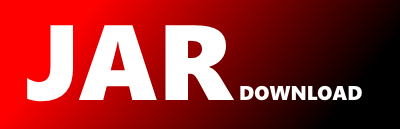
com.amazonaws.services.s3control.model.ObjectLambdaConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-s3control Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.s3control.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A configuration used when creating an Object Lambda Access Point.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ObjectLambdaConfiguration implements Serializable, Cloneable {
/**
*
* Standard access point associated with the Object Lambda Access Point.
*
*/
private String supportingAccessPoint;
/**
*
* A container for whether the CloudWatch metrics configuration is enabled.
*
*/
private Boolean cloudWatchMetricsEnabled;
/**
*
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
*
*/
private java.util.List allowedFeatures;
/**
*
* A container for transformation configurations for an Object Lambda Access Point.
*
*/
private java.util.List transformationConfigurations;
/**
*
* Standard access point associated with the Object Lambda Access Point.
*
*
* @param supportingAccessPoint
* Standard access point associated with the Object Lambda Access Point.
*/
public void setSupportingAccessPoint(String supportingAccessPoint) {
this.supportingAccessPoint = supportingAccessPoint;
}
/**
*
* Standard access point associated with the Object Lambda Access Point.
*
*
* @return Standard access point associated with the Object Lambda Access Point.
*/
public String getSupportingAccessPoint() {
return this.supportingAccessPoint;
}
/**
*
* Standard access point associated with the Object Lambda Access Point.
*
*
* @param supportingAccessPoint
* Standard access point associated with the Object Lambda Access Point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ObjectLambdaConfiguration withSupportingAccessPoint(String supportingAccessPoint) {
setSupportingAccessPoint(supportingAccessPoint);
return this;
}
/**
*
* A container for whether the CloudWatch metrics configuration is enabled.
*
*
* @param cloudWatchMetricsEnabled
* A container for whether the CloudWatch metrics configuration is enabled.
*/
public void setCloudWatchMetricsEnabled(Boolean cloudWatchMetricsEnabled) {
this.cloudWatchMetricsEnabled = cloudWatchMetricsEnabled;
}
/**
*
* A container for whether the CloudWatch metrics configuration is enabled.
*
*
* @return A container for whether the CloudWatch metrics configuration is enabled.
*/
public Boolean getCloudWatchMetricsEnabled() {
return this.cloudWatchMetricsEnabled;
}
/**
*
* A container for whether the CloudWatch metrics configuration is enabled.
*
*
* @param cloudWatchMetricsEnabled
* A container for whether the CloudWatch metrics configuration is enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ObjectLambdaConfiguration withCloudWatchMetricsEnabled(Boolean cloudWatchMetricsEnabled) {
setCloudWatchMetricsEnabled(cloudWatchMetricsEnabled);
return this;
}
/**
*
* A container for whether the CloudWatch metrics configuration is enabled.
*
*
* @return A container for whether the CloudWatch metrics configuration is enabled.
*/
public Boolean isCloudWatchMetricsEnabled() {
return this.cloudWatchMetricsEnabled;
}
/**
*
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
*
*
* @return A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
* @see ObjectLambdaAllowedFeature
*/
public java.util.List getAllowedFeatures() {
return allowedFeatures;
}
/**
*
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
*
*
* @param allowedFeatures
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
* @see ObjectLambdaAllowedFeature
*/
public void setAllowedFeatures(java.util.Collection allowedFeatures) {
if (allowedFeatures == null) {
this.allowedFeatures = null;
return;
}
this.allowedFeatures = new java.util.ArrayList(allowedFeatures);
}
/**
*
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAllowedFeatures(java.util.Collection)} or {@link #withAllowedFeatures(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param allowedFeatures
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ObjectLambdaAllowedFeature
*/
public ObjectLambdaConfiguration withAllowedFeatures(String... allowedFeatures) {
if (this.allowedFeatures == null) {
setAllowedFeatures(new java.util.ArrayList(allowedFeatures.length));
}
for (String ele : allowedFeatures) {
this.allowedFeatures.add(ele);
}
return this;
}
/**
*
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
*
*
* @param allowedFeatures
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ObjectLambdaAllowedFeature
*/
public ObjectLambdaConfiguration withAllowedFeatures(java.util.Collection allowedFeatures) {
setAllowedFeatures(allowedFeatures);
return this;
}
/**
*
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
*
*
* @param allowedFeatures
* A container for allowed features. Valid inputs are GetObject-Range
and
* GetObject-PartNumber
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ObjectLambdaAllowedFeature
*/
public ObjectLambdaConfiguration withAllowedFeatures(ObjectLambdaAllowedFeature... allowedFeatures) {
java.util.ArrayList allowedFeaturesCopy = new java.util.ArrayList(allowedFeatures.length);
for (ObjectLambdaAllowedFeature value : allowedFeatures) {
allowedFeaturesCopy.add(value.toString());
}
if (getAllowedFeatures() == null) {
setAllowedFeatures(allowedFeaturesCopy);
} else {
getAllowedFeatures().addAll(allowedFeaturesCopy);
}
return this;
}
/**
*
* A container for transformation configurations for an Object Lambda Access Point.
*
*
* @return A container for transformation configurations for an Object Lambda Access Point.
*/
public java.util.List getTransformationConfigurations() {
return transformationConfigurations;
}
/**
*
* A container for transformation configurations for an Object Lambda Access Point.
*
*
* @param transformationConfigurations
* A container for transformation configurations for an Object Lambda Access Point.
*/
public void setTransformationConfigurations(java.util.Collection transformationConfigurations) {
if (transformationConfigurations == null) {
this.transformationConfigurations = null;
return;
}
this.transformationConfigurations = new java.util.ArrayList(transformationConfigurations);
}
/**
*
* A container for transformation configurations for an Object Lambda Access Point.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTransformationConfigurations(java.util.Collection)} or
* {@link #withTransformationConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param transformationConfigurations
* A container for transformation configurations for an Object Lambda Access Point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ObjectLambdaConfiguration withTransformationConfigurations(ObjectLambdaTransformationConfiguration... transformationConfigurations) {
if (this.transformationConfigurations == null) {
setTransformationConfigurations(new java.util.ArrayList(transformationConfigurations.length));
}
for (ObjectLambdaTransformationConfiguration ele : transformationConfigurations) {
this.transformationConfigurations.add(ele);
}
return this;
}
/**
*
* A container for transformation configurations for an Object Lambda Access Point.
*
*
* @param transformationConfigurations
* A container for transformation configurations for an Object Lambda Access Point.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ObjectLambdaConfiguration withTransformationConfigurations(java.util.Collection transformationConfigurations) {
setTransformationConfigurations(transformationConfigurations);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSupportingAccessPoint() != null)
sb.append("SupportingAccessPoint: ").append(getSupportingAccessPoint()).append(",");
if (getCloudWatchMetricsEnabled() != null)
sb.append("CloudWatchMetricsEnabled: ").append(getCloudWatchMetricsEnabled()).append(",");
if (getAllowedFeatures() != null)
sb.append("AllowedFeatures: ").append(getAllowedFeatures()).append(",");
if (getTransformationConfigurations() != null)
sb.append("TransformationConfigurations: ").append(getTransformationConfigurations());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ObjectLambdaConfiguration == false)
return false;
ObjectLambdaConfiguration other = (ObjectLambdaConfiguration) obj;
if (other.getSupportingAccessPoint() == null ^ this.getSupportingAccessPoint() == null)
return false;
if (other.getSupportingAccessPoint() != null && other.getSupportingAccessPoint().equals(this.getSupportingAccessPoint()) == false)
return false;
if (other.getCloudWatchMetricsEnabled() == null ^ this.getCloudWatchMetricsEnabled() == null)
return false;
if (other.getCloudWatchMetricsEnabled() != null && other.getCloudWatchMetricsEnabled().equals(this.getCloudWatchMetricsEnabled()) == false)
return false;
if (other.getAllowedFeatures() == null ^ this.getAllowedFeatures() == null)
return false;
if (other.getAllowedFeatures() != null && other.getAllowedFeatures().equals(this.getAllowedFeatures()) == false)
return false;
if (other.getTransformationConfigurations() == null ^ this.getTransformationConfigurations() == null)
return false;
if (other.getTransformationConfigurations() != null && other.getTransformationConfigurations().equals(this.getTransformationConfigurations()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSupportingAccessPoint() == null) ? 0 : getSupportingAccessPoint().hashCode());
hashCode = prime * hashCode + ((getCloudWatchMetricsEnabled() == null) ? 0 : getCloudWatchMetricsEnabled().hashCode());
hashCode = prime * hashCode + ((getAllowedFeatures() == null) ? 0 : getAllowedFeatures().hashCode());
hashCode = prime * hashCode + ((getTransformationConfigurations() == null) ? 0 : getTransformationConfigurations().hashCode());
return hashCode;
}
@Override
public ObjectLambdaConfiguration clone() {
try {
return (ObjectLambdaConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}