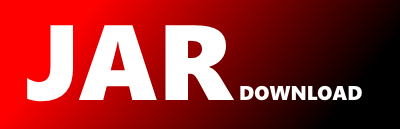
com.amazonaws.services.s3control.model.S3CopyObjectOperation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-s3control Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.s3control.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Contains the configuration parameters for a PUT Copy object operation. S3 Batch Operations passes every object to the
* underlying PUT Copy object API. For more information about the parameters for this operation, see PUT Object - Copy.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class S3CopyObjectOperation implements Serializable, Cloneable {
/**
*
* Specifies the destination bucket ARN for the batch copy operation. For example, to copy objects to a bucket named
* "destinationBucket", set the TargetResource to "arn:aws:s3:::destinationBucket".
*
*/
private String targetResource;
/** */
private String cannedAccessControlList;
/** */
private java.util.List accessControlGrants;
/** */
private String metadataDirective;
/** */
private java.util.Date modifiedSinceConstraint;
/**
*
* If you don't provide this parameter, Amazon S3 copies all the metadata from the original objects. If you specify
* an empty set, the new objects will have no tags. Otherwise, Amazon S3 assigns the supplied tags to the new
* objects.
*
*/
private S3ObjectMetadata newObjectMetadata;
/** */
private java.util.List newObjectTagging;
/**
*
* Specifies an optional metadata property for website redirects, x-amz-website-redirect-location
.
* Allows webpage redirects if the object is accessed through a website endpoint.
*
*/
private String redirectLocation;
/** */
private Boolean requesterPays;
/** */
private String storageClass;
/** */
private java.util.Date unModifiedSinceConstraint;
/** */
private String sSEAwsKmsKeyId;
/**
*
* Specifies the folder prefix into which you would like the objects to be copied. For example, to copy objects into
* a folder named Folder1
in the destination bucket, set the TargetKeyPrefix to Folder1
.
*
*/
private String targetKeyPrefix;
/**
*
* The legal hold status to be applied to all objects in the Batch Operations job.
*
*/
private String objectLockLegalHoldStatus;
/**
*
* The retention mode to be applied to all objects in the Batch Operations job.
*
*/
private String objectLockMode;
/**
*
* The date when the applied object retention configuration expires on all objects in the Batch Operations job.
*
*/
private java.util.Date objectLockRetainUntilDate;
/**
*
* Specifies whether Amazon S3 should use an S3 Bucket Key for object encryption with server-side encryption using
* Amazon Web Services KMS (SSE-KMS). Setting this header to true
causes Amazon S3 to use an S3 Bucket
* Key for object encryption with SSE-KMS.
*
*
* Specifying this header with an object action doesn’t affect bucket-level settings for S3 Bucket
* Key.
*
*/
private Boolean bucketKeyEnabled;
/**
*
* Indicates the algorithm you want Amazon S3 to use to create the checksum. For more information see Checking object
* integrity in the Amazon S3 User Guide.
*
*/
private String checksumAlgorithm;
/**
*
* Specifies the destination bucket ARN for the batch copy operation. For example, to copy objects to a bucket named
* "destinationBucket", set the TargetResource to "arn:aws:s3:::destinationBucket".
*
*
* @param targetResource
* Specifies the destination bucket ARN for the batch copy operation. For example, to copy objects to a
* bucket named "destinationBucket", set the TargetResource to "arn:aws:s3:::destinationBucket".
*/
public void setTargetResource(String targetResource) {
this.targetResource = targetResource;
}
/**
*
* Specifies the destination bucket ARN for the batch copy operation. For example, to copy objects to a bucket named
* "destinationBucket", set the TargetResource to "arn:aws:s3:::destinationBucket".
*
*
* @return Specifies the destination bucket ARN for the batch copy operation. For example, to copy objects to a
* bucket named "destinationBucket", set the TargetResource to "arn:aws:s3:::destinationBucket".
*/
public String getTargetResource() {
return this.targetResource;
}
/**
*
* Specifies the destination bucket ARN for the batch copy operation. For example, to copy objects to a bucket named
* "destinationBucket", set the TargetResource to "arn:aws:s3:::destinationBucket".
*
*
* @param targetResource
* Specifies the destination bucket ARN for the batch copy operation. For example, to copy objects to a
* bucket named "destinationBucket", set the TargetResource to "arn:aws:s3:::destinationBucket".
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withTargetResource(String targetResource) {
setTargetResource(targetResource);
return this;
}
/**
*
*
* @param cannedAccessControlList
* @see S3CannedAccessControlList
*/
public void setCannedAccessControlList(String cannedAccessControlList) {
this.cannedAccessControlList = cannedAccessControlList;
}
/**
*
*
* @return
* @see S3CannedAccessControlList
*/
public String getCannedAccessControlList() {
return this.cannedAccessControlList;
}
/**
*
*
* @param cannedAccessControlList
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3CannedAccessControlList
*/
public S3CopyObjectOperation withCannedAccessControlList(String cannedAccessControlList) {
setCannedAccessControlList(cannedAccessControlList);
return this;
}
/**
*
*
* @param cannedAccessControlList
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3CannedAccessControlList
*/
public S3CopyObjectOperation withCannedAccessControlList(S3CannedAccessControlList cannedAccessControlList) {
this.cannedAccessControlList = cannedAccessControlList.toString();
return this;
}
/**
*
*
* @return
*/
public java.util.List getAccessControlGrants() {
return accessControlGrants;
}
/**
*
*
* @param accessControlGrants
*/
public void setAccessControlGrants(java.util.Collection accessControlGrants) {
if (accessControlGrants == null) {
this.accessControlGrants = null;
return;
}
this.accessControlGrants = new java.util.ArrayList(accessControlGrants);
}
/**
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAccessControlGrants(java.util.Collection)} or {@link #withAccessControlGrants(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param accessControlGrants
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withAccessControlGrants(S3Grant... accessControlGrants) {
if (this.accessControlGrants == null) {
setAccessControlGrants(new java.util.ArrayList(accessControlGrants.length));
}
for (S3Grant ele : accessControlGrants) {
this.accessControlGrants.add(ele);
}
return this;
}
/**
*
*
* @param accessControlGrants
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withAccessControlGrants(java.util.Collection accessControlGrants) {
setAccessControlGrants(accessControlGrants);
return this;
}
/**
*
*
* @param metadataDirective
* @see S3MetadataDirective
*/
public void setMetadataDirective(String metadataDirective) {
this.metadataDirective = metadataDirective;
}
/**
*
*
* @return
* @see S3MetadataDirective
*/
public String getMetadataDirective() {
return this.metadataDirective;
}
/**
*
*
* @param metadataDirective
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3MetadataDirective
*/
public S3CopyObjectOperation withMetadataDirective(String metadataDirective) {
setMetadataDirective(metadataDirective);
return this;
}
/**
*
*
* @param metadataDirective
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3MetadataDirective
*/
public S3CopyObjectOperation withMetadataDirective(S3MetadataDirective metadataDirective) {
this.metadataDirective = metadataDirective.toString();
return this;
}
/**
*
*
* @param modifiedSinceConstraint
*/
public void setModifiedSinceConstraint(java.util.Date modifiedSinceConstraint) {
this.modifiedSinceConstraint = modifiedSinceConstraint;
}
/**
*
*
* @return
*/
public java.util.Date getModifiedSinceConstraint() {
return this.modifiedSinceConstraint;
}
/**
*
*
* @param modifiedSinceConstraint
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withModifiedSinceConstraint(java.util.Date modifiedSinceConstraint) {
setModifiedSinceConstraint(modifiedSinceConstraint);
return this;
}
/**
*
* If you don't provide this parameter, Amazon S3 copies all the metadata from the original objects. If you specify
* an empty set, the new objects will have no tags. Otherwise, Amazon S3 assigns the supplied tags to the new
* objects.
*
*
* @param newObjectMetadata
* If you don't provide this parameter, Amazon S3 copies all the metadata from the original objects. If you
* specify an empty set, the new objects will have no tags. Otherwise, Amazon S3 assigns the supplied tags to
* the new objects.
*/
public void setNewObjectMetadata(S3ObjectMetadata newObjectMetadata) {
this.newObjectMetadata = newObjectMetadata;
}
/**
*
* If you don't provide this parameter, Amazon S3 copies all the metadata from the original objects. If you specify
* an empty set, the new objects will have no tags. Otherwise, Amazon S3 assigns the supplied tags to the new
* objects.
*
*
* @return If you don't provide this parameter, Amazon S3 copies all the metadata from the original objects. If you
* specify an empty set, the new objects will have no tags. Otherwise, Amazon S3 assigns the supplied tags
* to the new objects.
*/
public S3ObjectMetadata getNewObjectMetadata() {
return this.newObjectMetadata;
}
/**
*
* If you don't provide this parameter, Amazon S3 copies all the metadata from the original objects. If you specify
* an empty set, the new objects will have no tags. Otherwise, Amazon S3 assigns the supplied tags to the new
* objects.
*
*
* @param newObjectMetadata
* If you don't provide this parameter, Amazon S3 copies all the metadata from the original objects. If you
* specify an empty set, the new objects will have no tags. Otherwise, Amazon S3 assigns the supplied tags to
* the new objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withNewObjectMetadata(S3ObjectMetadata newObjectMetadata) {
setNewObjectMetadata(newObjectMetadata);
return this;
}
/**
*
*
* @return
*/
public java.util.List getNewObjectTagging() {
return newObjectTagging;
}
/**
*
*
* @param newObjectTagging
*/
public void setNewObjectTagging(java.util.Collection newObjectTagging) {
if (newObjectTagging == null) {
this.newObjectTagging = null;
return;
}
this.newObjectTagging = new java.util.ArrayList(newObjectTagging);
}
/**
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNewObjectTagging(java.util.Collection)} or {@link #withNewObjectTagging(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param newObjectTagging
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withNewObjectTagging(S3Tag... newObjectTagging) {
if (this.newObjectTagging == null) {
setNewObjectTagging(new java.util.ArrayList(newObjectTagging.length));
}
for (S3Tag ele : newObjectTagging) {
this.newObjectTagging.add(ele);
}
return this;
}
/**
*
*
* @param newObjectTagging
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withNewObjectTagging(java.util.Collection newObjectTagging) {
setNewObjectTagging(newObjectTagging);
return this;
}
/**
*
* Specifies an optional metadata property for website redirects, x-amz-website-redirect-location
.
* Allows webpage redirects if the object is accessed through a website endpoint.
*
*
* @param redirectLocation
* Specifies an optional metadata property for website redirects,
* x-amz-website-redirect-location
. Allows webpage redirects if the object is accessed through a
* website endpoint.
*/
public void setRedirectLocation(String redirectLocation) {
this.redirectLocation = redirectLocation;
}
/**
*
* Specifies an optional metadata property for website redirects, x-amz-website-redirect-location
.
* Allows webpage redirects if the object is accessed through a website endpoint.
*
*
* @return Specifies an optional metadata property for website redirects,
* x-amz-website-redirect-location
. Allows webpage redirects if the object is accessed through
* a website endpoint.
*/
public String getRedirectLocation() {
return this.redirectLocation;
}
/**
*
* Specifies an optional metadata property for website redirects, x-amz-website-redirect-location
.
* Allows webpage redirects if the object is accessed through a website endpoint.
*
*
* @param redirectLocation
* Specifies an optional metadata property for website redirects,
* x-amz-website-redirect-location
. Allows webpage redirects if the object is accessed through a
* website endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withRedirectLocation(String redirectLocation) {
setRedirectLocation(redirectLocation);
return this;
}
/**
*
*
* @param requesterPays
*/
public void setRequesterPays(Boolean requesterPays) {
this.requesterPays = requesterPays;
}
/**
*
*
* @return
*/
public Boolean getRequesterPays() {
return this.requesterPays;
}
/**
*
*
* @param requesterPays
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withRequesterPays(Boolean requesterPays) {
setRequesterPays(requesterPays);
return this;
}
/**
*
*
* @return
*/
public Boolean isRequesterPays() {
return this.requesterPays;
}
/**
*
*
* @param storageClass
* @see S3StorageClass
*/
public void setStorageClass(String storageClass) {
this.storageClass = storageClass;
}
/**
*
*
* @return
* @see S3StorageClass
*/
public String getStorageClass() {
return this.storageClass;
}
/**
*
*
* @param storageClass
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3StorageClass
*/
public S3CopyObjectOperation withStorageClass(String storageClass) {
setStorageClass(storageClass);
return this;
}
/**
*
*
* @param storageClass
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3StorageClass
*/
public S3CopyObjectOperation withStorageClass(S3StorageClass storageClass) {
this.storageClass = storageClass.toString();
return this;
}
/**
*
*
* @param unModifiedSinceConstraint
*/
public void setUnModifiedSinceConstraint(java.util.Date unModifiedSinceConstraint) {
this.unModifiedSinceConstraint = unModifiedSinceConstraint;
}
/**
*
*
* @return
*/
public java.util.Date getUnModifiedSinceConstraint() {
return this.unModifiedSinceConstraint;
}
/**
*
*
* @param unModifiedSinceConstraint
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withUnModifiedSinceConstraint(java.util.Date unModifiedSinceConstraint) {
setUnModifiedSinceConstraint(unModifiedSinceConstraint);
return this;
}
/**
*
*
* @param sSEAwsKmsKeyId
*/
public void setSSEAwsKmsKeyId(String sSEAwsKmsKeyId) {
this.sSEAwsKmsKeyId = sSEAwsKmsKeyId;
}
/**
*
*
* @return
*/
public String getSSEAwsKmsKeyId() {
return this.sSEAwsKmsKeyId;
}
/**
*
*
* @param sSEAwsKmsKeyId
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withSSEAwsKmsKeyId(String sSEAwsKmsKeyId) {
setSSEAwsKmsKeyId(sSEAwsKmsKeyId);
return this;
}
/**
*
* Specifies the folder prefix into which you would like the objects to be copied. For example, to copy objects into
* a folder named Folder1
in the destination bucket, set the TargetKeyPrefix to Folder1
.
*
*
* @param targetKeyPrefix
* Specifies the folder prefix into which you would like the objects to be copied. For example, to copy
* objects into a folder named Folder1
in the destination bucket, set the TargetKeyPrefix to
* Folder1
.
*/
public void setTargetKeyPrefix(String targetKeyPrefix) {
this.targetKeyPrefix = targetKeyPrefix;
}
/**
*
* Specifies the folder prefix into which you would like the objects to be copied. For example, to copy objects into
* a folder named Folder1
in the destination bucket, set the TargetKeyPrefix to Folder1
.
*
*
* @return Specifies the folder prefix into which you would like the objects to be copied. For example, to copy
* objects into a folder named Folder1
in the destination bucket, set the TargetKeyPrefix to
* Folder1
.
*/
public String getTargetKeyPrefix() {
return this.targetKeyPrefix;
}
/**
*
* Specifies the folder prefix into which you would like the objects to be copied. For example, to copy objects into
* a folder named Folder1
in the destination bucket, set the TargetKeyPrefix to Folder1
.
*
*
* @param targetKeyPrefix
* Specifies the folder prefix into which you would like the objects to be copied. For example, to copy
* objects into a folder named Folder1
in the destination bucket, set the TargetKeyPrefix to
* Folder1
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withTargetKeyPrefix(String targetKeyPrefix) {
setTargetKeyPrefix(targetKeyPrefix);
return this;
}
/**
*
* The legal hold status to be applied to all objects in the Batch Operations job.
*
*
* @param objectLockLegalHoldStatus
* The legal hold status to be applied to all objects in the Batch Operations job.
* @see S3ObjectLockLegalHoldStatus
*/
public void setObjectLockLegalHoldStatus(String objectLockLegalHoldStatus) {
this.objectLockLegalHoldStatus = objectLockLegalHoldStatus;
}
/**
*
* The legal hold status to be applied to all objects in the Batch Operations job.
*
*
* @return The legal hold status to be applied to all objects in the Batch Operations job.
* @see S3ObjectLockLegalHoldStatus
*/
public String getObjectLockLegalHoldStatus() {
return this.objectLockLegalHoldStatus;
}
/**
*
* The legal hold status to be applied to all objects in the Batch Operations job.
*
*
* @param objectLockLegalHoldStatus
* The legal hold status to be applied to all objects in the Batch Operations job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ObjectLockLegalHoldStatus
*/
public S3CopyObjectOperation withObjectLockLegalHoldStatus(String objectLockLegalHoldStatus) {
setObjectLockLegalHoldStatus(objectLockLegalHoldStatus);
return this;
}
/**
*
* The legal hold status to be applied to all objects in the Batch Operations job.
*
*
* @param objectLockLegalHoldStatus
* The legal hold status to be applied to all objects in the Batch Operations job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ObjectLockLegalHoldStatus
*/
public S3CopyObjectOperation withObjectLockLegalHoldStatus(S3ObjectLockLegalHoldStatus objectLockLegalHoldStatus) {
this.objectLockLegalHoldStatus = objectLockLegalHoldStatus.toString();
return this;
}
/**
*
* The retention mode to be applied to all objects in the Batch Operations job.
*
*
* @param objectLockMode
* The retention mode to be applied to all objects in the Batch Operations job.
* @see S3ObjectLockMode
*/
public void setObjectLockMode(String objectLockMode) {
this.objectLockMode = objectLockMode;
}
/**
*
* The retention mode to be applied to all objects in the Batch Operations job.
*
*
* @return The retention mode to be applied to all objects in the Batch Operations job.
* @see S3ObjectLockMode
*/
public String getObjectLockMode() {
return this.objectLockMode;
}
/**
*
* The retention mode to be applied to all objects in the Batch Operations job.
*
*
* @param objectLockMode
* The retention mode to be applied to all objects in the Batch Operations job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ObjectLockMode
*/
public S3CopyObjectOperation withObjectLockMode(String objectLockMode) {
setObjectLockMode(objectLockMode);
return this;
}
/**
*
* The retention mode to be applied to all objects in the Batch Operations job.
*
*
* @param objectLockMode
* The retention mode to be applied to all objects in the Batch Operations job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ObjectLockMode
*/
public S3CopyObjectOperation withObjectLockMode(S3ObjectLockMode objectLockMode) {
this.objectLockMode = objectLockMode.toString();
return this;
}
/**
*
* The date when the applied object retention configuration expires on all objects in the Batch Operations job.
*
*
* @param objectLockRetainUntilDate
* The date when the applied object retention configuration expires on all objects in the Batch Operations
* job.
*/
public void setObjectLockRetainUntilDate(java.util.Date objectLockRetainUntilDate) {
this.objectLockRetainUntilDate = objectLockRetainUntilDate;
}
/**
*
* The date when the applied object retention configuration expires on all objects in the Batch Operations job.
*
*
* @return The date when the applied object retention configuration expires on all objects in the Batch Operations
* job.
*/
public java.util.Date getObjectLockRetainUntilDate() {
return this.objectLockRetainUntilDate;
}
/**
*
* The date when the applied object retention configuration expires on all objects in the Batch Operations job.
*
*
* @param objectLockRetainUntilDate
* The date when the applied object retention configuration expires on all objects in the Batch Operations
* job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withObjectLockRetainUntilDate(java.util.Date objectLockRetainUntilDate) {
setObjectLockRetainUntilDate(objectLockRetainUntilDate);
return this;
}
/**
*
* Specifies whether Amazon S3 should use an S3 Bucket Key for object encryption with server-side encryption using
* Amazon Web Services KMS (SSE-KMS). Setting this header to true
causes Amazon S3 to use an S3 Bucket
* Key for object encryption with SSE-KMS.
*
*
* Specifying this header with an object action doesn’t affect bucket-level settings for S3 Bucket
* Key.
*
*
* @param bucketKeyEnabled
* Specifies whether Amazon S3 should use an S3 Bucket Key for object encryption with server-side encryption
* using Amazon Web Services KMS (SSE-KMS). Setting this header to true
causes Amazon S3 to use
* an S3 Bucket Key for object encryption with SSE-KMS.
*
* Specifying this header with an object action doesn’t affect bucket-level settings for S3
* Bucket Key.
*/
public void setBucketKeyEnabled(Boolean bucketKeyEnabled) {
this.bucketKeyEnabled = bucketKeyEnabled;
}
/**
*
* Specifies whether Amazon S3 should use an S3 Bucket Key for object encryption with server-side encryption using
* Amazon Web Services KMS (SSE-KMS). Setting this header to true
causes Amazon S3 to use an S3 Bucket
* Key for object encryption with SSE-KMS.
*
*
* Specifying this header with an object action doesn’t affect bucket-level settings for S3 Bucket
* Key.
*
*
* @return Specifies whether Amazon S3 should use an S3 Bucket Key for object encryption with server-side encryption
* using Amazon Web Services KMS (SSE-KMS). Setting this header to true
causes Amazon S3 to use
* an S3 Bucket Key for object encryption with SSE-KMS.
*
* Specifying this header with an object action doesn’t affect bucket-level settings for S3
* Bucket Key.
*/
public Boolean getBucketKeyEnabled() {
return this.bucketKeyEnabled;
}
/**
*
* Specifies whether Amazon S3 should use an S3 Bucket Key for object encryption with server-side encryption using
* Amazon Web Services KMS (SSE-KMS). Setting this header to true
causes Amazon S3 to use an S3 Bucket
* Key for object encryption with SSE-KMS.
*
*
* Specifying this header with an object action doesn’t affect bucket-level settings for S3 Bucket
* Key.
*
*
* @param bucketKeyEnabled
* Specifies whether Amazon S3 should use an S3 Bucket Key for object encryption with server-side encryption
* using Amazon Web Services KMS (SSE-KMS). Setting this header to true
causes Amazon S3 to use
* an S3 Bucket Key for object encryption with SSE-KMS.
*
* Specifying this header with an object action doesn’t affect bucket-level settings for S3
* Bucket Key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3CopyObjectOperation withBucketKeyEnabled(Boolean bucketKeyEnabled) {
setBucketKeyEnabled(bucketKeyEnabled);
return this;
}
/**
*
* Specifies whether Amazon S3 should use an S3 Bucket Key for object encryption with server-side encryption using
* Amazon Web Services KMS (SSE-KMS). Setting this header to true
causes Amazon S3 to use an S3 Bucket
* Key for object encryption with SSE-KMS.
*
*
* Specifying this header with an object action doesn’t affect bucket-level settings for S3 Bucket
* Key.
*
*
* @return Specifies whether Amazon S3 should use an S3 Bucket Key for object encryption with server-side encryption
* using Amazon Web Services KMS (SSE-KMS). Setting this header to true
causes Amazon S3 to use
* an S3 Bucket Key for object encryption with SSE-KMS.
*
* Specifying this header with an object action doesn’t affect bucket-level settings for S3
* Bucket Key.
*/
public Boolean isBucketKeyEnabled() {
return this.bucketKeyEnabled;
}
/**
*
* Indicates the algorithm you want Amazon S3 to use to create the checksum. For more information see Checking object
* integrity in the Amazon S3 User Guide.
*
*
* @param checksumAlgorithm
* Indicates the algorithm you want Amazon S3 to use to create the checksum. For more information see Checking object
* integrity in the Amazon S3 User Guide.
* @see S3ChecksumAlgorithm
*/
public void setChecksumAlgorithm(String checksumAlgorithm) {
this.checksumAlgorithm = checksumAlgorithm;
}
/**
*
* Indicates the algorithm you want Amazon S3 to use to create the checksum. For more information see Checking object
* integrity in the Amazon S3 User Guide.
*
*
* @return Indicates the algorithm you want Amazon S3 to use to create the checksum. For more information see Checking object
* integrity in the Amazon S3 User Guide.
* @see S3ChecksumAlgorithm
*/
public String getChecksumAlgorithm() {
return this.checksumAlgorithm;
}
/**
*
* Indicates the algorithm you want Amazon S3 to use to create the checksum. For more information see Checking object
* integrity in the Amazon S3 User Guide.
*
*
* @param checksumAlgorithm
* Indicates the algorithm you want Amazon S3 to use to create the checksum. For more information see Checking object
* integrity in the Amazon S3 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ChecksumAlgorithm
*/
public S3CopyObjectOperation withChecksumAlgorithm(String checksumAlgorithm) {
setChecksumAlgorithm(checksumAlgorithm);
return this;
}
/**
*
* Indicates the algorithm you want Amazon S3 to use to create the checksum. For more information see Checking object
* integrity in the Amazon S3 User Guide.
*
*
* @param checksumAlgorithm
* Indicates the algorithm you want Amazon S3 to use to create the checksum. For more information see Checking object
* integrity in the Amazon S3 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ChecksumAlgorithm
*/
public S3CopyObjectOperation withChecksumAlgorithm(S3ChecksumAlgorithm checksumAlgorithm) {
this.checksumAlgorithm = checksumAlgorithm.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTargetResource() != null)
sb.append("TargetResource: ").append(getTargetResource()).append(",");
if (getCannedAccessControlList() != null)
sb.append("CannedAccessControlList: ").append(getCannedAccessControlList()).append(",");
if (getAccessControlGrants() != null)
sb.append("AccessControlGrants: ").append(getAccessControlGrants()).append(",");
if (getMetadataDirective() != null)
sb.append("MetadataDirective: ").append(getMetadataDirective()).append(",");
if (getModifiedSinceConstraint() != null)
sb.append("ModifiedSinceConstraint: ").append(getModifiedSinceConstraint()).append(",");
if (getNewObjectMetadata() != null)
sb.append("NewObjectMetadata: ").append(getNewObjectMetadata()).append(",");
if (getNewObjectTagging() != null)
sb.append("NewObjectTagging: ").append(getNewObjectTagging()).append(",");
if (getRedirectLocation() != null)
sb.append("RedirectLocation: ").append(getRedirectLocation()).append(",");
if (getRequesterPays() != null)
sb.append("RequesterPays: ").append(getRequesterPays()).append(",");
if (getStorageClass() != null)
sb.append("StorageClass: ").append(getStorageClass()).append(",");
if (getUnModifiedSinceConstraint() != null)
sb.append("UnModifiedSinceConstraint: ").append(getUnModifiedSinceConstraint()).append(",");
if (getSSEAwsKmsKeyId() != null)
sb.append("SSEAwsKmsKeyId: ").append(getSSEAwsKmsKeyId()).append(",");
if (getTargetKeyPrefix() != null)
sb.append("TargetKeyPrefix: ").append(getTargetKeyPrefix()).append(",");
if (getObjectLockLegalHoldStatus() != null)
sb.append("ObjectLockLegalHoldStatus: ").append(getObjectLockLegalHoldStatus()).append(",");
if (getObjectLockMode() != null)
sb.append("ObjectLockMode: ").append(getObjectLockMode()).append(",");
if (getObjectLockRetainUntilDate() != null)
sb.append("ObjectLockRetainUntilDate: ").append(getObjectLockRetainUntilDate()).append(",");
if (getBucketKeyEnabled() != null)
sb.append("BucketKeyEnabled: ").append(getBucketKeyEnabled()).append(",");
if (getChecksumAlgorithm() != null)
sb.append("ChecksumAlgorithm: ").append(getChecksumAlgorithm());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof S3CopyObjectOperation == false)
return false;
S3CopyObjectOperation other = (S3CopyObjectOperation) obj;
if (other.getTargetResource() == null ^ this.getTargetResource() == null)
return false;
if (other.getTargetResource() != null && other.getTargetResource().equals(this.getTargetResource()) == false)
return false;
if (other.getCannedAccessControlList() == null ^ this.getCannedAccessControlList() == null)
return false;
if (other.getCannedAccessControlList() != null && other.getCannedAccessControlList().equals(this.getCannedAccessControlList()) == false)
return false;
if (other.getAccessControlGrants() == null ^ this.getAccessControlGrants() == null)
return false;
if (other.getAccessControlGrants() != null && other.getAccessControlGrants().equals(this.getAccessControlGrants()) == false)
return false;
if (other.getMetadataDirective() == null ^ this.getMetadataDirective() == null)
return false;
if (other.getMetadataDirective() != null && other.getMetadataDirective().equals(this.getMetadataDirective()) == false)
return false;
if (other.getModifiedSinceConstraint() == null ^ this.getModifiedSinceConstraint() == null)
return false;
if (other.getModifiedSinceConstraint() != null && other.getModifiedSinceConstraint().equals(this.getModifiedSinceConstraint()) == false)
return false;
if (other.getNewObjectMetadata() == null ^ this.getNewObjectMetadata() == null)
return false;
if (other.getNewObjectMetadata() != null && other.getNewObjectMetadata().equals(this.getNewObjectMetadata()) == false)
return false;
if (other.getNewObjectTagging() == null ^ this.getNewObjectTagging() == null)
return false;
if (other.getNewObjectTagging() != null && other.getNewObjectTagging().equals(this.getNewObjectTagging()) == false)
return false;
if (other.getRedirectLocation() == null ^ this.getRedirectLocation() == null)
return false;
if (other.getRedirectLocation() != null && other.getRedirectLocation().equals(this.getRedirectLocation()) == false)
return false;
if (other.getRequesterPays() == null ^ this.getRequesterPays() == null)
return false;
if (other.getRequesterPays() != null && other.getRequesterPays().equals(this.getRequesterPays()) == false)
return false;
if (other.getStorageClass() == null ^ this.getStorageClass() == null)
return false;
if (other.getStorageClass() != null && other.getStorageClass().equals(this.getStorageClass()) == false)
return false;
if (other.getUnModifiedSinceConstraint() == null ^ this.getUnModifiedSinceConstraint() == null)
return false;
if (other.getUnModifiedSinceConstraint() != null && other.getUnModifiedSinceConstraint().equals(this.getUnModifiedSinceConstraint()) == false)
return false;
if (other.getSSEAwsKmsKeyId() == null ^ this.getSSEAwsKmsKeyId() == null)
return false;
if (other.getSSEAwsKmsKeyId() != null && other.getSSEAwsKmsKeyId().equals(this.getSSEAwsKmsKeyId()) == false)
return false;
if (other.getTargetKeyPrefix() == null ^ this.getTargetKeyPrefix() == null)
return false;
if (other.getTargetKeyPrefix() != null && other.getTargetKeyPrefix().equals(this.getTargetKeyPrefix()) == false)
return false;
if (other.getObjectLockLegalHoldStatus() == null ^ this.getObjectLockLegalHoldStatus() == null)
return false;
if (other.getObjectLockLegalHoldStatus() != null && other.getObjectLockLegalHoldStatus().equals(this.getObjectLockLegalHoldStatus()) == false)
return false;
if (other.getObjectLockMode() == null ^ this.getObjectLockMode() == null)
return false;
if (other.getObjectLockMode() != null && other.getObjectLockMode().equals(this.getObjectLockMode()) == false)
return false;
if (other.getObjectLockRetainUntilDate() == null ^ this.getObjectLockRetainUntilDate() == null)
return false;
if (other.getObjectLockRetainUntilDate() != null && other.getObjectLockRetainUntilDate().equals(this.getObjectLockRetainUntilDate()) == false)
return false;
if (other.getBucketKeyEnabled() == null ^ this.getBucketKeyEnabled() == null)
return false;
if (other.getBucketKeyEnabled() != null && other.getBucketKeyEnabled().equals(this.getBucketKeyEnabled()) == false)
return false;
if (other.getChecksumAlgorithm() == null ^ this.getChecksumAlgorithm() == null)
return false;
if (other.getChecksumAlgorithm() != null && other.getChecksumAlgorithm().equals(this.getChecksumAlgorithm()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTargetResource() == null) ? 0 : getTargetResource().hashCode());
hashCode = prime * hashCode + ((getCannedAccessControlList() == null) ? 0 : getCannedAccessControlList().hashCode());
hashCode = prime * hashCode + ((getAccessControlGrants() == null) ? 0 : getAccessControlGrants().hashCode());
hashCode = prime * hashCode + ((getMetadataDirective() == null) ? 0 : getMetadataDirective().hashCode());
hashCode = prime * hashCode + ((getModifiedSinceConstraint() == null) ? 0 : getModifiedSinceConstraint().hashCode());
hashCode = prime * hashCode + ((getNewObjectMetadata() == null) ? 0 : getNewObjectMetadata().hashCode());
hashCode = prime * hashCode + ((getNewObjectTagging() == null) ? 0 : getNewObjectTagging().hashCode());
hashCode = prime * hashCode + ((getRedirectLocation() == null) ? 0 : getRedirectLocation().hashCode());
hashCode = prime * hashCode + ((getRequesterPays() == null) ? 0 : getRequesterPays().hashCode());
hashCode = prime * hashCode + ((getStorageClass() == null) ? 0 : getStorageClass().hashCode());
hashCode = prime * hashCode + ((getUnModifiedSinceConstraint() == null) ? 0 : getUnModifiedSinceConstraint().hashCode());
hashCode = prime * hashCode + ((getSSEAwsKmsKeyId() == null) ? 0 : getSSEAwsKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getTargetKeyPrefix() == null) ? 0 : getTargetKeyPrefix().hashCode());
hashCode = prime * hashCode + ((getObjectLockLegalHoldStatus() == null) ? 0 : getObjectLockLegalHoldStatus().hashCode());
hashCode = prime * hashCode + ((getObjectLockMode() == null) ? 0 : getObjectLockMode().hashCode());
hashCode = prime * hashCode + ((getObjectLockRetainUntilDate() == null) ? 0 : getObjectLockRetainUntilDate().hashCode());
hashCode = prime * hashCode + ((getBucketKeyEnabled() == null) ? 0 : getBucketKeyEnabled().hashCode());
hashCode = prime * hashCode + ((getChecksumAlgorithm() == null) ? 0 : getChecksumAlgorithm().hashCode());
return hashCode;
}
@Override
public S3CopyObjectOperation clone() {
try {
return (S3CopyObjectOperation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}