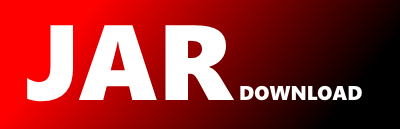
com.amazonaws.services.s3control.model.CreateAccessGrantsInstanceResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-s3control Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.s3control.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAccessGrantsInstanceResult extends com.amazonaws.AmazonWebServiceResult
implements Serializable, Cloneable {
/**
*
* The date and time when you created the S3 Access Grants instance.
*
*/
private java.util.Date createdAt;
/**
*
* The ID of the S3 Access Grants instance. The ID is default
. You can have one S3 Access Grants
* instance per Region per account.
*
*/
private String accessGrantsInstanceId;
/**
*
* The Amazon Resource Name (ARN) of the S3 Access Grants instance.
*
*/
private String accessGrantsInstanceArn;
/**
*
* If you associated your S3 Access Grants instance with an Amazon Web Services IAM Identity Center instance, this
* field returns the Amazon Resource Name (ARN) of the IAM Identity Center instance application; a subresource of
* the original Identity Center instance passed in the request. S3 Access Grants creates this Identity Center
* application for this specific S3 Access Grants instance.
*
*/
private String identityCenterArn;
/**
*
* The date and time when you created the S3 Access Grants instance.
*
*
* @param createdAt
* The date and time when you created the S3 Access Grants instance.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time when you created the S3 Access Grants instance.
*
*
* @return The date and time when you created the S3 Access Grants instance.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time when you created the S3 Access Grants instance.
*
*
* @param createdAt
* The date and time when you created the S3 Access Grants instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessGrantsInstanceResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The ID of the S3 Access Grants instance. The ID is default
. You can have one S3 Access Grants
* instance per Region per account.
*
*
* @param accessGrantsInstanceId
* The ID of the S3 Access Grants instance. The ID is default
. You can have one S3 Access Grants
* instance per Region per account.
*/
public void setAccessGrantsInstanceId(String accessGrantsInstanceId) {
this.accessGrantsInstanceId = accessGrantsInstanceId;
}
/**
*
* The ID of the S3 Access Grants instance. The ID is default
. You can have one S3 Access Grants
* instance per Region per account.
*
*
* @return The ID of the S3 Access Grants instance. The ID is default
. You can have one S3 Access
* Grants instance per Region per account.
*/
public String getAccessGrantsInstanceId() {
return this.accessGrantsInstanceId;
}
/**
*
* The ID of the S3 Access Grants instance. The ID is default
. You can have one S3 Access Grants
* instance per Region per account.
*
*
* @param accessGrantsInstanceId
* The ID of the S3 Access Grants instance. The ID is default
. You can have one S3 Access Grants
* instance per Region per account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessGrantsInstanceResult withAccessGrantsInstanceId(String accessGrantsInstanceId) {
setAccessGrantsInstanceId(accessGrantsInstanceId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the S3 Access Grants instance.
*
*
* @param accessGrantsInstanceArn
* The Amazon Resource Name (ARN) of the S3 Access Grants instance.
*/
public void setAccessGrantsInstanceArn(String accessGrantsInstanceArn) {
this.accessGrantsInstanceArn = accessGrantsInstanceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the S3 Access Grants instance.
*
*
* @return The Amazon Resource Name (ARN) of the S3 Access Grants instance.
*/
public String getAccessGrantsInstanceArn() {
return this.accessGrantsInstanceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the S3 Access Grants instance.
*
*
* @param accessGrantsInstanceArn
* The Amazon Resource Name (ARN) of the S3 Access Grants instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessGrantsInstanceResult withAccessGrantsInstanceArn(String accessGrantsInstanceArn) {
setAccessGrantsInstanceArn(accessGrantsInstanceArn);
return this;
}
/**
*
* If you associated your S3 Access Grants instance with an Amazon Web Services IAM Identity Center instance, this
* field returns the Amazon Resource Name (ARN) of the IAM Identity Center instance application; a subresource of
* the original Identity Center instance passed in the request. S3 Access Grants creates this Identity Center
* application for this specific S3 Access Grants instance.
*
*
* @param identityCenterArn
* If you associated your S3 Access Grants instance with an Amazon Web Services IAM Identity Center instance,
* this field returns the Amazon Resource Name (ARN) of the IAM Identity Center instance application; a
* subresource of the original Identity Center instance passed in the request. S3 Access Grants creates this
* Identity Center application for this specific S3 Access Grants instance.
*/
public void setIdentityCenterArn(String identityCenterArn) {
this.identityCenterArn = identityCenterArn;
}
/**
*
* If you associated your S3 Access Grants instance with an Amazon Web Services IAM Identity Center instance, this
* field returns the Amazon Resource Name (ARN) of the IAM Identity Center instance application; a subresource of
* the original Identity Center instance passed in the request. S3 Access Grants creates this Identity Center
* application for this specific S3 Access Grants instance.
*
*
* @return If you associated your S3 Access Grants instance with an Amazon Web Services IAM Identity Center
* instance, this field returns the Amazon Resource Name (ARN) of the IAM Identity Center instance
* application; a subresource of the original Identity Center instance passed in the request. S3 Access
* Grants creates this Identity Center application for this specific S3 Access Grants instance.
*/
public String getIdentityCenterArn() {
return this.identityCenterArn;
}
/**
*
* If you associated your S3 Access Grants instance with an Amazon Web Services IAM Identity Center instance, this
* field returns the Amazon Resource Name (ARN) of the IAM Identity Center instance application; a subresource of
* the original Identity Center instance passed in the request. S3 Access Grants creates this Identity Center
* application for this specific S3 Access Grants instance.
*
*
* @param identityCenterArn
* If you associated your S3 Access Grants instance with an Amazon Web Services IAM Identity Center instance,
* this field returns the Amazon Resource Name (ARN) of the IAM Identity Center instance application; a
* subresource of the original Identity Center instance passed in the request. S3 Access Grants creates this
* Identity Center application for this specific S3 Access Grants instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAccessGrantsInstanceResult withIdentityCenterArn(String identityCenterArn) {
setIdentityCenterArn(identityCenterArn);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getAccessGrantsInstanceId() != null)
sb.append("AccessGrantsInstanceId: ").append(getAccessGrantsInstanceId()).append(",");
if (getAccessGrantsInstanceArn() != null)
sb.append("AccessGrantsInstanceArn: ").append(getAccessGrantsInstanceArn()).append(",");
if (getIdentityCenterArn() != null)
sb.append("IdentityCenterArn: ").append(getIdentityCenterArn());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAccessGrantsInstanceResult == false)
return false;
CreateAccessGrantsInstanceResult other = (CreateAccessGrantsInstanceResult) obj;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getAccessGrantsInstanceId() == null ^ this.getAccessGrantsInstanceId() == null)
return false;
if (other.getAccessGrantsInstanceId() != null && other.getAccessGrantsInstanceId().equals(this.getAccessGrantsInstanceId()) == false)
return false;
if (other.getAccessGrantsInstanceArn() == null ^ this.getAccessGrantsInstanceArn() == null)
return false;
if (other.getAccessGrantsInstanceArn() != null && other.getAccessGrantsInstanceArn().equals(this.getAccessGrantsInstanceArn()) == false)
return false;
if (other.getIdentityCenterArn() == null ^ this.getIdentityCenterArn() == null)
return false;
if (other.getIdentityCenterArn() != null && other.getIdentityCenterArn().equals(this.getIdentityCenterArn()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getAccessGrantsInstanceId() == null) ? 0 : getAccessGrantsInstanceId().hashCode());
hashCode = prime * hashCode + ((getAccessGrantsInstanceArn() == null) ? 0 : getAccessGrantsInstanceArn().hashCode());
hashCode = prime * hashCode + ((getIdentityCenterArn() == null) ? 0 : getIdentityCenterArn().hashCode());
return hashCode;
}
@Override
public CreateAccessGrantsInstanceResult clone() {
try {
return (CreateAccessGrantsInstanceResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}