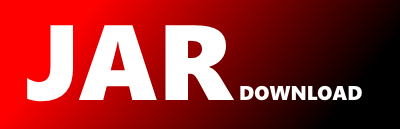
com.amazonaws.services.s3control.model.JobDescriptor Maven / Gradle / Ivy
Show all versions of aws-java-sdk-s3control Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.s3control.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A container element for the job configuration and status information returned by a Describe Job
request.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class JobDescriptor implements Serializable, Cloneable {
/**
*
* The ID for the specified job.
*
*/
private String jobId;
/**
*
* Indicates whether confirmation is required before Amazon S3 begins running the specified job. Confirmation is
* required only for jobs created through the Amazon S3 console.
*
*/
private Boolean confirmationRequired;
/**
*
* The description for this job, if one was provided in this job's Create Job
request.
*
*/
private String description;
/**
*
* The Amazon Resource Name (ARN) for this job.
*
*/
private String jobArn;
/**
*
* The current status of the specified job.
*
*/
private String status;
/**
*
* The configuration information for the specified job's manifest object.
*
*/
private JobManifest manifest;
/**
*
* The operation that the specified job is configured to run on the objects listed in the manifest.
*
*/
private JobOperation operation;
/**
*
* The priority of the specified job.
*
*/
private Integer priority;
/**
*
* Describes the total number of tasks that the specified job has run, the number of tasks that succeeded, and the
* number of tasks that failed.
*
*/
private JobProgressSummary progressSummary;
/**
*
* The reason for updating the job.
*
*/
private String statusUpdateReason;
/**
*
* If the specified job failed, this field contains information describing the failure.
*
*/
private java.util.List failureReasons;
/**
*
* Contains the configuration information for the job-completion report if you requested one in the
* Create Job
request.
*
*/
private JobReport report;
/**
*
* A timestamp indicating when this job was created.
*
*/
private java.util.Date creationTime;
/**
*
* A timestamp indicating when this job terminated. A job's termination date is the date and time when it succeeded,
* failed, or was canceled.
*
*/
private java.util.Date terminationDate;
/**
*
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role assigned to run the tasks for
* this job.
*
*/
private String roleArn;
/**
*
* The timestamp when this job was suspended, if it has been suspended.
*
*/
private java.util.Date suspendedDate;
/**
*
* The reason why the specified job was suspended. A job is only suspended if you create it through the Amazon S3
* console. When you create the job, it enters the Suspended
state to await confirmation before
* running. After you confirm the job, it automatically exits the Suspended
state.
*
*/
private String suspendedCause;
/**
*
* The manifest generator that was used to generate a job manifest for this job.
*
*/
private JobManifestGenerator manifestGenerator;
/**
*
* The attribute of the JobDescriptor containing details about the job's generated manifest.
*
*/
private S3GeneratedManifestDescriptor generatedManifestDescriptor;
/**
*
* The ID for the specified job.
*
*
* @param jobId
* The ID for the specified job.
*/
public void setJobId(String jobId) {
this.jobId = jobId;
}
/**
*
* The ID for the specified job.
*
*
* @return The ID for the specified job.
*/
public String getJobId() {
return this.jobId;
}
/**
*
* The ID for the specified job.
*
*
* @param jobId
* The ID for the specified job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withJobId(String jobId) {
setJobId(jobId);
return this;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 begins running the specified job. Confirmation is
* required only for jobs created through the Amazon S3 console.
*
*
* @param confirmationRequired
* Indicates whether confirmation is required before Amazon S3 begins running the specified job. Confirmation
* is required only for jobs created through the Amazon S3 console.
*/
public void setConfirmationRequired(Boolean confirmationRequired) {
this.confirmationRequired = confirmationRequired;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 begins running the specified job. Confirmation is
* required only for jobs created through the Amazon S3 console.
*
*
* @return Indicates whether confirmation is required before Amazon S3 begins running the specified job.
* Confirmation is required only for jobs created through the Amazon S3 console.
*/
public Boolean getConfirmationRequired() {
return this.confirmationRequired;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 begins running the specified job. Confirmation is
* required only for jobs created through the Amazon S3 console.
*
*
* @param confirmationRequired
* Indicates whether confirmation is required before Amazon S3 begins running the specified job. Confirmation
* is required only for jobs created through the Amazon S3 console.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withConfirmationRequired(Boolean confirmationRequired) {
setConfirmationRequired(confirmationRequired);
return this;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 begins running the specified job. Confirmation is
* required only for jobs created through the Amazon S3 console.
*
*
* @return Indicates whether confirmation is required before Amazon S3 begins running the specified job.
* Confirmation is required only for jobs created through the Amazon S3 console.
*/
public Boolean isConfirmationRequired() {
return this.confirmationRequired;
}
/**
*
* The description for this job, if one was provided in this job's Create Job
request.
*
*
* @param description
* The description for this job, if one was provided in this job's Create Job
request.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description for this job, if one was provided in this job's Create Job
request.
*
*
* @return The description for this job, if one was provided in this job's Create Job
request.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description for this job, if one was provided in this job's Create Job
request.
*
*
* @param description
* The description for this job, if one was provided in this job's Create Job
request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for this job.
*
*
* @param jobArn
* The Amazon Resource Name (ARN) for this job.
*/
public void setJobArn(String jobArn) {
this.jobArn = jobArn;
}
/**
*
* The Amazon Resource Name (ARN) for this job.
*
*
* @return The Amazon Resource Name (ARN) for this job.
*/
public String getJobArn() {
return this.jobArn;
}
/**
*
* The Amazon Resource Name (ARN) for this job.
*
*
* @param jobArn
* The Amazon Resource Name (ARN) for this job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withJobArn(String jobArn) {
setJobArn(jobArn);
return this;
}
/**
*
* The current status of the specified job.
*
*
* @param status
* The current status of the specified job.
* @see JobStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the specified job.
*
*
* @return The current status of the specified job.
* @see JobStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the specified job.
*
*
* @param status
* The current status of the specified job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobStatus
*/
public JobDescriptor withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the specified job.
*
*
* @param status
* The current status of the specified job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobStatus
*/
public JobDescriptor withStatus(JobStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The configuration information for the specified job's manifest object.
*
*
* @param manifest
* The configuration information for the specified job's manifest object.
*/
public void setManifest(JobManifest manifest) {
this.manifest = manifest;
}
/**
*
* The configuration information for the specified job's manifest object.
*
*
* @return The configuration information for the specified job's manifest object.
*/
public JobManifest getManifest() {
return this.manifest;
}
/**
*
* The configuration information for the specified job's manifest object.
*
*
* @param manifest
* The configuration information for the specified job's manifest object.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withManifest(JobManifest manifest) {
setManifest(manifest);
return this;
}
/**
*
* The operation that the specified job is configured to run on the objects listed in the manifest.
*
*
* @param operation
* The operation that the specified job is configured to run on the objects listed in the manifest.
*/
public void setOperation(JobOperation operation) {
this.operation = operation;
}
/**
*
* The operation that the specified job is configured to run on the objects listed in the manifest.
*
*
* @return The operation that the specified job is configured to run on the objects listed in the manifest.
*/
public JobOperation getOperation() {
return this.operation;
}
/**
*
* The operation that the specified job is configured to run on the objects listed in the manifest.
*
*
* @param operation
* The operation that the specified job is configured to run on the objects listed in the manifest.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withOperation(JobOperation operation) {
setOperation(operation);
return this;
}
/**
*
* The priority of the specified job.
*
*
* @param priority
* The priority of the specified job.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* The priority of the specified job.
*
*
* @return The priority of the specified job.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* The priority of the specified job.
*
*
* @param priority
* The priority of the specified job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* Describes the total number of tasks that the specified job has run, the number of tasks that succeeded, and the
* number of tasks that failed.
*
*
* @param progressSummary
* Describes the total number of tasks that the specified job has run, the number of tasks that succeeded,
* and the number of tasks that failed.
*/
public void setProgressSummary(JobProgressSummary progressSummary) {
this.progressSummary = progressSummary;
}
/**
*
* Describes the total number of tasks that the specified job has run, the number of tasks that succeeded, and the
* number of tasks that failed.
*
*
* @return Describes the total number of tasks that the specified job has run, the number of tasks that succeeded,
* and the number of tasks that failed.
*/
public JobProgressSummary getProgressSummary() {
return this.progressSummary;
}
/**
*
* Describes the total number of tasks that the specified job has run, the number of tasks that succeeded, and the
* number of tasks that failed.
*
*
* @param progressSummary
* Describes the total number of tasks that the specified job has run, the number of tasks that succeeded,
* and the number of tasks that failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withProgressSummary(JobProgressSummary progressSummary) {
setProgressSummary(progressSummary);
return this;
}
/**
*
* The reason for updating the job.
*
*
* @param statusUpdateReason
* The reason for updating the job.
*/
public void setStatusUpdateReason(String statusUpdateReason) {
this.statusUpdateReason = statusUpdateReason;
}
/**
*
* The reason for updating the job.
*
*
* @return The reason for updating the job.
*/
public String getStatusUpdateReason() {
return this.statusUpdateReason;
}
/**
*
* The reason for updating the job.
*
*
* @param statusUpdateReason
* The reason for updating the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withStatusUpdateReason(String statusUpdateReason) {
setStatusUpdateReason(statusUpdateReason);
return this;
}
/**
*
* If the specified job failed, this field contains information describing the failure.
*
*
* @return If the specified job failed, this field contains information describing the failure.
*/
public java.util.List getFailureReasons() {
return failureReasons;
}
/**
*
* If the specified job failed, this field contains information describing the failure.
*
*
* @param failureReasons
* If the specified job failed, this field contains information describing the failure.
*/
public void setFailureReasons(java.util.Collection failureReasons) {
if (failureReasons == null) {
this.failureReasons = null;
return;
}
this.failureReasons = new java.util.ArrayList(failureReasons);
}
/**
*
* If the specified job failed, this field contains information describing the failure.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFailureReasons(java.util.Collection)} or {@link #withFailureReasons(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param failureReasons
* If the specified job failed, this field contains information describing the failure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withFailureReasons(JobFailure... failureReasons) {
if (this.failureReasons == null) {
setFailureReasons(new java.util.ArrayList(failureReasons.length));
}
for (JobFailure ele : failureReasons) {
this.failureReasons.add(ele);
}
return this;
}
/**
*
* If the specified job failed, this field contains information describing the failure.
*
*
* @param failureReasons
* If the specified job failed, this field contains information describing the failure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withFailureReasons(java.util.Collection failureReasons) {
setFailureReasons(failureReasons);
return this;
}
/**
*
* Contains the configuration information for the job-completion report if you requested one in the
* Create Job
request.
*
*
* @param report
* Contains the configuration information for the job-completion report if you requested one in the
* Create Job
request.
*/
public void setReport(JobReport report) {
this.report = report;
}
/**
*
* Contains the configuration information for the job-completion report if you requested one in the
* Create Job
request.
*
*
* @return Contains the configuration information for the job-completion report if you requested one in the
* Create Job
request.
*/
public JobReport getReport() {
return this.report;
}
/**
*
* Contains the configuration information for the job-completion report if you requested one in the
* Create Job
request.
*
*
* @param report
* Contains the configuration information for the job-completion report if you requested one in the
* Create Job
request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withReport(JobReport report) {
setReport(report);
return this;
}
/**
*
* A timestamp indicating when this job was created.
*
*
* @param creationTime
* A timestamp indicating when this job was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* A timestamp indicating when this job was created.
*
*
* @return A timestamp indicating when this job was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* A timestamp indicating when this job was created.
*
*
* @param creationTime
* A timestamp indicating when this job was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* A timestamp indicating when this job terminated. A job's termination date is the date and time when it succeeded,
* failed, or was canceled.
*
*
* @param terminationDate
* A timestamp indicating when this job terminated. A job's termination date is the date and time when it
* succeeded, failed, or was canceled.
*/
public void setTerminationDate(java.util.Date terminationDate) {
this.terminationDate = terminationDate;
}
/**
*
* A timestamp indicating when this job terminated. A job's termination date is the date and time when it succeeded,
* failed, or was canceled.
*
*
* @return A timestamp indicating when this job terminated. A job's termination date is the date and time when it
* succeeded, failed, or was canceled.
*/
public java.util.Date getTerminationDate() {
return this.terminationDate;
}
/**
*
* A timestamp indicating when this job terminated. A job's termination date is the date and time when it succeeded,
* failed, or was canceled.
*
*
* @param terminationDate
* A timestamp indicating when this job terminated. A job's termination date is the date and time when it
* succeeded, failed, or was canceled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withTerminationDate(java.util.Date terminationDate) {
setTerminationDate(terminationDate);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role assigned to run the tasks for
* this job.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role assigned to run the tasks
* for this job.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role assigned to run the tasks for
* this job.
*
*
* @return The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role assigned to run the
* tasks for this job.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role assigned to run the tasks for
* this job.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role assigned to run the tasks
* for this job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The timestamp when this job was suspended, if it has been suspended.
*
*
* @param suspendedDate
* The timestamp when this job was suspended, if it has been suspended.
*/
public void setSuspendedDate(java.util.Date suspendedDate) {
this.suspendedDate = suspendedDate;
}
/**
*
* The timestamp when this job was suspended, if it has been suspended.
*
*
* @return The timestamp when this job was suspended, if it has been suspended.
*/
public java.util.Date getSuspendedDate() {
return this.suspendedDate;
}
/**
*
* The timestamp when this job was suspended, if it has been suspended.
*
*
* @param suspendedDate
* The timestamp when this job was suspended, if it has been suspended.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withSuspendedDate(java.util.Date suspendedDate) {
setSuspendedDate(suspendedDate);
return this;
}
/**
*
* The reason why the specified job was suspended. A job is only suspended if you create it through the Amazon S3
* console. When you create the job, it enters the Suspended
state to await confirmation before
* running. After you confirm the job, it automatically exits the Suspended
state.
*
*
* @param suspendedCause
* The reason why the specified job was suspended. A job is only suspended if you create it through the
* Amazon S3 console. When you create the job, it enters the Suspended
state to await
* confirmation before running. After you confirm the job, it automatically exits the Suspended
* state.
*/
public void setSuspendedCause(String suspendedCause) {
this.suspendedCause = suspendedCause;
}
/**
*
* The reason why the specified job was suspended. A job is only suspended if you create it through the Amazon S3
* console. When you create the job, it enters the Suspended
state to await confirmation before
* running. After you confirm the job, it automatically exits the Suspended
state.
*
*
* @return The reason why the specified job was suspended. A job is only suspended if you create it through the
* Amazon S3 console. When you create the job, it enters the Suspended
state to await
* confirmation before running. After you confirm the job, it automatically exits the Suspended
* state.
*/
public String getSuspendedCause() {
return this.suspendedCause;
}
/**
*
* The reason why the specified job was suspended. A job is only suspended if you create it through the Amazon S3
* console. When you create the job, it enters the Suspended
state to await confirmation before
* running. After you confirm the job, it automatically exits the Suspended
state.
*
*
* @param suspendedCause
* The reason why the specified job was suspended. A job is only suspended if you create it through the
* Amazon S3 console. When you create the job, it enters the Suspended
state to await
* confirmation before running. After you confirm the job, it automatically exits the Suspended
* state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withSuspendedCause(String suspendedCause) {
setSuspendedCause(suspendedCause);
return this;
}
/**
*
* The manifest generator that was used to generate a job manifest for this job.
*
*
* @param manifestGenerator
* The manifest generator that was used to generate a job manifest for this job.
*/
public void setManifestGenerator(JobManifestGenerator manifestGenerator) {
this.manifestGenerator = manifestGenerator;
}
/**
*
* The manifest generator that was used to generate a job manifest for this job.
*
*
* @return The manifest generator that was used to generate a job manifest for this job.
*/
public JobManifestGenerator getManifestGenerator() {
return this.manifestGenerator;
}
/**
*
* The manifest generator that was used to generate a job manifest for this job.
*
*
* @param manifestGenerator
* The manifest generator that was used to generate a job manifest for this job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withManifestGenerator(JobManifestGenerator manifestGenerator) {
setManifestGenerator(manifestGenerator);
return this;
}
/**
*
* The attribute of the JobDescriptor containing details about the job's generated manifest.
*
*
* @param generatedManifestDescriptor
* The attribute of the JobDescriptor containing details about the job's generated manifest.
*/
public void setGeneratedManifestDescriptor(S3GeneratedManifestDescriptor generatedManifestDescriptor) {
this.generatedManifestDescriptor = generatedManifestDescriptor;
}
/**
*
* The attribute of the JobDescriptor containing details about the job's generated manifest.
*
*
* @return The attribute of the JobDescriptor containing details about the job's generated manifest.
*/
public S3GeneratedManifestDescriptor getGeneratedManifestDescriptor() {
return this.generatedManifestDescriptor;
}
/**
*
* The attribute of the JobDescriptor containing details about the job's generated manifest.
*
*
* @param generatedManifestDescriptor
* The attribute of the JobDescriptor containing details about the job's generated manifest.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public JobDescriptor withGeneratedManifestDescriptor(S3GeneratedManifestDescriptor generatedManifestDescriptor) {
setGeneratedManifestDescriptor(generatedManifestDescriptor);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getJobId() != null)
sb.append("JobId: ").append(getJobId()).append(",");
if (getConfirmationRequired() != null)
sb.append("ConfirmationRequired: ").append(getConfirmationRequired()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getJobArn() != null)
sb.append("JobArn: ").append(getJobArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getManifest() != null)
sb.append("Manifest: ").append(getManifest()).append(",");
if (getOperation() != null)
sb.append("Operation: ").append(getOperation()).append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getProgressSummary() != null)
sb.append("ProgressSummary: ").append(getProgressSummary()).append(",");
if (getStatusUpdateReason() != null)
sb.append("StatusUpdateReason: ").append(getStatusUpdateReason()).append(",");
if (getFailureReasons() != null)
sb.append("FailureReasons: ").append(getFailureReasons()).append(",");
if (getReport() != null)
sb.append("Report: ").append(getReport()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getTerminationDate() != null)
sb.append("TerminationDate: ").append(getTerminationDate()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getSuspendedDate() != null)
sb.append("SuspendedDate: ").append(getSuspendedDate()).append(",");
if (getSuspendedCause() != null)
sb.append("SuspendedCause: ").append(getSuspendedCause()).append(",");
if (getManifestGenerator() != null)
sb.append("ManifestGenerator: ").append(getManifestGenerator()).append(",");
if (getGeneratedManifestDescriptor() != null)
sb.append("GeneratedManifestDescriptor: ").append(getGeneratedManifestDescriptor());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof JobDescriptor == false)
return false;
JobDescriptor other = (JobDescriptor) obj;
if (other.getJobId() == null ^ this.getJobId() == null)
return false;
if (other.getJobId() != null && other.getJobId().equals(this.getJobId()) == false)
return false;
if (other.getConfirmationRequired() == null ^ this.getConfirmationRequired() == null)
return false;
if (other.getConfirmationRequired() != null && other.getConfirmationRequired().equals(this.getConfirmationRequired()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getJobArn() == null ^ this.getJobArn() == null)
return false;
if (other.getJobArn() != null && other.getJobArn().equals(this.getJobArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getManifest() == null ^ this.getManifest() == null)
return false;
if (other.getManifest() != null && other.getManifest().equals(this.getManifest()) == false)
return false;
if (other.getOperation() == null ^ this.getOperation() == null)
return false;
if (other.getOperation() != null && other.getOperation().equals(this.getOperation()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getProgressSummary() == null ^ this.getProgressSummary() == null)
return false;
if (other.getProgressSummary() != null && other.getProgressSummary().equals(this.getProgressSummary()) == false)
return false;
if (other.getStatusUpdateReason() == null ^ this.getStatusUpdateReason() == null)
return false;
if (other.getStatusUpdateReason() != null && other.getStatusUpdateReason().equals(this.getStatusUpdateReason()) == false)
return false;
if (other.getFailureReasons() == null ^ this.getFailureReasons() == null)
return false;
if (other.getFailureReasons() != null && other.getFailureReasons().equals(this.getFailureReasons()) == false)
return false;
if (other.getReport() == null ^ this.getReport() == null)
return false;
if (other.getReport() != null && other.getReport().equals(this.getReport()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getTerminationDate() == null ^ this.getTerminationDate() == null)
return false;
if (other.getTerminationDate() != null && other.getTerminationDate().equals(this.getTerminationDate()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getSuspendedDate() == null ^ this.getSuspendedDate() == null)
return false;
if (other.getSuspendedDate() != null && other.getSuspendedDate().equals(this.getSuspendedDate()) == false)
return false;
if (other.getSuspendedCause() == null ^ this.getSuspendedCause() == null)
return false;
if (other.getSuspendedCause() != null && other.getSuspendedCause().equals(this.getSuspendedCause()) == false)
return false;
if (other.getManifestGenerator() == null ^ this.getManifestGenerator() == null)
return false;
if (other.getManifestGenerator() != null && other.getManifestGenerator().equals(this.getManifestGenerator()) == false)
return false;
if (other.getGeneratedManifestDescriptor() == null ^ this.getGeneratedManifestDescriptor() == null)
return false;
if (other.getGeneratedManifestDescriptor() != null && other.getGeneratedManifestDescriptor().equals(this.getGeneratedManifestDescriptor()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getJobId() == null) ? 0 : getJobId().hashCode());
hashCode = prime * hashCode + ((getConfirmationRequired() == null) ? 0 : getConfirmationRequired().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getJobArn() == null) ? 0 : getJobArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getManifest() == null) ? 0 : getManifest().hashCode());
hashCode = prime * hashCode + ((getOperation() == null) ? 0 : getOperation().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getProgressSummary() == null) ? 0 : getProgressSummary().hashCode());
hashCode = prime * hashCode + ((getStatusUpdateReason() == null) ? 0 : getStatusUpdateReason().hashCode());
hashCode = prime * hashCode + ((getFailureReasons() == null) ? 0 : getFailureReasons().hashCode());
hashCode = prime * hashCode + ((getReport() == null) ? 0 : getReport().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getTerminationDate() == null) ? 0 : getTerminationDate().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getSuspendedDate() == null) ? 0 : getSuspendedDate().hashCode());
hashCode = prime * hashCode + ((getSuspendedCause() == null) ? 0 : getSuspendedCause().hashCode());
hashCode = prime * hashCode + ((getManifestGenerator() == null) ? 0 : getManifestGenerator().hashCode());
hashCode = prime * hashCode + ((getGeneratedManifestDescriptor() == null) ? 0 : getGeneratedManifestDescriptor().hashCode());
return hashCode;
}
@Override
public JobDescriptor clone() {
try {
return (JobDescriptor) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}