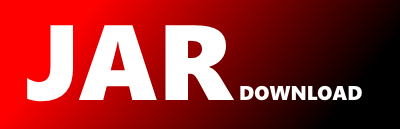
com.amazonaws.services.s3control.model.CreateJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-s3control Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.s3control.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Web Services account ID that creates the job.
*
*/
private String accountId;
/**
*
* Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required for jobs
* created through the Amazon S3 console.
*
*/
private Boolean confirmationRequired;
/**
*
* The action that you want this job to perform on every object listed in the manifest. For more information about
* the available actions, see Operations in the Amazon S3
* User Guide.
*
*/
private JobOperation operation;
/**
*
* Configuration parameters for the optional job-completion report.
*
*/
private JobReport report;
/**
*
* An idempotency token to ensure that you don't accidentally submit the same request twice. You can use any string
* up to the maximum length.
*
*/
private String clientRequestToken;
/**
*
* Configuration parameters for the manifest.
*
*/
private JobManifest manifest;
/**
*
* A description for this job. You can use any string within the permitted length. Descriptions don't need to be
* unique and can be used for multiple jobs.
*
*/
private String description;
/**
*
* The numerical priority for this job. Higher numbers indicate higher priority.
*
*/
private Integer priority;
/**
*
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role that Batch Operations will use
* to run this job's action on every object in the manifest.
*
*/
private String roleArn;
/**
*
* A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
*
*/
private java.util.List tags;
/**
*
* The attribute container for the ManifestGenerator details. Jobs must be created with either a manifest file or a
* ManifestGenerator, but not both.
*
*/
private JobManifestGenerator manifestGenerator;
/**
*
* The Amazon Web Services account ID that creates the job.
*
*
* @param accountId
* The Amazon Web Services account ID that creates the job.
*/
public void setAccountId(String accountId) {
this.accountId = accountId;
}
/**
*
* The Amazon Web Services account ID that creates the job.
*
*
* @return The Amazon Web Services account ID that creates the job.
*/
public String getAccountId() {
return this.accountId;
}
/**
*
* The Amazon Web Services account ID that creates the job.
*
*
* @param accountId
* The Amazon Web Services account ID that creates the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withAccountId(String accountId) {
setAccountId(accountId);
return this;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required for jobs
* created through the Amazon S3 console.
*
*
* @param confirmationRequired
* Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required
* for jobs created through the Amazon S3 console.
*/
public void setConfirmationRequired(Boolean confirmationRequired) {
this.confirmationRequired = confirmationRequired;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required for jobs
* created through the Amazon S3 console.
*
*
* @return Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required
* for jobs created through the Amazon S3 console.
*/
public Boolean getConfirmationRequired() {
return this.confirmationRequired;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required for jobs
* created through the Amazon S3 console.
*
*
* @param confirmationRequired
* Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required
* for jobs created through the Amazon S3 console.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withConfirmationRequired(Boolean confirmationRequired) {
setConfirmationRequired(confirmationRequired);
return this;
}
/**
*
* Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required for jobs
* created through the Amazon S3 console.
*
*
* @return Indicates whether confirmation is required before Amazon S3 runs the job. Confirmation is only required
* for jobs created through the Amazon S3 console.
*/
public Boolean isConfirmationRequired() {
return this.confirmationRequired;
}
/**
*
* The action that you want this job to perform on every object listed in the manifest. For more information about
* the available actions, see Operations in the Amazon S3
* User Guide.
*
*
* @param operation
* The action that you want this job to perform on every object listed in the manifest. For more information
* about the available actions, see Operations in the
* Amazon S3 User Guide.
*/
public void setOperation(JobOperation operation) {
this.operation = operation;
}
/**
*
* The action that you want this job to perform on every object listed in the manifest. For more information about
* the available actions, see Operations in the Amazon S3
* User Guide.
*
*
* @return The action that you want this job to perform on every object listed in the manifest. For more information
* about the available actions, see Operations in the
* Amazon S3 User Guide.
*/
public JobOperation getOperation() {
return this.operation;
}
/**
*
* The action that you want this job to perform on every object listed in the manifest. For more information about
* the available actions, see Operations in the Amazon S3
* User Guide.
*
*
* @param operation
* The action that you want this job to perform on every object listed in the manifest. For more information
* about the available actions, see Operations in the
* Amazon S3 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withOperation(JobOperation operation) {
setOperation(operation);
return this;
}
/**
*
* Configuration parameters for the optional job-completion report.
*
*
* @param report
* Configuration parameters for the optional job-completion report.
*/
public void setReport(JobReport report) {
this.report = report;
}
/**
*
* Configuration parameters for the optional job-completion report.
*
*
* @return Configuration parameters for the optional job-completion report.
*/
public JobReport getReport() {
return this.report;
}
/**
*
* Configuration parameters for the optional job-completion report.
*
*
* @param report
* Configuration parameters for the optional job-completion report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withReport(JobReport report) {
setReport(report);
return this;
}
/**
*
* An idempotency token to ensure that you don't accidentally submit the same request twice. You can use any string
* up to the maximum length.
*
*
* @param clientRequestToken
* An idempotency token to ensure that you don't accidentally submit the same request twice. You can use any
* string up to the maximum length.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* An idempotency token to ensure that you don't accidentally submit the same request twice. You can use any string
* up to the maximum length.
*
*
* @return An idempotency token to ensure that you don't accidentally submit the same request twice. You can use any
* string up to the maximum length.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* An idempotency token to ensure that you don't accidentally submit the same request twice. You can use any string
* up to the maximum length.
*
*
* @param clientRequestToken
* An idempotency token to ensure that you don't accidentally submit the same request twice. You can use any
* string up to the maximum length.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* Configuration parameters for the manifest.
*
*
* @param manifest
* Configuration parameters for the manifest.
*/
public void setManifest(JobManifest manifest) {
this.manifest = manifest;
}
/**
*
* Configuration parameters for the manifest.
*
*
* @return Configuration parameters for the manifest.
*/
public JobManifest getManifest() {
return this.manifest;
}
/**
*
* Configuration parameters for the manifest.
*
*
* @param manifest
* Configuration parameters for the manifest.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withManifest(JobManifest manifest) {
setManifest(manifest);
return this;
}
/**
*
* A description for this job. You can use any string within the permitted length. Descriptions don't need to be
* unique and can be used for multiple jobs.
*
*
* @param description
* A description for this job. You can use any string within the permitted length. Descriptions don't need to
* be unique and can be used for multiple jobs.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description for this job. You can use any string within the permitted length. Descriptions don't need to be
* unique and can be used for multiple jobs.
*
*
* @return A description for this job. You can use any string within the permitted length. Descriptions don't need
* to be unique and can be used for multiple jobs.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description for this job. You can use any string within the permitted length. Descriptions don't need to be
* unique and can be used for multiple jobs.
*
*
* @param description
* A description for this job. You can use any string within the permitted length. Descriptions don't need to
* be unique and can be used for multiple jobs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The numerical priority for this job. Higher numbers indicate higher priority.
*
*
* @param priority
* The numerical priority for this job. Higher numbers indicate higher priority.
*/
public void setPriority(Integer priority) {
this.priority = priority;
}
/**
*
* The numerical priority for this job. Higher numbers indicate higher priority.
*
*
* @return The numerical priority for this job. Higher numbers indicate higher priority.
*/
public Integer getPriority() {
return this.priority;
}
/**
*
* The numerical priority for this job. Higher numbers indicate higher priority.
*
*
* @param priority
* The numerical priority for this job. Higher numbers indicate higher priority.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withPriority(Integer priority) {
setPriority(priority);
return this;
}
/**
*
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role that Batch Operations will use
* to run this job's action on every object in the manifest.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role that Batch Operations
* will use to run this job's action on every object in the manifest.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role that Batch Operations will use
* to run this job's action on every object in the manifest.
*
*
* @return The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role that Batch Operations
* will use to run this job's action on every object in the manifest.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role that Batch Operations will use
* to run this job's action on every object in the manifest.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) for the Identity and Access Management (IAM) role that Batch Operations
* will use to run this job's action on every object in the manifest.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
*
*
* @return A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
*
*
* @param tags
* A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withTags(S3Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (S3Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
*
*
* @param tags
* A set of tags to associate with the S3 Batch Operations job. This is an optional parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The attribute container for the ManifestGenerator details. Jobs must be created with either a manifest file or a
* ManifestGenerator, but not both.
*
*
* @param manifestGenerator
* The attribute container for the ManifestGenerator details. Jobs must be created with either a manifest
* file or a ManifestGenerator, but not both.
*/
public void setManifestGenerator(JobManifestGenerator manifestGenerator) {
this.manifestGenerator = manifestGenerator;
}
/**
*
* The attribute container for the ManifestGenerator details. Jobs must be created with either a manifest file or a
* ManifestGenerator, but not both.
*
*
* @return The attribute container for the ManifestGenerator details. Jobs must be created with either a manifest
* file or a ManifestGenerator, but not both.
*/
public JobManifestGenerator getManifestGenerator() {
return this.manifestGenerator;
}
/**
*
* The attribute container for the ManifestGenerator details. Jobs must be created with either a manifest file or a
* ManifestGenerator, but not both.
*
*
* @param manifestGenerator
* The attribute container for the ManifestGenerator details. Jobs must be created with either a manifest
* file or a ManifestGenerator, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateJobRequest withManifestGenerator(JobManifestGenerator manifestGenerator) {
setManifestGenerator(manifestGenerator);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAccountId() != null)
sb.append("AccountId: ").append(getAccountId()).append(",");
if (getConfirmationRequired() != null)
sb.append("ConfirmationRequired: ").append(getConfirmationRequired()).append(",");
if (getOperation() != null)
sb.append("Operation: ").append(getOperation()).append(",");
if (getReport() != null)
sb.append("Report: ").append(getReport()).append(",");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken()).append(",");
if (getManifest() != null)
sb.append("Manifest: ").append(getManifest()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getPriority() != null)
sb.append("Priority: ").append(getPriority()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getManifestGenerator() != null)
sb.append("ManifestGenerator: ").append(getManifestGenerator());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateJobRequest == false)
return false;
CreateJobRequest other = (CreateJobRequest) obj;
if (other.getAccountId() == null ^ this.getAccountId() == null)
return false;
if (other.getAccountId() != null && other.getAccountId().equals(this.getAccountId()) == false)
return false;
if (other.getConfirmationRequired() == null ^ this.getConfirmationRequired() == null)
return false;
if (other.getConfirmationRequired() != null && other.getConfirmationRequired().equals(this.getConfirmationRequired()) == false)
return false;
if (other.getOperation() == null ^ this.getOperation() == null)
return false;
if (other.getOperation() != null && other.getOperation().equals(this.getOperation()) == false)
return false;
if (other.getReport() == null ^ this.getReport() == null)
return false;
if (other.getReport() != null && other.getReport().equals(this.getReport()) == false)
return false;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getManifest() == null ^ this.getManifest() == null)
return false;
if (other.getManifest() != null && other.getManifest().equals(this.getManifest()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getPriority() == null ^ this.getPriority() == null)
return false;
if (other.getPriority() != null && other.getPriority().equals(this.getPriority()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getManifestGenerator() == null ^ this.getManifestGenerator() == null)
return false;
if (other.getManifestGenerator() != null && other.getManifestGenerator().equals(this.getManifestGenerator()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAccountId() == null) ? 0 : getAccountId().hashCode());
hashCode = prime * hashCode + ((getConfirmationRequired() == null) ? 0 : getConfirmationRequired().hashCode());
hashCode = prime * hashCode + ((getOperation() == null) ? 0 : getOperation().hashCode());
hashCode = prime * hashCode + ((getReport() == null) ? 0 : getReport().hashCode());
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getManifest() == null) ? 0 : getManifest().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getPriority() == null) ? 0 : getPriority().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getManifestGenerator() == null) ? 0 : getManifestGenerator().hashCode());
return hashCode;
}
@Override
public CreateJobRequest clone() {
return (CreateJobRequest) super.clone();
}
}