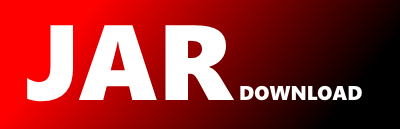
com.amazonaws.services.sagemaker.model.ClarifyInferenceConfig Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The inference configuration parameter for the model container.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ClarifyInferenceConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* Provides the JMESPath expression to extract the features from a model container input in JSON Lines format. For
* example, if FeaturesAttribute
is the JMESPath expression 'myfeatures'
, it extracts a
* list of features [1,2,3]
from request data '{"myfeatures":[1,2,3]}'
.
*
*/
private String featuresAttribute;
/**
*
* A template string used to format a JSON record into an acceptable model container input. For example, a
* ContentTemplate
string '{"myfeatures":$features}'
will format a list of features
* [1,2,3]
into the record string '{"myfeatures":[1,2,3]}'
. Required only when the model
* container input is in JSON Lines format.
*
*/
private String contentTemplate;
/**
*
* The maximum number of records in a request that the model container can process when querying the model container
* for the predictions of a synthetic dataset. A record is a unit of input data that inference can be made on, for example, a single
* line in CSV data. If MaxRecordCount
is 1
, the model container expects one record per
* request. A value of 2 or greater means that the model expects batch requests, which can reduce overhead and speed
* up the inferencing process. If this parameter is not provided, the explainer will tune the record count per
* request according to the model container's capacity at runtime.
*
*/
private Integer maxRecordCount;
/**
*
* The maximum payload size (MB) allowed of a request from the explainer to the model container. Defaults to
* 6
MB.
*
*/
private Integer maxPayloadInMB;
/**
*
* A zero-based index used to extract a probability value (score) or list from model container output in CSV format.
* If this value is not provided, the entire model container output will be treated as a probability value (score)
* or list.
*
*
* Example for a single class model: If the model container output consists of a string-formatted prediction
* label followed by its probability: '1,0.6'
, set ProbabilityIndex
to 1
to
* select the probability value 0.6
.
*
*
* Example for a multiclass model: If the model container output consists of a string-formatted prediction
* label followed by its probability: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set
* ProbabilityIndex
to 1
to select the probability values [0.1,0.6,0.3]
.
*
*/
private Integer probabilityIndex;
/**
*
* A zero-based index used to extract a label header or list of label headers from model container output in CSV
* format.
*
*
* Example for a multiclass model: If the model container output consists of label headers followed by
* probabilities: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set LabelIndex
to
* 0
to select the label headers ['cat','dog','fish']
.
*
*/
private Integer labelIndex;
/**
*
* A JMESPath expression used to extract the probability (or score) from the model container output if the model
* container is in JSON Lines format.
*
*
* Example: If the model container output of a single request is
* '{"predicted_label":1,"probability":0.6}'
, then set ProbabilityAttribute
to
* 'probability'
.
*
*/
private String probabilityAttribute;
/**
*
* A JMESPath expression used to locate the list of label headers in the model container output.
*
*
* Example: If the model container output of a batch request is
* '{"labels":["cat","dog","fish"],"probability":[0.6,0.3,0.1]}'
, then set LabelAttribute
* to 'labels'
to extract the list of label headers ["cat","dog","fish"]
*
*/
private String labelAttribute;
/**
*
* For multiclass classification problems, the label headers are the names of the classes. Otherwise, the label
* header is the name of the predicted label. These are used to help readability for the output of the
* InvokeEndpoint
API. See the response section under Invoke the endpoint in the Developer Guide for more information. If there are
* no label headers in the model container output, provide them manually using this parameter.
*
*/
private java.util.List labelHeaders;
/**
*
* The names of the features. If provided, these are included in the endpoint response payload to help readability
* of the InvokeEndpoint
output. See the Response section under Invoke the endpoint in the Developer Guide for more information.
*
*/
private java.util.List featureHeaders;
/**
*
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example, ['text']
).
* If FeatureTypes
is not provided, the explainer infers the feature types based on the baseline data.
* The feature types are included in the endpoint response payload. For additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
*
*/
private java.util.List featureTypes;
/**
*
* Provides the JMESPath expression to extract the features from a model container input in JSON Lines format. For
* example, if FeaturesAttribute
is the JMESPath expression 'myfeatures'
, it extracts a
* list of features [1,2,3]
from request data '{"myfeatures":[1,2,3]}'
.
*
*
* @param featuresAttribute
* Provides the JMESPath expression to extract the features from a model container input in JSON Lines
* format. For example, if FeaturesAttribute
is the JMESPath expression
* 'myfeatures'
, it extracts a list of features [1,2,3]
from request data
* '{"myfeatures":[1,2,3]}'
.
*/
public void setFeaturesAttribute(String featuresAttribute) {
this.featuresAttribute = featuresAttribute;
}
/**
*
* Provides the JMESPath expression to extract the features from a model container input in JSON Lines format. For
* example, if FeaturesAttribute
is the JMESPath expression 'myfeatures'
, it extracts a
* list of features [1,2,3]
from request data '{"myfeatures":[1,2,3]}'
.
*
*
* @return Provides the JMESPath expression to extract the features from a model container input in JSON Lines
* format. For example, if FeaturesAttribute
is the JMESPath expression
* 'myfeatures'
, it extracts a list of features [1,2,3]
from request data
* '{"myfeatures":[1,2,3]}'
.
*/
public String getFeaturesAttribute() {
return this.featuresAttribute;
}
/**
*
* Provides the JMESPath expression to extract the features from a model container input in JSON Lines format. For
* example, if FeaturesAttribute
is the JMESPath expression 'myfeatures'
, it extracts a
* list of features [1,2,3]
from request data '{"myfeatures":[1,2,3]}'
.
*
*
* @param featuresAttribute
* Provides the JMESPath expression to extract the features from a model container input in JSON Lines
* format. For example, if FeaturesAttribute
is the JMESPath expression
* 'myfeatures'
, it extracts a list of features [1,2,3]
from request data
* '{"myfeatures":[1,2,3]}'
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withFeaturesAttribute(String featuresAttribute) {
setFeaturesAttribute(featuresAttribute);
return this;
}
/**
*
* A template string used to format a JSON record into an acceptable model container input. For example, a
* ContentTemplate
string '{"myfeatures":$features}'
will format a list of features
* [1,2,3]
into the record string '{"myfeatures":[1,2,3]}'
. Required only when the model
* container input is in JSON Lines format.
*
*
* @param contentTemplate
* A template string used to format a JSON record into an acceptable model container input. For example, a
* ContentTemplate
string '{"myfeatures":$features}'
will format a list of features
* [1,2,3]
into the record string '{"myfeatures":[1,2,3]}'
. Required only when the
* model container input is in JSON Lines format.
*/
public void setContentTemplate(String contentTemplate) {
this.contentTemplate = contentTemplate;
}
/**
*
* A template string used to format a JSON record into an acceptable model container input. For example, a
* ContentTemplate
string '{"myfeatures":$features}'
will format a list of features
* [1,2,3]
into the record string '{"myfeatures":[1,2,3]}'
. Required only when the model
* container input is in JSON Lines format.
*
*
* @return A template string used to format a JSON record into an acceptable model container input. For example, a
* ContentTemplate
string '{"myfeatures":$features}'
will format a list of
* features [1,2,3]
into the record string '{"myfeatures":[1,2,3]}'
. Required only
* when the model container input is in JSON Lines format.
*/
public String getContentTemplate() {
return this.contentTemplate;
}
/**
*
* A template string used to format a JSON record into an acceptable model container input. For example, a
* ContentTemplate
string '{"myfeatures":$features}'
will format a list of features
* [1,2,3]
into the record string '{"myfeatures":[1,2,3]}'
. Required only when the model
* container input is in JSON Lines format.
*
*
* @param contentTemplate
* A template string used to format a JSON record into an acceptable model container input. For example, a
* ContentTemplate
string '{"myfeatures":$features}'
will format a list of features
* [1,2,3]
into the record string '{"myfeatures":[1,2,3]}'
. Required only when the
* model container input is in JSON Lines format.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withContentTemplate(String contentTemplate) {
setContentTemplate(contentTemplate);
return this;
}
/**
*
* The maximum number of records in a request that the model container can process when querying the model container
* for the predictions of a synthetic dataset. A record is a unit of input data that inference can be made on, for example, a single
* line in CSV data. If MaxRecordCount
is 1
, the model container expects one record per
* request. A value of 2 or greater means that the model expects batch requests, which can reduce overhead and speed
* up the inferencing process. If this parameter is not provided, the explainer will tune the record count per
* request according to the model container's capacity at runtime.
*
*
* @param maxRecordCount
* The maximum number of records in a request that the model container can process when querying the model
* container for the predictions of a synthetic dataset. A record is a unit of input data that inference can be made on, for example, a
* single line in CSV data. If MaxRecordCount
is 1
, the model container expects one
* record per request. A value of 2 or greater means that the model expects batch requests, which can reduce
* overhead and speed up the inferencing process. If this parameter is not provided, the explainer will tune
* the record count per request according to the model container's capacity at runtime.
*/
public void setMaxRecordCount(Integer maxRecordCount) {
this.maxRecordCount = maxRecordCount;
}
/**
*
* The maximum number of records in a request that the model container can process when querying the model container
* for the predictions of a synthetic dataset. A record is a unit of input data that inference can be made on, for example, a single
* line in CSV data. If MaxRecordCount
is 1
, the model container expects one record per
* request. A value of 2 or greater means that the model expects batch requests, which can reduce overhead and speed
* up the inferencing process. If this parameter is not provided, the explainer will tune the record count per
* request according to the model container's capacity at runtime.
*
*
* @return The maximum number of records in a request that the model container can process when querying the model
* container for the predictions of a synthetic dataset. A record is a unit of input data that inference can be made on, for example, a
* single line in CSV data. If MaxRecordCount
is 1
, the model container expects
* one record per request. A value of 2 or greater means that the model expects batch requests, which can
* reduce overhead and speed up the inferencing process. If this parameter is not provided, the explainer
* will tune the record count per request according to the model container's capacity at runtime.
*/
public Integer getMaxRecordCount() {
return this.maxRecordCount;
}
/**
*
* The maximum number of records in a request that the model container can process when querying the model container
* for the predictions of a synthetic dataset. A record is a unit of input data that inference can be made on, for example, a single
* line in CSV data. If MaxRecordCount
is 1
, the model container expects one record per
* request. A value of 2 or greater means that the model expects batch requests, which can reduce overhead and speed
* up the inferencing process. If this parameter is not provided, the explainer will tune the record count per
* request according to the model container's capacity at runtime.
*
*
* @param maxRecordCount
* The maximum number of records in a request that the model container can process when querying the model
* container for the predictions of a synthetic dataset. A record is a unit of input data that inference can be made on, for example, a
* single line in CSV data. If MaxRecordCount
is 1
, the model container expects one
* record per request. A value of 2 or greater means that the model expects batch requests, which can reduce
* overhead and speed up the inferencing process. If this parameter is not provided, the explainer will tune
* the record count per request according to the model container's capacity at runtime.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withMaxRecordCount(Integer maxRecordCount) {
setMaxRecordCount(maxRecordCount);
return this;
}
/**
*
* The maximum payload size (MB) allowed of a request from the explainer to the model container. Defaults to
* 6
MB.
*
*
* @param maxPayloadInMB
* The maximum payload size (MB) allowed of a request from the explainer to the model container. Defaults to
* 6
MB.
*/
public void setMaxPayloadInMB(Integer maxPayloadInMB) {
this.maxPayloadInMB = maxPayloadInMB;
}
/**
*
* The maximum payload size (MB) allowed of a request from the explainer to the model container. Defaults to
* 6
MB.
*
*
* @return The maximum payload size (MB) allowed of a request from the explainer to the model container. Defaults to
* 6
MB.
*/
public Integer getMaxPayloadInMB() {
return this.maxPayloadInMB;
}
/**
*
* The maximum payload size (MB) allowed of a request from the explainer to the model container. Defaults to
* 6
MB.
*
*
* @param maxPayloadInMB
* The maximum payload size (MB) allowed of a request from the explainer to the model container. Defaults to
* 6
MB.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withMaxPayloadInMB(Integer maxPayloadInMB) {
setMaxPayloadInMB(maxPayloadInMB);
return this;
}
/**
*
* A zero-based index used to extract a probability value (score) or list from model container output in CSV format.
* If this value is not provided, the entire model container output will be treated as a probability value (score)
* or list.
*
*
* Example for a single class model: If the model container output consists of a string-formatted prediction
* label followed by its probability: '1,0.6'
, set ProbabilityIndex
to 1
to
* select the probability value 0.6
.
*
*
* Example for a multiclass model: If the model container output consists of a string-formatted prediction
* label followed by its probability: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set
* ProbabilityIndex
to 1
to select the probability values [0.1,0.6,0.3]
.
*
*
* @param probabilityIndex
* A zero-based index used to extract a probability value (score) or list from model container output in CSV
* format. If this value is not provided, the entire model container output will be treated as a probability
* value (score) or list.
*
* Example for a single class model: If the model container output consists of a string-formatted
* prediction label followed by its probability: '1,0.6'
, set ProbabilityIndex
to
* 1
to select the probability value 0.6
.
*
*
* Example for a multiclass model: If the model container output consists of a string-formatted
* prediction label followed by its probability: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
,
* set ProbabilityIndex
to 1
to select the probability values
* [0.1,0.6,0.3]
.
*/
public void setProbabilityIndex(Integer probabilityIndex) {
this.probabilityIndex = probabilityIndex;
}
/**
*
* A zero-based index used to extract a probability value (score) or list from model container output in CSV format.
* If this value is not provided, the entire model container output will be treated as a probability value (score)
* or list.
*
*
* Example for a single class model: If the model container output consists of a string-formatted prediction
* label followed by its probability: '1,0.6'
, set ProbabilityIndex
to 1
to
* select the probability value 0.6
.
*
*
* Example for a multiclass model: If the model container output consists of a string-formatted prediction
* label followed by its probability: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set
* ProbabilityIndex
to 1
to select the probability values [0.1,0.6,0.3]
.
*
*
* @return A zero-based index used to extract a probability value (score) or list from model container output in CSV
* format. If this value is not provided, the entire model container output will be treated as a probability
* value (score) or list.
*
* Example for a single class model: If the model container output consists of a string-formatted
* prediction label followed by its probability: '1,0.6'
, set ProbabilityIndex
to
* 1
to select the probability value 0.6
.
*
*
* Example for a multiclass model: If the model container output consists of a string-formatted
* prediction label followed by its probability: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
* , set ProbabilityIndex
to 1
to select the probability values
* [0.1,0.6,0.3]
.
*/
public Integer getProbabilityIndex() {
return this.probabilityIndex;
}
/**
*
* A zero-based index used to extract a probability value (score) or list from model container output in CSV format.
* If this value is not provided, the entire model container output will be treated as a probability value (score)
* or list.
*
*
* Example for a single class model: If the model container output consists of a string-formatted prediction
* label followed by its probability: '1,0.6'
, set ProbabilityIndex
to 1
to
* select the probability value 0.6
.
*
*
* Example for a multiclass model: If the model container output consists of a string-formatted prediction
* label followed by its probability: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set
* ProbabilityIndex
to 1
to select the probability values [0.1,0.6,0.3]
.
*
*
* @param probabilityIndex
* A zero-based index used to extract a probability value (score) or list from model container output in CSV
* format. If this value is not provided, the entire model container output will be treated as a probability
* value (score) or list.
*
* Example for a single class model: If the model container output consists of a string-formatted
* prediction label followed by its probability: '1,0.6'
, set ProbabilityIndex
to
* 1
to select the probability value 0.6
.
*
*
* Example for a multiclass model: If the model container output consists of a string-formatted
* prediction label followed by its probability: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
,
* set ProbabilityIndex
to 1
to select the probability values
* [0.1,0.6,0.3]
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withProbabilityIndex(Integer probabilityIndex) {
setProbabilityIndex(probabilityIndex);
return this;
}
/**
*
* A zero-based index used to extract a label header or list of label headers from model container output in CSV
* format.
*
*
* Example for a multiclass model: If the model container output consists of label headers followed by
* probabilities: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set LabelIndex
to
* 0
to select the label headers ['cat','dog','fish']
.
*
*
* @param labelIndex
* A zero-based index used to extract a label header or list of label headers from model container output in
* CSV format.
*
* Example for a multiclass model: If the model container output consists of label headers followed by
* probabilities: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set LabelIndex
to
* 0
to select the label headers ['cat','dog','fish']
.
*/
public void setLabelIndex(Integer labelIndex) {
this.labelIndex = labelIndex;
}
/**
*
* A zero-based index used to extract a label header or list of label headers from model container output in CSV
* format.
*
*
* Example for a multiclass model: If the model container output consists of label headers followed by
* probabilities: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set LabelIndex
to
* 0
to select the label headers ['cat','dog','fish']
.
*
*
* @return A zero-based index used to extract a label header or list of label headers from model container output in
* CSV format.
*
* Example for a multiclass model: If the model container output consists of label headers followed
* by probabilities: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set
* LabelIndex
to 0
to select the label headers ['cat','dog','fish']
.
*/
public Integer getLabelIndex() {
return this.labelIndex;
}
/**
*
* A zero-based index used to extract a label header or list of label headers from model container output in CSV
* format.
*
*
* Example for a multiclass model: If the model container output consists of label headers followed by
* probabilities: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set LabelIndex
to
* 0
to select the label headers ['cat','dog','fish']
.
*
*
* @param labelIndex
* A zero-based index used to extract a label header or list of label headers from model container output in
* CSV format.
*
* Example for a multiclass model: If the model container output consists of label headers followed by
* probabilities: '"[\'cat\',\'dog\',\'fish\']","[0.1,0.6,0.3]"'
, set LabelIndex
to
* 0
to select the label headers ['cat','dog','fish']
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withLabelIndex(Integer labelIndex) {
setLabelIndex(labelIndex);
return this;
}
/**
*
* A JMESPath expression used to extract the probability (or score) from the model container output if the model
* container is in JSON Lines format.
*
*
* Example: If the model container output of a single request is
* '{"predicted_label":1,"probability":0.6}'
, then set ProbabilityAttribute
to
* 'probability'
.
*
*
* @param probabilityAttribute
* A JMESPath expression used to extract the probability (or score) from the model container output if the
* model container is in JSON Lines format.
*
* Example: If the model container output of a single request is
* '{"predicted_label":1,"probability":0.6}'
, then set ProbabilityAttribute
to
* 'probability'
.
*/
public void setProbabilityAttribute(String probabilityAttribute) {
this.probabilityAttribute = probabilityAttribute;
}
/**
*
* A JMESPath expression used to extract the probability (or score) from the model container output if the model
* container is in JSON Lines format.
*
*
* Example: If the model container output of a single request is
* '{"predicted_label":1,"probability":0.6}'
, then set ProbabilityAttribute
to
* 'probability'
.
*
*
* @return A JMESPath expression used to extract the probability (or score) from the model container output if the
* model container is in JSON Lines format.
*
* Example: If the model container output of a single request is
* '{"predicted_label":1,"probability":0.6}'
, then set ProbabilityAttribute
to
* 'probability'
.
*/
public String getProbabilityAttribute() {
return this.probabilityAttribute;
}
/**
*
* A JMESPath expression used to extract the probability (or score) from the model container output if the model
* container is in JSON Lines format.
*
*
* Example: If the model container output of a single request is
* '{"predicted_label":1,"probability":0.6}'
, then set ProbabilityAttribute
to
* 'probability'
.
*
*
* @param probabilityAttribute
* A JMESPath expression used to extract the probability (or score) from the model container output if the
* model container is in JSON Lines format.
*
* Example: If the model container output of a single request is
* '{"predicted_label":1,"probability":0.6}'
, then set ProbabilityAttribute
to
* 'probability'
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withProbabilityAttribute(String probabilityAttribute) {
setProbabilityAttribute(probabilityAttribute);
return this;
}
/**
*
* A JMESPath expression used to locate the list of label headers in the model container output.
*
*
* Example: If the model container output of a batch request is
* '{"labels":["cat","dog","fish"],"probability":[0.6,0.3,0.1]}'
, then set LabelAttribute
* to 'labels'
to extract the list of label headers ["cat","dog","fish"]
*
*
* @param labelAttribute
* A JMESPath expression used to locate the list of label headers in the model container output.
*
* Example: If the model container output of a batch request is
* '{"labels":["cat","dog","fish"],"probability":[0.6,0.3,0.1]}'
, then set
* LabelAttribute
to 'labels'
to extract the list of label headers
* ["cat","dog","fish"]
*/
public void setLabelAttribute(String labelAttribute) {
this.labelAttribute = labelAttribute;
}
/**
*
* A JMESPath expression used to locate the list of label headers in the model container output.
*
*
* Example: If the model container output of a batch request is
* '{"labels":["cat","dog","fish"],"probability":[0.6,0.3,0.1]}'
, then set LabelAttribute
* to 'labels'
to extract the list of label headers ["cat","dog","fish"]
*
*
* @return A JMESPath expression used to locate the list of label headers in the model container output.
*
* Example: If the model container output of a batch request is
* '{"labels":["cat","dog","fish"],"probability":[0.6,0.3,0.1]}'
, then set
* LabelAttribute
to 'labels'
to extract the list of label headers
* ["cat","dog","fish"]
*/
public String getLabelAttribute() {
return this.labelAttribute;
}
/**
*
* A JMESPath expression used to locate the list of label headers in the model container output.
*
*
* Example: If the model container output of a batch request is
* '{"labels":["cat","dog","fish"],"probability":[0.6,0.3,0.1]}'
, then set LabelAttribute
* to 'labels'
to extract the list of label headers ["cat","dog","fish"]
*
*
* @param labelAttribute
* A JMESPath expression used to locate the list of label headers in the model container output.
*
* Example: If the model container output of a batch request is
* '{"labels":["cat","dog","fish"],"probability":[0.6,0.3,0.1]}'
, then set
* LabelAttribute
to 'labels'
to extract the list of label headers
* ["cat","dog","fish"]
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withLabelAttribute(String labelAttribute) {
setLabelAttribute(labelAttribute);
return this;
}
/**
*
* For multiclass classification problems, the label headers are the names of the classes. Otherwise, the label
* header is the name of the predicted label. These are used to help readability for the output of the
* InvokeEndpoint
API. See the response section under Invoke the endpoint in the Developer Guide for more information. If there are
* no label headers in the model container output, provide them manually using this parameter.
*
*
* @return For multiclass classification problems, the label headers are the names of the classes. Otherwise, the
* label header is the name of the predicted label. These are used to help readability for the output of the
* InvokeEndpoint
API. See the response section under Invoke the endpoint in the Developer Guide for more information. If
* there are no label headers in the model container output, provide them manually using this parameter.
*/
public java.util.List getLabelHeaders() {
return labelHeaders;
}
/**
*
* For multiclass classification problems, the label headers are the names of the classes. Otherwise, the label
* header is the name of the predicted label. These are used to help readability for the output of the
* InvokeEndpoint
API. See the response section under Invoke the endpoint in the Developer Guide for more information. If there are
* no label headers in the model container output, provide them manually using this parameter.
*
*
* @param labelHeaders
* For multiclass classification problems, the label headers are the names of the classes. Otherwise, the
* label header is the name of the predicted label. These are used to help readability for the output of the
* InvokeEndpoint
API. See the response section under Invoke the endpoint in the Developer Guide for more information. If
* there are no label headers in the model container output, provide them manually using this parameter.
*/
public void setLabelHeaders(java.util.Collection labelHeaders) {
if (labelHeaders == null) {
this.labelHeaders = null;
return;
}
this.labelHeaders = new java.util.ArrayList(labelHeaders);
}
/**
*
* For multiclass classification problems, the label headers are the names of the classes. Otherwise, the label
* header is the name of the predicted label. These are used to help readability for the output of the
* InvokeEndpoint
API. See the response section under Invoke the endpoint in the Developer Guide for more information. If there are
* no label headers in the model container output, provide them manually using this parameter.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLabelHeaders(java.util.Collection)} or {@link #withLabelHeaders(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param labelHeaders
* For multiclass classification problems, the label headers are the names of the classes. Otherwise, the
* label header is the name of the predicted label. These are used to help readability for the output of the
* InvokeEndpoint
API. See the response section under Invoke the endpoint in the Developer Guide for more information. If
* there are no label headers in the model container output, provide them manually using this parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withLabelHeaders(String... labelHeaders) {
if (this.labelHeaders == null) {
setLabelHeaders(new java.util.ArrayList(labelHeaders.length));
}
for (String ele : labelHeaders) {
this.labelHeaders.add(ele);
}
return this;
}
/**
*
* For multiclass classification problems, the label headers are the names of the classes. Otherwise, the label
* header is the name of the predicted label. These are used to help readability for the output of the
* InvokeEndpoint
API. See the response section under Invoke the endpoint in the Developer Guide for more information. If there are
* no label headers in the model container output, provide them manually using this parameter.
*
*
* @param labelHeaders
* For multiclass classification problems, the label headers are the names of the classes. Otherwise, the
* label header is the name of the predicted label. These are used to help readability for the output of the
* InvokeEndpoint
API. See the response section under Invoke the endpoint in the Developer Guide for more information. If
* there are no label headers in the model container output, provide them manually using this parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withLabelHeaders(java.util.Collection labelHeaders) {
setLabelHeaders(labelHeaders);
return this;
}
/**
*
* The names of the features. If provided, these are included in the endpoint response payload to help readability
* of the InvokeEndpoint
output. See the Response section under Invoke the endpoint in the Developer Guide for more information.
*
*
* @return The names of the features. If provided, these are included in the endpoint response payload to help
* readability of the InvokeEndpoint
output. See the Response section under Invoke the endpoint in the Developer Guide for more information.
*/
public java.util.List getFeatureHeaders() {
return featureHeaders;
}
/**
*
* The names of the features. If provided, these are included in the endpoint response payload to help readability
* of the InvokeEndpoint
output. See the Response section under Invoke the endpoint in the Developer Guide for more information.
*
*
* @param featureHeaders
* The names of the features. If provided, these are included in the endpoint response payload to help
* readability of the InvokeEndpoint
output. See the Response section under Invoke the endpoint in the Developer Guide for more information.
*/
public void setFeatureHeaders(java.util.Collection featureHeaders) {
if (featureHeaders == null) {
this.featureHeaders = null;
return;
}
this.featureHeaders = new java.util.ArrayList(featureHeaders);
}
/**
*
* The names of the features. If provided, these are included in the endpoint response payload to help readability
* of the InvokeEndpoint
output. See the Response section under Invoke the endpoint in the Developer Guide for more information.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFeatureHeaders(java.util.Collection)} or {@link #withFeatureHeaders(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param featureHeaders
* The names of the features. If provided, these are included in the endpoint response payload to help
* readability of the InvokeEndpoint
output. See the Response section under Invoke the endpoint in the Developer Guide for more information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withFeatureHeaders(String... featureHeaders) {
if (this.featureHeaders == null) {
setFeatureHeaders(new java.util.ArrayList(featureHeaders.length));
}
for (String ele : featureHeaders) {
this.featureHeaders.add(ele);
}
return this;
}
/**
*
* The names of the features. If provided, these are included in the endpoint response payload to help readability
* of the InvokeEndpoint
output. See the Response section under Invoke the endpoint in the Developer Guide for more information.
*
*
* @param featureHeaders
* The names of the features. If provided, these are included in the endpoint response payload to help
* readability of the InvokeEndpoint
output. See the Response section under Invoke the endpoint in the Developer Guide for more information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClarifyInferenceConfig withFeatureHeaders(java.util.Collection featureHeaders) {
setFeatureHeaders(featureHeaders);
return this;
}
/**
*
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example, ['text']
).
* If FeatureTypes
is not provided, the explainer infers the feature types based on the baseline data.
* The feature types are included in the endpoint response payload. For additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
*
*
* @return A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example,
* ['text']
). If FeatureTypes
is not provided, the explainer infers the feature
* types based on the baseline data. The feature types are included in the endpoint response payload. For
* additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
* @see ClarifyFeatureType
*/
public java.util.List getFeatureTypes() {
return featureTypes;
}
/**
*
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example, ['text']
).
* If FeatureTypes
is not provided, the explainer infers the feature types based on the baseline data.
* The feature types are included in the endpoint response payload. For additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
*
*
* @param featureTypes
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example,
* ['text']
). If FeatureTypes
is not provided, the explainer infers the feature
* types based on the baseline data. The feature types are included in the endpoint response payload. For
* additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
* @see ClarifyFeatureType
*/
public void setFeatureTypes(java.util.Collection featureTypes) {
if (featureTypes == null) {
this.featureTypes = null;
return;
}
this.featureTypes = new java.util.ArrayList(featureTypes);
}
/**
*
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example, ['text']
).
* If FeatureTypes
is not provided, the explainer infers the feature types based on the baseline data.
* The feature types are included in the endpoint response payload. For additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFeatureTypes(java.util.Collection)} or {@link #withFeatureTypes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param featureTypes
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example,
* ['text']
). If FeatureTypes
is not provided, the explainer infers the feature
* types based on the baseline data. The feature types are included in the endpoint response payload. For
* additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ClarifyFeatureType
*/
public ClarifyInferenceConfig withFeatureTypes(String... featureTypes) {
if (this.featureTypes == null) {
setFeatureTypes(new java.util.ArrayList(featureTypes.length));
}
for (String ele : featureTypes) {
this.featureTypes.add(ele);
}
return this;
}
/**
*
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example, ['text']
).
* If FeatureTypes
is not provided, the explainer infers the feature types based on the baseline data.
* The feature types are included in the endpoint response payload. For additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
*
*
* @param featureTypes
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example,
* ['text']
). If FeatureTypes
is not provided, the explainer infers the feature
* types based on the baseline data. The feature types are included in the endpoint response payload. For
* additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ClarifyFeatureType
*/
public ClarifyInferenceConfig withFeatureTypes(java.util.Collection featureTypes) {
setFeatureTypes(featureTypes);
return this;
}
/**
*
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example, ['text']
).
* If FeatureTypes
is not provided, the explainer infers the feature types based on the baseline data.
* The feature types are included in the endpoint response payload. For additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
*
*
* @param featureTypes
* A list of data types of the features (optional). Applicable only to NLP explainability. If provided,
* FeatureTypes
must have at least one 'text'
string (for example,
* ['text']
). If FeatureTypes
is not provided, the explainer infers the feature
* types based on the baseline data. The feature types are included in the endpoint response payload. For
* additional information see the response section under Invoke the endpoint in the Developer Guide for more information.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ClarifyFeatureType
*/
public ClarifyInferenceConfig withFeatureTypes(ClarifyFeatureType... featureTypes) {
java.util.ArrayList featureTypesCopy = new java.util.ArrayList(featureTypes.length);
for (ClarifyFeatureType value : featureTypes) {
featureTypesCopy.add(value.toString());
}
if (getFeatureTypes() == null) {
setFeatureTypes(featureTypesCopy);
} else {
getFeatureTypes().addAll(featureTypesCopy);
}
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFeaturesAttribute() != null)
sb.append("FeaturesAttribute: ").append(getFeaturesAttribute()).append(",");
if (getContentTemplate() != null)
sb.append("ContentTemplate: ").append(getContentTemplate()).append(",");
if (getMaxRecordCount() != null)
sb.append("MaxRecordCount: ").append(getMaxRecordCount()).append(",");
if (getMaxPayloadInMB() != null)
sb.append("MaxPayloadInMB: ").append(getMaxPayloadInMB()).append(",");
if (getProbabilityIndex() != null)
sb.append("ProbabilityIndex: ").append(getProbabilityIndex()).append(",");
if (getLabelIndex() != null)
sb.append("LabelIndex: ").append(getLabelIndex()).append(",");
if (getProbabilityAttribute() != null)
sb.append("ProbabilityAttribute: ").append(getProbabilityAttribute()).append(",");
if (getLabelAttribute() != null)
sb.append("LabelAttribute: ").append(getLabelAttribute()).append(",");
if (getLabelHeaders() != null)
sb.append("LabelHeaders: ").append(getLabelHeaders()).append(",");
if (getFeatureHeaders() != null)
sb.append("FeatureHeaders: ").append(getFeatureHeaders()).append(",");
if (getFeatureTypes() != null)
sb.append("FeatureTypes: ").append(getFeatureTypes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ClarifyInferenceConfig == false)
return false;
ClarifyInferenceConfig other = (ClarifyInferenceConfig) obj;
if (other.getFeaturesAttribute() == null ^ this.getFeaturesAttribute() == null)
return false;
if (other.getFeaturesAttribute() != null && other.getFeaturesAttribute().equals(this.getFeaturesAttribute()) == false)
return false;
if (other.getContentTemplate() == null ^ this.getContentTemplate() == null)
return false;
if (other.getContentTemplate() != null && other.getContentTemplate().equals(this.getContentTemplate()) == false)
return false;
if (other.getMaxRecordCount() == null ^ this.getMaxRecordCount() == null)
return false;
if (other.getMaxRecordCount() != null && other.getMaxRecordCount().equals(this.getMaxRecordCount()) == false)
return false;
if (other.getMaxPayloadInMB() == null ^ this.getMaxPayloadInMB() == null)
return false;
if (other.getMaxPayloadInMB() != null && other.getMaxPayloadInMB().equals(this.getMaxPayloadInMB()) == false)
return false;
if (other.getProbabilityIndex() == null ^ this.getProbabilityIndex() == null)
return false;
if (other.getProbabilityIndex() != null && other.getProbabilityIndex().equals(this.getProbabilityIndex()) == false)
return false;
if (other.getLabelIndex() == null ^ this.getLabelIndex() == null)
return false;
if (other.getLabelIndex() != null && other.getLabelIndex().equals(this.getLabelIndex()) == false)
return false;
if (other.getProbabilityAttribute() == null ^ this.getProbabilityAttribute() == null)
return false;
if (other.getProbabilityAttribute() != null && other.getProbabilityAttribute().equals(this.getProbabilityAttribute()) == false)
return false;
if (other.getLabelAttribute() == null ^ this.getLabelAttribute() == null)
return false;
if (other.getLabelAttribute() != null && other.getLabelAttribute().equals(this.getLabelAttribute()) == false)
return false;
if (other.getLabelHeaders() == null ^ this.getLabelHeaders() == null)
return false;
if (other.getLabelHeaders() != null && other.getLabelHeaders().equals(this.getLabelHeaders()) == false)
return false;
if (other.getFeatureHeaders() == null ^ this.getFeatureHeaders() == null)
return false;
if (other.getFeatureHeaders() != null && other.getFeatureHeaders().equals(this.getFeatureHeaders()) == false)
return false;
if (other.getFeatureTypes() == null ^ this.getFeatureTypes() == null)
return false;
if (other.getFeatureTypes() != null && other.getFeatureTypes().equals(this.getFeatureTypes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFeaturesAttribute() == null) ? 0 : getFeaturesAttribute().hashCode());
hashCode = prime * hashCode + ((getContentTemplate() == null) ? 0 : getContentTemplate().hashCode());
hashCode = prime * hashCode + ((getMaxRecordCount() == null) ? 0 : getMaxRecordCount().hashCode());
hashCode = prime * hashCode + ((getMaxPayloadInMB() == null) ? 0 : getMaxPayloadInMB().hashCode());
hashCode = prime * hashCode + ((getProbabilityIndex() == null) ? 0 : getProbabilityIndex().hashCode());
hashCode = prime * hashCode + ((getLabelIndex() == null) ? 0 : getLabelIndex().hashCode());
hashCode = prime * hashCode + ((getProbabilityAttribute() == null) ? 0 : getProbabilityAttribute().hashCode());
hashCode = prime * hashCode + ((getLabelAttribute() == null) ? 0 : getLabelAttribute().hashCode());
hashCode = prime * hashCode + ((getLabelHeaders() == null) ? 0 : getLabelHeaders().hashCode());
hashCode = prime * hashCode + ((getFeatureHeaders() == null) ? 0 : getFeatureHeaders().hashCode());
hashCode = prime * hashCode + ((getFeatureTypes() == null) ? 0 : getFeatureTypes().hashCode());
return hashCode;
}
@Override
public ClarifyInferenceConfig clone() {
try {
return (ClarifyInferenceConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.ClarifyInferenceConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}