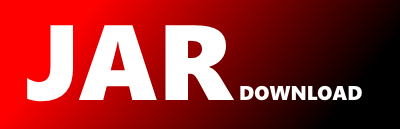
com.amazonaws.services.sagemaker.model.CreateAutoMLJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAutoMLJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Identifies an Autopilot job. The name must be unique to your account and is case insensitive.
*
*/
private String autoMLJobName;
/**
*
* An array of channel objects that describes the input data and its location. Each channel is a named input source.
* Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is required
* for the training dataset. There is not a minimum number of rows required for the validation dataset.
*
*/
private java.util.List inputDataConfig;
/**
*
* Provides information about encryption and the Amazon S3 output path needed to store artifacts from an AutoML job.
* Format(s) supported: CSV.
*
*/
private AutoMLOutputDataConfig outputDataConfig;
/**
*
* Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
*
*/
private String problemType;
/**
*
* Specifies a metric to minimize or maximize as the objective of a job. If not specified, the default objective
* metric depends on the problem type. See AutoMLJobObjective for the default values.
*
*/
private AutoMLJobObjective autoMLJobObjective;
/**
*
* A collection of settings used to configure an AutoML job.
*
*/
private AutoMLJobConfig autoMLJobConfig;
/**
*
* The ARN of the role that is used to access the data.
*
*/
private String roleArn;
/**
*
* Generates possible candidates without training the models. A candidate is a combination of data preprocessors,
* algorithms, and algorithm parameter settings.
*
*/
private Boolean generateCandidateDefinitionsOnly;
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web ServicesResources.
* Tag keys must be unique per resource.
*
*/
private java.util.List tags;
/**
*
* Specifies how to generate the endpoint name for an automatic one-click Autopilot model deployment.
*
*/
private ModelDeployConfig modelDeployConfig;
/**
*
* Identifies an Autopilot job. The name must be unique to your account and is case insensitive.
*
*
* @param autoMLJobName
* Identifies an Autopilot job. The name must be unique to your account and is case insensitive.
*/
public void setAutoMLJobName(String autoMLJobName) {
this.autoMLJobName = autoMLJobName;
}
/**
*
* Identifies an Autopilot job. The name must be unique to your account and is case insensitive.
*
*
* @return Identifies an Autopilot job. The name must be unique to your account and is case insensitive.
*/
public String getAutoMLJobName() {
return this.autoMLJobName;
}
/**
*
* Identifies an Autopilot job. The name must be unique to your account and is case insensitive.
*
*
* @param autoMLJobName
* Identifies an Autopilot job. The name must be unique to your account and is case insensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withAutoMLJobName(String autoMLJobName) {
setAutoMLJobName(autoMLJobName);
return this;
}
/**
*
* An array of channel objects that describes the input data and its location. Each channel is a named input source.
* Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is required
* for the training dataset. There is not a minimum number of rows required for the validation dataset.
*
*
* @return An array of channel objects that describes the input data and its location. Each channel is a named input
* source. Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is
* required for the training dataset. There is not a minimum number of rows required for the validation
* dataset.
*/
public java.util.List getInputDataConfig() {
return inputDataConfig;
}
/**
*
* An array of channel objects that describes the input data and its location. Each channel is a named input source.
* Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is required
* for the training dataset. There is not a minimum number of rows required for the validation dataset.
*
*
* @param inputDataConfig
* An array of channel objects that describes the input data and its location. Each channel is a named input
* source. Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is
* required for the training dataset. There is not a minimum number of rows required for the validation
* dataset.
*/
public void setInputDataConfig(java.util.Collection inputDataConfig) {
if (inputDataConfig == null) {
this.inputDataConfig = null;
return;
}
this.inputDataConfig = new java.util.ArrayList(inputDataConfig);
}
/**
*
* An array of channel objects that describes the input data and its location. Each channel is a named input source.
* Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is required
* for the training dataset. There is not a minimum number of rows required for the validation dataset.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInputDataConfig(java.util.Collection)} or {@link #withInputDataConfig(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param inputDataConfig
* An array of channel objects that describes the input data and its location. Each channel is a named input
* source. Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is
* required for the training dataset. There is not a minimum number of rows required for the validation
* dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withInputDataConfig(AutoMLChannel... inputDataConfig) {
if (this.inputDataConfig == null) {
setInputDataConfig(new java.util.ArrayList(inputDataConfig.length));
}
for (AutoMLChannel ele : inputDataConfig) {
this.inputDataConfig.add(ele);
}
return this;
}
/**
*
* An array of channel objects that describes the input data and its location. Each channel is a named input source.
* Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is required
* for the training dataset. There is not a minimum number of rows required for the validation dataset.
*
*
* @param inputDataConfig
* An array of channel objects that describes the input data and its location. Each channel is a named input
* source. Similar to InputDataConfig
supported by HyperParameterTrainingJobDefinition. Format(s) supported: CSV, Parquet. A minimum of 500 rows is
* required for the training dataset. There is not a minimum number of rows required for the validation
* dataset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withInputDataConfig(java.util.Collection inputDataConfig) {
setInputDataConfig(inputDataConfig);
return this;
}
/**
*
* Provides information about encryption and the Amazon S3 output path needed to store artifacts from an AutoML job.
* Format(s) supported: CSV.
*
*
* @param outputDataConfig
* Provides information about encryption and the Amazon S3 output path needed to store artifacts from an
* AutoML job. Format(s) supported: CSV.
*/
public void setOutputDataConfig(AutoMLOutputDataConfig outputDataConfig) {
this.outputDataConfig = outputDataConfig;
}
/**
*
* Provides information about encryption and the Amazon S3 output path needed to store artifacts from an AutoML job.
* Format(s) supported: CSV.
*
*
* @return Provides information about encryption and the Amazon S3 output path needed to store artifacts from an
* AutoML job. Format(s) supported: CSV.
*/
public AutoMLOutputDataConfig getOutputDataConfig() {
return this.outputDataConfig;
}
/**
*
* Provides information about encryption and the Amazon S3 output path needed to store artifacts from an AutoML job.
* Format(s) supported: CSV.
*
*
* @param outputDataConfig
* Provides information about encryption and the Amazon S3 output path needed to store artifacts from an
* AutoML job. Format(s) supported: CSV.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withOutputDataConfig(AutoMLOutputDataConfig outputDataConfig) {
setOutputDataConfig(outputDataConfig);
return this;
}
/**
*
* Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
*
*
* @param problemType
* Defines the type of supervised learning problem available for the candidates. For more information, see SageMaker Autopilot problem types.
* @see ProblemType
*/
public void setProblemType(String problemType) {
this.problemType = problemType;
}
/**
*
* Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
*
*
* @return Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
* @see ProblemType
*/
public String getProblemType() {
return this.problemType;
}
/**
*
* Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
*
*
* @param problemType
* Defines the type of supervised learning problem available for the candidates. For more information, see SageMaker Autopilot problem types.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProblemType
*/
public CreateAutoMLJobRequest withProblemType(String problemType) {
setProblemType(problemType);
return this;
}
/**
*
* Defines the type of supervised learning problem available for the candidates. For more information, see
* SageMaker Autopilot problem types.
*
*
* @param problemType
* Defines the type of supervised learning problem available for the candidates. For more information, see SageMaker Autopilot problem types.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProblemType
*/
public CreateAutoMLJobRequest withProblemType(ProblemType problemType) {
this.problemType = problemType.toString();
return this;
}
/**
*
* Specifies a metric to minimize or maximize as the objective of a job. If not specified, the default objective
* metric depends on the problem type. See AutoMLJobObjective for the default values.
*
*
* @param autoMLJobObjective
* Specifies a metric to minimize or maximize as the objective of a job. If not specified, the default
* objective metric depends on the problem type. See AutoMLJobObjective for the default values.
*/
public void setAutoMLJobObjective(AutoMLJobObjective autoMLJobObjective) {
this.autoMLJobObjective = autoMLJobObjective;
}
/**
*
* Specifies a metric to minimize or maximize as the objective of a job. If not specified, the default objective
* metric depends on the problem type. See AutoMLJobObjective for the default values.
*
*
* @return Specifies a metric to minimize or maximize as the objective of a job. If not specified, the default
* objective metric depends on the problem type. See AutoMLJobObjective for the default values.
*/
public AutoMLJobObjective getAutoMLJobObjective() {
return this.autoMLJobObjective;
}
/**
*
* Specifies a metric to minimize or maximize as the objective of a job. If not specified, the default objective
* metric depends on the problem type. See AutoMLJobObjective for the default values.
*
*
* @param autoMLJobObjective
* Specifies a metric to minimize or maximize as the objective of a job. If not specified, the default
* objective metric depends on the problem type. See AutoMLJobObjective for the default values.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withAutoMLJobObjective(AutoMLJobObjective autoMLJobObjective) {
setAutoMLJobObjective(autoMLJobObjective);
return this;
}
/**
*
* A collection of settings used to configure an AutoML job.
*
*
* @param autoMLJobConfig
* A collection of settings used to configure an AutoML job.
*/
public void setAutoMLJobConfig(AutoMLJobConfig autoMLJobConfig) {
this.autoMLJobConfig = autoMLJobConfig;
}
/**
*
* A collection of settings used to configure an AutoML job.
*
*
* @return A collection of settings used to configure an AutoML job.
*/
public AutoMLJobConfig getAutoMLJobConfig() {
return this.autoMLJobConfig;
}
/**
*
* A collection of settings used to configure an AutoML job.
*
*
* @param autoMLJobConfig
* A collection of settings used to configure an AutoML job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withAutoMLJobConfig(AutoMLJobConfig autoMLJobConfig) {
setAutoMLJobConfig(autoMLJobConfig);
return this;
}
/**
*
* The ARN of the role that is used to access the data.
*
*
* @param roleArn
* The ARN of the role that is used to access the data.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The ARN of the role that is used to access the data.
*
*
* @return The ARN of the role that is used to access the data.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The ARN of the role that is used to access the data.
*
*
* @param roleArn
* The ARN of the role that is used to access the data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* Generates possible candidates without training the models. A candidate is a combination of data preprocessors,
* algorithms, and algorithm parameter settings.
*
*
* @param generateCandidateDefinitionsOnly
* Generates possible candidates without training the models. A candidate is a combination of data
* preprocessors, algorithms, and algorithm parameter settings.
*/
public void setGenerateCandidateDefinitionsOnly(Boolean generateCandidateDefinitionsOnly) {
this.generateCandidateDefinitionsOnly = generateCandidateDefinitionsOnly;
}
/**
*
* Generates possible candidates without training the models. A candidate is a combination of data preprocessors,
* algorithms, and algorithm parameter settings.
*
*
* @return Generates possible candidates without training the models. A candidate is a combination of data
* preprocessors, algorithms, and algorithm parameter settings.
*/
public Boolean getGenerateCandidateDefinitionsOnly() {
return this.generateCandidateDefinitionsOnly;
}
/**
*
* Generates possible candidates without training the models. A candidate is a combination of data preprocessors,
* algorithms, and algorithm parameter settings.
*
*
* @param generateCandidateDefinitionsOnly
* Generates possible candidates without training the models. A candidate is a combination of data
* preprocessors, algorithms, and algorithm parameter settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withGenerateCandidateDefinitionsOnly(Boolean generateCandidateDefinitionsOnly) {
setGenerateCandidateDefinitionsOnly(generateCandidateDefinitionsOnly);
return this;
}
/**
*
* Generates possible candidates without training the models. A candidate is a combination of data preprocessors,
* algorithms, and algorithm parameter settings.
*
*
* @return Generates possible candidates without training the models. A candidate is a combination of data
* preprocessors, algorithms, and algorithm parameter settings.
*/
public Boolean isGenerateCandidateDefinitionsOnly() {
return this.generateCandidateDefinitionsOnly;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web ServicesResources.
* Tag keys must be unique per resource.
*
*
* @return An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web
* ServicesResources. Tag keys must be unique per resource.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web ServicesResources.
* Tag keys must be unique per resource.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web
* ServicesResources. Tag keys must be unique per resource.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web ServicesResources.
* Tag keys must be unique per resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web
* ServicesResources. Tag keys must be unique per resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web ServicesResources.
* Tag keys must be unique per resource.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web
* ServicesResources. Tag keys must be unique per resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Specifies how to generate the endpoint name for an automatic one-click Autopilot model deployment.
*
*
* @param modelDeployConfig
* Specifies how to generate the endpoint name for an automatic one-click Autopilot model deployment.
*/
public void setModelDeployConfig(ModelDeployConfig modelDeployConfig) {
this.modelDeployConfig = modelDeployConfig;
}
/**
*
* Specifies how to generate the endpoint name for an automatic one-click Autopilot model deployment.
*
*
* @return Specifies how to generate the endpoint name for an automatic one-click Autopilot model deployment.
*/
public ModelDeployConfig getModelDeployConfig() {
return this.modelDeployConfig;
}
/**
*
* Specifies how to generate the endpoint name for an automatic one-click Autopilot model deployment.
*
*
* @param modelDeployConfig
* Specifies how to generate the endpoint name for an automatic one-click Autopilot model deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAutoMLJobRequest withModelDeployConfig(ModelDeployConfig modelDeployConfig) {
setModelDeployConfig(modelDeployConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAutoMLJobName() != null)
sb.append("AutoMLJobName: ").append(getAutoMLJobName()).append(",");
if (getInputDataConfig() != null)
sb.append("InputDataConfig: ").append(getInputDataConfig()).append(",");
if (getOutputDataConfig() != null)
sb.append("OutputDataConfig: ").append(getOutputDataConfig()).append(",");
if (getProblemType() != null)
sb.append("ProblemType: ").append(getProblemType()).append(",");
if (getAutoMLJobObjective() != null)
sb.append("AutoMLJobObjective: ").append(getAutoMLJobObjective()).append(",");
if (getAutoMLJobConfig() != null)
sb.append("AutoMLJobConfig: ").append(getAutoMLJobConfig()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getGenerateCandidateDefinitionsOnly() != null)
sb.append("GenerateCandidateDefinitionsOnly: ").append(getGenerateCandidateDefinitionsOnly()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getModelDeployConfig() != null)
sb.append("ModelDeployConfig: ").append(getModelDeployConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAutoMLJobRequest == false)
return false;
CreateAutoMLJobRequest other = (CreateAutoMLJobRequest) obj;
if (other.getAutoMLJobName() == null ^ this.getAutoMLJobName() == null)
return false;
if (other.getAutoMLJobName() != null && other.getAutoMLJobName().equals(this.getAutoMLJobName()) == false)
return false;
if (other.getInputDataConfig() == null ^ this.getInputDataConfig() == null)
return false;
if (other.getInputDataConfig() != null && other.getInputDataConfig().equals(this.getInputDataConfig()) == false)
return false;
if (other.getOutputDataConfig() == null ^ this.getOutputDataConfig() == null)
return false;
if (other.getOutputDataConfig() != null && other.getOutputDataConfig().equals(this.getOutputDataConfig()) == false)
return false;
if (other.getProblemType() == null ^ this.getProblemType() == null)
return false;
if (other.getProblemType() != null && other.getProblemType().equals(this.getProblemType()) == false)
return false;
if (other.getAutoMLJobObjective() == null ^ this.getAutoMLJobObjective() == null)
return false;
if (other.getAutoMLJobObjective() != null && other.getAutoMLJobObjective().equals(this.getAutoMLJobObjective()) == false)
return false;
if (other.getAutoMLJobConfig() == null ^ this.getAutoMLJobConfig() == null)
return false;
if (other.getAutoMLJobConfig() != null && other.getAutoMLJobConfig().equals(this.getAutoMLJobConfig()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getGenerateCandidateDefinitionsOnly() == null ^ this.getGenerateCandidateDefinitionsOnly() == null)
return false;
if (other.getGenerateCandidateDefinitionsOnly() != null
&& other.getGenerateCandidateDefinitionsOnly().equals(this.getGenerateCandidateDefinitionsOnly()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getModelDeployConfig() == null ^ this.getModelDeployConfig() == null)
return false;
if (other.getModelDeployConfig() != null && other.getModelDeployConfig().equals(this.getModelDeployConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAutoMLJobName() == null) ? 0 : getAutoMLJobName().hashCode());
hashCode = prime * hashCode + ((getInputDataConfig() == null) ? 0 : getInputDataConfig().hashCode());
hashCode = prime * hashCode + ((getOutputDataConfig() == null) ? 0 : getOutputDataConfig().hashCode());
hashCode = prime * hashCode + ((getProblemType() == null) ? 0 : getProblemType().hashCode());
hashCode = prime * hashCode + ((getAutoMLJobObjective() == null) ? 0 : getAutoMLJobObjective().hashCode());
hashCode = prime * hashCode + ((getAutoMLJobConfig() == null) ? 0 : getAutoMLJobConfig().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getGenerateCandidateDefinitionsOnly() == null) ? 0 : getGenerateCandidateDefinitionsOnly().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getModelDeployConfig() == null) ? 0 : getModelDeployConfig().hashCode());
return hashCode;
}
@Override
public CreateAutoMLJobRequest clone() {
return (CreateAutoMLJobRequest) super.clone();
}
}