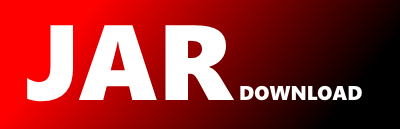
com.amazonaws.services.sagemaker.model.CreateCompilationJobRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateCompilationJobRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A name for the model compilation job. The name must be unique within the Amazon Web Services Region and within
* your Amazon Web Services account.
*
*/
private String compilationJobName;
/**
*
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your behalf.
*
*
* During model compilation, Amazon SageMaker needs your permission to:
*
*
* -
*
* Read input data from an S3 bucket
*
*
* -
*
* Write model artifacts to an S3 bucket
*
*
* -
*
* Write logs to Amazon CloudWatch Logs
*
*
* -
*
* Publish metrics to Amazon CloudWatch
*
*
*
*
* You grant permissions for all of these tasks to an IAM role. To pass this role to Amazon SageMaker, the caller of
* this API must have the iam:PassRole
permission. For more information, see Amazon SageMaker Roles.
*
*/
private String roleArn;
/**
*
* The Amazon Resource Name (ARN) of a versioned model package. Provide either a ModelPackageVersionArn
* or an InputConfig
object in the request syntax. The presence of both objects in the
* CreateCompilationJob
request will return an exception.
*
*/
private String modelPackageVersionArn;
/**
*
* Provides information about the location of input model artifacts, the name and shape of the expected data inputs,
* and the framework in which the model was trained.
*
*/
private InputConfig inputConfig;
/**
*
* Provides information about the output location for the compiled model and the target device the model runs on.
*
*/
private OutputConfig outputConfig;
/**
*
* A VpcConfig object
* that specifies the VPC that you want your compilation job to connect to. Control access to your models by
* configuring the VPC. For more information, see Protect Compilation Jobs by Using an Amazon
* Virtual Private Cloud.
*
*/
private NeoVpcConfig vpcConfig;
/**
*
* Specifies a limit to how long a model compilation job can run. When the job reaches the time limit, Amazon
* SageMaker ends the compilation job. Use this API to cap model training costs.
*
*/
private StoppingCondition stoppingCondition;
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*/
private java.util.List tags;
/**
*
* A name for the model compilation job. The name must be unique within the Amazon Web Services Region and within
* your Amazon Web Services account.
*
*
* @param compilationJobName
* A name for the model compilation job. The name must be unique within the Amazon Web Services Region and
* within your Amazon Web Services account.
*/
public void setCompilationJobName(String compilationJobName) {
this.compilationJobName = compilationJobName;
}
/**
*
* A name for the model compilation job. The name must be unique within the Amazon Web Services Region and within
* your Amazon Web Services account.
*
*
* @return A name for the model compilation job. The name must be unique within the Amazon Web Services Region and
* within your Amazon Web Services account.
*/
public String getCompilationJobName() {
return this.compilationJobName;
}
/**
*
* A name for the model compilation job. The name must be unique within the Amazon Web Services Region and within
* your Amazon Web Services account.
*
*
* @param compilationJobName
* A name for the model compilation job. The name must be unique within the Amazon Web Services Region and
* within your Amazon Web Services account.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCompilationJobRequest withCompilationJobName(String compilationJobName) {
setCompilationJobName(compilationJobName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your behalf.
*
*
* During model compilation, Amazon SageMaker needs your permission to:
*
*
* -
*
* Read input data from an S3 bucket
*
*
* -
*
* Write model artifacts to an S3 bucket
*
*
* -
*
* Write logs to Amazon CloudWatch Logs
*
*
* -
*
* Publish metrics to Amazon CloudWatch
*
*
*
*
* You grant permissions for all of these tasks to an IAM role. To pass this role to Amazon SageMaker, the caller of
* this API must have the iam:PassRole
permission. For more information, see Amazon SageMaker Roles.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your
* behalf.
*
* During model compilation, Amazon SageMaker needs your permission to:
*
*
* -
*
* Read input data from an S3 bucket
*
*
* -
*
* Write model artifacts to an S3 bucket
*
*
* -
*
* Write logs to Amazon CloudWatch Logs
*
*
* -
*
* Publish metrics to Amazon CloudWatch
*
*
*
*
* You grant permissions for all of these tasks to an IAM role. To pass this role to Amazon SageMaker, the
* caller of this API must have the iam:PassRole
permission. For more information, see Amazon SageMaker Roles.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your behalf.
*
*
* During model compilation, Amazon SageMaker needs your permission to:
*
*
* -
*
* Read input data from an S3 bucket
*
*
* -
*
* Write model artifacts to an S3 bucket
*
*
* -
*
* Write logs to Amazon CloudWatch Logs
*
*
* -
*
* Publish metrics to Amazon CloudWatch
*
*
*
*
* You grant permissions for all of these tasks to an IAM role. To pass this role to Amazon SageMaker, the caller of
* this API must have the iam:PassRole
permission. For more information, see Amazon SageMaker Roles.
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your
* behalf.
*
* During model compilation, Amazon SageMaker needs your permission to:
*
*
* -
*
* Read input data from an S3 bucket
*
*
* -
*
* Write model artifacts to an S3 bucket
*
*
* -
*
* Write logs to Amazon CloudWatch Logs
*
*
* -
*
* Publish metrics to Amazon CloudWatch
*
*
*
*
* You grant permissions for all of these tasks to an IAM role. To pass this role to Amazon SageMaker, the
* caller of this API must have the iam:PassRole
permission. For more information, see Amazon SageMaker Roles.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your behalf.
*
*
* During model compilation, Amazon SageMaker needs your permission to:
*
*
* -
*
* Read input data from an S3 bucket
*
*
* -
*
* Write model artifacts to an S3 bucket
*
*
* -
*
* Write logs to Amazon CloudWatch Logs
*
*
* -
*
* Publish metrics to Amazon CloudWatch
*
*
*
*
* You grant permissions for all of these tasks to an IAM role. To pass this role to Amazon SageMaker, the caller of
* this API must have the iam:PassRole
permission. For more information, see Amazon SageMaker Roles.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of an IAM role that enables Amazon SageMaker to perform tasks on your
* behalf.
*
* During model compilation, Amazon SageMaker needs your permission to:
*
*
* -
*
* Read input data from an S3 bucket
*
*
* -
*
* Write model artifacts to an S3 bucket
*
*
* -
*
* Write logs to Amazon CloudWatch Logs
*
*
* -
*
* Publish metrics to Amazon CloudWatch
*
*
*
*
* You grant permissions for all of these tasks to an IAM role. To pass this role to Amazon SageMaker, the
* caller of this API must have the iam:PassRole
permission. For more information, see Amazon SageMaker Roles.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCompilationJobRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of a versioned model package. Provide either a ModelPackageVersionArn
* or an InputConfig
object in the request syntax. The presence of both objects in the
* CreateCompilationJob
request will return an exception.
*
*
* @param modelPackageVersionArn
* The Amazon Resource Name (ARN) of a versioned model package. Provide either a
* ModelPackageVersionArn
or an InputConfig
object in the request syntax. The
* presence of both objects in the CreateCompilationJob
request will return an exception.
*/
public void setModelPackageVersionArn(String modelPackageVersionArn) {
this.modelPackageVersionArn = modelPackageVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of a versioned model package. Provide either a ModelPackageVersionArn
* or an InputConfig
object in the request syntax. The presence of both objects in the
* CreateCompilationJob
request will return an exception.
*
*
* @return The Amazon Resource Name (ARN) of a versioned model package. Provide either a
* ModelPackageVersionArn
or an InputConfig
object in the request syntax. The
* presence of both objects in the CreateCompilationJob
request will return an exception.
*/
public String getModelPackageVersionArn() {
return this.modelPackageVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of a versioned model package. Provide either a ModelPackageVersionArn
* or an InputConfig
object in the request syntax. The presence of both objects in the
* CreateCompilationJob
request will return an exception.
*
*
* @param modelPackageVersionArn
* The Amazon Resource Name (ARN) of a versioned model package. Provide either a
* ModelPackageVersionArn
or an InputConfig
object in the request syntax. The
* presence of both objects in the CreateCompilationJob
request will return an exception.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCompilationJobRequest withModelPackageVersionArn(String modelPackageVersionArn) {
setModelPackageVersionArn(modelPackageVersionArn);
return this;
}
/**
*
* Provides information about the location of input model artifacts, the name and shape of the expected data inputs,
* and the framework in which the model was trained.
*
*
* @param inputConfig
* Provides information about the location of input model artifacts, the name and shape of the expected data
* inputs, and the framework in which the model was trained.
*/
public void setInputConfig(InputConfig inputConfig) {
this.inputConfig = inputConfig;
}
/**
*
* Provides information about the location of input model artifacts, the name and shape of the expected data inputs,
* and the framework in which the model was trained.
*
*
* @return Provides information about the location of input model artifacts, the name and shape of the expected data
* inputs, and the framework in which the model was trained.
*/
public InputConfig getInputConfig() {
return this.inputConfig;
}
/**
*
* Provides information about the location of input model artifacts, the name and shape of the expected data inputs,
* and the framework in which the model was trained.
*
*
* @param inputConfig
* Provides information about the location of input model artifacts, the name and shape of the expected data
* inputs, and the framework in which the model was trained.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCompilationJobRequest withInputConfig(InputConfig inputConfig) {
setInputConfig(inputConfig);
return this;
}
/**
*
* Provides information about the output location for the compiled model and the target device the model runs on.
*
*
* @param outputConfig
* Provides information about the output location for the compiled model and the target device the model runs
* on.
*/
public void setOutputConfig(OutputConfig outputConfig) {
this.outputConfig = outputConfig;
}
/**
*
* Provides information about the output location for the compiled model and the target device the model runs on.
*
*
* @return Provides information about the output location for the compiled model and the target device the model
* runs on.
*/
public OutputConfig getOutputConfig() {
return this.outputConfig;
}
/**
*
* Provides information about the output location for the compiled model and the target device the model runs on.
*
*
* @param outputConfig
* Provides information about the output location for the compiled model and the target device the model runs
* on.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCompilationJobRequest withOutputConfig(OutputConfig outputConfig) {
setOutputConfig(outputConfig);
return this;
}
/**
*
* A VpcConfig object
* that specifies the VPC that you want your compilation job to connect to. Control access to your models by
* configuring the VPC. For more information, see Protect Compilation Jobs by Using an Amazon
* Virtual Private Cloud.
*
*
* @param vpcConfig
* A VpcConfig
* object that specifies the VPC that you want your compilation job to connect to. Control access to your
* models by configuring the VPC. For more information, see Protect Compilation Jobs by Using an
* Amazon Virtual Private Cloud.
*/
public void setVpcConfig(NeoVpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
*
* A VpcConfig object
* that specifies the VPC that you want your compilation job to connect to. Control access to your models by
* configuring the VPC. For more information, see Protect Compilation Jobs by Using an Amazon
* Virtual Private Cloud.
*
*
* @return A VpcConfig
* object that specifies the VPC that you want your compilation job to connect to. Control access to your
* models by configuring the VPC. For more information, see Protect Compilation Jobs by Using an
* Amazon Virtual Private Cloud.
*/
public NeoVpcConfig getVpcConfig() {
return this.vpcConfig;
}
/**
*
* A VpcConfig object
* that specifies the VPC that you want your compilation job to connect to. Control access to your models by
* configuring the VPC. For more information, see Protect Compilation Jobs by Using an Amazon
* Virtual Private Cloud.
*
*
* @param vpcConfig
* A VpcConfig
* object that specifies the VPC that you want your compilation job to connect to. Control access to your
* models by configuring the VPC. For more information, see Protect Compilation Jobs by Using an
* Amazon Virtual Private Cloud.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCompilationJobRequest withVpcConfig(NeoVpcConfig vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
*
* Specifies a limit to how long a model compilation job can run. When the job reaches the time limit, Amazon
* SageMaker ends the compilation job. Use this API to cap model training costs.
*
*
* @param stoppingCondition
* Specifies a limit to how long a model compilation job can run. When the job reaches the time limit, Amazon
* SageMaker ends the compilation job. Use this API to cap model training costs.
*/
public void setStoppingCondition(StoppingCondition stoppingCondition) {
this.stoppingCondition = stoppingCondition;
}
/**
*
* Specifies a limit to how long a model compilation job can run. When the job reaches the time limit, Amazon
* SageMaker ends the compilation job. Use this API to cap model training costs.
*
*
* @return Specifies a limit to how long a model compilation job can run. When the job reaches the time limit,
* Amazon SageMaker ends the compilation job. Use this API to cap model training costs.
*/
public StoppingCondition getStoppingCondition() {
return this.stoppingCondition;
}
/**
*
* Specifies a limit to how long a model compilation job can run. When the job reaches the time limit, Amazon
* SageMaker ends the compilation job. Use this API to cap model training costs.
*
*
* @param stoppingCondition
* Specifies a limit to how long a model compilation job can run. When the job reaches the time limit, Amazon
* SageMaker ends the compilation job. Use this API to cap model training costs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCompilationJobRequest withStoppingCondition(StoppingCondition stoppingCondition) {
setStoppingCondition(stoppingCondition);
return this;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @return An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCompilationJobRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCompilationJobRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCompilationJobName() != null)
sb.append("CompilationJobName: ").append(getCompilationJobName()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getModelPackageVersionArn() != null)
sb.append("ModelPackageVersionArn: ").append(getModelPackageVersionArn()).append(",");
if (getInputConfig() != null)
sb.append("InputConfig: ").append(getInputConfig()).append(",");
if (getOutputConfig() != null)
sb.append("OutputConfig: ").append(getOutputConfig()).append(",");
if (getVpcConfig() != null)
sb.append("VpcConfig: ").append(getVpcConfig()).append(",");
if (getStoppingCondition() != null)
sb.append("StoppingCondition: ").append(getStoppingCondition()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateCompilationJobRequest == false)
return false;
CreateCompilationJobRequest other = (CreateCompilationJobRequest) obj;
if (other.getCompilationJobName() == null ^ this.getCompilationJobName() == null)
return false;
if (other.getCompilationJobName() != null && other.getCompilationJobName().equals(this.getCompilationJobName()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getModelPackageVersionArn() == null ^ this.getModelPackageVersionArn() == null)
return false;
if (other.getModelPackageVersionArn() != null && other.getModelPackageVersionArn().equals(this.getModelPackageVersionArn()) == false)
return false;
if (other.getInputConfig() == null ^ this.getInputConfig() == null)
return false;
if (other.getInputConfig() != null && other.getInputConfig().equals(this.getInputConfig()) == false)
return false;
if (other.getOutputConfig() == null ^ this.getOutputConfig() == null)
return false;
if (other.getOutputConfig() != null && other.getOutputConfig().equals(this.getOutputConfig()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null && other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
if (other.getStoppingCondition() == null ^ this.getStoppingCondition() == null)
return false;
if (other.getStoppingCondition() != null && other.getStoppingCondition().equals(this.getStoppingCondition()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCompilationJobName() == null) ? 0 : getCompilationJobName().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getModelPackageVersionArn() == null) ? 0 : getModelPackageVersionArn().hashCode());
hashCode = prime * hashCode + ((getInputConfig() == null) ? 0 : getInputConfig().hashCode());
hashCode = prime * hashCode + ((getOutputConfig() == null) ? 0 : getOutputConfig().hashCode());
hashCode = prime * hashCode + ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
hashCode = prime * hashCode + ((getStoppingCondition() == null) ? 0 : getStoppingCondition().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateCompilationJobRequest clone() {
return (CreateCompilationJobRequest) super.clone();
}
}