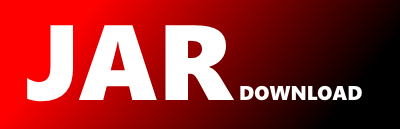
com.amazonaws.services.sagemaker.model.CreateEndpointConfigRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateEndpointConfigRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the endpoint configuration. You specify this name in a CreateEndpoint
* request.
*
*/
private String endpointConfigName;
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint.
*
*/
private java.util.List productionVariants;
private DataCaptureConfig dataCaptureConfig;
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*/
private java.util.List tags;
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to encrypt
* data on the storage volume attached to the ML compute instance that hosts the endpoint.
*
*
* The KmsKeyId can be any of the following formats:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Alias name: alias/ExampleAlias
*
*
* -
*
* Alias name ARN: arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
*
*
*
*
* The KMS key policy must grant permission to the IAM role that you specify in your CreateEndpoint
,
* UpdateEndpoint
requests. For more information, refer to the Amazon Web Services Key Management
* Service section Using Key
* Policies in Amazon Web Services KMS
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage volumes are
* encrypted using a hardware module on the instance. You can't request a KmsKeyId
when using an
* instance type with local storage. If any of the models that you specify in the ProductionVariants
* parameter use nitro-based instances with local storage, do not specify a value for the KmsKeyId
* parameter. If you specify a value for KmsKeyId
when using any nitro-based instances with local
* storage, the call to CreateEndpointConfig
fails.
*
*
* For a list of instance types that support local instance storage, see Instance
* Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*
*/
private String kmsKeyId;
/**
*
* Specifies configuration for how an endpoint performs asynchronous inference. This is a required field in order
* for your Endpoint to be invoked using InvokeEndpointAsync.
*
*/
private AsyncInferenceConfig asyncInferenceConfig;
/**
*
* A member of CreateEndpointConfig
that enables explainers.
*
*/
private ExplainerConfig explainerConfig;
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint in
* shadow mode with production traffic replicated from the model specified on ProductionVariants
. If
* you use this field, you can only specify one variant for ProductionVariants
and one variant for
* ShadowProductionVariants
.
*
*/
private java.util.List shadowProductionVariants;
/**
*
* The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to perform actions on your behalf.
* For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to Amazon SageMaker, the caller of this action must have the
* iam:PassRole
permission.
*
*
*/
private String executionRoleArn;
private VpcConfig vpcConfig;
/**
*
* Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or outbound
* network calls can be made to or from the model containers.
*
*/
private Boolean enableNetworkIsolation;
/**
*
* The name of the endpoint configuration. You specify this name in a CreateEndpoint
* request.
*
*
* @param endpointConfigName
* The name of the endpoint configuration. You specify this name in a CreateEndpoint request.
*/
public void setEndpointConfigName(String endpointConfigName) {
this.endpointConfigName = endpointConfigName;
}
/**
*
* The name of the endpoint configuration. You specify this name in a CreateEndpoint
* request.
*
*
* @return The name of the endpoint configuration. You specify this name in a CreateEndpoint request.
*/
public String getEndpointConfigName() {
return this.endpointConfigName;
}
/**
*
* The name of the endpoint configuration. You specify this name in a CreateEndpoint
* request.
*
*
* @param endpointConfigName
* The name of the endpoint configuration. You specify this name in a CreateEndpoint request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withEndpointConfigName(String endpointConfigName) {
setEndpointConfigName(endpointConfigName);
return this;
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint.
*
*
* @return An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint.
*/
public java.util.List getProductionVariants() {
return productionVariants;
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint.
*
*
* @param productionVariants
* An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint.
*/
public void setProductionVariants(java.util.Collection productionVariants) {
if (productionVariants == null) {
this.productionVariants = null;
return;
}
this.productionVariants = new java.util.ArrayList(productionVariants);
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setProductionVariants(java.util.Collection)} or {@link #withProductionVariants(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param productionVariants
* An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withProductionVariants(ProductionVariant... productionVariants) {
if (this.productionVariants == null) {
setProductionVariants(new java.util.ArrayList(productionVariants.length));
}
for (ProductionVariant ele : productionVariants) {
this.productionVariants.add(ele);
}
return this;
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint.
*
*
* @param productionVariants
* An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withProductionVariants(java.util.Collection productionVariants) {
setProductionVariants(productionVariants);
return this;
}
/**
* @param dataCaptureConfig
*/
public void setDataCaptureConfig(DataCaptureConfig dataCaptureConfig) {
this.dataCaptureConfig = dataCaptureConfig;
}
/**
* @return
*/
public DataCaptureConfig getDataCaptureConfig() {
return this.dataCaptureConfig;
}
/**
* @param dataCaptureConfig
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withDataCaptureConfig(DataCaptureConfig dataCaptureConfig) {
setDataCaptureConfig(dataCaptureConfig);
return this;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @return An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to encrypt
* data on the storage volume attached to the ML compute instance that hosts the endpoint.
*
*
* The KmsKeyId can be any of the following formats:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Alias name: alias/ExampleAlias
*
*
* -
*
* Alias name ARN: arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
*
*
*
*
* The KMS key policy must grant permission to the IAM role that you specify in your CreateEndpoint
,
* UpdateEndpoint
requests. For more information, refer to the Amazon Web Services Key Management
* Service section Using Key
* Policies in Amazon Web Services KMS
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage volumes are
* encrypted using a hardware module on the instance. You can't request a KmsKeyId
when using an
* instance type with local storage. If any of the models that you specify in the ProductionVariants
* parameter use nitro-based instances with local storage, do not specify a value for the KmsKeyId
* parameter. If you specify a value for KmsKeyId
when using any nitro-based instances with local
* storage, the call to CreateEndpointConfig
fails.
*
*
* For a list of instance types that support local instance storage, see Instance
* Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*
*
* @param kmsKeyId
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to
* encrypt data on the storage volume attached to the ML compute instance that hosts the endpoint.
*
* The KmsKeyId can be any of the following formats:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Alias name: alias/ExampleAlias
*
*
* -
*
* Alias name ARN: arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
*
*
*
*
* The KMS key policy must grant permission to the IAM role that you specify in your
* CreateEndpoint
, UpdateEndpoint
requests. For more information, refer to the
* Amazon Web Services Key Management Service section Using Key Policies in
* Amazon Web Services KMS
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage volumes
* are encrypted using a hardware module on the instance. You can't request a KmsKeyId
when
* using an instance type with local storage. If any of the models that you specify in the
* ProductionVariants
parameter use nitro-based instances with local storage, do not specify a
* value for the KmsKeyId
parameter. If you specify a value for KmsKeyId
when using
* any nitro-based instances with local storage, the call to CreateEndpointConfig
fails.
*
*
* For a list of instance types that support local instance storage, see Instance Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to encrypt
* data on the storage volume attached to the ML compute instance that hosts the endpoint.
*
*
* The KmsKeyId can be any of the following formats:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Alias name: alias/ExampleAlias
*
*
* -
*
* Alias name ARN: arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
*
*
*
*
* The KMS key policy must grant permission to the IAM role that you specify in your CreateEndpoint
,
* UpdateEndpoint
requests. For more information, refer to the Amazon Web Services Key Management
* Service section Using Key
* Policies in Amazon Web Services KMS
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage volumes are
* encrypted using a hardware module on the instance. You can't request a KmsKeyId
when using an
* instance type with local storage. If any of the models that you specify in the ProductionVariants
* parameter use nitro-based instances with local storage, do not specify a value for the KmsKeyId
* parameter. If you specify a value for KmsKeyId
when using any nitro-based instances with local
* storage, the call to CreateEndpointConfig
fails.
*
*
* For a list of instance types that support local instance storage, see Instance
* Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*
*
* @return The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to
* encrypt data on the storage volume attached to the ML compute instance that hosts the endpoint.
*
* The KmsKeyId can be any of the following formats:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Alias name: alias/ExampleAlias
*
*
* -
*
* Alias name ARN: arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
*
*
*
*
* The KMS key policy must grant permission to the IAM role that you specify in your
* CreateEndpoint
, UpdateEndpoint
requests. For more information, refer to the
* Amazon Web Services Key Management Service section Using Key Policies in
* Amazon Web Services KMS
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage
* volumes are encrypted using a hardware module on the instance. You can't request a KmsKeyId
* when using an instance type with local storage. If any of the models that you specify in the
* ProductionVariants
parameter use nitro-based instances with local storage, do not specify a
* value for the KmsKeyId
parameter. If you specify a value for KmsKeyId
when
* using any nitro-based instances with local storage, the call to CreateEndpointConfig
fails.
*
*
* For a list of instance types that support local instance storage, see Instance Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to encrypt
* data on the storage volume attached to the ML compute instance that hosts the endpoint.
*
*
* The KmsKeyId can be any of the following formats:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Alias name: alias/ExampleAlias
*
*
* -
*
* Alias name ARN: arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
*
*
*
*
* The KMS key policy must grant permission to the IAM role that you specify in your CreateEndpoint
,
* UpdateEndpoint
requests. For more information, refer to the Amazon Web Services Key Management
* Service section Using Key
* Policies in Amazon Web Services KMS
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage volumes are
* encrypted using a hardware module on the instance. You can't request a KmsKeyId
when using an
* instance type with local storage. If any of the models that you specify in the ProductionVariants
* parameter use nitro-based instances with local storage, do not specify a value for the KmsKeyId
* parameter. If you specify a value for KmsKeyId
when using any nitro-based instances with local
* storage, the call to CreateEndpointConfig
fails.
*
*
* For a list of instance types that support local instance storage, see Instance
* Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
*
*
* @param kmsKeyId
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to
* encrypt data on the storage volume attached to the ML compute instance that hosts the endpoint.
*
* The KmsKeyId can be any of the following formats:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-west-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Alias name: alias/ExampleAlias
*
*
* -
*
* Alias name ARN: arn:aws:kms:us-west-2:111122223333:alias/ExampleAlias
*
*
*
*
* The KMS key policy must grant permission to the IAM role that you specify in your
* CreateEndpoint
, UpdateEndpoint
requests. For more information, refer to the
* Amazon Web Services Key Management Service section Using Key Policies in
* Amazon Web Services KMS
*
*
*
* Certain Nitro-based instances include local storage, dependent on the instance type. Local storage volumes
* are encrypted using a hardware module on the instance. You can't request a KmsKeyId
when
* using an instance type with local storage. If any of the models that you specify in the
* ProductionVariants
parameter use nitro-based instances with local storage, do not specify a
* value for the KmsKeyId
parameter. If you specify a value for KmsKeyId
when using
* any nitro-based instances with local storage, the call to CreateEndpointConfig
fails.
*
*
* For a list of instance types that support local instance storage, see Instance Store Volumes.
*
*
* For more information about local instance storage encryption, see SSD Instance Store
* Volumes.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* Specifies configuration for how an endpoint performs asynchronous inference. This is a required field in order
* for your Endpoint to be invoked using InvokeEndpointAsync.
*
*
* @param asyncInferenceConfig
* Specifies configuration for how an endpoint performs asynchronous inference. This is a required field in
* order for your Endpoint to be invoked using InvokeEndpointAsync.
*/
public void setAsyncInferenceConfig(AsyncInferenceConfig asyncInferenceConfig) {
this.asyncInferenceConfig = asyncInferenceConfig;
}
/**
*
* Specifies configuration for how an endpoint performs asynchronous inference. This is a required field in order
* for your Endpoint to be invoked using InvokeEndpointAsync.
*
*
* @return Specifies configuration for how an endpoint performs asynchronous inference. This is a required field in
* order for your Endpoint to be invoked using InvokeEndpointAsync.
*/
public AsyncInferenceConfig getAsyncInferenceConfig() {
return this.asyncInferenceConfig;
}
/**
*
* Specifies configuration for how an endpoint performs asynchronous inference. This is a required field in order
* for your Endpoint to be invoked using InvokeEndpointAsync.
*
*
* @param asyncInferenceConfig
* Specifies configuration for how an endpoint performs asynchronous inference. This is a required field in
* order for your Endpoint to be invoked using InvokeEndpointAsync.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withAsyncInferenceConfig(AsyncInferenceConfig asyncInferenceConfig) {
setAsyncInferenceConfig(asyncInferenceConfig);
return this;
}
/**
*
* A member of CreateEndpointConfig
that enables explainers.
*
*
* @param explainerConfig
* A member of CreateEndpointConfig
that enables explainers.
*/
public void setExplainerConfig(ExplainerConfig explainerConfig) {
this.explainerConfig = explainerConfig;
}
/**
*
* A member of CreateEndpointConfig
that enables explainers.
*
*
* @return A member of CreateEndpointConfig
that enables explainers.
*/
public ExplainerConfig getExplainerConfig() {
return this.explainerConfig;
}
/**
*
* A member of CreateEndpointConfig
that enables explainers.
*
*
* @param explainerConfig
* A member of CreateEndpointConfig
that enables explainers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withExplainerConfig(ExplainerConfig explainerConfig) {
setExplainerConfig(explainerConfig);
return this;
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint in
* shadow mode with production traffic replicated from the model specified on ProductionVariants
. If
* you use this field, you can only specify one variant for ProductionVariants
and one variant for
* ShadowProductionVariants
.
*
*
* @return An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint in shadow mode with production traffic replicated from the model specified on
* ProductionVariants
. If you use this field, you can only specify one variant for
* ProductionVariants
and one variant for ShadowProductionVariants
.
*/
public java.util.List getShadowProductionVariants() {
return shadowProductionVariants;
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint in
* shadow mode with production traffic replicated from the model specified on ProductionVariants
. If
* you use this field, you can only specify one variant for ProductionVariants
and one variant for
* ShadowProductionVariants
.
*
*
* @param shadowProductionVariants
* An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint in shadow mode with production traffic replicated from the model specified on
* ProductionVariants
. If you use this field, you can only specify one variant for
* ProductionVariants
and one variant for ShadowProductionVariants
.
*/
public void setShadowProductionVariants(java.util.Collection shadowProductionVariants) {
if (shadowProductionVariants == null) {
this.shadowProductionVariants = null;
return;
}
this.shadowProductionVariants = new java.util.ArrayList(shadowProductionVariants);
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint in
* shadow mode with production traffic replicated from the model specified on ProductionVariants
. If
* you use this field, you can only specify one variant for ProductionVariants
and one variant for
* ShadowProductionVariants
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setShadowProductionVariants(java.util.Collection)} or
* {@link #withShadowProductionVariants(java.util.Collection)} if you want to override the existing values.
*
*
* @param shadowProductionVariants
* An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint in shadow mode with production traffic replicated from the model specified on
* ProductionVariants
. If you use this field, you can only specify one variant for
* ProductionVariants
and one variant for ShadowProductionVariants
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withShadowProductionVariants(ProductionVariant... shadowProductionVariants) {
if (this.shadowProductionVariants == null) {
setShadowProductionVariants(new java.util.ArrayList(shadowProductionVariants.length));
}
for (ProductionVariant ele : shadowProductionVariants) {
this.shadowProductionVariants.add(ele);
}
return this;
}
/**
*
* An array of ProductionVariant
objects, one for each model that you want to host at this endpoint in
* shadow mode with production traffic replicated from the model specified on ProductionVariants
. If
* you use this field, you can only specify one variant for ProductionVariants
and one variant for
* ShadowProductionVariants
.
*
*
* @param shadowProductionVariants
* An array of ProductionVariant
objects, one for each model that you want to host at this
* endpoint in shadow mode with production traffic replicated from the model specified on
* ProductionVariants
. If you use this field, you can only specify one variant for
* ProductionVariants
and one variant for ShadowProductionVariants
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withShadowProductionVariants(java.util.Collection shadowProductionVariants) {
setShadowProductionVariants(shadowProductionVariants);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to perform actions on your behalf.
* For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to Amazon SageMaker, the caller of this action must have the
* iam:PassRole
permission.
*
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to perform actions on your
* behalf. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to Amazon SageMaker, the caller of this action must have the
* iam:PassRole
permission.
*
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to perform actions on your behalf.
* For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to Amazon SageMaker, the caller of this action must have the
* iam:PassRole
permission.
*
*
*
* @return The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to perform actions on your
* behalf. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to Amazon SageMaker, the caller of this action must have the
* iam:PassRole
permission.
*
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to perform actions on your behalf.
* For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to Amazon SageMaker, the caller of this action must have the
* iam:PassRole
permission.
*
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of an IAM role that Amazon SageMaker can assume to perform actions on your
* behalf. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to Amazon SageMaker, the caller of this action must have the
* iam:PassRole
permission.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
* @param vpcConfig
*/
public void setVpcConfig(VpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
* @return
*/
public VpcConfig getVpcConfig() {
return this.vpcConfig;
}
/**
* @param vpcConfig
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withVpcConfig(VpcConfig vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
*
* Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or outbound
* network calls can be made to or from the model containers.
*
*
* @param enableNetworkIsolation
* Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or
* outbound network calls can be made to or from the model containers.
*/
public void setEnableNetworkIsolation(Boolean enableNetworkIsolation) {
this.enableNetworkIsolation = enableNetworkIsolation;
}
/**
*
* Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or outbound
* network calls can be made to or from the model containers.
*
*
* @return Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or
* outbound network calls can be made to or from the model containers.
*/
public Boolean getEnableNetworkIsolation() {
return this.enableNetworkIsolation;
}
/**
*
* Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or outbound
* network calls can be made to or from the model containers.
*
*
* @param enableNetworkIsolation
* Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or
* outbound network calls can be made to or from the model containers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateEndpointConfigRequest withEnableNetworkIsolation(Boolean enableNetworkIsolation) {
setEnableNetworkIsolation(enableNetworkIsolation);
return this;
}
/**
*
* Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or outbound
* network calls can be made to or from the model containers.
*
*
* @return Sets whether all model containers deployed to the endpoint are isolated. If they are, no inbound or
* outbound network calls can be made to or from the model containers.
*/
public Boolean isEnableNetworkIsolation() {
return this.enableNetworkIsolation;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEndpointConfigName() != null)
sb.append("EndpointConfigName: ").append(getEndpointConfigName()).append(",");
if (getProductionVariants() != null)
sb.append("ProductionVariants: ").append(getProductionVariants()).append(",");
if (getDataCaptureConfig() != null)
sb.append("DataCaptureConfig: ").append(getDataCaptureConfig()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getAsyncInferenceConfig() != null)
sb.append("AsyncInferenceConfig: ").append(getAsyncInferenceConfig()).append(",");
if (getExplainerConfig() != null)
sb.append("ExplainerConfig: ").append(getExplainerConfig()).append(",");
if (getShadowProductionVariants() != null)
sb.append("ShadowProductionVariants: ").append(getShadowProductionVariants()).append(",");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getVpcConfig() != null)
sb.append("VpcConfig: ").append(getVpcConfig()).append(",");
if (getEnableNetworkIsolation() != null)
sb.append("EnableNetworkIsolation: ").append(getEnableNetworkIsolation());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateEndpointConfigRequest == false)
return false;
CreateEndpointConfigRequest other = (CreateEndpointConfigRequest) obj;
if (other.getEndpointConfigName() == null ^ this.getEndpointConfigName() == null)
return false;
if (other.getEndpointConfigName() != null && other.getEndpointConfigName().equals(this.getEndpointConfigName()) == false)
return false;
if (other.getProductionVariants() == null ^ this.getProductionVariants() == null)
return false;
if (other.getProductionVariants() != null && other.getProductionVariants().equals(this.getProductionVariants()) == false)
return false;
if (other.getDataCaptureConfig() == null ^ this.getDataCaptureConfig() == null)
return false;
if (other.getDataCaptureConfig() != null && other.getDataCaptureConfig().equals(this.getDataCaptureConfig()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getAsyncInferenceConfig() == null ^ this.getAsyncInferenceConfig() == null)
return false;
if (other.getAsyncInferenceConfig() != null && other.getAsyncInferenceConfig().equals(this.getAsyncInferenceConfig()) == false)
return false;
if (other.getExplainerConfig() == null ^ this.getExplainerConfig() == null)
return false;
if (other.getExplainerConfig() != null && other.getExplainerConfig().equals(this.getExplainerConfig()) == false)
return false;
if (other.getShadowProductionVariants() == null ^ this.getShadowProductionVariants() == null)
return false;
if (other.getShadowProductionVariants() != null && other.getShadowProductionVariants().equals(this.getShadowProductionVariants()) == false)
return false;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null && other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
if (other.getEnableNetworkIsolation() == null ^ this.getEnableNetworkIsolation() == null)
return false;
if (other.getEnableNetworkIsolation() != null && other.getEnableNetworkIsolation().equals(this.getEnableNetworkIsolation()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEndpointConfigName() == null) ? 0 : getEndpointConfigName().hashCode());
hashCode = prime * hashCode + ((getProductionVariants() == null) ? 0 : getProductionVariants().hashCode());
hashCode = prime * hashCode + ((getDataCaptureConfig() == null) ? 0 : getDataCaptureConfig().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getAsyncInferenceConfig() == null) ? 0 : getAsyncInferenceConfig().hashCode());
hashCode = prime * hashCode + ((getExplainerConfig() == null) ? 0 : getExplainerConfig().hashCode());
hashCode = prime * hashCode + ((getShadowProductionVariants() == null) ? 0 : getShadowProductionVariants().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
hashCode = prime * hashCode + ((getEnableNetworkIsolation() == null) ? 0 : getEnableNetworkIsolation().hashCode());
return hashCode;
}
@Override
public CreateEndpointConfigRequest clone() {
return (CreateEndpointConfigRequest) super.clone();
}
}