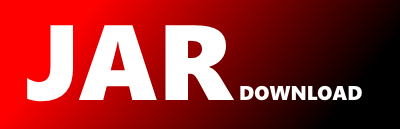
com.amazonaws.services.sagemaker.model.CreateFeatureGroupRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateFeatureGroupRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the FeatureGroup
. The name must be unique within an Amazon Web Services Region in an
* Amazon Web Services account.
*
*
* The name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only include alphanumeric characters, underscores, and hyphens. Spaces are not allowed.
*
*
*
*/
private String featureGroupName;
/**
*
* The name of the Feature
whose value uniquely identifies a Record
defined in the
* FeatureStore
. Only the latest record per identifier value will be stored in the
* OnlineStore
. RecordIdentifierFeatureName
must be one of feature definitions' names.
*
*
* You use the RecordIdentifierFeatureName
to access data in a FeatureStore
.
*
*
* This name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only contains alphanumeric characters, hyphens, underscores. Spaces are not allowed.
*
*
*
*/
private String recordIdentifierFeatureName;
/**
*
* The name of the feature that stores the EventTime
of a Record
in a
* FeatureGroup
.
*
*
* An EventTime
is a point in time when a new event occurs that corresponds to the creation or update
* of a Record
in a FeatureGroup
. All Records
in the
* FeatureGroup
must have a corresponding EventTime
.
*
*
* An EventTime
can be a String
or Fractional
.
*
*
* -
*
* Fractional
: EventTime
feature values must be a Unix timestamp in seconds.
*
*
* -
*
* String
: EventTime
feature values must be an ISO-8601 string in the format. The
* following formats are supported yyyy-MM-dd'T'HH:mm:ssZ
and yyyy-MM-dd'T'HH:mm:ss.SSSZ
* where yyyy
, MM
, and dd
represent the year, month, and day respectively and
* HH
, mm
, ss
, and if applicable, SSS
represent the hour, month,
* second and milliseconds respsectively. 'T'
and Z
are constants.
*
*
*
*/
private String eventTimeFeatureName;
/**
*
* A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
, write_time
,
* api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
*
*/
private java.util.List featureDefinitions;
/**
*
* You can turn the OnlineStore
on or off by specifying True
for the
* EnableOnlineStore
flag in OnlineStoreConfig
.
*
*
* You can also include an Amazon Web Services KMS key ID (KMSKeyId
) for at-rest encryption of the
* OnlineStore
.
*
*
* The default value is False
.
*
*/
private OnlineStoreConfig onlineStoreConfig;
/**
*
* Use this to configure an OfflineFeatureStore
. This parameter allows you to specify:
*
*
* -
*
* The Amazon Simple Storage Service (Amazon S3) location of an OfflineStore
.
*
*
* -
*
* A configuration for an Amazon Web Services Glue or Amazon Web Services Hive data catalog.
*
*
* -
*
* An KMS encryption key to encrypt the Amazon S3 location used for OfflineStore
. If KMS encryption key
* is not specified, by default we encrypt all data at rest using Amazon Web Services KMS key. By defining your bucket-level key for SSE, you
* can reduce Amazon Web Services KMS requests costs by up to 99 percent.
*
*
* -
*
* Format for the offline store table. Supported formats are Glue (Default) and Apache Iceberg.
*
*
*
*
* To learn more about this parameter, see OfflineStoreConfig.
*
*/
private OfflineStoreConfig offlineStoreConfig;
private ThroughputConfig throughputConfig;
/**
*
* The Amazon Resource Name (ARN) of the IAM execution role used to persist data into the OfflineStore
* if an OfflineStoreConfig
is provided.
*
*/
private String roleArn;
/**
*
* A free-form description of a FeatureGroup
.
*
*/
private String description;
/**
*
* Tags used to identify Features
in each FeatureGroup
.
*
*/
private java.util.List tags;
/**
*
* The name of the FeatureGroup
. The name must be unique within an Amazon Web Services Region in an
* Amazon Web Services account.
*
*
* The name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only include alphanumeric characters, underscores, and hyphens. Spaces are not allowed.
*
*
*
*
* @param featureGroupName
* The name of the FeatureGroup
. The name must be unique within an Amazon Web Services Region in
* an Amazon Web Services account.
*
* The name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only include alphanumeric characters, underscores, and hyphens. Spaces are not allowed.
*
*
*/
public void setFeatureGroupName(String featureGroupName) {
this.featureGroupName = featureGroupName;
}
/**
*
* The name of the FeatureGroup
. The name must be unique within an Amazon Web Services Region in an
* Amazon Web Services account.
*
*
* The name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only include alphanumeric characters, underscores, and hyphens. Spaces are not allowed.
*
*
*
*
* @return The name of the FeatureGroup
. The name must be unique within an Amazon Web Services Region
* in an Amazon Web Services account.
*
* The name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only include alphanumeric characters, underscores, and hyphens. Spaces are not allowed.
*
*
*/
public String getFeatureGroupName() {
return this.featureGroupName;
}
/**
*
* The name of the FeatureGroup
. The name must be unique within an Amazon Web Services Region in an
* Amazon Web Services account.
*
*
* The name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only include alphanumeric characters, underscores, and hyphens. Spaces are not allowed.
*
*
*
*
* @param featureGroupName
* The name of the FeatureGroup
. The name must be unique within an Amazon Web Services Region in
* an Amazon Web Services account.
*
* The name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only include alphanumeric characters, underscores, and hyphens. Spaces are not allowed.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withFeatureGroupName(String featureGroupName) {
setFeatureGroupName(featureGroupName);
return this;
}
/**
*
* The name of the Feature
whose value uniquely identifies a Record
defined in the
* FeatureStore
. Only the latest record per identifier value will be stored in the
* OnlineStore
. RecordIdentifierFeatureName
must be one of feature definitions' names.
*
*
* You use the RecordIdentifierFeatureName
to access data in a FeatureStore
.
*
*
* This name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only contains alphanumeric characters, hyphens, underscores. Spaces are not allowed.
*
*
*
*
* @param recordIdentifierFeatureName
* The name of the Feature
whose value uniquely identifies a Record
defined in the
* FeatureStore
. Only the latest record per identifier value will be stored in the
* OnlineStore
. RecordIdentifierFeatureName
must be one of feature definitions'
* names.
*
* You use the RecordIdentifierFeatureName
to access data in a FeatureStore
.
*
*
* This name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only contains alphanumeric characters, hyphens, underscores. Spaces are not allowed.
*
*
*/
public void setRecordIdentifierFeatureName(String recordIdentifierFeatureName) {
this.recordIdentifierFeatureName = recordIdentifierFeatureName;
}
/**
*
* The name of the Feature
whose value uniquely identifies a Record
defined in the
* FeatureStore
. Only the latest record per identifier value will be stored in the
* OnlineStore
. RecordIdentifierFeatureName
must be one of feature definitions' names.
*
*
* You use the RecordIdentifierFeatureName
to access data in a FeatureStore
.
*
*
* This name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only contains alphanumeric characters, hyphens, underscores. Spaces are not allowed.
*
*
*
*
* @return The name of the Feature
whose value uniquely identifies a Record
defined in the
* FeatureStore
. Only the latest record per identifier value will be stored in the
* OnlineStore
. RecordIdentifierFeatureName
must be one of feature definitions'
* names.
*
* You use the RecordIdentifierFeatureName
to access data in a FeatureStore
.
*
*
* This name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only contains alphanumeric characters, hyphens, underscores. Spaces are not allowed.
*
*
*/
public String getRecordIdentifierFeatureName() {
return this.recordIdentifierFeatureName;
}
/**
*
* The name of the Feature
whose value uniquely identifies a Record
defined in the
* FeatureStore
. Only the latest record per identifier value will be stored in the
* OnlineStore
. RecordIdentifierFeatureName
must be one of feature definitions' names.
*
*
* You use the RecordIdentifierFeatureName
to access data in a FeatureStore
.
*
*
* This name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only contains alphanumeric characters, hyphens, underscores. Spaces are not allowed.
*
*
*
*
* @param recordIdentifierFeatureName
* The name of the Feature
whose value uniquely identifies a Record
defined in the
* FeatureStore
. Only the latest record per identifier value will be stored in the
* OnlineStore
. RecordIdentifierFeatureName
must be one of feature definitions'
* names.
*
* You use the RecordIdentifierFeatureName
to access data in a FeatureStore
.
*
*
* This name:
*
*
* -
*
* Must start with an alphanumeric character.
*
*
* -
*
* Can only contains alphanumeric characters, hyphens, underscores. Spaces are not allowed.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withRecordIdentifierFeatureName(String recordIdentifierFeatureName) {
setRecordIdentifierFeatureName(recordIdentifierFeatureName);
return this;
}
/**
*
* The name of the feature that stores the EventTime
of a Record
in a
* FeatureGroup
.
*
*
* An EventTime
is a point in time when a new event occurs that corresponds to the creation or update
* of a Record
in a FeatureGroup
. All Records
in the
* FeatureGroup
must have a corresponding EventTime
.
*
*
* An EventTime
can be a String
or Fractional
.
*
*
* -
*
* Fractional
: EventTime
feature values must be a Unix timestamp in seconds.
*
*
* -
*
* String
: EventTime
feature values must be an ISO-8601 string in the format. The
* following formats are supported yyyy-MM-dd'T'HH:mm:ssZ
and yyyy-MM-dd'T'HH:mm:ss.SSSZ
* where yyyy
, MM
, and dd
represent the year, month, and day respectively and
* HH
, mm
, ss
, and if applicable, SSS
represent the hour, month,
* second and milliseconds respsectively. 'T'
and Z
are constants.
*
*
*
*
* @param eventTimeFeatureName
* The name of the feature that stores the EventTime
of a Record
in a
* FeatureGroup
.
*
* An EventTime
is a point in time when a new event occurs that corresponds to the creation or
* update of a Record
in a FeatureGroup
. All Records
in the
* FeatureGroup
must have a corresponding EventTime
.
*
*
* An EventTime
can be a String
or Fractional
.
*
*
* -
*
* Fractional
: EventTime
feature values must be a Unix timestamp in seconds.
*
*
* -
*
* String
: EventTime
feature values must be an ISO-8601 string in the format. The
* following formats are supported yyyy-MM-dd'T'HH:mm:ssZ
and
* yyyy-MM-dd'T'HH:mm:ss.SSSZ
where yyyy
, MM
, and dd
* represent the year, month, and day respectively and HH
, mm
, ss
, and
* if applicable, SSS
represent the hour, month, second and milliseconds respsectively.
* 'T'
and Z
are constants.
*
*
*/
public void setEventTimeFeatureName(String eventTimeFeatureName) {
this.eventTimeFeatureName = eventTimeFeatureName;
}
/**
*
* The name of the feature that stores the EventTime
of a Record
in a
* FeatureGroup
.
*
*
* An EventTime
is a point in time when a new event occurs that corresponds to the creation or update
* of a Record
in a FeatureGroup
. All Records
in the
* FeatureGroup
must have a corresponding EventTime
.
*
*
* An EventTime
can be a String
or Fractional
.
*
*
* -
*
* Fractional
: EventTime
feature values must be a Unix timestamp in seconds.
*
*
* -
*
* String
: EventTime
feature values must be an ISO-8601 string in the format. The
* following formats are supported yyyy-MM-dd'T'HH:mm:ssZ
and yyyy-MM-dd'T'HH:mm:ss.SSSZ
* where yyyy
, MM
, and dd
represent the year, month, and day respectively and
* HH
, mm
, ss
, and if applicable, SSS
represent the hour, month,
* second and milliseconds respsectively. 'T'
and Z
are constants.
*
*
*
*
* @return The name of the feature that stores the EventTime
of a Record
in a
* FeatureGroup
.
*
* An EventTime
is a point in time when a new event occurs that corresponds to the creation or
* update of a Record
in a FeatureGroup
. All Records
in the
* FeatureGroup
must have a corresponding EventTime
.
*
*
* An EventTime
can be a String
or Fractional
.
*
*
* -
*
* Fractional
: EventTime
feature values must be a Unix timestamp in seconds.
*
*
* -
*
* String
: EventTime
feature values must be an ISO-8601 string in the format. The
* following formats are supported yyyy-MM-dd'T'HH:mm:ssZ
and
* yyyy-MM-dd'T'HH:mm:ss.SSSZ
where yyyy
, MM
, and dd
* represent the year, month, and day respectively and HH
, mm
, ss
,
* and if applicable, SSS
represent the hour, month, second and milliseconds respsectively.
* 'T'
and Z
are constants.
*
*
*/
public String getEventTimeFeatureName() {
return this.eventTimeFeatureName;
}
/**
*
* The name of the feature that stores the EventTime
of a Record
in a
* FeatureGroup
.
*
*
* An EventTime
is a point in time when a new event occurs that corresponds to the creation or update
* of a Record
in a FeatureGroup
. All Records
in the
* FeatureGroup
must have a corresponding EventTime
.
*
*
* An EventTime
can be a String
or Fractional
.
*
*
* -
*
* Fractional
: EventTime
feature values must be a Unix timestamp in seconds.
*
*
* -
*
* String
: EventTime
feature values must be an ISO-8601 string in the format. The
* following formats are supported yyyy-MM-dd'T'HH:mm:ssZ
and yyyy-MM-dd'T'HH:mm:ss.SSSZ
* where yyyy
, MM
, and dd
represent the year, month, and day respectively and
* HH
, mm
, ss
, and if applicable, SSS
represent the hour, month,
* second and milliseconds respsectively. 'T'
and Z
are constants.
*
*
*
*
* @param eventTimeFeatureName
* The name of the feature that stores the EventTime
of a Record
in a
* FeatureGroup
.
*
* An EventTime
is a point in time when a new event occurs that corresponds to the creation or
* update of a Record
in a FeatureGroup
. All Records
in the
* FeatureGroup
must have a corresponding EventTime
.
*
*
* An EventTime
can be a String
or Fractional
.
*
*
* -
*
* Fractional
: EventTime
feature values must be a Unix timestamp in seconds.
*
*
* -
*
* String
: EventTime
feature values must be an ISO-8601 string in the format. The
* following formats are supported yyyy-MM-dd'T'HH:mm:ssZ
and
* yyyy-MM-dd'T'HH:mm:ss.SSSZ
where yyyy
, MM
, and dd
* represent the year, month, and day respectively and HH
, mm
, ss
, and
* if applicable, SSS
represent the hour, month, second and milliseconds respsectively.
* 'T'
and Z
are constants.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withEventTimeFeatureName(String eventTimeFeatureName) {
setEventTimeFeatureName(eventTimeFeatureName);
return this;
}
/**
*
* A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
, write_time
,
* api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
*
*
* @return A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
,
* write_time
, api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
*/
public java.util.List getFeatureDefinitions() {
return featureDefinitions;
}
/**
*
* A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
, write_time
,
* api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
*
*
* @param featureDefinitions
* A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
, write_time
, api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
*/
public void setFeatureDefinitions(java.util.Collection featureDefinitions) {
if (featureDefinitions == null) {
this.featureDefinitions = null;
return;
}
this.featureDefinitions = new java.util.ArrayList(featureDefinitions);
}
/**
*
* A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
, write_time
,
* api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFeatureDefinitions(java.util.Collection)} or {@link #withFeatureDefinitions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param featureDefinitions
* A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
, write_time
, api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withFeatureDefinitions(FeatureDefinition... featureDefinitions) {
if (this.featureDefinitions == null) {
setFeatureDefinitions(new java.util.ArrayList(featureDefinitions.length));
}
for (FeatureDefinition ele : featureDefinitions) {
this.featureDefinitions.add(ele);
}
return this;
}
/**
*
* A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
, write_time
,
* api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
*
*
* @param featureDefinitions
* A list of Feature
names and types. Name
and Type
is compulsory per
* Feature
.
*
* Valid feature FeatureType
s are Integral
, Fractional
and
* String
.
*
*
* FeatureName
s cannot be any of the following: is_deleted
, write_time
, api_invocation_time
*
*
* You can create up to 2,500 FeatureDefinition
s per FeatureGroup
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withFeatureDefinitions(java.util.Collection featureDefinitions) {
setFeatureDefinitions(featureDefinitions);
return this;
}
/**
*
* You can turn the OnlineStore
on or off by specifying True
for the
* EnableOnlineStore
flag in OnlineStoreConfig
.
*
*
* You can also include an Amazon Web Services KMS key ID (KMSKeyId
) for at-rest encryption of the
* OnlineStore
.
*
*
* The default value is False
.
*
*
* @param onlineStoreConfig
* You can turn the OnlineStore
on or off by specifying True
for the
* EnableOnlineStore
flag in OnlineStoreConfig
.
*
* You can also include an Amazon Web Services KMS key ID (KMSKeyId
) for at-rest encryption of
* the OnlineStore
.
*
*
* The default value is False
.
*/
public void setOnlineStoreConfig(OnlineStoreConfig onlineStoreConfig) {
this.onlineStoreConfig = onlineStoreConfig;
}
/**
*
* You can turn the OnlineStore
on or off by specifying True
for the
* EnableOnlineStore
flag in OnlineStoreConfig
.
*
*
* You can also include an Amazon Web Services KMS key ID (KMSKeyId
) for at-rest encryption of the
* OnlineStore
.
*
*
* The default value is False
.
*
*
* @return You can turn the OnlineStore
on or off by specifying True
for the
* EnableOnlineStore
flag in OnlineStoreConfig
.
*
* You can also include an Amazon Web Services KMS key ID (KMSKeyId
) for at-rest encryption of
* the OnlineStore
.
*
*
* The default value is False
.
*/
public OnlineStoreConfig getOnlineStoreConfig() {
return this.onlineStoreConfig;
}
/**
*
* You can turn the OnlineStore
on or off by specifying True
for the
* EnableOnlineStore
flag in OnlineStoreConfig
.
*
*
* You can also include an Amazon Web Services KMS key ID (KMSKeyId
) for at-rest encryption of the
* OnlineStore
.
*
*
* The default value is False
.
*
*
* @param onlineStoreConfig
* You can turn the OnlineStore
on or off by specifying True
for the
* EnableOnlineStore
flag in OnlineStoreConfig
.
*
* You can also include an Amazon Web Services KMS key ID (KMSKeyId
) for at-rest encryption of
* the OnlineStore
.
*
*
* The default value is False
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withOnlineStoreConfig(OnlineStoreConfig onlineStoreConfig) {
setOnlineStoreConfig(onlineStoreConfig);
return this;
}
/**
*
* Use this to configure an OfflineFeatureStore
. This parameter allows you to specify:
*
*
* -
*
* The Amazon Simple Storage Service (Amazon S3) location of an OfflineStore
.
*
*
* -
*
* A configuration for an Amazon Web Services Glue or Amazon Web Services Hive data catalog.
*
*
* -
*
* An KMS encryption key to encrypt the Amazon S3 location used for OfflineStore
. If KMS encryption key
* is not specified, by default we encrypt all data at rest using Amazon Web Services KMS key. By defining your bucket-level key for SSE, you
* can reduce Amazon Web Services KMS requests costs by up to 99 percent.
*
*
* -
*
* Format for the offline store table. Supported formats are Glue (Default) and Apache Iceberg.
*
*
*
*
* To learn more about this parameter, see OfflineStoreConfig.
*
*
* @param offlineStoreConfig
* Use this to configure an OfflineFeatureStore
. This parameter allows you to specify:
*
* -
*
* The Amazon Simple Storage Service (Amazon S3) location of an OfflineStore
.
*
*
* -
*
* A configuration for an Amazon Web Services Glue or Amazon Web Services Hive data catalog.
*
*
* -
*
* An KMS encryption key to encrypt the Amazon S3 location used for OfflineStore
. If KMS
* encryption key is not specified, by default we encrypt all data at rest using Amazon Web Services KMS key.
* By defining your bucket-level key for SSE,
* you can reduce Amazon Web Services KMS requests costs by up to 99 percent.
*
*
* -
*
* Format for the offline store table. Supported formats are Glue (Default) and Apache Iceberg.
*
*
*
*
* To learn more about this parameter, see OfflineStoreConfig.
*/
public void setOfflineStoreConfig(OfflineStoreConfig offlineStoreConfig) {
this.offlineStoreConfig = offlineStoreConfig;
}
/**
*
* Use this to configure an OfflineFeatureStore
. This parameter allows you to specify:
*
*
* -
*
* The Amazon Simple Storage Service (Amazon S3) location of an OfflineStore
.
*
*
* -
*
* A configuration for an Amazon Web Services Glue or Amazon Web Services Hive data catalog.
*
*
* -
*
* An KMS encryption key to encrypt the Amazon S3 location used for OfflineStore
. If KMS encryption key
* is not specified, by default we encrypt all data at rest using Amazon Web Services KMS key. By defining your bucket-level key for SSE, you
* can reduce Amazon Web Services KMS requests costs by up to 99 percent.
*
*
* -
*
* Format for the offline store table. Supported formats are Glue (Default) and Apache Iceberg.
*
*
*
*
* To learn more about this parameter, see OfflineStoreConfig.
*
*
* @return Use this to configure an OfflineFeatureStore
. This parameter allows you to specify:
*
* -
*
* The Amazon Simple Storage Service (Amazon S3) location of an OfflineStore
.
*
*
* -
*
* A configuration for an Amazon Web Services Glue or Amazon Web Services Hive data catalog.
*
*
* -
*
* An KMS encryption key to encrypt the Amazon S3 location used for OfflineStore
. If KMS
* encryption key is not specified, by default we encrypt all data at rest using Amazon Web Services KMS
* key. By defining your bucket-level key for
* SSE, you can reduce Amazon Web Services KMS requests costs by up to 99 percent.
*
*
* -
*
* Format for the offline store table. Supported formats are Glue (Default) and Apache Iceberg.
*
*
*
*
* To learn more about this parameter, see OfflineStoreConfig.
*/
public OfflineStoreConfig getOfflineStoreConfig() {
return this.offlineStoreConfig;
}
/**
*
* Use this to configure an OfflineFeatureStore
. This parameter allows you to specify:
*
*
* -
*
* The Amazon Simple Storage Service (Amazon S3) location of an OfflineStore
.
*
*
* -
*
* A configuration for an Amazon Web Services Glue or Amazon Web Services Hive data catalog.
*
*
* -
*
* An KMS encryption key to encrypt the Amazon S3 location used for OfflineStore
. If KMS encryption key
* is not specified, by default we encrypt all data at rest using Amazon Web Services KMS key. By defining your bucket-level key for SSE, you
* can reduce Amazon Web Services KMS requests costs by up to 99 percent.
*
*
* -
*
* Format for the offline store table. Supported formats are Glue (Default) and Apache Iceberg.
*
*
*
*
* To learn more about this parameter, see OfflineStoreConfig.
*
*
* @param offlineStoreConfig
* Use this to configure an OfflineFeatureStore
. This parameter allows you to specify:
*
* -
*
* The Amazon Simple Storage Service (Amazon S3) location of an OfflineStore
.
*
*
* -
*
* A configuration for an Amazon Web Services Glue or Amazon Web Services Hive data catalog.
*
*
* -
*
* An KMS encryption key to encrypt the Amazon S3 location used for OfflineStore
. If KMS
* encryption key is not specified, by default we encrypt all data at rest using Amazon Web Services KMS key.
* By defining your bucket-level key for SSE,
* you can reduce Amazon Web Services KMS requests costs by up to 99 percent.
*
*
* -
*
* Format for the offline store table. Supported formats are Glue (Default) and Apache Iceberg.
*
*
*
*
* To learn more about this parameter, see OfflineStoreConfig.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withOfflineStoreConfig(OfflineStoreConfig offlineStoreConfig) {
setOfflineStoreConfig(offlineStoreConfig);
return this;
}
/**
* @param throughputConfig
*/
public void setThroughputConfig(ThroughputConfig throughputConfig) {
this.throughputConfig = throughputConfig;
}
/**
* @return
*/
public ThroughputConfig getThroughputConfig() {
return this.throughputConfig;
}
/**
* @param throughputConfig
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withThroughputConfig(ThroughputConfig throughputConfig) {
setThroughputConfig(throughputConfig);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM execution role used to persist data into the OfflineStore
* if an OfflineStoreConfig
is provided.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM execution role used to persist data into the
* OfflineStore
if an OfflineStoreConfig
is provided.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM execution role used to persist data into the OfflineStore
* if an OfflineStoreConfig
is provided.
*
*
* @return The Amazon Resource Name (ARN) of the IAM execution role used to persist data into the
* OfflineStore
if an OfflineStoreConfig
is provided.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM execution role used to persist data into the OfflineStore
* if an OfflineStoreConfig
is provided.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM execution role used to persist data into the
* OfflineStore
if an OfflineStoreConfig
is provided.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* A free-form description of a FeatureGroup
.
*
*
* @param description
* A free-form description of a FeatureGroup
.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A free-form description of a FeatureGroup
.
*
*
* @return A free-form description of a FeatureGroup
.
*/
public String getDescription() {
return this.description;
}
/**
*
* A free-form description of a FeatureGroup
.
*
*
* @param description
* A free-form description of a FeatureGroup
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Tags used to identify Features
in each FeatureGroup
.
*
*
* @return Tags used to identify Features
in each FeatureGroup
.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* Tags used to identify Features
in each FeatureGroup
.
*
*
* @param tags
* Tags used to identify Features
in each FeatureGroup
.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* Tags used to identify Features
in each FeatureGroup
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Tags used to identify Features
in each FeatureGroup
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Tags used to identify Features
in each FeatureGroup
.
*
*
* @param tags
* Tags used to identify Features
in each FeatureGroup
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFeatureGroupRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFeatureGroupName() != null)
sb.append("FeatureGroupName: ").append(getFeatureGroupName()).append(",");
if (getRecordIdentifierFeatureName() != null)
sb.append("RecordIdentifierFeatureName: ").append(getRecordIdentifierFeatureName()).append(",");
if (getEventTimeFeatureName() != null)
sb.append("EventTimeFeatureName: ").append(getEventTimeFeatureName()).append(",");
if (getFeatureDefinitions() != null)
sb.append("FeatureDefinitions: ").append(getFeatureDefinitions()).append(",");
if (getOnlineStoreConfig() != null)
sb.append("OnlineStoreConfig: ").append(getOnlineStoreConfig()).append(",");
if (getOfflineStoreConfig() != null)
sb.append("OfflineStoreConfig: ").append(getOfflineStoreConfig()).append(",");
if (getThroughputConfig() != null)
sb.append("ThroughputConfig: ").append(getThroughputConfig()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateFeatureGroupRequest == false)
return false;
CreateFeatureGroupRequest other = (CreateFeatureGroupRequest) obj;
if (other.getFeatureGroupName() == null ^ this.getFeatureGroupName() == null)
return false;
if (other.getFeatureGroupName() != null && other.getFeatureGroupName().equals(this.getFeatureGroupName()) == false)
return false;
if (other.getRecordIdentifierFeatureName() == null ^ this.getRecordIdentifierFeatureName() == null)
return false;
if (other.getRecordIdentifierFeatureName() != null && other.getRecordIdentifierFeatureName().equals(this.getRecordIdentifierFeatureName()) == false)
return false;
if (other.getEventTimeFeatureName() == null ^ this.getEventTimeFeatureName() == null)
return false;
if (other.getEventTimeFeatureName() != null && other.getEventTimeFeatureName().equals(this.getEventTimeFeatureName()) == false)
return false;
if (other.getFeatureDefinitions() == null ^ this.getFeatureDefinitions() == null)
return false;
if (other.getFeatureDefinitions() != null && other.getFeatureDefinitions().equals(this.getFeatureDefinitions()) == false)
return false;
if (other.getOnlineStoreConfig() == null ^ this.getOnlineStoreConfig() == null)
return false;
if (other.getOnlineStoreConfig() != null && other.getOnlineStoreConfig().equals(this.getOnlineStoreConfig()) == false)
return false;
if (other.getOfflineStoreConfig() == null ^ this.getOfflineStoreConfig() == null)
return false;
if (other.getOfflineStoreConfig() != null && other.getOfflineStoreConfig().equals(this.getOfflineStoreConfig()) == false)
return false;
if (other.getThroughputConfig() == null ^ this.getThroughputConfig() == null)
return false;
if (other.getThroughputConfig() != null && other.getThroughputConfig().equals(this.getThroughputConfig()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFeatureGroupName() == null) ? 0 : getFeatureGroupName().hashCode());
hashCode = prime * hashCode + ((getRecordIdentifierFeatureName() == null) ? 0 : getRecordIdentifierFeatureName().hashCode());
hashCode = prime * hashCode + ((getEventTimeFeatureName() == null) ? 0 : getEventTimeFeatureName().hashCode());
hashCode = prime * hashCode + ((getFeatureDefinitions() == null) ? 0 : getFeatureDefinitions().hashCode());
hashCode = prime * hashCode + ((getOnlineStoreConfig() == null) ? 0 : getOnlineStoreConfig().hashCode());
hashCode = prime * hashCode + ((getOfflineStoreConfig() == null) ? 0 : getOfflineStoreConfig().hashCode());
hashCode = prime * hashCode + ((getThroughputConfig() == null) ? 0 : getThroughputConfig().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateFeatureGroupRequest clone() {
return (CreateFeatureGroupRequest) super.clone();
}
}