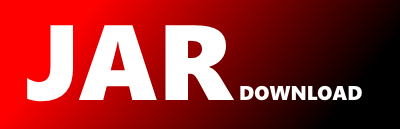
com.amazonaws.services.sagemaker.model.CreateFlowDefinitionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateFlowDefinitionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of your flow definition.
*
*/
private String flowDefinitionName;
/**
*
* Container for configuring the source of human task requests. Use to specify if Amazon Rekognition or Amazon
* Textract is used as an integration source.
*
*/
private HumanLoopRequestSource humanLoopRequestSource;
/**
*
* An object containing information about the events that trigger a human workflow.
*
*/
private HumanLoopActivationConfig humanLoopActivationConfig;
/**
*
* An object containing information about the tasks the human reviewers will perform.
*
*/
private HumanLoopConfig humanLoopConfig;
/**
*
* An object containing information about where the human review results will be uploaded.
*
*/
private FlowDefinitionOutputConfig outputConfig;
/**
*
* The Amazon Resource Name (ARN) of the role needed to call other services on your behalf. For example,
* arn:aws:iam::1234567890:role/service-role/AmazonSageMaker-ExecutionRole-20180111T151298
.
*
*/
private String roleArn;
/**
*
* An array of key-value pairs that contain metadata to help you categorize and organize a flow definition. Each tag
* consists of a key and a value, both of which you define.
*
*/
private java.util.List tags;
/**
*
* The name of your flow definition.
*
*
* @param flowDefinitionName
* The name of your flow definition.
*/
public void setFlowDefinitionName(String flowDefinitionName) {
this.flowDefinitionName = flowDefinitionName;
}
/**
*
* The name of your flow definition.
*
*
* @return The name of your flow definition.
*/
public String getFlowDefinitionName() {
return this.flowDefinitionName;
}
/**
*
* The name of your flow definition.
*
*
* @param flowDefinitionName
* The name of your flow definition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFlowDefinitionRequest withFlowDefinitionName(String flowDefinitionName) {
setFlowDefinitionName(flowDefinitionName);
return this;
}
/**
*
* Container for configuring the source of human task requests. Use to specify if Amazon Rekognition or Amazon
* Textract is used as an integration source.
*
*
* @param humanLoopRequestSource
* Container for configuring the source of human task requests. Use to specify if Amazon Rekognition or
* Amazon Textract is used as an integration source.
*/
public void setHumanLoopRequestSource(HumanLoopRequestSource humanLoopRequestSource) {
this.humanLoopRequestSource = humanLoopRequestSource;
}
/**
*
* Container for configuring the source of human task requests. Use to specify if Amazon Rekognition or Amazon
* Textract is used as an integration source.
*
*
* @return Container for configuring the source of human task requests. Use to specify if Amazon Rekognition or
* Amazon Textract is used as an integration source.
*/
public HumanLoopRequestSource getHumanLoopRequestSource() {
return this.humanLoopRequestSource;
}
/**
*
* Container for configuring the source of human task requests. Use to specify if Amazon Rekognition or Amazon
* Textract is used as an integration source.
*
*
* @param humanLoopRequestSource
* Container for configuring the source of human task requests. Use to specify if Amazon Rekognition or
* Amazon Textract is used as an integration source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFlowDefinitionRequest withHumanLoopRequestSource(HumanLoopRequestSource humanLoopRequestSource) {
setHumanLoopRequestSource(humanLoopRequestSource);
return this;
}
/**
*
* An object containing information about the events that trigger a human workflow.
*
*
* @param humanLoopActivationConfig
* An object containing information about the events that trigger a human workflow.
*/
public void setHumanLoopActivationConfig(HumanLoopActivationConfig humanLoopActivationConfig) {
this.humanLoopActivationConfig = humanLoopActivationConfig;
}
/**
*
* An object containing information about the events that trigger a human workflow.
*
*
* @return An object containing information about the events that trigger a human workflow.
*/
public HumanLoopActivationConfig getHumanLoopActivationConfig() {
return this.humanLoopActivationConfig;
}
/**
*
* An object containing information about the events that trigger a human workflow.
*
*
* @param humanLoopActivationConfig
* An object containing information about the events that trigger a human workflow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFlowDefinitionRequest withHumanLoopActivationConfig(HumanLoopActivationConfig humanLoopActivationConfig) {
setHumanLoopActivationConfig(humanLoopActivationConfig);
return this;
}
/**
*
* An object containing information about the tasks the human reviewers will perform.
*
*
* @param humanLoopConfig
* An object containing information about the tasks the human reviewers will perform.
*/
public void setHumanLoopConfig(HumanLoopConfig humanLoopConfig) {
this.humanLoopConfig = humanLoopConfig;
}
/**
*
* An object containing information about the tasks the human reviewers will perform.
*
*
* @return An object containing information about the tasks the human reviewers will perform.
*/
public HumanLoopConfig getHumanLoopConfig() {
return this.humanLoopConfig;
}
/**
*
* An object containing information about the tasks the human reviewers will perform.
*
*
* @param humanLoopConfig
* An object containing information about the tasks the human reviewers will perform.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFlowDefinitionRequest withHumanLoopConfig(HumanLoopConfig humanLoopConfig) {
setHumanLoopConfig(humanLoopConfig);
return this;
}
/**
*
* An object containing information about where the human review results will be uploaded.
*
*
* @param outputConfig
* An object containing information about where the human review results will be uploaded.
*/
public void setOutputConfig(FlowDefinitionOutputConfig outputConfig) {
this.outputConfig = outputConfig;
}
/**
*
* An object containing information about where the human review results will be uploaded.
*
*
* @return An object containing information about where the human review results will be uploaded.
*/
public FlowDefinitionOutputConfig getOutputConfig() {
return this.outputConfig;
}
/**
*
* An object containing information about where the human review results will be uploaded.
*
*
* @param outputConfig
* An object containing information about where the human review results will be uploaded.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFlowDefinitionRequest withOutputConfig(FlowDefinitionOutputConfig outputConfig) {
setOutputConfig(outputConfig);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the role needed to call other services on your behalf. For example,
* arn:aws:iam::1234567890:role/service-role/AmazonSageMaker-ExecutionRole-20180111T151298
.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the role needed to call other services on your behalf. For example,
* arn:aws:iam::1234567890:role/service-role/AmazonSageMaker-ExecutionRole-20180111T151298
.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the role needed to call other services on your behalf. For example,
* arn:aws:iam::1234567890:role/service-role/AmazonSageMaker-ExecutionRole-20180111T151298
.
*
*
* @return The Amazon Resource Name (ARN) of the role needed to call other services on your behalf. For example,
* arn:aws:iam::1234567890:role/service-role/AmazonSageMaker-ExecutionRole-20180111T151298
.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the role needed to call other services on your behalf. For example,
* arn:aws:iam::1234567890:role/service-role/AmazonSageMaker-ExecutionRole-20180111T151298
.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the role needed to call other services on your behalf. For example,
* arn:aws:iam::1234567890:role/service-role/AmazonSageMaker-ExecutionRole-20180111T151298
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFlowDefinitionRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* An array of key-value pairs that contain metadata to help you categorize and organize a flow definition. Each tag
* consists of a key and a value, both of which you define.
*
*
* @return An array of key-value pairs that contain metadata to help you categorize and organize a flow definition.
* Each tag consists of a key and a value, both of which you define.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of key-value pairs that contain metadata to help you categorize and organize a flow definition. Each tag
* consists of a key and a value, both of which you define.
*
*
* @param tags
* An array of key-value pairs that contain metadata to help you categorize and organize a flow definition.
* Each tag consists of a key and a value, both of which you define.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of key-value pairs that contain metadata to help you categorize and organize a flow definition. Each tag
* consists of a key and a value, both of which you define.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of key-value pairs that contain metadata to help you categorize and organize a flow definition.
* Each tag consists of a key and a value, both of which you define.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFlowDefinitionRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of key-value pairs that contain metadata to help you categorize and organize a flow definition. Each tag
* consists of a key and a value, both of which you define.
*
*
* @param tags
* An array of key-value pairs that contain metadata to help you categorize and organize a flow definition.
* Each tag consists of a key and a value, both of which you define.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateFlowDefinitionRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFlowDefinitionName() != null)
sb.append("FlowDefinitionName: ").append(getFlowDefinitionName()).append(",");
if (getHumanLoopRequestSource() != null)
sb.append("HumanLoopRequestSource: ").append(getHumanLoopRequestSource()).append(",");
if (getHumanLoopActivationConfig() != null)
sb.append("HumanLoopActivationConfig: ").append(getHumanLoopActivationConfig()).append(",");
if (getHumanLoopConfig() != null)
sb.append("HumanLoopConfig: ").append(getHumanLoopConfig()).append(",");
if (getOutputConfig() != null)
sb.append("OutputConfig: ").append(getOutputConfig()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateFlowDefinitionRequest == false)
return false;
CreateFlowDefinitionRequest other = (CreateFlowDefinitionRequest) obj;
if (other.getFlowDefinitionName() == null ^ this.getFlowDefinitionName() == null)
return false;
if (other.getFlowDefinitionName() != null && other.getFlowDefinitionName().equals(this.getFlowDefinitionName()) == false)
return false;
if (other.getHumanLoopRequestSource() == null ^ this.getHumanLoopRequestSource() == null)
return false;
if (other.getHumanLoopRequestSource() != null && other.getHumanLoopRequestSource().equals(this.getHumanLoopRequestSource()) == false)
return false;
if (other.getHumanLoopActivationConfig() == null ^ this.getHumanLoopActivationConfig() == null)
return false;
if (other.getHumanLoopActivationConfig() != null && other.getHumanLoopActivationConfig().equals(this.getHumanLoopActivationConfig()) == false)
return false;
if (other.getHumanLoopConfig() == null ^ this.getHumanLoopConfig() == null)
return false;
if (other.getHumanLoopConfig() != null && other.getHumanLoopConfig().equals(this.getHumanLoopConfig()) == false)
return false;
if (other.getOutputConfig() == null ^ this.getOutputConfig() == null)
return false;
if (other.getOutputConfig() != null && other.getOutputConfig().equals(this.getOutputConfig()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFlowDefinitionName() == null) ? 0 : getFlowDefinitionName().hashCode());
hashCode = prime * hashCode + ((getHumanLoopRequestSource() == null) ? 0 : getHumanLoopRequestSource().hashCode());
hashCode = prime * hashCode + ((getHumanLoopActivationConfig() == null) ? 0 : getHumanLoopActivationConfig().hashCode());
hashCode = prime * hashCode + ((getHumanLoopConfig() == null) ? 0 : getHumanLoopConfig().hashCode());
hashCode = prime * hashCode + ((getOutputConfig() == null) ? 0 : getOutputConfig().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateFlowDefinitionRequest clone() {
return (CreateFlowDefinitionRequest) super.clone();
}
}