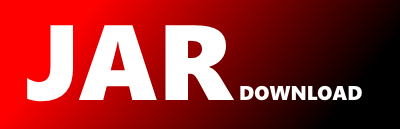
com.amazonaws.services.sagemaker.model.CreateModelRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateModelRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the new model.
*
*/
private String modelName;
/**
*
* The location of the primary docker image containing inference code, associated artifacts, and custom environment
* map that the inference code uses when the model is deployed for predictions.
*
*/
private ContainerDefinition primaryContainer;
/**
*
* Specifies the containers in the inference pipeline.
*
*/
private java.util.List containers;
/**
*
* Specifies details of how containers in a multi-container endpoint are called.
*
*/
private InferenceExecutionConfig inferenceExecutionConfig;
/**
*
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access model artifacts and docker
* image for deployment on ML compute instances or for batch transform jobs. Deploying on ML compute instances is
* part of model hosting. For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*/
private String executionRoleArn;
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*/
private java.util.List tags;
/**
*
* A VpcConfig object
* that specifies the VPC that you want your model to connect to. Control access to and from your model container by
* configuring the VPC. VpcConfig
is used in hosting services and in batch transform. For more
* information, see Protect Endpoints by
* Using an Amazon Virtual Private Cloud and Protect Data in Batch Transform Jobs by
* Using an Amazon Virtual Private Cloud.
*
*/
private VpcConfig vpcConfig;
/**
*
* Isolates the model container. No inbound or outbound network calls can be made to or from the model container.
*
*/
private Boolean enableNetworkIsolation;
/**
*
* The name of the new model.
*
*
* @param modelName
* The name of the new model.
*/
public void setModelName(String modelName) {
this.modelName = modelName;
}
/**
*
* The name of the new model.
*
*
* @return The name of the new model.
*/
public String getModelName() {
return this.modelName;
}
/**
*
* The name of the new model.
*
*
* @param modelName
* The name of the new model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withModelName(String modelName) {
setModelName(modelName);
return this;
}
/**
*
* The location of the primary docker image containing inference code, associated artifacts, and custom environment
* map that the inference code uses when the model is deployed for predictions.
*
*
* @param primaryContainer
* The location of the primary docker image containing inference code, associated artifacts, and custom
* environment map that the inference code uses when the model is deployed for predictions.
*/
public void setPrimaryContainer(ContainerDefinition primaryContainer) {
this.primaryContainer = primaryContainer;
}
/**
*
* The location of the primary docker image containing inference code, associated artifacts, and custom environment
* map that the inference code uses when the model is deployed for predictions.
*
*
* @return The location of the primary docker image containing inference code, associated artifacts, and custom
* environment map that the inference code uses when the model is deployed for predictions.
*/
public ContainerDefinition getPrimaryContainer() {
return this.primaryContainer;
}
/**
*
* The location of the primary docker image containing inference code, associated artifacts, and custom environment
* map that the inference code uses when the model is deployed for predictions.
*
*
* @param primaryContainer
* The location of the primary docker image containing inference code, associated artifacts, and custom
* environment map that the inference code uses when the model is deployed for predictions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withPrimaryContainer(ContainerDefinition primaryContainer) {
setPrimaryContainer(primaryContainer);
return this;
}
/**
*
* Specifies the containers in the inference pipeline.
*
*
* @return Specifies the containers in the inference pipeline.
*/
public java.util.List getContainers() {
return containers;
}
/**
*
* Specifies the containers in the inference pipeline.
*
*
* @param containers
* Specifies the containers in the inference pipeline.
*/
public void setContainers(java.util.Collection containers) {
if (containers == null) {
this.containers = null;
return;
}
this.containers = new java.util.ArrayList(containers);
}
/**
*
* Specifies the containers in the inference pipeline.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setContainers(java.util.Collection)} or {@link #withContainers(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param containers
* Specifies the containers in the inference pipeline.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withContainers(ContainerDefinition... containers) {
if (this.containers == null) {
setContainers(new java.util.ArrayList(containers.length));
}
for (ContainerDefinition ele : containers) {
this.containers.add(ele);
}
return this;
}
/**
*
* Specifies the containers in the inference pipeline.
*
*
* @param containers
* Specifies the containers in the inference pipeline.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withContainers(java.util.Collection containers) {
setContainers(containers);
return this;
}
/**
*
* Specifies details of how containers in a multi-container endpoint are called.
*
*
* @param inferenceExecutionConfig
* Specifies details of how containers in a multi-container endpoint are called.
*/
public void setInferenceExecutionConfig(InferenceExecutionConfig inferenceExecutionConfig) {
this.inferenceExecutionConfig = inferenceExecutionConfig;
}
/**
*
* Specifies details of how containers in a multi-container endpoint are called.
*
*
* @return Specifies details of how containers in a multi-container endpoint are called.
*/
public InferenceExecutionConfig getInferenceExecutionConfig() {
return this.inferenceExecutionConfig;
}
/**
*
* Specifies details of how containers in a multi-container endpoint are called.
*
*
* @param inferenceExecutionConfig
* Specifies details of how containers in a multi-container endpoint are called.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withInferenceExecutionConfig(InferenceExecutionConfig inferenceExecutionConfig) {
setInferenceExecutionConfig(inferenceExecutionConfig);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access model artifacts and docker
* image for deployment on ML compute instances or for batch transform jobs. Deploying on ML compute instances is
* part of model hosting. For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access model artifacts and
* docker image for deployment on ML compute instances or for batch transform jobs. Deploying on ML compute
* instances is part of model hosting. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access model artifacts and docker
* image for deployment on ML compute instances or for batch transform jobs. Deploying on ML compute instances is
* part of model hosting. For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*
* @return The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access model artifacts and
* docker image for deployment on ML compute instances or for batch transform jobs. Deploying on ML compute
* instances is part of model hosting. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access model artifacts and docker
* image for deployment on ML compute instances or for batch transform jobs. Deploying on ML compute instances is
* part of model hosting. For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access model artifacts and
* docker image for deployment on ML compute instances or for batch transform jobs. Deploying on ML compute
* instances is part of model hosting. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @return An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* A VpcConfig object
* that specifies the VPC that you want your model to connect to. Control access to and from your model container by
* configuring the VPC. VpcConfig
is used in hosting services and in batch transform. For more
* information, see Protect Endpoints by
* Using an Amazon Virtual Private Cloud and Protect Data in Batch Transform Jobs by
* Using an Amazon Virtual Private Cloud.
*
*
* @param vpcConfig
* A VpcConfig
* object that specifies the VPC that you want your model to connect to. Control access to and from your
* model container by configuring the VPC. VpcConfig
is used in hosting services and in batch
* transform. For more information, see Protect Endpoints by Using an Amazon
* Virtual Private Cloud and Protect Data in Batch Transform Jobs
* by Using an Amazon Virtual Private Cloud.
*/
public void setVpcConfig(VpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
*
* A VpcConfig object
* that specifies the VPC that you want your model to connect to. Control access to and from your model container by
* configuring the VPC. VpcConfig
is used in hosting services and in batch transform. For more
* information, see Protect Endpoints by
* Using an Amazon Virtual Private Cloud and Protect Data in Batch Transform Jobs by
* Using an Amazon Virtual Private Cloud.
*
*
* @return A VpcConfig
* object that specifies the VPC that you want your model to connect to. Control access to and from your
* model container by configuring the VPC. VpcConfig
is used in hosting services and in batch
* transform. For more information, see Protect Endpoints by Using an Amazon
* Virtual Private Cloud and Protect Data in Batch Transform
* Jobs by Using an Amazon Virtual Private Cloud.
*/
public VpcConfig getVpcConfig() {
return this.vpcConfig;
}
/**
*
* A VpcConfig object
* that specifies the VPC that you want your model to connect to. Control access to and from your model container by
* configuring the VPC. VpcConfig
is used in hosting services and in batch transform. For more
* information, see Protect Endpoints by
* Using an Amazon Virtual Private Cloud and Protect Data in Batch Transform Jobs by
* Using an Amazon Virtual Private Cloud.
*
*
* @param vpcConfig
* A VpcConfig
* object that specifies the VPC that you want your model to connect to. Control access to and from your
* model container by configuring the VPC. VpcConfig
is used in hosting services and in batch
* transform. For more information, see Protect Endpoints by Using an Amazon
* Virtual Private Cloud and Protect Data in Batch Transform Jobs
* by Using an Amazon Virtual Private Cloud.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withVpcConfig(VpcConfig vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
*
* Isolates the model container. No inbound or outbound network calls can be made to or from the model container.
*
*
* @param enableNetworkIsolation
* Isolates the model container. No inbound or outbound network calls can be made to or from the model
* container.
*/
public void setEnableNetworkIsolation(Boolean enableNetworkIsolation) {
this.enableNetworkIsolation = enableNetworkIsolation;
}
/**
*
* Isolates the model container. No inbound or outbound network calls can be made to or from the model container.
*
*
* @return Isolates the model container. No inbound or outbound network calls can be made to or from the model
* container.
*/
public Boolean getEnableNetworkIsolation() {
return this.enableNetworkIsolation;
}
/**
*
* Isolates the model container. No inbound or outbound network calls can be made to or from the model container.
*
*
* @param enableNetworkIsolation
* Isolates the model container. No inbound or outbound network calls can be made to or from the model
* container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateModelRequest withEnableNetworkIsolation(Boolean enableNetworkIsolation) {
setEnableNetworkIsolation(enableNetworkIsolation);
return this;
}
/**
*
* Isolates the model container. No inbound or outbound network calls can be made to or from the model container.
*
*
* @return Isolates the model container. No inbound or outbound network calls can be made to or from the model
* container.
*/
public Boolean isEnableNetworkIsolation() {
return this.enableNetworkIsolation;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getModelName() != null)
sb.append("ModelName: ").append(getModelName()).append(",");
if (getPrimaryContainer() != null)
sb.append("PrimaryContainer: ").append(getPrimaryContainer()).append(",");
if (getContainers() != null)
sb.append("Containers: ").append(getContainers()).append(",");
if (getInferenceExecutionConfig() != null)
sb.append("InferenceExecutionConfig: ").append(getInferenceExecutionConfig()).append(",");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getVpcConfig() != null)
sb.append("VpcConfig: ").append(getVpcConfig()).append(",");
if (getEnableNetworkIsolation() != null)
sb.append("EnableNetworkIsolation: ").append(getEnableNetworkIsolation());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateModelRequest == false)
return false;
CreateModelRequest other = (CreateModelRequest) obj;
if (other.getModelName() == null ^ this.getModelName() == null)
return false;
if (other.getModelName() != null && other.getModelName().equals(this.getModelName()) == false)
return false;
if (other.getPrimaryContainer() == null ^ this.getPrimaryContainer() == null)
return false;
if (other.getPrimaryContainer() != null && other.getPrimaryContainer().equals(this.getPrimaryContainer()) == false)
return false;
if (other.getContainers() == null ^ this.getContainers() == null)
return false;
if (other.getContainers() != null && other.getContainers().equals(this.getContainers()) == false)
return false;
if (other.getInferenceExecutionConfig() == null ^ this.getInferenceExecutionConfig() == null)
return false;
if (other.getInferenceExecutionConfig() != null && other.getInferenceExecutionConfig().equals(this.getInferenceExecutionConfig()) == false)
return false;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null && other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
if (other.getEnableNetworkIsolation() == null ^ this.getEnableNetworkIsolation() == null)
return false;
if (other.getEnableNetworkIsolation() != null && other.getEnableNetworkIsolation().equals(this.getEnableNetworkIsolation()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getModelName() == null) ? 0 : getModelName().hashCode());
hashCode = prime * hashCode + ((getPrimaryContainer() == null) ? 0 : getPrimaryContainer().hashCode());
hashCode = prime * hashCode + ((getContainers() == null) ? 0 : getContainers().hashCode());
hashCode = prime * hashCode + ((getInferenceExecutionConfig() == null) ? 0 : getInferenceExecutionConfig().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
hashCode = prime * hashCode + ((getEnableNetworkIsolation() == null) ? 0 : getEnableNetworkIsolation().hashCode());
return hashCode;
}
@Override
public CreateModelRequest clone() {
return (CreateModelRequest) super.clone();
}
}