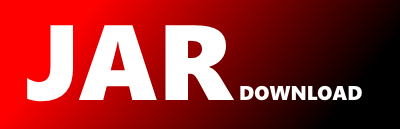
com.amazonaws.services.sagemaker.model.CreateNotebookInstanceRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateNotebookInstanceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the new notebook instance.
*
*/
private String notebookInstanceName;
/**
*
* The type of ML compute instance to launch for the notebook instance.
*
*/
private String instanceType;
/**
*
* The ID of the subnet in a VPC to which you would like to have a connectivity from your ML compute instance.
*
*/
private String subnetId;
/**
*
* The VPC security group IDs, in the form sg-xxxxxxxx. The security groups must be for the same VPC as specified in
* the subnet.
*
*/
private java.util.List securityGroupIds;
/**
*
* When you send any requests to Amazon Web Services resources from the notebook instance, SageMaker assumes this
* role to perform tasks on your behalf. You must grant this role necessary permissions so SageMaker can perform
* these tasks. The policy must allow the SageMaker service principal (sagemaker.amazonaws.com) permissions to
* assume this role. For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*/
private String roleArn;
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to encrypt
* data on the storage volume attached to your notebook instance. The KMS key you provide must be enabled. For
* information, see Enabling and
* Disabling Keys in the Amazon Web Services Key Management Service Developer Guide.
*
*/
private String kmsKeyId;
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*/
private java.util.List tags;
/**
*
* The name of a lifecycle configuration to associate with the notebook instance. For information about lifestyle
* configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance.
*
*/
private String lifecycleConfigName;
/**
*
* Sets whether SageMaker provides internet access to the notebook instance. If you set this to
* Disabled
this notebook instance is able to access resources only in your VPC, and is not be able to
* connect to SageMaker training and endpoint services unless you configure a NAT Gateway in your VPC.
*
*
* For more information, see Notebook Instances Are Internet-Enabled by Default. You can set the value of this parameter to
* Disabled
only if you set a value for the SubnetId
parameter.
*
*/
private String directInternetAccess;
/**
*
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
*
*/
private Integer volumeSizeInGB;
/**
*
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only one
* instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*/
private java.util.List acceleratorTypes;
/**
*
* A Git repository to associate with the notebook instance as its default code repository. This can be either the
* name of a Git repository stored as a resource in your account, or the URL of a Git repository in Amazon Web Services CodeCommit or
* in any other Git repository. When you open a notebook instance, it opens in the directory that contains this
* repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*/
private String defaultCodeRepository;
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*/
private java.util.List additionalCodeRepositories;
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* Lifecycle configurations need root access to be able to set up a notebook instance. Because of this, lifecycle
* configurations associated with a notebook instance always run with root access even if you disable root access
* for users.
*
*
*/
private String rootAccess;
/**
*
* The platform identifier of the notebook instance runtime environment.
*
*/
private String platformIdentifier;
/**
*
* Information on the IMDS configuration of the notebook instance
*
*/
private InstanceMetadataServiceConfiguration instanceMetadataServiceConfiguration;
/**
*
* The name of the new notebook instance.
*
*
* @param notebookInstanceName
* The name of the new notebook instance.
*/
public void setNotebookInstanceName(String notebookInstanceName) {
this.notebookInstanceName = notebookInstanceName;
}
/**
*
* The name of the new notebook instance.
*
*
* @return The name of the new notebook instance.
*/
public String getNotebookInstanceName() {
return this.notebookInstanceName;
}
/**
*
* The name of the new notebook instance.
*
*
* @param notebookInstanceName
* The name of the new notebook instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withNotebookInstanceName(String notebookInstanceName) {
setNotebookInstanceName(notebookInstanceName);
return this;
}
/**
*
* The type of ML compute instance to launch for the notebook instance.
*
*
* @param instanceType
* The type of ML compute instance to launch for the notebook instance.
* @see InstanceType
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
*
* The type of ML compute instance to launch for the notebook instance.
*
*
* @return The type of ML compute instance to launch for the notebook instance.
* @see InstanceType
*/
public String getInstanceType() {
return this.instanceType;
}
/**
*
* The type of ML compute instance to launch for the notebook instance.
*
*
* @param instanceType
* The type of ML compute instance to launch for the notebook instance.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public CreateNotebookInstanceRequest withInstanceType(String instanceType) {
setInstanceType(instanceType);
return this;
}
/**
*
* The type of ML compute instance to launch for the notebook instance.
*
*
* @param instanceType
* The type of ML compute instance to launch for the notebook instance.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public CreateNotebookInstanceRequest withInstanceType(InstanceType instanceType) {
this.instanceType = instanceType.toString();
return this;
}
/**
*
* The ID of the subnet in a VPC to which you would like to have a connectivity from your ML compute instance.
*
*
* @param subnetId
* The ID of the subnet in a VPC to which you would like to have a connectivity from your ML compute
* instance.
*/
public void setSubnetId(String subnetId) {
this.subnetId = subnetId;
}
/**
*
* The ID of the subnet in a VPC to which you would like to have a connectivity from your ML compute instance.
*
*
* @return The ID of the subnet in a VPC to which you would like to have a connectivity from your ML compute
* instance.
*/
public String getSubnetId() {
return this.subnetId;
}
/**
*
* The ID of the subnet in a VPC to which you would like to have a connectivity from your ML compute instance.
*
*
* @param subnetId
* The ID of the subnet in a VPC to which you would like to have a connectivity from your ML compute
* instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withSubnetId(String subnetId) {
setSubnetId(subnetId);
return this;
}
/**
*
* The VPC security group IDs, in the form sg-xxxxxxxx. The security groups must be for the same VPC as specified in
* the subnet.
*
*
* @return The VPC security group IDs, in the form sg-xxxxxxxx. The security groups must be for the same VPC as
* specified in the subnet.
*/
public java.util.List getSecurityGroupIds() {
return securityGroupIds;
}
/**
*
* The VPC security group IDs, in the form sg-xxxxxxxx. The security groups must be for the same VPC as specified in
* the subnet.
*
*
* @param securityGroupIds
* The VPC security group IDs, in the form sg-xxxxxxxx. The security groups must be for the same VPC as
* specified in the subnet.
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
this.securityGroupIds = new java.util.ArrayList(securityGroupIds);
}
/**
*
* The VPC security group IDs, in the form sg-xxxxxxxx. The security groups must be for the same VPC as specified in
* the subnet.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroupIds(java.util.Collection)} or {@link #withSecurityGroupIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param securityGroupIds
* The VPC security group IDs, in the form sg-xxxxxxxx. The security groups must be for the same VPC as
* specified in the subnet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withSecurityGroupIds(String... securityGroupIds) {
if (this.securityGroupIds == null) {
setSecurityGroupIds(new java.util.ArrayList(securityGroupIds.length));
}
for (String ele : securityGroupIds) {
this.securityGroupIds.add(ele);
}
return this;
}
/**
*
* The VPC security group IDs, in the form sg-xxxxxxxx. The security groups must be for the same VPC as specified in
* the subnet.
*
*
* @param securityGroupIds
* The VPC security group IDs, in the form sg-xxxxxxxx. The security groups must be for the same VPC as
* specified in the subnet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withSecurityGroupIds(java.util.Collection securityGroupIds) {
setSecurityGroupIds(securityGroupIds);
return this;
}
/**
*
* When you send any requests to Amazon Web Services resources from the notebook instance, SageMaker assumes this
* role to perform tasks on your behalf. You must grant this role necessary permissions so SageMaker can perform
* these tasks. The policy must allow the SageMaker service principal (sagemaker.amazonaws.com) permissions to
* assume this role. For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*
* @param roleArn
* When you send any requests to Amazon Web Services resources from the notebook instance, SageMaker assumes
* this role to perform tasks on your behalf. You must grant this role necessary permissions so SageMaker can
* perform these tasks. The policy must allow the SageMaker service principal (sagemaker.amazonaws.com)
* permissions to assume this role. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* When you send any requests to Amazon Web Services resources from the notebook instance, SageMaker assumes this
* role to perform tasks on your behalf. You must grant this role necessary permissions so SageMaker can perform
* these tasks. The policy must allow the SageMaker service principal (sagemaker.amazonaws.com) permissions to
* assume this role. For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*
* @return When you send any requests to Amazon Web Services resources from the notebook instance, SageMaker assumes
* this role to perform tasks on your behalf. You must grant this role necessary permissions so SageMaker
* can perform these tasks. The policy must allow the SageMaker service principal (sagemaker.amazonaws.com)
* permissions to assume this role. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* When you send any requests to Amazon Web Services resources from the notebook instance, SageMaker assumes this
* role to perform tasks on your behalf. You must grant this role necessary permissions so SageMaker can perform
* these tasks. The policy must allow the SageMaker service principal (sagemaker.amazonaws.com) permissions to
* assume this role. For more information, see SageMaker Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*
* @param roleArn
* When you send any requests to Amazon Web Services resources from the notebook instance, SageMaker assumes
* this role to perform tasks on your behalf. You must grant this role necessary permissions so SageMaker can
* perform these tasks. The policy must allow the SageMaker service principal (sagemaker.amazonaws.com)
* permissions to assume this role. For more information, see SageMaker Roles.
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to encrypt
* data on the storage volume attached to your notebook instance. The KMS key you provide must be enabled. For
* information, see Enabling and
* Disabling Keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* @param kmsKeyId
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to
* encrypt data on the storage volume attached to your notebook instance. The KMS key you provide must be
* enabled. For information, see Enabling and Disabling
* Keys in the Amazon Web Services Key Management Service Developer Guide.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to encrypt
* data on the storage volume attached to your notebook instance. The KMS key you provide must be enabled. For
* information, see Enabling and
* Disabling Keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to
* encrypt data on the storage volume attached to your notebook instance. The KMS key you provide must be
* enabled. For information, see Enabling and Disabling
* Keys in the Amazon Web Services Key Management Service Developer Guide.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to encrypt
* data on the storage volume attached to your notebook instance. The KMS key you provide must be enabled. For
* information, see Enabling and
* Disabling Keys in the Amazon Web Services Key Management Service Developer Guide.
*
*
* @param kmsKeyId
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service key that SageMaker uses to
* encrypt data on the storage volume attached to your notebook instance. The KMS key you provide must be
* enabled. For information, see Enabling and Disabling
* Keys in the Amazon Web Services Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @return An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in different ways,
* for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services Resources.
*
*
* @param tags
* An array of key-value pairs. You can use tags to categorize your Amazon Web Services resources in
* different ways, for example, by purpose, owner, or environment. For more information, see Tagging Amazon Web Services
* Resources.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The name of a lifecycle configuration to associate with the notebook instance. For information about lifestyle
* configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance.
*
*
* @param lifecycleConfigName
* The name of a lifecycle configuration to associate with the notebook instance. For information about
* lifestyle configurations, see Step 2.1: (Optional)
* Customize a Notebook Instance.
*/
public void setLifecycleConfigName(String lifecycleConfigName) {
this.lifecycleConfigName = lifecycleConfigName;
}
/**
*
* The name of a lifecycle configuration to associate with the notebook instance. For information about lifestyle
* configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance.
*
*
* @return The name of a lifecycle configuration to associate with the notebook instance. For information about
* lifestyle configurations, see Step 2.1:
* (Optional) Customize a Notebook Instance.
*/
public String getLifecycleConfigName() {
return this.lifecycleConfigName;
}
/**
*
* The name of a lifecycle configuration to associate with the notebook instance. For information about lifestyle
* configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance.
*
*
* @param lifecycleConfigName
* The name of a lifecycle configuration to associate with the notebook instance. For information about
* lifestyle configurations, see Step 2.1: (Optional)
* Customize a Notebook Instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withLifecycleConfigName(String lifecycleConfigName) {
setLifecycleConfigName(lifecycleConfigName);
return this;
}
/**
*
* Sets whether SageMaker provides internet access to the notebook instance. If you set this to
* Disabled
this notebook instance is able to access resources only in your VPC, and is not be able to
* connect to SageMaker training and endpoint services unless you configure a NAT Gateway in your VPC.
*
*
* For more information, see Notebook Instances Are Internet-Enabled by Default. You can set the value of this parameter to
* Disabled
only if you set a value for the SubnetId
parameter.
*
*
* @param directInternetAccess
* Sets whether SageMaker provides internet access to the notebook instance. If you set this to
* Disabled
this notebook instance is able to access resources only in your VPC, and is not be
* able to connect to SageMaker training and endpoint services unless you configure a NAT Gateway in your
* VPC.
*
* For more information, see Notebook Instances Are Internet-Enabled by Default. You can set the value of this parameter to
* Disabled
only if you set a value for the SubnetId
parameter.
* @see DirectInternetAccess
*/
public void setDirectInternetAccess(String directInternetAccess) {
this.directInternetAccess = directInternetAccess;
}
/**
*
* Sets whether SageMaker provides internet access to the notebook instance. If you set this to
* Disabled
this notebook instance is able to access resources only in your VPC, and is not be able to
* connect to SageMaker training and endpoint services unless you configure a NAT Gateway in your VPC.
*
*
* For more information, see Notebook Instances Are Internet-Enabled by Default. You can set the value of this parameter to
* Disabled
only if you set a value for the SubnetId
parameter.
*
*
* @return Sets whether SageMaker provides internet access to the notebook instance. If you set this to
* Disabled
this notebook instance is able to access resources only in your VPC, and is not be
* able to connect to SageMaker training and endpoint services unless you configure a NAT Gateway in your
* VPC.
*
* For more information, see Notebook Instances Are Internet-Enabled by Default. You can set the value of this parameter to
* Disabled
only if you set a value for the SubnetId
parameter.
* @see DirectInternetAccess
*/
public String getDirectInternetAccess() {
return this.directInternetAccess;
}
/**
*
* Sets whether SageMaker provides internet access to the notebook instance. If you set this to
* Disabled
this notebook instance is able to access resources only in your VPC, and is not be able to
* connect to SageMaker training and endpoint services unless you configure a NAT Gateway in your VPC.
*
*
* For more information, see Notebook Instances Are Internet-Enabled by Default. You can set the value of this parameter to
* Disabled
only if you set a value for the SubnetId
parameter.
*
*
* @param directInternetAccess
* Sets whether SageMaker provides internet access to the notebook instance. If you set this to
* Disabled
this notebook instance is able to access resources only in your VPC, and is not be
* able to connect to SageMaker training and endpoint services unless you configure a NAT Gateway in your
* VPC.
*
* For more information, see Notebook Instances Are Internet-Enabled by Default. You can set the value of this parameter to
* Disabled
only if you set a value for the SubnetId
parameter.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DirectInternetAccess
*/
public CreateNotebookInstanceRequest withDirectInternetAccess(String directInternetAccess) {
setDirectInternetAccess(directInternetAccess);
return this;
}
/**
*
* Sets whether SageMaker provides internet access to the notebook instance. If you set this to
* Disabled
this notebook instance is able to access resources only in your VPC, and is not be able to
* connect to SageMaker training and endpoint services unless you configure a NAT Gateway in your VPC.
*
*
* For more information, see Notebook Instances Are Internet-Enabled by Default. You can set the value of this parameter to
* Disabled
only if you set a value for the SubnetId
parameter.
*
*
* @param directInternetAccess
* Sets whether SageMaker provides internet access to the notebook instance. If you set this to
* Disabled
this notebook instance is able to access resources only in your VPC, and is not be
* able to connect to SageMaker training and endpoint services unless you configure a NAT Gateway in your
* VPC.
*
* For more information, see Notebook Instances Are Internet-Enabled by Default. You can set the value of this parameter to
* Disabled
only if you set a value for the SubnetId
parameter.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DirectInternetAccess
*/
public CreateNotebookInstanceRequest withDirectInternetAccess(DirectInternetAccess directInternetAccess) {
this.directInternetAccess = directInternetAccess.toString();
return this;
}
/**
*
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
*
*
* @param volumeSizeInGB
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
*/
public void setVolumeSizeInGB(Integer volumeSizeInGB) {
this.volumeSizeInGB = volumeSizeInGB;
}
/**
*
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
*
*
* @return The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
*/
public Integer getVolumeSizeInGB() {
return this.volumeSizeInGB;
}
/**
*
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
*
*
* @param volumeSizeInGB
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withVolumeSizeInGB(Integer volumeSizeInGB) {
setVolumeSizeInGB(volumeSizeInGB);
return this;
}
/**
*
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only one
* instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @return A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only
* one instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see NotebookInstanceAcceleratorType
*/
public java.util.List getAcceleratorTypes() {
return acceleratorTypes;
}
/**
*
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only one
* instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorTypes
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only
* one instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see NotebookInstanceAcceleratorType
*/
public void setAcceleratorTypes(java.util.Collection acceleratorTypes) {
if (acceleratorTypes == null) {
this.acceleratorTypes = null;
return;
}
this.acceleratorTypes = new java.util.ArrayList(acceleratorTypes);
}
/**
*
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only one
* instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAcceleratorTypes(java.util.Collection)} or {@link #withAcceleratorTypes(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param acceleratorTypes
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only
* one instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotebookInstanceAcceleratorType
*/
public CreateNotebookInstanceRequest withAcceleratorTypes(String... acceleratorTypes) {
if (this.acceleratorTypes == null) {
setAcceleratorTypes(new java.util.ArrayList(acceleratorTypes.length));
}
for (String ele : acceleratorTypes) {
this.acceleratorTypes.add(ele);
}
return this;
}
/**
*
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only one
* instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorTypes
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only
* one instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotebookInstanceAcceleratorType
*/
public CreateNotebookInstanceRequest withAcceleratorTypes(java.util.Collection acceleratorTypes) {
setAcceleratorTypes(acceleratorTypes);
return this;
}
/**
*
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only one
* instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorTypes
* A list of Elastic Inference (EI) instance types to associate with this notebook instance. Currently, only
* one instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotebookInstanceAcceleratorType
*/
public CreateNotebookInstanceRequest withAcceleratorTypes(NotebookInstanceAcceleratorType... acceleratorTypes) {
java.util.ArrayList acceleratorTypesCopy = new java.util.ArrayList(acceleratorTypes.length);
for (NotebookInstanceAcceleratorType value : acceleratorTypes) {
acceleratorTypesCopy.add(value.toString());
}
if (getAcceleratorTypes() == null) {
setAcceleratorTypes(acceleratorTypesCopy);
} else {
getAcceleratorTypes().addAll(acceleratorTypesCopy);
}
return this;
}
/**
*
* A Git repository to associate with the notebook instance as its default code repository. This can be either the
* name of a Git repository stored as a resource in your account, or the URL of a Git repository in Amazon Web Services CodeCommit or
* in any other Git repository. When you open a notebook instance, it opens in the directory that contains this
* repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @param defaultCodeRepository
* A Git repository to associate with the notebook instance as its default code repository. This can be
* either the name of a Git repository stored as a resource in your account, or the URL of a Git repository
* in Amazon Web Services
* CodeCommit or in any other Git repository. When you open a notebook instance, it opens in the
* directory that contains this repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*/
public void setDefaultCodeRepository(String defaultCodeRepository) {
this.defaultCodeRepository = defaultCodeRepository;
}
/**
*
* A Git repository to associate with the notebook instance as its default code repository. This can be either the
* name of a Git repository stored as a resource in your account, or the URL of a Git repository in Amazon Web Services CodeCommit or
* in any other Git repository. When you open a notebook instance, it opens in the directory that contains this
* repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @return A Git repository to associate with the notebook instance as its default code repository. This can be
* either the name of a Git repository stored as a resource in your account, or the URL of a Git repository
* in Amazon Web Services
* CodeCommit or in any other Git repository. When you open a notebook instance, it opens in the
* directory that contains this repository. For more information, see Associating Git Repositories
* with SageMaker Notebook Instances.
*/
public String getDefaultCodeRepository() {
return this.defaultCodeRepository;
}
/**
*
* A Git repository to associate with the notebook instance as its default code repository. This can be either the
* name of a Git repository stored as a resource in your account, or the URL of a Git repository in Amazon Web Services CodeCommit or
* in any other Git repository. When you open a notebook instance, it opens in the directory that contains this
* repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @param defaultCodeRepository
* A Git repository to associate with the notebook instance as its default code repository. This can be
* either the name of a Git repository stored as a resource in your account, or the URL of a Git repository
* in Amazon Web Services
* CodeCommit or in any other Git repository. When you open a notebook instance, it opens in the
* directory that contains this repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withDefaultCodeRepository(String defaultCodeRepository) {
setDefaultCodeRepository(defaultCodeRepository);
return this;
}
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @return An array of up to three Git repositories to associate with the notebook instance. These can be either the
* names of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services
* CodeCommit or in any other Git repository. These repositories are cloned at the same level as the
* default repository of your notebook instance. For more information, see Associating Git Repositories
* with SageMaker Notebook Instances.
*/
public java.util.List getAdditionalCodeRepositories() {
return additionalCodeRepositories;
}
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @param additionalCodeRepositories
* An array of up to three Git repositories to associate with the notebook instance. These can be either the
* names of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services
* CodeCommit or in any other Git repository. These repositories are cloned at the same level as the
* default repository of your notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*/
public void setAdditionalCodeRepositories(java.util.Collection additionalCodeRepositories) {
if (additionalCodeRepositories == null) {
this.additionalCodeRepositories = null;
return;
}
this.additionalCodeRepositories = new java.util.ArrayList(additionalCodeRepositories);
}
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdditionalCodeRepositories(java.util.Collection)} or
* {@link #withAdditionalCodeRepositories(java.util.Collection)} if you want to override the existing values.
*
*
* @param additionalCodeRepositories
* An array of up to three Git repositories to associate with the notebook instance. These can be either the
* names of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services
* CodeCommit or in any other Git repository. These repositories are cloned at the same level as the
* default repository of your notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withAdditionalCodeRepositories(String... additionalCodeRepositories) {
if (this.additionalCodeRepositories == null) {
setAdditionalCodeRepositories(new java.util.ArrayList(additionalCodeRepositories.length));
}
for (String ele : additionalCodeRepositories) {
this.additionalCodeRepositories.add(ele);
}
return this;
}
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @param additionalCodeRepositories
* An array of up to three Git repositories to associate with the notebook instance. These can be either the
* names of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services
* CodeCommit or in any other Git repository. These repositories are cloned at the same level as the
* default repository of your notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withAdditionalCodeRepositories(java.util.Collection additionalCodeRepositories) {
setAdditionalCodeRepositories(additionalCodeRepositories);
return this;
}
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* Lifecycle configurations need root access to be able to set up a notebook instance. Because of this, lifecycle
* configurations associated with a notebook instance always run with root access even if you disable root access
* for users.
*
*
*
* @param rootAccess
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
* Lifecycle configurations need root access to be able to set up a notebook instance. Because of this,
* lifecycle configurations associated with a notebook instance always run with root access even if you
* disable root access for users.
*
* @see RootAccess
*/
public void setRootAccess(String rootAccess) {
this.rootAccess = rootAccess;
}
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* Lifecycle configurations need root access to be able to set up a notebook instance. Because of this, lifecycle
* configurations associated with a notebook instance always run with root access even if you disable root access
* for users.
*
*
*
* @return Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
* Lifecycle configurations need root access to be able to set up a notebook instance. Because of this,
* lifecycle configurations associated with a notebook instance always run with root access even if you
* disable root access for users.
*
* @see RootAccess
*/
public String getRootAccess() {
return this.rootAccess;
}
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* Lifecycle configurations need root access to be able to set up a notebook instance. Because of this, lifecycle
* configurations associated with a notebook instance always run with root access even if you disable root access
* for users.
*
*
*
* @param rootAccess
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
* Lifecycle configurations need root access to be able to set up a notebook instance. Because of this,
* lifecycle configurations associated with a notebook instance always run with root access even if you
* disable root access for users.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RootAccess
*/
public CreateNotebookInstanceRequest withRootAccess(String rootAccess) {
setRootAccess(rootAccess);
return this;
}
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* Lifecycle configurations need root access to be able to set up a notebook instance. Because of this, lifecycle
* configurations associated with a notebook instance always run with root access even if you disable root access
* for users.
*
*
*
* @param rootAccess
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
* Lifecycle configurations need root access to be able to set up a notebook instance. Because of this,
* lifecycle configurations associated with a notebook instance always run with root access even if you
* disable root access for users.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RootAccess
*/
public CreateNotebookInstanceRequest withRootAccess(RootAccess rootAccess) {
this.rootAccess = rootAccess.toString();
return this;
}
/**
*
* The platform identifier of the notebook instance runtime environment.
*
*
* @param platformIdentifier
* The platform identifier of the notebook instance runtime environment.
*/
public void setPlatformIdentifier(String platformIdentifier) {
this.platformIdentifier = platformIdentifier;
}
/**
*
* The platform identifier of the notebook instance runtime environment.
*
*
* @return The platform identifier of the notebook instance runtime environment.
*/
public String getPlatformIdentifier() {
return this.platformIdentifier;
}
/**
*
* The platform identifier of the notebook instance runtime environment.
*
*
* @param platformIdentifier
* The platform identifier of the notebook instance runtime environment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withPlatformIdentifier(String platformIdentifier) {
setPlatformIdentifier(platformIdentifier);
return this;
}
/**
*
* Information on the IMDS configuration of the notebook instance
*
*
* @param instanceMetadataServiceConfiguration
* Information on the IMDS configuration of the notebook instance
*/
public void setInstanceMetadataServiceConfiguration(InstanceMetadataServiceConfiguration instanceMetadataServiceConfiguration) {
this.instanceMetadataServiceConfiguration = instanceMetadataServiceConfiguration;
}
/**
*
* Information on the IMDS configuration of the notebook instance
*
*
* @return Information on the IMDS configuration of the notebook instance
*/
public InstanceMetadataServiceConfiguration getInstanceMetadataServiceConfiguration() {
return this.instanceMetadataServiceConfiguration;
}
/**
*
* Information on the IMDS configuration of the notebook instance
*
*
* @param instanceMetadataServiceConfiguration
* Information on the IMDS configuration of the notebook instance
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateNotebookInstanceRequest withInstanceMetadataServiceConfiguration(InstanceMetadataServiceConfiguration instanceMetadataServiceConfiguration) {
setInstanceMetadataServiceConfiguration(instanceMetadataServiceConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getNotebookInstanceName() != null)
sb.append("NotebookInstanceName: ").append(getNotebookInstanceName()).append(",");
if (getInstanceType() != null)
sb.append("InstanceType: ").append(getInstanceType()).append(",");
if (getSubnetId() != null)
sb.append("SubnetId: ").append(getSubnetId()).append(",");
if (getSecurityGroupIds() != null)
sb.append("SecurityGroupIds: ").append(getSecurityGroupIds()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getLifecycleConfigName() != null)
sb.append("LifecycleConfigName: ").append(getLifecycleConfigName()).append(",");
if (getDirectInternetAccess() != null)
sb.append("DirectInternetAccess: ").append(getDirectInternetAccess()).append(",");
if (getVolumeSizeInGB() != null)
sb.append("VolumeSizeInGB: ").append(getVolumeSizeInGB()).append(",");
if (getAcceleratorTypes() != null)
sb.append("AcceleratorTypes: ").append(getAcceleratorTypes()).append(",");
if (getDefaultCodeRepository() != null)
sb.append("DefaultCodeRepository: ").append(getDefaultCodeRepository()).append(",");
if (getAdditionalCodeRepositories() != null)
sb.append("AdditionalCodeRepositories: ").append(getAdditionalCodeRepositories()).append(",");
if (getRootAccess() != null)
sb.append("RootAccess: ").append(getRootAccess()).append(",");
if (getPlatformIdentifier() != null)
sb.append("PlatformIdentifier: ").append(getPlatformIdentifier()).append(",");
if (getInstanceMetadataServiceConfiguration() != null)
sb.append("InstanceMetadataServiceConfiguration: ").append(getInstanceMetadataServiceConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateNotebookInstanceRequest == false)
return false;
CreateNotebookInstanceRequest other = (CreateNotebookInstanceRequest) obj;
if (other.getNotebookInstanceName() == null ^ this.getNotebookInstanceName() == null)
return false;
if (other.getNotebookInstanceName() != null && other.getNotebookInstanceName().equals(this.getNotebookInstanceName()) == false)
return false;
if (other.getInstanceType() == null ^ this.getInstanceType() == null)
return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false)
return false;
if (other.getSubnetId() == null ^ this.getSubnetId() == null)
return false;
if (other.getSubnetId() != null && other.getSubnetId().equals(this.getSubnetId()) == false)
return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null)
return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getLifecycleConfigName() == null ^ this.getLifecycleConfigName() == null)
return false;
if (other.getLifecycleConfigName() != null && other.getLifecycleConfigName().equals(this.getLifecycleConfigName()) == false)
return false;
if (other.getDirectInternetAccess() == null ^ this.getDirectInternetAccess() == null)
return false;
if (other.getDirectInternetAccess() != null && other.getDirectInternetAccess().equals(this.getDirectInternetAccess()) == false)
return false;
if (other.getVolumeSizeInGB() == null ^ this.getVolumeSizeInGB() == null)
return false;
if (other.getVolumeSizeInGB() != null && other.getVolumeSizeInGB().equals(this.getVolumeSizeInGB()) == false)
return false;
if (other.getAcceleratorTypes() == null ^ this.getAcceleratorTypes() == null)
return false;
if (other.getAcceleratorTypes() != null && other.getAcceleratorTypes().equals(this.getAcceleratorTypes()) == false)
return false;
if (other.getDefaultCodeRepository() == null ^ this.getDefaultCodeRepository() == null)
return false;
if (other.getDefaultCodeRepository() != null && other.getDefaultCodeRepository().equals(this.getDefaultCodeRepository()) == false)
return false;
if (other.getAdditionalCodeRepositories() == null ^ this.getAdditionalCodeRepositories() == null)
return false;
if (other.getAdditionalCodeRepositories() != null && other.getAdditionalCodeRepositories().equals(this.getAdditionalCodeRepositories()) == false)
return false;
if (other.getRootAccess() == null ^ this.getRootAccess() == null)
return false;
if (other.getRootAccess() != null && other.getRootAccess().equals(this.getRootAccess()) == false)
return false;
if (other.getPlatformIdentifier() == null ^ this.getPlatformIdentifier() == null)
return false;
if (other.getPlatformIdentifier() != null && other.getPlatformIdentifier().equals(this.getPlatformIdentifier()) == false)
return false;
if (other.getInstanceMetadataServiceConfiguration() == null ^ this.getInstanceMetadataServiceConfiguration() == null)
return false;
if (other.getInstanceMetadataServiceConfiguration() != null
&& other.getInstanceMetadataServiceConfiguration().equals(this.getInstanceMetadataServiceConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getNotebookInstanceName() == null) ? 0 : getNotebookInstanceName().hashCode());
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getSubnetId() == null) ? 0 : getSubnetId().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getLifecycleConfigName() == null) ? 0 : getLifecycleConfigName().hashCode());
hashCode = prime * hashCode + ((getDirectInternetAccess() == null) ? 0 : getDirectInternetAccess().hashCode());
hashCode = prime * hashCode + ((getVolumeSizeInGB() == null) ? 0 : getVolumeSizeInGB().hashCode());
hashCode = prime * hashCode + ((getAcceleratorTypes() == null) ? 0 : getAcceleratorTypes().hashCode());
hashCode = prime * hashCode + ((getDefaultCodeRepository() == null) ? 0 : getDefaultCodeRepository().hashCode());
hashCode = prime * hashCode + ((getAdditionalCodeRepositories() == null) ? 0 : getAdditionalCodeRepositories().hashCode());
hashCode = prime * hashCode + ((getRootAccess() == null) ? 0 : getRootAccess().hashCode());
hashCode = prime * hashCode + ((getPlatformIdentifier() == null) ? 0 : getPlatformIdentifier().hashCode());
hashCode = prime * hashCode + ((getInstanceMetadataServiceConfiguration() == null) ? 0 : getInstanceMetadataServiceConfiguration().hashCode());
return hashCode;
}
@Override
public CreateNotebookInstanceRequest clone() {
return (CreateNotebookInstanceRequest) super.clone();
}
}