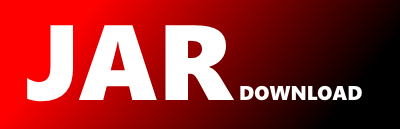
com.amazonaws.services.sagemaker.model.CreateTrialComponentRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateTrialComponentRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the component. The name must be unique in your Amazon Web Services account and is not case-sensitive.
*
*/
private String trialComponentName;
/**
*
* The name of the component as displayed. The name doesn't need to be unique. If DisplayName
isn't
* specified, TrialComponentName
is displayed.
*
*/
private String displayName;
/**
*
* The status of the component. States include:
*
*
* -
*
* InProgress
*
*
* -
*
* Completed
*
*
* -
*
* Failed
*
*
*
*/
private TrialComponentStatus status;
/**
*
* When the component started.
*
*/
private java.util.Date startTime;
/**
*
* When the component ended.
*
*/
private java.util.Date endTime;
/**
*
* The hyperparameters for the component.
*
*/
private java.util.Map parameters;
/**
*
* The input artifacts for the component. Examples of input artifacts are datasets, algorithms, hyperparameters,
* source code, and instance types.
*
*/
private java.util.Map inputArtifacts;
/**
*
* The output artifacts for the component. Examples of output artifacts are metrics, snapshots, logs, and images.
*
*/
private java.util.Map outputArtifacts;
private MetadataProperties metadataProperties;
/**
*
* A list of tags to associate with the component. You can use Search API to search on the
* tags.
*
*/
private java.util.List tags;
/**
*
* The name of the component. The name must be unique in your Amazon Web Services account and is not case-sensitive.
*
*
* @param trialComponentName
* The name of the component. The name must be unique in your Amazon Web Services account and is not
* case-sensitive.
*/
public void setTrialComponentName(String trialComponentName) {
this.trialComponentName = trialComponentName;
}
/**
*
* The name of the component. The name must be unique in your Amazon Web Services account and is not case-sensitive.
*
*
* @return The name of the component. The name must be unique in your Amazon Web Services account and is not
* case-sensitive.
*/
public String getTrialComponentName() {
return this.trialComponentName;
}
/**
*
* The name of the component. The name must be unique in your Amazon Web Services account and is not case-sensitive.
*
*
* @param trialComponentName
* The name of the component. The name must be unique in your Amazon Web Services account and is not
* case-sensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withTrialComponentName(String trialComponentName) {
setTrialComponentName(trialComponentName);
return this;
}
/**
*
* The name of the component as displayed. The name doesn't need to be unique. If DisplayName
isn't
* specified, TrialComponentName
is displayed.
*
*
* @param displayName
* The name of the component as displayed. The name doesn't need to be unique. If DisplayName
* isn't specified, TrialComponentName
is displayed.
*/
public void setDisplayName(String displayName) {
this.displayName = displayName;
}
/**
*
* The name of the component as displayed. The name doesn't need to be unique. If DisplayName
isn't
* specified, TrialComponentName
is displayed.
*
*
* @return The name of the component as displayed. The name doesn't need to be unique. If DisplayName
* isn't specified, TrialComponentName
is displayed.
*/
public String getDisplayName() {
return this.displayName;
}
/**
*
* The name of the component as displayed. The name doesn't need to be unique. If DisplayName
isn't
* specified, TrialComponentName
is displayed.
*
*
* @param displayName
* The name of the component as displayed. The name doesn't need to be unique. If DisplayName
* isn't specified, TrialComponentName
is displayed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withDisplayName(String displayName) {
setDisplayName(displayName);
return this;
}
/**
*
* The status of the component. States include:
*
*
* -
*
* InProgress
*
*
* -
*
* Completed
*
*
* -
*
* Failed
*
*
*
*
* @param status
* The status of the component. States include:
*
* -
*
* InProgress
*
*
* -
*
* Completed
*
*
* -
*
* Failed
*
*
*/
public void setStatus(TrialComponentStatus status) {
this.status = status;
}
/**
*
* The status of the component. States include:
*
*
* -
*
* InProgress
*
*
* -
*
* Completed
*
*
* -
*
* Failed
*
*
*
*
* @return The status of the component. States include:
*
* -
*
* InProgress
*
*
* -
*
* Completed
*
*
* -
*
* Failed
*
*
*/
public TrialComponentStatus getStatus() {
return this.status;
}
/**
*
* The status of the component. States include:
*
*
* -
*
* InProgress
*
*
* -
*
* Completed
*
*
* -
*
* Failed
*
*
*
*
* @param status
* The status of the component. States include:
*
* -
*
* InProgress
*
*
* -
*
* Completed
*
*
* -
*
* Failed
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withStatus(TrialComponentStatus status) {
setStatus(status);
return this;
}
/**
*
* When the component started.
*
*
* @param startTime
* When the component started.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* When the component started.
*
*
* @return When the component started.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* When the component started.
*
*
* @param startTime
* When the component started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* When the component ended.
*
*
* @param endTime
* When the component ended.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* When the component ended.
*
*
* @return When the component ended.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* When the component ended.
*
*
* @param endTime
* When the component ended.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* The hyperparameters for the component.
*
*
* @return The hyperparameters for the component.
*/
public java.util.Map getParameters() {
return parameters;
}
/**
*
* The hyperparameters for the component.
*
*
* @param parameters
* The hyperparameters for the component.
*/
public void setParameters(java.util.Map parameters) {
this.parameters = parameters;
}
/**
*
* The hyperparameters for the component.
*
*
* @param parameters
* The hyperparameters for the component.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withParameters(java.util.Map parameters) {
setParameters(parameters);
return this;
}
/**
* Add a single Parameters entry
*
* @see CreateTrialComponentRequest#withParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest addParametersEntry(String key, TrialComponentParameterValue value) {
if (null == this.parameters) {
this.parameters = new java.util.HashMap();
}
if (this.parameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.parameters.put(key, value);
return this;
}
/**
* Removes all the entries added into Parameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest clearParametersEntries() {
this.parameters = null;
return this;
}
/**
*
* The input artifacts for the component. Examples of input artifacts are datasets, algorithms, hyperparameters,
* source code, and instance types.
*
*
* @return The input artifacts for the component. Examples of input artifacts are datasets, algorithms,
* hyperparameters, source code, and instance types.
*/
public java.util.Map getInputArtifacts() {
return inputArtifacts;
}
/**
*
* The input artifacts for the component. Examples of input artifacts are datasets, algorithms, hyperparameters,
* source code, and instance types.
*
*
* @param inputArtifacts
* The input artifacts for the component. Examples of input artifacts are datasets, algorithms,
* hyperparameters, source code, and instance types.
*/
public void setInputArtifacts(java.util.Map inputArtifacts) {
this.inputArtifacts = inputArtifacts;
}
/**
*
* The input artifacts for the component. Examples of input artifacts are datasets, algorithms, hyperparameters,
* source code, and instance types.
*
*
* @param inputArtifacts
* The input artifacts for the component. Examples of input artifacts are datasets, algorithms,
* hyperparameters, source code, and instance types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withInputArtifacts(java.util.Map inputArtifacts) {
setInputArtifacts(inputArtifacts);
return this;
}
/**
* Add a single InputArtifacts entry
*
* @see CreateTrialComponentRequest#withInputArtifacts
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest addInputArtifactsEntry(String key, TrialComponentArtifact value) {
if (null == this.inputArtifacts) {
this.inputArtifacts = new java.util.HashMap();
}
if (this.inputArtifacts.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.inputArtifacts.put(key, value);
return this;
}
/**
* Removes all the entries added into InputArtifacts.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest clearInputArtifactsEntries() {
this.inputArtifacts = null;
return this;
}
/**
*
* The output artifacts for the component. Examples of output artifacts are metrics, snapshots, logs, and images.
*
*
* @return The output artifacts for the component. Examples of output artifacts are metrics, snapshots, logs, and
* images.
*/
public java.util.Map getOutputArtifacts() {
return outputArtifacts;
}
/**
*
* The output artifacts for the component. Examples of output artifacts are metrics, snapshots, logs, and images.
*
*
* @param outputArtifacts
* The output artifacts for the component. Examples of output artifacts are metrics, snapshots, logs, and
* images.
*/
public void setOutputArtifacts(java.util.Map outputArtifacts) {
this.outputArtifacts = outputArtifacts;
}
/**
*
* The output artifacts for the component. Examples of output artifacts are metrics, snapshots, logs, and images.
*
*
* @param outputArtifacts
* The output artifacts for the component. Examples of output artifacts are metrics, snapshots, logs, and
* images.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withOutputArtifacts(java.util.Map outputArtifacts) {
setOutputArtifacts(outputArtifacts);
return this;
}
/**
* Add a single OutputArtifacts entry
*
* @see CreateTrialComponentRequest#withOutputArtifacts
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest addOutputArtifactsEntry(String key, TrialComponentArtifact value) {
if (null == this.outputArtifacts) {
this.outputArtifacts = new java.util.HashMap();
}
if (this.outputArtifacts.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.outputArtifacts.put(key, value);
return this;
}
/**
* Removes all the entries added into OutputArtifacts.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest clearOutputArtifactsEntries() {
this.outputArtifacts = null;
return this;
}
/**
* @param metadataProperties
*/
public void setMetadataProperties(MetadataProperties metadataProperties) {
this.metadataProperties = metadataProperties;
}
/**
* @return
*/
public MetadataProperties getMetadataProperties() {
return this.metadataProperties;
}
/**
* @param metadataProperties
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withMetadataProperties(MetadataProperties metadataProperties) {
setMetadataProperties(metadataProperties);
return this;
}
/**
*
* A list of tags to associate with the component. You can use Search API to search on the
* tags.
*
*
* @return A list of tags to associate with the component. You can use Search API to search
* on the tags.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of tags to associate with the component. You can use Search API to search on the
* tags.
*
*
* @param tags
* A list of tags to associate with the component. You can use Search API to search
* on the tags.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of tags to associate with the component. You can use Search API to search on the
* tags.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags to associate with the component. You can use Search API to search
* on the tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags to associate with the component. You can use Search API to search on the
* tags.
*
*
* @param tags
* A list of tags to associate with the component. You can use Search API to search
* on the tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateTrialComponentRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTrialComponentName() != null)
sb.append("TrialComponentName: ").append(getTrialComponentName()).append(",");
if (getDisplayName() != null)
sb.append("DisplayName: ").append(getDisplayName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters()).append(",");
if (getInputArtifacts() != null)
sb.append("InputArtifacts: ").append(getInputArtifacts()).append(",");
if (getOutputArtifacts() != null)
sb.append("OutputArtifacts: ").append(getOutputArtifacts()).append(",");
if (getMetadataProperties() != null)
sb.append("MetadataProperties: ").append(getMetadataProperties()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateTrialComponentRequest == false)
return false;
CreateTrialComponentRequest other = (CreateTrialComponentRequest) obj;
if (other.getTrialComponentName() == null ^ this.getTrialComponentName() == null)
return false;
if (other.getTrialComponentName() != null && other.getTrialComponentName().equals(this.getTrialComponentName()) == false)
return false;
if (other.getDisplayName() == null ^ this.getDisplayName() == null)
return false;
if (other.getDisplayName() != null && other.getDisplayName().equals(this.getDisplayName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getInputArtifacts() == null ^ this.getInputArtifacts() == null)
return false;
if (other.getInputArtifacts() != null && other.getInputArtifacts().equals(this.getInputArtifacts()) == false)
return false;
if (other.getOutputArtifacts() == null ^ this.getOutputArtifacts() == null)
return false;
if (other.getOutputArtifacts() != null && other.getOutputArtifacts().equals(this.getOutputArtifacts()) == false)
return false;
if (other.getMetadataProperties() == null ^ this.getMetadataProperties() == null)
return false;
if (other.getMetadataProperties() != null && other.getMetadataProperties().equals(this.getMetadataProperties()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTrialComponentName() == null) ? 0 : getTrialComponentName().hashCode());
hashCode = prime * hashCode + ((getDisplayName() == null) ? 0 : getDisplayName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getInputArtifacts() == null) ? 0 : getInputArtifacts().hashCode());
hashCode = prime * hashCode + ((getOutputArtifacts() == null) ? 0 : getOutputArtifacts().hashCode());
hashCode = prime * hashCode + ((getMetadataProperties() == null) ? 0 : getMetadataProperties().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateTrialComponentRequest clone() {
return (CreateTrialComponentRequest) super.clone();
}
}