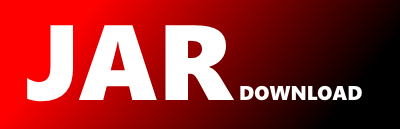
com.amazonaws.services.sagemaker.model.CreateWorkteamRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateWorkteamRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the work team. Use this name to identify the work team.
*
*/
private String workteamName;
/**
*
* The name of the workforce.
*
*/
private String workforceName;
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up the
* work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private workforces
* created using Amazon Cognito use CognitoMemberDefinition
. For workforces created using your own OIDC
* identity provider (IdP) use OidcMemberDefinition
. Do not provide input for both of these parameters
* in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user groups
* within the user pool used to create a workforce. All of the CognitoMemberDefinition
objects that
* make up the member definition must have the same ClientId
and UserPool
values. To add a
* Amazon Cognito user group to an existing worker pool, see Adding groups to a User Pool. For more
* information about user pools, see Amazon Cognito
* User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your private
* work team in OidcMemberDefinition
by listing those groups in Groups
.
*
*/
private java.util.List memberDefinitions;
/**
*
* A description of the work team.
*
*/
private String description;
/**
*
* Configures notification of workers regarding available or expiring work items.
*
*/
private NotificationConfiguration notificationConfiguration;
/**
*
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using supported
* IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a Amazon S3 presigned
* URL.
*
*/
private WorkerAccessConfiguration workerAccessConfiguration;
/**
*
* An array of key-value pairs.
*
*
* For more information, see Resource
* Tag and Using
* Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*
*/
private java.util.List tags;
/**
*
* The name of the work team. Use this name to identify the work team.
*
*
* @param workteamName
* The name of the work team. Use this name to identify the work team.
*/
public void setWorkteamName(String workteamName) {
this.workteamName = workteamName;
}
/**
*
* The name of the work team. Use this name to identify the work team.
*
*
* @return The name of the work team. Use this name to identify the work team.
*/
public String getWorkteamName() {
return this.workteamName;
}
/**
*
* The name of the work team. Use this name to identify the work team.
*
*
* @param workteamName
* The name of the work team. Use this name to identify the work team.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateWorkteamRequest withWorkteamName(String workteamName) {
setWorkteamName(workteamName);
return this;
}
/**
*
* The name of the workforce.
*
*
* @param workforceName
* The name of the workforce.
*/
public void setWorkforceName(String workforceName) {
this.workforceName = workforceName;
}
/**
*
* The name of the workforce.
*
*
* @return The name of the workforce.
*/
public String getWorkforceName() {
return this.workforceName;
}
/**
*
* The name of the workforce.
*
*
* @param workforceName
* The name of the workforce.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateWorkteamRequest withWorkforceName(String workforceName) {
setWorkforceName(workforceName);
return this;
}
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up the
* work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private workforces
* created using Amazon Cognito use CognitoMemberDefinition
. For workforces created using your own OIDC
* identity provider (IdP) use OidcMemberDefinition
. Do not provide input for both of these parameters
* in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user groups
* within the user pool used to create a workforce. All of the CognitoMemberDefinition
objects that
* make up the member definition must have the same ClientId
and UserPool
values. To add a
* Amazon Cognito user group to an existing worker pool, see Adding groups to a User Pool. For more
* information about user pools, see Amazon Cognito
* User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your private
* work team in OidcMemberDefinition
by listing those groups in Groups
.
*
*
* @return A list of MemberDefinition
objects that contains objects that identify the workers that make
* up the work team.
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
. Do not provide input
* for both of these parameters in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user
* groups within the user pool used to create a workforce. All of the
* CognitoMemberDefinition
objects that make up the member definition must have the same
* ClientId
and UserPool
values. To add a Amazon Cognito user group to an existing
* worker pool, see Adding groups to a User Pool. For more information about user pools, see
*
* Amazon Cognito User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your
* private work team in OidcMemberDefinition
by listing those groups in Groups
.
*/
public java.util.List getMemberDefinitions() {
return memberDefinitions;
}
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up the
* work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private workforces
* created using Amazon Cognito use CognitoMemberDefinition
. For workforces created using your own OIDC
* identity provider (IdP) use OidcMemberDefinition
. Do not provide input for both of these parameters
* in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user groups
* within the user pool used to create a workforce. All of the CognitoMemberDefinition
objects that
* make up the member definition must have the same ClientId
and UserPool
values. To add a
* Amazon Cognito user group to an existing worker pool, see Adding groups to a User Pool. For more
* information about user pools, see Amazon Cognito
* User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your private
* work team in OidcMemberDefinition
by listing those groups in Groups
.
*
*
* @param memberDefinitions
* A list of MemberDefinition
objects that contains objects that identify the workers that make
* up the work team.
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
. Do not provide input
* for both of these parameters in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user
* groups within the user pool used to create a workforce. All of the
* CognitoMemberDefinition
objects that make up the member definition must have the same
* ClientId
and UserPool
values. To add a Amazon Cognito user group to an existing
* worker pool, see Adding groups to a User Pool. For more information about user pools, see
* Amazon
* Cognito User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your
* private work team in OidcMemberDefinition
by listing those groups in Groups
.
*/
public void setMemberDefinitions(java.util.Collection memberDefinitions) {
if (memberDefinitions == null) {
this.memberDefinitions = null;
return;
}
this.memberDefinitions = new java.util.ArrayList(memberDefinitions);
}
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up the
* work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private workforces
* created using Amazon Cognito use CognitoMemberDefinition
. For workforces created using your own OIDC
* identity provider (IdP) use OidcMemberDefinition
. Do not provide input for both of these parameters
* in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user groups
* within the user pool used to create a workforce. All of the CognitoMemberDefinition
objects that
* make up the member definition must have the same ClientId
and UserPool
values. To add a
* Amazon Cognito user group to an existing worker pool, see Adding groups to a User Pool. For more
* information about user pools, see Amazon Cognito
* User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your private
* work team in OidcMemberDefinition
by listing those groups in Groups
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMemberDefinitions(java.util.Collection)} or {@link #withMemberDefinitions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param memberDefinitions
* A list of MemberDefinition
objects that contains objects that identify the workers that make
* up the work team.
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
. Do not provide input
* for both of these parameters in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user
* groups within the user pool used to create a workforce. All of the
* CognitoMemberDefinition
objects that make up the member definition must have the same
* ClientId
and UserPool
values. To add a Amazon Cognito user group to an existing
* worker pool, see Adding groups to a User Pool. For more information about user pools, see
* Amazon
* Cognito User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your
* private work team in OidcMemberDefinition
by listing those groups in Groups
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateWorkteamRequest withMemberDefinitions(MemberDefinition... memberDefinitions) {
if (this.memberDefinitions == null) {
setMemberDefinitions(new java.util.ArrayList(memberDefinitions.length));
}
for (MemberDefinition ele : memberDefinitions) {
this.memberDefinitions.add(ele);
}
return this;
}
/**
*
* A list of MemberDefinition
objects that contains objects that identify the workers that make up the
* work team.
*
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private workforces
* created using Amazon Cognito use CognitoMemberDefinition
. For workforces created using your own OIDC
* identity provider (IdP) use OidcMemberDefinition
. Do not provide input for both of these parameters
* in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user groups
* within the user pool used to create a workforce. All of the CognitoMemberDefinition
objects that
* make up the member definition must have the same ClientId
and UserPool
values. To add a
* Amazon Cognito user group to an existing worker pool, see Adding groups to a User Pool. For more
* information about user pools, see Amazon Cognito
* User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your private
* work team in OidcMemberDefinition
by listing those groups in Groups
.
*
*
* @param memberDefinitions
* A list of MemberDefinition
objects that contains objects that identify the workers that make
* up the work team.
*
* Workforces can be created using Amazon Cognito or your own OIDC Identity Provider (IdP). For private
* workforces created using Amazon Cognito use CognitoMemberDefinition
. For workforces created
* using your own OIDC identity provider (IdP) use OidcMemberDefinition
. Do not provide input
* for both of these parameters in a single request.
*
*
* For workforces created using Amazon Cognito, private work teams correspond to Amazon Cognito user
* groups within the user pool used to create a workforce. All of the
* CognitoMemberDefinition
objects that make up the member definition must have the same
* ClientId
and UserPool
values. To add a Amazon Cognito user group to an existing
* worker pool, see Adding groups to a User Pool. For more information about user pools, see
* Amazon
* Cognito User Pools.
*
*
* For workforces created using your own OIDC IdP, specify the user groups that you want to include in your
* private work team in OidcMemberDefinition
by listing those groups in Groups
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateWorkteamRequest withMemberDefinitions(java.util.Collection memberDefinitions) {
setMemberDefinitions(memberDefinitions);
return this;
}
/**
*
* A description of the work team.
*
*
* @param description
* A description of the work team.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the work team.
*
*
* @return A description of the work team.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the work team.
*
*
* @param description
* A description of the work team.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateWorkteamRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Configures notification of workers regarding available or expiring work items.
*
*
* @param notificationConfiguration
* Configures notification of workers regarding available or expiring work items.
*/
public void setNotificationConfiguration(NotificationConfiguration notificationConfiguration) {
this.notificationConfiguration = notificationConfiguration;
}
/**
*
* Configures notification of workers regarding available or expiring work items.
*
*
* @return Configures notification of workers regarding available or expiring work items.
*/
public NotificationConfiguration getNotificationConfiguration() {
return this.notificationConfiguration;
}
/**
*
* Configures notification of workers regarding available or expiring work items.
*
*
* @param notificationConfiguration
* Configures notification of workers regarding available or expiring work items.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateWorkteamRequest withNotificationConfiguration(NotificationConfiguration notificationConfiguration) {
setNotificationConfiguration(notificationConfiguration);
return this;
}
/**
*
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using supported
* IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a Amazon S3 presigned
* URL.
*
*
* @param workerAccessConfiguration
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using
* supported IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a
* Amazon S3 presigned URL.
*/
public void setWorkerAccessConfiguration(WorkerAccessConfiguration workerAccessConfiguration) {
this.workerAccessConfiguration = workerAccessConfiguration;
}
/**
*
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using supported
* IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a Amazon S3 presigned
* URL.
*
*
* @return Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using
* supported IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a
* Amazon S3 presigned URL.
*/
public WorkerAccessConfiguration getWorkerAccessConfiguration() {
return this.workerAccessConfiguration;
}
/**
*
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using supported
* IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a Amazon S3 presigned
* URL.
*
*
* @param workerAccessConfiguration
* Use this optional parameter to constrain access to an Amazon S3 resource based on the IP address using
* supported IAM global condition keys. The Amazon S3 resource is accessed in the worker portal using a
* Amazon S3 presigned URL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateWorkteamRequest withWorkerAccessConfiguration(WorkerAccessConfiguration workerAccessConfiguration) {
setWorkerAccessConfiguration(workerAccessConfiguration);
return this;
}
/**
*
* An array of key-value pairs.
*
*
* For more information, see Resource
* Tag and Using
* Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*
*
* @return An array of key-value pairs.
*
* For more information, see Resource Tag and Using
* Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* An array of key-value pairs.
*
*
* For more information, see Resource
* Tag and Using
* Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*
*
* @param tags
* An array of key-value pairs.
*
* For more information, see Resource Tag and Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* An array of key-value pairs.
*
*
* For more information, see Resource
* Tag and Using
* Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* An array of key-value pairs.
*
* For more information, see Resource Tag and Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateWorkteamRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* An array of key-value pairs.
*
*
* For more information, see Resource
* Tag and Using
* Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
*
*
* @param tags
* An array of key-value pairs.
*
* For more information, see Resource Tag and Using Cost Allocation Tags in the Amazon Web Services Billing and Cost Management User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateWorkteamRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWorkteamName() != null)
sb.append("WorkteamName: ").append(getWorkteamName()).append(",");
if (getWorkforceName() != null)
sb.append("WorkforceName: ").append(getWorkforceName()).append(",");
if (getMemberDefinitions() != null)
sb.append("MemberDefinitions: ").append(getMemberDefinitions()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getNotificationConfiguration() != null)
sb.append("NotificationConfiguration: ").append(getNotificationConfiguration()).append(",");
if (getWorkerAccessConfiguration() != null)
sb.append("WorkerAccessConfiguration: ").append(getWorkerAccessConfiguration()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateWorkteamRequest == false)
return false;
CreateWorkteamRequest other = (CreateWorkteamRequest) obj;
if (other.getWorkteamName() == null ^ this.getWorkteamName() == null)
return false;
if (other.getWorkteamName() != null && other.getWorkteamName().equals(this.getWorkteamName()) == false)
return false;
if (other.getWorkforceName() == null ^ this.getWorkforceName() == null)
return false;
if (other.getWorkforceName() != null && other.getWorkforceName().equals(this.getWorkforceName()) == false)
return false;
if (other.getMemberDefinitions() == null ^ this.getMemberDefinitions() == null)
return false;
if (other.getMemberDefinitions() != null && other.getMemberDefinitions().equals(this.getMemberDefinitions()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getNotificationConfiguration() == null ^ this.getNotificationConfiguration() == null)
return false;
if (other.getNotificationConfiguration() != null && other.getNotificationConfiguration().equals(this.getNotificationConfiguration()) == false)
return false;
if (other.getWorkerAccessConfiguration() == null ^ this.getWorkerAccessConfiguration() == null)
return false;
if (other.getWorkerAccessConfiguration() != null && other.getWorkerAccessConfiguration().equals(this.getWorkerAccessConfiguration()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWorkteamName() == null) ? 0 : getWorkteamName().hashCode());
hashCode = prime * hashCode + ((getWorkforceName() == null) ? 0 : getWorkforceName().hashCode());
hashCode = prime * hashCode + ((getMemberDefinitions() == null) ? 0 : getMemberDefinitions().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getNotificationConfiguration() == null) ? 0 : getNotificationConfiguration().hashCode());
hashCode = prime * hashCode + ((getWorkerAccessConfiguration() == null) ? 0 : getWorkerAccessConfiguration().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateWorkteamRequest clone() {
return (CreateWorkteamRequest) super.clone();
}
}