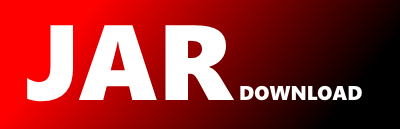
com.amazonaws.services.sagemaker.model.DataQualityAppSpecification Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about the container that a data quality monitoring job runs.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DataQualityAppSpecification implements Serializable, Cloneable, StructuredPojo {
/**
*
* The container image that the data quality monitoring job runs.
*
*/
private String imageUri;
/**
*
* The entrypoint for a container used to run a monitoring job.
*
*/
private java.util.List containerEntrypoint;
/**
*
* The arguments to send to the container that the monitoring job runs.
*
*/
private java.util.List containerArguments;
/**
*
* An Amazon S3 URI to a script that is called per row prior to running analysis. It can base64 decode the payload
* and convert it into a flattened JSON so that the built-in container can use the converted data. Applicable only
* for the built-in (first party) containers.
*
*/
private String recordPreprocessorSourceUri;
/**
*
* An Amazon S3 URI to a script that is called after analysis has been performed. Applicable only for the built-in
* (first party) containers.
*
*/
private String postAnalyticsProcessorSourceUri;
/**
*
* Sets the environment variables in the container that the monitoring job runs.
*
*/
private java.util.Map environment;
/**
*
* The container image that the data quality monitoring job runs.
*
*
* @param imageUri
* The container image that the data quality monitoring job runs.
*/
public void setImageUri(String imageUri) {
this.imageUri = imageUri;
}
/**
*
* The container image that the data quality monitoring job runs.
*
*
* @return The container image that the data quality monitoring job runs.
*/
public String getImageUri() {
return this.imageUri;
}
/**
*
* The container image that the data quality monitoring job runs.
*
*
* @param imageUri
* The container image that the data quality monitoring job runs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification withImageUri(String imageUri) {
setImageUri(imageUri);
return this;
}
/**
*
* The entrypoint for a container used to run a monitoring job.
*
*
* @return The entrypoint for a container used to run a monitoring job.
*/
public java.util.List getContainerEntrypoint() {
return containerEntrypoint;
}
/**
*
* The entrypoint for a container used to run a monitoring job.
*
*
* @param containerEntrypoint
* The entrypoint for a container used to run a monitoring job.
*/
public void setContainerEntrypoint(java.util.Collection containerEntrypoint) {
if (containerEntrypoint == null) {
this.containerEntrypoint = null;
return;
}
this.containerEntrypoint = new java.util.ArrayList(containerEntrypoint);
}
/**
*
* The entrypoint for a container used to run a monitoring job.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setContainerEntrypoint(java.util.Collection)} or {@link #withContainerEntrypoint(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param containerEntrypoint
* The entrypoint for a container used to run a monitoring job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification withContainerEntrypoint(String... containerEntrypoint) {
if (this.containerEntrypoint == null) {
setContainerEntrypoint(new java.util.ArrayList(containerEntrypoint.length));
}
for (String ele : containerEntrypoint) {
this.containerEntrypoint.add(ele);
}
return this;
}
/**
*
* The entrypoint for a container used to run a monitoring job.
*
*
* @param containerEntrypoint
* The entrypoint for a container used to run a monitoring job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification withContainerEntrypoint(java.util.Collection containerEntrypoint) {
setContainerEntrypoint(containerEntrypoint);
return this;
}
/**
*
* The arguments to send to the container that the monitoring job runs.
*
*
* @return The arguments to send to the container that the monitoring job runs.
*/
public java.util.List getContainerArguments() {
return containerArguments;
}
/**
*
* The arguments to send to the container that the monitoring job runs.
*
*
* @param containerArguments
* The arguments to send to the container that the monitoring job runs.
*/
public void setContainerArguments(java.util.Collection containerArguments) {
if (containerArguments == null) {
this.containerArguments = null;
return;
}
this.containerArguments = new java.util.ArrayList(containerArguments);
}
/**
*
* The arguments to send to the container that the monitoring job runs.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setContainerArguments(java.util.Collection)} or {@link #withContainerArguments(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param containerArguments
* The arguments to send to the container that the monitoring job runs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification withContainerArguments(String... containerArguments) {
if (this.containerArguments == null) {
setContainerArguments(new java.util.ArrayList(containerArguments.length));
}
for (String ele : containerArguments) {
this.containerArguments.add(ele);
}
return this;
}
/**
*
* The arguments to send to the container that the monitoring job runs.
*
*
* @param containerArguments
* The arguments to send to the container that the monitoring job runs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification withContainerArguments(java.util.Collection containerArguments) {
setContainerArguments(containerArguments);
return this;
}
/**
*
* An Amazon S3 URI to a script that is called per row prior to running analysis. It can base64 decode the payload
* and convert it into a flattened JSON so that the built-in container can use the converted data. Applicable only
* for the built-in (first party) containers.
*
*
* @param recordPreprocessorSourceUri
* An Amazon S3 URI to a script that is called per row prior to running analysis. It can base64 decode the
* payload and convert it into a flattened JSON so that the built-in container can use the converted data.
* Applicable only for the built-in (first party) containers.
*/
public void setRecordPreprocessorSourceUri(String recordPreprocessorSourceUri) {
this.recordPreprocessorSourceUri = recordPreprocessorSourceUri;
}
/**
*
* An Amazon S3 URI to a script that is called per row prior to running analysis. It can base64 decode the payload
* and convert it into a flattened JSON so that the built-in container can use the converted data. Applicable only
* for the built-in (first party) containers.
*
*
* @return An Amazon S3 URI to a script that is called per row prior to running analysis. It can base64 decode the
* payload and convert it into a flattened JSON so that the built-in container can use the converted data.
* Applicable only for the built-in (first party) containers.
*/
public String getRecordPreprocessorSourceUri() {
return this.recordPreprocessorSourceUri;
}
/**
*
* An Amazon S3 URI to a script that is called per row prior to running analysis. It can base64 decode the payload
* and convert it into a flattened JSON so that the built-in container can use the converted data. Applicable only
* for the built-in (first party) containers.
*
*
* @param recordPreprocessorSourceUri
* An Amazon S3 URI to a script that is called per row prior to running analysis. It can base64 decode the
* payload and convert it into a flattened JSON so that the built-in container can use the converted data.
* Applicable only for the built-in (first party) containers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification withRecordPreprocessorSourceUri(String recordPreprocessorSourceUri) {
setRecordPreprocessorSourceUri(recordPreprocessorSourceUri);
return this;
}
/**
*
* An Amazon S3 URI to a script that is called after analysis has been performed. Applicable only for the built-in
* (first party) containers.
*
*
* @param postAnalyticsProcessorSourceUri
* An Amazon S3 URI to a script that is called after analysis has been performed. Applicable only for the
* built-in (first party) containers.
*/
public void setPostAnalyticsProcessorSourceUri(String postAnalyticsProcessorSourceUri) {
this.postAnalyticsProcessorSourceUri = postAnalyticsProcessorSourceUri;
}
/**
*
* An Amazon S3 URI to a script that is called after analysis has been performed. Applicable only for the built-in
* (first party) containers.
*
*
* @return An Amazon S3 URI to a script that is called after analysis has been performed. Applicable only for the
* built-in (first party) containers.
*/
public String getPostAnalyticsProcessorSourceUri() {
return this.postAnalyticsProcessorSourceUri;
}
/**
*
* An Amazon S3 URI to a script that is called after analysis has been performed. Applicable only for the built-in
* (first party) containers.
*
*
* @param postAnalyticsProcessorSourceUri
* An Amazon S3 URI to a script that is called after analysis has been performed. Applicable only for the
* built-in (first party) containers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification withPostAnalyticsProcessorSourceUri(String postAnalyticsProcessorSourceUri) {
setPostAnalyticsProcessorSourceUri(postAnalyticsProcessorSourceUri);
return this;
}
/**
*
* Sets the environment variables in the container that the monitoring job runs.
*
*
* @return Sets the environment variables in the container that the monitoring job runs.
*/
public java.util.Map getEnvironment() {
return environment;
}
/**
*
* Sets the environment variables in the container that the monitoring job runs.
*
*
* @param environment
* Sets the environment variables in the container that the monitoring job runs.
*/
public void setEnvironment(java.util.Map environment) {
this.environment = environment;
}
/**
*
* Sets the environment variables in the container that the monitoring job runs.
*
*
* @param environment
* Sets the environment variables in the container that the monitoring job runs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification withEnvironment(java.util.Map environment) {
setEnvironment(environment);
return this;
}
/**
* Add a single Environment entry
*
* @see DataQualityAppSpecification#withEnvironment
* @returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification addEnvironmentEntry(String key, String value) {
if (null == this.environment) {
this.environment = new java.util.HashMap();
}
if (this.environment.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.environment.put(key, value);
return this;
}
/**
* Removes all the entries added into Environment.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DataQualityAppSpecification clearEnvironmentEntries() {
this.environment = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getImageUri() != null)
sb.append("ImageUri: ").append(getImageUri()).append(",");
if (getContainerEntrypoint() != null)
sb.append("ContainerEntrypoint: ").append(getContainerEntrypoint()).append(",");
if (getContainerArguments() != null)
sb.append("ContainerArguments: ").append(getContainerArguments()).append(",");
if (getRecordPreprocessorSourceUri() != null)
sb.append("RecordPreprocessorSourceUri: ").append(getRecordPreprocessorSourceUri()).append(",");
if (getPostAnalyticsProcessorSourceUri() != null)
sb.append("PostAnalyticsProcessorSourceUri: ").append(getPostAnalyticsProcessorSourceUri()).append(",");
if (getEnvironment() != null)
sb.append("Environment: ").append(getEnvironment());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DataQualityAppSpecification == false)
return false;
DataQualityAppSpecification other = (DataQualityAppSpecification) obj;
if (other.getImageUri() == null ^ this.getImageUri() == null)
return false;
if (other.getImageUri() != null && other.getImageUri().equals(this.getImageUri()) == false)
return false;
if (other.getContainerEntrypoint() == null ^ this.getContainerEntrypoint() == null)
return false;
if (other.getContainerEntrypoint() != null && other.getContainerEntrypoint().equals(this.getContainerEntrypoint()) == false)
return false;
if (other.getContainerArguments() == null ^ this.getContainerArguments() == null)
return false;
if (other.getContainerArguments() != null && other.getContainerArguments().equals(this.getContainerArguments()) == false)
return false;
if (other.getRecordPreprocessorSourceUri() == null ^ this.getRecordPreprocessorSourceUri() == null)
return false;
if (other.getRecordPreprocessorSourceUri() != null && other.getRecordPreprocessorSourceUri().equals(this.getRecordPreprocessorSourceUri()) == false)
return false;
if (other.getPostAnalyticsProcessorSourceUri() == null ^ this.getPostAnalyticsProcessorSourceUri() == null)
return false;
if (other.getPostAnalyticsProcessorSourceUri() != null
&& other.getPostAnalyticsProcessorSourceUri().equals(this.getPostAnalyticsProcessorSourceUri()) == false)
return false;
if (other.getEnvironment() == null ^ this.getEnvironment() == null)
return false;
if (other.getEnvironment() != null && other.getEnvironment().equals(this.getEnvironment()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getImageUri() == null) ? 0 : getImageUri().hashCode());
hashCode = prime * hashCode + ((getContainerEntrypoint() == null) ? 0 : getContainerEntrypoint().hashCode());
hashCode = prime * hashCode + ((getContainerArguments() == null) ? 0 : getContainerArguments().hashCode());
hashCode = prime * hashCode + ((getRecordPreprocessorSourceUri() == null) ? 0 : getRecordPreprocessorSourceUri().hashCode());
hashCode = prime * hashCode + ((getPostAnalyticsProcessorSourceUri() == null) ? 0 : getPostAnalyticsProcessorSourceUri().hashCode());
hashCode = prime * hashCode + ((getEnvironment() == null) ? 0 : getEnvironment().hashCode());
return hashCode;
}
@Override
public DataQualityAppSpecification clone() {
try {
return (DataQualityAppSpecification) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.DataQualityAppSpecificationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}