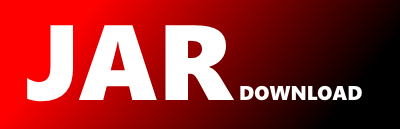
com.amazonaws.services.sagemaker.model.DescribeDomainResult Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeDomainResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The domain's Amazon Resource Name (ARN).
*
*/
private String domainArn;
/**
*
* The domain ID.
*
*/
private String domainId;
/**
*
* The domain name.
*
*/
private String domainName;
/**
*
* The ID of the Amazon Elastic File System managed by this Domain.
*
*/
private String homeEfsFileSystemId;
/**
*
* The IAM Identity Center managed application instance ID.
*
*/
private String singleSignOnManagedApplicationInstanceId;
/**
*
* The ARN of the application managed by SageMaker in IAM Identity Center. This value is only returned for domains
* created after October 1, 2023.
*
*/
private String singleSignOnApplicationArn;
/**
*
* The status.
*
*/
private String status;
/**
*
* The creation time.
*
*/
private java.util.Date creationTime;
/**
*
* The last modified time.
*
*/
private java.util.Date lastModifiedTime;
/**
*
* The failure reason.
*
*/
private String failureReason;
/**
*
* The ID of the security group that authorizes traffic between the RSessionGateway
apps and the
* RStudioServerPro
app.
*
*/
private String securityGroupIdForDomainBoundary;
/**
*
* The domain's authentication mode.
*
*/
private String authMode;
/**
*
* Settings which are applied to UserProfiles in this domain if settings are not explicitly specified in a given
* UserProfile.
*
*/
private UserSettings defaultUserSettings;
/**
*
* A collection of Domain
settings.
*
*/
private DomainSettings domainSettings;
/**
*
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which allows
* direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
*
*/
private String appNetworkAccessType;
/**
*
* Use KmsKeyId
.
*
*/
@Deprecated
private String homeEfsFileSystemKmsKeyId;
/**
*
* The VPC subnets that the domain uses for communication.
*
*/
private java.util.List subnetIds;
/**
*
* The domain's URL.
*
*/
private String url;
/**
*
* The ID of the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*/
private String vpcId;
/**
*
* The Amazon Web Services KMS customer managed key used to encrypt the EFS volume attached to the domain.
*
*/
private String kmsKeyId;
/**
*
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is VPCOnly
* and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided.
*
*/
private String appSecurityGroupManagement;
/**
*
* The default settings used to create a space.
*
*/
private DefaultSpaceSettings defaultSpaceSettings;
/**
*
* The domain's Amazon Resource Name (ARN).
*
*
* @param domainArn
* The domain's Amazon Resource Name (ARN).
*/
public void setDomainArn(String domainArn) {
this.domainArn = domainArn;
}
/**
*
* The domain's Amazon Resource Name (ARN).
*
*
* @return The domain's Amazon Resource Name (ARN).
*/
public String getDomainArn() {
return this.domainArn;
}
/**
*
* The domain's Amazon Resource Name (ARN).
*
*
* @param domainArn
* The domain's Amazon Resource Name (ARN).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withDomainArn(String domainArn) {
setDomainArn(domainArn);
return this;
}
/**
*
* The domain ID.
*
*
* @param domainId
* The domain ID.
*/
public void setDomainId(String domainId) {
this.domainId = domainId;
}
/**
*
* The domain ID.
*
*
* @return The domain ID.
*/
public String getDomainId() {
return this.domainId;
}
/**
*
* The domain ID.
*
*
* @param domainId
* The domain ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withDomainId(String domainId) {
setDomainId(domainId);
return this;
}
/**
*
* The domain name.
*
*
* @param domainName
* The domain name.
*/
public void setDomainName(String domainName) {
this.domainName = domainName;
}
/**
*
* The domain name.
*
*
* @return The domain name.
*/
public String getDomainName() {
return this.domainName;
}
/**
*
* The domain name.
*
*
* @param domainName
* The domain name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withDomainName(String domainName) {
setDomainName(domainName);
return this;
}
/**
*
* The ID of the Amazon Elastic File System managed by this Domain.
*
*
* @param homeEfsFileSystemId
* The ID of the Amazon Elastic File System managed by this Domain.
*/
public void setHomeEfsFileSystemId(String homeEfsFileSystemId) {
this.homeEfsFileSystemId = homeEfsFileSystemId;
}
/**
*
* The ID of the Amazon Elastic File System managed by this Domain.
*
*
* @return The ID of the Amazon Elastic File System managed by this Domain.
*/
public String getHomeEfsFileSystemId() {
return this.homeEfsFileSystemId;
}
/**
*
* The ID of the Amazon Elastic File System managed by this Domain.
*
*
* @param homeEfsFileSystemId
* The ID of the Amazon Elastic File System managed by this Domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withHomeEfsFileSystemId(String homeEfsFileSystemId) {
setHomeEfsFileSystemId(homeEfsFileSystemId);
return this;
}
/**
*
* The IAM Identity Center managed application instance ID.
*
*
* @param singleSignOnManagedApplicationInstanceId
* The IAM Identity Center managed application instance ID.
*/
public void setSingleSignOnManagedApplicationInstanceId(String singleSignOnManagedApplicationInstanceId) {
this.singleSignOnManagedApplicationInstanceId = singleSignOnManagedApplicationInstanceId;
}
/**
*
* The IAM Identity Center managed application instance ID.
*
*
* @return The IAM Identity Center managed application instance ID.
*/
public String getSingleSignOnManagedApplicationInstanceId() {
return this.singleSignOnManagedApplicationInstanceId;
}
/**
*
* The IAM Identity Center managed application instance ID.
*
*
* @param singleSignOnManagedApplicationInstanceId
* The IAM Identity Center managed application instance ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withSingleSignOnManagedApplicationInstanceId(String singleSignOnManagedApplicationInstanceId) {
setSingleSignOnManagedApplicationInstanceId(singleSignOnManagedApplicationInstanceId);
return this;
}
/**
*
* The ARN of the application managed by SageMaker in IAM Identity Center. This value is only returned for domains
* created after October 1, 2023.
*
*
* @param singleSignOnApplicationArn
* The ARN of the application managed by SageMaker in IAM Identity Center. This value is only returned for
* domains created after October 1, 2023.
*/
public void setSingleSignOnApplicationArn(String singleSignOnApplicationArn) {
this.singleSignOnApplicationArn = singleSignOnApplicationArn;
}
/**
*
* The ARN of the application managed by SageMaker in IAM Identity Center. This value is only returned for domains
* created after October 1, 2023.
*
*
* @return The ARN of the application managed by SageMaker in IAM Identity Center. This value is only returned for
* domains created after October 1, 2023.
*/
public String getSingleSignOnApplicationArn() {
return this.singleSignOnApplicationArn;
}
/**
*
* The ARN of the application managed by SageMaker in IAM Identity Center. This value is only returned for domains
* created after October 1, 2023.
*
*
* @param singleSignOnApplicationArn
* The ARN of the application managed by SageMaker in IAM Identity Center. This value is only returned for
* domains created after October 1, 2023.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withSingleSignOnApplicationArn(String singleSignOnApplicationArn) {
setSingleSignOnApplicationArn(singleSignOnApplicationArn);
return this;
}
/**
*
* The status.
*
*
* @param status
* The status.
* @see DomainStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status.
*
*
* @return The status.
* @see DomainStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status.
*
*
* @param status
* The status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DomainStatus
*/
public DescribeDomainResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status.
*
*
* @param status
* The status.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DomainStatus
*/
public DescribeDomainResult withStatus(DomainStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The creation time.
*
*
* @param creationTime
* The creation time.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* The creation time.
*
*
* @return The creation time.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* The creation time.
*
*
* @param creationTime
* The creation time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* The last modified time.
*
*
* @param lastModifiedTime
* The last modified time.
*/
public void setLastModifiedTime(java.util.Date lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
/**
*
* The last modified time.
*
*
* @return The last modified time.
*/
public java.util.Date getLastModifiedTime() {
return this.lastModifiedTime;
}
/**
*
* The last modified time.
*
*
* @param lastModifiedTime
* The last modified time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withLastModifiedTime(java.util.Date lastModifiedTime) {
setLastModifiedTime(lastModifiedTime);
return this;
}
/**
*
* The failure reason.
*
*
* @param failureReason
* The failure reason.
*/
public void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
/**
*
* The failure reason.
*
*
* @return The failure reason.
*/
public String getFailureReason() {
return this.failureReason;
}
/**
*
* The failure reason.
*
*
* @param failureReason
* The failure reason.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withFailureReason(String failureReason) {
setFailureReason(failureReason);
return this;
}
/**
*
* The ID of the security group that authorizes traffic between the RSessionGateway
apps and the
* RStudioServerPro
app.
*
*
* @param securityGroupIdForDomainBoundary
* The ID of the security group that authorizes traffic between the RSessionGateway
apps and the
* RStudioServerPro
app.
*/
public void setSecurityGroupIdForDomainBoundary(String securityGroupIdForDomainBoundary) {
this.securityGroupIdForDomainBoundary = securityGroupIdForDomainBoundary;
}
/**
*
* The ID of the security group that authorizes traffic between the RSessionGateway
apps and the
* RStudioServerPro
app.
*
*
* @return The ID of the security group that authorizes traffic between the RSessionGateway
apps and
* the RStudioServerPro
app.
*/
public String getSecurityGroupIdForDomainBoundary() {
return this.securityGroupIdForDomainBoundary;
}
/**
*
* The ID of the security group that authorizes traffic between the RSessionGateway
apps and the
* RStudioServerPro
app.
*
*
* @param securityGroupIdForDomainBoundary
* The ID of the security group that authorizes traffic between the RSessionGateway
apps and the
* RStudioServerPro
app.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withSecurityGroupIdForDomainBoundary(String securityGroupIdForDomainBoundary) {
setSecurityGroupIdForDomainBoundary(securityGroupIdForDomainBoundary);
return this;
}
/**
*
* The domain's authentication mode.
*
*
* @param authMode
* The domain's authentication mode.
* @see AuthMode
*/
public void setAuthMode(String authMode) {
this.authMode = authMode;
}
/**
*
* The domain's authentication mode.
*
*
* @return The domain's authentication mode.
* @see AuthMode
*/
public String getAuthMode() {
return this.authMode;
}
/**
*
* The domain's authentication mode.
*
*
* @param authMode
* The domain's authentication mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthMode
*/
public DescribeDomainResult withAuthMode(String authMode) {
setAuthMode(authMode);
return this;
}
/**
*
* The domain's authentication mode.
*
*
* @param authMode
* The domain's authentication mode.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AuthMode
*/
public DescribeDomainResult withAuthMode(AuthMode authMode) {
this.authMode = authMode.toString();
return this;
}
/**
*
* Settings which are applied to UserProfiles in this domain if settings are not explicitly specified in a given
* UserProfile.
*
*
* @param defaultUserSettings
* Settings which are applied to UserProfiles in this domain if settings are not explicitly specified in a
* given UserProfile.
*/
public void setDefaultUserSettings(UserSettings defaultUserSettings) {
this.defaultUserSettings = defaultUserSettings;
}
/**
*
* Settings which are applied to UserProfiles in this domain if settings are not explicitly specified in a given
* UserProfile.
*
*
* @return Settings which are applied to UserProfiles in this domain if settings are not explicitly specified in a
* given UserProfile.
*/
public UserSettings getDefaultUserSettings() {
return this.defaultUserSettings;
}
/**
*
* Settings which are applied to UserProfiles in this domain if settings are not explicitly specified in a given
* UserProfile.
*
*
* @param defaultUserSettings
* Settings which are applied to UserProfiles in this domain if settings are not explicitly specified in a
* given UserProfile.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withDefaultUserSettings(UserSettings defaultUserSettings) {
setDefaultUserSettings(defaultUserSettings);
return this;
}
/**
*
* A collection of Domain
settings.
*
*
* @param domainSettings
* A collection of Domain
settings.
*/
public void setDomainSettings(DomainSettings domainSettings) {
this.domainSettings = domainSettings;
}
/**
*
* A collection of Domain
settings.
*
*
* @return A collection of Domain
settings.
*/
public DomainSettings getDomainSettings() {
return this.domainSettings;
}
/**
*
* A collection of Domain
settings.
*
*
* @param domainSettings
* A collection of Domain
settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withDomainSettings(DomainSettings domainSettings) {
setDomainSettings(domainSettings);
return this;
}
/**
*
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which allows
* direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
*
*
* @param appNetworkAccessType
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which
* allows direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
* @see AppNetworkAccessType
*/
public void setAppNetworkAccessType(String appNetworkAccessType) {
this.appNetworkAccessType = appNetworkAccessType;
}
/**
*
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which allows
* direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
*
*
* @return Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which
* allows direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
* @see AppNetworkAccessType
*/
public String getAppNetworkAccessType() {
return this.appNetworkAccessType;
}
/**
*
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which allows
* direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
*
*
* @param appNetworkAccessType
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which
* allows direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppNetworkAccessType
*/
public DescribeDomainResult withAppNetworkAccessType(String appNetworkAccessType) {
setAppNetworkAccessType(appNetworkAccessType);
return this;
}
/**
*
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which allows
* direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
*
*
* @param appNetworkAccessType
* Specifies the VPC used for non-EFS traffic. The default value is PublicInternetOnly
.
*
* -
*
* PublicInternetOnly
- Non-EFS traffic is through a VPC managed by Amazon SageMaker, which
* allows direct internet access
*
*
* -
*
* VpcOnly
- All traffic is through the specified VPC and subnets
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppNetworkAccessType
*/
public DescribeDomainResult withAppNetworkAccessType(AppNetworkAccessType appNetworkAccessType) {
this.appNetworkAccessType = appNetworkAccessType.toString();
return this;
}
/**
*
* Use KmsKeyId
.
*
*
* @param homeEfsFileSystemKmsKeyId
* Use KmsKeyId
.
*/
@Deprecated
public void setHomeEfsFileSystemKmsKeyId(String homeEfsFileSystemKmsKeyId) {
this.homeEfsFileSystemKmsKeyId = homeEfsFileSystemKmsKeyId;
}
/**
*
* Use KmsKeyId
.
*
*
* @return Use KmsKeyId
.
*/
@Deprecated
public String getHomeEfsFileSystemKmsKeyId() {
return this.homeEfsFileSystemKmsKeyId;
}
/**
*
* Use KmsKeyId
.
*
*
* @param homeEfsFileSystemKmsKeyId
* Use KmsKeyId
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
@Deprecated
public DescribeDomainResult withHomeEfsFileSystemKmsKeyId(String homeEfsFileSystemKmsKeyId) {
setHomeEfsFileSystemKmsKeyId(homeEfsFileSystemKmsKeyId);
return this;
}
/**
*
* The VPC subnets that the domain uses for communication.
*
*
* @return The VPC subnets that the domain uses for communication.
*/
public java.util.List getSubnetIds() {
return subnetIds;
}
/**
*
* The VPC subnets that the domain uses for communication.
*
*
* @param subnetIds
* The VPC subnets that the domain uses for communication.
*/
public void setSubnetIds(java.util.Collection subnetIds) {
if (subnetIds == null) {
this.subnetIds = null;
return;
}
this.subnetIds = new java.util.ArrayList(subnetIds);
}
/**
*
* The VPC subnets that the domain uses for communication.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubnetIds(java.util.Collection)} or {@link #withSubnetIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param subnetIds
* The VPC subnets that the domain uses for communication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withSubnetIds(String... subnetIds) {
if (this.subnetIds == null) {
setSubnetIds(new java.util.ArrayList(subnetIds.length));
}
for (String ele : subnetIds) {
this.subnetIds.add(ele);
}
return this;
}
/**
*
* The VPC subnets that the domain uses for communication.
*
*
* @param subnetIds
* The VPC subnets that the domain uses for communication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withSubnetIds(java.util.Collection subnetIds) {
setSubnetIds(subnetIds);
return this;
}
/**
*
* The domain's URL.
*
*
* @param url
* The domain's URL.
*/
public void setUrl(String url) {
this.url = url;
}
/**
*
* The domain's URL.
*
*
* @return The domain's URL.
*/
public String getUrl() {
return this.url;
}
/**
*
* The domain's URL.
*
*
* @param url
* The domain's URL.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withUrl(String url) {
setUrl(url);
return this;
}
/**
*
* The ID of the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*
* @param vpcId
* The ID of the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*/
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
/**
*
* The ID of the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*
* @return The ID of the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*/
public String getVpcId() {
return this.vpcId;
}
/**
*
* The ID of the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*
* @param vpcId
* The ID of the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withVpcId(String vpcId) {
setVpcId(vpcId);
return this;
}
/**
*
* The Amazon Web Services KMS customer managed key used to encrypt the EFS volume attached to the domain.
*
*
* @param kmsKeyId
* The Amazon Web Services KMS customer managed key used to encrypt the EFS volume attached to the domain.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The Amazon Web Services KMS customer managed key used to encrypt the EFS volume attached to the domain.
*
*
* @return The Amazon Web Services KMS customer managed key used to encrypt the EFS volume attached to the domain.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The Amazon Web Services KMS customer managed key used to encrypt the EFS volume attached to the domain.
*
*
* @param kmsKeyId
* The Amazon Web Services KMS customer managed key used to encrypt the EFS volume attached to the domain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is VPCOnly
* and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided.
*
*
* @param appSecurityGroupManagement
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is
* VPCOnly
and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
* is provided.
* @see AppSecurityGroupManagement
*/
public void setAppSecurityGroupManagement(String appSecurityGroupManagement) {
this.appSecurityGroupManagement = appSecurityGroupManagement;
}
/**
*
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is VPCOnly
* and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided.
*
*
* @return The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is
* VPCOnly
and
* DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided.
* @see AppSecurityGroupManagement
*/
public String getAppSecurityGroupManagement() {
return this.appSecurityGroupManagement;
}
/**
*
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is VPCOnly
* and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided.
*
*
* @param appSecurityGroupManagement
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is
* VPCOnly
and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
* is provided.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppSecurityGroupManagement
*/
public DescribeDomainResult withAppSecurityGroupManagement(String appSecurityGroupManagement) {
setAppSecurityGroupManagement(appSecurityGroupManagement);
return this;
}
/**
*
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is VPCOnly
* and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
is provided.
*
*
* @param appSecurityGroupManagement
* The entity that creates and manages the required security groups for inter-app communication in
* VPCOnly
mode. Required when CreateDomain.AppNetworkAccessType
is
* VPCOnly
and DomainSettings.RStudioServerProDomainSettings.DomainExecutionRoleArn
* is provided.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AppSecurityGroupManagement
*/
public DescribeDomainResult withAppSecurityGroupManagement(AppSecurityGroupManagement appSecurityGroupManagement) {
this.appSecurityGroupManagement = appSecurityGroupManagement.toString();
return this;
}
/**
*
* The default settings used to create a space.
*
*
* @param defaultSpaceSettings
* The default settings used to create a space.
*/
public void setDefaultSpaceSettings(DefaultSpaceSettings defaultSpaceSettings) {
this.defaultSpaceSettings = defaultSpaceSettings;
}
/**
*
* The default settings used to create a space.
*
*
* @return The default settings used to create a space.
*/
public DefaultSpaceSettings getDefaultSpaceSettings() {
return this.defaultSpaceSettings;
}
/**
*
* The default settings used to create a space.
*
*
* @param defaultSpaceSettings
* The default settings used to create a space.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeDomainResult withDefaultSpaceSettings(DefaultSpaceSettings defaultSpaceSettings) {
setDefaultSpaceSettings(defaultSpaceSettings);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDomainArn() != null)
sb.append("DomainArn: ").append(getDomainArn()).append(",");
if (getDomainId() != null)
sb.append("DomainId: ").append(getDomainId()).append(",");
if (getDomainName() != null)
sb.append("DomainName: ").append(getDomainName()).append(",");
if (getHomeEfsFileSystemId() != null)
sb.append("HomeEfsFileSystemId: ").append(getHomeEfsFileSystemId()).append(",");
if (getSingleSignOnManagedApplicationInstanceId() != null)
sb.append("SingleSignOnManagedApplicationInstanceId: ").append(getSingleSignOnManagedApplicationInstanceId()).append(",");
if (getSingleSignOnApplicationArn() != null)
sb.append("SingleSignOnApplicationArn: ").append(getSingleSignOnApplicationArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getLastModifiedTime() != null)
sb.append("LastModifiedTime: ").append(getLastModifiedTime()).append(",");
if (getFailureReason() != null)
sb.append("FailureReason: ").append(getFailureReason()).append(",");
if (getSecurityGroupIdForDomainBoundary() != null)
sb.append("SecurityGroupIdForDomainBoundary: ").append(getSecurityGroupIdForDomainBoundary()).append(",");
if (getAuthMode() != null)
sb.append("AuthMode: ").append(getAuthMode()).append(",");
if (getDefaultUserSettings() != null)
sb.append("DefaultUserSettings: ").append(getDefaultUserSettings()).append(",");
if (getDomainSettings() != null)
sb.append("DomainSettings: ").append(getDomainSettings()).append(",");
if (getAppNetworkAccessType() != null)
sb.append("AppNetworkAccessType: ").append(getAppNetworkAccessType()).append(",");
if (getHomeEfsFileSystemKmsKeyId() != null)
sb.append("HomeEfsFileSystemKmsKeyId: ").append(getHomeEfsFileSystemKmsKeyId()).append(",");
if (getSubnetIds() != null)
sb.append("SubnetIds: ").append(getSubnetIds()).append(",");
if (getUrl() != null)
sb.append("Url: ").append(getUrl()).append(",");
if (getVpcId() != null)
sb.append("VpcId: ").append(getVpcId()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getAppSecurityGroupManagement() != null)
sb.append("AppSecurityGroupManagement: ").append(getAppSecurityGroupManagement()).append(",");
if (getDefaultSpaceSettings() != null)
sb.append("DefaultSpaceSettings: ").append(getDefaultSpaceSettings());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeDomainResult == false)
return false;
DescribeDomainResult other = (DescribeDomainResult) obj;
if (other.getDomainArn() == null ^ this.getDomainArn() == null)
return false;
if (other.getDomainArn() != null && other.getDomainArn().equals(this.getDomainArn()) == false)
return false;
if (other.getDomainId() == null ^ this.getDomainId() == null)
return false;
if (other.getDomainId() != null && other.getDomainId().equals(this.getDomainId()) == false)
return false;
if (other.getDomainName() == null ^ this.getDomainName() == null)
return false;
if (other.getDomainName() != null && other.getDomainName().equals(this.getDomainName()) == false)
return false;
if (other.getHomeEfsFileSystemId() == null ^ this.getHomeEfsFileSystemId() == null)
return false;
if (other.getHomeEfsFileSystemId() != null && other.getHomeEfsFileSystemId().equals(this.getHomeEfsFileSystemId()) == false)
return false;
if (other.getSingleSignOnManagedApplicationInstanceId() == null ^ this.getSingleSignOnManagedApplicationInstanceId() == null)
return false;
if (other.getSingleSignOnManagedApplicationInstanceId() != null
&& other.getSingleSignOnManagedApplicationInstanceId().equals(this.getSingleSignOnManagedApplicationInstanceId()) == false)
return false;
if (other.getSingleSignOnApplicationArn() == null ^ this.getSingleSignOnApplicationArn() == null)
return false;
if (other.getSingleSignOnApplicationArn() != null && other.getSingleSignOnApplicationArn().equals(this.getSingleSignOnApplicationArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getLastModifiedTime() == null ^ this.getLastModifiedTime() == null)
return false;
if (other.getLastModifiedTime() != null && other.getLastModifiedTime().equals(this.getLastModifiedTime()) == false)
return false;
if (other.getFailureReason() == null ^ this.getFailureReason() == null)
return false;
if (other.getFailureReason() != null && other.getFailureReason().equals(this.getFailureReason()) == false)
return false;
if (other.getSecurityGroupIdForDomainBoundary() == null ^ this.getSecurityGroupIdForDomainBoundary() == null)
return false;
if (other.getSecurityGroupIdForDomainBoundary() != null
&& other.getSecurityGroupIdForDomainBoundary().equals(this.getSecurityGroupIdForDomainBoundary()) == false)
return false;
if (other.getAuthMode() == null ^ this.getAuthMode() == null)
return false;
if (other.getAuthMode() != null && other.getAuthMode().equals(this.getAuthMode()) == false)
return false;
if (other.getDefaultUserSettings() == null ^ this.getDefaultUserSettings() == null)
return false;
if (other.getDefaultUserSettings() != null && other.getDefaultUserSettings().equals(this.getDefaultUserSettings()) == false)
return false;
if (other.getDomainSettings() == null ^ this.getDomainSettings() == null)
return false;
if (other.getDomainSettings() != null && other.getDomainSettings().equals(this.getDomainSettings()) == false)
return false;
if (other.getAppNetworkAccessType() == null ^ this.getAppNetworkAccessType() == null)
return false;
if (other.getAppNetworkAccessType() != null && other.getAppNetworkAccessType().equals(this.getAppNetworkAccessType()) == false)
return false;
if (other.getHomeEfsFileSystemKmsKeyId() == null ^ this.getHomeEfsFileSystemKmsKeyId() == null)
return false;
if (other.getHomeEfsFileSystemKmsKeyId() != null && other.getHomeEfsFileSystemKmsKeyId().equals(this.getHomeEfsFileSystemKmsKeyId()) == false)
return false;
if (other.getSubnetIds() == null ^ this.getSubnetIds() == null)
return false;
if (other.getSubnetIds() != null && other.getSubnetIds().equals(this.getSubnetIds()) == false)
return false;
if (other.getUrl() == null ^ this.getUrl() == null)
return false;
if (other.getUrl() != null && other.getUrl().equals(this.getUrl()) == false)
return false;
if (other.getVpcId() == null ^ this.getVpcId() == null)
return false;
if (other.getVpcId() != null && other.getVpcId().equals(this.getVpcId()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getAppSecurityGroupManagement() == null ^ this.getAppSecurityGroupManagement() == null)
return false;
if (other.getAppSecurityGroupManagement() != null && other.getAppSecurityGroupManagement().equals(this.getAppSecurityGroupManagement()) == false)
return false;
if (other.getDefaultSpaceSettings() == null ^ this.getDefaultSpaceSettings() == null)
return false;
if (other.getDefaultSpaceSettings() != null && other.getDefaultSpaceSettings().equals(this.getDefaultSpaceSettings()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDomainArn() == null) ? 0 : getDomainArn().hashCode());
hashCode = prime * hashCode + ((getDomainId() == null) ? 0 : getDomainId().hashCode());
hashCode = prime * hashCode + ((getDomainName() == null) ? 0 : getDomainName().hashCode());
hashCode = prime * hashCode + ((getHomeEfsFileSystemId() == null) ? 0 : getHomeEfsFileSystemId().hashCode());
hashCode = prime * hashCode + ((getSingleSignOnManagedApplicationInstanceId() == null) ? 0 : getSingleSignOnManagedApplicationInstanceId().hashCode());
hashCode = prime * hashCode + ((getSingleSignOnApplicationArn() == null) ? 0 : getSingleSignOnApplicationArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTime() == null) ? 0 : getLastModifiedTime().hashCode());
hashCode = prime * hashCode + ((getFailureReason() == null) ? 0 : getFailureReason().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIdForDomainBoundary() == null) ? 0 : getSecurityGroupIdForDomainBoundary().hashCode());
hashCode = prime * hashCode + ((getAuthMode() == null) ? 0 : getAuthMode().hashCode());
hashCode = prime * hashCode + ((getDefaultUserSettings() == null) ? 0 : getDefaultUserSettings().hashCode());
hashCode = prime * hashCode + ((getDomainSettings() == null) ? 0 : getDomainSettings().hashCode());
hashCode = prime * hashCode + ((getAppNetworkAccessType() == null) ? 0 : getAppNetworkAccessType().hashCode());
hashCode = prime * hashCode + ((getHomeEfsFileSystemKmsKeyId() == null) ? 0 : getHomeEfsFileSystemKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getSubnetIds() == null) ? 0 : getSubnetIds().hashCode());
hashCode = prime * hashCode + ((getUrl() == null) ? 0 : getUrl().hashCode());
hashCode = prime * hashCode + ((getVpcId() == null) ? 0 : getVpcId().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getAppSecurityGroupManagement() == null) ? 0 : getAppSecurityGroupManagement().hashCode());
hashCode = prime * hashCode + ((getDefaultSpaceSettings() == null) ? 0 : getDefaultSpaceSettings().hashCode());
return hashCode;
}
@Override
public DescribeDomainResult clone() {
try {
return (DescribeDomainResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}