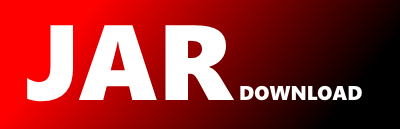
com.amazonaws.services.sagemaker.model.DescribeModelCardExportJobResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeModelCardExportJobResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the model card export job to describe.
*
*/
private String modelCardExportJobName;
/**
*
* The Amazon Resource Name (ARN) of the model card export job.
*
*/
private String modelCardExportJobArn;
/**
*
* The completion status of the model card export job.
*
*
* -
*
* InProgress
: The model card export job is in progress.
*
*
* -
*
* Completed
: The model card export job is complete.
*
*
* -
*
* Failed
: The model card export job failed. To see the reason for the failure, see the
* FailureReason
field in the response to a DescribeModelCardExportJob
call.
*
*
*
*/
private String status;
/**
*
* The name or Amazon Resource Name (ARN) of the model card that the model export job exports.
*
*/
private String modelCardName;
/**
*
* The version of the model card that the model export job exports.
*
*/
private Integer modelCardVersion;
/**
*
* The export output details for the model card.
*
*/
private ModelCardExportOutputConfig outputConfig;
/**
*
* The date and time that the model export job was created.
*
*/
private java.util.Date createdAt;
/**
*
* The date and time that the model export job was last modified.
*
*/
private java.util.Date lastModifiedAt;
/**
*
* The failure reason if the model export job fails.
*
*/
private String failureReason;
/**
*
* The exported model card artifacts.
*
*/
private ModelCardExportArtifacts exportArtifacts;
/**
*
* The name of the model card export job to describe.
*
*
* @param modelCardExportJobName
* The name of the model card export job to describe.
*/
public void setModelCardExportJobName(String modelCardExportJobName) {
this.modelCardExportJobName = modelCardExportJobName;
}
/**
*
* The name of the model card export job to describe.
*
*
* @return The name of the model card export job to describe.
*/
public String getModelCardExportJobName() {
return this.modelCardExportJobName;
}
/**
*
* The name of the model card export job to describe.
*
*
* @param modelCardExportJobName
* The name of the model card export job to describe.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelCardExportJobResult withModelCardExportJobName(String modelCardExportJobName) {
setModelCardExportJobName(modelCardExportJobName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the model card export job.
*
*
* @param modelCardExportJobArn
* The Amazon Resource Name (ARN) of the model card export job.
*/
public void setModelCardExportJobArn(String modelCardExportJobArn) {
this.modelCardExportJobArn = modelCardExportJobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model card export job.
*
*
* @return The Amazon Resource Name (ARN) of the model card export job.
*/
public String getModelCardExportJobArn() {
return this.modelCardExportJobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model card export job.
*
*
* @param modelCardExportJobArn
* The Amazon Resource Name (ARN) of the model card export job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelCardExportJobResult withModelCardExportJobArn(String modelCardExportJobArn) {
setModelCardExportJobArn(modelCardExportJobArn);
return this;
}
/**
*
* The completion status of the model card export job.
*
*
* -
*
* InProgress
: The model card export job is in progress.
*
*
* -
*
* Completed
: The model card export job is complete.
*
*
* -
*
* Failed
: The model card export job failed. To see the reason for the failure, see the
* FailureReason
field in the response to a DescribeModelCardExportJob
call.
*
*
*
*
* @param status
* The completion status of the model card export job.
*
* -
*
* InProgress
: The model card export job is in progress.
*
*
* -
*
* Completed
: The model card export job is complete.
*
*
* -
*
* Failed
: The model card export job failed. To see the reason for the failure, see the
* FailureReason
field in the response to a DescribeModelCardExportJob
call.
*
*
* @see ModelCardExportJobStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The completion status of the model card export job.
*
*
* -
*
* InProgress
: The model card export job is in progress.
*
*
* -
*
* Completed
: The model card export job is complete.
*
*
* -
*
* Failed
: The model card export job failed. To see the reason for the failure, see the
* FailureReason
field in the response to a DescribeModelCardExportJob
call.
*
*
*
*
* @return The completion status of the model card export job.
*
* -
*
* InProgress
: The model card export job is in progress.
*
*
* -
*
* Completed
: The model card export job is complete.
*
*
* -
*
* Failed
: The model card export job failed. To see the reason for the failure, see the
* FailureReason
field in the response to a DescribeModelCardExportJob
call.
*
*
* @see ModelCardExportJobStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The completion status of the model card export job.
*
*
* -
*
* InProgress
: The model card export job is in progress.
*
*
* -
*
* Completed
: The model card export job is complete.
*
*
* -
*
* Failed
: The model card export job failed. To see the reason for the failure, see the
* FailureReason
field in the response to a DescribeModelCardExportJob
call.
*
*
*
*
* @param status
* The completion status of the model card export job.
*
* -
*
* InProgress
: The model card export job is in progress.
*
*
* -
*
* Completed
: The model card export job is complete.
*
*
* -
*
* Failed
: The model card export job failed. To see the reason for the failure, see the
* FailureReason
field in the response to a DescribeModelCardExportJob
call.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelCardExportJobStatus
*/
public DescribeModelCardExportJobResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The completion status of the model card export job.
*
*
* -
*
* InProgress
: The model card export job is in progress.
*
*
* -
*
* Completed
: The model card export job is complete.
*
*
* -
*
* Failed
: The model card export job failed. To see the reason for the failure, see the
* FailureReason
field in the response to a DescribeModelCardExportJob
call.
*
*
*
*
* @param status
* The completion status of the model card export job.
*
* -
*
* InProgress
: The model card export job is in progress.
*
*
* -
*
* Completed
: The model card export job is complete.
*
*
* -
*
* Failed
: The model card export job failed. To see the reason for the failure, see the
* FailureReason
field in the response to a DescribeModelCardExportJob
call.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelCardExportJobStatus
*/
public DescribeModelCardExportJobResult withStatus(ModelCardExportJobStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The name or Amazon Resource Name (ARN) of the model card that the model export job exports.
*
*
* @param modelCardName
* The name or Amazon Resource Name (ARN) of the model card that the model export job exports.
*/
public void setModelCardName(String modelCardName) {
this.modelCardName = modelCardName;
}
/**
*
* The name or Amazon Resource Name (ARN) of the model card that the model export job exports.
*
*
* @return The name or Amazon Resource Name (ARN) of the model card that the model export job exports.
*/
public String getModelCardName() {
return this.modelCardName;
}
/**
*
* The name or Amazon Resource Name (ARN) of the model card that the model export job exports.
*
*
* @param modelCardName
* The name or Amazon Resource Name (ARN) of the model card that the model export job exports.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelCardExportJobResult withModelCardName(String modelCardName) {
setModelCardName(modelCardName);
return this;
}
/**
*
* The version of the model card that the model export job exports.
*
*
* @param modelCardVersion
* The version of the model card that the model export job exports.
*/
public void setModelCardVersion(Integer modelCardVersion) {
this.modelCardVersion = modelCardVersion;
}
/**
*
* The version of the model card that the model export job exports.
*
*
* @return The version of the model card that the model export job exports.
*/
public Integer getModelCardVersion() {
return this.modelCardVersion;
}
/**
*
* The version of the model card that the model export job exports.
*
*
* @param modelCardVersion
* The version of the model card that the model export job exports.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelCardExportJobResult withModelCardVersion(Integer modelCardVersion) {
setModelCardVersion(modelCardVersion);
return this;
}
/**
*
* The export output details for the model card.
*
*
* @param outputConfig
* The export output details for the model card.
*/
public void setOutputConfig(ModelCardExportOutputConfig outputConfig) {
this.outputConfig = outputConfig;
}
/**
*
* The export output details for the model card.
*
*
* @return The export output details for the model card.
*/
public ModelCardExportOutputConfig getOutputConfig() {
return this.outputConfig;
}
/**
*
* The export output details for the model card.
*
*
* @param outputConfig
* The export output details for the model card.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelCardExportJobResult withOutputConfig(ModelCardExportOutputConfig outputConfig) {
setOutputConfig(outputConfig);
return this;
}
/**
*
* The date and time that the model export job was created.
*
*
* @param createdAt
* The date and time that the model export job was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The date and time that the model export job was created.
*
*
* @return The date and time that the model export job was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The date and time that the model export job was created.
*
*
* @param createdAt
* The date and time that the model export job was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelCardExportJobResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The date and time that the model export job was last modified.
*
*
* @param lastModifiedAt
* The date and time that the model export job was last modified.
*/
public void setLastModifiedAt(java.util.Date lastModifiedAt) {
this.lastModifiedAt = lastModifiedAt;
}
/**
*
* The date and time that the model export job was last modified.
*
*
* @return The date and time that the model export job was last modified.
*/
public java.util.Date getLastModifiedAt() {
return this.lastModifiedAt;
}
/**
*
* The date and time that the model export job was last modified.
*
*
* @param lastModifiedAt
* The date and time that the model export job was last modified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelCardExportJobResult withLastModifiedAt(java.util.Date lastModifiedAt) {
setLastModifiedAt(lastModifiedAt);
return this;
}
/**
*
* The failure reason if the model export job fails.
*
*
* @param failureReason
* The failure reason if the model export job fails.
*/
public void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
/**
*
* The failure reason if the model export job fails.
*
*
* @return The failure reason if the model export job fails.
*/
public String getFailureReason() {
return this.failureReason;
}
/**
*
* The failure reason if the model export job fails.
*
*
* @param failureReason
* The failure reason if the model export job fails.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelCardExportJobResult withFailureReason(String failureReason) {
setFailureReason(failureReason);
return this;
}
/**
*
* The exported model card artifacts.
*
*
* @param exportArtifacts
* The exported model card artifacts.
*/
public void setExportArtifacts(ModelCardExportArtifacts exportArtifacts) {
this.exportArtifacts = exportArtifacts;
}
/**
*
* The exported model card artifacts.
*
*
* @return The exported model card artifacts.
*/
public ModelCardExportArtifacts getExportArtifacts() {
return this.exportArtifacts;
}
/**
*
* The exported model card artifacts.
*
*
* @param exportArtifacts
* The exported model card artifacts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelCardExportJobResult withExportArtifacts(ModelCardExportArtifacts exportArtifacts) {
setExportArtifacts(exportArtifacts);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getModelCardExportJobName() != null)
sb.append("ModelCardExportJobName: ").append(getModelCardExportJobName()).append(",");
if (getModelCardExportJobArn() != null)
sb.append("ModelCardExportJobArn: ").append(getModelCardExportJobArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getModelCardName() != null)
sb.append("ModelCardName: ").append(getModelCardName()).append(",");
if (getModelCardVersion() != null)
sb.append("ModelCardVersion: ").append(getModelCardVersion()).append(",");
if (getOutputConfig() != null)
sb.append("OutputConfig: ").append(getOutputConfig()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getLastModifiedAt() != null)
sb.append("LastModifiedAt: ").append(getLastModifiedAt()).append(",");
if (getFailureReason() != null)
sb.append("FailureReason: ").append(getFailureReason()).append(",");
if (getExportArtifacts() != null)
sb.append("ExportArtifacts: ").append(getExportArtifacts());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeModelCardExportJobResult == false)
return false;
DescribeModelCardExportJobResult other = (DescribeModelCardExportJobResult) obj;
if (other.getModelCardExportJobName() == null ^ this.getModelCardExportJobName() == null)
return false;
if (other.getModelCardExportJobName() != null && other.getModelCardExportJobName().equals(this.getModelCardExportJobName()) == false)
return false;
if (other.getModelCardExportJobArn() == null ^ this.getModelCardExportJobArn() == null)
return false;
if (other.getModelCardExportJobArn() != null && other.getModelCardExportJobArn().equals(this.getModelCardExportJobArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getModelCardName() == null ^ this.getModelCardName() == null)
return false;
if (other.getModelCardName() != null && other.getModelCardName().equals(this.getModelCardName()) == false)
return false;
if (other.getModelCardVersion() == null ^ this.getModelCardVersion() == null)
return false;
if (other.getModelCardVersion() != null && other.getModelCardVersion().equals(this.getModelCardVersion()) == false)
return false;
if (other.getOutputConfig() == null ^ this.getOutputConfig() == null)
return false;
if (other.getOutputConfig() != null && other.getOutputConfig().equals(this.getOutputConfig()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getLastModifiedAt() == null ^ this.getLastModifiedAt() == null)
return false;
if (other.getLastModifiedAt() != null && other.getLastModifiedAt().equals(this.getLastModifiedAt()) == false)
return false;
if (other.getFailureReason() == null ^ this.getFailureReason() == null)
return false;
if (other.getFailureReason() != null && other.getFailureReason().equals(this.getFailureReason()) == false)
return false;
if (other.getExportArtifacts() == null ^ this.getExportArtifacts() == null)
return false;
if (other.getExportArtifacts() != null && other.getExportArtifacts().equals(this.getExportArtifacts()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getModelCardExportJobName() == null) ? 0 : getModelCardExportJobName().hashCode());
hashCode = prime * hashCode + ((getModelCardExportJobArn() == null) ? 0 : getModelCardExportJobArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getModelCardName() == null) ? 0 : getModelCardName().hashCode());
hashCode = prime * hashCode + ((getModelCardVersion() == null) ? 0 : getModelCardVersion().hashCode());
hashCode = prime * hashCode + ((getOutputConfig() == null) ? 0 : getOutputConfig().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getLastModifiedAt() == null) ? 0 : getLastModifiedAt().hashCode());
hashCode = prime * hashCode + ((getFailureReason() == null) ? 0 : getFailureReason().hashCode());
hashCode = prime * hashCode + ((getExportArtifacts() == null) ? 0 : getExportArtifacts().hashCode());
return hashCode;
}
@Override
public DescribeModelCardExportJobResult clone() {
try {
return (DescribeModelCardExportJobResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}