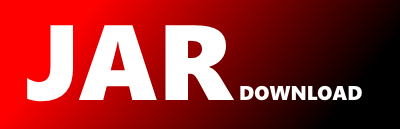
com.amazonaws.services.sagemaker.model.DescribeModelPackageResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeModelPackageResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the model package being described.
*
*/
private String modelPackageName;
/**
*
* If the model is a versioned model, the name of the model group that the versioned model belongs to.
*
*/
private String modelPackageGroupName;
/**
*
* The version of the model package.
*
*/
private Integer modelPackageVersion;
/**
*
* The Amazon Resource Name (ARN) of the model package.
*
*/
private String modelPackageArn;
/**
*
* A brief summary of the model package.
*
*/
private String modelPackageDescription;
/**
*
* A timestamp specifying when the model package was created.
*
*/
private java.util.Date creationTime;
/**
*
* Details about inference jobs that you can run with models based on this model package.
*
*/
private InferenceSpecification inferenceSpecification;
/**
*
* Details about the algorithm that was used to create the model package.
*
*/
private SourceAlgorithmSpecification sourceAlgorithmSpecification;
/**
*
* Configurations for one or more transform jobs that SageMaker runs to test the model package.
*
*/
private ModelPackageValidationSpecification validationSpecification;
/**
*
* The current status of the model package.
*
*/
private String modelPackageStatus;
/**
*
* Details about the current status of the model package.
*
*/
private ModelPackageStatusDetails modelPackageStatusDetails;
/**
*
* Whether the model package is certified for listing on Amazon Web Services Marketplace.
*
*/
private Boolean certifyForMarketplace;
/**
*
* The approval status of the model package.
*
*/
private String modelApprovalStatus;
private UserContext createdBy;
private MetadataProperties metadataProperties;
/**
*
* Metrics for the model.
*
*/
private ModelMetrics modelMetrics;
/**
*
* The last time that the model package was modified.
*
*/
private java.util.Date lastModifiedTime;
private UserContext lastModifiedBy;
/**
*
* A description provided for the model approval.
*
*/
private String approvalDescription;
/**
*
* The machine learning domain of the model package you specified. Common machine learning domains include computer
* vision and natural language processing.
*
*/
private String domain;
/**
*
* The machine learning task you specified that your model package accomplishes. Common machine learning tasks
* include object detection and image classification.
*
*/
private String task;
/**
*
* The Amazon Simple Storage Service (Amazon S3) path where the sample payload are stored. This path points to a
* single gzip compressed tar archive (.tar.gz suffix).
*
*/
private String samplePayloadUrl;
/**
*
* The metadata properties associated with the model package versions.
*
*/
private java.util.Map customerMetadataProperties;
/**
*
* Represents the drift check baselines that can be used when the model monitor is set using the model package. For
* more information, see the topic on Drift Detection against Previous Baselines in SageMaker Pipelines in the Amazon SageMaker Developer
* Guide.
*
*/
private DriftCheckBaselines driftCheckBaselines;
/**
*
* An array of additional Inference Specification objects. Each additional Inference Specification specifies
* artifacts based on this model package that can be used on inference endpoints. Generally used with SageMaker Neo
* to store the compiled artifacts.
*
*/
private java.util.List additionalInferenceSpecifications;
/**
*
* Indicates if you want to skip model validation.
*
*/
private String skipModelValidation;
/**
*
* The URI of the source for the model package.
*
*/
private String sourceUri;
/**
*
* The KMS Key ID (KMSKeyId
) used for encryption of model package information.
*
*/
private ModelPackageSecurityConfig securityConfig;
/**
*
* The model card associated with the model package. Since ModelPackageModelCard
is tied to a model
* package, it is a specific usage of a model card and its schema is simplified compared to the schema of
* ModelCard
. The ModelPackageModelCard
schema does not include
* model_package_details
, and model_overview
is composed of the model_creator
* and model_artifact
properties. For more information about the model package model card schema, see
* Model
* package model card schema. For more information about the model card associated with the model package, see
* View the Details of a Model
* Version.
*
*/
private ModelPackageModelCard modelCard;
/**
*
* The name of the model package being described.
*
*
* @param modelPackageName
* The name of the model package being described.
*/
public void setModelPackageName(String modelPackageName) {
this.modelPackageName = modelPackageName;
}
/**
*
* The name of the model package being described.
*
*
* @return The name of the model package being described.
*/
public String getModelPackageName() {
return this.modelPackageName;
}
/**
*
* The name of the model package being described.
*
*
* @param modelPackageName
* The name of the model package being described.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withModelPackageName(String modelPackageName) {
setModelPackageName(modelPackageName);
return this;
}
/**
*
* If the model is a versioned model, the name of the model group that the versioned model belongs to.
*
*
* @param modelPackageGroupName
* If the model is a versioned model, the name of the model group that the versioned model belongs to.
*/
public void setModelPackageGroupName(String modelPackageGroupName) {
this.modelPackageGroupName = modelPackageGroupName;
}
/**
*
* If the model is a versioned model, the name of the model group that the versioned model belongs to.
*
*
* @return If the model is a versioned model, the name of the model group that the versioned model belongs to.
*/
public String getModelPackageGroupName() {
return this.modelPackageGroupName;
}
/**
*
* If the model is a versioned model, the name of the model group that the versioned model belongs to.
*
*
* @param modelPackageGroupName
* If the model is a versioned model, the name of the model group that the versioned model belongs to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withModelPackageGroupName(String modelPackageGroupName) {
setModelPackageGroupName(modelPackageGroupName);
return this;
}
/**
*
* The version of the model package.
*
*
* @param modelPackageVersion
* The version of the model package.
*/
public void setModelPackageVersion(Integer modelPackageVersion) {
this.modelPackageVersion = modelPackageVersion;
}
/**
*
* The version of the model package.
*
*
* @return The version of the model package.
*/
public Integer getModelPackageVersion() {
return this.modelPackageVersion;
}
/**
*
* The version of the model package.
*
*
* @param modelPackageVersion
* The version of the model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withModelPackageVersion(Integer modelPackageVersion) {
setModelPackageVersion(modelPackageVersion);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the model package.
*
*
* @param modelPackageArn
* The Amazon Resource Name (ARN) of the model package.
*/
public void setModelPackageArn(String modelPackageArn) {
this.modelPackageArn = modelPackageArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model package.
*
*
* @return The Amazon Resource Name (ARN) of the model package.
*/
public String getModelPackageArn() {
return this.modelPackageArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model package.
*
*
* @param modelPackageArn
* The Amazon Resource Name (ARN) of the model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withModelPackageArn(String modelPackageArn) {
setModelPackageArn(modelPackageArn);
return this;
}
/**
*
* A brief summary of the model package.
*
*
* @param modelPackageDescription
* A brief summary of the model package.
*/
public void setModelPackageDescription(String modelPackageDescription) {
this.modelPackageDescription = modelPackageDescription;
}
/**
*
* A brief summary of the model package.
*
*
* @return A brief summary of the model package.
*/
public String getModelPackageDescription() {
return this.modelPackageDescription;
}
/**
*
* A brief summary of the model package.
*
*
* @param modelPackageDescription
* A brief summary of the model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withModelPackageDescription(String modelPackageDescription) {
setModelPackageDescription(modelPackageDescription);
return this;
}
/**
*
* A timestamp specifying when the model package was created.
*
*
* @param creationTime
* A timestamp specifying when the model package was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* A timestamp specifying when the model package was created.
*
*
* @return A timestamp specifying when the model package was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* A timestamp specifying when the model package was created.
*
*
* @param creationTime
* A timestamp specifying when the model package was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* Details about inference jobs that you can run with models based on this model package.
*
*
* @param inferenceSpecification
* Details about inference jobs that you can run with models based on this model package.
*/
public void setInferenceSpecification(InferenceSpecification inferenceSpecification) {
this.inferenceSpecification = inferenceSpecification;
}
/**
*
* Details about inference jobs that you can run with models based on this model package.
*
*
* @return Details about inference jobs that you can run with models based on this model package.
*/
public InferenceSpecification getInferenceSpecification() {
return this.inferenceSpecification;
}
/**
*
* Details about inference jobs that you can run with models based on this model package.
*
*
* @param inferenceSpecification
* Details about inference jobs that you can run with models based on this model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withInferenceSpecification(InferenceSpecification inferenceSpecification) {
setInferenceSpecification(inferenceSpecification);
return this;
}
/**
*
* Details about the algorithm that was used to create the model package.
*
*
* @param sourceAlgorithmSpecification
* Details about the algorithm that was used to create the model package.
*/
public void setSourceAlgorithmSpecification(SourceAlgorithmSpecification sourceAlgorithmSpecification) {
this.sourceAlgorithmSpecification = sourceAlgorithmSpecification;
}
/**
*
* Details about the algorithm that was used to create the model package.
*
*
* @return Details about the algorithm that was used to create the model package.
*/
public SourceAlgorithmSpecification getSourceAlgorithmSpecification() {
return this.sourceAlgorithmSpecification;
}
/**
*
* Details about the algorithm that was used to create the model package.
*
*
* @param sourceAlgorithmSpecification
* Details about the algorithm that was used to create the model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withSourceAlgorithmSpecification(SourceAlgorithmSpecification sourceAlgorithmSpecification) {
setSourceAlgorithmSpecification(sourceAlgorithmSpecification);
return this;
}
/**
*
* Configurations for one or more transform jobs that SageMaker runs to test the model package.
*
*
* @param validationSpecification
* Configurations for one or more transform jobs that SageMaker runs to test the model package.
*/
public void setValidationSpecification(ModelPackageValidationSpecification validationSpecification) {
this.validationSpecification = validationSpecification;
}
/**
*
* Configurations for one or more transform jobs that SageMaker runs to test the model package.
*
*
* @return Configurations for one or more transform jobs that SageMaker runs to test the model package.
*/
public ModelPackageValidationSpecification getValidationSpecification() {
return this.validationSpecification;
}
/**
*
* Configurations for one or more transform jobs that SageMaker runs to test the model package.
*
*
* @param validationSpecification
* Configurations for one or more transform jobs that SageMaker runs to test the model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withValidationSpecification(ModelPackageValidationSpecification validationSpecification) {
setValidationSpecification(validationSpecification);
return this;
}
/**
*
* The current status of the model package.
*
*
* @param modelPackageStatus
* The current status of the model package.
* @see ModelPackageStatus
*/
public void setModelPackageStatus(String modelPackageStatus) {
this.modelPackageStatus = modelPackageStatus;
}
/**
*
* The current status of the model package.
*
*
* @return The current status of the model package.
* @see ModelPackageStatus
*/
public String getModelPackageStatus() {
return this.modelPackageStatus;
}
/**
*
* The current status of the model package.
*
*
* @param modelPackageStatus
* The current status of the model package.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelPackageStatus
*/
public DescribeModelPackageResult withModelPackageStatus(String modelPackageStatus) {
setModelPackageStatus(modelPackageStatus);
return this;
}
/**
*
* The current status of the model package.
*
*
* @param modelPackageStatus
* The current status of the model package.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelPackageStatus
*/
public DescribeModelPackageResult withModelPackageStatus(ModelPackageStatus modelPackageStatus) {
this.modelPackageStatus = modelPackageStatus.toString();
return this;
}
/**
*
* Details about the current status of the model package.
*
*
* @param modelPackageStatusDetails
* Details about the current status of the model package.
*/
public void setModelPackageStatusDetails(ModelPackageStatusDetails modelPackageStatusDetails) {
this.modelPackageStatusDetails = modelPackageStatusDetails;
}
/**
*
* Details about the current status of the model package.
*
*
* @return Details about the current status of the model package.
*/
public ModelPackageStatusDetails getModelPackageStatusDetails() {
return this.modelPackageStatusDetails;
}
/**
*
* Details about the current status of the model package.
*
*
* @param modelPackageStatusDetails
* Details about the current status of the model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withModelPackageStatusDetails(ModelPackageStatusDetails modelPackageStatusDetails) {
setModelPackageStatusDetails(modelPackageStatusDetails);
return this;
}
/**
*
* Whether the model package is certified for listing on Amazon Web Services Marketplace.
*
*
* @param certifyForMarketplace
* Whether the model package is certified for listing on Amazon Web Services Marketplace.
*/
public void setCertifyForMarketplace(Boolean certifyForMarketplace) {
this.certifyForMarketplace = certifyForMarketplace;
}
/**
*
* Whether the model package is certified for listing on Amazon Web Services Marketplace.
*
*
* @return Whether the model package is certified for listing on Amazon Web Services Marketplace.
*/
public Boolean getCertifyForMarketplace() {
return this.certifyForMarketplace;
}
/**
*
* Whether the model package is certified for listing on Amazon Web Services Marketplace.
*
*
* @param certifyForMarketplace
* Whether the model package is certified for listing on Amazon Web Services Marketplace.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withCertifyForMarketplace(Boolean certifyForMarketplace) {
setCertifyForMarketplace(certifyForMarketplace);
return this;
}
/**
*
* Whether the model package is certified for listing on Amazon Web Services Marketplace.
*
*
* @return Whether the model package is certified for listing on Amazon Web Services Marketplace.
*/
public Boolean isCertifyForMarketplace() {
return this.certifyForMarketplace;
}
/**
*
* The approval status of the model package.
*
*
* @param modelApprovalStatus
* The approval status of the model package.
* @see ModelApprovalStatus
*/
public void setModelApprovalStatus(String modelApprovalStatus) {
this.modelApprovalStatus = modelApprovalStatus;
}
/**
*
* The approval status of the model package.
*
*
* @return The approval status of the model package.
* @see ModelApprovalStatus
*/
public String getModelApprovalStatus() {
return this.modelApprovalStatus;
}
/**
*
* The approval status of the model package.
*
*
* @param modelApprovalStatus
* The approval status of the model package.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelApprovalStatus
*/
public DescribeModelPackageResult withModelApprovalStatus(String modelApprovalStatus) {
setModelApprovalStatus(modelApprovalStatus);
return this;
}
/**
*
* The approval status of the model package.
*
*
* @param modelApprovalStatus
* The approval status of the model package.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelApprovalStatus
*/
public DescribeModelPackageResult withModelApprovalStatus(ModelApprovalStatus modelApprovalStatus) {
this.modelApprovalStatus = modelApprovalStatus.toString();
return this;
}
/**
* @param createdBy
*/
public void setCreatedBy(UserContext createdBy) {
this.createdBy = createdBy;
}
/**
* @return
*/
public UserContext getCreatedBy() {
return this.createdBy;
}
/**
* @param createdBy
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withCreatedBy(UserContext createdBy) {
setCreatedBy(createdBy);
return this;
}
/**
* @param metadataProperties
*/
public void setMetadataProperties(MetadataProperties metadataProperties) {
this.metadataProperties = metadataProperties;
}
/**
* @return
*/
public MetadataProperties getMetadataProperties() {
return this.metadataProperties;
}
/**
* @param metadataProperties
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withMetadataProperties(MetadataProperties metadataProperties) {
setMetadataProperties(metadataProperties);
return this;
}
/**
*
* Metrics for the model.
*
*
* @param modelMetrics
* Metrics for the model.
*/
public void setModelMetrics(ModelMetrics modelMetrics) {
this.modelMetrics = modelMetrics;
}
/**
*
* Metrics for the model.
*
*
* @return Metrics for the model.
*/
public ModelMetrics getModelMetrics() {
return this.modelMetrics;
}
/**
*
* Metrics for the model.
*
*
* @param modelMetrics
* Metrics for the model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withModelMetrics(ModelMetrics modelMetrics) {
setModelMetrics(modelMetrics);
return this;
}
/**
*
* The last time that the model package was modified.
*
*
* @param lastModifiedTime
* The last time that the model package was modified.
*/
public void setLastModifiedTime(java.util.Date lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
/**
*
* The last time that the model package was modified.
*
*
* @return The last time that the model package was modified.
*/
public java.util.Date getLastModifiedTime() {
return this.lastModifiedTime;
}
/**
*
* The last time that the model package was modified.
*
*
* @param lastModifiedTime
* The last time that the model package was modified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withLastModifiedTime(java.util.Date lastModifiedTime) {
setLastModifiedTime(lastModifiedTime);
return this;
}
/**
* @param lastModifiedBy
*/
public void setLastModifiedBy(UserContext lastModifiedBy) {
this.lastModifiedBy = lastModifiedBy;
}
/**
* @return
*/
public UserContext getLastModifiedBy() {
return this.lastModifiedBy;
}
/**
* @param lastModifiedBy
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withLastModifiedBy(UserContext lastModifiedBy) {
setLastModifiedBy(lastModifiedBy);
return this;
}
/**
*
* A description provided for the model approval.
*
*
* @param approvalDescription
* A description provided for the model approval.
*/
public void setApprovalDescription(String approvalDescription) {
this.approvalDescription = approvalDescription;
}
/**
*
* A description provided for the model approval.
*
*
* @return A description provided for the model approval.
*/
public String getApprovalDescription() {
return this.approvalDescription;
}
/**
*
* A description provided for the model approval.
*
*
* @param approvalDescription
* A description provided for the model approval.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withApprovalDescription(String approvalDescription) {
setApprovalDescription(approvalDescription);
return this;
}
/**
*
* The machine learning domain of the model package you specified. Common machine learning domains include computer
* vision and natural language processing.
*
*
* @param domain
* The machine learning domain of the model package you specified. Common machine learning domains include
* computer vision and natural language processing.
*/
public void setDomain(String domain) {
this.domain = domain;
}
/**
*
* The machine learning domain of the model package you specified. Common machine learning domains include computer
* vision and natural language processing.
*
*
* @return The machine learning domain of the model package you specified. Common machine learning domains include
* computer vision and natural language processing.
*/
public String getDomain() {
return this.domain;
}
/**
*
* The machine learning domain of the model package you specified. Common machine learning domains include computer
* vision and natural language processing.
*
*
* @param domain
* The machine learning domain of the model package you specified. Common machine learning domains include
* computer vision and natural language processing.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withDomain(String domain) {
setDomain(domain);
return this;
}
/**
*
* The machine learning task you specified that your model package accomplishes. Common machine learning tasks
* include object detection and image classification.
*
*
* @param task
* The machine learning task you specified that your model package accomplishes. Common machine learning
* tasks include object detection and image classification.
*/
public void setTask(String task) {
this.task = task;
}
/**
*
* The machine learning task you specified that your model package accomplishes. Common machine learning tasks
* include object detection and image classification.
*
*
* @return The machine learning task you specified that your model package accomplishes. Common machine learning
* tasks include object detection and image classification.
*/
public String getTask() {
return this.task;
}
/**
*
* The machine learning task you specified that your model package accomplishes. Common machine learning tasks
* include object detection and image classification.
*
*
* @param task
* The machine learning task you specified that your model package accomplishes. Common machine learning
* tasks include object detection and image classification.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withTask(String task) {
setTask(task);
return this;
}
/**
*
* The Amazon Simple Storage Service (Amazon S3) path where the sample payload are stored. This path points to a
* single gzip compressed tar archive (.tar.gz suffix).
*
*
* @param samplePayloadUrl
* The Amazon Simple Storage Service (Amazon S3) path where the sample payload are stored. This path points
* to a single gzip compressed tar archive (.tar.gz suffix).
*/
public void setSamplePayloadUrl(String samplePayloadUrl) {
this.samplePayloadUrl = samplePayloadUrl;
}
/**
*
* The Amazon Simple Storage Service (Amazon S3) path where the sample payload are stored. This path points to a
* single gzip compressed tar archive (.tar.gz suffix).
*
*
* @return The Amazon Simple Storage Service (Amazon S3) path where the sample payload are stored. This path points
* to a single gzip compressed tar archive (.tar.gz suffix).
*/
public String getSamplePayloadUrl() {
return this.samplePayloadUrl;
}
/**
*
* The Amazon Simple Storage Service (Amazon S3) path where the sample payload are stored. This path points to a
* single gzip compressed tar archive (.tar.gz suffix).
*
*
* @param samplePayloadUrl
* The Amazon Simple Storage Service (Amazon S3) path where the sample payload are stored. This path points
* to a single gzip compressed tar archive (.tar.gz suffix).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withSamplePayloadUrl(String samplePayloadUrl) {
setSamplePayloadUrl(samplePayloadUrl);
return this;
}
/**
*
* The metadata properties associated with the model package versions.
*
*
* @return The metadata properties associated with the model package versions.
*/
public java.util.Map getCustomerMetadataProperties() {
return customerMetadataProperties;
}
/**
*
* The metadata properties associated with the model package versions.
*
*
* @param customerMetadataProperties
* The metadata properties associated with the model package versions.
*/
public void setCustomerMetadataProperties(java.util.Map customerMetadataProperties) {
this.customerMetadataProperties = customerMetadataProperties;
}
/**
*
* The metadata properties associated with the model package versions.
*
*
* @param customerMetadataProperties
* The metadata properties associated with the model package versions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withCustomerMetadataProperties(java.util.Map customerMetadataProperties) {
setCustomerMetadataProperties(customerMetadataProperties);
return this;
}
/**
* Add a single CustomerMetadataProperties entry
*
* @see DescribeModelPackageResult#withCustomerMetadataProperties
* @returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult addCustomerMetadataPropertiesEntry(String key, String value) {
if (null == this.customerMetadataProperties) {
this.customerMetadataProperties = new java.util.HashMap();
}
if (this.customerMetadataProperties.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.customerMetadataProperties.put(key, value);
return this;
}
/**
* Removes all the entries added into CustomerMetadataProperties.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult clearCustomerMetadataPropertiesEntries() {
this.customerMetadataProperties = null;
return this;
}
/**
*
* Represents the drift check baselines that can be used when the model monitor is set using the model package. For
* more information, see the topic on Drift Detection against Previous Baselines in SageMaker Pipelines in the Amazon SageMaker Developer
* Guide.
*
*
* @param driftCheckBaselines
* Represents the drift check baselines that can be used when the model monitor is set using the model
* package. For more information, see the topic on Drift Detection against Previous Baselines in SageMaker Pipelines in the Amazon SageMaker
* Developer Guide.
*/
public void setDriftCheckBaselines(DriftCheckBaselines driftCheckBaselines) {
this.driftCheckBaselines = driftCheckBaselines;
}
/**
*
* Represents the drift check baselines that can be used when the model monitor is set using the model package. For
* more information, see the topic on Drift Detection against Previous Baselines in SageMaker Pipelines in the Amazon SageMaker Developer
* Guide.
*
*
* @return Represents the drift check baselines that can be used when the model monitor is set using the model
* package. For more information, see the topic on Drift Detection against Previous Baselines in SageMaker Pipelines in the Amazon SageMaker
* Developer Guide.
*/
public DriftCheckBaselines getDriftCheckBaselines() {
return this.driftCheckBaselines;
}
/**
*
* Represents the drift check baselines that can be used when the model monitor is set using the model package. For
* more information, see the topic on Drift Detection against Previous Baselines in SageMaker Pipelines in the Amazon SageMaker Developer
* Guide.
*
*
* @param driftCheckBaselines
* Represents the drift check baselines that can be used when the model monitor is set using the model
* package. For more information, see the topic on Drift Detection against Previous Baselines in SageMaker Pipelines in the Amazon SageMaker
* Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withDriftCheckBaselines(DriftCheckBaselines driftCheckBaselines) {
setDriftCheckBaselines(driftCheckBaselines);
return this;
}
/**
*
* An array of additional Inference Specification objects. Each additional Inference Specification specifies
* artifacts based on this model package that can be used on inference endpoints. Generally used with SageMaker Neo
* to store the compiled artifacts.
*
*
* @return An array of additional Inference Specification objects. Each additional Inference Specification specifies
* artifacts based on this model package that can be used on inference endpoints. Generally used with
* SageMaker Neo to store the compiled artifacts.
*/
public java.util.List getAdditionalInferenceSpecifications() {
return additionalInferenceSpecifications;
}
/**
*
* An array of additional Inference Specification objects. Each additional Inference Specification specifies
* artifacts based on this model package that can be used on inference endpoints. Generally used with SageMaker Neo
* to store the compiled artifacts.
*
*
* @param additionalInferenceSpecifications
* An array of additional Inference Specification objects. Each additional Inference Specification specifies
* artifacts based on this model package that can be used on inference endpoints. Generally used with
* SageMaker Neo to store the compiled artifacts.
*/
public void setAdditionalInferenceSpecifications(java.util.Collection additionalInferenceSpecifications) {
if (additionalInferenceSpecifications == null) {
this.additionalInferenceSpecifications = null;
return;
}
this.additionalInferenceSpecifications = new java.util.ArrayList(additionalInferenceSpecifications);
}
/**
*
* An array of additional Inference Specification objects. Each additional Inference Specification specifies
* artifacts based on this model package that can be used on inference endpoints. Generally used with SageMaker Neo
* to store the compiled artifacts.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdditionalInferenceSpecifications(java.util.Collection)} or
* {@link #withAdditionalInferenceSpecifications(java.util.Collection)} if you want to override the existing values.
*
*
* @param additionalInferenceSpecifications
* An array of additional Inference Specification objects. Each additional Inference Specification specifies
* artifacts based on this model package that can be used on inference endpoints. Generally used with
* SageMaker Neo to store the compiled artifacts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withAdditionalInferenceSpecifications(AdditionalInferenceSpecificationDefinition... additionalInferenceSpecifications) {
if (this.additionalInferenceSpecifications == null) {
setAdditionalInferenceSpecifications(new java.util.ArrayList(additionalInferenceSpecifications.length));
}
for (AdditionalInferenceSpecificationDefinition ele : additionalInferenceSpecifications) {
this.additionalInferenceSpecifications.add(ele);
}
return this;
}
/**
*
* An array of additional Inference Specification objects. Each additional Inference Specification specifies
* artifacts based on this model package that can be used on inference endpoints. Generally used with SageMaker Neo
* to store the compiled artifacts.
*
*
* @param additionalInferenceSpecifications
* An array of additional Inference Specification objects. Each additional Inference Specification specifies
* artifacts based on this model package that can be used on inference endpoints. Generally used with
* SageMaker Neo to store the compiled artifacts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withAdditionalInferenceSpecifications(
java.util.Collection additionalInferenceSpecifications) {
setAdditionalInferenceSpecifications(additionalInferenceSpecifications);
return this;
}
/**
*
* Indicates if you want to skip model validation.
*
*
* @param skipModelValidation
* Indicates if you want to skip model validation.
* @see SkipModelValidation
*/
public void setSkipModelValidation(String skipModelValidation) {
this.skipModelValidation = skipModelValidation;
}
/**
*
* Indicates if you want to skip model validation.
*
*
* @return Indicates if you want to skip model validation.
* @see SkipModelValidation
*/
public String getSkipModelValidation() {
return this.skipModelValidation;
}
/**
*
* Indicates if you want to skip model validation.
*
*
* @param skipModelValidation
* Indicates if you want to skip model validation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SkipModelValidation
*/
public DescribeModelPackageResult withSkipModelValidation(String skipModelValidation) {
setSkipModelValidation(skipModelValidation);
return this;
}
/**
*
* Indicates if you want to skip model validation.
*
*
* @param skipModelValidation
* Indicates if you want to skip model validation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SkipModelValidation
*/
public DescribeModelPackageResult withSkipModelValidation(SkipModelValidation skipModelValidation) {
this.skipModelValidation = skipModelValidation.toString();
return this;
}
/**
*
* The URI of the source for the model package.
*
*
* @param sourceUri
* The URI of the source for the model package.
*/
public void setSourceUri(String sourceUri) {
this.sourceUri = sourceUri;
}
/**
*
* The URI of the source for the model package.
*
*
* @return The URI of the source for the model package.
*/
public String getSourceUri() {
return this.sourceUri;
}
/**
*
* The URI of the source for the model package.
*
*
* @param sourceUri
* The URI of the source for the model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withSourceUri(String sourceUri) {
setSourceUri(sourceUri);
return this;
}
/**
*
* The KMS Key ID (KMSKeyId
) used for encryption of model package information.
*
*
* @param securityConfig
* The KMS Key ID (KMSKeyId
) used for encryption of model package information.
*/
public void setSecurityConfig(ModelPackageSecurityConfig securityConfig) {
this.securityConfig = securityConfig;
}
/**
*
* The KMS Key ID (KMSKeyId
) used for encryption of model package information.
*
*
* @return The KMS Key ID (KMSKeyId
) used for encryption of model package information.
*/
public ModelPackageSecurityConfig getSecurityConfig() {
return this.securityConfig;
}
/**
*
* The KMS Key ID (KMSKeyId
) used for encryption of model package information.
*
*
* @param securityConfig
* The KMS Key ID (KMSKeyId
) used for encryption of model package information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withSecurityConfig(ModelPackageSecurityConfig securityConfig) {
setSecurityConfig(securityConfig);
return this;
}
/**
*
* The model card associated with the model package. Since ModelPackageModelCard
is tied to a model
* package, it is a specific usage of a model card and its schema is simplified compared to the schema of
* ModelCard
. The ModelPackageModelCard
schema does not include
* model_package_details
, and model_overview
is composed of the model_creator
* and model_artifact
properties. For more information about the model package model card schema, see
* Model
* package model card schema. For more information about the model card associated with the model package, see
* View the Details of a Model
* Version.
*
*
* @param modelCard
* The model card associated with the model package. Since ModelPackageModelCard
is tied to a
* model package, it is a specific usage of a model card and its schema is simplified compared to the schema
* of ModelCard
. The ModelPackageModelCard
schema does not include
* model_package_details
, and model_overview
is composed of the
* model_creator
and model_artifact
properties. For more information about the
* model package model card schema, see Model
* package model card schema. For more information about the model card associated with the model
* package, see View
* the Details of a Model Version.
*/
public void setModelCard(ModelPackageModelCard modelCard) {
this.modelCard = modelCard;
}
/**
*
* The model card associated with the model package. Since ModelPackageModelCard
is tied to a model
* package, it is a specific usage of a model card and its schema is simplified compared to the schema of
* ModelCard
. The ModelPackageModelCard
schema does not include
* model_package_details
, and model_overview
is composed of the model_creator
* and model_artifact
properties. For more information about the model package model card schema, see
* Model
* package model card schema. For more information about the model card associated with the model package, see
* View the Details of a Model
* Version.
*
*
* @return The model card associated with the model package. Since ModelPackageModelCard
is tied to a
* model package, it is a specific usage of a model card and its schema is simplified compared to the schema
* of ModelCard
. The ModelPackageModelCard
schema does not include
* model_package_details
, and model_overview
is composed of the
* model_creator
and model_artifact
properties. For more information about the
* model package model card schema, see Model package model card schema. For more information about the model card associated with the model
* package, see View
* the Details of a Model Version.
*/
public ModelPackageModelCard getModelCard() {
return this.modelCard;
}
/**
*
* The model card associated with the model package. Since ModelPackageModelCard
is tied to a model
* package, it is a specific usage of a model card and its schema is simplified compared to the schema of
* ModelCard
. The ModelPackageModelCard
schema does not include
* model_package_details
, and model_overview
is composed of the model_creator
* and model_artifact
properties. For more information about the model package model card schema, see
* Model
* package model card schema. For more information about the model card associated with the model package, see
* View the Details of a Model
* Version.
*
*
* @param modelCard
* The model card associated with the model package. Since ModelPackageModelCard
is tied to a
* model package, it is a specific usage of a model card and its schema is simplified compared to the schema
* of ModelCard
. The ModelPackageModelCard
schema does not include
* model_package_details
, and model_overview
is composed of the
* model_creator
and model_artifact
properties. For more information about the
* model package model card schema, see Model
* package model card schema. For more information about the model card associated with the model
* package, see View
* the Details of a Model Version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeModelPackageResult withModelCard(ModelPackageModelCard modelCard) {
setModelCard(modelCard);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getModelPackageName() != null)
sb.append("ModelPackageName: ").append(getModelPackageName()).append(",");
if (getModelPackageGroupName() != null)
sb.append("ModelPackageGroupName: ").append(getModelPackageGroupName()).append(",");
if (getModelPackageVersion() != null)
sb.append("ModelPackageVersion: ").append(getModelPackageVersion()).append(",");
if (getModelPackageArn() != null)
sb.append("ModelPackageArn: ").append(getModelPackageArn()).append(",");
if (getModelPackageDescription() != null)
sb.append("ModelPackageDescription: ").append(getModelPackageDescription()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getInferenceSpecification() != null)
sb.append("InferenceSpecification: ").append(getInferenceSpecification()).append(",");
if (getSourceAlgorithmSpecification() != null)
sb.append("SourceAlgorithmSpecification: ").append(getSourceAlgorithmSpecification()).append(",");
if (getValidationSpecification() != null)
sb.append("ValidationSpecification: ").append(getValidationSpecification()).append(",");
if (getModelPackageStatus() != null)
sb.append("ModelPackageStatus: ").append(getModelPackageStatus()).append(",");
if (getModelPackageStatusDetails() != null)
sb.append("ModelPackageStatusDetails: ").append(getModelPackageStatusDetails()).append(",");
if (getCertifyForMarketplace() != null)
sb.append("CertifyForMarketplace: ").append(getCertifyForMarketplace()).append(",");
if (getModelApprovalStatus() != null)
sb.append("ModelApprovalStatus: ").append(getModelApprovalStatus()).append(",");
if (getCreatedBy() != null)
sb.append("CreatedBy: ").append(getCreatedBy()).append(",");
if (getMetadataProperties() != null)
sb.append("MetadataProperties: ").append(getMetadataProperties()).append(",");
if (getModelMetrics() != null)
sb.append("ModelMetrics: ").append(getModelMetrics()).append(",");
if (getLastModifiedTime() != null)
sb.append("LastModifiedTime: ").append(getLastModifiedTime()).append(",");
if (getLastModifiedBy() != null)
sb.append("LastModifiedBy: ").append(getLastModifiedBy()).append(",");
if (getApprovalDescription() != null)
sb.append("ApprovalDescription: ").append(getApprovalDescription()).append(",");
if (getDomain() != null)
sb.append("Domain: ").append(getDomain()).append(",");
if (getTask() != null)
sb.append("Task: ").append(getTask()).append(",");
if (getSamplePayloadUrl() != null)
sb.append("SamplePayloadUrl: ").append(getSamplePayloadUrl()).append(",");
if (getCustomerMetadataProperties() != null)
sb.append("CustomerMetadataProperties: ").append(getCustomerMetadataProperties()).append(",");
if (getDriftCheckBaselines() != null)
sb.append("DriftCheckBaselines: ").append(getDriftCheckBaselines()).append(",");
if (getAdditionalInferenceSpecifications() != null)
sb.append("AdditionalInferenceSpecifications: ").append(getAdditionalInferenceSpecifications()).append(",");
if (getSkipModelValidation() != null)
sb.append("SkipModelValidation: ").append(getSkipModelValidation()).append(",");
if (getSourceUri() != null)
sb.append("SourceUri: ").append(getSourceUri()).append(",");
if (getSecurityConfig() != null)
sb.append("SecurityConfig: ").append(getSecurityConfig()).append(",");
if (getModelCard() != null)
sb.append("ModelCard: ").append(getModelCard());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeModelPackageResult == false)
return false;
DescribeModelPackageResult other = (DescribeModelPackageResult) obj;
if (other.getModelPackageName() == null ^ this.getModelPackageName() == null)
return false;
if (other.getModelPackageName() != null && other.getModelPackageName().equals(this.getModelPackageName()) == false)
return false;
if (other.getModelPackageGroupName() == null ^ this.getModelPackageGroupName() == null)
return false;
if (other.getModelPackageGroupName() != null && other.getModelPackageGroupName().equals(this.getModelPackageGroupName()) == false)
return false;
if (other.getModelPackageVersion() == null ^ this.getModelPackageVersion() == null)
return false;
if (other.getModelPackageVersion() != null && other.getModelPackageVersion().equals(this.getModelPackageVersion()) == false)
return false;
if (other.getModelPackageArn() == null ^ this.getModelPackageArn() == null)
return false;
if (other.getModelPackageArn() != null && other.getModelPackageArn().equals(this.getModelPackageArn()) == false)
return false;
if (other.getModelPackageDescription() == null ^ this.getModelPackageDescription() == null)
return false;
if (other.getModelPackageDescription() != null && other.getModelPackageDescription().equals(this.getModelPackageDescription()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getInferenceSpecification() == null ^ this.getInferenceSpecification() == null)
return false;
if (other.getInferenceSpecification() != null && other.getInferenceSpecification().equals(this.getInferenceSpecification()) == false)
return false;
if (other.getSourceAlgorithmSpecification() == null ^ this.getSourceAlgorithmSpecification() == null)
return false;
if (other.getSourceAlgorithmSpecification() != null && other.getSourceAlgorithmSpecification().equals(this.getSourceAlgorithmSpecification()) == false)
return false;
if (other.getValidationSpecification() == null ^ this.getValidationSpecification() == null)
return false;
if (other.getValidationSpecification() != null && other.getValidationSpecification().equals(this.getValidationSpecification()) == false)
return false;
if (other.getModelPackageStatus() == null ^ this.getModelPackageStatus() == null)
return false;
if (other.getModelPackageStatus() != null && other.getModelPackageStatus().equals(this.getModelPackageStatus()) == false)
return false;
if (other.getModelPackageStatusDetails() == null ^ this.getModelPackageStatusDetails() == null)
return false;
if (other.getModelPackageStatusDetails() != null && other.getModelPackageStatusDetails().equals(this.getModelPackageStatusDetails()) == false)
return false;
if (other.getCertifyForMarketplace() == null ^ this.getCertifyForMarketplace() == null)
return false;
if (other.getCertifyForMarketplace() != null && other.getCertifyForMarketplace().equals(this.getCertifyForMarketplace()) == false)
return false;
if (other.getModelApprovalStatus() == null ^ this.getModelApprovalStatus() == null)
return false;
if (other.getModelApprovalStatus() != null && other.getModelApprovalStatus().equals(this.getModelApprovalStatus()) == false)
return false;
if (other.getCreatedBy() == null ^ this.getCreatedBy() == null)
return false;
if (other.getCreatedBy() != null && other.getCreatedBy().equals(this.getCreatedBy()) == false)
return false;
if (other.getMetadataProperties() == null ^ this.getMetadataProperties() == null)
return false;
if (other.getMetadataProperties() != null && other.getMetadataProperties().equals(this.getMetadataProperties()) == false)
return false;
if (other.getModelMetrics() == null ^ this.getModelMetrics() == null)
return false;
if (other.getModelMetrics() != null && other.getModelMetrics().equals(this.getModelMetrics()) == false)
return false;
if (other.getLastModifiedTime() == null ^ this.getLastModifiedTime() == null)
return false;
if (other.getLastModifiedTime() != null && other.getLastModifiedTime().equals(this.getLastModifiedTime()) == false)
return false;
if (other.getLastModifiedBy() == null ^ this.getLastModifiedBy() == null)
return false;
if (other.getLastModifiedBy() != null && other.getLastModifiedBy().equals(this.getLastModifiedBy()) == false)
return false;
if (other.getApprovalDescription() == null ^ this.getApprovalDescription() == null)
return false;
if (other.getApprovalDescription() != null && other.getApprovalDescription().equals(this.getApprovalDescription()) == false)
return false;
if (other.getDomain() == null ^ this.getDomain() == null)
return false;
if (other.getDomain() != null && other.getDomain().equals(this.getDomain()) == false)
return false;
if (other.getTask() == null ^ this.getTask() == null)
return false;
if (other.getTask() != null && other.getTask().equals(this.getTask()) == false)
return false;
if (other.getSamplePayloadUrl() == null ^ this.getSamplePayloadUrl() == null)
return false;
if (other.getSamplePayloadUrl() != null && other.getSamplePayloadUrl().equals(this.getSamplePayloadUrl()) == false)
return false;
if (other.getCustomerMetadataProperties() == null ^ this.getCustomerMetadataProperties() == null)
return false;
if (other.getCustomerMetadataProperties() != null && other.getCustomerMetadataProperties().equals(this.getCustomerMetadataProperties()) == false)
return false;
if (other.getDriftCheckBaselines() == null ^ this.getDriftCheckBaselines() == null)
return false;
if (other.getDriftCheckBaselines() != null && other.getDriftCheckBaselines().equals(this.getDriftCheckBaselines()) == false)
return false;
if (other.getAdditionalInferenceSpecifications() == null ^ this.getAdditionalInferenceSpecifications() == null)
return false;
if (other.getAdditionalInferenceSpecifications() != null
&& other.getAdditionalInferenceSpecifications().equals(this.getAdditionalInferenceSpecifications()) == false)
return false;
if (other.getSkipModelValidation() == null ^ this.getSkipModelValidation() == null)
return false;
if (other.getSkipModelValidation() != null && other.getSkipModelValidation().equals(this.getSkipModelValidation()) == false)
return false;
if (other.getSourceUri() == null ^ this.getSourceUri() == null)
return false;
if (other.getSourceUri() != null && other.getSourceUri().equals(this.getSourceUri()) == false)
return false;
if (other.getSecurityConfig() == null ^ this.getSecurityConfig() == null)
return false;
if (other.getSecurityConfig() != null && other.getSecurityConfig().equals(this.getSecurityConfig()) == false)
return false;
if (other.getModelCard() == null ^ this.getModelCard() == null)
return false;
if (other.getModelCard() != null && other.getModelCard().equals(this.getModelCard()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getModelPackageName() == null) ? 0 : getModelPackageName().hashCode());
hashCode = prime * hashCode + ((getModelPackageGroupName() == null) ? 0 : getModelPackageGroupName().hashCode());
hashCode = prime * hashCode + ((getModelPackageVersion() == null) ? 0 : getModelPackageVersion().hashCode());
hashCode = prime * hashCode + ((getModelPackageArn() == null) ? 0 : getModelPackageArn().hashCode());
hashCode = prime * hashCode + ((getModelPackageDescription() == null) ? 0 : getModelPackageDescription().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getInferenceSpecification() == null) ? 0 : getInferenceSpecification().hashCode());
hashCode = prime * hashCode + ((getSourceAlgorithmSpecification() == null) ? 0 : getSourceAlgorithmSpecification().hashCode());
hashCode = prime * hashCode + ((getValidationSpecification() == null) ? 0 : getValidationSpecification().hashCode());
hashCode = prime * hashCode + ((getModelPackageStatus() == null) ? 0 : getModelPackageStatus().hashCode());
hashCode = prime * hashCode + ((getModelPackageStatusDetails() == null) ? 0 : getModelPackageStatusDetails().hashCode());
hashCode = prime * hashCode + ((getCertifyForMarketplace() == null) ? 0 : getCertifyForMarketplace().hashCode());
hashCode = prime * hashCode + ((getModelApprovalStatus() == null) ? 0 : getModelApprovalStatus().hashCode());
hashCode = prime * hashCode + ((getCreatedBy() == null) ? 0 : getCreatedBy().hashCode());
hashCode = prime * hashCode + ((getMetadataProperties() == null) ? 0 : getMetadataProperties().hashCode());
hashCode = prime * hashCode + ((getModelMetrics() == null) ? 0 : getModelMetrics().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTime() == null) ? 0 : getLastModifiedTime().hashCode());
hashCode = prime * hashCode + ((getLastModifiedBy() == null) ? 0 : getLastModifiedBy().hashCode());
hashCode = prime * hashCode + ((getApprovalDescription() == null) ? 0 : getApprovalDescription().hashCode());
hashCode = prime * hashCode + ((getDomain() == null) ? 0 : getDomain().hashCode());
hashCode = prime * hashCode + ((getTask() == null) ? 0 : getTask().hashCode());
hashCode = prime * hashCode + ((getSamplePayloadUrl() == null) ? 0 : getSamplePayloadUrl().hashCode());
hashCode = prime * hashCode + ((getCustomerMetadataProperties() == null) ? 0 : getCustomerMetadataProperties().hashCode());
hashCode = prime * hashCode + ((getDriftCheckBaselines() == null) ? 0 : getDriftCheckBaselines().hashCode());
hashCode = prime * hashCode + ((getAdditionalInferenceSpecifications() == null) ? 0 : getAdditionalInferenceSpecifications().hashCode());
hashCode = prime * hashCode + ((getSkipModelValidation() == null) ? 0 : getSkipModelValidation().hashCode());
hashCode = prime * hashCode + ((getSourceUri() == null) ? 0 : getSourceUri().hashCode());
hashCode = prime * hashCode + ((getSecurityConfig() == null) ? 0 : getSecurityConfig().hashCode());
hashCode = prime * hashCode + ((getModelCard() == null) ? 0 : getModelCard().hashCode());
return hashCode;
}
@Override
public DescribeModelPackageResult clone() {
try {
return (DescribeModelPackageResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}