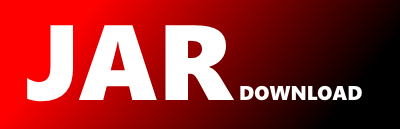
com.amazonaws.services.sagemaker.model.DescribeTransformJobResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeTransformJobResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the transform job.
*
*/
private String transformJobName;
/**
*
* The Amazon Resource Name (ARN) of the transform job.
*
*/
private String transformJobArn;
/**
*
* The status of the transform job. If the transform job failed, the reason is returned in the
* FailureReason
field.
*
*/
private String transformJobStatus;
/**
*
* If the transform job failed, FailureReason
describes why it failed. A transform job creates a log
* file, which includes error messages, and stores it as an Amazon S3 object. For more information, see Log Amazon SageMaker Events with
* Amazon CloudWatch.
*
*/
private String failureReason;
/**
*
* The name of the model used in the transform job.
*
*/
private String modelName;
/**
*
* The maximum number of parallel requests on each instance node that can be launched in a transform job. The
* default value is 1.
*
*/
private Integer maxConcurrentTransforms;
/**
*
* The timeout and maximum number of retries for processing a transform job invocation.
*
*/
private ModelClientConfig modelClientConfig;
/**
*
* The maximum payload size, in MB, used in the transform job.
*
*/
private Integer maxPayloadInMB;
/**
*
* Specifies the number of records to include in a mini-batch for an HTTP inference request. A record is
* a single unit of input data that inference can be made on. For example, a single line in a CSV file is a record.
*
*
* To enable the batch strategy, you must set SplitType
to Line
, RecordIO
, or
* TFRecord
.
*
*/
private String batchStrategy;
/**
*
* The environment variables to set in the Docker container. We support up to 16 key and values entries in the map.
*
*/
private java.util.Map environment;
/**
*
* Describes the dataset to be transformed and the Amazon S3 location where it is stored.
*
*/
private TransformInput transformInput;
/**
*
* Identifies the Amazon S3 location where you want Amazon SageMaker to save the results from the transform job.
*
*/
private TransformOutput transformOutput;
/**
*
* Configuration to control how SageMaker captures inference data.
*
*/
private BatchDataCaptureConfig dataCaptureConfig;
/**
*
* Describes the resources, including ML instance types and ML instance count, to use for the transform job.
*
*/
private TransformResources transformResources;
/**
*
* A timestamp that shows when the transform Job was created.
*
*/
private java.util.Date creationTime;
/**
*
* Indicates when the transform job starts on ML instances. You are billed for the time interval between this time
* and the value of TransformEndTime
.
*
*/
private java.util.Date transformStartTime;
/**
*
* Indicates when the transform job has been completed, or has stopped or failed. You are billed for the time
* interval between this time and the value of TransformStartTime
.
*
*/
private java.util.Date transformEndTime;
/**
*
* The Amazon Resource Name (ARN) of the Amazon SageMaker Ground Truth labeling job that created the transform or
* training job.
*
*/
private String labelingJobArn;
/**
*
* The Amazon Resource Name (ARN) of the AutoML transform job.
*
*/
private String autoMLJobArn;
private DataProcessing dataProcessing;
private ExperimentConfig experimentConfig;
/**
*
* The name of the transform job.
*
*
* @param transformJobName
* The name of the transform job.
*/
public void setTransformJobName(String transformJobName) {
this.transformJobName = transformJobName;
}
/**
*
* The name of the transform job.
*
*
* @return The name of the transform job.
*/
public String getTransformJobName() {
return this.transformJobName;
}
/**
*
* The name of the transform job.
*
*
* @param transformJobName
* The name of the transform job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withTransformJobName(String transformJobName) {
setTransformJobName(transformJobName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the transform job.
*
*
* @param transformJobArn
* The Amazon Resource Name (ARN) of the transform job.
*/
public void setTransformJobArn(String transformJobArn) {
this.transformJobArn = transformJobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the transform job.
*
*
* @return The Amazon Resource Name (ARN) of the transform job.
*/
public String getTransformJobArn() {
return this.transformJobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the transform job.
*
*
* @param transformJobArn
* The Amazon Resource Name (ARN) of the transform job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withTransformJobArn(String transformJobArn) {
setTransformJobArn(transformJobArn);
return this;
}
/**
*
* The status of the transform job. If the transform job failed, the reason is returned in the
* FailureReason
field.
*
*
* @param transformJobStatus
* The status of the transform job. If the transform job failed, the reason is returned in the
* FailureReason
field.
* @see TransformJobStatus
*/
public void setTransformJobStatus(String transformJobStatus) {
this.transformJobStatus = transformJobStatus;
}
/**
*
* The status of the transform job. If the transform job failed, the reason is returned in the
* FailureReason
field.
*
*
* @return The status of the transform job. If the transform job failed, the reason is returned in the
* FailureReason
field.
* @see TransformJobStatus
*/
public String getTransformJobStatus() {
return this.transformJobStatus;
}
/**
*
* The status of the transform job. If the transform job failed, the reason is returned in the
* FailureReason
field.
*
*
* @param transformJobStatus
* The status of the transform job. If the transform job failed, the reason is returned in the
* FailureReason
field.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TransformJobStatus
*/
public DescribeTransformJobResult withTransformJobStatus(String transformJobStatus) {
setTransformJobStatus(transformJobStatus);
return this;
}
/**
*
* The status of the transform job. If the transform job failed, the reason is returned in the
* FailureReason
field.
*
*
* @param transformJobStatus
* The status of the transform job. If the transform job failed, the reason is returned in the
* FailureReason
field.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TransformJobStatus
*/
public DescribeTransformJobResult withTransformJobStatus(TransformJobStatus transformJobStatus) {
this.transformJobStatus = transformJobStatus.toString();
return this;
}
/**
*
* If the transform job failed, FailureReason
describes why it failed. A transform job creates a log
* file, which includes error messages, and stores it as an Amazon S3 object. For more information, see Log Amazon SageMaker Events with
* Amazon CloudWatch.
*
*
* @param failureReason
* If the transform job failed, FailureReason
describes why it failed. A transform job creates a
* log file, which includes error messages, and stores it as an Amazon S3 object. For more information, see
* Log Amazon SageMaker
* Events with Amazon CloudWatch.
*/
public void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
/**
*
* If the transform job failed, FailureReason
describes why it failed. A transform job creates a log
* file, which includes error messages, and stores it as an Amazon S3 object. For more information, see Log Amazon SageMaker Events with
* Amazon CloudWatch.
*
*
* @return If the transform job failed, FailureReason
describes why it failed. A transform job creates
* a log file, which includes error messages, and stores it as an Amazon S3 object. For more information,
* see Log Amazon
* SageMaker Events with Amazon CloudWatch.
*/
public String getFailureReason() {
return this.failureReason;
}
/**
*
* If the transform job failed, FailureReason
describes why it failed. A transform job creates a log
* file, which includes error messages, and stores it as an Amazon S3 object. For more information, see Log Amazon SageMaker Events with
* Amazon CloudWatch.
*
*
* @param failureReason
* If the transform job failed, FailureReason
describes why it failed. A transform job creates a
* log file, which includes error messages, and stores it as an Amazon S3 object. For more information, see
* Log Amazon SageMaker
* Events with Amazon CloudWatch.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withFailureReason(String failureReason) {
setFailureReason(failureReason);
return this;
}
/**
*
* The name of the model used in the transform job.
*
*
* @param modelName
* The name of the model used in the transform job.
*/
public void setModelName(String modelName) {
this.modelName = modelName;
}
/**
*
* The name of the model used in the transform job.
*
*
* @return The name of the model used in the transform job.
*/
public String getModelName() {
return this.modelName;
}
/**
*
* The name of the model used in the transform job.
*
*
* @param modelName
* The name of the model used in the transform job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withModelName(String modelName) {
setModelName(modelName);
return this;
}
/**
*
* The maximum number of parallel requests on each instance node that can be launched in a transform job. The
* default value is 1.
*
*
* @param maxConcurrentTransforms
* The maximum number of parallel requests on each instance node that can be launched in a transform job. The
* default value is 1.
*/
public void setMaxConcurrentTransforms(Integer maxConcurrentTransforms) {
this.maxConcurrentTransforms = maxConcurrentTransforms;
}
/**
*
* The maximum number of parallel requests on each instance node that can be launched in a transform job. The
* default value is 1.
*
*
* @return The maximum number of parallel requests on each instance node that can be launched in a transform job.
* The default value is 1.
*/
public Integer getMaxConcurrentTransforms() {
return this.maxConcurrentTransforms;
}
/**
*
* The maximum number of parallel requests on each instance node that can be launched in a transform job. The
* default value is 1.
*
*
* @param maxConcurrentTransforms
* The maximum number of parallel requests on each instance node that can be launched in a transform job. The
* default value is 1.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withMaxConcurrentTransforms(Integer maxConcurrentTransforms) {
setMaxConcurrentTransforms(maxConcurrentTransforms);
return this;
}
/**
*
* The timeout and maximum number of retries for processing a transform job invocation.
*
*
* @param modelClientConfig
* The timeout and maximum number of retries for processing a transform job invocation.
*/
public void setModelClientConfig(ModelClientConfig modelClientConfig) {
this.modelClientConfig = modelClientConfig;
}
/**
*
* The timeout and maximum number of retries for processing a transform job invocation.
*
*
* @return The timeout and maximum number of retries for processing a transform job invocation.
*/
public ModelClientConfig getModelClientConfig() {
return this.modelClientConfig;
}
/**
*
* The timeout and maximum number of retries for processing a transform job invocation.
*
*
* @param modelClientConfig
* The timeout and maximum number of retries for processing a transform job invocation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withModelClientConfig(ModelClientConfig modelClientConfig) {
setModelClientConfig(modelClientConfig);
return this;
}
/**
*
* The maximum payload size, in MB, used in the transform job.
*
*
* @param maxPayloadInMB
* The maximum payload size, in MB, used in the transform job.
*/
public void setMaxPayloadInMB(Integer maxPayloadInMB) {
this.maxPayloadInMB = maxPayloadInMB;
}
/**
*
* The maximum payload size, in MB, used in the transform job.
*
*
* @return The maximum payload size, in MB, used in the transform job.
*/
public Integer getMaxPayloadInMB() {
return this.maxPayloadInMB;
}
/**
*
* The maximum payload size, in MB, used in the transform job.
*
*
* @param maxPayloadInMB
* The maximum payload size, in MB, used in the transform job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withMaxPayloadInMB(Integer maxPayloadInMB) {
setMaxPayloadInMB(maxPayloadInMB);
return this;
}
/**
*
* Specifies the number of records to include in a mini-batch for an HTTP inference request. A record is
* a single unit of input data that inference can be made on. For example, a single line in a CSV file is a record.
*
*
* To enable the batch strategy, you must set SplitType
to Line
, RecordIO
, or
* TFRecord
.
*
*
* @param batchStrategy
* Specifies the number of records to include in a mini-batch for an HTTP inference request. A record
* is a single unit of input data that inference can be made on. For example, a single line in a CSV
* file is a record.
*
* To enable the batch strategy, you must set SplitType
to Line
,
* RecordIO
, or TFRecord
.
* @see BatchStrategy
*/
public void setBatchStrategy(String batchStrategy) {
this.batchStrategy = batchStrategy;
}
/**
*
* Specifies the number of records to include in a mini-batch for an HTTP inference request. A record is
* a single unit of input data that inference can be made on. For example, a single line in a CSV file is a record.
*
*
* To enable the batch strategy, you must set SplitType
to Line
, RecordIO
, or
* TFRecord
.
*
*
* @return Specifies the number of records to include in a mini-batch for an HTTP inference request. A record
* is a single unit of input data that inference can be made on. For example, a single line in a CSV
* file is a record.
*
* To enable the batch strategy, you must set SplitType
to Line
,
* RecordIO
, or TFRecord
.
* @see BatchStrategy
*/
public String getBatchStrategy() {
return this.batchStrategy;
}
/**
*
* Specifies the number of records to include in a mini-batch for an HTTP inference request. A record is
* a single unit of input data that inference can be made on. For example, a single line in a CSV file is a record.
*
*
* To enable the batch strategy, you must set SplitType
to Line
, RecordIO
, or
* TFRecord
.
*
*
* @param batchStrategy
* Specifies the number of records to include in a mini-batch for an HTTP inference request. A record
* is a single unit of input data that inference can be made on. For example, a single line in a CSV
* file is a record.
*
* To enable the batch strategy, you must set SplitType
to Line
,
* RecordIO
, or TFRecord
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BatchStrategy
*/
public DescribeTransformJobResult withBatchStrategy(String batchStrategy) {
setBatchStrategy(batchStrategy);
return this;
}
/**
*
* Specifies the number of records to include in a mini-batch for an HTTP inference request. A record is
* a single unit of input data that inference can be made on. For example, a single line in a CSV file is a record.
*
*
* To enable the batch strategy, you must set SplitType
to Line
, RecordIO
, or
* TFRecord
.
*
*
* @param batchStrategy
* Specifies the number of records to include in a mini-batch for an HTTP inference request. A record
* is a single unit of input data that inference can be made on. For example, a single line in a CSV
* file is a record.
*
* To enable the batch strategy, you must set SplitType
to Line
,
* RecordIO
, or TFRecord
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see BatchStrategy
*/
public DescribeTransformJobResult withBatchStrategy(BatchStrategy batchStrategy) {
this.batchStrategy = batchStrategy.toString();
return this;
}
/**
*
* The environment variables to set in the Docker container. We support up to 16 key and values entries in the map.
*
*
* @return The environment variables to set in the Docker container. We support up to 16 key and values entries in
* the map.
*/
public java.util.Map getEnvironment() {
return environment;
}
/**
*
* The environment variables to set in the Docker container. We support up to 16 key and values entries in the map.
*
*
* @param environment
* The environment variables to set in the Docker container. We support up to 16 key and values entries in
* the map.
*/
public void setEnvironment(java.util.Map environment) {
this.environment = environment;
}
/**
*
* The environment variables to set in the Docker container. We support up to 16 key and values entries in the map.
*
*
* @param environment
* The environment variables to set in the Docker container. We support up to 16 key and values entries in
* the map.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withEnvironment(java.util.Map environment) {
setEnvironment(environment);
return this;
}
/**
* Add a single Environment entry
*
* @see DescribeTransformJobResult#withEnvironment
* @returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult addEnvironmentEntry(String key, String value) {
if (null == this.environment) {
this.environment = new java.util.HashMap();
}
if (this.environment.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.environment.put(key, value);
return this;
}
/**
* Removes all the entries added into Environment.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult clearEnvironmentEntries() {
this.environment = null;
return this;
}
/**
*
* Describes the dataset to be transformed and the Amazon S3 location where it is stored.
*
*
* @param transformInput
* Describes the dataset to be transformed and the Amazon S3 location where it is stored.
*/
public void setTransformInput(TransformInput transformInput) {
this.transformInput = transformInput;
}
/**
*
* Describes the dataset to be transformed and the Amazon S3 location where it is stored.
*
*
* @return Describes the dataset to be transformed and the Amazon S3 location where it is stored.
*/
public TransformInput getTransformInput() {
return this.transformInput;
}
/**
*
* Describes the dataset to be transformed and the Amazon S3 location where it is stored.
*
*
* @param transformInput
* Describes the dataset to be transformed and the Amazon S3 location where it is stored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withTransformInput(TransformInput transformInput) {
setTransformInput(transformInput);
return this;
}
/**
*
* Identifies the Amazon S3 location where you want Amazon SageMaker to save the results from the transform job.
*
*
* @param transformOutput
* Identifies the Amazon S3 location where you want Amazon SageMaker to save the results from the transform
* job.
*/
public void setTransformOutput(TransformOutput transformOutput) {
this.transformOutput = transformOutput;
}
/**
*
* Identifies the Amazon S3 location where you want Amazon SageMaker to save the results from the transform job.
*
*
* @return Identifies the Amazon S3 location where you want Amazon SageMaker to save the results from the transform
* job.
*/
public TransformOutput getTransformOutput() {
return this.transformOutput;
}
/**
*
* Identifies the Amazon S3 location where you want Amazon SageMaker to save the results from the transform job.
*
*
* @param transformOutput
* Identifies the Amazon S3 location where you want Amazon SageMaker to save the results from the transform
* job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withTransformOutput(TransformOutput transformOutput) {
setTransformOutput(transformOutput);
return this;
}
/**
*
* Configuration to control how SageMaker captures inference data.
*
*
* @param dataCaptureConfig
* Configuration to control how SageMaker captures inference data.
*/
public void setDataCaptureConfig(BatchDataCaptureConfig dataCaptureConfig) {
this.dataCaptureConfig = dataCaptureConfig;
}
/**
*
* Configuration to control how SageMaker captures inference data.
*
*
* @return Configuration to control how SageMaker captures inference data.
*/
public BatchDataCaptureConfig getDataCaptureConfig() {
return this.dataCaptureConfig;
}
/**
*
* Configuration to control how SageMaker captures inference data.
*
*
* @param dataCaptureConfig
* Configuration to control how SageMaker captures inference data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withDataCaptureConfig(BatchDataCaptureConfig dataCaptureConfig) {
setDataCaptureConfig(dataCaptureConfig);
return this;
}
/**
*
* Describes the resources, including ML instance types and ML instance count, to use for the transform job.
*
*
* @param transformResources
* Describes the resources, including ML instance types and ML instance count, to use for the transform job.
*/
public void setTransformResources(TransformResources transformResources) {
this.transformResources = transformResources;
}
/**
*
* Describes the resources, including ML instance types and ML instance count, to use for the transform job.
*
*
* @return Describes the resources, including ML instance types and ML instance count, to use for the transform job.
*/
public TransformResources getTransformResources() {
return this.transformResources;
}
/**
*
* Describes the resources, including ML instance types and ML instance count, to use for the transform job.
*
*
* @param transformResources
* Describes the resources, including ML instance types and ML instance count, to use for the transform job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withTransformResources(TransformResources transformResources) {
setTransformResources(transformResources);
return this;
}
/**
*
* A timestamp that shows when the transform Job was created.
*
*
* @param creationTime
* A timestamp that shows when the transform Job was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* A timestamp that shows when the transform Job was created.
*
*
* @return A timestamp that shows when the transform Job was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* A timestamp that shows when the transform Job was created.
*
*
* @param creationTime
* A timestamp that shows when the transform Job was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* Indicates when the transform job starts on ML instances. You are billed for the time interval between this time
* and the value of TransformEndTime
.
*
*
* @param transformStartTime
* Indicates when the transform job starts on ML instances. You are billed for the time interval between this
* time and the value of TransformEndTime
.
*/
public void setTransformStartTime(java.util.Date transformStartTime) {
this.transformStartTime = transformStartTime;
}
/**
*
* Indicates when the transform job starts on ML instances. You are billed for the time interval between this time
* and the value of TransformEndTime
.
*
*
* @return Indicates when the transform job starts on ML instances. You are billed for the time interval between
* this time and the value of TransformEndTime
.
*/
public java.util.Date getTransformStartTime() {
return this.transformStartTime;
}
/**
*
* Indicates when the transform job starts on ML instances. You are billed for the time interval between this time
* and the value of TransformEndTime
.
*
*
* @param transformStartTime
* Indicates when the transform job starts on ML instances. You are billed for the time interval between this
* time and the value of TransformEndTime
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withTransformStartTime(java.util.Date transformStartTime) {
setTransformStartTime(transformStartTime);
return this;
}
/**
*
* Indicates when the transform job has been completed, or has stopped or failed. You are billed for the time
* interval between this time and the value of TransformStartTime
.
*
*
* @param transformEndTime
* Indicates when the transform job has been completed, or has stopped or failed. You are billed for the time
* interval between this time and the value of TransformStartTime
.
*/
public void setTransformEndTime(java.util.Date transformEndTime) {
this.transformEndTime = transformEndTime;
}
/**
*
* Indicates when the transform job has been completed, or has stopped or failed. You are billed for the time
* interval between this time and the value of TransformStartTime
.
*
*
* @return Indicates when the transform job has been completed, or has stopped or failed. You are billed for the
* time interval between this time and the value of TransformStartTime
.
*/
public java.util.Date getTransformEndTime() {
return this.transformEndTime;
}
/**
*
* Indicates when the transform job has been completed, or has stopped or failed. You are billed for the time
* interval between this time and the value of TransformStartTime
.
*
*
* @param transformEndTime
* Indicates when the transform job has been completed, or has stopped or failed. You are billed for the time
* interval between this time and the value of TransformStartTime
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withTransformEndTime(java.util.Date transformEndTime) {
setTransformEndTime(transformEndTime);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SageMaker Ground Truth labeling job that created the transform or
* training job.
*
*
* @param labelingJobArn
* The Amazon Resource Name (ARN) of the Amazon SageMaker Ground Truth labeling job that created the
* transform or training job.
*/
public void setLabelingJobArn(String labelingJobArn) {
this.labelingJobArn = labelingJobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SageMaker Ground Truth labeling job that created the transform or
* training job.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon SageMaker Ground Truth labeling job that created the
* transform or training job.
*/
public String getLabelingJobArn() {
return this.labelingJobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon SageMaker Ground Truth labeling job that created the transform or
* training job.
*
*
* @param labelingJobArn
* The Amazon Resource Name (ARN) of the Amazon SageMaker Ground Truth labeling job that created the
* transform or training job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withLabelingJobArn(String labelingJobArn) {
setLabelingJobArn(labelingJobArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the AutoML transform job.
*
*
* @param autoMLJobArn
* The Amazon Resource Name (ARN) of the AutoML transform job.
*/
public void setAutoMLJobArn(String autoMLJobArn) {
this.autoMLJobArn = autoMLJobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the AutoML transform job.
*
*
* @return The Amazon Resource Name (ARN) of the AutoML transform job.
*/
public String getAutoMLJobArn() {
return this.autoMLJobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the AutoML transform job.
*
*
* @param autoMLJobArn
* The Amazon Resource Name (ARN) of the AutoML transform job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withAutoMLJobArn(String autoMLJobArn) {
setAutoMLJobArn(autoMLJobArn);
return this;
}
/**
* @param dataProcessing
*/
public void setDataProcessing(DataProcessing dataProcessing) {
this.dataProcessing = dataProcessing;
}
/**
* @return
*/
public DataProcessing getDataProcessing() {
return this.dataProcessing;
}
/**
* @param dataProcessing
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withDataProcessing(DataProcessing dataProcessing) {
setDataProcessing(dataProcessing);
return this;
}
/**
* @param experimentConfig
*/
public void setExperimentConfig(ExperimentConfig experimentConfig) {
this.experimentConfig = experimentConfig;
}
/**
* @return
*/
public ExperimentConfig getExperimentConfig() {
return this.experimentConfig;
}
/**
* @param experimentConfig
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeTransformJobResult withExperimentConfig(ExperimentConfig experimentConfig) {
setExperimentConfig(experimentConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTransformJobName() != null)
sb.append("TransformJobName: ").append(getTransformJobName()).append(",");
if (getTransformJobArn() != null)
sb.append("TransformJobArn: ").append(getTransformJobArn()).append(",");
if (getTransformJobStatus() != null)
sb.append("TransformJobStatus: ").append(getTransformJobStatus()).append(",");
if (getFailureReason() != null)
sb.append("FailureReason: ").append(getFailureReason()).append(",");
if (getModelName() != null)
sb.append("ModelName: ").append(getModelName()).append(",");
if (getMaxConcurrentTransforms() != null)
sb.append("MaxConcurrentTransforms: ").append(getMaxConcurrentTransforms()).append(",");
if (getModelClientConfig() != null)
sb.append("ModelClientConfig: ").append(getModelClientConfig()).append(",");
if (getMaxPayloadInMB() != null)
sb.append("MaxPayloadInMB: ").append(getMaxPayloadInMB()).append(",");
if (getBatchStrategy() != null)
sb.append("BatchStrategy: ").append(getBatchStrategy()).append(",");
if (getEnvironment() != null)
sb.append("Environment: ").append(getEnvironment()).append(",");
if (getTransformInput() != null)
sb.append("TransformInput: ").append(getTransformInput()).append(",");
if (getTransformOutput() != null)
sb.append("TransformOutput: ").append(getTransformOutput()).append(",");
if (getDataCaptureConfig() != null)
sb.append("DataCaptureConfig: ").append(getDataCaptureConfig()).append(",");
if (getTransformResources() != null)
sb.append("TransformResources: ").append(getTransformResources()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getTransformStartTime() != null)
sb.append("TransformStartTime: ").append(getTransformStartTime()).append(",");
if (getTransformEndTime() != null)
sb.append("TransformEndTime: ").append(getTransformEndTime()).append(",");
if (getLabelingJobArn() != null)
sb.append("LabelingJobArn: ").append(getLabelingJobArn()).append(",");
if (getAutoMLJobArn() != null)
sb.append("AutoMLJobArn: ").append(getAutoMLJobArn()).append(",");
if (getDataProcessing() != null)
sb.append("DataProcessing: ").append(getDataProcessing()).append(",");
if (getExperimentConfig() != null)
sb.append("ExperimentConfig: ").append(getExperimentConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeTransformJobResult == false)
return false;
DescribeTransformJobResult other = (DescribeTransformJobResult) obj;
if (other.getTransformJobName() == null ^ this.getTransformJobName() == null)
return false;
if (other.getTransformJobName() != null && other.getTransformJobName().equals(this.getTransformJobName()) == false)
return false;
if (other.getTransformJobArn() == null ^ this.getTransformJobArn() == null)
return false;
if (other.getTransformJobArn() != null && other.getTransformJobArn().equals(this.getTransformJobArn()) == false)
return false;
if (other.getTransformJobStatus() == null ^ this.getTransformJobStatus() == null)
return false;
if (other.getTransformJobStatus() != null && other.getTransformJobStatus().equals(this.getTransformJobStatus()) == false)
return false;
if (other.getFailureReason() == null ^ this.getFailureReason() == null)
return false;
if (other.getFailureReason() != null && other.getFailureReason().equals(this.getFailureReason()) == false)
return false;
if (other.getModelName() == null ^ this.getModelName() == null)
return false;
if (other.getModelName() != null && other.getModelName().equals(this.getModelName()) == false)
return false;
if (other.getMaxConcurrentTransforms() == null ^ this.getMaxConcurrentTransforms() == null)
return false;
if (other.getMaxConcurrentTransforms() != null && other.getMaxConcurrentTransforms().equals(this.getMaxConcurrentTransforms()) == false)
return false;
if (other.getModelClientConfig() == null ^ this.getModelClientConfig() == null)
return false;
if (other.getModelClientConfig() != null && other.getModelClientConfig().equals(this.getModelClientConfig()) == false)
return false;
if (other.getMaxPayloadInMB() == null ^ this.getMaxPayloadInMB() == null)
return false;
if (other.getMaxPayloadInMB() != null && other.getMaxPayloadInMB().equals(this.getMaxPayloadInMB()) == false)
return false;
if (other.getBatchStrategy() == null ^ this.getBatchStrategy() == null)
return false;
if (other.getBatchStrategy() != null && other.getBatchStrategy().equals(this.getBatchStrategy()) == false)
return false;
if (other.getEnvironment() == null ^ this.getEnvironment() == null)
return false;
if (other.getEnvironment() != null && other.getEnvironment().equals(this.getEnvironment()) == false)
return false;
if (other.getTransformInput() == null ^ this.getTransformInput() == null)
return false;
if (other.getTransformInput() != null && other.getTransformInput().equals(this.getTransformInput()) == false)
return false;
if (other.getTransformOutput() == null ^ this.getTransformOutput() == null)
return false;
if (other.getTransformOutput() != null && other.getTransformOutput().equals(this.getTransformOutput()) == false)
return false;
if (other.getDataCaptureConfig() == null ^ this.getDataCaptureConfig() == null)
return false;
if (other.getDataCaptureConfig() != null && other.getDataCaptureConfig().equals(this.getDataCaptureConfig()) == false)
return false;
if (other.getTransformResources() == null ^ this.getTransformResources() == null)
return false;
if (other.getTransformResources() != null && other.getTransformResources().equals(this.getTransformResources()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getTransformStartTime() == null ^ this.getTransformStartTime() == null)
return false;
if (other.getTransformStartTime() != null && other.getTransformStartTime().equals(this.getTransformStartTime()) == false)
return false;
if (other.getTransformEndTime() == null ^ this.getTransformEndTime() == null)
return false;
if (other.getTransformEndTime() != null && other.getTransformEndTime().equals(this.getTransformEndTime()) == false)
return false;
if (other.getLabelingJobArn() == null ^ this.getLabelingJobArn() == null)
return false;
if (other.getLabelingJobArn() != null && other.getLabelingJobArn().equals(this.getLabelingJobArn()) == false)
return false;
if (other.getAutoMLJobArn() == null ^ this.getAutoMLJobArn() == null)
return false;
if (other.getAutoMLJobArn() != null && other.getAutoMLJobArn().equals(this.getAutoMLJobArn()) == false)
return false;
if (other.getDataProcessing() == null ^ this.getDataProcessing() == null)
return false;
if (other.getDataProcessing() != null && other.getDataProcessing().equals(this.getDataProcessing()) == false)
return false;
if (other.getExperimentConfig() == null ^ this.getExperimentConfig() == null)
return false;
if (other.getExperimentConfig() != null && other.getExperimentConfig().equals(this.getExperimentConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTransformJobName() == null) ? 0 : getTransformJobName().hashCode());
hashCode = prime * hashCode + ((getTransformJobArn() == null) ? 0 : getTransformJobArn().hashCode());
hashCode = prime * hashCode + ((getTransformJobStatus() == null) ? 0 : getTransformJobStatus().hashCode());
hashCode = prime * hashCode + ((getFailureReason() == null) ? 0 : getFailureReason().hashCode());
hashCode = prime * hashCode + ((getModelName() == null) ? 0 : getModelName().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrentTransforms() == null) ? 0 : getMaxConcurrentTransforms().hashCode());
hashCode = prime * hashCode + ((getModelClientConfig() == null) ? 0 : getModelClientConfig().hashCode());
hashCode = prime * hashCode + ((getMaxPayloadInMB() == null) ? 0 : getMaxPayloadInMB().hashCode());
hashCode = prime * hashCode + ((getBatchStrategy() == null) ? 0 : getBatchStrategy().hashCode());
hashCode = prime * hashCode + ((getEnvironment() == null) ? 0 : getEnvironment().hashCode());
hashCode = prime * hashCode + ((getTransformInput() == null) ? 0 : getTransformInput().hashCode());
hashCode = prime * hashCode + ((getTransformOutput() == null) ? 0 : getTransformOutput().hashCode());
hashCode = prime * hashCode + ((getDataCaptureConfig() == null) ? 0 : getDataCaptureConfig().hashCode());
hashCode = prime * hashCode + ((getTransformResources() == null) ? 0 : getTransformResources().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getTransformStartTime() == null) ? 0 : getTransformStartTime().hashCode());
hashCode = prime * hashCode + ((getTransformEndTime() == null) ? 0 : getTransformEndTime().hashCode());
hashCode = prime * hashCode + ((getLabelingJobArn() == null) ? 0 : getLabelingJobArn().hashCode());
hashCode = prime * hashCode + ((getAutoMLJobArn() == null) ? 0 : getAutoMLJobArn().hashCode());
hashCode = prime * hashCode + ((getDataProcessing() == null) ? 0 : getDataProcessing().hashCode());
hashCode = prime * hashCode + ((getExperimentConfig() == null) ? 0 : getExperimentConfig().hashCode());
return hashCode;
}
@Override
public DescribeTransformJobResult clone() {
try {
return (DescribeTransformJobResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}