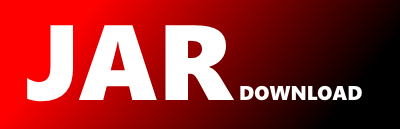
com.amazonaws.services.sagemaker.model.EndpointInput Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Input object for the endpoint
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EndpointInput implements Serializable, Cloneable, StructuredPojo {
/**
*
* An endpoint in customer's account which has enabled DataCaptureConfig
enabled.
*
*/
private String endpointName;
/**
*
* Path to the filesystem where the endpoint data is available to the container.
*
*/
private String localPath;
/**
*
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful for
* small files that fit in memory. Defaults to File
.
*
*/
private String s3InputMode;
/**
*
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults to
* FullyReplicated
*
*/
private String s3DataDistributionType;
/**
*
* The attributes of the input data that are the input features.
*
*/
private String featuresAttribute;
/**
*
* The attribute of the input data that represents the ground truth label.
*
*/
private String inferenceAttribute;
/**
*
* In a classification problem, the attribute that represents the class probability.
*
*/
private String probabilityAttribute;
/**
*
* The threshold for the class probability to be evaluated as a positive result.
*
*/
private Double probabilityThresholdAttribute;
/**
*
* If specified, monitoring jobs substract this time from the start time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*/
private String startTimeOffset;
/**
*
* If specified, monitoring jobs substract this time from the end time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*/
private String endTimeOffset;
/**
*
* The attributes of the input data to exclude from the analysis.
*
*/
private String excludeFeaturesAttribute;
/**
*
* An endpoint in customer's account which has enabled DataCaptureConfig
enabled.
*
*
* @param endpointName
* An endpoint in customer's account which has enabled DataCaptureConfig
enabled.
*/
public void setEndpointName(String endpointName) {
this.endpointName = endpointName;
}
/**
*
* An endpoint in customer's account which has enabled DataCaptureConfig
enabled.
*
*
* @return An endpoint in customer's account which has enabled DataCaptureConfig
enabled.
*/
public String getEndpointName() {
return this.endpointName;
}
/**
*
* An endpoint in customer's account which has enabled DataCaptureConfig
enabled.
*
*
* @param endpointName
* An endpoint in customer's account which has enabled DataCaptureConfig
enabled.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointInput withEndpointName(String endpointName) {
setEndpointName(endpointName);
return this;
}
/**
*
* Path to the filesystem where the endpoint data is available to the container.
*
*
* @param localPath
* Path to the filesystem where the endpoint data is available to the container.
*/
public void setLocalPath(String localPath) {
this.localPath = localPath;
}
/**
*
* Path to the filesystem where the endpoint data is available to the container.
*
*
* @return Path to the filesystem where the endpoint data is available to the container.
*/
public String getLocalPath() {
return this.localPath;
}
/**
*
* Path to the filesystem where the endpoint data is available to the container.
*
*
* @param localPath
* Path to the filesystem where the endpoint data is available to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointInput withLocalPath(String localPath) {
setLocalPath(localPath);
return this;
}
/**
*
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful for
* small files that fit in memory. Defaults to File
.
*
*
* @param s3InputMode
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful
* for small files that fit in memory. Defaults to File
.
* @see ProcessingS3InputMode
*/
public void setS3InputMode(String s3InputMode) {
this.s3InputMode = s3InputMode;
}
/**
*
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful for
* small files that fit in memory. Defaults to File
.
*
*
* @return Whether the Pipe
or File
is used as the input mode for transferring data for
* the monitoring job. Pipe
mode is recommended for large datasets. File
mode is
* useful for small files that fit in memory. Defaults to File
.
* @see ProcessingS3InputMode
*/
public String getS3InputMode() {
return this.s3InputMode;
}
/**
*
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful for
* small files that fit in memory. Defaults to File
.
*
*
* @param s3InputMode
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful
* for small files that fit in memory. Defaults to File
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProcessingS3InputMode
*/
public EndpointInput withS3InputMode(String s3InputMode) {
setS3InputMode(s3InputMode);
return this;
}
/**
*
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful for
* small files that fit in memory. Defaults to File
.
*
*
* @param s3InputMode
* Whether the Pipe
or File
is used as the input mode for transferring data for the
* monitoring job. Pipe
mode is recommended for large datasets. File
mode is useful
* for small files that fit in memory. Defaults to File
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProcessingS3InputMode
*/
public EndpointInput withS3InputMode(ProcessingS3InputMode s3InputMode) {
this.s3InputMode = s3InputMode.toString();
return this;
}
/**
*
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults to
* FullyReplicated
*
*
* @param s3DataDistributionType
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults
* to FullyReplicated
* @see ProcessingS3DataDistributionType
*/
public void setS3DataDistributionType(String s3DataDistributionType) {
this.s3DataDistributionType = s3DataDistributionType;
}
/**
*
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults to
* FullyReplicated
*
*
* @return Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults
* to FullyReplicated
* @see ProcessingS3DataDistributionType
*/
public String getS3DataDistributionType() {
return this.s3DataDistributionType;
}
/**
*
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults to
* FullyReplicated
*
*
* @param s3DataDistributionType
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults
* to FullyReplicated
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProcessingS3DataDistributionType
*/
public EndpointInput withS3DataDistributionType(String s3DataDistributionType) {
setS3DataDistributionType(s3DataDistributionType);
return this;
}
/**
*
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults to
* FullyReplicated
*
*
* @param s3DataDistributionType
* Whether input data distributed in Amazon S3 is fully replicated or sharded by an Amazon S3 key. Defaults
* to FullyReplicated
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProcessingS3DataDistributionType
*/
public EndpointInput withS3DataDistributionType(ProcessingS3DataDistributionType s3DataDistributionType) {
this.s3DataDistributionType = s3DataDistributionType.toString();
return this;
}
/**
*
* The attributes of the input data that are the input features.
*
*
* @param featuresAttribute
* The attributes of the input data that are the input features.
*/
public void setFeaturesAttribute(String featuresAttribute) {
this.featuresAttribute = featuresAttribute;
}
/**
*
* The attributes of the input data that are the input features.
*
*
* @return The attributes of the input data that are the input features.
*/
public String getFeaturesAttribute() {
return this.featuresAttribute;
}
/**
*
* The attributes of the input data that are the input features.
*
*
* @param featuresAttribute
* The attributes of the input data that are the input features.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointInput withFeaturesAttribute(String featuresAttribute) {
setFeaturesAttribute(featuresAttribute);
return this;
}
/**
*
* The attribute of the input data that represents the ground truth label.
*
*
* @param inferenceAttribute
* The attribute of the input data that represents the ground truth label.
*/
public void setInferenceAttribute(String inferenceAttribute) {
this.inferenceAttribute = inferenceAttribute;
}
/**
*
* The attribute of the input data that represents the ground truth label.
*
*
* @return The attribute of the input data that represents the ground truth label.
*/
public String getInferenceAttribute() {
return this.inferenceAttribute;
}
/**
*
* The attribute of the input data that represents the ground truth label.
*
*
* @param inferenceAttribute
* The attribute of the input data that represents the ground truth label.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointInput withInferenceAttribute(String inferenceAttribute) {
setInferenceAttribute(inferenceAttribute);
return this;
}
/**
*
* In a classification problem, the attribute that represents the class probability.
*
*
* @param probabilityAttribute
* In a classification problem, the attribute that represents the class probability.
*/
public void setProbabilityAttribute(String probabilityAttribute) {
this.probabilityAttribute = probabilityAttribute;
}
/**
*
* In a classification problem, the attribute that represents the class probability.
*
*
* @return In a classification problem, the attribute that represents the class probability.
*/
public String getProbabilityAttribute() {
return this.probabilityAttribute;
}
/**
*
* In a classification problem, the attribute that represents the class probability.
*
*
* @param probabilityAttribute
* In a classification problem, the attribute that represents the class probability.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointInput withProbabilityAttribute(String probabilityAttribute) {
setProbabilityAttribute(probabilityAttribute);
return this;
}
/**
*
* The threshold for the class probability to be evaluated as a positive result.
*
*
* @param probabilityThresholdAttribute
* The threshold for the class probability to be evaluated as a positive result.
*/
public void setProbabilityThresholdAttribute(Double probabilityThresholdAttribute) {
this.probabilityThresholdAttribute = probabilityThresholdAttribute;
}
/**
*
* The threshold for the class probability to be evaluated as a positive result.
*
*
* @return The threshold for the class probability to be evaluated as a positive result.
*/
public Double getProbabilityThresholdAttribute() {
return this.probabilityThresholdAttribute;
}
/**
*
* The threshold for the class probability to be evaluated as a positive result.
*
*
* @param probabilityThresholdAttribute
* The threshold for the class probability to be evaluated as a positive result.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointInput withProbabilityThresholdAttribute(Double probabilityThresholdAttribute) {
setProbabilityThresholdAttribute(probabilityThresholdAttribute);
return this;
}
/**
*
* If specified, monitoring jobs substract this time from the start time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*
* @param startTimeOffset
* If specified, monitoring jobs substract this time from the start time. For information about using offsets
* for scheduling monitoring jobs, see Schedule
* Model Quality Monitoring Jobs.
*/
public void setStartTimeOffset(String startTimeOffset) {
this.startTimeOffset = startTimeOffset;
}
/**
*
* If specified, monitoring jobs substract this time from the start time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*
* @return If specified, monitoring jobs substract this time from the start time. For information about using
* offsets for scheduling monitoring jobs, see Schedule
* Model Quality Monitoring Jobs.
*/
public String getStartTimeOffset() {
return this.startTimeOffset;
}
/**
*
* If specified, monitoring jobs substract this time from the start time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*
* @param startTimeOffset
* If specified, monitoring jobs substract this time from the start time. For information about using offsets
* for scheduling monitoring jobs, see Schedule
* Model Quality Monitoring Jobs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointInput withStartTimeOffset(String startTimeOffset) {
setStartTimeOffset(startTimeOffset);
return this;
}
/**
*
* If specified, monitoring jobs substract this time from the end time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*
* @param endTimeOffset
* If specified, monitoring jobs substract this time from the end time. For information about using offsets
* for scheduling monitoring jobs, see Schedule
* Model Quality Monitoring Jobs.
*/
public void setEndTimeOffset(String endTimeOffset) {
this.endTimeOffset = endTimeOffset;
}
/**
*
* If specified, monitoring jobs substract this time from the end time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*
* @return If specified, monitoring jobs substract this time from the end time. For information about using offsets
* for scheduling monitoring jobs, see Schedule
* Model Quality Monitoring Jobs.
*/
public String getEndTimeOffset() {
return this.endTimeOffset;
}
/**
*
* If specified, monitoring jobs substract this time from the end time. For information about using offsets for
* scheduling monitoring jobs, see Schedule Model
* Quality Monitoring Jobs.
*
*
* @param endTimeOffset
* If specified, monitoring jobs substract this time from the end time. For information about using offsets
* for scheduling monitoring jobs, see Schedule
* Model Quality Monitoring Jobs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointInput withEndTimeOffset(String endTimeOffset) {
setEndTimeOffset(endTimeOffset);
return this;
}
/**
*
* The attributes of the input data to exclude from the analysis.
*
*
* @param excludeFeaturesAttribute
* The attributes of the input data to exclude from the analysis.
*/
public void setExcludeFeaturesAttribute(String excludeFeaturesAttribute) {
this.excludeFeaturesAttribute = excludeFeaturesAttribute;
}
/**
*
* The attributes of the input data to exclude from the analysis.
*
*
* @return The attributes of the input data to exclude from the analysis.
*/
public String getExcludeFeaturesAttribute() {
return this.excludeFeaturesAttribute;
}
/**
*
* The attributes of the input data to exclude from the analysis.
*
*
* @param excludeFeaturesAttribute
* The attributes of the input data to exclude from the analysis.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EndpointInput withExcludeFeaturesAttribute(String excludeFeaturesAttribute) {
setExcludeFeaturesAttribute(excludeFeaturesAttribute);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEndpointName() != null)
sb.append("EndpointName: ").append(getEndpointName()).append(",");
if (getLocalPath() != null)
sb.append("LocalPath: ").append(getLocalPath()).append(",");
if (getS3InputMode() != null)
sb.append("S3InputMode: ").append(getS3InputMode()).append(",");
if (getS3DataDistributionType() != null)
sb.append("S3DataDistributionType: ").append(getS3DataDistributionType()).append(",");
if (getFeaturesAttribute() != null)
sb.append("FeaturesAttribute: ").append(getFeaturesAttribute()).append(",");
if (getInferenceAttribute() != null)
sb.append("InferenceAttribute: ").append(getInferenceAttribute()).append(",");
if (getProbabilityAttribute() != null)
sb.append("ProbabilityAttribute: ").append(getProbabilityAttribute()).append(",");
if (getProbabilityThresholdAttribute() != null)
sb.append("ProbabilityThresholdAttribute: ").append(getProbabilityThresholdAttribute()).append(",");
if (getStartTimeOffset() != null)
sb.append("StartTimeOffset: ").append(getStartTimeOffset()).append(",");
if (getEndTimeOffset() != null)
sb.append("EndTimeOffset: ").append(getEndTimeOffset()).append(",");
if (getExcludeFeaturesAttribute() != null)
sb.append("ExcludeFeaturesAttribute: ").append(getExcludeFeaturesAttribute());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EndpointInput == false)
return false;
EndpointInput other = (EndpointInput) obj;
if (other.getEndpointName() == null ^ this.getEndpointName() == null)
return false;
if (other.getEndpointName() != null && other.getEndpointName().equals(this.getEndpointName()) == false)
return false;
if (other.getLocalPath() == null ^ this.getLocalPath() == null)
return false;
if (other.getLocalPath() != null && other.getLocalPath().equals(this.getLocalPath()) == false)
return false;
if (other.getS3InputMode() == null ^ this.getS3InputMode() == null)
return false;
if (other.getS3InputMode() != null && other.getS3InputMode().equals(this.getS3InputMode()) == false)
return false;
if (other.getS3DataDistributionType() == null ^ this.getS3DataDistributionType() == null)
return false;
if (other.getS3DataDistributionType() != null && other.getS3DataDistributionType().equals(this.getS3DataDistributionType()) == false)
return false;
if (other.getFeaturesAttribute() == null ^ this.getFeaturesAttribute() == null)
return false;
if (other.getFeaturesAttribute() != null && other.getFeaturesAttribute().equals(this.getFeaturesAttribute()) == false)
return false;
if (other.getInferenceAttribute() == null ^ this.getInferenceAttribute() == null)
return false;
if (other.getInferenceAttribute() != null && other.getInferenceAttribute().equals(this.getInferenceAttribute()) == false)
return false;
if (other.getProbabilityAttribute() == null ^ this.getProbabilityAttribute() == null)
return false;
if (other.getProbabilityAttribute() != null && other.getProbabilityAttribute().equals(this.getProbabilityAttribute()) == false)
return false;
if (other.getProbabilityThresholdAttribute() == null ^ this.getProbabilityThresholdAttribute() == null)
return false;
if (other.getProbabilityThresholdAttribute() != null
&& other.getProbabilityThresholdAttribute().equals(this.getProbabilityThresholdAttribute()) == false)
return false;
if (other.getStartTimeOffset() == null ^ this.getStartTimeOffset() == null)
return false;
if (other.getStartTimeOffset() != null && other.getStartTimeOffset().equals(this.getStartTimeOffset()) == false)
return false;
if (other.getEndTimeOffset() == null ^ this.getEndTimeOffset() == null)
return false;
if (other.getEndTimeOffset() != null && other.getEndTimeOffset().equals(this.getEndTimeOffset()) == false)
return false;
if (other.getExcludeFeaturesAttribute() == null ^ this.getExcludeFeaturesAttribute() == null)
return false;
if (other.getExcludeFeaturesAttribute() != null && other.getExcludeFeaturesAttribute().equals(this.getExcludeFeaturesAttribute()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEndpointName() == null) ? 0 : getEndpointName().hashCode());
hashCode = prime * hashCode + ((getLocalPath() == null) ? 0 : getLocalPath().hashCode());
hashCode = prime * hashCode + ((getS3InputMode() == null) ? 0 : getS3InputMode().hashCode());
hashCode = prime * hashCode + ((getS3DataDistributionType() == null) ? 0 : getS3DataDistributionType().hashCode());
hashCode = prime * hashCode + ((getFeaturesAttribute() == null) ? 0 : getFeaturesAttribute().hashCode());
hashCode = prime * hashCode + ((getInferenceAttribute() == null) ? 0 : getInferenceAttribute().hashCode());
hashCode = prime * hashCode + ((getProbabilityAttribute() == null) ? 0 : getProbabilityAttribute().hashCode());
hashCode = prime * hashCode + ((getProbabilityThresholdAttribute() == null) ? 0 : getProbabilityThresholdAttribute().hashCode());
hashCode = prime * hashCode + ((getStartTimeOffset() == null) ? 0 : getStartTimeOffset().hashCode());
hashCode = prime * hashCode + ((getEndTimeOffset() == null) ? 0 : getEndTimeOffset().hashCode());
hashCode = prime * hashCode + ((getExcludeFeaturesAttribute() == null) ? 0 : getExcludeFeaturesAttribute().hashCode());
return hashCode;
}
@Override
public EndpointInput clone() {
try {
return (EndpointInput) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.EndpointInputMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}