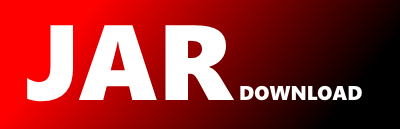
com.amazonaws.services.sagemaker.model.HumanLoopConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the work to be performed by human workers.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class HumanLoopConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* Amazon Resource Name (ARN) of a team of workers. To learn more about the types of workforces and work teams you
* can create and use with Amazon A2I, see Create and Manage
* Workforces.
*
*/
private String workteamArn;
/**
*
* The Amazon Resource Name (ARN) of the human task user interface.
*
*
* You can use standard HTML and Crowd HTML Elements to create a custom worker task template. You use this template
* to create a human task UI.
*
*
* To learn how to create a custom HTML template, see Create Custom Worker Task
* Template.
*
*
* To learn how to create a human task UI, which is a worker task template that can be used in a flow definition,
* see Create and Delete
* a Worker Task Templates.
*
*/
private String humanTaskUiArn;
/**
*
* A title for the human worker task.
*
*/
private String taskTitle;
/**
*
* A description for the human worker task.
*
*/
private String taskDescription;
/**
*
* The number of distinct workers who will perform the same task on each object. For example, if
* TaskCount
is set to 3
for an image classification labeling job, three workers will
* classify each input image. Increasing TaskCount
can improve label accuracy.
*
*/
private Integer taskCount;
/**
*
* The length of time that a task remains available for review by human workers.
*
*/
private Integer taskAvailabilityLifetimeInSeconds;
/**
*
* The amount of time that a worker has to complete a task. The default value is 3,600 seconds (1 hour).
*
*/
private Integer taskTimeLimitInSeconds;
/**
*
* Keywords used to describe the task so that workers can discover the task.
*
*/
private java.util.List taskKeywords;
private PublicWorkforceTaskPrice publicWorkforceTaskPrice;
/**
*
* Amazon Resource Name (ARN) of a team of workers. To learn more about the types of workforces and work teams you
* can create and use with Amazon A2I, see Create and Manage
* Workforces.
*
*
* @param workteamArn
* Amazon Resource Name (ARN) of a team of workers. To learn more about the types of workforces and work
* teams you can create and use with Amazon A2I, see Create and Manage
* Workforces.
*/
public void setWorkteamArn(String workteamArn) {
this.workteamArn = workteamArn;
}
/**
*
* Amazon Resource Name (ARN) of a team of workers. To learn more about the types of workforces and work teams you
* can create and use with Amazon A2I, see Create and Manage
* Workforces.
*
*
* @return Amazon Resource Name (ARN) of a team of workers. To learn more about the types of workforces and work
* teams you can create and use with Amazon A2I, see Create and Manage
* Workforces.
*/
public String getWorkteamArn() {
return this.workteamArn;
}
/**
*
* Amazon Resource Name (ARN) of a team of workers. To learn more about the types of workforces and work teams you
* can create and use with Amazon A2I, see Create and Manage
* Workforces.
*
*
* @param workteamArn
* Amazon Resource Name (ARN) of a team of workers. To learn more about the types of workforces and work
* teams you can create and use with Amazon A2I, see Create and Manage
* Workforces.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withWorkteamArn(String workteamArn) {
setWorkteamArn(workteamArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the human task user interface.
*
*
* You can use standard HTML and Crowd HTML Elements to create a custom worker task template. You use this template
* to create a human task UI.
*
*
* To learn how to create a custom HTML template, see Create Custom Worker Task
* Template.
*
*
* To learn how to create a human task UI, which is a worker task template that can be used in a flow definition,
* see Create and Delete
* a Worker Task Templates.
*
*
* @param humanTaskUiArn
* The Amazon Resource Name (ARN) of the human task user interface.
*
* You can use standard HTML and Crowd HTML Elements to create a custom worker task template. You use this
* template to create a human task UI.
*
*
* To learn how to create a custom HTML template, see Create Custom Worker Task
* Template.
*
*
* To learn how to create a human task UI, which is a worker task template that can be used in a flow
* definition, see Create and Delete
* a Worker Task Templates.
*/
public void setHumanTaskUiArn(String humanTaskUiArn) {
this.humanTaskUiArn = humanTaskUiArn;
}
/**
*
* The Amazon Resource Name (ARN) of the human task user interface.
*
*
* You can use standard HTML and Crowd HTML Elements to create a custom worker task template. You use this template
* to create a human task UI.
*
*
* To learn how to create a custom HTML template, see Create Custom Worker Task
* Template.
*
*
* To learn how to create a human task UI, which is a worker task template that can be used in a flow definition,
* see Create and Delete
* a Worker Task Templates.
*
*
* @return The Amazon Resource Name (ARN) of the human task user interface.
*
* You can use standard HTML and Crowd HTML Elements to create a custom worker task template. You use this
* template to create a human task UI.
*
*
* To learn how to create a custom HTML template, see Create Custom Worker
* Task Template.
*
*
* To learn how to create a human task UI, which is a worker task template that can be used in a flow
* definition, see Create and Delete
* a Worker Task Templates.
*/
public String getHumanTaskUiArn() {
return this.humanTaskUiArn;
}
/**
*
* The Amazon Resource Name (ARN) of the human task user interface.
*
*
* You can use standard HTML and Crowd HTML Elements to create a custom worker task template. You use this template
* to create a human task UI.
*
*
* To learn how to create a custom HTML template, see Create Custom Worker Task
* Template.
*
*
* To learn how to create a human task UI, which is a worker task template that can be used in a flow definition,
* see Create and Delete
* a Worker Task Templates.
*
*
* @param humanTaskUiArn
* The Amazon Resource Name (ARN) of the human task user interface.
*
* You can use standard HTML and Crowd HTML Elements to create a custom worker task template. You use this
* template to create a human task UI.
*
*
* To learn how to create a custom HTML template, see Create Custom Worker Task
* Template.
*
*
* To learn how to create a human task UI, which is a worker task template that can be used in a flow
* definition, see Create and Delete
* a Worker Task Templates.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withHumanTaskUiArn(String humanTaskUiArn) {
setHumanTaskUiArn(humanTaskUiArn);
return this;
}
/**
*
* A title for the human worker task.
*
*
* @param taskTitle
* A title for the human worker task.
*/
public void setTaskTitle(String taskTitle) {
this.taskTitle = taskTitle;
}
/**
*
* A title for the human worker task.
*
*
* @return A title for the human worker task.
*/
public String getTaskTitle() {
return this.taskTitle;
}
/**
*
* A title for the human worker task.
*
*
* @param taskTitle
* A title for the human worker task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withTaskTitle(String taskTitle) {
setTaskTitle(taskTitle);
return this;
}
/**
*
* A description for the human worker task.
*
*
* @param taskDescription
* A description for the human worker task.
*/
public void setTaskDescription(String taskDescription) {
this.taskDescription = taskDescription;
}
/**
*
* A description for the human worker task.
*
*
* @return A description for the human worker task.
*/
public String getTaskDescription() {
return this.taskDescription;
}
/**
*
* A description for the human worker task.
*
*
* @param taskDescription
* A description for the human worker task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withTaskDescription(String taskDescription) {
setTaskDescription(taskDescription);
return this;
}
/**
*
* The number of distinct workers who will perform the same task on each object. For example, if
* TaskCount
is set to 3
for an image classification labeling job, three workers will
* classify each input image. Increasing TaskCount
can improve label accuracy.
*
*
* @param taskCount
* The number of distinct workers who will perform the same task on each object. For example, if
* TaskCount
is set to 3
for an image classification labeling job, three workers
* will classify each input image. Increasing TaskCount
can improve label accuracy.
*/
public void setTaskCount(Integer taskCount) {
this.taskCount = taskCount;
}
/**
*
* The number of distinct workers who will perform the same task on each object. For example, if
* TaskCount
is set to 3
for an image classification labeling job, three workers will
* classify each input image. Increasing TaskCount
can improve label accuracy.
*
*
* @return The number of distinct workers who will perform the same task on each object. For example, if
* TaskCount
is set to 3
for an image classification labeling job, three workers
* will classify each input image. Increasing TaskCount
can improve label accuracy.
*/
public Integer getTaskCount() {
return this.taskCount;
}
/**
*
* The number of distinct workers who will perform the same task on each object. For example, if
* TaskCount
is set to 3
for an image classification labeling job, three workers will
* classify each input image. Increasing TaskCount
can improve label accuracy.
*
*
* @param taskCount
* The number of distinct workers who will perform the same task on each object. For example, if
* TaskCount
is set to 3
for an image classification labeling job, three workers
* will classify each input image. Increasing TaskCount
can improve label accuracy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withTaskCount(Integer taskCount) {
setTaskCount(taskCount);
return this;
}
/**
*
* The length of time that a task remains available for review by human workers.
*
*
* @param taskAvailabilityLifetimeInSeconds
* The length of time that a task remains available for review by human workers.
*/
public void setTaskAvailabilityLifetimeInSeconds(Integer taskAvailabilityLifetimeInSeconds) {
this.taskAvailabilityLifetimeInSeconds = taskAvailabilityLifetimeInSeconds;
}
/**
*
* The length of time that a task remains available for review by human workers.
*
*
* @return The length of time that a task remains available for review by human workers.
*/
public Integer getTaskAvailabilityLifetimeInSeconds() {
return this.taskAvailabilityLifetimeInSeconds;
}
/**
*
* The length of time that a task remains available for review by human workers.
*
*
* @param taskAvailabilityLifetimeInSeconds
* The length of time that a task remains available for review by human workers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withTaskAvailabilityLifetimeInSeconds(Integer taskAvailabilityLifetimeInSeconds) {
setTaskAvailabilityLifetimeInSeconds(taskAvailabilityLifetimeInSeconds);
return this;
}
/**
*
* The amount of time that a worker has to complete a task. The default value is 3,600 seconds (1 hour).
*
*
* @param taskTimeLimitInSeconds
* The amount of time that a worker has to complete a task. The default value is 3,600 seconds (1 hour).
*/
public void setTaskTimeLimitInSeconds(Integer taskTimeLimitInSeconds) {
this.taskTimeLimitInSeconds = taskTimeLimitInSeconds;
}
/**
*
* The amount of time that a worker has to complete a task. The default value is 3,600 seconds (1 hour).
*
*
* @return The amount of time that a worker has to complete a task. The default value is 3,600 seconds (1 hour).
*/
public Integer getTaskTimeLimitInSeconds() {
return this.taskTimeLimitInSeconds;
}
/**
*
* The amount of time that a worker has to complete a task. The default value is 3,600 seconds (1 hour).
*
*
* @param taskTimeLimitInSeconds
* The amount of time that a worker has to complete a task. The default value is 3,600 seconds (1 hour).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withTaskTimeLimitInSeconds(Integer taskTimeLimitInSeconds) {
setTaskTimeLimitInSeconds(taskTimeLimitInSeconds);
return this;
}
/**
*
* Keywords used to describe the task so that workers can discover the task.
*
*
* @return Keywords used to describe the task so that workers can discover the task.
*/
public java.util.List getTaskKeywords() {
return taskKeywords;
}
/**
*
* Keywords used to describe the task so that workers can discover the task.
*
*
* @param taskKeywords
* Keywords used to describe the task so that workers can discover the task.
*/
public void setTaskKeywords(java.util.Collection taskKeywords) {
if (taskKeywords == null) {
this.taskKeywords = null;
return;
}
this.taskKeywords = new java.util.ArrayList(taskKeywords);
}
/**
*
* Keywords used to describe the task so that workers can discover the task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTaskKeywords(java.util.Collection)} or {@link #withTaskKeywords(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param taskKeywords
* Keywords used to describe the task so that workers can discover the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withTaskKeywords(String... taskKeywords) {
if (this.taskKeywords == null) {
setTaskKeywords(new java.util.ArrayList(taskKeywords.length));
}
for (String ele : taskKeywords) {
this.taskKeywords.add(ele);
}
return this;
}
/**
*
* Keywords used to describe the task so that workers can discover the task.
*
*
* @param taskKeywords
* Keywords used to describe the task so that workers can discover the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withTaskKeywords(java.util.Collection taskKeywords) {
setTaskKeywords(taskKeywords);
return this;
}
/**
* @param publicWorkforceTaskPrice
*/
public void setPublicWorkforceTaskPrice(PublicWorkforceTaskPrice publicWorkforceTaskPrice) {
this.publicWorkforceTaskPrice = publicWorkforceTaskPrice;
}
/**
* @return
*/
public PublicWorkforceTaskPrice getPublicWorkforceTaskPrice() {
return this.publicWorkforceTaskPrice;
}
/**
* @param publicWorkforceTaskPrice
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HumanLoopConfig withPublicWorkforceTaskPrice(PublicWorkforceTaskPrice publicWorkforceTaskPrice) {
setPublicWorkforceTaskPrice(publicWorkforceTaskPrice);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getWorkteamArn() != null)
sb.append("WorkteamArn: ").append(getWorkteamArn()).append(",");
if (getHumanTaskUiArn() != null)
sb.append("HumanTaskUiArn: ").append(getHumanTaskUiArn()).append(",");
if (getTaskTitle() != null)
sb.append("TaskTitle: ").append(getTaskTitle()).append(",");
if (getTaskDescription() != null)
sb.append("TaskDescription: ").append(getTaskDescription()).append(",");
if (getTaskCount() != null)
sb.append("TaskCount: ").append(getTaskCount()).append(",");
if (getTaskAvailabilityLifetimeInSeconds() != null)
sb.append("TaskAvailabilityLifetimeInSeconds: ").append(getTaskAvailabilityLifetimeInSeconds()).append(",");
if (getTaskTimeLimitInSeconds() != null)
sb.append("TaskTimeLimitInSeconds: ").append(getTaskTimeLimitInSeconds()).append(",");
if (getTaskKeywords() != null)
sb.append("TaskKeywords: ").append(getTaskKeywords()).append(",");
if (getPublicWorkforceTaskPrice() != null)
sb.append("PublicWorkforceTaskPrice: ").append(getPublicWorkforceTaskPrice());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof HumanLoopConfig == false)
return false;
HumanLoopConfig other = (HumanLoopConfig) obj;
if (other.getWorkteamArn() == null ^ this.getWorkteamArn() == null)
return false;
if (other.getWorkteamArn() != null && other.getWorkteamArn().equals(this.getWorkteamArn()) == false)
return false;
if (other.getHumanTaskUiArn() == null ^ this.getHumanTaskUiArn() == null)
return false;
if (other.getHumanTaskUiArn() != null && other.getHumanTaskUiArn().equals(this.getHumanTaskUiArn()) == false)
return false;
if (other.getTaskTitle() == null ^ this.getTaskTitle() == null)
return false;
if (other.getTaskTitle() != null && other.getTaskTitle().equals(this.getTaskTitle()) == false)
return false;
if (other.getTaskDescription() == null ^ this.getTaskDescription() == null)
return false;
if (other.getTaskDescription() != null && other.getTaskDescription().equals(this.getTaskDescription()) == false)
return false;
if (other.getTaskCount() == null ^ this.getTaskCount() == null)
return false;
if (other.getTaskCount() != null && other.getTaskCount().equals(this.getTaskCount()) == false)
return false;
if (other.getTaskAvailabilityLifetimeInSeconds() == null ^ this.getTaskAvailabilityLifetimeInSeconds() == null)
return false;
if (other.getTaskAvailabilityLifetimeInSeconds() != null
&& other.getTaskAvailabilityLifetimeInSeconds().equals(this.getTaskAvailabilityLifetimeInSeconds()) == false)
return false;
if (other.getTaskTimeLimitInSeconds() == null ^ this.getTaskTimeLimitInSeconds() == null)
return false;
if (other.getTaskTimeLimitInSeconds() != null && other.getTaskTimeLimitInSeconds().equals(this.getTaskTimeLimitInSeconds()) == false)
return false;
if (other.getTaskKeywords() == null ^ this.getTaskKeywords() == null)
return false;
if (other.getTaskKeywords() != null && other.getTaskKeywords().equals(this.getTaskKeywords()) == false)
return false;
if (other.getPublicWorkforceTaskPrice() == null ^ this.getPublicWorkforceTaskPrice() == null)
return false;
if (other.getPublicWorkforceTaskPrice() != null && other.getPublicWorkforceTaskPrice().equals(this.getPublicWorkforceTaskPrice()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getWorkteamArn() == null) ? 0 : getWorkteamArn().hashCode());
hashCode = prime * hashCode + ((getHumanTaskUiArn() == null) ? 0 : getHumanTaskUiArn().hashCode());
hashCode = prime * hashCode + ((getTaskTitle() == null) ? 0 : getTaskTitle().hashCode());
hashCode = prime * hashCode + ((getTaskDescription() == null) ? 0 : getTaskDescription().hashCode());
hashCode = prime * hashCode + ((getTaskCount() == null) ? 0 : getTaskCount().hashCode());
hashCode = prime * hashCode + ((getTaskAvailabilityLifetimeInSeconds() == null) ? 0 : getTaskAvailabilityLifetimeInSeconds().hashCode());
hashCode = prime * hashCode + ((getTaskTimeLimitInSeconds() == null) ? 0 : getTaskTimeLimitInSeconds().hashCode());
hashCode = prime * hashCode + ((getTaskKeywords() == null) ? 0 : getTaskKeywords().hashCode());
hashCode = prime * hashCode + ((getPublicWorkforceTaskPrice() == null) ? 0 : getPublicWorkforceTaskPrice().hashCode());
return hashCode;
}
@Override
public HumanLoopConfig clone() {
try {
return (HumanLoopConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.HumanLoopConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}