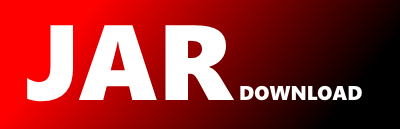
com.amazonaws.services.sagemaker.model.HyperParameterTrainingJobDefinition Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Defines the training jobs launched by a hyperparameter tuning job.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class HyperParameterTrainingJobDefinition implements Serializable, Cloneable, StructuredPojo {
/**
*
* The job definition name.
*
*/
private String definitionName;
private HyperParameterTuningJobObjective tuningObjective;
private ParameterRanges hyperParameterRanges;
/**
*
* Specifies the values of hyperparameters that do not change for the tuning job.
*
*/
private java.util.Map staticHyperParameters;
/**
*
* The HyperParameterAlgorithmSpecification object that specifies the resource algorithm to use for the training
* jobs that the tuning job launches.
*
*/
private HyperParameterAlgorithmSpecification algorithmSpecification;
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the training jobs that the tuning job launches.
*
*/
private String roleArn;
/**
*
* An array of Channel
* objects that specify the input for the training jobs that the tuning job launches.
*
*/
private java.util.List inputDataConfig;
/**
*
* The VpcConfig object
* that specifies the VPC that you want the training jobs that this hyperparameter tuning job launches to connect
* to. Control access to and from your training container by configuring the VPC. For more information, see Protect Training Jobs by Using an Amazon
* Virtual Private Cloud.
*
*/
private VpcConfig vpcConfig;
/**
*
* Specifies the path to the Amazon S3 bucket where you store model artifacts from the training jobs that the tuning
* job launches.
*
*/
private OutputDataConfig outputDataConfig;
/**
*
* The resources, including the compute instances and storage volumes, to use for the training jobs that the tuning
* job launches.
*
*
* Storage volumes store model artifacts and incremental states. Training algorithms might also use storage volumes
* for scratch space. If you want SageMaker to use the storage volume to store the training data, choose
* File
as the TrainingInputMode
in the algorithm specification. For distributed training
* algorithms, specify an instance count greater than 1.
*
*
*
* If you want to use hyperparameter optimization with instance type flexibility, use
* HyperParameterTuningResourceConfig
instead.
*
*
*/
private ResourceConfig resourceConfig;
/**
*
* The configuration for the hyperparameter tuning resources, including the compute instances and storage volumes,
* used for training jobs launched by the tuning job. By default, storage volumes hold model artifacts and
* incremental states. Choose File
for TrainingInputMode
in the
* AlgorithmSpecification
parameter to additionally store training data in the storage volume
* (optional).
*
*/
private HyperParameterTuningResourceConfig hyperParameterTuningResourceConfig;
/**
*
* Specifies a limit to how long a model hyperparameter training job can run. It also specifies how long a managed
* spot training job has to complete. When the job reaches the time limit, SageMaker ends the training job. Use this
* API to cap model training costs.
*
*/
private StoppingCondition stoppingCondition;
/**
*
* Isolates the training container. No inbound or outbound network calls can be made, except for calls between peers
* within a training cluster for distributed training. If network isolation is used for training jobs that are
* configured to use a VPC, SageMaker downloads and uploads customer data and model artifacts through the specified
* VPC, but the training container does not have network access.
*
*/
private Boolean enableNetworkIsolation;
/**
*
* To encrypt all communications between ML compute instances in distributed training, choose True
.
* Encryption provides greater security for distributed training, but training might take longer. How long it takes
* depends on the amount of communication between compute instances, especially if you use a deep learning algorithm
* in distributed training.
*
*/
private Boolean enableInterContainerTrafficEncryption;
/**
*
* A Boolean indicating whether managed spot training is enabled (True
) or not (False
).
*
*/
private Boolean enableManagedSpotTraining;
private CheckpointConfig checkpointConfig;
/**
*
* The number of times to retry the job when the job fails due to an InternalServerError
.
*
*/
private RetryStrategy retryStrategy;
/**
*
* An environment variable that you can pass into the SageMaker CreateTrainingJob
* API. You can use an existing environment variable from the training container or use your own. See Define metrics and variables for more information.
*
*
*
* The maximum number of items specified for Map Entries
refers to the maximum number of environment
* variables for each TrainingJobDefinition
and also the maximum for the hyperparameter tuning job
* itself. That is, the sum of the number of environment variables for all the training job definitions can't exceed
* the maximum number specified.
*
*
*/
private java.util.Map environment;
/**
*
* The job definition name.
*
*
* @param definitionName
* The job definition name.
*/
public void setDefinitionName(String definitionName) {
this.definitionName = definitionName;
}
/**
*
* The job definition name.
*
*
* @return The job definition name.
*/
public String getDefinitionName() {
return this.definitionName;
}
/**
*
* The job definition name.
*
*
* @param definitionName
* The job definition name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withDefinitionName(String definitionName) {
setDefinitionName(definitionName);
return this;
}
/**
* @param tuningObjective
*/
public void setTuningObjective(HyperParameterTuningJobObjective tuningObjective) {
this.tuningObjective = tuningObjective;
}
/**
* @return
*/
public HyperParameterTuningJobObjective getTuningObjective() {
return this.tuningObjective;
}
/**
* @param tuningObjective
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withTuningObjective(HyperParameterTuningJobObjective tuningObjective) {
setTuningObjective(tuningObjective);
return this;
}
/**
* @param hyperParameterRanges
*/
public void setHyperParameterRanges(ParameterRanges hyperParameterRanges) {
this.hyperParameterRanges = hyperParameterRanges;
}
/**
* @return
*/
public ParameterRanges getHyperParameterRanges() {
return this.hyperParameterRanges;
}
/**
* @param hyperParameterRanges
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withHyperParameterRanges(ParameterRanges hyperParameterRanges) {
setHyperParameterRanges(hyperParameterRanges);
return this;
}
/**
*
* Specifies the values of hyperparameters that do not change for the tuning job.
*
*
* @return Specifies the values of hyperparameters that do not change for the tuning job.
*/
public java.util.Map getStaticHyperParameters() {
return staticHyperParameters;
}
/**
*
* Specifies the values of hyperparameters that do not change for the tuning job.
*
*
* @param staticHyperParameters
* Specifies the values of hyperparameters that do not change for the tuning job.
*/
public void setStaticHyperParameters(java.util.Map staticHyperParameters) {
this.staticHyperParameters = staticHyperParameters;
}
/**
*
* Specifies the values of hyperparameters that do not change for the tuning job.
*
*
* @param staticHyperParameters
* Specifies the values of hyperparameters that do not change for the tuning job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withStaticHyperParameters(java.util.Map staticHyperParameters) {
setStaticHyperParameters(staticHyperParameters);
return this;
}
/**
* Add a single StaticHyperParameters entry
*
* @see HyperParameterTrainingJobDefinition#withStaticHyperParameters
* @returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition addStaticHyperParametersEntry(String key, String value) {
if (null == this.staticHyperParameters) {
this.staticHyperParameters = new java.util.HashMap();
}
if (this.staticHyperParameters.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.staticHyperParameters.put(key, value);
return this;
}
/**
* Removes all the entries added into StaticHyperParameters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition clearStaticHyperParametersEntries() {
this.staticHyperParameters = null;
return this;
}
/**
*
* The HyperParameterAlgorithmSpecification object that specifies the resource algorithm to use for the training
* jobs that the tuning job launches.
*
*
* @param algorithmSpecification
* The HyperParameterAlgorithmSpecification object that specifies the resource algorithm to use for the
* training jobs that the tuning job launches.
*/
public void setAlgorithmSpecification(HyperParameterAlgorithmSpecification algorithmSpecification) {
this.algorithmSpecification = algorithmSpecification;
}
/**
*
* The HyperParameterAlgorithmSpecification object that specifies the resource algorithm to use for the training
* jobs that the tuning job launches.
*
*
* @return The HyperParameterAlgorithmSpecification object that specifies the resource algorithm to use for the
* training jobs that the tuning job launches.
*/
public HyperParameterAlgorithmSpecification getAlgorithmSpecification() {
return this.algorithmSpecification;
}
/**
*
* The HyperParameterAlgorithmSpecification object that specifies the resource algorithm to use for the training
* jobs that the tuning job launches.
*
*
* @param algorithmSpecification
* The HyperParameterAlgorithmSpecification object that specifies the resource algorithm to use for the
* training jobs that the tuning job launches.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withAlgorithmSpecification(HyperParameterAlgorithmSpecification algorithmSpecification) {
setAlgorithmSpecification(algorithmSpecification);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the training jobs that the tuning job launches.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role associated with the training jobs that the tuning job
* launches.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the training jobs that the tuning job launches.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role associated with the training jobs that the tuning job
* launches.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the training jobs that the tuning job launches.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role associated with the training jobs that the tuning job
* launches.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* An array of Channel
* objects that specify the input for the training jobs that the tuning job launches.
*
*
* @return An array of Channel objects
* that specify the input for the training jobs that the tuning job launches.
*/
public java.util.List getInputDataConfig() {
return inputDataConfig;
}
/**
*
* An array of Channel
* objects that specify the input for the training jobs that the tuning job launches.
*
*
* @param inputDataConfig
* An array of Channel objects that
* specify the input for the training jobs that the tuning job launches.
*/
public void setInputDataConfig(java.util.Collection inputDataConfig) {
if (inputDataConfig == null) {
this.inputDataConfig = null;
return;
}
this.inputDataConfig = new java.util.ArrayList(inputDataConfig);
}
/**
*
* An array of Channel
* objects that specify the input for the training jobs that the tuning job launches.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInputDataConfig(java.util.Collection)} or {@link #withInputDataConfig(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param inputDataConfig
* An array of Channel objects that
* specify the input for the training jobs that the tuning job launches.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withInputDataConfig(Channel... inputDataConfig) {
if (this.inputDataConfig == null) {
setInputDataConfig(new java.util.ArrayList(inputDataConfig.length));
}
for (Channel ele : inputDataConfig) {
this.inputDataConfig.add(ele);
}
return this;
}
/**
*
* An array of Channel
* objects that specify the input for the training jobs that the tuning job launches.
*
*
* @param inputDataConfig
* An array of Channel objects that
* specify the input for the training jobs that the tuning job launches.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withInputDataConfig(java.util.Collection inputDataConfig) {
setInputDataConfig(inputDataConfig);
return this;
}
/**
*
* The VpcConfig object
* that specifies the VPC that you want the training jobs that this hyperparameter tuning job launches to connect
* to. Control access to and from your training container by configuring the VPC. For more information, see Protect Training Jobs by Using an Amazon
* Virtual Private Cloud.
*
*
* @param vpcConfig
* The VpcConfig
* object that specifies the VPC that you want the training jobs that this hyperparameter tuning job launches
* to connect to. Control access to and from your training container by configuring the VPC. For more
* information, see Protect Training
* Jobs by Using an Amazon Virtual Private Cloud.
*/
public void setVpcConfig(VpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
*
* The VpcConfig object
* that specifies the VPC that you want the training jobs that this hyperparameter tuning job launches to connect
* to. Control access to and from your training container by configuring the VPC. For more information, see Protect Training Jobs by Using an Amazon
* Virtual Private Cloud.
*
*
* @return The VpcConfig
* object that specifies the VPC that you want the training jobs that this hyperparameter tuning job
* launches to connect to. Control access to and from your training container by configuring the VPC. For
* more information, see Protect
* Training Jobs by Using an Amazon Virtual Private Cloud.
*/
public VpcConfig getVpcConfig() {
return this.vpcConfig;
}
/**
*
* The VpcConfig object
* that specifies the VPC that you want the training jobs that this hyperparameter tuning job launches to connect
* to. Control access to and from your training container by configuring the VPC. For more information, see Protect Training Jobs by Using an Amazon
* Virtual Private Cloud.
*
*
* @param vpcConfig
* The VpcConfig
* object that specifies the VPC that you want the training jobs that this hyperparameter tuning job launches
* to connect to. Control access to and from your training container by configuring the VPC. For more
* information, see Protect Training
* Jobs by Using an Amazon Virtual Private Cloud.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withVpcConfig(VpcConfig vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
*
* Specifies the path to the Amazon S3 bucket where you store model artifacts from the training jobs that the tuning
* job launches.
*
*
* @param outputDataConfig
* Specifies the path to the Amazon S3 bucket where you store model artifacts from the training jobs that the
* tuning job launches.
*/
public void setOutputDataConfig(OutputDataConfig outputDataConfig) {
this.outputDataConfig = outputDataConfig;
}
/**
*
* Specifies the path to the Amazon S3 bucket where you store model artifacts from the training jobs that the tuning
* job launches.
*
*
* @return Specifies the path to the Amazon S3 bucket where you store model artifacts from the training jobs that
* the tuning job launches.
*/
public OutputDataConfig getOutputDataConfig() {
return this.outputDataConfig;
}
/**
*
* Specifies the path to the Amazon S3 bucket where you store model artifacts from the training jobs that the tuning
* job launches.
*
*
* @param outputDataConfig
* Specifies the path to the Amazon S3 bucket where you store model artifacts from the training jobs that the
* tuning job launches.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withOutputDataConfig(OutputDataConfig outputDataConfig) {
setOutputDataConfig(outputDataConfig);
return this;
}
/**
*
* The resources, including the compute instances and storage volumes, to use for the training jobs that the tuning
* job launches.
*
*
* Storage volumes store model artifacts and incremental states. Training algorithms might also use storage volumes
* for scratch space. If you want SageMaker to use the storage volume to store the training data, choose
* File
as the TrainingInputMode
in the algorithm specification. For distributed training
* algorithms, specify an instance count greater than 1.
*
*
*
* If you want to use hyperparameter optimization with instance type flexibility, use
* HyperParameterTuningResourceConfig
instead.
*
*
*
* @param resourceConfig
* The resources, including the compute instances and storage volumes, to use for the training jobs that the
* tuning job launches.
*
* Storage volumes store model artifacts and incremental states. Training algorithms might also use storage
* volumes for scratch space. If you want SageMaker to use the storage volume to store the training data,
* choose File
as the TrainingInputMode
in the algorithm specification. For
* distributed training algorithms, specify an instance count greater than 1.
*
*
*
* If you want to use hyperparameter optimization with instance type flexibility, use
* HyperParameterTuningResourceConfig
instead.
*
*/
public void setResourceConfig(ResourceConfig resourceConfig) {
this.resourceConfig = resourceConfig;
}
/**
*
* The resources, including the compute instances and storage volumes, to use for the training jobs that the tuning
* job launches.
*
*
* Storage volumes store model artifacts and incremental states. Training algorithms might also use storage volumes
* for scratch space. If you want SageMaker to use the storage volume to store the training data, choose
* File
as the TrainingInputMode
in the algorithm specification. For distributed training
* algorithms, specify an instance count greater than 1.
*
*
*
* If you want to use hyperparameter optimization with instance type flexibility, use
* HyperParameterTuningResourceConfig
instead.
*
*
*
* @return The resources, including the compute instances and storage volumes, to use for the training jobs that the
* tuning job launches.
*
* Storage volumes store model artifacts and incremental states. Training algorithms might also use storage
* volumes for scratch space. If you want SageMaker to use the storage volume to store the training data,
* choose File
as the TrainingInputMode
in the algorithm specification. For
* distributed training algorithms, specify an instance count greater than 1.
*
*
*
* If you want to use hyperparameter optimization with instance type flexibility, use
* HyperParameterTuningResourceConfig
instead.
*
*/
public ResourceConfig getResourceConfig() {
return this.resourceConfig;
}
/**
*
* The resources, including the compute instances and storage volumes, to use for the training jobs that the tuning
* job launches.
*
*
* Storage volumes store model artifacts and incremental states. Training algorithms might also use storage volumes
* for scratch space. If you want SageMaker to use the storage volume to store the training data, choose
* File
as the TrainingInputMode
in the algorithm specification. For distributed training
* algorithms, specify an instance count greater than 1.
*
*
*
* If you want to use hyperparameter optimization with instance type flexibility, use
* HyperParameterTuningResourceConfig
instead.
*
*
*
* @param resourceConfig
* The resources, including the compute instances and storage volumes, to use for the training jobs that the
* tuning job launches.
*
* Storage volumes store model artifacts and incremental states. Training algorithms might also use storage
* volumes for scratch space. If you want SageMaker to use the storage volume to store the training data,
* choose File
as the TrainingInputMode
in the algorithm specification. For
* distributed training algorithms, specify an instance count greater than 1.
*
*
*
* If you want to use hyperparameter optimization with instance type flexibility, use
* HyperParameterTuningResourceConfig
instead.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withResourceConfig(ResourceConfig resourceConfig) {
setResourceConfig(resourceConfig);
return this;
}
/**
*
* The configuration for the hyperparameter tuning resources, including the compute instances and storage volumes,
* used for training jobs launched by the tuning job. By default, storage volumes hold model artifacts and
* incremental states. Choose File
for TrainingInputMode
in the
* AlgorithmSpecification
parameter to additionally store training data in the storage volume
* (optional).
*
*
* @param hyperParameterTuningResourceConfig
* The configuration for the hyperparameter tuning resources, including the compute instances and storage
* volumes, used for training jobs launched by the tuning job. By default, storage volumes hold model
* artifacts and incremental states. Choose File
for TrainingInputMode
in the
* AlgorithmSpecification
parameter to additionally store training data in the storage volume
* (optional).
*/
public void setHyperParameterTuningResourceConfig(HyperParameterTuningResourceConfig hyperParameterTuningResourceConfig) {
this.hyperParameterTuningResourceConfig = hyperParameterTuningResourceConfig;
}
/**
*
* The configuration for the hyperparameter tuning resources, including the compute instances and storage volumes,
* used for training jobs launched by the tuning job. By default, storage volumes hold model artifacts and
* incremental states. Choose File
for TrainingInputMode
in the
* AlgorithmSpecification
parameter to additionally store training data in the storage volume
* (optional).
*
*
* @return The configuration for the hyperparameter tuning resources, including the compute instances and storage
* volumes, used for training jobs launched by the tuning job. By default, storage volumes hold model
* artifacts and incremental states. Choose File
for TrainingInputMode
in the
* AlgorithmSpecification
parameter to additionally store training data in the storage volume
* (optional).
*/
public HyperParameterTuningResourceConfig getHyperParameterTuningResourceConfig() {
return this.hyperParameterTuningResourceConfig;
}
/**
*
* The configuration for the hyperparameter tuning resources, including the compute instances and storage volumes,
* used for training jobs launched by the tuning job. By default, storage volumes hold model artifacts and
* incremental states. Choose File
for TrainingInputMode
in the
* AlgorithmSpecification
parameter to additionally store training data in the storage volume
* (optional).
*
*
* @param hyperParameterTuningResourceConfig
* The configuration for the hyperparameter tuning resources, including the compute instances and storage
* volumes, used for training jobs launched by the tuning job. By default, storage volumes hold model
* artifacts and incremental states. Choose File
for TrainingInputMode
in the
* AlgorithmSpecification
parameter to additionally store training data in the storage volume
* (optional).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withHyperParameterTuningResourceConfig(HyperParameterTuningResourceConfig hyperParameterTuningResourceConfig) {
setHyperParameterTuningResourceConfig(hyperParameterTuningResourceConfig);
return this;
}
/**
*
* Specifies a limit to how long a model hyperparameter training job can run. It also specifies how long a managed
* spot training job has to complete. When the job reaches the time limit, SageMaker ends the training job. Use this
* API to cap model training costs.
*
*
* @param stoppingCondition
* Specifies a limit to how long a model hyperparameter training job can run. It also specifies how long a
* managed spot training job has to complete. When the job reaches the time limit, SageMaker ends the
* training job. Use this API to cap model training costs.
*/
public void setStoppingCondition(StoppingCondition stoppingCondition) {
this.stoppingCondition = stoppingCondition;
}
/**
*
* Specifies a limit to how long a model hyperparameter training job can run. It also specifies how long a managed
* spot training job has to complete. When the job reaches the time limit, SageMaker ends the training job. Use this
* API to cap model training costs.
*
*
* @return Specifies a limit to how long a model hyperparameter training job can run. It also specifies how long a
* managed spot training job has to complete. When the job reaches the time limit, SageMaker ends the
* training job. Use this API to cap model training costs.
*/
public StoppingCondition getStoppingCondition() {
return this.stoppingCondition;
}
/**
*
* Specifies a limit to how long a model hyperparameter training job can run. It also specifies how long a managed
* spot training job has to complete. When the job reaches the time limit, SageMaker ends the training job. Use this
* API to cap model training costs.
*
*
* @param stoppingCondition
* Specifies a limit to how long a model hyperparameter training job can run. It also specifies how long a
* managed spot training job has to complete. When the job reaches the time limit, SageMaker ends the
* training job. Use this API to cap model training costs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withStoppingCondition(StoppingCondition stoppingCondition) {
setStoppingCondition(stoppingCondition);
return this;
}
/**
*
* Isolates the training container. No inbound or outbound network calls can be made, except for calls between peers
* within a training cluster for distributed training. If network isolation is used for training jobs that are
* configured to use a VPC, SageMaker downloads and uploads customer data and model artifacts through the specified
* VPC, but the training container does not have network access.
*
*
* @param enableNetworkIsolation
* Isolates the training container. No inbound or outbound network calls can be made, except for calls
* between peers within a training cluster for distributed training. If network isolation is used for
* training jobs that are configured to use a VPC, SageMaker downloads and uploads customer data and model
* artifacts through the specified VPC, but the training container does not have network access.
*/
public void setEnableNetworkIsolation(Boolean enableNetworkIsolation) {
this.enableNetworkIsolation = enableNetworkIsolation;
}
/**
*
* Isolates the training container. No inbound or outbound network calls can be made, except for calls between peers
* within a training cluster for distributed training. If network isolation is used for training jobs that are
* configured to use a VPC, SageMaker downloads and uploads customer data and model artifacts through the specified
* VPC, but the training container does not have network access.
*
*
* @return Isolates the training container. No inbound or outbound network calls can be made, except for calls
* between peers within a training cluster for distributed training. If network isolation is used for
* training jobs that are configured to use a VPC, SageMaker downloads and uploads customer data and model
* artifacts through the specified VPC, but the training container does not have network access.
*/
public Boolean getEnableNetworkIsolation() {
return this.enableNetworkIsolation;
}
/**
*
* Isolates the training container. No inbound or outbound network calls can be made, except for calls between peers
* within a training cluster for distributed training. If network isolation is used for training jobs that are
* configured to use a VPC, SageMaker downloads and uploads customer data and model artifacts through the specified
* VPC, but the training container does not have network access.
*
*
* @param enableNetworkIsolation
* Isolates the training container. No inbound or outbound network calls can be made, except for calls
* between peers within a training cluster for distributed training. If network isolation is used for
* training jobs that are configured to use a VPC, SageMaker downloads and uploads customer data and model
* artifacts through the specified VPC, but the training container does not have network access.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withEnableNetworkIsolation(Boolean enableNetworkIsolation) {
setEnableNetworkIsolation(enableNetworkIsolation);
return this;
}
/**
*
* Isolates the training container. No inbound or outbound network calls can be made, except for calls between peers
* within a training cluster for distributed training. If network isolation is used for training jobs that are
* configured to use a VPC, SageMaker downloads and uploads customer data and model artifacts through the specified
* VPC, but the training container does not have network access.
*
*
* @return Isolates the training container. No inbound or outbound network calls can be made, except for calls
* between peers within a training cluster for distributed training. If network isolation is used for
* training jobs that are configured to use a VPC, SageMaker downloads and uploads customer data and model
* artifacts through the specified VPC, but the training container does not have network access.
*/
public Boolean isEnableNetworkIsolation() {
return this.enableNetworkIsolation;
}
/**
*
* To encrypt all communications between ML compute instances in distributed training, choose True
.
* Encryption provides greater security for distributed training, but training might take longer. How long it takes
* depends on the amount of communication between compute instances, especially if you use a deep learning algorithm
* in distributed training.
*
*
* @param enableInterContainerTrafficEncryption
* To encrypt all communications between ML compute instances in distributed training, choose
* True
. Encryption provides greater security for distributed training, but training might take
* longer. How long it takes depends on the amount of communication between compute instances, especially if
* you use a deep learning algorithm in distributed training.
*/
public void setEnableInterContainerTrafficEncryption(Boolean enableInterContainerTrafficEncryption) {
this.enableInterContainerTrafficEncryption = enableInterContainerTrafficEncryption;
}
/**
*
* To encrypt all communications between ML compute instances in distributed training, choose True
.
* Encryption provides greater security for distributed training, but training might take longer. How long it takes
* depends on the amount of communication between compute instances, especially if you use a deep learning algorithm
* in distributed training.
*
*
* @return To encrypt all communications between ML compute instances in distributed training, choose
* True
. Encryption provides greater security for distributed training, but training might take
* longer. How long it takes depends on the amount of communication between compute instances, especially if
* you use a deep learning algorithm in distributed training.
*/
public Boolean getEnableInterContainerTrafficEncryption() {
return this.enableInterContainerTrafficEncryption;
}
/**
*
* To encrypt all communications between ML compute instances in distributed training, choose True
.
* Encryption provides greater security for distributed training, but training might take longer. How long it takes
* depends on the amount of communication between compute instances, especially if you use a deep learning algorithm
* in distributed training.
*
*
* @param enableInterContainerTrafficEncryption
* To encrypt all communications between ML compute instances in distributed training, choose
* True
. Encryption provides greater security for distributed training, but training might take
* longer. How long it takes depends on the amount of communication between compute instances, especially if
* you use a deep learning algorithm in distributed training.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withEnableInterContainerTrafficEncryption(Boolean enableInterContainerTrafficEncryption) {
setEnableInterContainerTrafficEncryption(enableInterContainerTrafficEncryption);
return this;
}
/**
*
* To encrypt all communications between ML compute instances in distributed training, choose True
.
* Encryption provides greater security for distributed training, but training might take longer. How long it takes
* depends on the amount of communication between compute instances, especially if you use a deep learning algorithm
* in distributed training.
*
*
* @return To encrypt all communications between ML compute instances in distributed training, choose
* True
. Encryption provides greater security for distributed training, but training might take
* longer. How long it takes depends on the amount of communication between compute instances, especially if
* you use a deep learning algorithm in distributed training.
*/
public Boolean isEnableInterContainerTrafficEncryption() {
return this.enableInterContainerTrafficEncryption;
}
/**
*
* A Boolean indicating whether managed spot training is enabled (True
) or not (False
).
*
*
* @param enableManagedSpotTraining
* A Boolean indicating whether managed spot training is enabled (True
) or not (
* False
).
*/
public void setEnableManagedSpotTraining(Boolean enableManagedSpotTraining) {
this.enableManagedSpotTraining = enableManagedSpotTraining;
}
/**
*
* A Boolean indicating whether managed spot training is enabled (True
) or not (False
).
*
*
* @return A Boolean indicating whether managed spot training is enabled (True
) or not (
* False
).
*/
public Boolean getEnableManagedSpotTraining() {
return this.enableManagedSpotTraining;
}
/**
*
* A Boolean indicating whether managed spot training is enabled (True
) or not (False
).
*
*
* @param enableManagedSpotTraining
* A Boolean indicating whether managed spot training is enabled (True
) or not (
* False
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withEnableManagedSpotTraining(Boolean enableManagedSpotTraining) {
setEnableManagedSpotTraining(enableManagedSpotTraining);
return this;
}
/**
*
* A Boolean indicating whether managed spot training is enabled (True
) or not (False
).
*
*
* @return A Boolean indicating whether managed spot training is enabled (True
) or not (
* False
).
*/
public Boolean isEnableManagedSpotTraining() {
return this.enableManagedSpotTraining;
}
/**
* @param checkpointConfig
*/
public void setCheckpointConfig(CheckpointConfig checkpointConfig) {
this.checkpointConfig = checkpointConfig;
}
/**
* @return
*/
public CheckpointConfig getCheckpointConfig() {
return this.checkpointConfig;
}
/**
* @param checkpointConfig
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withCheckpointConfig(CheckpointConfig checkpointConfig) {
setCheckpointConfig(checkpointConfig);
return this;
}
/**
*
* The number of times to retry the job when the job fails due to an InternalServerError
.
*
*
* @param retryStrategy
* The number of times to retry the job when the job fails due to an InternalServerError
.
*/
public void setRetryStrategy(RetryStrategy retryStrategy) {
this.retryStrategy = retryStrategy;
}
/**
*
* The number of times to retry the job when the job fails due to an InternalServerError
.
*
*
* @return The number of times to retry the job when the job fails due to an InternalServerError
.
*/
public RetryStrategy getRetryStrategy() {
return this.retryStrategy;
}
/**
*
* The number of times to retry the job when the job fails due to an InternalServerError
.
*
*
* @param retryStrategy
* The number of times to retry the job when the job fails due to an InternalServerError
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withRetryStrategy(RetryStrategy retryStrategy) {
setRetryStrategy(retryStrategy);
return this;
}
/**
*
* An environment variable that you can pass into the SageMaker CreateTrainingJob
* API. You can use an existing environment variable from the training container or use your own. See Define metrics and variables for more information.
*
*
*
* The maximum number of items specified for Map Entries
refers to the maximum number of environment
* variables for each TrainingJobDefinition
and also the maximum for the hyperparameter tuning job
* itself. That is, the sum of the number of environment variables for all the training job definitions can't exceed
* the maximum number specified.
*
*
*
* @return An environment variable that you can pass into the SageMaker CreateTrainingJob API. You can use an existing environment variable from the training container or use your own. See Define metrics and variables for more information.
*
* The maximum number of items specified for Map Entries
refers to the maximum number of
* environment variables for each TrainingJobDefinition
and also the maximum for the
* hyperparameter tuning job itself. That is, the sum of the number of environment variables for all the
* training job definitions can't exceed the maximum number specified.
*
*/
public java.util.Map getEnvironment() {
return environment;
}
/**
*
* An environment variable that you can pass into the SageMaker CreateTrainingJob
* API. You can use an existing environment variable from the training container or use your own. See Define metrics and variables for more information.
*
*
*
* The maximum number of items specified for Map Entries
refers to the maximum number of environment
* variables for each TrainingJobDefinition
and also the maximum for the hyperparameter tuning job
* itself. That is, the sum of the number of environment variables for all the training job definitions can't exceed
* the maximum number specified.
*
*
*
* @param environment
* An environment variable that you can pass into the SageMaker CreateTrainingJob API. You can use an existing environment variable from the training container or use your own. See Define metrics and variables for more information.
*
* The maximum number of items specified for Map Entries
refers to the maximum number of
* environment variables for each TrainingJobDefinition
and also the maximum for the
* hyperparameter tuning job itself. That is, the sum of the number of environment variables for all the
* training job definitions can't exceed the maximum number specified.
*
*/
public void setEnvironment(java.util.Map environment) {
this.environment = environment;
}
/**
*
* An environment variable that you can pass into the SageMaker CreateTrainingJob
* API. You can use an existing environment variable from the training container or use your own. See Define metrics and variables for more information.
*
*
*
* The maximum number of items specified for Map Entries
refers to the maximum number of environment
* variables for each TrainingJobDefinition
and also the maximum for the hyperparameter tuning job
* itself. That is, the sum of the number of environment variables for all the training job definitions can't exceed
* the maximum number specified.
*
*
*
* @param environment
* An environment variable that you can pass into the SageMaker CreateTrainingJob API. You can use an existing environment variable from the training container or use your own. See Define metrics and variables for more information.
*
* The maximum number of items specified for Map Entries
refers to the maximum number of
* environment variables for each TrainingJobDefinition
and also the maximum for the
* hyperparameter tuning job itself. That is, the sum of the number of environment variables for all the
* training job definitions can't exceed the maximum number specified.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition withEnvironment(java.util.Map environment) {
setEnvironment(environment);
return this;
}
/**
* Add a single Environment entry
*
* @see HyperParameterTrainingJobDefinition#withEnvironment
* @returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition addEnvironmentEntry(String key, String value) {
if (null == this.environment) {
this.environment = new java.util.HashMap();
}
if (this.environment.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.environment.put(key, value);
return this;
}
/**
* Removes all the entries added into Environment.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTrainingJobDefinition clearEnvironmentEntries() {
this.environment = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDefinitionName() != null)
sb.append("DefinitionName: ").append(getDefinitionName()).append(",");
if (getTuningObjective() != null)
sb.append("TuningObjective: ").append(getTuningObjective()).append(",");
if (getHyperParameterRanges() != null)
sb.append("HyperParameterRanges: ").append(getHyperParameterRanges()).append(",");
if (getStaticHyperParameters() != null)
sb.append("StaticHyperParameters: ").append(getStaticHyperParameters()).append(",");
if (getAlgorithmSpecification() != null)
sb.append("AlgorithmSpecification: ").append(getAlgorithmSpecification()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getInputDataConfig() != null)
sb.append("InputDataConfig: ").append(getInputDataConfig()).append(",");
if (getVpcConfig() != null)
sb.append("VpcConfig: ").append(getVpcConfig()).append(",");
if (getOutputDataConfig() != null)
sb.append("OutputDataConfig: ").append(getOutputDataConfig()).append(",");
if (getResourceConfig() != null)
sb.append("ResourceConfig: ").append(getResourceConfig()).append(",");
if (getHyperParameterTuningResourceConfig() != null)
sb.append("HyperParameterTuningResourceConfig: ").append(getHyperParameterTuningResourceConfig()).append(",");
if (getStoppingCondition() != null)
sb.append("StoppingCondition: ").append(getStoppingCondition()).append(",");
if (getEnableNetworkIsolation() != null)
sb.append("EnableNetworkIsolation: ").append(getEnableNetworkIsolation()).append(",");
if (getEnableInterContainerTrafficEncryption() != null)
sb.append("EnableInterContainerTrafficEncryption: ").append(getEnableInterContainerTrafficEncryption()).append(",");
if (getEnableManagedSpotTraining() != null)
sb.append("EnableManagedSpotTraining: ").append(getEnableManagedSpotTraining()).append(",");
if (getCheckpointConfig() != null)
sb.append("CheckpointConfig: ").append(getCheckpointConfig()).append(",");
if (getRetryStrategy() != null)
sb.append("RetryStrategy: ").append(getRetryStrategy()).append(",");
if (getEnvironment() != null)
sb.append("Environment: ").append(getEnvironment());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof HyperParameterTrainingJobDefinition == false)
return false;
HyperParameterTrainingJobDefinition other = (HyperParameterTrainingJobDefinition) obj;
if (other.getDefinitionName() == null ^ this.getDefinitionName() == null)
return false;
if (other.getDefinitionName() != null && other.getDefinitionName().equals(this.getDefinitionName()) == false)
return false;
if (other.getTuningObjective() == null ^ this.getTuningObjective() == null)
return false;
if (other.getTuningObjective() != null && other.getTuningObjective().equals(this.getTuningObjective()) == false)
return false;
if (other.getHyperParameterRanges() == null ^ this.getHyperParameterRanges() == null)
return false;
if (other.getHyperParameterRanges() != null && other.getHyperParameterRanges().equals(this.getHyperParameterRanges()) == false)
return false;
if (other.getStaticHyperParameters() == null ^ this.getStaticHyperParameters() == null)
return false;
if (other.getStaticHyperParameters() != null && other.getStaticHyperParameters().equals(this.getStaticHyperParameters()) == false)
return false;
if (other.getAlgorithmSpecification() == null ^ this.getAlgorithmSpecification() == null)
return false;
if (other.getAlgorithmSpecification() != null && other.getAlgorithmSpecification().equals(this.getAlgorithmSpecification()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getInputDataConfig() == null ^ this.getInputDataConfig() == null)
return false;
if (other.getInputDataConfig() != null && other.getInputDataConfig().equals(this.getInputDataConfig()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null && other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
if (other.getOutputDataConfig() == null ^ this.getOutputDataConfig() == null)
return false;
if (other.getOutputDataConfig() != null && other.getOutputDataConfig().equals(this.getOutputDataConfig()) == false)
return false;
if (other.getResourceConfig() == null ^ this.getResourceConfig() == null)
return false;
if (other.getResourceConfig() != null && other.getResourceConfig().equals(this.getResourceConfig()) == false)
return false;
if (other.getHyperParameterTuningResourceConfig() == null ^ this.getHyperParameterTuningResourceConfig() == null)
return false;
if (other.getHyperParameterTuningResourceConfig() != null
&& other.getHyperParameterTuningResourceConfig().equals(this.getHyperParameterTuningResourceConfig()) == false)
return false;
if (other.getStoppingCondition() == null ^ this.getStoppingCondition() == null)
return false;
if (other.getStoppingCondition() != null && other.getStoppingCondition().equals(this.getStoppingCondition()) == false)
return false;
if (other.getEnableNetworkIsolation() == null ^ this.getEnableNetworkIsolation() == null)
return false;
if (other.getEnableNetworkIsolation() != null && other.getEnableNetworkIsolation().equals(this.getEnableNetworkIsolation()) == false)
return false;
if (other.getEnableInterContainerTrafficEncryption() == null ^ this.getEnableInterContainerTrafficEncryption() == null)
return false;
if (other.getEnableInterContainerTrafficEncryption() != null
&& other.getEnableInterContainerTrafficEncryption().equals(this.getEnableInterContainerTrafficEncryption()) == false)
return false;
if (other.getEnableManagedSpotTraining() == null ^ this.getEnableManagedSpotTraining() == null)
return false;
if (other.getEnableManagedSpotTraining() != null && other.getEnableManagedSpotTraining().equals(this.getEnableManagedSpotTraining()) == false)
return false;
if (other.getCheckpointConfig() == null ^ this.getCheckpointConfig() == null)
return false;
if (other.getCheckpointConfig() != null && other.getCheckpointConfig().equals(this.getCheckpointConfig()) == false)
return false;
if (other.getRetryStrategy() == null ^ this.getRetryStrategy() == null)
return false;
if (other.getRetryStrategy() != null && other.getRetryStrategy().equals(this.getRetryStrategy()) == false)
return false;
if (other.getEnvironment() == null ^ this.getEnvironment() == null)
return false;
if (other.getEnvironment() != null && other.getEnvironment().equals(this.getEnvironment()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDefinitionName() == null) ? 0 : getDefinitionName().hashCode());
hashCode = prime * hashCode + ((getTuningObjective() == null) ? 0 : getTuningObjective().hashCode());
hashCode = prime * hashCode + ((getHyperParameterRanges() == null) ? 0 : getHyperParameterRanges().hashCode());
hashCode = prime * hashCode + ((getStaticHyperParameters() == null) ? 0 : getStaticHyperParameters().hashCode());
hashCode = prime * hashCode + ((getAlgorithmSpecification() == null) ? 0 : getAlgorithmSpecification().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getInputDataConfig() == null) ? 0 : getInputDataConfig().hashCode());
hashCode = prime * hashCode + ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
hashCode = prime * hashCode + ((getOutputDataConfig() == null) ? 0 : getOutputDataConfig().hashCode());
hashCode = prime * hashCode + ((getResourceConfig() == null) ? 0 : getResourceConfig().hashCode());
hashCode = prime * hashCode + ((getHyperParameterTuningResourceConfig() == null) ? 0 : getHyperParameterTuningResourceConfig().hashCode());
hashCode = prime * hashCode + ((getStoppingCondition() == null) ? 0 : getStoppingCondition().hashCode());
hashCode = prime * hashCode + ((getEnableNetworkIsolation() == null) ? 0 : getEnableNetworkIsolation().hashCode());
hashCode = prime * hashCode + ((getEnableInterContainerTrafficEncryption() == null) ? 0 : getEnableInterContainerTrafficEncryption().hashCode());
hashCode = prime * hashCode + ((getEnableManagedSpotTraining() == null) ? 0 : getEnableManagedSpotTraining().hashCode());
hashCode = prime * hashCode + ((getCheckpointConfig() == null) ? 0 : getCheckpointConfig().hashCode());
hashCode = prime * hashCode + ((getRetryStrategy() == null) ? 0 : getRetryStrategy().hashCode());
hashCode = prime * hashCode + ((getEnvironment() == null) ? 0 : getEnvironment().hashCode());
return hashCode;
}
@Override
public HyperParameterTrainingJobDefinition clone() {
try {
return (HyperParameterTrainingJobDefinition) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.HyperParameterTrainingJobDefinitionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}