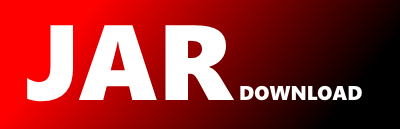
com.amazonaws.services.sagemaker.model.HyperParameterTuningJobConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Configures a hyperparameter tuning job.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class HyperParameterTuningJobConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* Specifies how hyperparameter tuning chooses the combinations of hyperparameter values to use for the training job
* it launches. For information about search strategies, see How
* Hyperparameter Tuning Works.
*
*/
private String strategy;
/**
*
* The configuration for the Hyperband
optimization strategy. This parameter should be provided only if
* Hyperband
is selected as the strategy for HyperParameterTuningJobConfig
.
*
*/
private HyperParameterTuningJobStrategyConfig strategyConfig;
/**
*
* The
* HyperParameterTuningJobObjective specifies the objective metric used to evaluate the performance of training
* jobs launched by this tuning job.
*
*/
private HyperParameterTuningJobObjective hyperParameterTuningJobObjective;
/**
*
* The ResourceLimits
* object that specifies the maximum number of training and parallel training jobs that can be used for this
* hyperparameter tuning job.
*
*/
private ResourceLimits resourceLimits;
/**
*
* The ParameterRanges
* object that specifies the ranges of hyperparameters that this tuning job searches over to find the optimal
* configuration for the highest model performance against your chosen objective metric.
*
*/
private ParameterRanges parameterRanges;
/**
*
* Specifies whether to use early stopping for training jobs launched by the hyperparameter tuning job. Because the
* Hyperband
strategy has its own advanced internal early stopping mechanism,
* TrainingJobEarlyStoppingType
must be OFF
to use Hyperband
. This parameter
* can take on one of the following values (the default value is OFF
):
*
*
* - OFF
* -
*
* Training jobs launched by the hyperparameter tuning job do not use early stopping.
*
*
* - AUTO
* -
*
* SageMaker stops training jobs launched by the hyperparameter tuning job when they are unlikely to perform better
* than previously completed training jobs. For more information, see Stop Training
* Jobs Early.
*
*
*
*/
private String trainingJobEarlyStoppingType;
/**
*
* The tuning job's completion criteria.
*
*/
private TuningJobCompletionCriteria tuningJobCompletionCriteria;
/**
*
* A value used to initialize a pseudo-random number generator. Setting a random seed and using the same seed later
* for the same tuning job will allow hyperparameter optimization to find more a consistent hyperparameter
* configuration between the two runs.
*
*/
private Integer randomSeed;
/**
*
* Specifies how hyperparameter tuning chooses the combinations of hyperparameter values to use for the training job
* it launches. For information about search strategies, see How
* Hyperparameter Tuning Works.
*
*
* @param strategy
* Specifies how hyperparameter tuning chooses the combinations of hyperparameter values to use for the
* training job it launches. For information about search strategies, see How
* Hyperparameter Tuning Works.
* @see HyperParameterTuningJobStrategyType
*/
public void setStrategy(String strategy) {
this.strategy = strategy;
}
/**
*
* Specifies how hyperparameter tuning chooses the combinations of hyperparameter values to use for the training job
* it launches. For information about search strategies, see How
* Hyperparameter Tuning Works.
*
*
* @return Specifies how hyperparameter tuning chooses the combinations of hyperparameter values to use for the
* training job it launches. For information about search strategies, see How
* Hyperparameter Tuning Works.
* @see HyperParameterTuningJobStrategyType
*/
public String getStrategy() {
return this.strategy;
}
/**
*
* Specifies how hyperparameter tuning chooses the combinations of hyperparameter values to use for the training job
* it launches. For information about search strategies, see How
* Hyperparameter Tuning Works.
*
*
* @param strategy
* Specifies how hyperparameter tuning chooses the combinations of hyperparameter values to use for the
* training job it launches. For information about search strategies, see How
* Hyperparameter Tuning Works.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HyperParameterTuningJobStrategyType
*/
public HyperParameterTuningJobConfig withStrategy(String strategy) {
setStrategy(strategy);
return this;
}
/**
*
* Specifies how hyperparameter tuning chooses the combinations of hyperparameter values to use for the training job
* it launches. For information about search strategies, see How
* Hyperparameter Tuning Works.
*
*
* @param strategy
* Specifies how hyperparameter tuning chooses the combinations of hyperparameter values to use for the
* training job it launches. For information about search strategies, see How
* Hyperparameter Tuning Works.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HyperParameterTuningJobStrategyType
*/
public HyperParameterTuningJobConfig withStrategy(HyperParameterTuningJobStrategyType strategy) {
this.strategy = strategy.toString();
return this;
}
/**
*
* The configuration for the Hyperband
optimization strategy. This parameter should be provided only if
* Hyperband
is selected as the strategy for HyperParameterTuningJobConfig
.
*
*
* @param strategyConfig
* The configuration for the Hyperband
optimization strategy. This parameter should be provided
* only if Hyperband
is selected as the strategy for HyperParameterTuningJobConfig
.
*/
public void setStrategyConfig(HyperParameterTuningJobStrategyConfig strategyConfig) {
this.strategyConfig = strategyConfig;
}
/**
*
* The configuration for the Hyperband
optimization strategy. This parameter should be provided only if
* Hyperband
is selected as the strategy for HyperParameterTuningJobConfig
.
*
*
* @return The configuration for the Hyperband
optimization strategy. This parameter should be provided
* only if Hyperband
is selected as the strategy for HyperParameterTuningJobConfig
* .
*/
public HyperParameterTuningJobStrategyConfig getStrategyConfig() {
return this.strategyConfig;
}
/**
*
* The configuration for the Hyperband
optimization strategy. This parameter should be provided only if
* Hyperband
is selected as the strategy for HyperParameterTuningJobConfig
.
*
*
* @param strategyConfig
* The configuration for the Hyperband
optimization strategy. This parameter should be provided
* only if Hyperband
is selected as the strategy for HyperParameterTuningJobConfig
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTuningJobConfig withStrategyConfig(HyperParameterTuningJobStrategyConfig strategyConfig) {
setStrategyConfig(strategyConfig);
return this;
}
/**
*
* The
* HyperParameterTuningJobObjective specifies the objective metric used to evaluate the performance of training
* jobs launched by this tuning job.
*
*
* @param hyperParameterTuningJobObjective
* The HyperParameterTuningJobObjective specifies the objective metric used to evaluate the performance of
* training jobs launched by this tuning job.
*/
public void setHyperParameterTuningJobObjective(HyperParameterTuningJobObjective hyperParameterTuningJobObjective) {
this.hyperParameterTuningJobObjective = hyperParameterTuningJobObjective;
}
/**
*
* The
* HyperParameterTuningJobObjective specifies the objective metric used to evaluate the performance of training
* jobs launched by this tuning job.
*
*
* @return The HyperParameterTuningJobObjective specifies the objective metric used to evaluate the performance of
* training jobs launched by this tuning job.
*/
public HyperParameterTuningJobObjective getHyperParameterTuningJobObjective() {
return this.hyperParameterTuningJobObjective;
}
/**
*
* The
* HyperParameterTuningJobObjective specifies the objective metric used to evaluate the performance of training
* jobs launched by this tuning job.
*
*
* @param hyperParameterTuningJobObjective
* The HyperParameterTuningJobObjective specifies the objective metric used to evaluate the performance of
* training jobs launched by this tuning job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTuningJobConfig withHyperParameterTuningJobObjective(HyperParameterTuningJobObjective hyperParameterTuningJobObjective) {
setHyperParameterTuningJobObjective(hyperParameterTuningJobObjective);
return this;
}
/**
*
* The ResourceLimits
* object that specifies the maximum number of training and parallel training jobs that can be used for this
* hyperparameter tuning job.
*
*
* @param resourceLimits
* The ResourceLimits
* object that specifies the maximum number of training and parallel training jobs that can be used for
* this hyperparameter tuning job.
*/
public void setResourceLimits(ResourceLimits resourceLimits) {
this.resourceLimits = resourceLimits;
}
/**
*
* The ResourceLimits
* object that specifies the maximum number of training and parallel training jobs that can be used for this
* hyperparameter tuning job.
*
*
* @return The ResourceLimits
* object that specifies the maximum number of training and parallel training jobs that can be used for
* this hyperparameter tuning job.
*/
public ResourceLimits getResourceLimits() {
return this.resourceLimits;
}
/**
*
* The ResourceLimits
* object that specifies the maximum number of training and parallel training jobs that can be used for this
* hyperparameter tuning job.
*
*
* @param resourceLimits
* The ResourceLimits
* object that specifies the maximum number of training and parallel training jobs that can be used for
* this hyperparameter tuning job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTuningJobConfig withResourceLimits(ResourceLimits resourceLimits) {
setResourceLimits(resourceLimits);
return this;
}
/**
*
* The ParameterRanges
* object that specifies the ranges of hyperparameters that this tuning job searches over to find the optimal
* configuration for the highest model performance against your chosen objective metric.
*
*
* @param parameterRanges
* The
* ParameterRanges object that specifies the ranges of hyperparameters that this tuning job searches over
* to find the optimal configuration for the highest model performance against your chosen objective metric.
*/
public void setParameterRanges(ParameterRanges parameterRanges) {
this.parameterRanges = parameterRanges;
}
/**
*
* The ParameterRanges
* object that specifies the ranges of hyperparameters that this tuning job searches over to find the optimal
* configuration for the highest model performance against your chosen objective metric.
*
*
* @return The ParameterRanges
* object that specifies the ranges of hyperparameters that this tuning job searches over to find the
* optimal configuration for the highest model performance against your chosen objective metric.
*/
public ParameterRanges getParameterRanges() {
return this.parameterRanges;
}
/**
*
* The ParameterRanges
* object that specifies the ranges of hyperparameters that this tuning job searches over to find the optimal
* configuration for the highest model performance against your chosen objective metric.
*
*
* @param parameterRanges
* The
* ParameterRanges object that specifies the ranges of hyperparameters that this tuning job searches over
* to find the optimal configuration for the highest model performance against your chosen objective metric.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTuningJobConfig withParameterRanges(ParameterRanges parameterRanges) {
setParameterRanges(parameterRanges);
return this;
}
/**
*
* Specifies whether to use early stopping for training jobs launched by the hyperparameter tuning job. Because the
* Hyperband
strategy has its own advanced internal early stopping mechanism,
* TrainingJobEarlyStoppingType
must be OFF
to use Hyperband
. This parameter
* can take on one of the following values (the default value is OFF
):
*
*
* - OFF
* -
*
* Training jobs launched by the hyperparameter tuning job do not use early stopping.
*
*
* - AUTO
* -
*
* SageMaker stops training jobs launched by the hyperparameter tuning job when they are unlikely to perform better
* than previously completed training jobs. For more information, see Stop Training
* Jobs Early.
*
*
*
*
* @param trainingJobEarlyStoppingType
* Specifies whether to use early stopping for training jobs launched by the hyperparameter tuning job.
* Because the Hyperband
strategy has its own advanced internal early stopping mechanism,
* TrainingJobEarlyStoppingType
must be OFF
to use Hyperband
. This
* parameter can take on one of the following values (the default value is OFF
):
*
* - OFF
* -
*
* Training jobs launched by the hyperparameter tuning job do not use early stopping.
*
*
* - AUTO
* -
*
* SageMaker stops training jobs launched by the hyperparameter tuning job when they are unlikely to perform
* better than previously completed training jobs. For more information, see Stop
* Training Jobs Early.
*
*
* @see TrainingJobEarlyStoppingType
*/
public void setTrainingJobEarlyStoppingType(String trainingJobEarlyStoppingType) {
this.trainingJobEarlyStoppingType = trainingJobEarlyStoppingType;
}
/**
*
* Specifies whether to use early stopping for training jobs launched by the hyperparameter tuning job. Because the
* Hyperband
strategy has its own advanced internal early stopping mechanism,
* TrainingJobEarlyStoppingType
must be OFF
to use Hyperband
. This parameter
* can take on one of the following values (the default value is OFF
):
*
*
* - OFF
* -
*
* Training jobs launched by the hyperparameter tuning job do not use early stopping.
*
*
* - AUTO
* -
*
* SageMaker stops training jobs launched by the hyperparameter tuning job when they are unlikely to perform better
* than previously completed training jobs. For more information, see Stop Training
* Jobs Early.
*
*
*
*
* @return Specifies whether to use early stopping for training jobs launched by the hyperparameter tuning job.
* Because the Hyperband
strategy has its own advanced internal early stopping mechanism,
* TrainingJobEarlyStoppingType
must be OFF
to use Hyperband
. This
* parameter can take on one of the following values (the default value is OFF
):
*
* - OFF
* -
*
* Training jobs launched by the hyperparameter tuning job do not use early stopping.
*
*
* - AUTO
* -
*
* SageMaker stops training jobs launched by the hyperparameter tuning job when they are unlikely to perform
* better than previously completed training jobs. For more information, see Stop
* Training Jobs Early.
*
*
* @see TrainingJobEarlyStoppingType
*/
public String getTrainingJobEarlyStoppingType() {
return this.trainingJobEarlyStoppingType;
}
/**
*
* Specifies whether to use early stopping for training jobs launched by the hyperparameter tuning job. Because the
* Hyperband
strategy has its own advanced internal early stopping mechanism,
* TrainingJobEarlyStoppingType
must be OFF
to use Hyperband
. This parameter
* can take on one of the following values (the default value is OFF
):
*
*
* - OFF
* -
*
* Training jobs launched by the hyperparameter tuning job do not use early stopping.
*
*
* - AUTO
* -
*
* SageMaker stops training jobs launched by the hyperparameter tuning job when they are unlikely to perform better
* than previously completed training jobs. For more information, see Stop Training
* Jobs Early.
*
*
*
*
* @param trainingJobEarlyStoppingType
* Specifies whether to use early stopping for training jobs launched by the hyperparameter tuning job.
* Because the Hyperband
strategy has its own advanced internal early stopping mechanism,
* TrainingJobEarlyStoppingType
must be OFF
to use Hyperband
. This
* parameter can take on one of the following values (the default value is OFF
):
*
* - OFF
* -
*
* Training jobs launched by the hyperparameter tuning job do not use early stopping.
*
*
* - AUTO
* -
*
* SageMaker stops training jobs launched by the hyperparameter tuning job when they are unlikely to perform
* better than previously completed training jobs. For more information, see Stop
* Training Jobs Early.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see TrainingJobEarlyStoppingType
*/
public HyperParameterTuningJobConfig withTrainingJobEarlyStoppingType(String trainingJobEarlyStoppingType) {
setTrainingJobEarlyStoppingType(trainingJobEarlyStoppingType);
return this;
}
/**
*
* Specifies whether to use early stopping for training jobs launched by the hyperparameter tuning job. Because the
* Hyperband
strategy has its own advanced internal early stopping mechanism,
* TrainingJobEarlyStoppingType
must be OFF
to use Hyperband
. This parameter
* can take on one of the following values (the default value is OFF
):
*
*
* - OFF
* -
*
* Training jobs launched by the hyperparameter tuning job do not use early stopping.
*
*
* - AUTO
* -
*
* SageMaker stops training jobs launched by the hyperparameter tuning job when they are unlikely to perform better
* than previously completed training jobs. For more information, see Stop Training
* Jobs Early.
*
*
*
*
* @param trainingJobEarlyStoppingType
* Specifies whether to use early stopping for training jobs launched by the hyperparameter tuning job.
* Because the Hyperband
strategy has its own advanced internal early stopping mechanism,
* TrainingJobEarlyStoppingType
must be OFF
to use Hyperband
. This
* parameter can take on one of the following values (the default value is OFF
):
*
* - OFF
* -
*
* Training jobs launched by the hyperparameter tuning job do not use early stopping.
*
*
* - AUTO
* -
*
* SageMaker stops training jobs launched by the hyperparameter tuning job when they are unlikely to perform
* better than previously completed training jobs. For more information, see Stop
* Training Jobs Early.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see TrainingJobEarlyStoppingType
*/
public HyperParameterTuningJobConfig withTrainingJobEarlyStoppingType(TrainingJobEarlyStoppingType trainingJobEarlyStoppingType) {
this.trainingJobEarlyStoppingType = trainingJobEarlyStoppingType.toString();
return this;
}
/**
*
* The tuning job's completion criteria.
*
*
* @param tuningJobCompletionCriteria
* The tuning job's completion criteria.
*/
public void setTuningJobCompletionCriteria(TuningJobCompletionCriteria tuningJobCompletionCriteria) {
this.tuningJobCompletionCriteria = tuningJobCompletionCriteria;
}
/**
*
* The tuning job's completion criteria.
*
*
* @return The tuning job's completion criteria.
*/
public TuningJobCompletionCriteria getTuningJobCompletionCriteria() {
return this.tuningJobCompletionCriteria;
}
/**
*
* The tuning job's completion criteria.
*
*
* @param tuningJobCompletionCriteria
* The tuning job's completion criteria.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTuningJobConfig withTuningJobCompletionCriteria(TuningJobCompletionCriteria tuningJobCompletionCriteria) {
setTuningJobCompletionCriteria(tuningJobCompletionCriteria);
return this;
}
/**
*
* A value used to initialize a pseudo-random number generator. Setting a random seed and using the same seed later
* for the same tuning job will allow hyperparameter optimization to find more a consistent hyperparameter
* configuration between the two runs.
*
*
* @param randomSeed
* A value used to initialize a pseudo-random number generator. Setting a random seed and using the same seed
* later for the same tuning job will allow hyperparameter optimization to find more a consistent
* hyperparameter configuration between the two runs.
*/
public void setRandomSeed(Integer randomSeed) {
this.randomSeed = randomSeed;
}
/**
*
* A value used to initialize a pseudo-random number generator. Setting a random seed and using the same seed later
* for the same tuning job will allow hyperparameter optimization to find more a consistent hyperparameter
* configuration between the two runs.
*
*
* @return A value used to initialize a pseudo-random number generator. Setting a random seed and using the same
* seed later for the same tuning job will allow hyperparameter optimization to find more a consistent
* hyperparameter configuration between the two runs.
*/
public Integer getRandomSeed() {
return this.randomSeed;
}
/**
*
* A value used to initialize a pseudo-random number generator. Setting a random seed and using the same seed later
* for the same tuning job will allow hyperparameter optimization to find more a consistent hyperparameter
* configuration between the two runs.
*
*
* @param randomSeed
* A value used to initialize a pseudo-random number generator. Setting a random seed and using the same seed
* later for the same tuning job will allow hyperparameter optimization to find more a consistent
* hyperparameter configuration between the two runs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public HyperParameterTuningJobConfig withRandomSeed(Integer randomSeed) {
setRandomSeed(randomSeed);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStrategy() != null)
sb.append("Strategy: ").append(getStrategy()).append(",");
if (getStrategyConfig() != null)
sb.append("StrategyConfig: ").append(getStrategyConfig()).append(",");
if (getHyperParameterTuningJobObjective() != null)
sb.append("HyperParameterTuningJobObjective: ").append(getHyperParameterTuningJobObjective()).append(",");
if (getResourceLimits() != null)
sb.append("ResourceLimits: ").append(getResourceLimits()).append(",");
if (getParameterRanges() != null)
sb.append("ParameterRanges: ").append(getParameterRanges()).append(",");
if (getTrainingJobEarlyStoppingType() != null)
sb.append("TrainingJobEarlyStoppingType: ").append(getTrainingJobEarlyStoppingType()).append(",");
if (getTuningJobCompletionCriteria() != null)
sb.append("TuningJobCompletionCriteria: ").append(getTuningJobCompletionCriteria()).append(",");
if (getRandomSeed() != null)
sb.append("RandomSeed: ").append(getRandomSeed());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof HyperParameterTuningJobConfig == false)
return false;
HyperParameterTuningJobConfig other = (HyperParameterTuningJobConfig) obj;
if (other.getStrategy() == null ^ this.getStrategy() == null)
return false;
if (other.getStrategy() != null && other.getStrategy().equals(this.getStrategy()) == false)
return false;
if (other.getStrategyConfig() == null ^ this.getStrategyConfig() == null)
return false;
if (other.getStrategyConfig() != null && other.getStrategyConfig().equals(this.getStrategyConfig()) == false)
return false;
if (other.getHyperParameterTuningJobObjective() == null ^ this.getHyperParameterTuningJobObjective() == null)
return false;
if (other.getHyperParameterTuningJobObjective() != null
&& other.getHyperParameterTuningJobObjective().equals(this.getHyperParameterTuningJobObjective()) == false)
return false;
if (other.getResourceLimits() == null ^ this.getResourceLimits() == null)
return false;
if (other.getResourceLimits() != null && other.getResourceLimits().equals(this.getResourceLimits()) == false)
return false;
if (other.getParameterRanges() == null ^ this.getParameterRanges() == null)
return false;
if (other.getParameterRanges() != null && other.getParameterRanges().equals(this.getParameterRanges()) == false)
return false;
if (other.getTrainingJobEarlyStoppingType() == null ^ this.getTrainingJobEarlyStoppingType() == null)
return false;
if (other.getTrainingJobEarlyStoppingType() != null && other.getTrainingJobEarlyStoppingType().equals(this.getTrainingJobEarlyStoppingType()) == false)
return false;
if (other.getTuningJobCompletionCriteria() == null ^ this.getTuningJobCompletionCriteria() == null)
return false;
if (other.getTuningJobCompletionCriteria() != null && other.getTuningJobCompletionCriteria().equals(this.getTuningJobCompletionCriteria()) == false)
return false;
if (other.getRandomSeed() == null ^ this.getRandomSeed() == null)
return false;
if (other.getRandomSeed() != null && other.getRandomSeed().equals(this.getRandomSeed()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStrategy() == null) ? 0 : getStrategy().hashCode());
hashCode = prime * hashCode + ((getStrategyConfig() == null) ? 0 : getStrategyConfig().hashCode());
hashCode = prime * hashCode + ((getHyperParameterTuningJobObjective() == null) ? 0 : getHyperParameterTuningJobObjective().hashCode());
hashCode = prime * hashCode + ((getResourceLimits() == null) ? 0 : getResourceLimits().hashCode());
hashCode = prime * hashCode + ((getParameterRanges() == null) ? 0 : getParameterRanges().hashCode());
hashCode = prime * hashCode + ((getTrainingJobEarlyStoppingType() == null) ? 0 : getTrainingJobEarlyStoppingType().hashCode());
hashCode = prime * hashCode + ((getTuningJobCompletionCriteria() == null) ? 0 : getTuningJobCompletionCriteria().hashCode());
hashCode = prime * hashCode + ((getRandomSeed() == null) ? 0 : getRandomSeed().hashCode());
return hashCode;
}
@Override
public HyperParameterTuningJobConfig clone() {
try {
return (HyperParameterTuningJobConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.HyperParameterTuningJobConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}