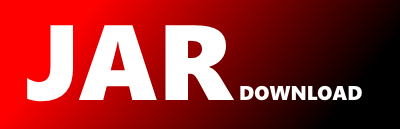
com.amazonaws.services.sagemaker.model.InferenceRecommendation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A list of recommendations made by Amazon SageMaker Inference Recommender.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class InferenceRecommendation implements Serializable, Cloneable, StructuredPojo {
/**
*
* The recommendation ID which uniquely identifies each recommendation.
*
*/
private String recommendationId;
/**
*
* The metrics used to decide what recommendation to make.
*
*/
private RecommendationMetrics metrics;
/**
*
* Defines the endpoint configuration parameters.
*
*/
private EndpointOutputConfiguration endpointConfiguration;
/**
*
* Defines the model configuration.
*
*/
private ModelConfiguration modelConfiguration;
/**
*
* A timestamp that shows when the benchmark completed.
*
*/
private java.util.Date invocationEndTime;
/**
*
* A timestamp that shows when the benchmark started.
*
*/
private java.util.Date invocationStartTime;
/**
*
* The recommendation ID which uniquely identifies each recommendation.
*
*
* @param recommendationId
* The recommendation ID which uniquely identifies each recommendation.
*/
public void setRecommendationId(String recommendationId) {
this.recommendationId = recommendationId;
}
/**
*
* The recommendation ID which uniquely identifies each recommendation.
*
*
* @return The recommendation ID which uniquely identifies each recommendation.
*/
public String getRecommendationId() {
return this.recommendationId;
}
/**
*
* The recommendation ID which uniquely identifies each recommendation.
*
*
* @param recommendationId
* The recommendation ID which uniquely identifies each recommendation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InferenceRecommendation withRecommendationId(String recommendationId) {
setRecommendationId(recommendationId);
return this;
}
/**
*
* The metrics used to decide what recommendation to make.
*
*
* @param metrics
* The metrics used to decide what recommendation to make.
*/
public void setMetrics(RecommendationMetrics metrics) {
this.metrics = metrics;
}
/**
*
* The metrics used to decide what recommendation to make.
*
*
* @return The metrics used to decide what recommendation to make.
*/
public RecommendationMetrics getMetrics() {
return this.metrics;
}
/**
*
* The metrics used to decide what recommendation to make.
*
*
* @param metrics
* The metrics used to decide what recommendation to make.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InferenceRecommendation withMetrics(RecommendationMetrics metrics) {
setMetrics(metrics);
return this;
}
/**
*
* Defines the endpoint configuration parameters.
*
*
* @param endpointConfiguration
* Defines the endpoint configuration parameters.
*/
public void setEndpointConfiguration(EndpointOutputConfiguration endpointConfiguration) {
this.endpointConfiguration = endpointConfiguration;
}
/**
*
* Defines the endpoint configuration parameters.
*
*
* @return Defines the endpoint configuration parameters.
*/
public EndpointOutputConfiguration getEndpointConfiguration() {
return this.endpointConfiguration;
}
/**
*
* Defines the endpoint configuration parameters.
*
*
* @param endpointConfiguration
* Defines the endpoint configuration parameters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InferenceRecommendation withEndpointConfiguration(EndpointOutputConfiguration endpointConfiguration) {
setEndpointConfiguration(endpointConfiguration);
return this;
}
/**
*
* Defines the model configuration.
*
*
* @param modelConfiguration
* Defines the model configuration.
*/
public void setModelConfiguration(ModelConfiguration modelConfiguration) {
this.modelConfiguration = modelConfiguration;
}
/**
*
* Defines the model configuration.
*
*
* @return Defines the model configuration.
*/
public ModelConfiguration getModelConfiguration() {
return this.modelConfiguration;
}
/**
*
* Defines the model configuration.
*
*
* @param modelConfiguration
* Defines the model configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InferenceRecommendation withModelConfiguration(ModelConfiguration modelConfiguration) {
setModelConfiguration(modelConfiguration);
return this;
}
/**
*
* A timestamp that shows when the benchmark completed.
*
*
* @param invocationEndTime
* A timestamp that shows when the benchmark completed.
*/
public void setInvocationEndTime(java.util.Date invocationEndTime) {
this.invocationEndTime = invocationEndTime;
}
/**
*
* A timestamp that shows when the benchmark completed.
*
*
* @return A timestamp that shows when the benchmark completed.
*/
public java.util.Date getInvocationEndTime() {
return this.invocationEndTime;
}
/**
*
* A timestamp that shows when the benchmark completed.
*
*
* @param invocationEndTime
* A timestamp that shows when the benchmark completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InferenceRecommendation withInvocationEndTime(java.util.Date invocationEndTime) {
setInvocationEndTime(invocationEndTime);
return this;
}
/**
*
* A timestamp that shows when the benchmark started.
*
*
* @param invocationStartTime
* A timestamp that shows when the benchmark started.
*/
public void setInvocationStartTime(java.util.Date invocationStartTime) {
this.invocationStartTime = invocationStartTime;
}
/**
*
* A timestamp that shows when the benchmark started.
*
*
* @return A timestamp that shows when the benchmark started.
*/
public java.util.Date getInvocationStartTime() {
return this.invocationStartTime;
}
/**
*
* A timestamp that shows when the benchmark started.
*
*
* @param invocationStartTime
* A timestamp that shows when the benchmark started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InferenceRecommendation withInvocationStartTime(java.util.Date invocationStartTime) {
setInvocationStartTime(invocationStartTime);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRecommendationId() != null)
sb.append("RecommendationId: ").append(getRecommendationId()).append(",");
if (getMetrics() != null)
sb.append("Metrics: ").append(getMetrics()).append(",");
if (getEndpointConfiguration() != null)
sb.append("EndpointConfiguration: ").append(getEndpointConfiguration()).append(",");
if (getModelConfiguration() != null)
sb.append("ModelConfiguration: ").append(getModelConfiguration()).append(",");
if (getInvocationEndTime() != null)
sb.append("InvocationEndTime: ").append(getInvocationEndTime()).append(",");
if (getInvocationStartTime() != null)
sb.append("InvocationStartTime: ").append(getInvocationStartTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof InferenceRecommendation == false)
return false;
InferenceRecommendation other = (InferenceRecommendation) obj;
if (other.getRecommendationId() == null ^ this.getRecommendationId() == null)
return false;
if (other.getRecommendationId() != null && other.getRecommendationId().equals(this.getRecommendationId()) == false)
return false;
if (other.getMetrics() == null ^ this.getMetrics() == null)
return false;
if (other.getMetrics() != null && other.getMetrics().equals(this.getMetrics()) == false)
return false;
if (other.getEndpointConfiguration() == null ^ this.getEndpointConfiguration() == null)
return false;
if (other.getEndpointConfiguration() != null && other.getEndpointConfiguration().equals(this.getEndpointConfiguration()) == false)
return false;
if (other.getModelConfiguration() == null ^ this.getModelConfiguration() == null)
return false;
if (other.getModelConfiguration() != null && other.getModelConfiguration().equals(this.getModelConfiguration()) == false)
return false;
if (other.getInvocationEndTime() == null ^ this.getInvocationEndTime() == null)
return false;
if (other.getInvocationEndTime() != null && other.getInvocationEndTime().equals(this.getInvocationEndTime()) == false)
return false;
if (other.getInvocationStartTime() == null ^ this.getInvocationStartTime() == null)
return false;
if (other.getInvocationStartTime() != null && other.getInvocationStartTime().equals(this.getInvocationStartTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRecommendationId() == null) ? 0 : getRecommendationId().hashCode());
hashCode = prime * hashCode + ((getMetrics() == null) ? 0 : getMetrics().hashCode());
hashCode = prime * hashCode + ((getEndpointConfiguration() == null) ? 0 : getEndpointConfiguration().hashCode());
hashCode = prime * hashCode + ((getModelConfiguration() == null) ? 0 : getModelConfiguration().hashCode());
hashCode = prime * hashCode + ((getInvocationEndTime() == null) ? 0 : getInvocationEndTime().hashCode());
hashCode = prime * hashCode + ((getInvocationStartTime() == null) ? 0 : getInvocationStartTime().hashCode());
return hashCode;
}
@Override
public InferenceRecommendation clone() {
try {
return (InferenceRecommendation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.InferenceRecommendationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}