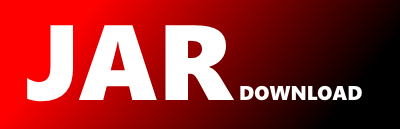
com.amazonaws.services.sagemaker.model.PendingProductionVariantSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The production variant summary for a deployment when an endpoint is creating or updating with the CreateEndpoint or UpdateEndpoint
* operations. Describes the VariantStatus
, weight and capacity for a production variant associated with
* an endpoint.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PendingProductionVariantSummary implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the variant.
*
*/
private String variantName;
/**
*
* An array of DeployedImage
objects that specify the Amazon EC2 Container Registry paths of the
* inference images deployed on instances of this ProductionVariant
.
*
*/
private java.util.List deployedImages;
/**
*
* The weight associated with the variant.
*
*/
private Float currentWeight;
/**
*
* The requested weight for the variant in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*
*/
private Float desiredWeight;
/**
*
* The number of instances associated with the variant.
*
*/
private Integer currentInstanceCount;
/**
*
* The number of instances requested in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*
*/
private Integer desiredInstanceCount;
/**
*
* The type of instances associated with the variant.
*
*/
private String instanceType;
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*/
private String acceleratorType;
/**
*
* The endpoint variant status which describes the current deployment stage status or operational status.
*
*/
private java.util.List variantStatus;
/**
*
* The serverless configuration for the endpoint.
*
*/
private ProductionVariantServerlessConfig currentServerlessConfig;
/**
*
* The serverless configuration requested for this deployment, as specified in the endpoint configuration for the
* endpoint.
*
*/
private ProductionVariantServerlessConfig desiredServerlessConfig;
/**
*
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or down
* to accommodate traffic.
*
*/
private ProductionVariantManagedInstanceScaling managedInstanceScaling;
/**
*
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*
*/
private ProductionVariantRoutingConfig routingConfig;
/**
*
* The name of the variant.
*
*
* @param variantName
* The name of the variant.
*/
public void setVariantName(String variantName) {
this.variantName = variantName;
}
/**
*
* The name of the variant.
*
*
* @return The name of the variant.
*/
public String getVariantName() {
return this.variantName;
}
/**
*
* The name of the variant.
*
*
* @param variantName
* The name of the variant.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withVariantName(String variantName) {
setVariantName(variantName);
return this;
}
/**
*
* An array of DeployedImage
objects that specify the Amazon EC2 Container Registry paths of the
* inference images deployed on instances of this ProductionVariant
.
*
*
* @return An array of DeployedImage
objects that specify the Amazon EC2 Container Registry paths of
* the inference images deployed on instances of this ProductionVariant
.
*/
public java.util.List getDeployedImages() {
return deployedImages;
}
/**
*
* An array of DeployedImage
objects that specify the Amazon EC2 Container Registry paths of the
* inference images deployed on instances of this ProductionVariant
.
*
*
* @param deployedImages
* An array of DeployedImage
objects that specify the Amazon EC2 Container Registry paths of the
* inference images deployed on instances of this ProductionVariant
.
*/
public void setDeployedImages(java.util.Collection deployedImages) {
if (deployedImages == null) {
this.deployedImages = null;
return;
}
this.deployedImages = new java.util.ArrayList(deployedImages);
}
/**
*
* An array of DeployedImage
objects that specify the Amazon EC2 Container Registry paths of the
* inference images deployed on instances of this ProductionVariant
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDeployedImages(java.util.Collection)} or {@link #withDeployedImages(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param deployedImages
* An array of DeployedImage
objects that specify the Amazon EC2 Container Registry paths of the
* inference images deployed on instances of this ProductionVariant
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withDeployedImages(DeployedImage... deployedImages) {
if (this.deployedImages == null) {
setDeployedImages(new java.util.ArrayList(deployedImages.length));
}
for (DeployedImage ele : deployedImages) {
this.deployedImages.add(ele);
}
return this;
}
/**
*
* An array of DeployedImage
objects that specify the Amazon EC2 Container Registry paths of the
* inference images deployed on instances of this ProductionVariant
.
*
*
* @param deployedImages
* An array of DeployedImage
objects that specify the Amazon EC2 Container Registry paths of the
* inference images deployed on instances of this ProductionVariant
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withDeployedImages(java.util.Collection deployedImages) {
setDeployedImages(deployedImages);
return this;
}
/**
*
* The weight associated with the variant.
*
*
* @param currentWeight
* The weight associated with the variant.
*/
public void setCurrentWeight(Float currentWeight) {
this.currentWeight = currentWeight;
}
/**
*
* The weight associated with the variant.
*
*
* @return The weight associated with the variant.
*/
public Float getCurrentWeight() {
return this.currentWeight;
}
/**
*
* The weight associated with the variant.
*
*
* @param currentWeight
* The weight associated with the variant.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withCurrentWeight(Float currentWeight) {
setCurrentWeight(currentWeight);
return this;
}
/**
*
* The requested weight for the variant in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*
*
* @param desiredWeight
* The requested weight for the variant in this deployment, as specified in the endpoint configuration for
* the endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*/
public void setDesiredWeight(Float desiredWeight) {
this.desiredWeight = desiredWeight;
}
/**
*
* The requested weight for the variant in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*
*
* @return The requested weight for the variant in this deployment, as specified in the endpoint configuration for
* the endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*/
public Float getDesiredWeight() {
return this.desiredWeight;
}
/**
*
* The requested weight for the variant in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*
*
* @param desiredWeight
* The requested weight for the variant in this deployment, as specified in the endpoint configuration for
* the endpoint. The value is taken from the request to the CreateEndpointConfig operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withDesiredWeight(Float desiredWeight) {
setDesiredWeight(desiredWeight);
return this;
}
/**
*
* The number of instances associated with the variant.
*
*
* @param currentInstanceCount
* The number of instances associated with the variant.
*/
public void setCurrentInstanceCount(Integer currentInstanceCount) {
this.currentInstanceCount = currentInstanceCount;
}
/**
*
* The number of instances associated with the variant.
*
*
* @return The number of instances associated with the variant.
*/
public Integer getCurrentInstanceCount() {
return this.currentInstanceCount;
}
/**
*
* The number of instances associated with the variant.
*
*
* @param currentInstanceCount
* The number of instances associated with the variant.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withCurrentInstanceCount(Integer currentInstanceCount) {
setCurrentInstanceCount(currentInstanceCount);
return this;
}
/**
*
* The number of instances requested in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*
*
* @param desiredInstanceCount
* The number of instances requested in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*/
public void setDesiredInstanceCount(Integer desiredInstanceCount) {
this.desiredInstanceCount = desiredInstanceCount;
}
/**
*
* The number of instances requested in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*
*
* @return The number of instances requested in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*/
public Integer getDesiredInstanceCount() {
return this.desiredInstanceCount;
}
/**
*
* The number of instances requested in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
*
*
* @param desiredInstanceCount
* The number of instances requested in this deployment, as specified in the endpoint configuration for the
* endpoint. The value is taken from the request to the CreateEndpointConfig operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withDesiredInstanceCount(Integer desiredInstanceCount) {
setDesiredInstanceCount(desiredInstanceCount);
return this;
}
/**
*
* The type of instances associated with the variant.
*
*
* @param instanceType
* The type of instances associated with the variant.
* @see ProductionVariantInstanceType
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
*
* The type of instances associated with the variant.
*
*
* @return The type of instances associated with the variant.
* @see ProductionVariantInstanceType
*/
public String getInstanceType() {
return this.instanceType;
}
/**
*
* The type of instances associated with the variant.
*
*
* @param instanceType
* The type of instances associated with the variant.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantInstanceType
*/
public PendingProductionVariantSummary withInstanceType(String instanceType) {
setInstanceType(instanceType);
return this;
}
/**
*
* The type of instances associated with the variant.
*
*
* @param instanceType
* The type of instances associated with the variant.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantInstanceType
*/
public PendingProductionVariantSummary withInstanceType(ProductionVariantInstanceType instanceType) {
this.instanceType = instanceType.toString();
return this;
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorType
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see ProductionVariantAcceleratorType
*/
public void setAcceleratorType(String acceleratorType) {
this.acceleratorType = acceleratorType;
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @return The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see ProductionVariantAcceleratorType
*/
public String getAcceleratorType() {
return this.acceleratorType;
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorType
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantAcceleratorType
*/
public PendingProductionVariantSummary withAcceleratorType(String acceleratorType) {
setAcceleratorType(acceleratorType);
return this;
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorType
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantAcceleratorType
*/
public PendingProductionVariantSummary withAcceleratorType(ProductionVariantAcceleratorType acceleratorType) {
this.acceleratorType = acceleratorType.toString();
return this;
}
/**
*
* The endpoint variant status which describes the current deployment stage status or operational status.
*
*
* @return The endpoint variant status which describes the current deployment stage status or operational status.
*/
public java.util.List getVariantStatus() {
return variantStatus;
}
/**
*
* The endpoint variant status which describes the current deployment stage status or operational status.
*
*
* @param variantStatus
* The endpoint variant status which describes the current deployment stage status or operational status.
*/
public void setVariantStatus(java.util.Collection variantStatus) {
if (variantStatus == null) {
this.variantStatus = null;
return;
}
this.variantStatus = new java.util.ArrayList(variantStatus);
}
/**
*
* The endpoint variant status which describes the current deployment stage status or operational status.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVariantStatus(java.util.Collection)} or {@link #withVariantStatus(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param variantStatus
* The endpoint variant status which describes the current deployment stage status or operational status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withVariantStatus(ProductionVariantStatus... variantStatus) {
if (this.variantStatus == null) {
setVariantStatus(new java.util.ArrayList(variantStatus.length));
}
for (ProductionVariantStatus ele : variantStatus) {
this.variantStatus.add(ele);
}
return this;
}
/**
*
* The endpoint variant status which describes the current deployment stage status or operational status.
*
*
* @param variantStatus
* The endpoint variant status which describes the current deployment stage status or operational status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withVariantStatus(java.util.Collection variantStatus) {
setVariantStatus(variantStatus);
return this;
}
/**
*
* The serverless configuration for the endpoint.
*
*
* @param currentServerlessConfig
* The serverless configuration for the endpoint.
*/
public void setCurrentServerlessConfig(ProductionVariantServerlessConfig currentServerlessConfig) {
this.currentServerlessConfig = currentServerlessConfig;
}
/**
*
* The serverless configuration for the endpoint.
*
*
* @return The serverless configuration for the endpoint.
*/
public ProductionVariantServerlessConfig getCurrentServerlessConfig() {
return this.currentServerlessConfig;
}
/**
*
* The serverless configuration for the endpoint.
*
*
* @param currentServerlessConfig
* The serverless configuration for the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withCurrentServerlessConfig(ProductionVariantServerlessConfig currentServerlessConfig) {
setCurrentServerlessConfig(currentServerlessConfig);
return this;
}
/**
*
* The serverless configuration requested for this deployment, as specified in the endpoint configuration for the
* endpoint.
*
*
* @param desiredServerlessConfig
* The serverless configuration requested for this deployment, as specified in the endpoint configuration for
* the endpoint.
*/
public void setDesiredServerlessConfig(ProductionVariantServerlessConfig desiredServerlessConfig) {
this.desiredServerlessConfig = desiredServerlessConfig;
}
/**
*
* The serverless configuration requested for this deployment, as specified in the endpoint configuration for the
* endpoint.
*
*
* @return The serverless configuration requested for this deployment, as specified in the endpoint configuration
* for the endpoint.
*/
public ProductionVariantServerlessConfig getDesiredServerlessConfig() {
return this.desiredServerlessConfig;
}
/**
*
* The serverless configuration requested for this deployment, as specified in the endpoint configuration for the
* endpoint.
*
*
* @param desiredServerlessConfig
* The serverless configuration requested for this deployment, as specified in the endpoint configuration for
* the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withDesiredServerlessConfig(ProductionVariantServerlessConfig desiredServerlessConfig) {
setDesiredServerlessConfig(desiredServerlessConfig);
return this;
}
/**
*
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or down
* to accommodate traffic.
*
*
* @param managedInstanceScaling
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or
* down to accommodate traffic.
*/
public void setManagedInstanceScaling(ProductionVariantManagedInstanceScaling managedInstanceScaling) {
this.managedInstanceScaling = managedInstanceScaling;
}
/**
*
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or down
* to accommodate traffic.
*
*
* @return Settings that control the range in the number of instances that the endpoint provisions as it scales up
* or down to accommodate traffic.
*/
public ProductionVariantManagedInstanceScaling getManagedInstanceScaling() {
return this.managedInstanceScaling;
}
/**
*
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or down
* to accommodate traffic.
*
*
* @param managedInstanceScaling
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or
* down to accommodate traffic.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withManagedInstanceScaling(ProductionVariantManagedInstanceScaling managedInstanceScaling) {
setManagedInstanceScaling(managedInstanceScaling);
return this;
}
/**
*
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*
*
* @param routingConfig
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*/
public void setRoutingConfig(ProductionVariantRoutingConfig routingConfig) {
this.routingConfig = routingConfig;
}
/**
*
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*
*
* @return Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*/
public ProductionVariantRoutingConfig getRoutingConfig() {
return this.routingConfig;
}
/**
*
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*
*
* @param routingConfig
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PendingProductionVariantSummary withRoutingConfig(ProductionVariantRoutingConfig routingConfig) {
setRoutingConfig(routingConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getVariantName() != null)
sb.append("VariantName: ").append(getVariantName()).append(",");
if (getDeployedImages() != null)
sb.append("DeployedImages: ").append(getDeployedImages()).append(",");
if (getCurrentWeight() != null)
sb.append("CurrentWeight: ").append(getCurrentWeight()).append(",");
if (getDesiredWeight() != null)
sb.append("DesiredWeight: ").append(getDesiredWeight()).append(",");
if (getCurrentInstanceCount() != null)
sb.append("CurrentInstanceCount: ").append(getCurrentInstanceCount()).append(",");
if (getDesiredInstanceCount() != null)
sb.append("DesiredInstanceCount: ").append(getDesiredInstanceCount()).append(",");
if (getInstanceType() != null)
sb.append("InstanceType: ").append(getInstanceType()).append(",");
if (getAcceleratorType() != null)
sb.append("AcceleratorType: ").append(getAcceleratorType()).append(",");
if (getVariantStatus() != null)
sb.append("VariantStatus: ").append(getVariantStatus()).append(",");
if (getCurrentServerlessConfig() != null)
sb.append("CurrentServerlessConfig: ").append(getCurrentServerlessConfig()).append(",");
if (getDesiredServerlessConfig() != null)
sb.append("DesiredServerlessConfig: ").append(getDesiredServerlessConfig()).append(",");
if (getManagedInstanceScaling() != null)
sb.append("ManagedInstanceScaling: ").append(getManagedInstanceScaling()).append(",");
if (getRoutingConfig() != null)
sb.append("RoutingConfig: ").append(getRoutingConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PendingProductionVariantSummary == false)
return false;
PendingProductionVariantSummary other = (PendingProductionVariantSummary) obj;
if (other.getVariantName() == null ^ this.getVariantName() == null)
return false;
if (other.getVariantName() != null && other.getVariantName().equals(this.getVariantName()) == false)
return false;
if (other.getDeployedImages() == null ^ this.getDeployedImages() == null)
return false;
if (other.getDeployedImages() != null && other.getDeployedImages().equals(this.getDeployedImages()) == false)
return false;
if (other.getCurrentWeight() == null ^ this.getCurrentWeight() == null)
return false;
if (other.getCurrentWeight() != null && other.getCurrentWeight().equals(this.getCurrentWeight()) == false)
return false;
if (other.getDesiredWeight() == null ^ this.getDesiredWeight() == null)
return false;
if (other.getDesiredWeight() != null && other.getDesiredWeight().equals(this.getDesiredWeight()) == false)
return false;
if (other.getCurrentInstanceCount() == null ^ this.getCurrentInstanceCount() == null)
return false;
if (other.getCurrentInstanceCount() != null && other.getCurrentInstanceCount().equals(this.getCurrentInstanceCount()) == false)
return false;
if (other.getDesiredInstanceCount() == null ^ this.getDesiredInstanceCount() == null)
return false;
if (other.getDesiredInstanceCount() != null && other.getDesiredInstanceCount().equals(this.getDesiredInstanceCount()) == false)
return false;
if (other.getInstanceType() == null ^ this.getInstanceType() == null)
return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false)
return false;
if (other.getAcceleratorType() == null ^ this.getAcceleratorType() == null)
return false;
if (other.getAcceleratorType() != null && other.getAcceleratorType().equals(this.getAcceleratorType()) == false)
return false;
if (other.getVariantStatus() == null ^ this.getVariantStatus() == null)
return false;
if (other.getVariantStatus() != null && other.getVariantStatus().equals(this.getVariantStatus()) == false)
return false;
if (other.getCurrentServerlessConfig() == null ^ this.getCurrentServerlessConfig() == null)
return false;
if (other.getCurrentServerlessConfig() != null && other.getCurrentServerlessConfig().equals(this.getCurrentServerlessConfig()) == false)
return false;
if (other.getDesiredServerlessConfig() == null ^ this.getDesiredServerlessConfig() == null)
return false;
if (other.getDesiredServerlessConfig() != null && other.getDesiredServerlessConfig().equals(this.getDesiredServerlessConfig()) == false)
return false;
if (other.getManagedInstanceScaling() == null ^ this.getManagedInstanceScaling() == null)
return false;
if (other.getManagedInstanceScaling() != null && other.getManagedInstanceScaling().equals(this.getManagedInstanceScaling()) == false)
return false;
if (other.getRoutingConfig() == null ^ this.getRoutingConfig() == null)
return false;
if (other.getRoutingConfig() != null && other.getRoutingConfig().equals(this.getRoutingConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getVariantName() == null) ? 0 : getVariantName().hashCode());
hashCode = prime * hashCode + ((getDeployedImages() == null) ? 0 : getDeployedImages().hashCode());
hashCode = prime * hashCode + ((getCurrentWeight() == null) ? 0 : getCurrentWeight().hashCode());
hashCode = prime * hashCode + ((getDesiredWeight() == null) ? 0 : getDesiredWeight().hashCode());
hashCode = prime * hashCode + ((getCurrentInstanceCount() == null) ? 0 : getCurrentInstanceCount().hashCode());
hashCode = prime * hashCode + ((getDesiredInstanceCount() == null) ? 0 : getDesiredInstanceCount().hashCode());
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getAcceleratorType() == null) ? 0 : getAcceleratorType().hashCode());
hashCode = prime * hashCode + ((getVariantStatus() == null) ? 0 : getVariantStatus().hashCode());
hashCode = prime * hashCode + ((getCurrentServerlessConfig() == null) ? 0 : getCurrentServerlessConfig().hashCode());
hashCode = prime * hashCode + ((getDesiredServerlessConfig() == null) ? 0 : getDesiredServerlessConfig().hashCode());
hashCode = prime * hashCode + ((getManagedInstanceScaling() == null) ? 0 : getManagedInstanceScaling().hashCode());
hashCode = prime * hashCode + ((getRoutingConfig() == null) ? 0 : getRoutingConfig().hashCode());
return hashCode;
}
@Override
public PendingProductionVariantSummary clone() {
try {
return (PendingProductionVariantSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.PendingProductionVariantSummaryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}