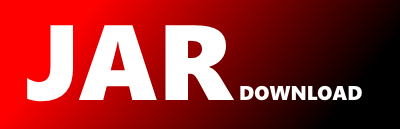
com.amazonaws.services.sagemaker.model.PipelineExecutionStep Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An execution of a step in a pipeline.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PipelineExecutionStep implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the step that is executed.
*
*/
private String stepName;
/**
*
* The display name of the step.
*
*/
private String stepDisplayName;
/**
*
* The description of the step.
*
*/
private String stepDescription;
/**
*
* The time that the step started executing.
*
*/
private java.util.Date startTime;
/**
*
* The time that the step stopped executing.
*
*/
private java.util.Date endTime;
/**
*
* The status of the step execution.
*
*/
private String stepStatus;
/**
*
* If this pipeline execution step was cached, details on the cache hit.
*
*/
private CacheHitResult cacheHitResult;
/**
*
* The reason why the step failed execution. This is only returned if the step failed its execution.
*
*/
private String failureReason;
/**
*
* Metadata to run the pipeline step.
*
*/
private PipelineExecutionStepMetadata metadata;
/**
*
* The current attempt of the execution step. For more information, see Retry Policy for SageMaker
* Pipelines steps.
*
*/
private Integer attemptCount;
/**
*
* The ARN from an execution of the current pipeline from which results are reused for this step.
*
*/
private SelectiveExecutionResult selectiveExecutionResult;
/**
*
* The name of the step that is executed.
*
*
* @param stepName
* The name of the step that is executed.
*/
public void setStepName(String stepName) {
this.stepName = stepName;
}
/**
*
* The name of the step that is executed.
*
*
* @return The name of the step that is executed.
*/
public String getStepName() {
return this.stepName;
}
/**
*
* The name of the step that is executed.
*
*
* @param stepName
* The name of the step that is executed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withStepName(String stepName) {
setStepName(stepName);
return this;
}
/**
*
* The display name of the step.
*
*
* @param stepDisplayName
* The display name of the step.
*/
public void setStepDisplayName(String stepDisplayName) {
this.stepDisplayName = stepDisplayName;
}
/**
*
* The display name of the step.
*
*
* @return The display name of the step.
*/
public String getStepDisplayName() {
return this.stepDisplayName;
}
/**
*
* The display name of the step.
*
*
* @param stepDisplayName
* The display name of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withStepDisplayName(String stepDisplayName) {
setStepDisplayName(stepDisplayName);
return this;
}
/**
*
* The description of the step.
*
*
* @param stepDescription
* The description of the step.
*/
public void setStepDescription(String stepDescription) {
this.stepDescription = stepDescription;
}
/**
*
* The description of the step.
*
*
* @return The description of the step.
*/
public String getStepDescription() {
return this.stepDescription;
}
/**
*
* The description of the step.
*
*
* @param stepDescription
* The description of the step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withStepDescription(String stepDescription) {
setStepDescription(stepDescription);
return this;
}
/**
*
* The time that the step started executing.
*
*
* @param startTime
* The time that the step started executing.
*/
public void setStartTime(java.util.Date startTime) {
this.startTime = startTime;
}
/**
*
* The time that the step started executing.
*
*
* @return The time that the step started executing.
*/
public java.util.Date getStartTime() {
return this.startTime;
}
/**
*
* The time that the step started executing.
*
*
* @param startTime
* The time that the step started executing.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withStartTime(java.util.Date startTime) {
setStartTime(startTime);
return this;
}
/**
*
* The time that the step stopped executing.
*
*
* @param endTime
* The time that the step stopped executing.
*/
public void setEndTime(java.util.Date endTime) {
this.endTime = endTime;
}
/**
*
* The time that the step stopped executing.
*
*
* @return The time that the step stopped executing.
*/
public java.util.Date getEndTime() {
return this.endTime;
}
/**
*
* The time that the step stopped executing.
*
*
* @param endTime
* The time that the step stopped executing.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withEndTime(java.util.Date endTime) {
setEndTime(endTime);
return this;
}
/**
*
* The status of the step execution.
*
*
* @param stepStatus
* The status of the step execution.
* @see StepStatus
*/
public void setStepStatus(String stepStatus) {
this.stepStatus = stepStatus;
}
/**
*
* The status of the step execution.
*
*
* @return The status of the step execution.
* @see StepStatus
*/
public String getStepStatus() {
return this.stepStatus;
}
/**
*
* The status of the step execution.
*
*
* @param stepStatus
* The status of the step execution.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StepStatus
*/
public PipelineExecutionStep withStepStatus(String stepStatus) {
setStepStatus(stepStatus);
return this;
}
/**
*
* The status of the step execution.
*
*
* @param stepStatus
* The status of the step execution.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StepStatus
*/
public PipelineExecutionStep withStepStatus(StepStatus stepStatus) {
this.stepStatus = stepStatus.toString();
return this;
}
/**
*
* If this pipeline execution step was cached, details on the cache hit.
*
*
* @param cacheHitResult
* If this pipeline execution step was cached, details on the cache hit.
*/
public void setCacheHitResult(CacheHitResult cacheHitResult) {
this.cacheHitResult = cacheHitResult;
}
/**
*
* If this pipeline execution step was cached, details on the cache hit.
*
*
* @return If this pipeline execution step was cached, details on the cache hit.
*/
public CacheHitResult getCacheHitResult() {
return this.cacheHitResult;
}
/**
*
* If this pipeline execution step was cached, details on the cache hit.
*
*
* @param cacheHitResult
* If this pipeline execution step was cached, details on the cache hit.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withCacheHitResult(CacheHitResult cacheHitResult) {
setCacheHitResult(cacheHitResult);
return this;
}
/**
*
* The reason why the step failed execution. This is only returned if the step failed its execution.
*
*
* @param failureReason
* The reason why the step failed execution. This is only returned if the step failed its execution.
*/
public void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
/**
*
* The reason why the step failed execution. This is only returned if the step failed its execution.
*
*
* @return The reason why the step failed execution. This is only returned if the step failed its execution.
*/
public String getFailureReason() {
return this.failureReason;
}
/**
*
* The reason why the step failed execution. This is only returned if the step failed its execution.
*
*
* @param failureReason
* The reason why the step failed execution. This is only returned if the step failed its execution.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withFailureReason(String failureReason) {
setFailureReason(failureReason);
return this;
}
/**
*
* Metadata to run the pipeline step.
*
*
* @param metadata
* Metadata to run the pipeline step.
*/
public void setMetadata(PipelineExecutionStepMetadata metadata) {
this.metadata = metadata;
}
/**
*
* Metadata to run the pipeline step.
*
*
* @return Metadata to run the pipeline step.
*/
public PipelineExecutionStepMetadata getMetadata() {
return this.metadata;
}
/**
*
* Metadata to run the pipeline step.
*
*
* @param metadata
* Metadata to run the pipeline step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withMetadata(PipelineExecutionStepMetadata metadata) {
setMetadata(metadata);
return this;
}
/**
*
* The current attempt of the execution step. For more information, see Retry Policy for SageMaker
* Pipelines steps.
*
*
* @param attemptCount
* The current attempt of the execution step. For more information, see Retry Policy for
* SageMaker Pipelines steps.
*/
public void setAttemptCount(Integer attemptCount) {
this.attemptCount = attemptCount;
}
/**
*
* The current attempt of the execution step. For more information, see Retry Policy for SageMaker
* Pipelines steps.
*
*
* @return The current attempt of the execution step. For more information, see Retry Policy for
* SageMaker Pipelines steps.
*/
public Integer getAttemptCount() {
return this.attemptCount;
}
/**
*
* The current attempt of the execution step. For more information, see Retry Policy for SageMaker
* Pipelines steps.
*
*
* @param attemptCount
* The current attempt of the execution step. For more information, see Retry Policy for
* SageMaker Pipelines steps.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withAttemptCount(Integer attemptCount) {
setAttemptCount(attemptCount);
return this;
}
/**
*
* The ARN from an execution of the current pipeline from which results are reused for this step.
*
*
* @param selectiveExecutionResult
* The ARN from an execution of the current pipeline from which results are reused for this step.
*/
public void setSelectiveExecutionResult(SelectiveExecutionResult selectiveExecutionResult) {
this.selectiveExecutionResult = selectiveExecutionResult;
}
/**
*
* The ARN from an execution of the current pipeline from which results are reused for this step.
*
*
* @return The ARN from an execution of the current pipeline from which results are reused for this step.
*/
public SelectiveExecutionResult getSelectiveExecutionResult() {
return this.selectiveExecutionResult;
}
/**
*
* The ARN from an execution of the current pipeline from which results are reused for this step.
*
*
* @param selectiveExecutionResult
* The ARN from an execution of the current pipeline from which results are reused for this step.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PipelineExecutionStep withSelectiveExecutionResult(SelectiveExecutionResult selectiveExecutionResult) {
setSelectiveExecutionResult(selectiveExecutionResult);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStepName() != null)
sb.append("StepName: ").append(getStepName()).append(",");
if (getStepDisplayName() != null)
sb.append("StepDisplayName: ").append(getStepDisplayName()).append(",");
if (getStepDescription() != null)
sb.append("StepDescription: ").append(getStepDescription()).append(",");
if (getStartTime() != null)
sb.append("StartTime: ").append(getStartTime()).append(",");
if (getEndTime() != null)
sb.append("EndTime: ").append(getEndTime()).append(",");
if (getStepStatus() != null)
sb.append("StepStatus: ").append(getStepStatus()).append(",");
if (getCacheHitResult() != null)
sb.append("CacheHitResult: ").append(getCacheHitResult()).append(",");
if (getFailureReason() != null)
sb.append("FailureReason: ").append(getFailureReason()).append(",");
if (getMetadata() != null)
sb.append("Metadata: ").append(getMetadata()).append(",");
if (getAttemptCount() != null)
sb.append("AttemptCount: ").append(getAttemptCount()).append(",");
if (getSelectiveExecutionResult() != null)
sb.append("SelectiveExecutionResult: ").append(getSelectiveExecutionResult());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PipelineExecutionStep == false)
return false;
PipelineExecutionStep other = (PipelineExecutionStep) obj;
if (other.getStepName() == null ^ this.getStepName() == null)
return false;
if (other.getStepName() != null && other.getStepName().equals(this.getStepName()) == false)
return false;
if (other.getStepDisplayName() == null ^ this.getStepDisplayName() == null)
return false;
if (other.getStepDisplayName() != null && other.getStepDisplayName().equals(this.getStepDisplayName()) == false)
return false;
if (other.getStepDescription() == null ^ this.getStepDescription() == null)
return false;
if (other.getStepDescription() != null && other.getStepDescription().equals(this.getStepDescription()) == false)
return false;
if (other.getStartTime() == null ^ this.getStartTime() == null)
return false;
if (other.getStartTime() != null && other.getStartTime().equals(this.getStartTime()) == false)
return false;
if (other.getEndTime() == null ^ this.getEndTime() == null)
return false;
if (other.getEndTime() != null && other.getEndTime().equals(this.getEndTime()) == false)
return false;
if (other.getStepStatus() == null ^ this.getStepStatus() == null)
return false;
if (other.getStepStatus() != null && other.getStepStatus().equals(this.getStepStatus()) == false)
return false;
if (other.getCacheHitResult() == null ^ this.getCacheHitResult() == null)
return false;
if (other.getCacheHitResult() != null && other.getCacheHitResult().equals(this.getCacheHitResult()) == false)
return false;
if (other.getFailureReason() == null ^ this.getFailureReason() == null)
return false;
if (other.getFailureReason() != null && other.getFailureReason().equals(this.getFailureReason()) == false)
return false;
if (other.getMetadata() == null ^ this.getMetadata() == null)
return false;
if (other.getMetadata() != null && other.getMetadata().equals(this.getMetadata()) == false)
return false;
if (other.getAttemptCount() == null ^ this.getAttemptCount() == null)
return false;
if (other.getAttemptCount() != null && other.getAttemptCount().equals(this.getAttemptCount()) == false)
return false;
if (other.getSelectiveExecutionResult() == null ^ this.getSelectiveExecutionResult() == null)
return false;
if (other.getSelectiveExecutionResult() != null && other.getSelectiveExecutionResult().equals(this.getSelectiveExecutionResult()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStepName() == null) ? 0 : getStepName().hashCode());
hashCode = prime * hashCode + ((getStepDisplayName() == null) ? 0 : getStepDisplayName().hashCode());
hashCode = prime * hashCode + ((getStepDescription() == null) ? 0 : getStepDescription().hashCode());
hashCode = prime * hashCode + ((getStartTime() == null) ? 0 : getStartTime().hashCode());
hashCode = prime * hashCode + ((getEndTime() == null) ? 0 : getEndTime().hashCode());
hashCode = prime * hashCode + ((getStepStatus() == null) ? 0 : getStepStatus().hashCode());
hashCode = prime * hashCode + ((getCacheHitResult() == null) ? 0 : getCacheHitResult().hashCode());
hashCode = prime * hashCode + ((getFailureReason() == null) ? 0 : getFailureReason().hashCode());
hashCode = prime * hashCode + ((getMetadata() == null) ? 0 : getMetadata().hashCode());
hashCode = prime * hashCode + ((getAttemptCount() == null) ? 0 : getAttemptCount().hashCode());
hashCode = prime * hashCode + ((getSelectiveExecutionResult() == null) ? 0 : getSelectiveExecutionResult().hashCode());
return hashCode;
}
@Override
public PipelineExecutionStep clone() {
try {
return (PipelineExecutionStep) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.PipelineExecutionStepMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}