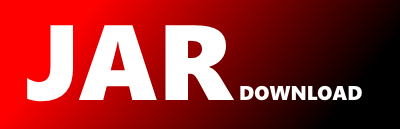
com.amazonaws.services.sagemaker.model.ProcessingOutput Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes the results of a processing job. The processing output must specify exactly one of either
* S3Output
or FeatureStoreOutput
types.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ProcessingOutput implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name for the processing job output.
*
*/
private String outputName;
/**
*
* Configuration for processing job outputs in Amazon S3.
*
*/
private ProcessingS3Output s3Output;
/**
*
* Configuration for processing job outputs in Amazon SageMaker Feature Store. This processing output type is only
* supported when AppManaged
is specified.
*
*/
private ProcessingFeatureStoreOutput featureStoreOutput;
/**
*
* When True
, output operations such as data upload are managed natively by the processing job
* application. When False
(default), output operations are managed by Amazon SageMaker.
*
*/
private Boolean appManaged;
/**
*
* The name for the processing job output.
*
*
* @param outputName
* The name for the processing job output.
*/
public void setOutputName(String outputName) {
this.outputName = outputName;
}
/**
*
* The name for the processing job output.
*
*
* @return The name for the processing job output.
*/
public String getOutputName() {
return this.outputName;
}
/**
*
* The name for the processing job output.
*
*
* @param outputName
* The name for the processing job output.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProcessingOutput withOutputName(String outputName) {
setOutputName(outputName);
return this;
}
/**
*
* Configuration for processing job outputs in Amazon S3.
*
*
* @param s3Output
* Configuration for processing job outputs in Amazon S3.
*/
public void setS3Output(ProcessingS3Output s3Output) {
this.s3Output = s3Output;
}
/**
*
* Configuration for processing job outputs in Amazon S3.
*
*
* @return Configuration for processing job outputs in Amazon S3.
*/
public ProcessingS3Output getS3Output() {
return this.s3Output;
}
/**
*
* Configuration for processing job outputs in Amazon S3.
*
*
* @param s3Output
* Configuration for processing job outputs in Amazon S3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProcessingOutput withS3Output(ProcessingS3Output s3Output) {
setS3Output(s3Output);
return this;
}
/**
*
* Configuration for processing job outputs in Amazon SageMaker Feature Store. This processing output type is only
* supported when AppManaged
is specified.
*
*
* @param featureStoreOutput
* Configuration for processing job outputs in Amazon SageMaker Feature Store. This processing output type is
* only supported when AppManaged
is specified.
*/
public void setFeatureStoreOutput(ProcessingFeatureStoreOutput featureStoreOutput) {
this.featureStoreOutput = featureStoreOutput;
}
/**
*
* Configuration for processing job outputs in Amazon SageMaker Feature Store. This processing output type is only
* supported when AppManaged
is specified.
*
*
* @return Configuration for processing job outputs in Amazon SageMaker Feature Store. This processing output type
* is only supported when AppManaged
is specified.
*/
public ProcessingFeatureStoreOutput getFeatureStoreOutput() {
return this.featureStoreOutput;
}
/**
*
* Configuration for processing job outputs in Amazon SageMaker Feature Store. This processing output type is only
* supported when AppManaged
is specified.
*
*
* @param featureStoreOutput
* Configuration for processing job outputs in Amazon SageMaker Feature Store. This processing output type is
* only supported when AppManaged
is specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProcessingOutput withFeatureStoreOutput(ProcessingFeatureStoreOutput featureStoreOutput) {
setFeatureStoreOutput(featureStoreOutput);
return this;
}
/**
*
* When True
, output operations such as data upload are managed natively by the processing job
* application. When False
(default), output operations are managed by Amazon SageMaker.
*
*
* @param appManaged
* When True
, output operations such as data upload are managed natively by the processing job
* application. When False
(default), output operations are managed by Amazon SageMaker.
*/
public void setAppManaged(Boolean appManaged) {
this.appManaged = appManaged;
}
/**
*
* When True
, output operations such as data upload are managed natively by the processing job
* application. When False
(default), output operations are managed by Amazon SageMaker.
*
*
* @return When True
, output operations such as data upload are managed natively by the processing job
* application. When False
(default), output operations are managed by Amazon SageMaker.
*/
public Boolean getAppManaged() {
return this.appManaged;
}
/**
*
* When True
, output operations such as data upload are managed natively by the processing job
* application. When False
(default), output operations are managed by Amazon SageMaker.
*
*
* @param appManaged
* When True
, output operations such as data upload are managed natively by the processing job
* application. When False
(default), output operations are managed by Amazon SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProcessingOutput withAppManaged(Boolean appManaged) {
setAppManaged(appManaged);
return this;
}
/**
*
* When True
, output operations such as data upload are managed natively by the processing job
* application. When False
(default), output operations are managed by Amazon SageMaker.
*
*
* @return When True
, output operations such as data upload are managed natively by the processing job
* application. When False
(default), output operations are managed by Amazon SageMaker.
*/
public Boolean isAppManaged() {
return this.appManaged;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOutputName() != null)
sb.append("OutputName: ").append(getOutputName()).append(",");
if (getS3Output() != null)
sb.append("S3Output: ").append(getS3Output()).append(",");
if (getFeatureStoreOutput() != null)
sb.append("FeatureStoreOutput: ").append(getFeatureStoreOutput()).append(",");
if (getAppManaged() != null)
sb.append("AppManaged: ").append(getAppManaged());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ProcessingOutput == false)
return false;
ProcessingOutput other = (ProcessingOutput) obj;
if (other.getOutputName() == null ^ this.getOutputName() == null)
return false;
if (other.getOutputName() != null && other.getOutputName().equals(this.getOutputName()) == false)
return false;
if (other.getS3Output() == null ^ this.getS3Output() == null)
return false;
if (other.getS3Output() != null && other.getS3Output().equals(this.getS3Output()) == false)
return false;
if (other.getFeatureStoreOutput() == null ^ this.getFeatureStoreOutput() == null)
return false;
if (other.getFeatureStoreOutput() != null && other.getFeatureStoreOutput().equals(this.getFeatureStoreOutput()) == false)
return false;
if (other.getAppManaged() == null ^ this.getAppManaged() == null)
return false;
if (other.getAppManaged() != null && other.getAppManaged().equals(this.getAppManaged()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOutputName() == null) ? 0 : getOutputName().hashCode());
hashCode = prime * hashCode + ((getS3Output() == null) ? 0 : getS3Output().hashCode());
hashCode = prime * hashCode + ((getFeatureStoreOutput() == null) ? 0 : getFeatureStoreOutput().hashCode());
hashCode = prime * hashCode + ((getAppManaged() == null) ? 0 : getAppManaged().hashCode());
return hashCode;
}
@Override
public ProcessingOutput clone() {
try {
return (ProcessingOutput) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.ProcessingOutputMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}