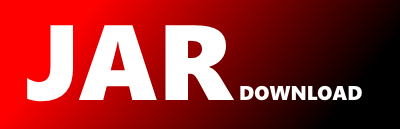
com.amazonaws.services.sagemaker.model.ProductionVariant Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Identifies a model that you want to host and the resources chosen to deploy for hosting it. If you are deploying
* multiple models, tell SageMaker how to distribute traffic among the models by specifying variant weights. For more
* information on production variants, check Production variants.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ProductionVariant implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the production variant.
*
*/
private String variantName;
/**
*
* The name of the model that you want to host. This is the name that you specified when creating the model.
*
*/
private String modelName;
/**
*
* Number of instances to launch initially.
*
*/
private Integer initialInstanceCount;
/**
*
* The ML compute instance type.
*
*/
private String instanceType;
/**
*
* Determines initial traffic distribution among all of the models that you specify in the endpoint configuration.
* The traffic to a production variant is determined by the ratio of the VariantWeight
to the sum of
* all VariantWeight
values across all ProductionVariants. If unspecified, it defaults to 1.0.
*
*/
private Float initialVariantWeight;
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*/
private String acceleratorType;
/**
*
* Specifies configuration for a core dump from the model container when the process crashes.
*
*/
private ProductionVariantCoreDumpConfig coreDumpConfig;
/**
*
* The serverless configuration for an endpoint. Specifies a serverless endpoint configuration instead of an
* instance-based endpoint configuration.
*
*/
private ProductionVariantServerlessConfig serverlessConfig;
/**
*
* The size, in GB, of the ML storage volume attached to individual inference instance associated with the
* production variant. Currently only Amazon EBS gp2 storage volumes are supported.
*
*/
private Integer volumeSizeInGB;
/**
*
* The timeout value, in seconds, to download and extract the model that you want to host from Amazon S3 to the
* individual inference instance associated with this production variant.
*
*/
private Integer modelDataDownloadTimeoutInSeconds;
/**
*
* The timeout value, in seconds, for your inference container to pass health check by SageMaker Hosting. For more
* information about health check, see How Your Container Should Respond to Health Check (Ping) Requests.
*
*/
private Integer containerStartupHealthCheckTimeoutInSeconds;
/**
*
* You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a production
* variant behind an endpoint. By default, SSM access is disabled for all production variants behind an endpoint.
* You can turn on or turn off SSM access for a production variant behind an existing endpoint by creating a new
* endpoint configuration and calling UpdateEndpoint
.
*
*/
private Boolean enableSSMAccess;
/**
*
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or down
* to accommodate traffic.
*
*/
private ProductionVariantManagedInstanceScaling managedInstanceScaling;
/**
*
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*
*/
private ProductionVariantRoutingConfig routingConfig;
/**
*
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services optimizes these
* configurations for different machine learning workloads.
*
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific software
* requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron driver versions.
*
*/
private String inferenceAmiVersion;
/**
*
* The name of the production variant.
*
*
* @param variantName
* The name of the production variant.
*/
public void setVariantName(String variantName) {
this.variantName = variantName;
}
/**
*
* The name of the production variant.
*
*
* @return The name of the production variant.
*/
public String getVariantName() {
return this.variantName;
}
/**
*
* The name of the production variant.
*
*
* @param variantName
* The name of the production variant.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withVariantName(String variantName) {
setVariantName(variantName);
return this;
}
/**
*
* The name of the model that you want to host. This is the name that you specified when creating the model.
*
*
* @param modelName
* The name of the model that you want to host. This is the name that you specified when creating the model.
*/
public void setModelName(String modelName) {
this.modelName = modelName;
}
/**
*
* The name of the model that you want to host. This is the name that you specified when creating the model.
*
*
* @return The name of the model that you want to host. This is the name that you specified when creating the model.
*/
public String getModelName() {
return this.modelName;
}
/**
*
* The name of the model that you want to host. This is the name that you specified when creating the model.
*
*
* @param modelName
* The name of the model that you want to host. This is the name that you specified when creating the model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withModelName(String modelName) {
setModelName(modelName);
return this;
}
/**
*
* Number of instances to launch initially.
*
*
* @param initialInstanceCount
* Number of instances to launch initially.
*/
public void setInitialInstanceCount(Integer initialInstanceCount) {
this.initialInstanceCount = initialInstanceCount;
}
/**
*
* Number of instances to launch initially.
*
*
* @return Number of instances to launch initially.
*/
public Integer getInitialInstanceCount() {
return this.initialInstanceCount;
}
/**
*
* Number of instances to launch initially.
*
*
* @param initialInstanceCount
* Number of instances to launch initially.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withInitialInstanceCount(Integer initialInstanceCount) {
setInitialInstanceCount(initialInstanceCount);
return this;
}
/**
*
* The ML compute instance type.
*
*
* @param instanceType
* The ML compute instance type.
* @see ProductionVariantInstanceType
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
*
* The ML compute instance type.
*
*
* @return The ML compute instance type.
* @see ProductionVariantInstanceType
*/
public String getInstanceType() {
return this.instanceType;
}
/**
*
* The ML compute instance type.
*
*
* @param instanceType
* The ML compute instance type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantInstanceType
*/
public ProductionVariant withInstanceType(String instanceType) {
setInstanceType(instanceType);
return this;
}
/**
*
* The ML compute instance type.
*
*
* @param instanceType
* The ML compute instance type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantInstanceType
*/
public ProductionVariant withInstanceType(ProductionVariantInstanceType instanceType) {
this.instanceType = instanceType.toString();
return this;
}
/**
*
* Determines initial traffic distribution among all of the models that you specify in the endpoint configuration.
* The traffic to a production variant is determined by the ratio of the VariantWeight
to the sum of
* all VariantWeight
values across all ProductionVariants. If unspecified, it defaults to 1.0.
*
*
* @param initialVariantWeight
* Determines initial traffic distribution among all of the models that you specify in the endpoint
* configuration. The traffic to a production variant is determined by the ratio of the
* VariantWeight
to the sum of all VariantWeight
values across all
* ProductionVariants. If unspecified, it defaults to 1.0.
*/
public void setInitialVariantWeight(Float initialVariantWeight) {
this.initialVariantWeight = initialVariantWeight;
}
/**
*
* Determines initial traffic distribution among all of the models that you specify in the endpoint configuration.
* The traffic to a production variant is determined by the ratio of the VariantWeight
to the sum of
* all VariantWeight
values across all ProductionVariants. If unspecified, it defaults to 1.0.
*
*
* @return Determines initial traffic distribution among all of the models that you specify in the endpoint
* configuration. The traffic to a production variant is determined by the ratio of the
* VariantWeight
to the sum of all VariantWeight
values across all
* ProductionVariants. If unspecified, it defaults to 1.0.
*/
public Float getInitialVariantWeight() {
return this.initialVariantWeight;
}
/**
*
* Determines initial traffic distribution among all of the models that you specify in the endpoint configuration.
* The traffic to a production variant is determined by the ratio of the VariantWeight
to the sum of
* all VariantWeight
values across all ProductionVariants. If unspecified, it defaults to 1.0.
*
*
* @param initialVariantWeight
* Determines initial traffic distribution among all of the models that you specify in the endpoint
* configuration. The traffic to a production variant is determined by the ratio of the
* VariantWeight
to the sum of all VariantWeight
values across all
* ProductionVariants. If unspecified, it defaults to 1.0.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withInitialVariantWeight(Float initialVariantWeight) {
setInitialVariantWeight(initialVariantWeight);
return this;
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorType
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see ProductionVariantAcceleratorType
*/
public void setAcceleratorType(String acceleratorType) {
this.acceleratorType = acceleratorType;
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @return The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see ProductionVariantAcceleratorType
*/
public String getAcceleratorType() {
return this.acceleratorType;
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorType
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantAcceleratorType
*/
public ProductionVariant withAcceleratorType(String acceleratorType) {
setAcceleratorType(acceleratorType);
return this;
}
/**
*
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide on-demand
* GPU computing for inference. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorType
* The size of the Elastic Inference (EI) instance to use for the production variant. EI instances provide
* on-demand GPU computing for inference. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantAcceleratorType
*/
public ProductionVariant withAcceleratorType(ProductionVariantAcceleratorType acceleratorType) {
this.acceleratorType = acceleratorType.toString();
return this;
}
/**
*
* Specifies configuration for a core dump from the model container when the process crashes.
*
*
* @param coreDumpConfig
* Specifies configuration for a core dump from the model container when the process crashes.
*/
public void setCoreDumpConfig(ProductionVariantCoreDumpConfig coreDumpConfig) {
this.coreDumpConfig = coreDumpConfig;
}
/**
*
* Specifies configuration for a core dump from the model container when the process crashes.
*
*
* @return Specifies configuration for a core dump from the model container when the process crashes.
*/
public ProductionVariantCoreDumpConfig getCoreDumpConfig() {
return this.coreDumpConfig;
}
/**
*
* Specifies configuration for a core dump from the model container when the process crashes.
*
*
* @param coreDumpConfig
* Specifies configuration for a core dump from the model container when the process crashes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withCoreDumpConfig(ProductionVariantCoreDumpConfig coreDumpConfig) {
setCoreDumpConfig(coreDumpConfig);
return this;
}
/**
*
* The serverless configuration for an endpoint. Specifies a serverless endpoint configuration instead of an
* instance-based endpoint configuration.
*
*
* @param serverlessConfig
* The serverless configuration for an endpoint. Specifies a serverless endpoint configuration instead of an
* instance-based endpoint configuration.
*/
public void setServerlessConfig(ProductionVariantServerlessConfig serverlessConfig) {
this.serverlessConfig = serverlessConfig;
}
/**
*
* The serverless configuration for an endpoint. Specifies a serverless endpoint configuration instead of an
* instance-based endpoint configuration.
*
*
* @return The serverless configuration for an endpoint. Specifies a serverless endpoint configuration instead of an
* instance-based endpoint configuration.
*/
public ProductionVariantServerlessConfig getServerlessConfig() {
return this.serverlessConfig;
}
/**
*
* The serverless configuration for an endpoint. Specifies a serverless endpoint configuration instead of an
* instance-based endpoint configuration.
*
*
* @param serverlessConfig
* The serverless configuration for an endpoint. Specifies a serverless endpoint configuration instead of an
* instance-based endpoint configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withServerlessConfig(ProductionVariantServerlessConfig serverlessConfig) {
setServerlessConfig(serverlessConfig);
return this;
}
/**
*
* The size, in GB, of the ML storage volume attached to individual inference instance associated with the
* production variant. Currently only Amazon EBS gp2 storage volumes are supported.
*
*
* @param volumeSizeInGB
* The size, in GB, of the ML storage volume attached to individual inference instance associated with the
* production variant. Currently only Amazon EBS gp2 storage volumes are supported.
*/
public void setVolumeSizeInGB(Integer volumeSizeInGB) {
this.volumeSizeInGB = volumeSizeInGB;
}
/**
*
* The size, in GB, of the ML storage volume attached to individual inference instance associated with the
* production variant. Currently only Amazon EBS gp2 storage volumes are supported.
*
*
* @return The size, in GB, of the ML storage volume attached to individual inference instance associated with the
* production variant. Currently only Amazon EBS gp2 storage volumes are supported.
*/
public Integer getVolumeSizeInGB() {
return this.volumeSizeInGB;
}
/**
*
* The size, in GB, of the ML storage volume attached to individual inference instance associated with the
* production variant. Currently only Amazon EBS gp2 storage volumes are supported.
*
*
* @param volumeSizeInGB
* The size, in GB, of the ML storage volume attached to individual inference instance associated with the
* production variant. Currently only Amazon EBS gp2 storage volumes are supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withVolumeSizeInGB(Integer volumeSizeInGB) {
setVolumeSizeInGB(volumeSizeInGB);
return this;
}
/**
*
* The timeout value, in seconds, to download and extract the model that you want to host from Amazon S3 to the
* individual inference instance associated with this production variant.
*
*
* @param modelDataDownloadTimeoutInSeconds
* The timeout value, in seconds, to download and extract the model that you want to host from Amazon S3 to
* the individual inference instance associated with this production variant.
*/
public void setModelDataDownloadTimeoutInSeconds(Integer modelDataDownloadTimeoutInSeconds) {
this.modelDataDownloadTimeoutInSeconds = modelDataDownloadTimeoutInSeconds;
}
/**
*
* The timeout value, in seconds, to download and extract the model that you want to host from Amazon S3 to the
* individual inference instance associated with this production variant.
*
*
* @return The timeout value, in seconds, to download and extract the model that you want to host from Amazon S3 to
* the individual inference instance associated with this production variant.
*/
public Integer getModelDataDownloadTimeoutInSeconds() {
return this.modelDataDownloadTimeoutInSeconds;
}
/**
*
* The timeout value, in seconds, to download and extract the model that you want to host from Amazon S3 to the
* individual inference instance associated with this production variant.
*
*
* @param modelDataDownloadTimeoutInSeconds
* The timeout value, in seconds, to download and extract the model that you want to host from Amazon S3 to
* the individual inference instance associated with this production variant.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withModelDataDownloadTimeoutInSeconds(Integer modelDataDownloadTimeoutInSeconds) {
setModelDataDownloadTimeoutInSeconds(modelDataDownloadTimeoutInSeconds);
return this;
}
/**
*
* The timeout value, in seconds, for your inference container to pass health check by SageMaker Hosting. For more
* information about health check, see How Your Container Should Respond to Health Check (Ping) Requests.
*
*
* @param containerStartupHealthCheckTimeoutInSeconds
* The timeout value, in seconds, for your inference container to pass health check by SageMaker Hosting. For
* more information about health check, see How Your Container Should Respond to Health Check (Ping) Requests.
*/
public void setContainerStartupHealthCheckTimeoutInSeconds(Integer containerStartupHealthCheckTimeoutInSeconds) {
this.containerStartupHealthCheckTimeoutInSeconds = containerStartupHealthCheckTimeoutInSeconds;
}
/**
*
* The timeout value, in seconds, for your inference container to pass health check by SageMaker Hosting. For more
* information about health check, see How Your Container Should Respond to Health Check (Ping) Requests.
*
*
* @return The timeout value, in seconds, for your inference container to pass health check by SageMaker Hosting.
* For more information about health check, see How Your Container Should Respond to Health Check (Ping) Requests.
*/
public Integer getContainerStartupHealthCheckTimeoutInSeconds() {
return this.containerStartupHealthCheckTimeoutInSeconds;
}
/**
*
* The timeout value, in seconds, for your inference container to pass health check by SageMaker Hosting. For more
* information about health check, see How Your Container Should Respond to Health Check (Ping) Requests.
*
*
* @param containerStartupHealthCheckTimeoutInSeconds
* The timeout value, in seconds, for your inference container to pass health check by SageMaker Hosting. For
* more information about health check, see How Your Container Should Respond to Health Check (Ping) Requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withContainerStartupHealthCheckTimeoutInSeconds(Integer containerStartupHealthCheckTimeoutInSeconds) {
setContainerStartupHealthCheckTimeoutInSeconds(containerStartupHealthCheckTimeoutInSeconds);
return this;
}
/**
*
* You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a production
* variant behind an endpoint. By default, SSM access is disabled for all production variants behind an endpoint.
* You can turn on or turn off SSM access for a production variant behind an existing endpoint by creating a new
* endpoint configuration and calling UpdateEndpoint
.
*
*
* @param enableSSMAccess
* You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a
* production variant behind an endpoint. By default, SSM access is disabled for all production variants
* behind an endpoint. You can turn on or turn off SSM access for a production variant behind an existing
* endpoint by creating a new endpoint configuration and calling UpdateEndpoint
.
*/
public void setEnableSSMAccess(Boolean enableSSMAccess) {
this.enableSSMAccess = enableSSMAccess;
}
/**
*
* You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a production
* variant behind an endpoint. By default, SSM access is disabled for all production variants behind an endpoint.
* You can turn on or turn off SSM access for a production variant behind an existing endpoint by creating a new
* endpoint configuration and calling UpdateEndpoint
.
*
*
* @return You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a
* production variant behind an endpoint. By default, SSM access is disabled for all production variants
* behind an endpoint. You can turn on or turn off SSM access for a production variant behind an existing
* endpoint by creating a new endpoint configuration and calling UpdateEndpoint
.
*/
public Boolean getEnableSSMAccess() {
return this.enableSSMAccess;
}
/**
*
* You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a production
* variant behind an endpoint. By default, SSM access is disabled for all production variants behind an endpoint.
* You can turn on or turn off SSM access for a production variant behind an existing endpoint by creating a new
* endpoint configuration and calling UpdateEndpoint
.
*
*
* @param enableSSMAccess
* You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a
* production variant behind an endpoint. By default, SSM access is disabled for all production variants
* behind an endpoint. You can turn on or turn off SSM access for a production variant behind an existing
* endpoint by creating a new endpoint configuration and calling UpdateEndpoint
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withEnableSSMAccess(Boolean enableSSMAccess) {
setEnableSSMAccess(enableSSMAccess);
return this;
}
/**
*
* You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a production
* variant behind an endpoint. By default, SSM access is disabled for all production variants behind an endpoint.
* You can turn on or turn off SSM access for a production variant behind an existing endpoint by creating a new
* endpoint configuration and calling UpdateEndpoint
.
*
*
* @return You can use this parameter to turn on native Amazon Web Services Systems Manager (SSM) access for a
* production variant behind an endpoint. By default, SSM access is disabled for all production variants
* behind an endpoint. You can turn on or turn off SSM access for a production variant behind an existing
* endpoint by creating a new endpoint configuration and calling UpdateEndpoint
.
*/
public Boolean isEnableSSMAccess() {
return this.enableSSMAccess;
}
/**
*
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or down
* to accommodate traffic.
*
*
* @param managedInstanceScaling
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or
* down to accommodate traffic.
*/
public void setManagedInstanceScaling(ProductionVariantManagedInstanceScaling managedInstanceScaling) {
this.managedInstanceScaling = managedInstanceScaling;
}
/**
*
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or down
* to accommodate traffic.
*
*
* @return Settings that control the range in the number of instances that the endpoint provisions as it scales up
* or down to accommodate traffic.
*/
public ProductionVariantManagedInstanceScaling getManagedInstanceScaling() {
return this.managedInstanceScaling;
}
/**
*
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or down
* to accommodate traffic.
*
*
* @param managedInstanceScaling
* Settings that control the range in the number of instances that the endpoint provisions as it scales up or
* down to accommodate traffic.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withManagedInstanceScaling(ProductionVariantManagedInstanceScaling managedInstanceScaling) {
setManagedInstanceScaling(managedInstanceScaling);
return this;
}
/**
*
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*
*
* @param routingConfig
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*/
public void setRoutingConfig(ProductionVariantRoutingConfig routingConfig) {
this.routingConfig = routingConfig;
}
/**
*
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*
*
* @return Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*/
public ProductionVariantRoutingConfig getRoutingConfig() {
return this.routingConfig;
}
/**
*
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
*
*
* @param routingConfig
* Settings that control how the endpoint routes incoming traffic to the instances that the endpoint hosts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ProductionVariant withRoutingConfig(ProductionVariantRoutingConfig routingConfig) {
setRoutingConfig(routingConfig);
return this;
}
/**
*
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services optimizes these
* configurations for different machine learning workloads.
*
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific software
* requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron driver versions.
*
*
* @param inferenceAmiVersion
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services
* optimizes these configurations for different machine learning workloads.
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific
* software requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron
* driver versions.
* @see ProductionVariantInferenceAmiVersion
*/
public void setInferenceAmiVersion(String inferenceAmiVersion) {
this.inferenceAmiVersion = inferenceAmiVersion;
}
/**
*
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services optimizes these
* configurations for different machine learning workloads.
*
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific software
* requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron driver versions.
*
*
* @return Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services
* optimizes these configurations for different machine learning workloads.
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific
* software requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron
* driver versions.
* @see ProductionVariantInferenceAmiVersion
*/
public String getInferenceAmiVersion() {
return this.inferenceAmiVersion;
}
/**
*
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services optimizes these
* configurations for different machine learning workloads.
*
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific software
* requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron driver versions.
*
*
* @param inferenceAmiVersion
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services
* optimizes these configurations for different machine learning workloads.
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific
* software requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron
* driver versions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantInferenceAmiVersion
*/
public ProductionVariant withInferenceAmiVersion(String inferenceAmiVersion) {
setInferenceAmiVersion(inferenceAmiVersion);
return this;
}
/**
*
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services optimizes these
* configurations for different machine learning workloads.
*
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific software
* requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron driver versions.
*
*
* @param inferenceAmiVersion
* Specifies an option from a collection of preconfigured Amazon Machine Image (AMI) images. Each image is
* configured by Amazon Web Services with a set of software and driver versions. Amazon Web Services
* optimizes these configurations for different machine learning workloads.
*
* By selecting an AMI version, you can ensure that your inference environment is compatible with specific
* software requirements, such as CUDA driver versions, Linux kernel versions, or Amazon Web Services Neuron
* driver versions.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ProductionVariantInferenceAmiVersion
*/
public ProductionVariant withInferenceAmiVersion(ProductionVariantInferenceAmiVersion inferenceAmiVersion) {
this.inferenceAmiVersion = inferenceAmiVersion.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getVariantName() != null)
sb.append("VariantName: ").append(getVariantName()).append(",");
if (getModelName() != null)
sb.append("ModelName: ").append(getModelName()).append(",");
if (getInitialInstanceCount() != null)
sb.append("InitialInstanceCount: ").append(getInitialInstanceCount()).append(",");
if (getInstanceType() != null)
sb.append("InstanceType: ").append(getInstanceType()).append(",");
if (getInitialVariantWeight() != null)
sb.append("InitialVariantWeight: ").append(getInitialVariantWeight()).append(",");
if (getAcceleratorType() != null)
sb.append("AcceleratorType: ").append(getAcceleratorType()).append(",");
if (getCoreDumpConfig() != null)
sb.append("CoreDumpConfig: ").append(getCoreDumpConfig()).append(",");
if (getServerlessConfig() != null)
sb.append("ServerlessConfig: ").append(getServerlessConfig()).append(",");
if (getVolumeSizeInGB() != null)
sb.append("VolumeSizeInGB: ").append(getVolumeSizeInGB()).append(",");
if (getModelDataDownloadTimeoutInSeconds() != null)
sb.append("ModelDataDownloadTimeoutInSeconds: ").append(getModelDataDownloadTimeoutInSeconds()).append(",");
if (getContainerStartupHealthCheckTimeoutInSeconds() != null)
sb.append("ContainerStartupHealthCheckTimeoutInSeconds: ").append(getContainerStartupHealthCheckTimeoutInSeconds()).append(",");
if (getEnableSSMAccess() != null)
sb.append("EnableSSMAccess: ").append(getEnableSSMAccess()).append(",");
if (getManagedInstanceScaling() != null)
sb.append("ManagedInstanceScaling: ").append(getManagedInstanceScaling()).append(",");
if (getRoutingConfig() != null)
sb.append("RoutingConfig: ").append(getRoutingConfig()).append(",");
if (getInferenceAmiVersion() != null)
sb.append("InferenceAmiVersion: ").append(getInferenceAmiVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ProductionVariant == false)
return false;
ProductionVariant other = (ProductionVariant) obj;
if (other.getVariantName() == null ^ this.getVariantName() == null)
return false;
if (other.getVariantName() != null && other.getVariantName().equals(this.getVariantName()) == false)
return false;
if (other.getModelName() == null ^ this.getModelName() == null)
return false;
if (other.getModelName() != null && other.getModelName().equals(this.getModelName()) == false)
return false;
if (other.getInitialInstanceCount() == null ^ this.getInitialInstanceCount() == null)
return false;
if (other.getInitialInstanceCount() != null && other.getInitialInstanceCount().equals(this.getInitialInstanceCount()) == false)
return false;
if (other.getInstanceType() == null ^ this.getInstanceType() == null)
return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false)
return false;
if (other.getInitialVariantWeight() == null ^ this.getInitialVariantWeight() == null)
return false;
if (other.getInitialVariantWeight() != null && other.getInitialVariantWeight().equals(this.getInitialVariantWeight()) == false)
return false;
if (other.getAcceleratorType() == null ^ this.getAcceleratorType() == null)
return false;
if (other.getAcceleratorType() != null && other.getAcceleratorType().equals(this.getAcceleratorType()) == false)
return false;
if (other.getCoreDumpConfig() == null ^ this.getCoreDumpConfig() == null)
return false;
if (other.getCoreDumpConfig() != null && other.getCoreDumpConfig().equals(this.getCoreDumpConfig()) == false)
return false;
if (other.getServerlessConfig() == null ^ this.getServerlessConfig() == null)
return false;
if (other.getServerlessConfig() != null && other.getServerlessConfig().equals(this.getServerlessConfig()) == false)
return false;
if (other.getVolumeSizeInGB() == null ^ this.getVolumeSizeInGB() == null)
return false;
if (other.getVolumeSizeInGB() != null && other.getVolumeSizeInGB().equals(this.getVolumeSizeInGB()) == false)
return false;
if (other.getModelDataDownloadTimeoutInSeconds() == null ^ this.getModelDataDownloadTimeoutInSeconds() == null)
return false;
if (other.getModelDataDownloadTimeoutInSeconds() != null
&& other.getModelDataDownloadTimeoutInSeconds().equals(this.getModelDataDownloadTimeoutInSeconds()) == false)
return false;
if (other.getContainerStartupHealthCheckTimeoutInSeconds() == null ^ this.getContainerStartupHealthCheckTimeoutInSeconds() == null)
return false;
if (other.getContainerStartupHealthCheckTimeoutInSeconds() != null
&& other.getContainerStartupHealthCheckTimeoutInSeconds().equals(this.getContainerStartupHealthCheckTimeoutInSeconds()) == false)
return false;
if (other.getEnableSSMAccess() == null ^ this.getEnableSSMAccess() == null)
return false;
if (other.getEnableSSMAccess() != null && other.getEnableSSMAccess().equals(this.getEnableSSMAccess()) == false)
return false;
if (other.getManagedInstanceScaling() == null ^ this.getManagedInstanceScaling() == null)
return false;
if (other.getManagedInstanceScaling() != null && other.getManagedInstanceScaling().equals(this.getManagedInstanceScaling()) == false)
return false;
if (other.getRoutingConfig() == null ^ this.getRoutingConfig() == null)
return false;
if (other.getRoutingConfig() != null && other.getRoutingConfig().equals(this.getRoutingConfig()) == false)
return false;
if (other.getInferenceAmiVersion() == null ^ this.getInferenceAmiVersion() == null)
return false;
if (other.getInferenceAmiVersion() != null && other.getInferenceAmiVersion().equals(this.getInferenceAmiVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getVariantName() == null) ? 0 : getVariantName().hashCode());
hashCode = prime * hashCode + ((getModelName() == null) ? 0 : getModelName().hashCode());
hashCode = prime * hashCode + ((getInitialInstanceCount() == null) ? 0 : getInitialInstanceCount().hashCode());
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getInitialVariantWeight() == null) ? 0 : getInitialVariantWeight().hashCode());
hashCode = prime * hashCode + ((getAcceleratorType() == null) ? 0 : getAcceleratorType().hashCode());
hashCode = prime * hashCode + ((getCoreDumpConfig() == null) ? 0 : getCoreDumpConfig().hashCode());
hashCode = prime * hashCode + ((getServerlessConfig() == null) ? 0 : getServerlessConfig().hashCode());
hashCode = prime * hashCode + ((getVolumeSizeInGB() == null) ? 0 : getVolumeSizeInGB().hashCode());
hashCode = prime * hashCode + ((getModelDataDownloadTimeoutInSeconds() == null) ? 0 : getModelDataDownloadTimeoutInSeconds().hashCode());
hashCode = prime * hashCode
+ ((getContainerStartupHealthCheckTimeoutInSeconds() == null) ? 0 : getContainerStartupHealthCheckTimeoutInSeconds().hashCode());
hashCode = prime * hashCode + ((getEnableSSMAccess() == null) ? 0 : getEnableSSMAccess().hashCode());
hashCode = prime * hashCode + ((getManagedInstanceScaling() == null) ? 0 : getManagedInstanceScaling().hashCode());
hashCode = prime * hashCode + ((getRoutingConfig() == null) ? 0 : getRoutingConfig().hashCode());
hashCode = prime * hashCode + ((getInferenceAmiVersion() == null) ? 0 : getInferenceAmiVersion().hashCode());
return hashCode;
}
@Override
public ProductionVariant clone() {
try {
return (ProductionVariant) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.ProductionVariantMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}