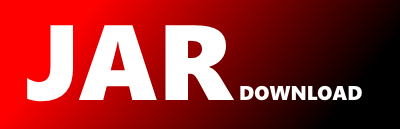
com.amazonaws.services.sagemaker.model.RecommendationJobInputConfig Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The input configuration of the recommendation job.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RecommendationJobInputConfig implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of a versioned model package.
*
*/
private String modelPackageVersionArn;
/**
*
* The name of the created model.
*
*/
private String modelName;
/**
*
* Specifies the maximum duration of the job, in seconds. The maximum value is 18,000 seconds.
*
*/
private Integer jobDurationInSeconds;
/**
*
* Specifies the traffic pattern of the job.
*
*/
private TrafficPattern trafficPattern;
/**
*
* Defines the resource limit of the job.
*
*/
private RecommendationJobResourceLimit resourceLimit;
/**
*
* Specifies the endpoint configuration to use for a job.
*
*/
private java.util.List endpointConfigurations;
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service (Amazon Web Services KMS) key that
* Amazon SageMaker uses to encrypt data on the storage volume attached to the ML compute instance that hosts the
* endpoint. This key will be passed to SageMaker Hosting for endpoint creation.
*
*
* The SageMaker execution role must have kms:CreateGrant
permission in order to encrypt data on the
* storage volume of the endpoints created for inference recommendation. The inference recommendation job will fail
* asynchronously during endpoint configuration creation if the role passed does not have
* kms:CreateGrant
permission.
*
*
* The KmsKeyId
can be any of the following formats:
*
*
* -
*
* // KMS Key ID
*
*
* "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key
*
*
* "arn:aws:kms:<region>:<account>:key/<key-id-12ab-34cd-56ef-1234567890ab>"
*
*
* -
*
* // KMS Key Alias
*
*
* "alias/ExampleAlias"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key Alias
*
*
* "arn:aws:kms:<region>:<account>:alias/<ExampleAlias>"
*
*
*
*
* For more information about key identifiers, see Key identifiers
* (KeyID) in the Amazon Web Services Key Management Service (Amazon Web Services KMS) documentation.
*
*/
private String volumeKmsKeyId;
/**
*
* Specifies mandatory fields for running an Inference Recommender job. The fields specified in
* ContainerConfig
override the corresponding fields in the model package.
*
*/
private RecommendationJobContainerConfig containerConfig;
/**
*
* Existing customer endpoints on which to run an Inference Recommender job.
*
*/
private java.util.List endpoints;
/**
*
* Inference Recommender provisions SageMaker endpoints with access to VPC in the inference recommendation job.
*
*/
private RecommendationJobVpcConfig vpcConfig;
/**
*
* The Amazon Resource Name (ARN) of a versioned model package.
*
*
* @param modelPackageVersionArn
* The Amazon Resource Name (ARN) of a versioned model package.
*/
public void setModelPackageVersionArn(String modelPackageVersionArn) {
this.modelPackageVersionArn = modelPackageVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of a versioned model package.
*
*
* @return The Amazon Resource Name (ARN) of a versioned model package.
*/
public String getModelPackageVersionArn() {
return this.modelPackageVersionArn;
}
/**
*
* The Amazon Resource Name (ARN) of a versioned model package.
*
*
* @param modelPackageVersionArn
* The Amazon Resource Name (ARN) of a versioned model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withModelPackageVersionArn(String modelPackageVersionArn) {
setModelPackageVersionArn(modelPackageVersionArn);
return this;
}
/**
*
* The name of the created model.
*
*
* @param modelName
* The name of the created model.
*/
public void setModelName(String modelName) {
this.modelName = modelName;
}
/**
*
* The name of the created model.
*
*
* @return The name of the created model.
*/
public String getModelName() {
return this.modelName;
}
/**
*
* The name of the created model.
*
*
* @param modelName
* The name of the created model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withModelName(String modelName) {
setModelName(modelName);
return this;
}
/**
*
* Specifies the maximum duration of the job, in seconds. The maximum value is 18,000 seconds.
*
*
* @param jobDurationInSeconds
* Specifies the maximum duration of the job, in seconds. The maximum value is 18,000 seconds.
*/
public void setJobDurationInSeconds(Integer jobDurationInSeconds) {
this.jobDurationInSeconds = jobDurationInSeconds;
}
/**
*
* Specifies the maximum duration of the job, in seconds. The maximum value is 18,000 seconds.
*
*
* @return Specifies the maximum duration of the job, in seconds. The maximum value is 18,000 seconds.
*/
public Integer getJobDurationInSeconds() {
return this.jobDurationInSeconds;
}
/**
*
* Specifies the maximum duration of the job, in seconds. The maximum value is 18,000 seconds.
*
*
* @param jobDurationInSeconds
* Specifies the maximum duration of the job, in seconds. The maximum value is 18,000 seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withJobDurationInSeconds(Integer jobDurationInSeconds) {
setJobDurationInSeconds(jobDurationInSeconds);
return this;
}
/**
*
* Specifies the traffic pattern of the job.
*
*
* @param trafficPattern
* Specifies the traffic pattern of the job.
*/
public void setTrafficPattern(TrafficPattern trafficPattern) {
this.trafficPattern = trafficPattern;
}
/**
*
* Specifies the traffic pattern of the job.
*
*
* @return Specifies the traffic pattern of the job.
*/
public TrafficPattern getTrafficPattern() {
return this.trafficPattern;
}
/**
*
* Specifies the traffic pattern of the job.
*
*
* @param trafficPattern
* Specifies the traffic pattern of the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withTrafficPattern(TrafficPattern trafficPattern) {
setTrafficPattern(trafficPattern);
return this;
}
/**
*
* Defines the resource limit of the job.
*
*
* @param resourceLimit
* Defines the resource limit of the job.
*/
public void setResourceLimit(RecommendationJobResourceLimit resourceLimit) {
this.resourceLimit = resourceLimit;
}
/**
*
* Defines the resource limit of the job.
*
*
* @return Defines the resource limit of the job.
*/
public RecommendationJobResourceLimit getResourceLimit() {
return this.resourceLimit;
}
/**
*
* Defines the resource limit of the job.
*
*
* @param resourceLimit
* Defines the resource limit of the job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withResourceLimit(RecommendationJobResourceLimit resourceLimit) {
setResourceLimit(resourceLimit);
return this;
}
/**
*
* Specifies the endpoint configuration to use for a job.
*
*
* @return Specifies the endpoint configuration to use for a job.
*/
public java.util.List getEndpointConfigurations() {
return endpointConfigurations;
}
/**
*
* Specifies the endpoint configuration to use for a job.
*
*
* @param endpointConfigurations
* Specifies the endpoint configuration to use for a job.
*/
public void setEndpointConfigurations(java.util.Collection endpointConfigurations) {
if (endpointConfigurations == null) {
this.endpointConfigurations = null;
return;
}
this.endpointConfigurations = new java.util.ArrayList(endpointConfigurations);
}
/**
*
* Specifies the endpoint configuration to use for a job.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEndpointConfigurations(java.util.Collection)} or
* {@link #withEndpointConfigurations(java.util.Collection)} if you want to override the existing values.
*
*
* @param endpointConfigurations
* Specifies the endpoint configuration to use for a job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withEndpointConfigurations(EndpointInputConfiguration... endpointConfigurations) {
if (this.endpointConfigurations == null) {
setEndpointConfigurations(new java.util.ArrayList(endpointConfigurations.length));
}
for (EndpointInputConfiguration ele : endpointConfigurations) {
this.endpointConfigurations.add(ele);
}
return this;
}
/**
*
* Specifies the endpoint configuration to use for a job.
*
*
* @param endpointConfigurations
* Specifies the endpoint configuration to use for a job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withEndpointConfigurations(java.util.Collection endpointConfigurations) {
setEndpointConfigurations(endpointConfigurations);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service (Amazon Web Services KMS) key that
* Amazon SageMaker uses to encrypt data on the storage volume attached to the ML compute instance that hosts the
* endpoint. This key will be passed to SageMaker Hosting for endpoint creation.
*
*
* The SageMaker execution role must have kms:CreateGrant
permission in order to encrypt data on the
* storage volume of the endpoints created for inference recommendation. The inference recommendation job will fail
* asynchronously during endpoint configuration creation if the role passed does not have
* kms:CreateGrant
permission.
*
*
* The KmsKeyId
can be any of the following formats:
*
*
* -
*
* // KMS Key ID
*
*
* "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key
*
*
* "arn:aws:kms:<region>:<account>:key/<key-id-12ab-34cd-56ef-1234567890ab>"
*
*
* -
*
* // KMS Key Alias
*
*
* "alias/ExampleAlias"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key Alias
*
*
* "arn:aws:kms:<region>:<account>:alias/<ExampleAlias>"
*
*
*
*
* For more information about key identifiers, see Key identifiers
* (KeyID) in the Amazon Web Services Key Management Service (Amazon Web Services KMS) documentation.
*
*
* @param volumeKmsKeyId
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service (Amazon Web Services KMS)
* key that Amazon SageMaker uses to encrypt data on the storage volume attached to the ML compute instance
* that hosts the endpoint. This key will be passed to SageMaker Hosting for endpoint creation.
*
* The SageMaker execution role must have kms:CreateGrant
permission in order to encrypt data on
* the storage volume of the endpoints created for inference recommendation. The inference recommendation job
* will fail asynchronously during endpoint configuration creation if the role passed does not have
* kms:CreateGrant
permission.
*
*
* The KmsKeyId
can be any of the following formats:
*
*
* -
*
* // KMS Key ID
*
*
* "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key
*
*
* "arn:aws:kms:<region>:<account>:key/<key-id-12ab-34cd-56ef-1234567890ab>"
*
*
* -
*
* // KMS Key Alias
*
*
* "alias/ExampleAlias"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key Alias
*
*
* "arn:aws:kms:<region>:<account>:alias/<ExampleAlias>"
*
*
*
*
* For more information about key identifiers, see Key identifiers
* (KeyID) in the Amazon Web Services Key Management Service (Amazon Web Services KMS) documentation.
*/
public void setVolumeKmsKeyId(String volumeKmsKeyId) {
this.volumeKmsKeyId = volumeKmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service (Amazon Web Services KMS) key that
* Amazon SageMaker uses to encrypt data on the storage volume attached to the ML compute instance that hosts the
* endpoint. This key will be passed to SageMaker Hosting for endpoint creation.
*
*
* The SageMaker execution role must have kms:CreateGrant
permission in order to encrypt data on the
* storage volume of the endpoints created for inference recommendation. The inference recommendation job will fail
* asynchronously during endpoint configuration creation if the role passed does not have
* kms:CreateGrant
permission.
*
*
* The KmsKeyId
can be any of the following formats:
*
*
* -
*
* // KMS Key ID
*
*
* "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key
*
*
* "arn:aws:kms:<region>:<account>:key/<key-id-12ab-34cd-56ef-1234567890ab>"
*
*
* -
*
* // KMS Key Alias
*
*
* "alias/ExampleAlias"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key Alias
*
*
* "arn:aws:kms:<region>:<account>:alias/<ExampleAlias>"
*
*
*
*
* For more information about key identifiers, see Key identifiers
* (KeyID) in the Amazon Web Services Key Management Service (Amazon Web Services KMS) documentation.
*
*
* @return The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service (Amazon Web Services KMS)
* key that Amazon SageMaker uses to encrypt data on the storage volume attached to the ML compute instance
* that hosts the endpoint. This key will be passed to SageMaker Hosting for endpoint creation.
*
* The SageMaker execution role must have kms:CreateGrant
permission in order to encrypt data
* on the storage volume of the endpoints created for inference recommendation. The inference recommendation
* job will fail asynchronously during endpoint configuration creation if the role passed does not have
* kms:CreateGrant
permission.
*
*
* The KmsKeyId
can be any of the following formats:
*
*
* -
*
* // KMS Key ID
*
*
* "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key
*
*
* "arn:aws:kms:<region>:<account>:key/<key-id-12ab-34cd-56ef-1234567890ab>"
*
*
* -
*
* // KMS Key Alias
*
*
* "alias/ExampleAlias"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key Alias
*
*
* "arn:aws:kms:<region>:<account>:alias/<ExampleAlias>"
*
*
*
*
* For more information about key identifiers, see Key identifiers
* (KeyID) in the Amazon Web Services Key Management Service (Amazon Web Services KMS) documentation.
*/
public String getVolumeKmsKeyId() {
return this.volumeKmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service (Amazon Web Services KMS) key that
* Amazon SageMaker uses to encrypt data on the storage volume attached to the ML compute instance that hosts the
* endpoint. This key will be passed to SageMaker Hosting for endpoint creation.
*
*
* The SageMaker execution role must have kms:CreateGrant
permission in order to encrypt data on the
* storage volume of the endpoints created for inference recommendation. The inference recommendation job will fail
* asynchronously during endpoint configuration creation if the role passed does not have
* kms:CreateGrant
permission.
*
*
* The KmsKeyId
can be any of the following formats:
*
*
* -
*
* // KMS Key ID
*
*
* "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key
*
*
* "arn:aws:kms:<region>:<account>:key/<key-id-12ab-34cd-56ef-1234567890ab>"
*
*
* -
*
* // KMS Key Alias
*
*
* "alias/ExampleAlias"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key Alias
*
*
* "arn:aws:kms:<region>:<account>:alias/<ExampleAlias>"
*
*
*
*
* For more information about key identifiers, see Key identifiers
* (KeyID) in the Amazon Web Services Key Management Service (Amazon Web Services KMS) documentation.
*
*
* @param volumeKmsKeyId
* The Amazon Resource Name (ARN) of a Amazon Web Services Key Management Service (Amazon Web Services KMS)
* key that Amazon SageMaker uses to encrypt data on the storage volume attached to the ML compute instance
* that hosts the endpoint. This key will be passed to SageMaker Hosting for endpoint creation.
*
* The SageMaker execution role must have kms:CreateGrant
permission in order to encrypt data on
* the storage volume of the endpoints created for inference recommendation. The inference recommendation job
* will fail asynchronously during endpoint configuration creation if the role passed does not have
* kms:CreateGrant
permission.
*
*
* The KmsKeyId
can be any of the following formats:
*
*
* -
*
* // KMS Key ID
*
*
* "1234abcd-12ab-34cd-56ef-1234567890ab"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key
*
*
* "arn:aws:kms:<region>:<account>:key/<key-id-12ab-34cd-56ef-1234567890ab>"
*
*
* -
*
* // KMS Key Alias
*
*
* "alias/ExampleAlias"
*
*
* -
*
* // Amazon Resource Name (ARN) of a KMS Key Alias
*
*
* "arn:aws:kms:<region>:<account>:alias/<ExampleAlias>"
*
*
*
*
* For more information about key identifiers, see Key identifiers
* (KeyID) in the Amazon Web Services Key Management Service (Amazon Web Services KMS) documentation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withVolumeKmsKeyId(String volumeKmsKeyId) {
setVolumeKmsKeyId(volumeKmsKeyId);
return this;
}
/**
*
* Specifies mandatory fields for running an Inference Recommender job. The fields specified in
* ContainerConfig
override the corresponding fields in the model package.
*
*
* @param containerConfig
* Specifies mandatory fields for running an Inference Recommender job. The fields specified in
* ContainerConfig
override the corresponding fields in the model package.
*/
public void setContainerConfig(RecommendationJobContainerConfig containerConfig) {
this.containerConfig = containerConfig;
}
/**
*
* Specifies mandatory fields for running an Inference Recommender job. The fields specified in
* ContainerConfig
override the corresponding fields in the model package.
*
*
* @return Specifies mandatory fields for running an Inference Recommender job. The fields specified in
* ContainerConfig
override the corresponding fields in the model package.
*/
public RecommendationJobContainerConfig getContainerConfig() {
return this.containerConfig;
}
/**
*
* Specifies mandatory fields for running an Inference Recommender job. The fields specified in
* ContainerConfig
override the corresponding fields in the model package.
*
*
* @param containerConfig
* Specifies mandatory fields for running an Inference Recommender job. The fields specified in
* ContainerConfig
override the corresponding fields in the model package.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withContainerConfig(RecommendationJobContainerConfig containerConfig) {
setContainerConfig(containerConfig);
return this;
}
/**
*
* Existing customer endpoints on which to run an Inference Recommender job.
*
*
* @return Existing customer endpoints on which to run an Inference Recommender job.
*/
public java.util.List getEndpoints() {
return endpoints;
}
/**
*
* Existing customer endpoints on which to run an Inference Recommender job.
*
*
* @param endpoints
* Existing customer endpoints on which to run an Inference Recommender job.
*/
public void setEndpoints(java.util.Collection endpoints) {
if (endpoints == null) {
this.endpoints = null;
return;
}
this.endpoints = new java.util.ArrayList(endpoints);
}
/**
*
* Existing customer endpoints on which to run an Inference Recommender job.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEndpoints(java.util.Collection)} or {@link #withEndpoints(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param endpoints
* Existing customer endpoints on which to run an Inference Recommender job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withEndpoints(EndpointInfo... endpoints) {
if (this.endpoints == null) {
setEndpoints(new java.util.ArrayList(endpoints.length));
}
for (EndpointInfo ele : endpoints) {
this.endpoints.add(ele);
}
return this;
}
/**
*
* Existing customer endpoints on which to run an Inference Recommender job.
*
*
* @param endpoints
* Existing customer endpoints on which to run an Inference Recommender job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withEndpoints(java.util.Collection endpoints) {
setEndpoints(endpoints);
return this;
}
/**
*
* Inference Recommender provisions SageMaker endpoints with access to VPC in the inference recommendation job.
*
*
* @param vpcConfig
* Inference Recommender provisions SageMaker endpoints with access to VPC in the inference recommendation
* job.
*/
public void setVpcConfig(RecommendationJobVpcConfig vpcConfig) {
this.vpcConfig = vpcConfig;
}
/**
*
* Inference Recommender provisions SageMaker endpoints with access to VPC in the inference recommendation job.
*
*
* @return Inference Recommender provisions SageMaker endpoints with access to VPC in the inference recommendation
* job.
*/
public RecommendationJobVpcConfig getVpcConfig() {
return this.vpcConfig;
}
/**
*
* Inference Recommender provisions SageMaker endpoints with access to VPC in the inference recommendation job.
*
*
* @param vpcConfig
* Inference Recommender provisions SageMaker endpoints with access to VPC in the inference recommendation
* job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RecommendationJobInputConfig withVpcConfig(RecommendationJobVpcConfig vpcConfig) {
setVpcConfig(vpcConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getModelPackageVersionArn() != null)
sb.append("ModelPackageVersionArn: ").append(getModelPackageVersionArn()).append(",");
if (getModelName() != null)
sb.append("ModelName: ").append(getModelName()).append(",");
if (getJobDurationInSeconds() != null)
sb.append("JobDurationInSeconds: ").append(getJobDurationInSeconds()).append(",");
if (getTrafficPattern() != null)
sb.append("TrafficPattern: ").append(getTrafficPattern()).append(",");
if (getResourceLimit() != null)
sb.append("ResourceLimit: ").append(getResourceLimit()).append(",");
if (getEndpointConfigurations() != null)
sb.append("EndpointConfigurations: ").append(getEndpointConfigurations()).append(",");
if (getVolumeKmsKeyId() != null)
sb.append("VolumeKmsKeyId: ").append(getVolumeKmsKeyId()).append(",");
if (getContainerConfig() != null)
sb.append("ContainerConfig: ").append(getContainerConfig()).append(",");
if (getEndpoints() != null)
sb.append("Endpoints: ").append(getEndpoints()).append(",");
if (getVpcConfig() != null)
sb.append("VpcConfig: ").append(getVpcConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RecommendationJobInputConfig == false)
return false;
RecommendationJobInputConfig other = (RecommendationJobInputConfig) obj;
if (other.getModelPackageVersionArn() == null ^ this.getModelPackageVersionArn() == null)
return false;
if (other.getModelPackageVersionArn() != null && other.getModelPackageVersionArn().equals(this.getModelPackageVersionArn()) == false)
return false;
if (other.getModelName() == null ^ this.getModelName() == null)
return false;
if (other.getModelName() != null && other.getModelName().equals(this.getModelName()) == false)
return false;
if (other.getJobDurationInSeconds() == null ^ this.getJobDurationInSeconds() == null)
return false;
if (other.getJobDurationInSeconds() != null && other.getJobDurationInSeconds().equals(this.getJobDurationInSeconds()) == false)
return false;
if (other.getTrafficPattern() == null ^ this.getTrafficPattern() == null)
return false;
if (other.getTrafficPattern() != null && other.getTrafficPattern().equals(this.getTrafficPattern()) == false)
return false;
if (other.getResourceLimit() == null ^ this.getResourceLimit() == null)
return false;
if (other.getResourceLimit() != null && other.getResourceLimit().equals(this.getResourceLimit()) == false)
return false;
if (other.getEndpointConfigurations() == null ^ this.getEndpointConfigurations() == null)
return false;
if (other.getEndpointConfigurations() != null && other.getEndpointConfigurations().equals(this.getEndpointConfigurations()) == false)
return false;
if (other.getVolumeKmsKeyId() == null ^ this.getVolumeKmsKeyId() == null)
return false;
if (other.getVolumeKmsKeyId() != null && other.getVolumeKmsKeyId().equals(this.getVolumeKmsKeyId()) == false)
return false;
if (other.getContainerConfig() == null ^ this.getContainerConfig() == null)
return false;
if (other.getContainerConfig() != null && other.getContainerConfig().equals(this.getContainerConfig()) == false)
return false;
if (other.getEndpoints() == null ^ this.getEndpoints() == null)
return false;
if (other.getEndpoints() != null && other.getEndpoints().equals(this.getEndpoints()) == false)
return false;
if (other.getVpcConfig() == null ^ this.getVpcConfig() == null)
return false;
if (other.getVpcConfig() != null && other.getVpcConfig().equals(this.getVpcConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getModelPackageVersionArn() == null) ? 0 : getModelPackageVersionArn().hashCode());
hashCode = prime * hashCode + ((getModelName() == null) ? 0 : getModelName().hashCode());
hashCode = prime * hashCode + ((getJobDurationInSeconds() == null) ? 0 : getJobDurationInSeconds().hashCode());
hashCode = prime * hashCode + ((getTrafficPattern() == null) ? 0 : getTrafficPattern().hashCode());
hashCode = prime * hashCode + ((getResourceLimit() == null) ? 0 : getResourceLimit().hashCode());
hashCode = prime * hashCode + ((getEndpointConfigurations() == null) ? 0 : getEndpointConfigurations().hashCode());
hashCode = prime * hashCode + ((getVolumeKmsKeyId() == null) ? 0 : getVolumeKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getContainerConfig() == null) ? 0 : getContainerConfig().hashCode());
hashCode = prime * hashCode + ((getEndpoints() == null) ? 0 : getEndpoints().hashCode());
hashCode = prime * hashCode + ((getVpcConfig() == null) ? 0 : getVpcConfig().hashCode());
return hashCode;
}
@Override
public RecommendationJobInputConfig clone() {
try {
return (RecommendationJobInputConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.RecommendationJobInputConfigMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}