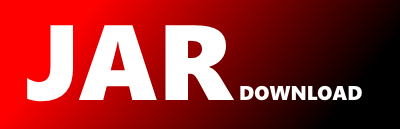
com.amazonaws.services.sagemaker.model.S3ModelDataSource Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Specifies the S3 location of ML model data to deploy.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class S3ModelDataSource implements Serializable, Cloneable, StructuredPojo {
/**
*
* Specifies the S3 path of ML model data to deploy.
*
*/
private String s3Uri;
/**
*
* Specifies the type of ML model data to deploy.
*
*
* If you choose S3Prefix
, S3Uri
identifies a key name prefix. SageMaker uses all objects
* that match the specified key name prefix as part of the ML model data to deploy. A valid key name prefix
* identified by S3Uri
always ends with a forward slash (/).
*
*
* If you choose S3Object
, S3Uri
identifies an object that is the ML model data to deploy.
*
*/
private String s3DataType;
/**
*
* Specifies how the ML model data is prepared.
*
*
* If you choose Gzip
and choose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that is a gzip-compressed TAR archive. SageMaker will attempt to
* decompress and untar the object during model deployment.
*
*
* If you choose None
and chooose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that represents an uncompressed ML model to deploy.
*
*
* If you choose None and choose S3Prefix
as the value of S3DataType
, S3Uri
* identifies a key name prefix, under which all objects represents the uncompressed ML model to deploy.
*
*
* If you choose None, then SageMaker will follow rules below when creating model data files under /opt/ml/model
* directory for use by your inference code:
*
*
* -
*
* If you choose S3Object
as the value of S3DataType
, then SageMaker will split the key of
* the S3 object referenced by S3Uri
by slash (/), and use the last part as the filename of the file
* holding the content of the S3 object.
*
*
* -
*
* If you choose S3Prefix
as the value of S3DataType
, then for each S3 object under the
* key name pefix referenced by S3Uri
, SageMaker will trim its key by the prefix, and use the remainder
* as the path (relative to /opt/ml/model
) of the file holding the content of the S3 object. SageMaker
* will split the remainder by slash (/), using intermediate parts as directory names and the last part as filename
* of the file holding the content of the S3 object.
*
*
* -
*
* Do not use any of the following as file names or directory names:
*
*
* -
*
* An empty or blank string
*
*
* -
*
* A string which contains null bytes
*
*
* -
*
* A string longer than 255 bytes
*
*
* -
*
* A single dot (.
)
*
*
* -
*
* A double dot (..
)
*
*
*
*
* -
*
* Ambiguous file names will result in model deployment failure. For example, if your uncompressed ML model consists
* of two S3 objects s3://mybucket/model/weights
and s3://mybucket/model/weights/part1
and
* you specify s3://mybucket/model/
as the value of S3Uri
and S3Prefix
as the
* value of S3DataType
, then it will result in name clash between /opt/ml/model/weights
(a
* regular file) and /opt/ml/model/weights/
(a directory).
*
*
* -
*
* Do not organize the model artifacts in S3 console using folders.
* When you create a folder in S3 console, S3 creates a 0-byte object with a key set to the folder name you provide.
* They key of the 0-byte object ends with a slash (/) which violates SageMaker restrictions on model artifact file
* names, leading to model deployment failure.
*
*
*
*/
private String compressionType;
/**
*
* Specifies the access configuration file for the ML model. You can explicitly accept the model end-user license
* agreement (EULA) within the ModelAccessConfig
. You are responsible for reviewing and complying with
* any applicable license terms and making sure they are acceptable for your use case before downloading or using a
* model.
*
*/
private ModelAccessConfig modelAccessConfig;
/**
*
* Configuration information for hub access.
*
*/
private InferenceHubAccessConfig hubAccessConfig;
/**
*
* Specifies the S3 path of ML model data to deploy.
*
*
* @param s3Uri
* Specifies the S3 path of ML model data to deploy.
*/
public void setS3Uri(String s3Uri) {
this.s3Uri = s3Uri;
}
/**
*
* Specifies the S3 path of ML model data to deploy.
*
*
* @return Specifies the S3 path of ML model data to deploy.
*/
public String getS3Uri() {
return this.s3Uri;
}
/**
*
* Specifies the S3 path of ML model data to deploy.
*
*
* @param s3Uri
* Specifies the S3 path of ML model data to deploy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3ModelDataSource withS3Uri(String s3Uri) {
setS3Uri(s3Uri);
return this;
}
/**
*
* Specifies the type of ML model data to deploy.
*
*
* If you choose S3Prefix
, S3Uri
identifies a key name prefix. SageMaker uses all objects
* that match the specified key name prefix as part of the ML model data to deploy. A valid key name prefix
* identified by S3Uri
always ends with a forward slash (/).
*
*
* If you choose S3Object
, S3Uri
identifies an object that is the ML model data to deploy.
*
*
* @param s3DataType
* Specifies the type of ML model data to deploy.
*
* If you choose S3Prefix
, S3Uri
identifies a key name prefix. SageMaker uses all
* objects that match the specified key name prefix as part of the ML model data to deploy. A valid key name
* prefix identified by S3Uri
always ends with a forward slash (/).
*
*
* If you choose S3Object
, S3Uri
identifies an object that is the ML model data to
* deploy.
* @see S3ModelDataType
*/
public void setS3DataType(String s3DataType) {
this.s3DataType = s3DataType;
}
/**
*
* Specifies the type of ML model data to deploy.
*
*
* If you choose S3Prefix
, S3Uri
identifies a key name prefix. SageMaker uses all objects
* that match the specified key name prefix as part of the ML model data to deploy. A valid key name prefix
* identified by S3Uri
always ends with a forward slash (/).
*
*
* If you choose S3Object
, S3Uri
identifies an object that is the ML model data to deploy.
*
*
* @return Specifies the type of ML model data to deploy.
*
* If you choose S3Prefix
, S3Uri
identifies a key name prefix. SageMaker uses all
* objects that match the specified key name prefix as part of the ML model data to deploy. A valid key name
* prefix identified by S3Uri
always ends with a forward slash (/).
*
*
* If you choose S3Object
, S3Uri
identifies an object that is the ML model data to
* deploy.
* @see S3ModelDataType
*/
public String getS3DataType() {
return this.s3DataType;
}
/**
*
* Specifies the type of ML model data to deploy.
*
*
* If you choose S3Prefix
, S3Uri
identifies a key name prefix. SageMaker uses all objects
* that match the specified key name prefix as part of the ML model data to deploy. A valid key name prefix
* identified by S3Uri
always ends with a forward slash (/).
*
*
* If you choose S3Object
, S3Uri
identifies an object that is the ML model data to deploy.
*
*
* @param s3DataType
* Specifies the type of ML model data to deploy.
*
* If you choose S3Prefix
, S3Uri
identifies a key name prefix. SageMaker uses all
* objects that match the specified key name prefix as part of the ML model data to deploy. A valid key name
* prefix identified by S3Uri
always ends with a forward slash (/).
*
*
* If you choose S3Object
, S3Uri
identifies an object that is the ML model data to
* deploy.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ModelDataType
*/
public S3ModelDataSource withS3DataType(String s3DataType) {
setS3DataType(s3DataType);
return this;
}
/**
*
* Specifies the type of ML model data to deploy.
*
*
* If you choose S3Prefix
, S3Uri
identifies a key name prefix. SageMaker uses all objects
* that match the specified key name prefix as part of the ML model data to deploy. A valid key name prefix
* identified by S3Uri
always ends with a forward slash (/).
*
*
* If you choose S3Object
, S3Uri
identifies an object that is the ML model data to deploy.
*
*
* @param s3DataType
* Specifies the type of ML model data to deploy.
*
* If you choose S3Prefix
, S3Uri
identifies a key name prefix. SageMaker uses all
* objects that match the specified key name prefix as part of the ML model data to deploy. A valid key name
* prefix identified by S3Uri
always ends with a forward slash (/).
*
*
* If you choose S3Object
, S3Uri
identifies an object that is the ML model data to
* deploy.
* @return Returns a reference to this object so that method calls can be chained together.
* @see S3ModelDataType
*/
public S3ModelDataSource withS3DataType(S3ModelDataType s3DataType) {
this.s3DataType = s3DataType.toString();
return this;
}
/**
*
* Specifies how the ML model data is prepared.
*
*
* If you choose Gzip
and choose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that is a gzip-compressed TAR archive. SageMaker will attempt to
* decompress and untar the object during model deployment.
*
*
* If you choose None
and chooose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that represents an uncompressed ML model to deploy.
*
*
* If you choose None and choose S3Prefix
as the value of S3DataType
, S3Uri
* identifies a key name prefix, under which all objects represents the uncompressed ML model to deploy.
*
*
* If you choose None, then SageMaker will follow rules below when creating model data files under /opt/ml/model
* directory for use by your inference code:
*
*
* -
*
* If you choose S3Object
as the value of S3DataType
, then SageMaker will split the key of
* the S3 object referenced by S3Uri
by slash (/), and use the last part as the filename of the file
* holding the content of the S3 object.
*
*
* -
*
* If you choose S3Prefix
as the value of S3DataType
, then for each S3 object under the
* key name pefix referenced by S3Uri
, SageMaker will trim its key by the prefix, and use the remainder
* as the path (relative to /opt/ml/model
) of the file holding the content of the S3 object. SageMaker
* will split the remainder by slash (/), using intermediate parts as directory names and the last part as filename
* of the file holding the content of the S3 object.
*
*
* -
*
* Do not use any of the following as file names or directory names:
*
*
* -
*
* An empty or blank string
*
*
* -
*
* A string which contains null bytes
*
*
* -
*
* A string longer than 255 bytes
*
*
* -
*
* A single dot (.
)
*
*
* -
*
* A double dot (..
)
*
*
*
*
* -
*
* Ambiguous file names will result in model deployment failure. For example, if your uncompressed ML model consists
* of two S3 objects s3://mybucket/model/weights
and s3://mybucket/model/weights/part1
and
* you specify s3://mybucket/model/
as the value of S3Uri
and S3Prefix
as the
* value of S3DataType
, then it will result in name clash between /opt/ml/model/weights
(a
* regular file) and /opt/ml/model/weights/
(a directory).
*
*
* -
*
* Do not organize the model artifacts in S3 console using folders.
* When you create a folder in S3 console, S3 creates a 0-byte object with a key set to the folder name you provide.
* They key of the 0-byte object ends with a slash (/) which violates SageMaker restrictions on model artifact file
* names, leading to model deployment failure.
*
*
*
*
* @param compressionType
* Specifies how the ML model data is prepared.
*
* If you choose Gzip
and choose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that is a gzip-compressed TAR archive. SageMaker will attempt to
* decompress and untar the object during model deployment.
*
*
* If you choose None
and chooose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that represents an uncompressed ML model to deploy.
*
*
* If you choose None and choose S3Prefix
as the value of S3DataType
,
* S3Uri
identifies a key name prefix, under which all objects represents the uncompressed ML
* model to deploy.
*
*
* If you choose None, then SageMaker will follow rules below when creating model data files under
* /opt/ml/model directory for use by your inference code:
*
*
* -
*
* If you choose S3Object
as the value of S3DataType
, then SageMaker will split the
* key of the S3 object referenced by S3Uri
by slash (/), and use the last part as the filename
* of the file holding the content of the S3 object.
*
*
* -
*
* If you choose S3Prefix
as the value of S3DataType
, then for each S3 object under
* the key name pefix referenced by S3Uri
, SageMaker will trim its key by the prefix, and use
* the remainder as the path (relative to /opt/ml/model
) of the file holding the content of the
* S3 object. SageMaker will split the remainder by slash (/), using intermediate parts as directory names
* and the last part as filename of the file holding the content of the S3 object.
*
*
* -
*
* Do not use any of the following as file names or directory names:
*
*
* -
*
* An empty or blank string
*
*
* -
*
* A string which contains null bytes
*
*
* -
*
* A string longer than 255 bytes
*
*
* -
*
* A single dot (.
)
*
*
* -
*
* A double dot (..
)
*
*
*
*
* -
*
* Ambiguous file names will result in model deployment failure. For example, if your uncompressed ML model
* consists of two S3 objects s3://mybucket/model/weights
and
* s3://mybucket/model/weights/part1
and you specify s3://mybucket/model/
as the
* value of S3Uri
and S3Prefix
as the value of S3DataType
, then it
* will result in name clash between /opt/ml/model/weights
(a regular file) and
* /opt/ml/model/weights/
(a directory).
*
*
* -
*
* Do not organize the model artifacts in S3 console using
* folders. When you create a folder in S3 console, S3 creates a 0-byte object with a key set to the
* folder name you provide. They key of the 0-byte object ends with a slash (/) which violates SageMaker
* restrictions on model artifact file names, leading to model deployment failure.
*
*
* @see ModelCompressionType
*/
public void setCompressionType(String compressionType) {
this.compressionType = compressionType;
}
/**
*
* Specifies how the ML model data is prepared.
*
*
* If you choose Gzip
and choose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that is a gzip-compressed TAR archive. SageMaker will attempt to
* decompress and untar the object during model deployment.
*
*
* If you choose None
and chooose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that represents an uncompressed ML model to deploy.
*
*
* If you choose None and choose S3Prefix
as the value of S3DataType
, S3Uri
* identifies a key name prefix, under which all objects represents the uncompressed ML model to deploy.
*
*
* If you choose None, then SageMaker will follow rules below when creating model data files under /opt/ml/model
* directory for use by your inference code:
*
*
* -
*
* If you choose S3Object
as the value of S3DataType
, then SageMaker will split the key of
* the S3 object referenced by S3Uri
by slash (/), and use the last part as the filename of the file
* holding the content of the S3 object.
*
*
* -
*
* If you choose S3Prefix
as the value of S3DataType
, then for each S3 object under the
* key name pefix referenced by S3Uri
, SageMaker will trim its key by the prefix, and use the remainder
* as the path (relative to /opt/ml/model
) of the file holding the content of the S3 object. SageMaker
* will split the remainder by slash (/), using intermediate parts as directory names and the last part as filename
* of the file holding the content of the S3 object.
*
*
* -
*
* Do not use any of the following as file names or directory names:
*
*
* -
*
* An empty or blank string
*
*
* -
*
* A string which contains null bytes
*
*
* -
*
* A string longer than 255 bytes
*
*
* -
*
* A single dot (.
)
*
*
* -
*
* A double dot (..
)
*
*
*
*
* -
*
* Ambiguous file names will result in model deployment failure. For example, if your uncompressed ML model consists
* of two S3 objects s3://mybucket/model/weights
and s3://mybucket/model/weights/part1
and
* you specify s3://mybucket/model/
as the value of S3Uri
and S3Prefix
as the
* value of S3DataType
, then it will result in name clash between /opt/ml/model/weights
(a
* regular file) and /opt/ml/model/weights/
(a directory).
*
*
* -
*
* Do not organize the model artifacts in S3 console using folders.
* When you create a folder in S3 console, S3 creates a 0-byte object with a key set to the folder name you provide.
* They key of the 0-byte object ends with a slash (/) which violates SageMaker restrictions on model artifact file
* names, leading to model deployment failure.
*
*
*
*
* @return Specifies how the ML model data is prepared.
*
* If you choose Gzip
and choose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that is a gzip-compressed TAR archive. SageMaker will attempt to
* decompress and untar the object during model deployment.
*
*
* If you choose None
and chooose S3Object
as the value of S3DataType
, S3Uri
identifies an object that represents an uncompressed ML model to deploy.
*
*
* If you choose None and choose S3Prefix
as the value of S3DataType
,
* S3Uri
identifies a key name prefix, under which all objects represents the uncompressed ML
* model to deploy.
*
*
* If you choose None, then SageMaker will follow rules below when creating model data files under
* /opt/ml/model directory for use by your inference code:
*
*
* -
*
* If you choose S3Object
as the value of S3DataType
, then SageMaker will split
* the key of the S3 object referenced by S3Uri
by slash (/), and use the last part as the
* filename of the file holding the content of the S3 object.
*
*
* -
*
* If you choose S3Prefix
as the value of S3DataType
, then for each S3 object
* under the key name pefix referenced by S3Uri
, SageMaker will trim its key by the prefix, and
* use the remainder as the path (relative to /opt/ml/model
) of the file holding the content of
* the S3 object. SageMaker will split the remainder by slash (/), using intermediate parts as directory
* names and the last part as filename of the file holding the content of the S3 object.
*
*
* -
*
* Do not use any of the following as file names or directory names:
*
*
* -
*
* An empty or blank string
*
*
* -
*
* A string which contains null bytes
*
*
* -
*
* A string longer than 255 bytes
*
*
* -
*
* A single dot (.
)
*
*
* -
*
* A double dot (..
)
*
*
*
*
* -
*
* Ambiguous file names will result in model deployment failure. For example, if your uncompressed ML model
* consists of two S3 objects s3://mybucket/model/weights
and
* s3://mybucket/model/weights/part1
and you specify s3://mybucket/model/
as the
* value of S3Uri
and S3Prefix
as the value of S3DataType
, then it
* will result in name clash between /opt/ml/model/weights
(a regular file) and
* /opt/ml/model/weights/
(a directory).
*
*
* -
*
* Do not organize the model artifacts in S3 console using
* folders. When you create a folder in S3 console, S3 creates a 0-byte object with a key set to the
* folder name you provide. They key of the 0-byte object ends with a slash (/) which violates SageMaker
* restrictions on model artifact file names, leading to model deployment failure.
*
*
* @see ModelCompressionType
*/
public String getCompressionType() {
return this.compressionType;
}
/**
*
* Specifies how the ML model data is prepared.
*
*
* If you choose Gzip
and choose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that is a gzip-compressed TAR archive. SageMaker will attempt to
* decompress and untar the object during model deployment.
*
*
* If you choose None
and chooose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that represents an uncompressed ML model to deploy.
*
*
* If you choose None and choose S3Prefix
as the value of S3DataType
, S3Uri
* identifies a key name prefix, under which all objects represents the uncompressed ML model to deploy.
*
*
* If you choose None, then SageMaker will follow rules below when creating model data files under /opt/ml/model
* directory for use by your inference code:
*
*
* -
*
* If you choose S3Object
as the value of S3DataType
, then SageMaker will split the key of
* the S3 object referenced by S3Uri
by slash (/), and use the last part as the filename of the file
* holding the content of the S3 object.
*
*
* -
*
* If you choose S3Prefix
as the value of S3DataType
, then for each S3 object under the
* key name pefix referenced by S3Uri
, SageMaker will trim its key by the prefix, and use the remainder
* as the path (relative to /opt/ml/model
) of the file holding the content of the S3 object. SageMaker
* will split the remainder by slash (/), using intermediate parts as directory names and the last part as filename
* of the file holding the content of the S3 object.
*
*
* -
*
* Do not use any of the following as file names or directory names:
*
*
* -
*
* An empty or blank string
*
*
* -
*
* A string which contains null bytes
*
*
* -
*
* A string longer than 255 bytes
*
*
* -
*
* A single dot (.
)
*
*
* -
*
* A double dot (..
)
*
*
*
*
* -
*
* Ambiguous file names will result in model deployment failure. For example, if your uncompressed ML model consists
* of two S3 objects s3://mybucket/model/weights
and s3://mybucket/model/weights/part1
and
* you specify s3://mybucket/model/
as the value of S3Uri
and S3Prefix
as the
* value of S3DataType
, then it will result in name clash between /opt/ml/model/weights
(a
* regular file) and /opt/ml/model/weights/
(a directory).
*
*
* -
*
* Do not organize the model artifacts in S3 console using folders.
* When you create a folder in S3 console, S3 creates a 0-byte object with a key set to the folder name you provide.
* They key of the 0-byte object ends with a slash (/) which violates SageMaker restrictions on model artifact file
* names, leading to model deployment failure.
*
*
*
*
* @param compressionType
* Specifies how the ML model data is prepared.
*
* If you choose Gzip
and choose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that is a gzip-compressed TAR archive. SageMaker will attempt to
* decompress and untar the object during model deployment.
*
*
* If you choose None
and chooose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that represents an uncompressed ML model to deploy.
*
*
* If you choose None and choose S3Prefix
as the value of S3DataType
,
* S3Uri
identifies a key name prefix, under which all objects represents the uncompressed ML
* model to deploy.
*
*
* If you choose None, then SageMaker will follow rules below when creating model data files under
* /opt/ml/model directory for use by your inference code:
*
*
* -
*
* If you choose S3Object
as the value of S3DataType
, then SageMaker will split the
* key of the S3 object referenced by S3Uri
by slash (/), and use the last part as the filename
* of the file holding the content of the S3 object.
*
*
* -
*
* If you choose S3Prefix
as the value of S3DataType
, then for each S3 object under
* the key name pefix referenced by S3Uri
, SageMaker will trim its key by the prefix, and use
* the remainder as the path (relative to /opt/ml/model
) of the file holding the content of the
* S3 object. SageMaker will split the remainder by slash (/), using intermediate parts as directory names
* and the last part as filename of the file holding the content of the S3 object.
*
*
* -
*
* Do not use any of the following as file names or directory names:
*
*
* -
*
* An empty or blank string
*
*
* -
*
* A string which contains null bytes
*
*
* -
*
* A string longer than 255 bytes
*
*
* -
*
* A single dot (.
)
*
*
* -
*
* A double dot (..
)
*
*
*
*
* -
*
* Ambiguous file names will result in model deployment failure. For example, if your uncompressed ML model
* consists of two S3 objects s3://mybucket/model/weights
and
* s3://mybucket/model/weights/part1
and you specify s3://mybucket/model/
as the
* value of S3Uri
and S3Prefix
as the value of S3DataType
, then it
* will result in name clash between /opt/ml/model/weights
(a regular file) and
* /opt/ml/model/weights/
(a directory).
*
*
* -
*
* Do not organize the model artifacts in S3 console using
* folders. When you create a folder in S3 console, S3 creates a 0-byte object with a key set to the
* folder name you provide. They key of the 0-byte object ends with a slash (/) which violates SageMaker
* restrictions on model artifact file names, leading to model deployment failure.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelCompressionType
*/
public S3ModelDataSource withCompressionType(String compressionType) {
setCompressionType(compressionType);
return this;
}
/**
*
* Specifies how the ML model data is prepared.
*
*
* If you choose Gzip
and choose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that is a gzip-compressed TAR archive. SageMaker will attempt to
* decompress and untar the object during model deployment.
*
*
* If you choose None
and chooose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that represents an uncompressed ML model to deploy.
*
*
* If you choose None and choose S3Prefix
as the value of S3DataType
, S3Uri
* identifies a key name prefix, under which all objects represents the uncompressed ML model to deploy.
*
*
* If you choose None, then SageMaker will follow rules below when creating model data files under /opt/ml/model
* directory for use by your inference code:
*
*
* -
*
* If you choose S3Object
as the value of S3DataType
, then SageMaker will split the key of
* the S3 object referenced by S3Uri
by slash (/), and use the last part as the filename of the file
* holding the content of the S3 object.
*
*
* -
*
* If you choose S3Prefix
as the value of S3DataType
, then for each S3 object under the
* key name pefix referenced by S3Uri
, SageMaker will trim its key by the prefix, and use the remainder
* as the path (relative to /opt/ml/model
) of the file holding the content of the S3 object. SageMaker
* will split the remainder by slash (/), using intermediate parts as directory names and the last part as filename
* of the file holding the content of the S3 object.
*
*
* -
*
* Do not use any of the following as file names or directory names:
*
*
* -
*
* An empty or blank string
*
*
* -
*
* A string which contains null bytes
*
*
* -
*
* A string longer than 255 bytes
*
*
* -
*
* A single dot (.
)
*
*
* -
*
* A double dot (..
)
*
*
*
*
* -
*
* Ambiguous file names will result in model deployment failure. For example, if your uncompressed ML model consists
* of two S3 objects s3://mybucket/model/weights
and s3://mybucket/model/weights/part1
and
* you specify s3://mybucket/model/
as the value of S3Uri
and S3Prefix
as the
* value of S3DataType
, then it will result in name clash between /opt/ml/model/weights
(a
* regular file) and /opt/ml/model/weights/
(a directory).
*
*
* -
*
* Do not organize the model artifacts in S3 console using folders.
* When you create a folder in S3 console, S3 creates a 0-byte object with a key set to the folder name you provide.
* They key of the 0-byte object ends with a slash (/) which violates SageMaker restrictions on model artifact file
* names, leading to model deployment failure.
*
*
*
*
* @param compressionType
* Specifies how the ML model data is prepared.
*
* If you choose Gzip
and choose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that is a gzip-compressed TAR archive. SageMaker will attempt to
* decompress and untar the object during model deployment.
*
*
* If you choose None
and chooose S3Object
as the value of S3DataType
,
* S3Uri
identifies an object that represents an uncompressed ML model to deploy.
*
*
* If you choose None and choose S3Prefix
as the value of S3DataType
,
* S3Uri
identifies a key name prefix, under which all objects represents the uncompressed ML
* model to deploy.
*
*
* If you choose None, then SageMaker will follow rules below when creating model data files under
* /opt/ml/model directory for use by your inference code:
*
*
* -
*
* If you choose S3Object
as the value of S3DataType
, then SageMaker will split the
* key of the S3 object referenced by S3Uri
by slash (/), and use the last part as the filename
* of the file holding the content of the S3 object.
*
*
* -
*
* If you choose S3Prefix
as the value of S3DataType
, then for each S3 object under
* the key name pefix referenced by S3Uri
, SageMaker will trim its key by the prefix, and use
* the remainder as the path (relative to /opt/ml/model
) of the file holding the content of the
* S3 object. SageMaker will split the remainder by slash (/), using intermediate parts as directory names
* and the last part as filename of the file holding the content of the S3 object.
*
*
* -
*
* Do not use any of the following as file names or directory names:
*
*
* -
*
* An empty or blank string
*
*
* -
*
* A string which contains null bytes
*
*
* -
*
* A string longer than 255 bytes
*
*
* -
*
* A single dot (.
)
*
*
* -
*
* A double dot (..
)
*
*
*
*
* -
*
* Ambiguous file names will result in model deployment failure. For example, if your uncompressed ML model
* consists of two S3 objects s3://mybucket/model/weights
and
* s3://mybucket/model/weights/part1
and you specify s3://mybucket/model/
as the
* value of S3Uri
and S3Prefix
as the value of S3DataType
, then it
* will result in name clash between /opt/ml/model/weights
(a regular file) and
* /opt/ml/model/weights/
(a directory).
*
*
* -
*
* Do not organize the model artifacts in S3 console using
* folders. When you create a folder in S3 console, S3 creates a 0-byte object with a key set to the
* folder name you provide. They key of the 0-byte object ends with a slash (/) which violates SageMaker
* restrictions on model artifact file names, leading to model deployment failure.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ModelCompressionType
*/
public S3ModelDataSource withCompressionType(ModelCompressionType compressionType) {
this.compressionType = compressionType.toString();
return this;
}
/**
*
* Specifies the access configuration file for the ML model. You can explicitly accept the model end-user license
* agreement (EULA) within the ModelAccessConfig
. You are responsible for reviewing and complying with
* any applicable license terms and making sure they are acceptable for your use case before downloading or using a
* model.
*
*
* @param modelAccessConfig
* Specifies the access configuration file for the ML model. You can explicitly accept the model end-user
* license agreement (EULA) within the ModelAccessConfig
. You are responsible for reviewing and
* complying with any applicable license terms and making sure they are acceptable for your use case before
* downloading or using a model.
*/
public void setModelAccessConfig(ModelAccessConfig modelAccessConfig) {
this.modelAccessConfig = modelAccessConfig;
}
/**
*
* Specifies the access configuration file for the ML model. You can explicitly accept the model end-user license
* agreement (EULA) within the ModelAccessConfig
. You are responsible for reviewing and complying with
* any applicable license terms and making sure they are acceptable for your use case before downloading or using a
* model.
*
*
* @return Specifies the access configuration file for the ML model. You can explicitly accept the model end-user
* license agreement (EULA) within the ModelAccessConfig
. You are responsible for reviewing and
* complying with any applicable license terms and making sure they are acceptable for your use case before
* downloading or using a model.
*/
public ModelAccessConfig getModelAccessConfig() {
return this.modelAccessConfig;
}
/**
*
* Specifies the access configuration file for the ML model. You can explicitly accept the model end-user license
* agreement (EULA) within the ModelAccessConfig
. You are responsible for reviewing and complying with
* any applicable license terms and making sure they are acceptable for your use case before downloading or using a
* model.
*
*
* @param modelAccessConfig
* Specifies the access configuration file for the ML model. You can explicitly accept the model end-user
* license agreement (EULA) within the ModelAccessConfig
. You are responsible for reviewing and
* complying with any applicable license terms and making sure they are acceptable for your use case before
* downloading or using a model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3ModelDataSource withModelAccessConfig(ModelAccessConfig modelAccessConfig) {
setModelAccessConfig(modelAccessConfig);
return this;
}
/**
*
* Configuration information for hub access.
*
*
* @param hubAccessConfig
* Configuration information for hub access.
*/
public void setHubAccessConfig(InferenceHubAccessConfig hubAccessConfig) {
this.hubAccessConfig = hubAccessConfig;
}
/**
*
* Configuration information for hub access.
*
*
* @return Configuration information for hub access.
*/
public InferenceHubAccessConfig getHubAccessConfig() {
return this.hubAccessConfig;
}
/**
*
* Configuration information for hub access.
*
*
* @param hubAccessConfig
* Configuration information for hub access.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public S3ModelDataSource withHubAccessConfig(InferenceHubAccessConfig hubAccessConfig) {
setHubAccessConfig(hubAccessConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getS3Uri() != null)
sb.append("S3Uri: ").append(getS3Uri()).append(",");
if (getS3DataType() != null)
sb.append("S3DataType: ").append(getS3DataType()).append(",");
if (getCompressionType() != null)
sb.append("CompressionType: ").append(getCompressionType()).append(",");
if (getModelAccessConfig() != null)
sb.append("ModelAccessConfig: ").append(getModelAccessConfig()).append(",");
if (getHubAccessConfig() != null)
sb.append("HubAccessConfig: ").append(getHubAccessConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof S3ModelDataSource == false)
return false;
S3ModelDataSource other = (S3ModelDataSource) obj;
if (other.getS3Uri() == null ^ this.getS3Uri() == null)
return false;
if (other.getS3Uri() != null && other.getS3Uri().equals(this.getS3Uri()) == false)
return false;
if (other.getS3DataType() == null ^ this.getS3DataType() == null)
return false;
if (other.getS3DataType() != null && other.getS3DataType().equals(this.getS3DataType()) == false)
return false;
if (other.getCompressionType() == null ^ this.getCompressionType() == null)
return false;
if (other.getCompressionType() != null && other.getCompressionType().equals(this.getCompressionType()) == false)
return false;
if (other.getModelAccessConfig() == null ^ this.getModelAccessConfig() == null)
return false;
if (other.getModelAccessConfig() != null && other.getModelAccessConfig().equals(this.getModelAccessConfig()) == false)
return false;
if (other.getHubAccessConfig() == null ^ this.getHubAccessConfig() == null)
return false;
if (other.getHubAccessConfig() != null && other.getHubAccessConfig().equals(this.getHubAccessConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getS3Uri() == null) ? 0 : getS3Uri().hashCode());
hashCode = prime * hashCode + ((getS3DataType() == null) ? 0 : getS3DataType().hashCode());
hashCode = prime * hashCode + ((getCompressionType() == null) ? 0 : getCompressionType().hashCode());
hashCode = prime * hashCode + ((getModelAccessConfig() == null) ? 0 : getModelAccessConfig().hashCode());
hashCode = prime * hashCode + ((getHubAccessConfig() == null) ? 0 : getHubAccessConfig().hashCode());
return hashCode;
}
@Override
public S3ModelDataSource clone() {
try {
return (S3ModelDataSource) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.S3ModelDataSourceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}