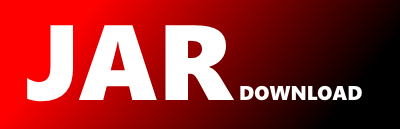
com.amazonaws.services.sagemaker.model.UpdateImageVersionRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateImageVersionRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the image.
*
*/
private String imageName;
/**
*
* The alias of the image version.
*
*/
private String alias;
/**
*
* The version of the image.
*
*/
private Integer version;
/**
*
* A list of aliases to add.
*
*/
private java.util.List aliasesToAdd;
/**
*
* A list of aliases to delete.
*
*/
private java.util.List aliasesToDelete;
/**
*
* The availability of the image version specified by the maintainer.
*
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set to be
* archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are no
* longer actively supported.
*
*
*
*/
private String vendorGuidance;
/**
*
* Indicates SageMaker job type compatibility.
*
*
* -
*
* TRAINING
: The image version is compatible with SageMaker training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker notebook kernels.
*
*
*
*/
private String jobType;
/**
*
* The machine learning framework vended in the image version.
*
*/
private String mLFramework;
/**
*
* The supported programming language and its version.
*
*/
private String programmingLang;
/**
*
* Indicates CPU or GPU compatibility.
*
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
*
*/
private String processor;
/**
*
* Indicates Horovod compatibility.
*
*/
private Boolean horovod;
/**
*
* The maintainer description of the image version.
*
*/
private String releaseNotes;
/**
*
* The name of the image.
*
*
* @param imageName
* The name of the image.
*/
public void setImageName(String imageName) {
this.imageName = imageName;
}
/**
*
* The name of the image.
*
*
* @return The name of the image.
*/
public String getImageName() {
return this.imageName;
}
/**
*
* The name of the image.
*
*
* @param imageName
* The name of the image.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withImageName(String imageName) {
setImageName(imageName);
return this;
}
/**
*
* The alias of the image version.
*
*
* @param alias
* The alias of the image version.
*/
public void setAlias(String alias) {
this.alias = alias;
}
/**
*
* The alias of the image version.
*
*
* @return The alias of the image version.
*/
public String getAlias() {
return this.alias;
}
/**
*
* The alias of the image version.
*
*
* @param alias
* The alias of the image version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withAlias(String alias) {
setAlias(alias);
return this;
}
/**
*
* The version of the image.
*
*
* @param version
* The version of the image.
*/
public void setVersion(Integer version) {
this.version = version;
}
/**
*
* The version of the image.
*
*
* @return The version of the image.
*/
public Integer getVersion() {
return this.version;
}
/**
*
* The version of the image.
*
*
* @param version
* The version of the image.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withVersion(Integer version) {
setVersion(version);
return this;
}
/**
*
* A list of aliases to add.
*
*
* @return A list of aliases to add.
*/
public java.util.List getAliasesToAdd() {
return aliasesToAdd;
}
/**
*
* A list of aliases to add.
*
*
* @param aliasesToAdd
* A list of aliases to add.
*/
public void setAliasesToAdd(java.util.Collection aliasesToAdd) {
if (aliasesToAdd == null) {
this.aliasesToAdd = null;
return;
}
this.aliasesToAdd = new java.util.ArrayList(aliasesToAdd);
}
/**
*
* A list of aliases to add.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAliasesToAdd(java.util.Collection)} or {@link #withAliasesToAdd(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param aliasesToAdd
* A list of aliases to add.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withAliasesToAdd(String... aliasesToAdd) {
if (this.aliasesToAdd == null) {
setAliasesToAdd(new java.util.ArrayList(aliasesToAdd.length));
}
for (String ele : aliasesToAdd) {
this.aliasesToAdd.add(ele);
}
return this;
}
/**
*
* A list of aliases to add.
*
*
* @param aliasesToAdd
* A list of aliases to add.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withAliasesToAdd(java.util.Collection aliasesToAdd) {
setAliasesToAdd(aliasesToAdd);
return this;
}
/**
*
* A list of aliases to delete.
*
*
* @return A list of aliases to delete.
*/
public java.util.List getAliasesToDelete() {
return aliasesToDelete;
}
/**
*
* A list of aliases to delete.
*
*
* @param aliasesToDelete
* A list of aliases to delete.
*/
public void setAliasesToDelete(java.util.Collection aliasesToDelete) {
if (aliasesToDelete == null) {
this.aliasesToDelete = null;
return;
}
this.aliasesToDelete = new java.util.ArrayList(aliasesToDelete);
}
/**
*
* A list of aliases to delete.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAliasesToDelete(java.util.Collection)} or {@link #withAliasesToDelete(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param aliasesToDelete
* A list of aliases to delete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withAliasesToDelete(String... aliasesToDelete) {
if (this.aliasesToDelete == null) {
setAliasesToDelete(new java.util.ArrayList(aliasesToDelete.length));
}
for (String ele : aliasesToDelete) {
this.aliasesToDelete.add(ele);
}
return this;
}
/**
*
* A list of aliases to delete.
*
*
* @param aliasesToDelete
* A list of aliases to delete.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withAliasesToDelete(java.util.Collection aliasesToDelete) {
setAliasesToDelete(aliasesToDelete);
return this;
}
/**
*
* The availability of the image version specified by the maintainer.
*
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set to be
* archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are no
* longer actively supported.
*
*
*
*
* @param vendorGuidance
* The availability of the image version specified by the maintainer.
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set
* to be archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are
* no longer actively supported.
*
*
* @see VendorGuidance
*/
public void setVendorGuidance(String vendorGuidance) {
this.vendorGuidance = vendorGuidance;
}
/**
*
* The availability of the image version specified by the maintainer.
*
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set to be
* archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are no
* longer actively supported.
*
*
*
*
* @return The availability of the image version specified by the maintainer.
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set
* to be archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are
* no longer actively supported.
*
*
* @see VendorGuidance
*/
public String getVendorGuidance() {
return this.vendorGuidance;
}
/**
*
* The availability of the image version specified by the maintainer.
*
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set to be
* archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are no
* longer actively supported.
*
*
*
*
* @param vendorGuidance
* The availability of the image version specified by the maintainer.
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set
* to be archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are
* no longer actively supported.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see VendorGuidance
*/
public UpdateImageVersionRequest withVendorGuidance(String vendorGuidance) {
setVendorGuidance(vendorGuidance);
return this;
}
/**
*
* The availability of the image version specified by the maintainer.
*
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set to be
* archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are no
* longer actively supported.
*
*
*
*
* @param vendorGuidance
* The availability of the image version specified by the maintainer.
*
* -
*
* NOT_PROVIDED
: The maintainers did not provide a status for image version stability.
*
*
* -
*
* STABLE
: The image version is stable.
*
*
* -
*
* TO_BE_ARCHIVED
: The image version is set to be archived. Custom image versions that are set
* to be archived are automatically archived after three months.
*
*
* -
*
* ARCHIVED
: The image version is archived. Archived image versions are not searchable and are
* no longer actively supported.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see VendorGuidance
*/
public UpdateImageVersionRequest withVendorGuidance(VendorGuidance vendorGuidance) {
this.vendorGuidance = vendorGuidance.toString();
return this;
}
/**
*
* Indicates SageMaker job type compatibility.
*
*
* -
*
* TRAINING
: The image version is compatible with SageMaker training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker notebook kernels.
*
*
*
*
* @param jobType
* Indicates SageMaker job type compatibility.
*
* -
*
* TRAINING
: The image version is compatible with SageMaker training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker notebook kernels.
*
*
* @see JobType
*/
public void setJobType(String jobType) {
this.jobType = jobType;
}
/**
*
* Indicates SageMaker job type compatibility.
*
*
* -
*
* TRAINING
: The image version is compatible with SageMaker training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker notebook kernels.
*
*
*
*
* @return Indicates SageMaker job type compatibility.
*
* -
*
* TRAINING
: The image version is compatible with SageMaker training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker notebook kernels.
*
*
* @see JobType
*/
public String getJobType() {
return this.jobType;
}
/**
*
* Indicates SageMaker job type compatibility.
*
*
* -
*
* TRAINING
: The image version is compatible with SageMaker training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker notebook kernels.
*
*
*
*
* @param jobType
* Indicates SageMaker job type compatibility.
*
* -
*
* TRAINING
: The image version is compatible with SageMaker training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker notebook kernels.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobType
*/
public UpdateImageVersionRequest withJobType(String jobType) {
setJobType(jobType);
return this;
}
/**
*
* Indicates SageMaker job type compatibility.
*
*
* -
*
* TRAINING
: The image version is compatible with SageMaker training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker notebook kernels.
*
*
*
*
* @param jobType
* Indicates SageMaker job type compatibility.
*
* -
*
* TRAINING
: The image version is compatible with SageMaker training jobs.
*
*
* -
*
* INFERENCE
: The image version is compatible with SageMaker inference jobs.
*
*
* -
*
* NOTEBOOK_KERNEL
: The image version is compatible with SageMaker notebook kernels.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see JobType
*/
public UpdateImageVersionRequest withJobType(JobType jobType) {
this.jobType = jobType.toString();
return this;
}
/**
*
* The machine learning framework vended in the image version.
*
*
* @param mLFramework
* The machine learning framework vended in the image version.
*/
public void setMLFramework(String mLFramework) {
this.mLFramework = mLFramework;
}
/**
*
* The machine learning framework vended in the image version.
*
*
* @return The machine learning framework vended in the image version.
*/
public String getMLFramework() {
return this.mLFramework;
}
/**
*
* The machine learning framework vended in the image version.
*
*
* @param mLFramework
* The machine learning framework vended in the image version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withMLFramework(String mLFramework) {
setMLFramework(mLFramework);
return this;
}
/**
*
* The supported programming language and its version.
*
*
* @param programmingLang
* The supported programming language and its version.
*/
public void setProgrammingLang(String programmingLang) {
this.programmingLang = programmingLang;
}
/**
*
* The supported programming language and its version.
*
*
* @return The supported programming language and its version.
*/
public String getProgrammingLang() {
return this.programmingLang;
}
/**
*
* The supported programming language and its version.
*
*
* @param programmingLang
* The supported programming language and its version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withProgrammingLang(String programmingLang) {
setProgrammingLang(programmingLang);
return this;
}
/**
*
* Indicates CPU or GPU compatibility.
*
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
*
*
* @param processor
* Indicates CPU or GPU compatibility.
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
* @see Processor
*/
public void setProcessor(String processor) {
this.processor = processor;
}
/**
*
* Indicates CPU or GPU compatibility.
*
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
*
*
* @return Indicates CPU or GPU compatibility.
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
* @see Processor
*/
public String getProcessor() {
return this.processor;
}
/**
*
* Indicates CPU or GPU compatibility.
*
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
*
*
* @param processor
* Indicates CPU or GPU compatibility.
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Processor
*/
public UpdateImageVersionRequest withProcessor(String processor) {
setProcessor(processor);
return this;
}
/**
*
* Indicates CPU or GPU compatibility.
*
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
*
*
* @param processor
* Indicates CPU or GPU compatibility.
*
* -
*
* CPU
: The image version is compatible with CPU.
*
*
* -
*
* GPU
: The image version is compatible with GPU.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Processor
*/
public UpdateImageVersionRequest withProcessor(Processor processor) {
this.processor = processor.toString();
return this;
}
/**
*
* Indicates Horovod compatibility.
*
*
* @param horovod
* Indicates Horovod compatibility.
*/
public void setHorovod(Boolean horovod) {
this.horovod = horovod;
}
/**
*
* Indicates Horovod compatibility.
*
*
* @return Indicates Horovod compatibility.
*/
public Boolean getHorovod() {
return this.horovod;
}
/**
*
* Indicates Horovod compatibility.
*
*
* @param horovod
* Indicates Horovod compatibility.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withHorovod(Boolean horovod) {
setHorovod(horovod);
return this;
}
/**
*
* Indicates Horovod compatibility.
*
*
* @return Indicates Horovod compatibility.
*/
public Boolean isHorovod() {
return this.horovod;
}
/**
*
* The maintainer description of the image version.
*
*
* @param releaseNotes
* The maintainer description of the image version.
*/
public void setReleaseNotes(String releaseNotes) {
this.releaseNotes = releaseNotes;
}
/**
*
* The maintainer description of the image version.
*
*
* @return The maintainer description of the image version.
*/
public String getReleaseNotes() {
return this.releaseNotes;
}
/**
*
* The maintainer description of the image version.
*
*
* @param releaseNotes
* The maintainer description of the image version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateImageVersionRequest withReleaseNotes(String releaseNotes) {
setReleaseNotes(releaseNotes);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getImageName() != null)
sb.append("ImageName: ").append(getImageName()).append(",");
if (getAlias() != null)
sb.append("Alias: ").append(getAlias()).append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion()).append(",");
if (getAliasesToAdd() != null)
sb.append("AliasesToAdd: ").append(getAliasesToAdd()).append(",");
if (getAliasesToDelete() != null)
sb.append("AliasesToDelete: ").append(getAliasesToDelete()).append(",");
if (getVendorGuidance() != null)
sb.append("VendorGuidance: ").append(getVendorGuidance()).append(",");
if (getJobType() != null)
sb.append("JobType: ").append(getJobType()).append(",");
if (getMLFramework() != null)
sb.append("MLFramework: ").append(getMLFramework()).append(",");
if (getProgrammingLang() != null)
sb.append("ProgrammingLang: ").append(getProgrammingLang()).append(",");
if (getProcessor() != null)
sb.append("Processor: ").append(getProcessor()).append(",");
if (getHorovod() != null)
sb.append("Horovod: ").append(getHorovod()).append(",");
if (getReleaseNotes() != null)
sb.append("ReleaseNotes: ").append(getReleaseNotes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateImageVersionRequest == false)
return false;
UpdateImageVersionRequest other = (UpdateImageVersionRequest) obj;
if (other.getImageName() == null ^ this.getImageName() == null)
return false;
if (other.getImageName() != null && other.getImageName().equals(this.getImageName()) == false)
return false;
if (other.getAlias() == null ^ this.getAlias() == null)
return false;
if (other.getAlias() != null && other.getAlias().equals(this.getAlias()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
if (other.getAliasesToAdd() == null ^ this.getAliasesToAdd() == null)
return false;
if (other.getAliasesToAdd() != null && other.getAliasesToAdd().equals(this.getAliasesToAdd()) == false)
return false;
if (other.getAliasesToDelete() == null ^ this.getAliasesToDelete() == null)
return false;
if (other.getAliasesToDelete() != null && other.getAliasesToDelete().equals(this.getAliasesToDelete()) == false)
return false;
if (other.getVendorGuidance() == null ^ this.getVendorGuidance() == null)
return false;
if (other.getVendorGuidance() != null && other.getVendorGuidance().equals(this.getVendorGuidance()) == false)
return false;
if (other.getJobType() == null ^ this.getJobType() == null)
return false;
if (other.getJobType() != null && other.getJobType().equals(this.getJobType()) == false)
return false;
if (other.getMLFramework() == null ^ this.getMLFramework() == null)
return false;
if (other.getMLFramework() != null && other.getMLFramework().equals(this.getMLFramework()) == false)
return false;
if (other.getProgrammingLang() == null ^ this.getProgrammingLang() == null)
return false;
if (other.getProgrammingLang() != null && other.getProgrammingLang().equals(this.getProgrammingLang()) == false)
return false;
if (other.getProcessor() == null ^ this.getProcessor() == null)
return false;
if (other.getProcessor() != null && other.getProcessor().equals(this.getProcessor()) == false)
return false;
if (other.getHorovod() == null ^ this.getHorovod() == null)
return false;
if (other.getHorovod() != null && other.getHorovod().equals(this.getHorovod()) == false)
return false;
if (other.getReleaseNotes() == null ^ this.getReleaseNotes() == null)
return false;
if (other.getReleaseNotes() != null && other.getReleaseNotes().equals(this.getReleaseNotes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getImageName() == null) ? 0 : getImageName().hashCode());
hashCode = prime * hashCode + ((getAlias() == null) ? 0 : getAlias().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
hashCode = prime * hashCode + ((getAliasesToAdd() == null) ? 0 : getAliasesToAdd().hashCode());
hashCode = prime * hashCode + ((getAliasesToDelete() == null) ? 0 : getAliasesToDelete().hashCode());
hashCode = prime * hashCode + ((getVendorGuidance() == null) ? 0 : getVendorGuidance().hashCode());
hashCode = prime * hashCode + ((getJobType() == null) ? 0 : getJobType().hashCode());
hashCode = prime * hashCode + ((getMLFramework() == null) ? 0 : getMLFramework().hashCode());
hashCode = prime * hashCode + ((getProgrammingLang() == null) ? 0 : getProgrammingLang().hashCode());
hashCode = prime * hashCode + ((getProcessor() == null) ? 0 : getProcessor().hashCode());
hashCode = prime * hashCode + ((getHorovod() == null) ? 0 : getHorovod().hashCode());
hashCode = prime * hashCode + ((getReleaseNotes() == null) ? 0 : getReleaseNotes().hashCode());
return hashCode;
}
@Override
public UpdateImageVersionRequest clone() {
return (UpdateImageVersionRequest) super.clone();
}
}