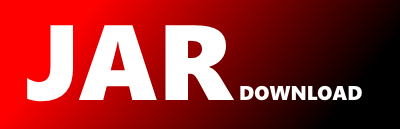
com.amazonaws.services.sagemaker.model.UpdateNotebookInstanceRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateNotebookInstanceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the notebook instance to update.
*
*/
private String notebookInstanceName;
/**
*
* The Amazon ML compute instance type.
*
*/
private String instanceType;
/**
*
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access the notebook instance. For
* more information, see SageMaker
* Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*/
private String roleArn;
/**
*
* The name of a lifecycle configuration to associate with the notebook instance. For information about lifestyle
* configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance.
*
*/
private String lifecycleConfigName;
/**
*
* Set to true
to remove the notebook instance lifecycle configuration currently associated with the
* notebook instance. This operation is idempotent. If you specify a lifecycle configuration that is not associated
* with the notebook instance when you call this method, it does not throw an error.
*
*/
private Boolean disassociateLifecycleConfig;
/**
*
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB. ML
* storage volumes are encrypted, so SageMaker can't determine the amount of available free space on the volume.
* Because of this, you can increase the volume size when you update a notebook instance, but you can't decrease the
* volume size. If you want to decrease the size of the ML storage volume in use, create a new notebook instance
* with the desired size.
*
*/
private Integer volumeSizeInGB;
/**
*
* The Git repository to associate with the notebook instance as its default code repository. This can be either the
* name of a Git repository stored as a resource in your account, or the URL of a Git repository in Amazon Web Services CodeCommit or
* in any other Git repository. When you open a notebook instance, it opens in the directory that contains this
* repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*/
private String defaultCodeRepository;
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*/
private java.util.List additionalCodeRepositories;
/**
*
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently only one
* EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*/
private java.util.List acceleratorTypes;
/**
*
* A list of the Elastic Inference (EI) instance types to remove from this notebook instance. This operation is
* idempotent. If you specify an accelerator type that is not associated with the notebook instance when you call
* this method, it does not throw an error.
*
*/
private Boolean disassociateAcceleratorTypes;
/**
*
* The name or URL of the default Git repository to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*/
private Boolean disassociateDefaultCodeRepository;
/**
*
* A list of names or URLs of the default Git repositories to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*/
private Boolean disassociateAdditionalCodeRepositories;
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* If you set this to Disabled
, users don't have root access on the notebook instance, but lifecycle
* configuration scripts still run with root permissions.
*
*
*/
private String rootAccess;
/**
*
* Information on the IMDS configuration of the notebook instance
*
*/
private InstanceMetadataServiceConfiguration instanceMetadataServiceConfiguration;
/**
*
* The name of the notebook instance to update.
*
*
* @param notebookInstanceName
* The name of the notebook instance to update.
*/
public void setNotebookInstanceName(String notebookInstanceName) {
this.notebookInstanceName = notebookInstanceName;
}
/**
*
* The name of the notebook instance to update.
*
*
* @return The name of the notebook instance to update.
*/
public String getNotebookInstanceName() {
return this.notebookInstanceName;
}
/**
*
* The name of the notebook instance to update.
*
*
* @param notebookInstanceName
* The name of the notebook instance to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withNotebookInstanceName(String notebookInstanceName) {
setNotebookInstanceName(notebookInstanceName);
return this;
}
/**
*
* The Amazon ML compute instance type.
*
*
* @param instanceType
* The Amazon ML compute instance type.
* @see InstanceType
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
*
* The Amazon ML compute instance type.
*
*
* @return The Amazon ML compute instance type.
* @see InstanceType
*/
public String getInstanceType() {
return this.instanceType;
}
/**
*
* The Amazon ML compute instance type.
*
*
* @param instanceType
* The Amazon ML compute instance type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public UpdateNotebookInstanceRequest withInstanceType(String instanceType) {
setInstanceType(instanceType);
return this;
}
/**
*
* The Amazon ML compute instance type.
*
*
* @param instanceType
* The Amazon ML compute instance type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public UpdateNotebookInstanceRequest withInstanceType(InstanceType instanceType) {
this.instanceType = instanceType.toString();
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access the notebook instance. For
* more information, see SageMaker
* Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access the notebook instance.
* For more information, see SageMaker Roles.
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access the notebook instance. For
* more information, see SageMaker
* Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*
* @return The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access the notebook instance.
* For more information, see SageMaker Roles.
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access the notebook instance. For
* more information, see SageMaker
* Roles.
*
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role that SageMaker can assume to access the notebook instance.
* For more information, see SageMaker Roles.
*
*
* To be able to pass this role to SageMaker, the caller of this API must have the iam:PassRole
* permission.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The name of a lifecycle configuration to associate with the notebook instance. For information about lifestyle
* configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance.
*
*
* @param lifecycleConfigName
* The name of a lifecycle configuration to associate with the notebook instance. For information about
* lifestyle configurations, see Step 2.1: (Optional)
* Customize a Notebook Instance.
*/
public void setLifecycleConfigName(String lifecycleConfigName) {
this.lifecycleConfigName = lifecycleConfigName;
}
/**
*
* The name of a lifecycle configuration to associate with the notebook instance. For information about lifestyle
* configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance.
*
*
* @return The name of a lifecycle configuration to associate with the notebook instance. For information about
* lifestyle configurations, see Step 2.1:
* (Optional) Customize a Notebook Instance.
*/
public String getLifecycleConfigName() {
return this.lifecycleConfigName;
}
/**
*
* The name of a lifecycle configuration to associate with the notebook instance. For information about lifestyle
* configurations, see Step
* 2.1: (Optional) Customize a Notebook Instance.
*
*
* @param lifecycleConfigName
* The name of a lifecycle configuration to associate with the notebook instance. For information about
* lifestyle configurations, see Step 2.1: (Optional)
* Customize a Notebook Instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withLifecycleConfigName(String lifecycleConfigName) {
setLifecycleConfigName(lifecycleConfigName);
return this;
}
/**
*
* Set to true
to remove the notebook instance lifecycle configuration currently associated with the
* notebook instance. This operation is idempotent. If you specify a lifecycle configuration that is not associated
* with the notebook instance when you call this method, it does not throw an error.
*
*
* @param disassociateLifecycleConfig
* Set to true
to remove the notebook instance lifecycle configuration currently associated with
* the notebook instance. This operation is idempotent. If you specify a lifecycle configuration that is not
* associated with the notebook instance when you call this method, it does not throw an error.
*/
public void setDisassociateLifecycleConfig(Boolean disassociateLifecycleConfig) {
this.disassociateLifecycleConfig = disassociateLifecycleConfig;
}
/**
*
* Set to true
to remove the notebook instance lifecycle configuration currently associated with the
* notebook instance. This operation is idempotent. If you specify a lifecycle configuration that is not associated
* with the notebook instance when you call this method, it does not throw an error.
*
*
* @return Set to true
to remove the notebook instance lifecycle configuration currently associated
* with the notebook instance. This operation is idempotent. If you specify a lifecycle configuration that
* is not associated with the notebook instance when you call this method, it does not throw an error.
*/
public Boolean getDisassociateLifecycleConfig() {
return this.disassociateLifecycleConfig;
}
/**
*
* Set to true
to remove the notebook instance lifecycle configuration currently associated with the
* notebook instance. This operation is idempotent. If you specify a lifecycle configuration that is not associated
* with the notebook instance when you call this method, it does not throw an error.
*
*
* @param disassociateLifecycleConfig
* Set to true
to remove the notebook instance lifecycle configuration currently associated with
* the notebook instance. This operation is idempotent. If you specify a lifecycle configuration that is not
* associated with the notebook instance when you call this method, it does not throw an error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withDisassociateLifecycleConfig(Boolean disassociateLifecycleConfig) {
setDisassociateLifecycleConfig(disassociateLifecycleConfig);
return this;
}
/**
*
* Set to true
to remove the notebook instance lifecycle configuration currently associated with the
* notebook instance. This operation is idempotent. If you specify a lifecycle configuration that is not associated
* with the notebook instance when you call this method, it does not throw an error.
*
*
* @return Set to true
to remove the notebook instance lifecycle configuration currently associated
* with the notebook instance. This operation is idempotent. If you specify a lifecycle configuration that
* is not associated with the notebook instance when you call this method, it does not throw an error.
*/
public Boolean isDisassociateLifecycleConfig() {
return this.disassociateLifecycleConfig;
}
/**
*
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB. ML
* storage volumes are encrypted, so SageMaker can't determine the amount of available free space on the volume.
* Because of this, you can increase the volume size when you update a notebook instance, but you can't decrease the
* volume size. If you want to decrease the size of the ML storage volume in use, create a new notebook instance
* with the desired size.
*
*
* @param volumeSizeInGB
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
* ML storage volumes are encrypted, so SageMaker can't determine the amount of available free space on the
* volume. Because of this, you can increase the volume size when you update a notebook instance, but you
* can't decrease the volume size. If you want to decrease the size of the ML storage volume in use, create a
* new notebook instance with the desired size.
*/
public void setVolumeSizeInGB(Integer volumeSizeInGB) {
this.volumeSizeInGB = volumeSizeInGB;
}
/**
*
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB. ML
* storage volumes are encrypted, so SageMaker can't determine the amount of available free space on the volume.
* Because of this, you can increase the volume size when you update a notebook instance, but you can't decrease the
* volume size. If you want to decrease the size of the ML storage volume in use, create a new notebook instance
* with the desired size.
*
*
* @return The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
* ML storage volumes are encrypted, so SageMaker can't determine the amount of available free space on the
* volume. Because of this, you can increase the volume size when you update a notebook instance, but you
* can't decrease the volume size. If you want to decrease the size of the ML storage volume in use, create
* a new notebook instance with the desired size.
*/
public Integer getVolumeSizeInGB() {
return this.volumeSizeInGB;
}
/**
*
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB. ML
* storage volumes are encrypted, so SageMaker can't determine the amount of available free space on the volume.
* Because of this, you can increase the volume size when you update a notebook instance, but you can't decrease the
* volume size. If you want to decrease the size of the ML storage volume in use, create a new notebook instance
* with the desired size.
*
*
* @param volumeSizeInGB
* The size, in GB, of the ML storage volume to attach to the notebook instance. The default value is 5 GB.
* ML storage volumes are encrypted, so SageMaker can't determine the amount of available free space on the
* volume. Because of this, you can increase the volume size when you update a notebook instance, but you
* can't decrease the volume size. If you want to decrease the size of the ML storage volume in use, create a
* new notebook instance with the desired size.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withVolumeSizeInGB(Integer volumeSizeInGB) {
setVolumeSizeInGB(volumeSizeInGB);
return this;
}
/**
*
* The Git repository to associate with the notebook instance as its default code repository. This can be either the
* name of a Git repository stored as a resource in your account, or the URL of a Git repository in Amazon Web Services CodeCommit or
* in any other Git repository. When you open a notebook instance, it opens in the directory that contains this
* repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @param defaultCodeRepository
* The Git repository to associate with the notebook instance as its default code repository. This can be
* either the name of a Git repository stored as a resource in your account, or the URL of a Git repository
* in Amazon Web Services
* CodeCommit or in any other Git repository. When you open a notebook instance, it opens in the
* directory that contains this repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*/
public void setDefaultCodeRepository(String defaultCodeRepository) {
this.defaultCodeRepository = defaultCodeRepository;
}
/**
*
* The Git repository to associate with the notebook instance as its default code repository. This can be either the
* name of a Git repository stored as a resource in your account, or the URL of a Git repository in Amazon Web Services CodeCommit or
* in any other Git repository. When you open a notebook instance, it opens in the directory that contains this
* repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @return The Git repository to associate with the notebook instance as its default code repository. This can be
* either the name of a Git repository stored as a resource in your account, or the URL of a Git repository
* in Amazon Web Services
* CodeCommit or in any other Git repository. When you open a notebook instance, it opens in the
* directory that contains this repository. For more information, see Associating Git Repositories
* with SageMaker Notebook Instances.
*/
public String getDefaultCodeRepository() {
return this.defaultCodeRepository;
}
/**
*
* The Git repository to associate with the notebook instance as its default code repository. This can be either the
* name of a Git repository stored as a resource in your account, or the URL of a Git repository in Amazon Web Services CodeCommit or
* in any other Git repository. When you open a notebook instance, it opens in the directory that contains this
* repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @param defaultCodeRepository
* The Git repository to associate with the notebook instance as its default code repository. This can be
* either the name of a Git repository stored as a resource in your account, or the URL of a Git repository
* in Amazon Web Services
* CodeCommit or in any other Git repository. When you open a notebook instance, it opens in the
* directory that contains this repository. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withDefaultCodeRepository(String defaultCodeRepository) {
setDefaultCodeRepository(defaultCodeRepository);
return this;
}
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @return An array of up to three Git repositories to associate with the notebook instance. These can be either the
* names of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services
* CodeCommit or in any other Git repository. These repositories are cloned at the same level as the
* default repository of your notebook instance. For more information, see Associating Git Repositories
* with SageMaker Notebook Instances.
*/
public java.util.List getAdditionalCodeRepositories() {
return additionalCodeRepositories;
}
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @param additionalCodeRepositories
* An array of up to three Git repositories to associate with the notebook instance. These can be either the
* names of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services
* CodeCommit or in any other Git repository. These repositories are cloned at the same level as the
* default repository of your notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*/
public void setAdditionalCodeRepositories(java.util.Collection additionalCodeRepositories) {
if (additionalCodeRepositories == null) {
this.additionalCodeRepositories = null;
return;
}
this.additionalCodeRepositories = new java.util.ArrayList(additionalCodeRepositories);
}
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAdditionalCodeRepositories(java.util.Collection)} or
* {@link #withAdditionalCodeRepositories(java.util.Collection)} if you want to override the existing values.
*
*
* @param additionalCodeRepositories
* An array of up to three Git repositories to associate with the notebook instance. These can be either the
* names of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services
* CodeCommit or in any other Git repository. These repositories are cloned at the same level as the
* default repository of your notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withAdditionalCodeRepositories(String... additionalCodeRepositories) {
if (this.additionalCodeRepositories == null) {
setAdditionalCodeRepositories(new java.util.ArrayList(additionalCodeRepositories.length));
}
for (String ele : additionalCodeRepositories) {
this.additionalCodeRepositories.add(ele);
}
return this;
}
/**
*
* An array of up to three Git repositories to associate with the notebook instance. These can be either the names
* of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services CodeCommit or
* in any other Git repository. These repositories are cloned at the same level as the default repository of your
* notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
*
*
* @param additionalCodeRepositories
* An array of up to three Git repositories to associate with the notebook instance. These can be either the
* names of Git repositories stored as resources in your account, or the URL of Git repositories in Amazon Web Services
* CodeCommit or in any other Git repository. These repositories are cloned at the same level as the
* default repository of your notebook instance. For more information, see Associating Git Repositories with
* SageMaker Notebook Instances.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withAdditionalCodeRepositories(java.util.Collection additionalCodeRepositories) {
setAdditionalCodeRepositories(additionalCodeRepositories);
return this;
}
/**
*
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently only one
* EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @return A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently
* only one EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see NotebookInstanceAcceleratorType
*/
public java.util.List getAcceleratorTypes() {
return acceleratorTypes;
}
/**
*
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently only one
* EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorTypes
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently
* only one EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @see NotebookInstanceAcceleratorType
*/
public void setAcceleratorTypes(java.util.Collection acceleratorTypes) {
if (acceleratorTypes == null) {
this.acceleratorTypes = null;
return;
}
this.acceleratorTypes = new java.util.ArrayList(acceleratorTypes);
}
/**
*
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently only one
* EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAcceleratorTypes(java.util.Collection)} or {@link #withAcceleratorTypes(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param acceleratorTypes
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently
* only one EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotebookInstanceAcceleratorType
*/
public UpdateNotebookInstanceRequest withAcceleratorTypes(String... acceleratorTypes) {
if (this.acceleratorTypes == null) {
setAcceleratorTypes(new java.util.ArrayList(acceleratorTypes.length));
}
for (String ele : acceleratorTypes) {
this.acceleratorTypes.add(ele);
}
return this;
}
/**
*
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently only one
* EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorTypes
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently
* only one EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotebookInstanceAcceleratorType
*/
public UpdateNotebookInstanceRequest withAcceleratorTypes(java.util.Collection acceleratorTypes) {
setAcceleratorTypes(acceleratorTypes);
return this;
}
/**
*
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently only one
* EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon SageMaker.
*
*
* @param acceleratorTypes
* A list of the Elastic Inference (EI) instance types to associate with this notebook instance. Currently
* only one EI instance type can be associated with a notebook instance. For more information, see Using Elastic Inference in Amazon
* SageMaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NotebookInstanceAcceleratorType
*/
public UpdateNotebookInstanceRequest withAcceleratorTypes(NotebookInstanceAcceleratorType... acceleratorTypes) {
java.util.ArrayList acceleratorTypesCopy = new java.util.ArrayList(acceleratorTypes.length);
for (NotebookInstanceAcceleratorType value : acceleratorTypes) {
acceleratorTypesCopy.add(value.toString());
}
if (getAcceleratorTypes() == null) {
setAcceleratorTypes(acceleratorTypesCopy);
} else {
getAcceleratorTypes().addAll(acceleratorTypesCopy);
}
return this;
}
/**
*
* A list of the Elastic Inference (EI) instance types to remove from this notebook instance. This operation is
* idempotent. If you specify an accelerator type that is not associated with the notebook instance when you call
* this method, it does not throw an error.
*
*
* @param disassociateAcceleratorTypes
* A list of the Elastic Inference (EI) instance types to remove from this notebook instance. This operation
* is idempotent. If you specify an accelerator type that is not associated with the notebook instance when
* you call this method, it does not throw an error.
*/
public void setDisassociateAcceleratorTypes(Boolean disassociateAcceleratorTypes) {
this.disassociateAcceleratorTypes = disassociateAcceleratorTypes;
}
/**
*
* A list of the Elastic Inference (EI) instance types to remove from this notebook instance. This operation is
* idempotent. If you specify an accelerator type that is not associated with the notebook instance when you call
* this method, it does not throw an error.
*
*
* @return A list of the Elastic Inference (EI) instance types to remove from this notebook instance. This operation
* is idempotent. If you specify an accelerator type that is not associated with the notebook instance when
* you call this method, it does not throw an error.
*/
public Boolean getDisassociateAcceleratorTypes() {
return this.disassociateAcceleratorTypes;
}
/**
*
* A list of the Elastic Inference (EI) instance types to remove from this notebook instance. This operation is
* idempotent. If you specify an accelerator type that is not associated with the notebook instance when you call
* this method, it does not throw an error.
*
*
* @param disassociateAcceleratorTypes
* A list of the Elastic Inference (EI) instance types to remove from this notebook instance. This operation
* is idempotent. If you specify an accelerator type that is not associated with the notebook instance when
* you call this method, it does not throw an error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withDisassociateAcceleratorTypes(Boolean disassociateAcceleratorTypes) {
setDisassociateAcceleratorTypes(disassociateAcceleratorTypes);
return this;
}
/**
*
* A list of the Elastic Inference (EI) instance types to remove from this notebook instance. This operation is
* idempotent. If you specify an accelerator type that is not associated with the notebook instance when you call
* this method, it does not throw an error.
*
*
* @return A list of the Elastic Inference (EI) instance types to remove from this notebook instance. This operation
* is idempotent. If you specify an accelerator type that is not associated with the notebook instance when
* you call this method, it does not throw an error.
*/
public Boolean isDisassociateAcceleratorTypes() {
return this.disassociateAcceleratorTypes;
}
/**
*
* The name or URL of the default Git repository to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*
* @param disassociateDefaultCodeRepository
* The name or URL of the default Git repository to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you
* call this method, it does not throw an error.
*/
public void setDisassociateDefaultCodeRepository(Boolean disassociateDefaultCodeRepository) {
this.disassociateDefaultCodeRepository = disassociateDefaultCodeRepository;
}
/**
*
* The name or URL of the default Git repository to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*
* @return The name or URL of the default Git repository to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you
* call this method, it does not throw an error.
*/
public Boolean getDisassociateDefaultCodeRepository() {
return this.disassociateDefaultCodeRepository;
}
/**
*
* The name or URL of the default Git repository to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*
* @param disassociateDefaultCodeRepository
* The name or URL of the default Git repository to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you
* call this method, it does not throw an error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withDisassociateDefaultCodeRepository(Boolean disassociateDefaultCodeRepository) {
setDisassociateDefaultCodeRepository(disassociateDefaultCodeRepository);
return this;
}
/**
*
* The name or URL of the default Git repository to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*
* @return The name or URL of the default Git repository to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you
* call this method, it does not throw an error.
*/
public Boolean isDisassociateDefaultCodeRepository() {
return this.disassociateDefaultCodeRepository;
}
/**
*
* A list of names or URLs of the default Git repositories to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*
* @param disassociateAdditionalCodeRepositories
* A list of names or URLs of the default Git repositories to remove from this notebook instance. This
* operation is idempotent. If you specify a Git repository that is not associated with the notebook instance
* when you call this method, it does not throw an error.
*/
public void setDisassociateAdditionalCodeRepositories(Boolean disassociateAdditionalCodeRepositories) {
this.disassociateAdditionalCodeRepositories = disassociateAdditionalCodeRepositories;
}
/**
*
* A list of names or URLs of the default Git repositories to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*
* @return A list of names or URLs of the default Git repositories to remove from this notebook instance. This
* operation is idempotent. If you specify a Git repository that is not associated with the notebook
* instance when you call this method, it does not throw an error.
*/
public Boolean getDisassociateAdditionalCodeRepositories() {
return this.disassociateAdditionalCodeRepositories;
}
/**
*
* A list of names or URLs of the default Git repositories to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*
* @param disassociateAdditionalCodeRepositories
* A list of names or URLs of the default Git repositories to remove from this notebook instance. This
* operation is idempotent. If you specify a Git repository that is not associated with the notebook instance
* when you call this method, it does not throw an error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withDisassociateAdditionalCodeRepositories(Boolean disassociateAdditionalCodeRepositories) {
setDisassociateAdditionalCodeRepositories(disassociateAdditionalCodeRepositories);
return this;
}
/**
*
* A list of names or URLs of the default Git repositories to remove from this notebook instance. This operation is
* idempotent. If you specify a Git repository that is not associated with the notebook instance when you call this
* method, it does not throw an error.
*
*
* @return A list of names or URLs of the default Git repositories to remove from this notebook instance. This
* operation is idempotent. If you specify a Git repository that is not associated with the notebook
* instance when you call this method, it does not throw an error.
*/
public Boolean isDisassociateAdditionalCodeRepositories() {
return this.disassociateAdditionalCodeRepositories;
}
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* If you set this to Disabled
, users don't have root access on the notebook instance, but lifecycle
* configuration scripts still run with root permissions.
*
*
*
* @param rootAccess
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
* If you set this to Disabled
, users don't have root access on the notebook instance, but
* lifecycle configuration scripts still run with root permissions.
*
* @see RootAccess
*/
public void setRootAccess(String rootAccess) {
this.rootAccess = rootAccess;
}
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* If you set this to Disabled
, users don't have root access on the notebook instance, but lifecycle
* configuration scripts still run with root permissions.
*
*
*
* @return Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
* If you set this to Disabled
, users don't have root access on the notebook instance, but
* lifecycle configuration scripts still run with root permissions.
*
* @see RootAccess
*/
public String getRootAccess() {
return this.rootAccess;
}
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* If you set this to Disabled
, users don't have root access on the notebook instance, but lifecycle
* configuration scripts still run with root permissions.
*
*
*
* @param rootAccess
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
* If you set this to Disabled
, users don't have root access on the notebook instance, but
* lifecycle configuration scripts still run with root permissions.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RootAccess
*/
public UpdateNotebookInstanceRequest withRootAccess(String rootAccess) {
setRootAccess(rootAccess);
return this;
}
/**
*
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
*
*
* If you set this to Disabled
, users don't have root access on the notebook instance, but lifecycle
* configuration scripts still run with root permissions.
*
*
*
* @param rootAccess
* Whether root access is enabled or disabled for users of the notebook instance. The default value is
* Enabled
.
*
* If you set this to Disabled
, users don't have root access on the notebook instance, but
* lifecycle configuration scripts still run with root permissions.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see RootAccess
*/
public UpdateNotebookInstanceRequest withRootAccess(RootAccess rootAccess) {
this.rootAccess = rootAccess.toString();
return this;
}
/**
*
* Information on the IMDS configuration of the notebook instance
*
*
* @param instanceMetadataServiceConfiguration
* Information on the IMDS configuration of the notebook instance
*/
public void setInstanceMetadataServiceConfiguration(InstanceMetadataServiceConfiguration instanceMetadataServiceConfiguration) {
this.instanceMetadataServiceConfiguration = instanceMetadataServiceConfiguration;
}
/**
*
* Information on the IMDS configuration of the notebook instance
*
*
* @return Information on the IMDS configuration of the notebook instance
*/
public InstanceMetadataServiceConfiguration getInstanceMetadataServiceConfiguration() {
return this.instanceMetadataServiceConfiguration;
}
/**
*
* Information on the IMDS configuration of the notebook instance
*
*
* @param instanceMetadataServiceConfiguration
* Information on the IMDS configuration of the notebook instance
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateNotebookInstanceRequest withInstanceMetadataServiceConfiguration(InstanceMetadataServiceConfiguration instanceMetadataServiceConfiguration) {
setInstanceMetadataServiceConfiguration(instanceMetadataServiceConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getNotebookInstanceName() != null)
sb.append("NotebookInstanceName: ").append(getNotebookInstanceName()).append(",");
if (getInstanceType() != null)
sb.append("InstanceType: ").append(getInstanceType()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getLifecycleConfigName() != null)
sb.append("LifecycleConfigName: ").append(getLifecycleConfigName()).append(",");
if (getDisassociateLifecycleConfig() != null)
sb.append("DisassociateLifecycleConfig: ").append(getDisassociateLifecycleConfig()).append(",");
if (getVolumeSizeInGB() != null)
sb.append("VolumeSizeInGB: ").append(getVolumeSizeInGB()).append(",");
if (getDefaultCodeRepository() != null)
sb.append("DefaultCodeRepository: ").append(getDefaultCodeRepository()).append(",");
if (getAdditionalCodeRepositories() != null)
sb.append("AdditionalCodeRepositories: ").append(getAdditionalCodeRepositories()).append(",");
if (getAcceleratorTypes() != null)
sb.append("AcceleratorTypes: ").append(getAcceleratorTypes()).append(",");
if (getDisassociateAcceleratorTypes() != null)
sb.append("DisassociateAcceleratorTypes: ").append(getDisassociateAcceleratorTypes()).append(",");
if (getDisassociateDefaultCodeRepository() != null)
sb.append("DisassociateDefaultCodeRepository: ").append(getDisassociateDefaultCodeRepository()).append(",");
if (getDisassociateAdditionalCodeRepositories() != null)
sb.append("DisassociateAdditionalCodeRepositories: ").append(getDisassociateAdditionalCodeRepositories()).append(",");
if (getRootAccess() != null)
sb.append("RootAccess: ").append(getRootAccess()).append(",");
if (getInstanceMetadataServiceConfiguration() != null)
sb.append("InstanceMetadataServiceConfiguration: ").append(getInstanceMetadataServiceConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateNotebookInstanceRequest == false)
return false;
UpdateNotebookInstanceRequest other = (UpdateNotebookInstanceRequest) obj;
if (other.getNotebookInstanceName() == null ^ this.getNotebookInstanceName() == null)
return false;
if (other.getNotebookInstanceName() != null && other.getNotebookInstanceName().equals(this.getNotebookInstanceName()) == false)
return false;
if (other.getInstanceType() == null ^ this.getInstanceType() == null)
return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getLifecycleConfigName() == null ^ this.getLifecycleConfigName() == null)
return false;
if (other.getLifecycleConfigName() != null && other.getLifecycleConfigName().equals(this.getLifecycleConfigName()) == false)
return false;
if (other.getDisassociateLifecycleConfig() == null ^ this.getDisassociateLifecycleConfig() == null)
return false;
if (other.getDisassociateLifecycleConfig() != null && other.getDisassociateLifecycleConfig().equals(this.getDisassociateLifecycleConfig()) == false)
return false;
if (other.getVolumeSizeInGB() == null ^ this.getVolumeSizeInGB() == null)
return false;
if (other.getVolumeSizeInGB() != null && other.getVolumeSizeInGB().equals(this.getVolumeSizeInGB()) == false)
return false;
if (other.getDefaultCodeRepository() == null ^ this.getDefaultCodeRepository() == null)
return false;
if (other.getDefaultCodeRepository() != null && other.getDefaultCodeRepository().equals(this.getDefaultCodeRepository()) == false)
return false;
if (other.getAdditionalCodeRepositories() == null ^ this.getAdditionalCodeRepositories() == null)
return false;
if (other.getAdditionalCodeRepositories() != null && other.getAdditionalCodeRepositories().equals(this.getAdditionalCodeRepositories()) == false)
return false;
if (other.getAcceleratorTypes() == null ^ this.getAcceleratorTypes() == null)
return false;
if (other.getAcceleratorTypes() != null && other.getAcceleratorTypes().equals(this.getAcceleratorTypes()) == false)
return false;
if (other.getDisassociateAcceleratorTypes() == null ^ this.getDisassociateAcceleratorTypes() == null)
return false;
if (other.getDisassociateAcceleratorTypes() != null && other.getDisassociateAcceleratorTypes().equals(this.getDisassociateAcceleratorTypes()) == false)
return false;
if (other.getDisassociateDefaultCodeRepository() == null ^ this.getDisassociateDefaultCodeRepository() == null)
return false;
if (other.getDisassociateDefaultCodeRepository() != null
&& other.getDisassociateDefaultCodeRepository().equals(this.getDisassociateDefaultCodeRepository()) == false)
return false;
if (other.getDisassociateAdditionalCodeRepositories() == null ^ this.getDisassociateAdditionalCodeRepositories() == null)
return false;
if (other.getDisassociateAdditionalCodeRepositories() != null
&& other.getDisassociateAdditionalCodeRepositories().equals(this.getDisassociateAdditionalCodeRepositories()) == false)
return false;
if (other.getRootAccess() == null ^ this.getRootAccess() == null)
return false;
if (other.getRootAccess() != null && other.getRootAccess().equals(this.getRootAccess()) == false)
return false;
if (other.getInstanceMetadataServiceConfiguration() == null ^ this.getInstanceMetadataServiceConfiguration() == null)
return false;
if (other.getInstanceMetadataServiceConfiguration() != null
&& other.getInstanceMetadataServiceConfiguration().equals(this.getInstanceMetadataServiceConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getNotebookInstanceName() == null) ? 0 : getNotebookInstanceName().hashCode());
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getLifecycleConfigName() == null) ? 0 : getLifecycleConfigName().hashCode());
hashCode = prime * hashCode + ((getDisassociateLifecycleConfig() == null) ? 0 : getDisassociateLifecycleConfig().hashCode());
hashCode = prime * hashCode + ((getVolumeSizeInGB() == null) ? 0 : getVolumeSizeInGB().hashCode());
hashCode = prime * hashCode + ((getDefaultCodeRepository() == null) ? 0 : getDefaultCodeRepository().hashCode());
hashCode = prime * hashCode + ((getAdditionalCodeRepositories() == null) ? 0 : getAdditionalCodeRepositories().hashCode());
hashCode = prime * hashCode + ((getAcceleratorTypes() == null) ? 0 : getAcceleratorTypes().hashCode());
hashCode = prime * hashCode + ((getDisassociateAcceleratorTypes() == null) ? 0 : getDisassociateAcceleratorTypes().hashCode());
hashCode = prime * hashCode + ((getDisassociateDefaultCodeRepository() == null) ? 0 : getDisassociateDefaultCodeRepository().hashCode());
hashCode = prime * hashCode + ((getDisassociateAdditionalCodeRepositories() == null) ? 0 : getDisassociateAdditionalCodeRepositories().hashCode());
hashCode = prime * hashCode + ((getRootAccess() == null) ? 0 : getRootAccess().hashCode());
hashCode = prime * hashCode + ((getInstanceMetadataServiceConfiguration() == null) ? 0 : getInstanceMetadataServiceConfiguration().hashCode());
return hashCode;
}
@Override
public UpdateNotebookInstanceRequest clone() {
return (UpdateNotebookInstanceRequest) super.clone();
}
}