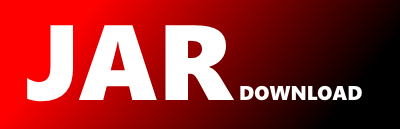
com.amazonaws.services.sagemaker.model.UserSettings Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemaker Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemaker.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A collection of settings that apply to users in a domain. These settings are specified when the
* CreateUserProfile
API is called, and as DefaultUserSettings
when the
* CreateDomain
API is called.
*
*
* SecurityGroups
is aggregated when specified in both calls. For all other settings in
* UserSettings
, the values specified in CreateUserProfile
take precedence over those
* specified in CreateDomain
.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UserSettings implements Serializable, Cloneable, StructuredPojo {
/**
*
* The execution role for the user.
*
*/
private String executionRole;
/**
*
* The security groups for the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*
* Optional when the CreateDomain.AppNetworkAccessType
parameter is set to
* PublicInternetOnly
.
*
*
* Required when the CreateDomain.AppNetworkAccessType
parameter is set to VpcOnly
, unless
* specified as part of the DefaultUserSettings
for the domain.
*
*
* Amazon SageMaker adds a security group to allow NFS traffic from Amazon SageMaker Studio. Therefore, the number
* of security groups that you can specify is one less than the maximum number shown.
*
*/
private java.util.List securityGroups;
/**
*
* Specifies options for sharing Amazon SageMaker Studio notebooks.
*
*/
private SharingSettings sharingSettings;
/**
*
* The Jupyter server's app settings.
*
*/
private JupyterServerAppSettings jupyterServerAppSettings;
/**
*
* The kernel gateway app settings.
*
*/
private KernelGatewayAppSettings kernelGatewayAppSettings;
/**
*
* The TensorBoard app settings.
*
*/
private TensorBoardAppSettings tensorBoardAppSettings;
/**
*
* A collection of settings that configure user interaction with the RStudioServerPro
app.
*
*/
private RStudioServerProAppSettings rStudioServerProAppSettings;
/**
*
* A collection of settings that configure the RSessionGateway
app.
*
*/
private RSessionAppSettings rSessionAppSettings;
/**
*
* The Canvas app settings.
*
*/
private CanvasAppSettings canvasAppSettings;
/**
*
* The Code Editor application settings.
*
*/
private CodeEditorAppSettings codeEditorAppSettings;
/**
*
* The settings for the JupyterLab application.
*
*/
private JupyterLabAppSettings jupyterLabAppSettings;
/**
*
* The storage settings for a space.
*
*/
private DefaultSpaceStorageSettings spaceStorageSettings;
/**
*
* The default experience that the user is directed to when accessing the domain. The supported values are:
*
*
* -
*
* studio::
: Indicates that Studio is the default experience. This value can only be passed if
* StudioWebPortal
is set to ENABLED
.
*
*
* -
*
* app:JupyterServer:
: Indicates that Studio Classic is the default experience.
*
*
*
*/
private String defaultLandingUri;
/**
*
* Whether the user can access Studio. If this value is set to DISABLED
, the user cannot access Studio,
* even if that is the default experience for the domain.
*
*/
private String studioWebPortal;
/**
*
* Details about the POSIX identity that is used for file system operations.
*
*/
private CustomPosixUserConfig customPosixUserConfig;
/**
*
* The settings for assigning a custom file system to a user profile. Permitted users can access this file system in
* Amazon SageMaker Studio.
*
*/
private java.util.List customFileSystemConfigs;
/**
*
* Studio settings. If these settings are applied on a user level, they take priority over the settings applied on a
* domain level.
*
*/
private StudioWebPortalSettings studioWebPortalSettings;
/**
*
* The execution role for the user.
*
*
* @param executionRole
* The execution role for the user.
*/
public void setExecutionRole(String executionRole) {
this.executionRole = executionRole;
}
/**
*
* The execution role for the user.
*
*
* @return The execution role for the user.
*/
public String getExecutionRole() {
return this.executionRole;
}
/**
*
* The execution role for the user.
*
*
* @param executionRole
* The execution role for the user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withExecutionRole(String executionRole) {
setExecutionRole(executionRole);
return this;
}
/**
*
* The security groups for the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*
* Optional when the CreateDomain.AppNetworkAccessType
parameter is set to
* PublicInternetOnly
.
*
*
* Required when the CreateDomain.AppNetworkAccessType
parameter is set to VpcOnly
, unless
* specified as part of the DefaultUserSettings
for the domain.
*
*
* Amazon SageMaker adds a security group to allow NFS traffic from Amazon SageMaker Studio. Therefore, the number
* of security groups that you can specify is one less than the maximum number shown.
*
*
* @return The security groups for the Amazon Virtual Private Cloud (VPC) that the domain uses for
* communication.
*
* Optional when the CreateDomain.AppNetworkAccessType
parameter is set to
* PublicInternetOnly
.
*
*
* Required when the CreateDomain.AppNetworkAccessType
parameter is set to VpcOnly
* , unless specified as part of the DefaultUserSettings
for the domain.
*
*
* Amazon SageMaker adds a security group to allow NFS traffic from Amazon SageMaker Studio. Therefore, the
* number of security groups that you can specify is one less than the maximum number shown.
*/
public java.util.List getSecurityGroups() {
return securityGroups;
}
/**
*
* The security groups for the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*
* Optional when the CreateDomain.AppNetworkAccessType
parameter is set to
* PublicInternetOnly
.
*
*
* Required when the CreateDomain.AppNetworkAccessType
parameter is set to VpcOnly
, unless
* specified as part of the DefaultUserSettings
for the domain.
*
*
* Amazon SageMaker adds a security group to allow NFS traffic from Amazon SageMaker Studio. Therefore, the number
* of security groups that you can specify is one less than the maximum number shown.
*
*
* @param securityGroups
* The security groups for the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
* Optional when the CreateDomain.AppNetworkAccessType
parameter is set to
* PublicInternetOnly
.
*
*
* Required when the CreateDomain.AppNetworkAccessType
parameter is set to VpcOnly
,
* unless specified as part of the DefaultUserSettings
for the domain.
*
*
* Amazon SageMaker adds a security group to allow NFS traffic from Amazon SageMaker Studio. Therefore, the
* number of security groups that you can specify is one less than the maximum number shown.
*/
public void setSecurityGroups(java.util.Collection securityGroups) {
if (securityGroups == null) {
this.securityGroups = null;
return;
}
this.securityGroups = new java.util.ArrayList(securityGroups);
}
/**
*
* The security groups for the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*
* Optional when the CreateDomain.AppNetworkAccessType
parameter is set to
* PublicInternetOnly
.
*
*
* Required when the CreateDomain.AppNetworkAccessType
parameter is set to VpcOnly
, unless
* specified as part of the DefaultUserSettings
for the domain.
*
*
* Amazon SageMaker adds a security group to allow NFS traffic from Amazon SageMaker Studio. Therefore, the number
* of security groups that you can specify is one less than the maximum number shown.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroups(java.util.Collection)} or {@link #withSecurityGroups(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param securityGroups
* The security groups for the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
* Optional when the CreateDomain.AppNetworkAccessType
parameter is set to
* PublicInternetOnly
.
*
*
* Required when the CreateDomain.AppNetworkAccessType
parameter is set to VpcOnly
,
* unless specified as part of the DefaultUserSettings
for the domain.
*
*
* Amazon SageMaker adds a security group to allow NFS traffic from Amazon SageMaker Studio. Therefore, the
* number of security groups that you can specify is one less than the maximum number shown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withSecurityGroups(String... securityGroups) {
if (this.securityGroups == null) {
setSecurityGroups(new java.util.ArrayList(securityGroups.length));
}
for (String ele : securityGroups) {
this.securityGroups.add(ele);
}
return this;
}
/**
*
* The security groups for the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
*
* Optional when the CreateDomain.AppNetworkAccessType
parameter is set to
* PublicInternetOnly
.
*
*
* Required when the CreateDomain.AppNetworkAccessType
parameter is set to VpcOnly
, unless
* specified as part of the DefaultUserSettings
for the domain.
*
*
* Amazon SageMaker adds a security group to allow NFS traffic from Amazon SageMaker Studio. Therefore, the number
* of security groups that you can specify is one less than the maximum number shown.
*
*
* @param securityGroups
* The security groups for the Amazon Virtual Private Cloud (VPC) that the domain uses for communication.
*
* Optional when the CreateDomain.AppNetworkAccessType
parameter is set to
* PublicInternetOnly
.
*
*
* Required when the CreateDomain.AppNetworkAccessType
parameter is set to VpcOnly
,
* unless specified as part of the DefaultUserSettings
for the domain.
*
*
* Amazon SageMaker adds a security group to allow NFS traffic from Amazon SageMaker Studio. Therefore, the
* number of security groups that you can specify is one less than the maximum number shown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withSecurityGroups(java.util.Collection securityGroups) {
setSecurityGroups(securityGroups);
return this;
}
/**
*
* Specifies options for sharing Amazon SageMaker Studio notebooks.
*
*
* @param sharingSettings
* Specifies options for sharing Amazon SageMaker Studio notebooks.
*/
public void setSharingSettings(SharingSettings sharingSettings) {
this.sharingSettings = sharingSettings;
}
/**
*
* Specifies options for sharing Amazon SageMaker Studio notebooks.
*
*
* @return Specifies options for sharing Amazon SageMaker Studio notebooks.
*/
public SharingSettings getSharingSettings() {
return this.sharingSettings;
}
/**
*
* Specifies options for sharing Amazon SageMaker Studio notebooks.
*
*
* @param sharingSettings
* Specifies options for sharing Amazon SageMaker Studio notebooks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withSharingSettings(SharingSettings sharingSettings) {
setSharingSettings(sharingSettings);
return this;
}
/**
*
* The Jupyter server's app settings.
*
*
* @param jupyterServerAppSettings
* The Jupyter server's app settings.
*/
public void setJupyterServerAppSettings(JupyterServerAppSettings jupyterServerAppSettings) {
this.jupyterServerAppSettings = jupyterServerAppSettings;
}
/**
*
* The Jupyter server's app settings.
*
*
* @return The Jupyter server's app settings.
*/
public JupyterServerAppSettings getJupyterServerAppSettings() {
return this.jupyterServerAppSettings;
}
/**
*
* The Jupyter server's app settings.
*
*
* @param jupyterServerAppSettings
* The Jupyter server's app settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withJupyterServerAppSettings(JupyterServerAppSettings jupyterServerAppSettings) {
setJupyterServerAppSettings(jupyterServerAppSettings);
return this;
}
/**
*
* The kernel gateway app settings.
*
*
* @param kernelGatewayAppSettings
* The kernel gateway app settings.
*/
public void setKernelGatewayAppSettings(KernelGatewayAppSettings kernelGatewayAppSettings) {
this.kernelGatewayAppSettings = kernelGatewayAppSettings;
}
/**
*
* The kernel gateway app settings.
*
*
* @return The kernel gateway app settings.
*/
public KernelGatewayAppSettings getKernelGatewayAppSettings() {
return this.kernelGatewayAppSettings;
}
/**
*
* The kernel gateway app settings.
*
*
* @param kernelGatewayAppSettings
* The kernel gateway app settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withKernelGatewayAppSettings(KernelGatewayAppSettings kernelGatewayAppSettings) {
setKernelGatewayAppSettings(kernelGatewayAppSettings);
return this;
}
/**
*
* The TensorBoard app settings.
*
*
* @param tensorBoardAppSettings
* The TensorBoard app settings.
*/
public void setTensorBoardAppSettings(TensorBoardAppSettings tensorBoardAppSettings) {
this.tensorBoardAppSettings = tensorBoardAppSettings;
}
/**
*
* The TensorBoard app settings.
*
*
* @return The TensorBoard app settings.
*/
public TensorBoardAppSettings getTensorBoardAppSettings() {
return this.tensorBoardAppSettings;
}
/**
*
* The TensorBoard app settings.
*
*
* @param tensorBoardAppSettings
* The TensorBoard app settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withTensorBoardAppSettings(TensorBoardAppSettings tensorBoardAppSettings) {
setTensorBoardAppSettings(tensorBoardAppSettings);
return this;
}
/**
*
* A collection of settings that configure user interaction with the RStudioServerPro
app.
*
*
* @param rStudioServerProAppSettings
* A collection of settings that configure user interaction with the RStudioServerPro
app.
*/
public void setRStudioServerProAppSettings(RStudioServerProAppSettings rStudioServerProAppSettings) {
this.rStudioServerProAppSettings = rStudioServerProAppSettings;
}
/**
*
* A collection of settings that configure user interaction with the RStudioServerPro
app.
*
*
* @return A collection of settings that configure user interaction with the RStudioServerPro
app.
*/
public RStudioServerProAppSettings getRStudioServerProAppSettings() {
return this.rStudioServerProAppSettings;
}
/**
*
* A collection of settings that configure user interaction with the RStudioServerPro
app.
*
*
* @param rStudioServerProAppSettings
* A collection of settings that configure user interaction with the RStudioServerPro
app.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withRStudioServerProAppSettings(RStudioServerProAppSettings rStudioServerProAppSettings) {
setRStudioServerProAppSettings(rStudioServerProAppSettings);
return this;
}
/**
*
* A collection of settings that configure the RSessionGateway
app.
*
*
* @param rSessionAppSettings
* A collection of settings that configure the RSessionGateway
app.
*/
public void setRSessionAppSettings(RSessionAppSettings rSessionAppSettings) {
this.rSessionAppSettings = rSessionAppSettings;
}
/**
*
* A collection of settings that configure the RSessionGateway
app.
*
*
* @return A collection of settings that configure the RSessionGateway
app.
*/
public RSessionAppSettings getRSessionAppSettings() {
return this.rSessionAppSettings;
}
/**
*
* A collection of settings that configure the RSessionGateway
app.
*
*
* @param rSessionAppSettings
* A collection of settings that configure the RSessionGateway
app.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withRSessionAppSettings(RSessionAppSettings rSessionAppSettings) {
setRSessionAppSettings(rSessionAppSettings);
return this;
}
/**
*
* The Canvas app settings.
*
*
* @param canvasAppSettings
* The Canvas app settings.
*/
public void setCanvasAppSettings(CanvasAppSettings canvasAppSettings) {
this.canvasAppSettings = canvasAppSettings;
}
/**
*
* The Canvas app settings.
*
*
* @return The Canvas app settings.
*/
public CanvasAppSettings getCanvasAppSettings() {
return this.canvasAppSettings;
}
/**
*
* The Canvas app settings.
*
*
* @param canvasAppSettings
* The Canvas app settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withCanvasAppSettings(CanvasAppSettings canvasAppSettings) {
setCanvasAppSettings(canvasAppSettings);
return this;
}
/**
*
* The Code Editor application settings.
*
*
* @param codeEditorAppSettings
* The Code Editor application settings.
*/
public void setCodeEditorAppSettings(CodeEditorAppSettings codeEditorAppSettings) {
this.codeEditorAppSettings = codeEditorAppSettings;
}
/**
*
* The Code Editor application settings.
*
*
* @return The Code Editor application settings.
*/
public CodeEditorAppSettings getCodeEditorAppSettings() {
return this.codeEditorAppSettings;
}
/**
*
* The Code Editor application settings.
*
*
* @param codeEditorAppSettings
* The Code Editor application settings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withCodeEditorAppSettings(CodeEditorAppSettings codeEditorAppSettings) {
setCodeEditorAppSettings(codeEditorAppSettings);
return this;
}
/**
*
* The settings for the JupyterLab application.
*
*
* @param jupyterLabAppSettings
* The settings for the JupyterLab application.
*/
public void setJupyterLabAppSettings(JupyterLabAppSettings jupyterLabAppSettings) {
this.jupyterLabAppSettings = jupyterLabAppSettings;
}
/**
*
* The settings for the JupyterLab application.
*
*
* @return The settings for the JupyterLab application.
*/
public JupyterLabAppSettings getJupyterLabAppSettings() {
return this.jupyterLabAppSettings;
}
/**
*
* The settings for the JupyterLab application.
*
*
* @param jupyterLabAppSettings
* The settings for the JupyterLab application.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withJupyterLabAppSettings(JupyterLabAppSettings jupyterLabAppSettings) {
setJupyterLabAppSettings(jupyterLabAppSettings);
return this;
}
/**
*
* The storage settings for a space.
*
*
* @param spaceStorageSettings
* The storage settings for a space.
*/
public void setSpaceStorageSettings(DefaultSpaceStorageSettings spaceStorageSettings) {
this.spaceStorageSettings = spaceStorageSettings;
}
/**
*
* The storage settings for a space.
*
*
* @return The storage settings for a space.
*/
public DefaultSpaceStorageSettings getSpaceStorageSettings() {
return this.spaceStorageSettings;
}
/**
*
* The storage settings for a space.
*
*
* @param spaceStorageSettings
* The storage settings for a space.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withSpaceStorageSettings(DefaultSpaceStorageSettings spaceStorageSettings) {
setSpaceStorageSettings(spaceStorageSettings);
return this;
}
/**
*
* The default experience that the user is directed to when accessing the domain. The supported values are:
*
*
* -
*
* studio::
: Indicates that Studio is the default experience. This value can only be passed if
* StudioWebPortal
is set to ENABLED
.
*
*
* -
*
* app:JupyterServer:
: Indicates that Studio Classic is the default experience.
*
*
*
*
* @param defaultLandingUri
* The default experience that the user is directed to when accessing the domain. The supported values
* are:
*
* -
*
* studio::
: Indicates that Studio is the default experience. This value can only be passed if
* StudioWebPortal
is set to ENABLED
.
*
*
* -
*
* app:JupyterServer:
: Indicates that Studio Classic is the default experience.
*
*
*/
public void setDefaultLandingUri(String defaultLandingUri) {
this.defaultLandingUri = defaultLandingUri;
}
/**
*
* The default experience that the user is directed to when accessing the domain. The supported values are:
*
*
* -
*
* studio::
: Indicates that Studio is the default experience. This value can only be passed if
* StudioWebPortal
is set to ENABLED
.
*
*
* -
*
* app:JupyterServer:
: Indicates that Studio Classic is the default experience.
*
*
*
*
* @return The default experience that the user is directed to when accessing the domain. The supported values
* are:
*
* -
*
* studio::
: Indicates that Studio is the default experience. This value can only be passed if
* StudioWebPortal
is set to ENABLED
.
*
*
* -
*
* app:JupyterServer:
: Indicates that Studio Classic is the default experience.
*
*
*/
public String getDefaultLandingUri() {
return this.defaultLandingUri;
}
/**
*
* The default experience that the user is directed to when accessing the domain. The supported values are:
*
*
* -
*
* studio::
: Indicates that Studio is the default experience. This value can only be passed if
* StudioWebPortal
is set to ENABLED
.
*
*
* -
*
* app:JupyterServer:
: Indicates that Studio Classic is the default experience.
*
*
*
*
* @param defaultLandingUri
* The default experience that the user is directed to when accessing the domain. The supported values
* are:
*
* -
*
* studio::
: Indicates that Studio is the default experience. This value can only be passed if
* StudioWebPortal
is set to ENABLED
.
*
*
* -
*
* app:JupyterServer:
: Indicates that Studio Classic is the default experience.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withDefaultLandingUri(String defaultLandingUri) {
setDefaultLandingUri(defaultLandingUri);
return this;
}
/**
*
* Whether the user can access Studio. If this value is set to DISABLED
, the user cannot access Studio,
* even if that is the default experience for the domain.
*
*
* @param studioWebPortal
* Whether the user can access Studio. If this value is set to DISABLED
, the user cannot access
* Studio, even if that is the default experience for the domain.
* @see StudioWebPortal
*/
public void setStudioWebPortal(String studioWebPortal) {
this.studioWebPortal = studioWebPortal;
}
/**
*
* Whether the user can access Studio. If this value is set to DISABLED
, the user cannot access Studio,
* even if that is the default experience for the domain.
*
*
* @return Whether the user can access Studio. If this value is set to DISABLED
, the user cannot access
* Studio, even if that is the default experience for the domain.
* @see StudioWebPortal
*/
public String getStudioWebPortal() {
return this.studioWebPortal;
}
/**
*
* Whether the user can access Studio. If this value is set to DISABLED
, the user cannot access Studio,
* even if that is the default experience for the domain.
*
*
* @param studioWebPortal
* Whether the user can access Studio. If this value is set to DISABLED
, the user cannot access
* Studio, even if that is the default experience for the domain.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StudioWebPortal
*/
public UserSettings withStudioWebPortal(String studioWebPortal) {
setStudioWebPortal(studioWebPortal);
return this;
}
/**
*
* Whether the user can access Studio. If this value is set to DISABLED
, the user cannot access Studio,
* even if that is the default experience for the domain.
*
*
* @param studioWebPortal
* Whether the user can access Studio. If this value is set to DISABLED
, the user cannot access
* Studio, even if that is the default experience for the domain.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StudioWebPortal
*/
public UserSettings withStudioWebPortal(StudioWebPortal studioWebPortal) {
this.studioWebPortal = studioWebPortal.toString();
return this;
}
/**
*
* Details about the POSIX identity that is used for file system operations.
*
*
* @param customPosixUserConfig
* Details about the POSIX identity that is used for file system operations.
*/
public void setCustomPosixUserConfig(CustomPosixUserConfig customPosixUserConfig) {
this.customPosixUserConfig = customPosixUserConfig;
}
/**
*
* Details about the POSIX identity that is used for file system operations.
*
*
* @return Details about the POSIX identity that is used for file system operations.
*/
public CustomPosixUserConfig getCustomPosixUserConfig() {
return this.customPosixUserConfig;
}
/**
*
* Details about the POSIX identity that is used for file system operations.
*
*
* @param customPosixUserConfig
* Details about the POSIX identity that is used for file system operations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withCustomPosixUserConfig(CustomPosixUserConfig customPosixUserConfig) {
setCustomPosixUserConfig(customPosixUserConfig);
return this;
}
/**
*
* The settings for assigning a custom file system to a user profile. Permitted users can access this file system in
* Amazon SageMaker Studio.
*
*
* @return The settings for assigning a custom file system to a user profile. Permitted users can access this file
* system in Amazon SageMaker Studio.
*/
public java.util.List getCustomFileSystemConfigs() {
return customFileSystemConfigs;
}
/**
*
* The settings for assigning a custom file system to a user profile. Permitted users can access this file system in
* Amazon SageMaker Studio.
*
*
* @param customFileSystemConfigs
* The settings for assigning a custom file system to a user profile. Permitted users can access this file
* system in Amazon SageMaker Studio.
*/
public void setCustomFileSystemConfigs(java.util.Collection customFileSystemConfigs) {
if (customFileSystemConfigs == null) {
this.customFileSystemConfigs = null;
return;
}
this.customFileSystemConfigs = new java.util.ArrayList(customFileSystemConfigs);
}
/**
*
* The settings for assigning a custom file system to a user profile. Permitted users can access this file system in
* Amazon SageMaker Studio.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCustomFileSystemConfigs(java.util.Collection)} or
* {@link #withCustomFileSystemConfigs(java.util.Collection)} if you want to override the existing values.
*
*
* @param customFileSystemConfigs
* The settings for assigning a custom file system to a user profile. Permitted users can access this file
* system in Amazon SageMaker Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withCustomFileSystemConfigs(CustomFileSystemConfig... customFileSystemConfigs) {
if (this.customFileSystemConfigs == null) {
setCustomFileSystemConfigs(new java.util.ArrayList(customFileSystemConfigs.length));
}
for (CustomFileSystemConfig ele : customFileSystemConfigs) {
this.customFileSystemConfigs.add(ele);
}
return this;
}
/**
*
* The settings for assigning a custom file system to a user profile. Permitted users can access this file system in
* Amazon SageMaker Studio.
*
*
* @param customFileSystemConfigs
* The settings for assigning a custom file system to a user profile. Permitted users can access this file
* system in Amazon SageMaker Studio.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withCustomFileSystemConfigs(java.util.Collection customFileSystemConfigs) {
setCustomFileSystemConfigs(customFileSystemConfigs);
return this;
}
/**
*
* Studio settings. If these settings are applied on a user level, they take priority over the settings applied on a
* domain level.
*
*
* @param studioWebPortalSettings
* Studio settings. If these settings are applied on a user level, they take priority over the settings
* applied on a domain level.
*/
public void setStudioWebPortalSettings(StudioWebPortalSettings studioWebPortalSettings) {
this.studioWebPortalSettings = studioWebPortalSettings;
}
/**
*
* Studio settings. If these settings are applied on a user level, they take priority over the settings applied on a
* domain level.
*
*
* @return Studio settings. If these settings are applied on a user level, they take priority over the settings
* applied on a domain level.
*/
public StudioWebPortalSettings getStudioWebPortalSettings() {
return this.studioWebPortalSettings;
}
/**
*
* Studio settings. If these settings are applied on a user level, they take priority over the settings applied on a
* domain level.
*
*
* @param studioWebPortalSettings
* Studio settings. If these settings are applied on a user level, they take priority over the settings
* applied on a domain level.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UserSettings withStudioWebPortalSettings(StudioWebPortalSettings studioWebPortalSettings) {
setStudioWebPortalSettings(studioWebPortalSettings);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getExecutionRole() != null)
sb.append("ExecutionRole: ").append(getExecutionRole()).append(",");
if (getSecurityGroups() != null)
sb.append("SecurityGroups: ").append(getSecurityGroups()).append(",");
if (getSharingSettings() != null)
sb.append("SharingSettings: ").append(getSharingSettings()).append(",");
if (getJupyterServerAppSettings() != null)
sb.append("JupyterServerAppSettings: ").append(getJupyterServerAppSettings()).append(",");
if (getKernelGatewayAppSettings() != null)
sb.append("KernelGatewayAppSettings: ").append(getKernelGatewayAppSettings()).append(",");
if (getTensorBoardAppSettings() != null)
sb.append("TensorBoardAppSettings: ").append(getTensorBoardAppSettings()).append(",");
if (getRStudioServerProAppSettings() != null)
sb.append("RStudioServerProAppSettings: ").append(getRStudioServerProAppSettings()).append(",");
if (getRSessionAppSettings() != null)
sb.append("RSessionAppSettings: ").append(getRSessionAppSettings()).append(",");
if (getCanvasAppSettings() != null)
sb.append("CanvasAppSettings: ").append(getCanvasAppSettings()).append(",");
if (getCodeEditorAppSettings() != null)
sb.append("CodeEditorAppSettings: ").append(getCodeEditorAppSettings()).append(",");
if (getJupyterLabAppSettings() != null)
sb.append("JupyterLabAppSettings: ").append(getJupyterLabAppSettings()).append(",");
if (getSpaceStorageSettings() != null)
sb.append("SpaceStorageSettings: ").append(getSpaceStorageSettings()).append(",");
if (getDefaultLandingUri() != null)
sb.append("DefaultLandingUri: ").append(getDefaultLandingUri()).append(",");
if (getStudioWebPortal() != null)
sb.append("StudioWebPortal: ").append(getStudioWebPortal()).append(",");
if (getCustomPosixUserConfig() != null)
sb.append("CustomPosixUserConfig: ").append(getCustomPosixUserConfig()).append(",");
if (getCustomFileSystemConfigs() != null)
sb.append("CustomFileSystemConfigs: ").append(getCustomFileSystemConfigs()).append(",");
if (getStudioWebPortalSettings() != null)
sb.append("StudioWebPortalSettings: ").append(getStudioWebPortalSettings());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UserSettings == false)
return false;
UserSettings other = (UserSettings) obj;
if (other.getExecutionRole() == null ^ this.getExecutionRole() == null)
return false;
if (other.getExecutionRole() != null && other.getExecutionRole().equals(this.getExecutionRole()) == false)
return false;
if (other.getSecurityGroups() == null ^ this.getSecurityGroups() == null)
return false;
if (other.getSecurityGroups() != null && other.getSecurityGroups().equals(this.getSecurityGroups()) == false)
return false;
if (other.getSharingSettings() == null ^ this.getSharingSettings() == null)
return false;
if (other.getSharingSettings() != null && other.getSharingSettings().equals(this.getSharingSettings()) == false)
return false;
if (other.getJupyterServerAppSettings() == null ^ this.getJupyterServerAppSettings() == null)
return false;
if (other.getJupyterServerAppSettings() != null && other.getJupyterServerAppSettings().equals(this.getJupyterServerAppSettings()) == false)
return false;
if (other.getKernelGatewayAppSettings() == null ^ this.getKernelGatewayAppSettings() == null)
return false;
if (other.getKernelGatewayAppSettings() != null && other.getKernelGatewayAppSettings().equals(this.getKernelGatewayAppSettings()) == false)
return false;
if (other.getTensorBoardAppSettings() == null ^ this.getTensorBoardAppSettings() == null)
return false;
if (other.getTensorBoardAppSettings() != null && other.getTensorBoardAppSettings().equals(this.getTensorBoardAppSettings()) == false)
return false;
if (other.getRStudioServerProAppSettings() == null ^ this.getRStudioServerProAppSettings() == null)
return false;
if (other.getRStudioServerProAppSettings() != null && other.getRStudioServerProAppSettings().equals(this.getRStudioServerProAppSettings()) == false)
return false;
if (other.getRSessionAppSettings() == null ^ this.getRSessionAppSettings() == null)
return false;
if (other.getRSessionAppSettings() != null && other.getRSessionAppSettings().equals(this.getRSessionAppSettings()) == false)
return false;
if (other.getCanvasAppSettings() == null ^ this.getCanvasAppSettings() == null)
return false;
if (other.getCanvasAppSettings() != null && other.getCanvasAppSettings().equals(this.getCanvasAppSettings()) == false)
return false;
if (other.getCodeEditorAppSettings() == null ^ this.getCodeEditorAppSettings() == null)
return false;
if (other.getCodeEditorAppSettings() != null && other.getCodeEditorAppSettings().equals(this.getCodeEditorAppSettings()) == false)
return false;
if (other.getJupyterLabAppSettings() == null ^ this.getJupyterLabAppSettings() == null)
return false;
if (other.getJupyterLabAppSettings() != null && other.getJupyterLabAppSettings().equals(this.getJupyterLabAppSettings()) == false)
return false;
if (other.getSpaceStorageSettings() == null ^ this.getSpaceStorageSettings() == null)
return false;
if (other.getSpaceStorageSettings() != null && other.getSpaceStorageSettings().equals(this.getSpaceStorageSettings()) == false)
return false;
if (other.getDefaultLandingUri() == null ^ this.getDefaultLandingUri() == null)
return false;
if (other.getDefaultLandingUri() != null && other.getDefaultLandingUri().equals(this.getDefaultLandingUri()) == false)
return false;
if (other.getStudioWebPortal() == null ^ this.getStudioWebPortal() == null)
return false;
if (other.getStudioWebPortal() != null && other.getStudioWebPortal().equals(this.getStudioWebPortal()) == false)
return false;
if (other.getCustomPosixUserConfig() == null ^ this.getCustomPosixUserConfig() == null)
return false;
if (other.getCustomPosixUserConfig() != null && other.getCustomPosixUserConfig().equals(this.getCustomPosixUserConfig()) == false)
return false;
if (other.getCustomFileSystemConfigs() == null ^ this.getCustomFileSystemConfigs() == null)
return false;
if (other.getCustomFileSystemConfigs() != null && other.getCustomFileSystemConfigs().equals(this.getCustomFileSystemConfigs()) == false)
return false;
if (other.getStudioWebPortalSettings() == null ^ this.getStudioWebPortalSettings() == null)
return false;
if (other.getStudioWebPortalSettings() != null && other.getStudioWebPortalSettings().equals(this.getStudioWebPortalSettings()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getExecutionRole() == null) ? 0 : getExecutionRole().hashCode());
hashCode = prime * hashCode + ((getSecurityGroups() == null) ? 0 : getSecurityGroups().hashCode());
hashCode = prime * hashCode + ((getSharingSettings() == null) ? 0 : getSharingSettings().hashCode());
hashCode = prime * hashCode + ((getJupyterServerAppSettings() == null) ? 0 : getJupyterServerAppSettings().hashCode());
hashCode = prime * hashCode + ((getKernelGatewayAppSettings() == null) ? 0 : getKernelGatewayAppSettings().hashCode());
hashCode = prime * hashCode + ((getTensorBoardAppSettings() == null) ? 0 : getTensorBoardAppSettings().hashCode());
hashCode = prime * hashCode + ((getRStudioServerProAppSettings() == null) ? 0 : getRStudioServerProAppSettings().hashCode());
hashCode = prime * hashCode + ((getRSessionAppSettings() == null) ? 0 : getRSessionAppSettings().hashCode());
hashCode = prime * hashCode + ((getCanvasAppSettings() == null) ? 0 : getCanvasAppSettings().hashCode());
hashCode = prime * hashCode + ((getCodeEditorAppSettings() == null) ? 0 : getCodeEditorAppSettings().hashCode());
hashCode = prime * hashCode + ((getJupyterLabAppSettings() == null) ? 0 : getJupyterLabAppSettings().hashCode());
hashCode = prime * hashCode + ((getSpaceStorageSettings() == null) ? 0 : getSpaceStorageSettings().hashCode());
hashCode = prime * hashCode + ((getDefaultLandingUri() == null) ? 0 : getDefaultLandingUri().hashCode());
hashCode = prime * hashCode + ((getStudioWebPortal() == null) ? 0 : getStudioWebPortal().hashCode());
hashCode = prime * hashCode + ((getCustomPosixUserConfig() == null) ? 0 : getCustomPosixUserConfig().hashCode());
hashCode = prime * hashCode + ((getCustomFileSystemConfigs() == null) ? 0 : getCustomFileSystemConfigs().hashCode());
hashCode = prime * hashCode + ((getStudioWebPortalSettings() == null) ? 0 : getStudioWebPortalSettings().hashCode());
return hashCode;
}
@Override
public UserSettings clone() {
try {
return (UserSettings) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.sagemaker.model.transform.UserSettingsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}