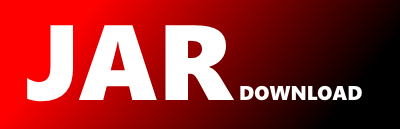
com.amazonaws.services.sagemakeredgemanager.model.SendHeartbeatRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemakeredgemanager Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemakeredgemanager.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class SendHeartbeatRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* For internal use. Returns a list of SageMaker Edge Manager agent operating metrics.
*
*/
private java.util.List agentMetrics;
/**
*
* Returns a list of models deployed on the the device.
*
*/
private java.util.List models;
/**
*
* Returns the version of the agent.
*
*/
private String agentVersion;
/**
*
* The unique name of the device.
*
*/
private String deviceName;
/**
*
* The name of the fleet that the device belongs to.
*
*/
private String deviceFleetName;
/**
*
* Returns the result of a deployment on the device.
*
*/
private DeploymentResult deploymentResult;
/**
*
* For internal use. Returns a list of SageMaker Edge Manager agent operating metrics.
*
*
* @return For internal use. Returns a list of SageMaker Edge Manager agent operating metrics.
*/
public java.util.List getAgentMetrics() {
return agentMetrics;
}
/**
*
* For internal use. Returns a list of SageMaker Edge Manager agent operating metrics.
*
*
* @param agentMetrics
* For internal use. Returns a list of SageMaker Edge Manager agent operating metrics.
*/
public void setAgentMetrics(java.util.Collection agentMetrics) {
if (agentMetrics == null) {
this.agentMetrics = null;
return;
}
this.agentMetrics = new java.util.ArrayList(agentMetrics);
}
/**
*
* For internal use. Returns a list of SageMaker Edge Manager agent operating metrics.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAgentMetrics(java.util.Collection)} or {@link #withAgentMetrics(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param agentMetrics
* For internal use. Returns a list of SageMaker Edge Manager agent operating metrics.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendHeartbeatRequest withAgentMetrics(EdgeMetric... agentMetrics) {
if (this.agentMetrics == null) {
setAgentMetrics(new java.util.ArrayList(agentMetrics.length));
}
for (EdgeMetric ele : agentMetrics) {
this.agentMetrics.add(ele);
}
return this;
}
/**
*
* For internal use. Returns a list of SageMaker Edge Manager agent operating metrics.
*
*
* @param agentMetrics
* For internal use. Returns a list of SageMaker Edge Manager agent operating metrics.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendHeartbeatRequest withAgentMetrics(java.util.Collection agentMetrics) {
setAgentMetrics(agentMetrics);
return this;
}
/**
*
* Returns a list of models deployed on the the device.
*
*
* @return Returns a list of models deployed on the the device.
*/
public java.util.List getModels() {
return models;
}
/**
*
* Returns a list of models deployed on the the device.
*
*
* @param models
* Returns a list of models deployed on the the device.
*/
public void setModels(java.util.Collection models) {
if (models == null) {
this.models = null;
return;
}
this.models = new java.util.ArrayList(models);
}
/**
*
* Returns a list of models deployed on the the device.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setModels(java.util.Collection)} or {@link #withModels(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param models
* Returns a list of models deployed on the the device.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendHeartbeatRequest withModels(Model... models) {
if (this.models == null) {
setModels(new java.util.ArrayList(models.length));
}
for (Model ele : models) {
this.models.add(ele);
}
return this;
}
/**
*
* Returns a list of models deployed on the the device.
*
*
* @param models
* Returns a list of models deployed on the the device.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendHeartbeatRequest withModels(java.util.Collection models) {
setModels(models);
return this;
}
/**
*
* Returns the version of the agent.
*
*
* @param agentVersion
* Returns the version of the agent.
*/
public void setAgentVersion(String agentVersion) {
this.agentVersion = agentVersion;
}
/**
*
* Returns the version of the agent.
*
*
* @return Returns the version of the agent.
*/
public String getAgentVersion() {
return this.agentVersion;
}
/**
*
* Returns the version of the agent.
*
*
* @param agentVersion
* Returns the version of the agent.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendHeartbeatRequest withAgentVersion(String agentVersion) {
setAgentVersion(agentVersion);
return this;
}
/**
*
* The unique name of the device.
*
*
* @param deviceName
* The unique name of the device.
*/
public void setDeviceName(String deviceName) {
this.deviceName = deviceName;
}
/**
*
* The unique name of the device.
*
*
* @return The unique name of the device.
*/
public String getDeviceName() {
return this.deviceName;
}
/**
*
* The unique name of the device.
*
*
* @param deviceName
* The unique name of the device.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendHeartbeatRequest withDeviceName(String deviceName) {
setDeviceName(deviceName);
return this;
}
/**
*
* The name of the fleet that the device belongs to.
*
*
* @param deviceFleetName
* The name of the fleet that the device belongs to.
*/
public void setDeviceFleetName(String deviceFleetName) {
this.deviceFleetName = deviceFleetName;
}
/**
*
* The name of the fleet that the device belongs to.
*
*
* @return The name of the fleet that the device belongs to.
*/
public String getDeviceFleetName() {
return this.deviceFleetName;
}
/**
*
* The name of the fleet that the device belongs to.
*
*
* @param deviceFleetName
* The name of the fleet that the device belongs to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendHeartbeatRequest withDeviceFleetName(String deviceFleetName) {
setDeviceFleetName(deviceFleetName);
return this;
}
/**
*
* Returns the result of a deployment on the device.
*
*
* @param deploymentResult
* Returns the result of a deployment on the device.
*/
public void setDeploymentResult(DeploymentResult deploymentResult) {
this.deploymentResult = deploymentResult;
}
/**
*
* Returns the result of a deployment on the device.
*
*
* @return Returns the result of a deployment on the device.
*/
public DeploymentResult getDeploymentResult() {
return this.deploymentResult;
}
/**
*
* Returns the result of a deployment on the device.
*
*
* @param deploymentResult
* Returns the result of a deployment on the device.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public SendHeartbeatRequest withDeploymentResult(DeploymentResult deploymentResult) {
setDeploymentResult(deploymentResult);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAgentMetrics() != null)
sb.append("AgentMetrics: ").append(getAgentMetrics()).append(",");
if (getModels() != null)
sb.append("Models: ").append(getModels()).append(",");
if (getAgentVersion() != null)
sb.append("AgentVersion: ").append(getAgentVersion()).append(",");
if (getDeviceName() != null)
sb.append("DeviceName: ").append(getDeviceName()).append(",");
if (getDeviceFleetName() != null)
sb.append("DeviceFleetName: ").append(getDeviceFleetName()).append(",");
if (getDeploymentResult() != null)
sb.append("DeploymentResult: ").append(getDeploymentResult());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof SendHeartbeatRequest == false)
return false;
SendHeartbeatRequest other = (SendHeartbeatRequest) obj;
if (other.getAgentMetrics() == null ^ this.getAgentMetrics() == null)
return false;
if (other.getAgentMetrics() != null && other.getAgentMetrics().equals(this.getAgentMetrics()) == false)
return false;
if (other.getModels() == null ^ this.getModels() == null)
return false;
if (other.getModels() != null && other.getModels().equals(this.getModels()) == false)
return false;
if (other.getAgentVersion() == null ^ this.getAgentVersion() == null)
return false;
if (other.getAgentVersion() != null && other.getAgentVersion().equals(this.getAgentVersion()) == false)
return false;
if (other.getDeviceName() == null ^ this.getDeviceName() == null)
return false;
if (other.getDeviceName() != null && other.getDeviceName().equals(this.getDeviceName()) == false)
return false;
if (other.getDeviceFleetName() == null ^ this.getDeviceFleetName() == null)
return false;
if (other.getDeviceFleetName() != null && other.getDeviceFleetName().equals(this.getDeviceFleetName()) == false)
return false;
if (other.getDeploymentResult() == null ^ this.getDeploymentResult() == null)
return false;
if (other.getDeploymentResult() != null && other.getDeploymentResult().equals(this.getDeploymentResult()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAgentMetrics() == null) ? 0 : getAgentMetrics().hashCode());
hashCode = prime * hashCode + ((getModels() == null) ? 0 : getModels().hashCode());
hashCode = prime * hashCode + ((getAgentVersion() == null) ? 0 : getAgentVersion().hashCode());
hashCode = prime * hashCode + ((getDeviceName() == null) ? 0 : getDeviceName().hashCode());
hashCode = prime * hashCode + ((getDeviceFleetName() == null) ? 0 : getDeviceFleetName().hashCode());
hashCode = prime * hashCode + ((getDeploymentResult() == null) ? 0 : getDeploymentResult().hashCode());
return hashCode;
}
@Override
public SendHeartbeatRequest clone() {
return (SendHeartbeatRequest) super.clone();
}
}