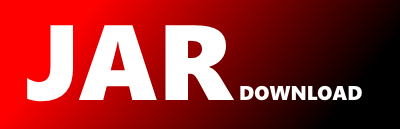
com.amazonaws.services.sagemakerruntime.model.InvokeEndpointAsyncRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemakerruntime Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemakerruntime.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class InvokeEndpointAsyncRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*
*/
private String endpointName;
/**
*
* The MIME type of the input data in the request body.
*
*/
private String contentType;
/**
*
* The desired MIME type of the inference response from the model container.
*
*/
private String accept;
/**
*
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to provide an ID that you can use to track a request or to provide other metadata that a service
* endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII characters as
* specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*/
private String customAttributes;
/**
*
* The identifier for the inference request. Amazon SageMaker will generate an identifier for you if none is
* specified.
*
*/
private String inferenceId;
/**
*
* The Amazon S3 URI where the inference request payload is stored.
*
*/
private String inputLocation;
/**
*
* Maximum age in seconds a request can be in the queue before it is marked as expired. The default is 6 hours, or
* 21,600 seconds.
*
*/
private Integer requestTTLSeconds;
/**
*
* Maximum amount of time in seconds a request can be processed before it is marked as expired. The default is 15
* minutes, or 900 seconds.
*
*/
private Integer invocationTimeoutSeconds;
/**
*
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*
*
* @param endpointName
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*/
public void setEndpointName(String endpointName) {
this.endpointName = endpointName;
}
/**
*
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*
*
* @return The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*/
public String getEndpointName() {
return this.endpointName;
}
/**
*
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*
*
* @param endpointName
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointAsyncRequest withEndpointName(String endpointName) {
setEndpointName(endpointName);
return this;
}
/**
*
* The MIME type of the input data in the request body.
*
*
* @param contentType
* The MIME type of the input data in the request body.
*/
public void setContentType(String contentType) {
this.contentType = contentType;
}
/**
*
* The MIME type of the input data in the request body.
*
*
* @return The MIME type of the input data in the request body.
*/
public String getContentType() {
return this.contentType;
}
/**
*
* The MIME type of the input data in the request body.
*
*
* @param contentType
* The MIME type of the input data in the request body.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointAsyncRequest withContentType(String contentType) {
setContentType(contentType);
return this;
}
/**
*
* The desired MIME type of the inference response from the model container.
*
*
* @param accept
* The desired MIME type of the inference response from the model container.
*/
public void setAccept(String accept) {
this.accept = accept;
}
/**
*
* The desired MIME type of the inference response from the model container.
*
*
* @return The desired MIME type of the inference response from the model container.
*/
public String getAccept() {
return this.accept;
}
/**
*
* The desired MIME type of the inference response from the model container.
*
*
* @param accept
* The desired MIME type of the inference response from the model container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointAsyncRequest withAccept(String accept) {
setAccept(accept);
return this;
}
/**
*
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to provide an ID that you can use to track a request or to provide other metadata that a service
* endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII characters as
* specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*
* @param customAttributes
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this
* value, for example, to provide an ID that you can use to track a request or to provide other metadata that
* a service endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII
* characters as specified in Section
* 3.3.6. Field Value Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If
* your code does not set this value in the response, an empty value is returned. For example, if a custom
* attribute represents the trace ID, your model can prepend the custom attribute with Trace ID:
* in your post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python
* SDK.
*/
public void setCustomAttributes(String customAttributes) {
this.customAttributes = customAttributes;
}
/**
*
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to provide an ID that you can use to track a request or to provide other metadata that a service
* endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII characters as
* specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*
* @return Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this
* value, for example, to provide an ID that you can use to track a request or to provide other metadata
* that a service endpoint was programmed to process. The value must consist of no more than 1024 visible
* US-ASCII characters as specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If
* your code does not set this value in the response, an empty value is returned. For example, if a custom
* attribute represents the trace ID, your model can prepend the custom attribute with
* Trace ID:
in your post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker
* Python SDK.
*/
public String getCustomAttributes() {
return this.customAttributes;
}
/**
*
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to provide an ID that you can use to track a request or to provide other metadata that a service
* endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII characters as
* specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*
* @param customAttributes
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this
* value, for example, to provide an ID that you can use to track a request or to provide other metadata that
* a service endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII
* characters as specified in Section
* 3.3.6. Field Value Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If
* your code does not set this value in the response, an empty value is returned. For example, if a custom
* attribute represents the trace ID, your model can prepend the custom attribute with Trace ID:
* in your post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python
* SDK.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointAsyncRequest withCustomAttributes(String customAttributes) {
setCustomAttributes(customAttributes);
return this;
}
/**
*
* The identifier for the inference request. Amazon SageMaker will generate an identifier for you if none is
* specified.
*
*
* @param inferenceId
* The identifier for the inference request. Amazon SageMaker will generate an identifier for you if none is
* specified.
*/
public void setInferenceId(String inferenceId) {
this.inferenceId = inferenceId;
}
/**
*
* The identifier for the inference request. Amazon SageMaker will generate an identifier for you if none is
* specified.
*
*
* @return The identifier for the inference request. Amazon SageMaker will generate an identifier for you if none is
* specified.
*/
public String getInferenceId() {
return this.inferenceId;
}
/**
*
* The identifier for the inference request. Amazon SageMaker will generate an identifier for you if none is
* specified.
*
*
* @param inferenceId
* The identifier for the inference request. Amazon SageMaker will generate an identifier for you if none is
* specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointAsyncRequest withInferenceId(String inferenceId) {
setInferenceId(inferenceId);
return this;
}
/**
*
* The Amazon S3 URI where the inference request payload is stored.
*
*
* @param inputLocation
* The Amazon S3 URI where the inference request payload is stored.
*/
public void setInputLocation(String inputLocation) {
this.inputLocation = inputLocation;
}
/**
*
* The Amazon S3 URI where the inference request payload is stored.
*
*
* @return The Amazon S3 URI where the inference request payload is stored.
*/
public String getInputLocation() {
return this.inputLocation;
}
/**
*
* The Amazon S3 URI where the inference request payload is stored.
*
*
* @param inputLocation
* The Amazon S3 URI where the inference request payload is stored.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointAsyncRequest withInputLocation(String inputLocation) {
setInputLocation(inputLocation);
return this;
}
/**
*
* Maximum age in seconds a request can be in the queue before it is marked as expired. The default is 6 hours, or
* 21,600 seconds.
*
*
* @param requestTTLSeconds
* Maximum age in seconds a request can be in the queue before it is marked as expired. The default is 6
* hours, or 21,600 seconds.
*/
public void setRequestTTLSeconds(Integer requestTTLSeconds) {
this.requestTTLSeconds = requestTTLSeconds;
}
/**
*
* Maximum age in seconds a request can be in the queue before it is marked as expired. The default is 6 hours, or
* 21,600 seconds.
*
*
* @return Maximum age in seconds a request can be in the queue before it is marked as expired. The default is 6
* hours, or 21,600 seconds.
*/
public Integer getRequestTTLSeconds() {
return this.requestTTLSeconds;
}
/**
*
* Maximum age in seconds a request can be in the queue before it is marked as expired. The default is 6 hours, or
* 21,600 seconds.
*
*
* @param requestTTLSeconds
* Maximum age in seconds a request can be in the queue before it is marked as expired. The default is 6
* hours, or 21,600 seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointAsyncRequest withRequestTTLSeconds(Integer requestTTLSeconds) {
setRequestTTLSeconds(requestTTLSeconds);
return this;
}
/**
*
* Maximum amount of time in seconds a request can be processed before it is marked as expired. The default is 15
* minutes, or 900 seconds.
*
*
* @param invocationTimeoutSeconds
* Maximum amount of time in seconds a request can be processed before it is marked as expired. The default
* is 15 minutes, or 900 seconds.
*/
public void setInvocationTimeoutSeconds(Integer invocationTimeoutSeconds) {
this.invocationTimeoutSeconds = invocationTimeoutSeconds;
}
/**
*
* Maximum amount of time in seconds a request can be processed before it is marked as expired. The default is 15
* minutes, or 900 seconds.
*
*
* @return Maximum amount of time in seconds a request can be processed before it is marked as expired. The default
* is 15 minutes, or 900 seconds.
*/
public Integer getInvocationTimeoutSeconds() {
return this.invocationTimeoutSeconds;
}
/**
*
* Maximum amount of time in seconds a request can be processed before it is marked as expired. The default is 15
* minutes, or 900 seconds.
*
*
* @param invocationTimeoutSeconds
* Maximum amount of time in seconds a request can be processed before it is marked as expired. The default
* is 15 minutes, or 900 seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointAsyncRequest withInvocationTimeoutSeconds(Integer invocationTimeoutSeconds) {
setInvocationTimeoutSeconds(invocationTimeoutSeconds);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEndpointName() != null)
sb.append("EndpointName: ").append(getEndpointName()).append(",");
if (getContentType() != null)
sb.append("ContentType: ").append(getContentType()).append(",");
if (getAccept() != null)
sb.append("Accept: ").append(getAccept()).append(",");
if (getCustomAttributes() != null)
sb.append("CustomAttributes: ").append("***Sensitive Data Redacted***").append(",");
if (getInferenceId() != null)
sb.append("InferenceId: ").append(getInferenceId()).append(",");
if (getInputLocation() != null)
sb.append("InputLocation: ").append(getInputLocation()).append(",");
if (getRequestTTLSeconds() != null)
sb.append("RequestTTLSeconds: ").append(getRequestTTLSeconds()).append(",");
if (getInvocationTimeoutSeconds() != null)
sb.append("InvocationTimeoutSeconds: ").append(getInvocationTimeoutSeconds());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof InvokeEndpointAsyncRequest == false)
return false;
InvokeEndpointAsyncRequest other = (InvokeEndpointAsyncRequest) obj;
if (other.getEndpointName() == null ^ this.getEndpointName() == null)
return false;
if (other.getEndpointName() != null && other.getEndpointName().equals(this.getEndpointName()) == false)
return false;
if (other.getContentType() == null ^ this.getContentType() == null)
return false;
if (other.getContentType() != null && other.getContentType().equals(this.getContentType()) == false)
return false;
if (other.getAccept() == null ^ this.getAccept() == null)
return false;
if (other.getAccept() != null && other.getAccept().equals(this.getAccept()) == false)
return false;
if (other.getCustomAttributes() == null ^ this.getCustomAttributes() == null)
return false;
if (other.getCustomAttributes() != null && other.getCustomAttributes().equals(this.getCustomAttributes()) == false)
return false;
if (other.getInferenceId() == null ^ this.getInferenceId() == null)
return false;
if (other.getInferenceId() != null && other.getInferenceId().equals(this.getInferenceId()) == false)
return false;
if (other.getInputLocation() == null ^ this.getInputLocation() == null)
return false;
if (other.getInputLocation() != null && other.getInputLocation().equals(this.getInputLocation()) == false)
return false;
if (other.getRequestTTLSeconds() == null ^ this.getRequestTTLSeconds() == null)
return false;
if (other.getRequestTTLSeconds() != null && other.getRequestTTLSeconds().equals(this.getRequestTTLSeconds()) == false)
return false;
if (other.getInvocationTimeoutSeconds() == null ^ this.getInvocationTimeoutSeconds() == null)
return false;
if (other.getInvocationTimeoutSeconds() != null && other.getInvocationTimeoutSeconds().equals(this.getInvocationTimeoutSeconds()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEndpointName() == null) ? 0 : getEndpointName().hashCode());
hashCode = prime * hashCode + ((getContentType() == null) ? 0 : getContentType().hashCode());
hashCode = prime * hashCode + ((getAccept() == null) ? 0 : getAccept().hashCode());
hashCode = prime * hashCode + ((getCustomAttributes() == null) ? 0 : getCustomAttributes().hashCode());
hashCode = prime * hashCode + ((getInferenceId() == null) ? 0 : getInferenceId().hashCode());
hashCode = prime * hashCode + ((getInputLocation() == null) ? 0 : getInputLocation().hashCode());
hashCode = prime * hashCode + ((getRequestTTLSeconds() == null) ? 0 : getRequestTTLSeconds().hashCode());
hashCode = prime * hashCode + ((getInvocationTimeoutSeconds() == null) ? 0 : getInvocationTimeoutSeconds().hashCode());
return hashCode;
}
@Override
public InvokeEndpointAsyncRequest clone() {
return (InvokeEndpointAsyncRequest) super.clone();
}
}