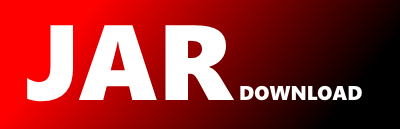
com.amazonaws.services.sagemakerruntime.model.InvokeEndpointRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-sagemakerruntime Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.sagemakerruntime.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class InvokeEndpointRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*
*/
private String endpointName;
/**
*
* Provides input data, in the format specified in the ContentType
request header. Amazon SageMaker
* passes all of the data in the body to the model.
*
*
* For information about the format of the request body, see Common Data Formats-Inference.
*
*/
private java.nio.ByteBuffer body;
/**
*
* The MIME type of the input data in the request body.
*
*/
private String contentType;
/**
*
* The desired MIME type of the inference response from the model container.
*
*/
private String accept;
/**
*
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to provide an ID that you can use to track a request or to provide other metadata that a service
* endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII characters as
* specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*/
private String customAttributes;
/**
*
* The model to request for inference when invoking a multi-model endpoint.
*
*/
private String targetModel;
/**
*
* Specify the production variant to send the inference request to when invoking an endpoint that is running two or
* more variants. Note that this parameter overrides the default behavior for the endpoint, which is to distribute
* the invocation traffic based on the variant weights.
*
*
* For information about how to use variant targeting to perform a/b testing, see Test models in production
*
*/
private String targetVariant;
/**
*
* If the endpoint hosts multiple containers and is configured to use direct invocation, this parameter specifies
* the host name of the container to invoke.
*
*/
private String targetContainerHostname;
/**
*
* If you provide a value, it is added to the captured data when you enable data capture on the endpoint. For
* information about data capture, see Capture Data.
*
*/
private String inferenceId;
/**
*
* An optional JMESPath expression used to override the EnableExplanations
parameter of the
* ClarifyExplainerConfig
API. See the EnableExplanations section in the developer guide for more information.
*
*/
private String enableExplanations;
/**
*
* If the endpoint hosts one or more inference components, this parameter specifies the name of inference component
* to invoke.
*
*/
private String inferenceComponentName;
/**
*
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*
*
* @param endpointName
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*/
public void setEndpointName(String endpointName) {
this.endpointName = endpointName;
}
/**
*
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*
*
* @return The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*/
public String getEndpointName() {
return this.endpointName;
}
/**
*
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
*
*
* @param endpointName
* The name of the endpoint that you specified when you created the endpoint using the CreateEndpoint API.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withEndpointName(String endpointName) {
setEndpointName(endpointName);
return this;
}
/**
*
* Provides input data, in the format specified in the ContentType
request header. Amazon SageMaker
* passes all of the data in the body to the model.
*
*
* For information about the format of the request body, see Common Data Formats-Inference.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param body
* Provides input data, in the format specified in the ContentType
request header. Amazon
* SageMaker passes all of the data in the body to the model.
*
* For information about the format of the request body, see Common Data
* Formats-Inference.
*/
public void setBody(java.nio.ByteBuffer body) {
this.body = body;
}
/**
*
* Provides input data, in the format specified in the ContentType
request header. Amazon SageMaker
* passes all of the data in the body to the model.
*
*
* For information about the format of the request body, see Common Data Formats-Inference.
*
*
* {@code ByteBuffer}s are stateful. Calling their {@code get} methods changes their {@code position}. We recommend
* using {@link java.nio.ByteBuffer#asReadOnlyBuffer()} to create a read-only view of the buffer with an independent
* {@code position}, and calling {@code get} methods on this rather than directly on the returned {@code ByteBuffer}.
* Doing so will ensure that anyone else using the {@code ByteBuffer} will not be affected by changes to the
* {@code position}.
*
*
* @return Provides input data, in the format specified in the ContentType
request header. Amazon
* SageMaker passes all of the data in the body to the model.
*
* For information about the format of the request body, see Common Data
* Formats-Inference.
*/
public java.nio.ByteBuffer getBody() {
return this.body;
}
/**
*
* Provides input data, in the format specified in the ContentType
request header. Amazon SageMaker
* passes all of the data in the body to the model.
*
*
* For information about the format of the request body, see Common Data Formats-Inference.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param body
* Provides input data, in the format specified in the ContentType
request header. Amazon
* SageMaker passes all of the data in the body to the model.
*
* For information about the format of the request body, see Common Data
* Formats-Inference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withBody(java.nio.ByteBuffer body) {
setBody(body);
return this;
}
/**
*
* The MIME type of the input data in the request body.
*
*
* @param contentType
* The MIME type of the input data in the request body.
*/
public void setContentType(String contentType) {
this.contentType = contentType;
}
/**
*
* The MIME type of the input data in the request body.
*
*
* @return The MIME type of the input data in the request body.
*/
public String getContentType() {
return this.contentType;
}
/**
*
* The MIME type of the input data in the request body.
*
*
* @param contentType
* The MIME type of the input data in the request body.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withContentType(String contentType) {
setContentType(contentType);
return this;
}
/**
*
* The desired MIME type of the inference response from the model container.
*
*
* @param accept
* The desired MIME type of the inference response from the model container.
*/
public void setAccept(String accept) {
this.accept = accept;
}
/**
*
* The desired MIME type of the inference response from the model container.
*
*
* @return The desired MIME type of the inference response from the model container.
*/
public String getAccept() {
return this.accept;
}
/**
*
* The desired MIME type of the inference response from the model container.
*
*
* @param accept
* The desired MIME type of the inference response from the model container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withAccept(String accept) {
setAccept(accept);
return this;
}
/**
*
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to provide an ID that you can use to track a request or to provide other metadata that a service
* endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII characters as
* specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*
* @param customAttributes
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this
* value, for example, to provide an ID that you can use to track a request or to provide other metadata that
* a service endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII
* characters as specified in Section
* 3.3.6. Field Value Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If
* your code does not set this value in the response, an empty value is returned. For example, if a custom
* attribute represents the trace ID, your model can prepend the custom attribute with Trace ID:
* in your post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python
* SDK.
*/
public void setCustomAttributes(String customAttributes) {
this.customAttributes = customAttributes;
}
/**
*
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to provide an ID that you can use to track a request or to provide other metadata that a service
* endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII characters as
* specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*
* @return Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this
* value, for example, to provide an ID that you can use to track a request or to provide other metadata
* that a service endpoint was programmed to process. The value must consist of no more than 1024 visible
* US-ASCII characters as specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If
* your code does not set this value in the response, an empty value is returned. For example, if a custom
* attribute represents the trace ID, your model can prepend the custom attribute with
* Trace ID:
in your post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker
* Python SDK.
*/
public String getCustomAttributes() {
return this.customAttributes;
}
/**
*
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this value, for
* example, to provide an ID that you can use to track a request or to provide other metadata that a service
* endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII characters as
* specified in Section 3.3.6. Field Value
* Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If your code
* does not set this value in the response, an empty value is returned. For example, if a custom attribute
* represents the trace ID, your model can prepend the custom attribute with Trace ID:
in your
* post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python SDK.
*
*
* @param customAttributes
* Provides additional information about a request for an inference submitted to a model hosted at an Amazon
* SageMaker endpoint. The information is an opaque value that is forwarded verbatim. You could use this
* value, for example, to provide an ID that you can use to track a request or to provide other metadata that
* a service endpoint was programmed to process. The value must consist of no more than 1024 visible US-ASCII
* characters as specified in Section
* 3.3.6. Field Value Components of the Hypertext Transfer Protocol (HTTP/1.1).
*
* The code in your model is responsible for setting or updating any custom attributes in the response. If
* your code does not set this value in the response, an empty value is returned. For example, if a custom
* attribute represents the trace ID, your model can prepend the custom attribute with Trace ID:
* in your post-processing function.
*
*
* This feature is currently supported in the Amazon Web Services SDKs but not in the Amazon SageMaker Python
* SDK.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withCustomAttributes(String customAttributes) {
setCustomAttributes(customAttributes);
return this;
}
/**
*
* The model to request for inference when invoking a multi-model endpoint.
*
*
* @param targetModel
* The model to request for inference when invoking a multi-model endpoint.
*/
public void setTargetModel(String targetModel) {
this.targetModel = targetModel;
}
/**
*
* The model to request for inference when invoking a multi-model endpoint.
*
*
* @return The model to request for inference when invoking a multi-model endpoint.
*/
public String getTargetModel() {
return this.targetModel;
}
/**
*
* The model to request for inference when invoking a multi-model endpoint.
*
*
* @param targetModel
* The model to request for inference when invoking a multi-model endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withTargetModel(String targetModel) {
setTargetModel(targetModel);
return this;
}
/**
*
* Specify the production variant to send the inference request to when invoking an endpoint that is running two or
* more variants. Note that this parameter overrides the default behavior for the endpoint, which is to distribute
* the invocation traffic based on the variant weights.
*
*
* For information about how to use variant targeting to perform a/b testing, see Test models in production
*
*
* @param targetVariant
* Specify the production variant to send the inference request to when invoking an endpoint that is running
* two or more variants. Note that this parameter overrides the default behavior for the endpoint, which is
* to distribute the invocation traffic based on the variant weights.
*
* For information about how to use variant targeting to perform a/b testing, see Test models in production
*/
public void setTargetVariant(String targetVariant) {
this.targetVariant = targetVariant;
}
/**
*
* Specify the production variant to send the inference request to when invoking an endpoint that is running two or
* more variants. Note that this parameter overrides the default behavior for the endpoint, which is to distribute
* the invocation traffic based on the variant weights.
*
*
* For information about how to use variant targeting to perform a/b testing, see Test models in production
*
*
* @return Specify the production variant to send the inference request to when invoking an endpoint that is running
* two or more variants. Note that this parameter overrides the default behavior for the endpoint, which is
* to distribute the invocation traffic based on the variant weights.
*
* For information about how to use variant targeting to perform a/b testing, see Test models in
* production
*/
public String getTargetVariant() {
return this.targetVariant;
}
/**
*
* Specify the production variant to send the inference request to when invoking an endpoint that is running two or
* more variants. Note that this parameter overrides the default behavior for the endpoint, which is to distribute
* the invocation traffic based on the variant weights.
*
*
* For information about how to use variant targeting to perform a/b testing, see Test models in production
*
*
* @param targetVariant
* Specify the production variant to send the inference request to when invoking an endpoint that is running
* two or more variants. Note that this parameter overrides the default behavior for the endpoint, which is
* to distribute the invocation traffic based on the variant weights.
*
* For information about how to use variant targeting to perform a/b testing, see Test models in production
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withTargetVariant(String targetVariant) {
setTargetVariant(targetVariant);
return this;
}
/**
*
* If the endpoint hosts multiple containers and is configured to use direct invocation, this parameter specifies
* the host name of the container to invoke.
*
*
* @param targetContainerHostname
* If the endpoint hosts multiple containers and is configured to use direct invocation, this parameter
* specifies the host name of the container to invoke.
*/
public void setTargetContainerHostname(String targetContainerHostname) {
this.targetContainerHostname = targetContainerHostname;
}
/**
*
* If the endpoint hosts multiple containers and is configured to use direct invocation, this parameter specifies
* the host name of the container to invoke.
*
*
* @return If the endpoint hosts multiple containers and is configured to use direct invocation, this parameter
* specifies the host name of the container to invoke.
*/
public String getTargetContainerHostname() {
return this.targetContainerHostname;
}
/**
*
* If the endpoint hosts multiple containers and is configured to use direct invocation, this parameter specifies
* the host name of the container to invoke.
*
*
* @param targetContainerHostname
* If the endpoint hosts multiple containers and is configured to use direct invocation, this parameter
* specifies the host name of the container to invoke.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withTargetContainerHostname(String targetContainerHostname) {
setTargetContainerHostname(targetContainerHostname);
return this;
}
/**
*
* If you provide a value, it is added to the captured data when you enable data capture on the endpoint. For
* information about data capture, see Capture Data.
*
*
* @param inferenceId
* If you provide a value, it is added to the captured data when you enable data capture on the endpoint. For
* information about data capture, see Capture Data.
*/
public void setInferenceId(String inferenceId) {
this.inferenceId = inferenceId;
}
/**
*
* If you provide a value, it is added to the captured data when you enable data capture on the endpoint. For
* information about data capture, see Capture Data.
*
*
* @return If you provide a value, it is added to the captured data when you enable data capture on the endpoint.
* For information about data capture, see Capture Data.
*/
public String getInferenceId() {
return this.inferenceId;
}
/**
*
* If you provide a value, it is added to the captured data when you enable data capture on the endpoint. For
* information about data capture, see Capture Data.
*
*
* @param inferenceId
* If you provide a value, it is added to the captured data when you enable data capture on the endpoint. For
* information about data capture, see Capture Data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withInferenceId(String inferenceId) {
setInferenceId(inferenceId);
return this;
}
/**
*
* An optional JMESPath expression used to override the EnableExplanations
parameter of the
* ClarifyExplainerConfig
API. See the EnableExplanations section in the developer guide for more information.
*
*
* @param enableExplanations
* An optional JMESPath expression used to override the EnableExplanations
parameter of the
* ClarifyExplainerConfig
API. See the EnableExplanations section in the developer guide for more information.
*/
public void setEnableExplanations(String enableExplanations) {
this.enableExplanations = enableExplanations;
}
/**
*
* An optional JMESPath expression used to override the EnableExplanations
parameter of the
* ClarifyExplainerConfig
API. See the EnableExplanations section in the developer guide for more information.
*
*
* @return An optional JMESPath expression used to override the EnableExplanations
parameter of the
* ClarifyExplainerConfig
API. See the EnableExplanations section in the developer guide for more information.
*/
public String getEnableExplanations() {
return this.enableExplanations;
}
/**
*
* An optional JMESPath expression used to override the EnableExplanations
parameter of the
* ClarifyExplainerConfig
API. See the EnableExplanations section in the developer guide for more information.
*
*
* @param enableExplanations
* An optional JMESPath expression used to override the EnableExplanations
parameter of the
* ClarifyExplainerConfig
API. See the EnableExplanations section in the developer guide for more information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withEnableExplanations(String enableExplanations) {
setEnableExplanations(enableExplanations);
return this;
}
/**
*
* If the endpoint hosts one or more inference components, this parameter specifies the name of inference component
* to invoke.
*
*
* @param inferenceComponentName
* If the endpoint hosts one or more inference components, this parameter specifies the name of inference
* component to invoke.
*/
public void setInferenceComponentName(String inferenceComponentName) {
this.inferenceComponentName = inferenceComponentName;
}
/**
*
* If the endpoint hosts one or more inference components, this parameter specifies the name of inference component
* to invoke.
*
*
* @return If the endpoint hosts one or more inference components, this parameter specifies the name of inference
* component to invoke.
*/
public String getInferenceComponentName() {
return this.inferenceComponentName;
}
/**
*
* If the endpoint hosts one or more inference components, this parameter specifies the name of inference component
* to invoke.
*
*
* @param inferenceComponentName
* If the endpoint hosts one or more inference components, this parameter specifies the name of inference
* component to invoke.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public InvokeEndpointRequest withInferenceComponentName(String inferenceComponentName) {
setInferenceComponentName(inferenceComponentName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEndpointName() != null)
sb.append("EndpointName: ").append(getEndpointName()).append(",");
if (getBody() != null)
sb.append("Body: ").append("***Sensitive Data Redacted***").append(",");
if (getContentType() != null)
sb.append("ContentType: ").append(getContentType()).append(",");
if (getAccept() != null)
sb.append("Accept: ").append(getAccept()).append(",");
if (getCustomAttributes() != null)
sb.append("CustomAttributes: ").append("***Sensitive Data Redacted***").append(",");
if (getTargetModel() != null)
sb.append("TargetModel: ").append(getTargetModel()).append(",");
if (getTargetVariant() != null)
sb.append("TargetVariant: ").append(getTargetVariant()).append(",");
if (getTargetContainerHostname() != null)
sb.append("TargetContainerHostname: ").append(getTargetContainerHostname()).append(",");
if (getInferenceId() != null)
sb.append("InferenceId: ").append(getInferenceId()).append(",");
if (getEnableExplanations() != null)
sb.append("EnableExplanations: ").append(getEnableExplanations()).append(",");
if (getInferenceComponentName() != null)
sb.append("InferenceComponentName: ").append(getInferenceComponentName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof InvokeEndpointRequest == false)
return false;
InvokeEndpointRequest other = (InvokeEndpointRequest) obj;
if (other.getEndpointName() == null ^ this.getEndpointName() == null)
return false;
if (other.getEndpointName() != null && other.getEndpointName().equals(this.getEndpointName()) == false)
return false;
if (other.getBody() == null ^ this.getBody() == null)
return false;
if (other.getBody() != null && other.getBody().equals(this.getBody()) == false)
return false;
if (other.getContentType() == null ^ this.getContentType() == null)
return false;
if (other.getContentType() != null && other.getContentType().equals(this.getContentType()) == false)
return false;
if (other.getAccept() == null ^ this.getAccept() == null)
return false;
if (other.getAccept() != null && other.getAccept().equals(this.getAccept()) == false)
return false;
if (other.getCustomAttributes() == null ^ this.getCustomAttributes() == null)
return false;
if (other.getCustomAttributes() != null && other.getCustomAttributes().equals(this.getCustomAttributes()) == false)
return false;
if (other.getTargetModel() == null ^ this.getTargetModel() == null)
return false;
if (other.getTargetModel() != null && other.getTargetModel().equals(this.getTargetModel()) == false)
return false;
if (other.getTargetVariant() == null ^ this.getTargetVariant() == null)
return false;
if (other.getTargetVariant() != null && other.getTargetVariant().equals(this.getTargetVariant()) == false)
return false;
if (other.getTargetContainerHostname() == null ^ this.getTargetContainerHostname() == null)
return false;
if (other.getTargetContainerHostname() != null && other.getTargetContainerHostname().equals(this.getTargetContainerHostname()) == false)
return false;
if (other.getInferenceId() == null ^ this.getInferenceId() == null)
return false;
if (other.getInferenceId() != null && other.getInferenceId().equals(this.getInferenceId()) == false)
return false;
if (other.getEnableExplanations() == null ^ this.getEnableExplanations() == null)
return false;
if (other.getEnableExplanations() != null && other.getEnableExplanations().equals(this.getEnableExplanations()) == false)
return false;
if (other.getInferenceComponentName() == null ^ this.getInferenceComponentName() == null)
return false;
if (other.getInferenceComponentName() != null && other.getInferenceComponentName().equals(this.getInferenceComponentName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEndpointName() == null) ? 0 : getEndpointName().hashCode());
hashCode = prime * hashCode + ((getBody() == null) ? 0 : getBody().hashCode());
hashCode = prime * hashCode + ((getContentType() == null) ? 0 : getContentType().hashCode());
hashCode = prime * hashCode + ((getAccept() == null) ? 0 : getAccept().hashCode());
hashCode = prime * hashCode + ((getCustomAttributes() == null) ? 0 : getCustomAttributes().hashCode());
hashCode = prime * hashCode + ((getTargetModel() == null) ? 0 : getTargetModel().hashCode());
hashCode = prime * hashCode + ((getTargetVariant() == null) ? 0 : getTargetVariant().hashCode());
hashCode = prime * hashCode + ((getTargetContainerHostname() == null) ? 0 : getTargetContainerHostname().hashCode());
hashCode = prime * hashCode + ((getInferenceId() == null) ? 0 : getInferenceId().hashCode());
hashCode = prime * hashCode + ((getEnableExplanations() == null) ? 0 : getEnableExplanations().hashCode());
hashCode = prime * hashCode + ((getInferenceComponentName() == null) ? 0 : getInferenceComponentName().hashCode());
return hashCode;
}
@Override
public InvokeEndpointRequest clone() {
return (InvokeEndpointRequest) super.clone();
}
}