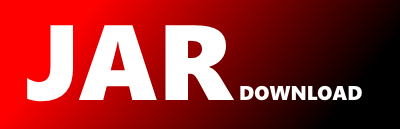
com.amazonaws.services.savingsplans.model.DescribeSavingsPlansOfferingsRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-savingsplans Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.savingsplans.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeSavingsPlansOfferingsRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The IDs of the offerings.
*
*/
private java.util.List offeringIds;
/**
*
* The payment options.
*
*/
private java.util.List paymentOptions;
/**
*
* The product type.
*
*/
private String productType;
/**
*
* The plan type.
*
*/
private java.util.List planTypes;
/**
*
* The durations, in seconds.
*
*/
private java.util.List durations;
/**
*
* The currencies.
*
*/
private java.util.List currencies;
/**
*
* The descriptions.
*
*/
private java.util.List descriptions;
/**
*
* The services.
*
*/
private java.util.List serviceCodes;
/**
*
* The usage details of the line item in the billing report.
*
*/
private java.util.List usageTypes;
/**
*
* The specific AWS operation for the line item in the billing report.
*
*/
private java.util.List operations;
/**
*
* The filters.
*
*/
private java.util.List filters;
/**
*
* The token for the next page of results.
*
*/
private String nextToken;
/**
*
* The maximum number of results to return with a single call. To retrieve additional results, make another call
* with the returned token value.
*
*/
private Integer maxResults;
/**
*
* The IDs of the offerings.
*
*
* @return The IDs of the offerings.
*/
public java.util.List getOfferingIds() {
return offeringIds;
}
/**
*
* The IDs of the offerings.
*
*
* @param offeringIds
* The IDs of the offerings.
*/
public void setOfferingIds(java.util.Collection offeringIds) {
if (offeringIds == null) {
this.offeringIds = null;
return;
}
this.offeringIds = new java.util.ArrayList(offeringIds);
}
/**
*
* The IDs of the offerings.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOfferingIds(java.util.Collection)} or {@link #withOfferingIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param offeringIds
* The IDs of the offerings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withOfferingIds(String... offeringIds) {
if (this.offeringIds == null) {
setOfferingIds(new java.util.ArrayList(offeringIds.length));
}
for (String ele : offeringIds) {
this.offeringIds.add(ele);
}
return this;
}
/**
*
* The IDs of the offerings.
*
*
* @param offeringIds
* The IDs of the offerings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withOfferingIds(java.util.Collection offeringIds) {
setOfferingIds(offeringIds);
return this;
}
/**
*
* The payment options.
*
*
* @return The payment options.
* @see SavingsPlanPaymentOption
*/
public java.util.List getPaymentOptions() {
return paymentOptions;
}
/**
*
* The payment options.
*
*
* @param paymentOptions
* The payment options.
* @see SavingsPlanPaymentOption
*/
public void setPaymentOptions(java.util.Collection paymentOptions) {
if (paymentOptions == null) {
this.paymentOptions = null;
return;
}
this.paymentOptions = new java.util.ArrayList(paymentOptions);
}
/**
*
* The payment options.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPaymentOptions(java.util.Collection)} or {@link #withPaymentOptions(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param paymentOptions
* The payment options.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanPaymentOption
*/
public DescribeSavingsPlansOfferingsRequest withPaymentOptions(String... paymentOptions) {
if (this.paymentOptions == null) {
setPaymentOptions(new java.util.ArrayList(paymentOptions.length));
}
for (String ele : paymentOptions) {
this.paymentOptions.add(ele);
}
return this;
}
/**
*
* The payment options.
*
*
* @param paymentOptions
* The payment options.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanPaymentOption
*/
public DescribeSavingsPlansOfferingsRequest withPaymentOptions(java.util.Collection paymentOptions) {
setPaymentOptions(paymentOptions);
return this;
}
/**
*
* The payment options.
*
*
* @param paymentOptions
* The payment options.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanPaymentOption
*/
public DescribeSavingsPlansOfferingsRequest withPaymentOptions(SavingsPlanPaymentOption... paymentOptions) {
java.util.ArrayList paymentOptionsCopy = new java.util.ArrayList(paymentOptions.length);
for (SavingsPlanPaymentOption value : paymentOptions) {
paymentOptionsCopy.add(value.toString());
}
if (getPaymentOptions() == null) {
setPaymentOptions(paymentOptionsCopy);
} else {
getPaymentOptions().addAll(paymentOptionsCopy);
}
return this;
}
/**
*
* The product type.
*
*
* @param productType
* The product type.
* @see SavingsPlanProductType
*/
public void setProductType(String productType) {
this.productType = productType;
}
/**
*
* The product type.
*
*
* @return The product type.
* @see SavingsPlanProductType
*/
public String getProductType() {
return this.productType;
}
/**
*
* The product type.
*
*
* @param productType
* The product type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanProductType
*/
public DescribeSavingsPlansOfferingsRequest withProductType(String productType) {
setProductType(productType);
return this;
}
/**
*
* The product type.
*
*
* @param productType
* The product type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanProductType
*/
public DescribeSavingsPlansOfferingsRequest withProductType(SavingsPlanProductType productType) {
this.productType = productType.toString();
return this;
}
/**
*
* The plan type.
*
*
* @return The plan type.
* @see SavingsPlanType
*/
public java.util.List getPlanTypes() {
return planTypes;
}
/**
*
* The plan type.
*
*
* @param planTypes
* The plan type.
* @see SavingsPlanType
*/
public void setPlanTypes(java.util.Collection planTypes) {
if (planTypes == null) {
this.planTypes = null;
return;
}
this.planTypes = new java.util.ArrayList(planTypes);
}
/**
*
* The plan type.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlanTypes(java.util.Collection)} or {@link #withPlanTypes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param planTypes
* The plan type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanType
*/
public DescribeSavingsPlansOfferingsRequest withPlanTypes(String... planTypes) {
if (this.planTypes == null) {
setPlanTypes(new java.util.ArrayList(planTypes.length));
}
for (String ele : planTypes) {
this.planTypes.add(ele);
}
return this;
}
/**
*
* The plan type.
*
*
* @param planTypes
* The plan type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanType
*/
public DescribeSavingsPlansOfferingsRequest withPlanTypes(java.util.Collection planTypes) {
setPlanTypes(planTypes);
return this;
}
/**
*
* The plan type.
*
*
* @param planTypes
* The plan type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanType
*/
public DescribeSavingsPlansOfferingsRequest withPlanTypes(SavingsPlanType... planTypes) {
java.util.ArrayList planTypesCopy = new java.util.ArrayList(planTypes.length);
for (SavingsPlanType value : planTypes) {
planTypesCopy.add(value.toString());
}
if (getPlanTypes() == null) {
setPlanTypes(planTypesCopy);
} else {
getPlanTypes().addAll(planTypesCopy);
}
return this;
}
/**
*
* The durations, in seconds.
*
*
* @return The durations, in seconds.
*/
public java.util.List getDurations() {
return durations;
}
/**
*
* The durations, in seconds.
*
*
* @param durations
* The durations, in seconds.
*/
public void setDurations(java.util.Collection durations) {
if (durations == null) {
this.durations = null;
return;
}
this.durations = new java.util.ArrayList(durations);
}
/**
*
* The durations, in seconds.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDurations(java.util.Collection)} or {@link #withDurations(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param durations
* The durations, in seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withDurations(Long... durations) {
if (this.durations == null) {
setDurations(new java.util.ArrayList(durations.length));
}
for (Long ele : durations) {
this.durations.add(ele);
}
return this;
}
/**
*
* The durations, in seconds.
*
*
* @param durations
* The durations, in seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withDurations(java.util.Collection durations) {
setDurations(durations);
return this;
}
/**
*
* The currencies.
*
*
* @return The currencies.
* @see CurrencyCode
*/
public java.util.List getCurrencies() {
return currencies;
}
/**
*
* The currencies.
*
*
* @param currencies
* The currencies.
* @see CurrencyCode
*/
public void setCurrencies(java.util.Collection currencies) {
if (currencies == null) {
this.currencies = null;
return;
}
this.currencies = new java.util.ArrayList(currencies);
}
/**
*
* The currencies.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCurrencies(java.util.Collection)} or {@link #withCurrencies(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param currencies
* The currencies.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CurrencyCode
*/
public DescribeSavingsPlansOfferingsRequest withCurrencies(String... currencies) {
if (this.currencies == null) {
setCurrencies(new java.util.ArrayList(currencies.length));
}
for (String ele : currencies) {
this.currencies.add(ele);
}
return this;
}
/**
*
* The currencies.
*
*
* @param currencies
* The currencies.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CurrencyCode
*/
public DescribeSavingsPlansOfferingsRequest withCurrencies(java.util.Collection currencies) {
setCurrencies(currencies);
return this;
}
/**
*
* The currencies.
*
*
* @param currencies
* The currencies.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CurrencyCode
*/
public DescribeSavingsPlansOfferingsRequest withCurrencies(CurrencyCode... currencies) {
java.util.ArrayList currenciesCopy = new java.util.ArrayList(currencies.length);
for (CurrencyCode value : currencies) {
currenciesCopy.add(value.toString());
}
if (getCurrencies() == null) {
setCurrencies(currenciesCopy);
} else {
getCurrencies().addAll(currenciesCopy);
}
return this;
}
/**
*
* The descriptions.
*
*
* @return The descriptions.
*/
public java.util.List getDescriptions() {
return descriptions;
}
/**
*
* The descriptions.
*
*
* @param descriptions
* The descriptions.
*/
public void setDescriptions(java.util.Collection descriptions) {
if (descriptions == null) {
this.descriptions = null;
return;
}
this.descriptions = new java.util.ArrayList(descriptions);
}
/**
*
* The descriptions.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDescriptions(java.util.Collection)} or {@link #withDescriptions(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param descriptions
* The descriptions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withDescriptions(String... descriptions) {
if (this.descriptions == null) {
setDescriptions(new java.util.ArrayList(descriptions.length));
}
for (String ele : descriptions) {
this.descriptions.add(ele);
}
return this;
}
/**
*
* The descriptions.
*
*
* @param descriptions
* The descriptions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withDescriptions(java.util.Collection descriptions) {
setDescriptions(descriptions);
return this;
}
/**
*
* The services.
*
*
* @return The services.
*/
public java.util.List getServiceCodes() {
return serviceCodes;
}
/**
*
* The services.
*
*
* @param serviceCodes
* The services.
*/
public void setServiceCodes(java.util.Collection serviceCodes) {
if (serviceCodes == null) {
this.serviceCodes = null;
return;
}
this.serviceCodes = new java.util.ArrayList(serviceCodes);
}
/**
*
* The services.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServiceCodes(java.util.Collection)} or {@link #withServiceCodes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param serviceCodes
* The services.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withServiceCodes(String... serviceCodes) {
if (this.serviceCodes == null) {
setServiceCodes(new java.util.ArrayList(serviceCodes.length));
}
for (String ele : serviceCodes) {
this.serviceCodes.add(ele);
}
return this;
}
/**
*
* The services.
*
*
* @param serviceCodes
* The services.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withServiceCodes(java.util.Collection serviceCodes) {
setServiceCodes(serviceCodes);
return this;
}
/**
*
* The usage details of the line item in the billing report.
*
*
* @return The usage details of the line item in the billing report.
*/
public java.util.List getUsageTypes() {
return usageTypes;
}
/**
*
* The usage details of the line item in the billing report.
*
*
* @param usageTypes
* The usage details of the line item in the billing report.
*/
public void setUsageTypes(java.util.Collection usageTypes) {
if (usageTypes == null) {
this.usageTypes = null;
return;
}
this.usageTypes = new java.util.ArrayList(usageTypes);
}
/**
*
* The usage details of the line item in the billing report.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUsageTypes(java.util.Collection)} or {@link #withUsageTypes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param usageTypes
* The usage details of the line item in the billing report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withUsageTypes(String... usageTypes) {
if (this.usageTypes == null) {
setUsageTypes(new java.util.ArrayList(usageTypes.length));
}
for (String ele : usageTypes) {
this.usageTypes.add(ele);
}
return this;
}
/**
*
* The usage details of the line item in the billing report.
*
*
* @param usageTypes
* The usage details of the line item in the billing report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withUsageTypes(java.util.Collection usageTypes) {
setUsageTypes(usageTypes);
return this;
}
/**
*
* The specific AWS operation for the line item in the billing report.
*
*
* @return The specific AWS operation for the line item in the billing report.
*/
public java.util.List getOperations() {
return operations;
}
/**
*
* The specific AWS operation for the line item in the billing report.
*
*
* @param operations
* The specific AWS operation for the line item in the billing report.
*/
public void setOperations(java.util.Collection operations) {
if (operations == null) {
this.operations = null;
return;
}
this.operations = new java.util.ArrayList(operations);
}
/**
*
* The specific AWS operation for the line item in the billing report.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOperations(java.util.Collection)} or {@link #withOperations(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param operations
* The specific AWS operation for the line item in the billing report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withOperations(String... operations) {
if (this.operations == null) {
setOperations(new java.util.ArrayList(operations.length));
}
for (String ele : operations) {
this.operations.add(ele);
}
return this;
}
/**
*
* The specific AWS operation for the line item in the billing report.
*
*
* @param operations
* The specific AWS operation for the line item in the billing report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withOperations(java.util.Collection operations) {
setOperations(operations);
return this;
}
/**
*
* The filters.
*
*
* @return The filters.
*/
public java.util.List getFilters() {
return filters;
}
/**
*
* The filters.
*
*
* @param filters
* The filters.
*/
public void setFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
return;
}
this.filters = new java.util.ArrayList(filters);
}
/**
*
* The filters.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFilters(java.util.Collection)} or {@link #withFilters(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param filters
* The filters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withFilters(SavingsPlanOfferingFilterElement... filters) {
if (this.filters == null) {
setFilters(new java.util.ArrayList(filters.length));
}
for (SavingsPlanOfferingFilterElement ele : filters) {
this.filters.add(ele);
}
return this;
}
/**
*
* The filters.
*
*
* @param filters
* The filters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withFilters(java.util.Collection filters) {
setFilters(filters);
return this;
}
/**
*
* The token for the next page of results.
*
*
* @param nextToken
* The token for the next page of results.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* The token for the next page of results.
*
*
* @return The token for the next page of results.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* The token for the next page of results.
*
*
* @param nextToken
* The token for the next page of results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* The maximum number of results to return with a single call. To retrieve additional results, make another call
* with the returned token value.
*
*
* @param maxResults
* The maximum number of results to return with a single call. To retrieve additional results, make another
* call with the returned token value.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of results to return with a single call. To retrieve additional results, make another call
* with the returned token value.
*
*
* @return The maximum number of results to return with a single call. To retrieve additional results, make another
* call with the returned token value.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of results to return with a single call. To retrieve additional results, make another call
* with the returned token value.
*
*
* @param maxResults
* The maximum number of results to return with a single call. To retrieve additional results, make another
* call with the returned token value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingsRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOfferingIds() != null)
sb.append("OfferingIds: ").append(getOfferingIds()).append(",");
if (getPaymentOptions() != null)
sb.append("PaymentOptions: ").append(getPaymentOptions()).append(",");
if (getProductType() != null)
sb.append("ProductType: ").append(getProductType()).append(",");
if (getPlanTypes() != null)
sb.append("PlanTypes: ").append(getPlanTypes()).append(",");
if (getDurations() != null)
sb.append("Durations: ").append(getDurations()).append(",");
if (getCurrencies() != null)
sb.append("Currencies: ").append(getCurrencies()).append(",");
if (getDescriptions() != null)
sb.append("Descriptions: ").append(getDescriptions()).append(",");
if (getServiceCodes() != null)
sb.append("ServiceCodes: ").append(getServiceCodes()).append(",");
if (getUsageTypes() != null)
sb.append("UsageTypes: ").append(getUsageTypes()).append(",");
if (getOperations() != null)
sb.append("Operations: ").append(getOperations()).append(",");
if (getFilters() != null)
sb.append("Filters: ").append(getFilters()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeSavingsPlansOfferingsRequest == false)
return false;
DescribeSavingsPlansOfferingsRequest other = (DescribeSavingsPlansOfferingsRequest) obj;
if (other.getOfferingIds() == null ^ this.getOfferingIds() == null)
return false;
if (other.getOfferingIds() != null && other.getOfferingIds().equals(this.getOfferingIds()) == false)
return false;
if (other.getPaymentOptions() == null ^ this.getPaymentOptions() == null)
return false;
if (other.getPaymentOptions() != null && other.getPaymentOptions().equals(this.getPaymentOptions()) == false)
return false;
if (other.getProductType() == null ^ this.getProductType() == null)
return false;
if (other.getProductType() != null && other.getProductType().equals(this.getProductType()) == false)
return false;
if (other.getPlanTypes() == null ^ this.getPlanTypes() == null)
return false;
if (other.getPlanTypes() != null && other.getPlanTypes().equals(this.getPlanTypes()) == false)
return false;
if (other.getDurations() == null ^ this.getDurations() == null)
return false;
if (other.getDurations() != null && other.getDurations().equals(this.getDurations()) == false)
return false;
if (other.getCurrencies() == null ^ this.getCurrencies() == null)
return false;
if (other.getCurrencies() != null && other.getCurrencies().equals(this.getCurrencies()) == false)
return false;
if (other.getDescriptions() == null ^ this.getDescriptions() == null)
return false;
if (other.getDescriptions() != null && other.getDescriptions().equals(this.getDescriptions()) == false)
return false;
if (other.getServiceCodes() == null ^ this.getServiceCodes() == null)
return false;
if (other.getServiceCodes() != null && other.getServiceCodes().equals(this.getServiceCodes()) == false)
return false;
if (other.getUsageTypes() == null ^ this.getUsageTypes() == null)
return false;
if (other.getUsageTypes() != null && other.getUsageTypes().equals(this.getUsageTypes()) == false)
return false;
if (other.getOperations() == null ^ this.getOperations() == null)
return false;
if (other.getOperations() != null && other.getOperations().equals(this.getOperations()) == false)
return false;
if (other.getFilters() == null ^ this.getFilters() == null)
return false;
if (other.getFilters() != null && other.getFilters().equals(this.getFilters()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOfferingIds() == null) ? 0 : getOfferingIds().hashCode());
hashCode = prime * hashCode + ((getPaymentOptions() == null) ? 0 : getPaymentOptions().hashCode());
hashCode = prime * hashCode + ((getProductType() == null) ? 0 : getProductType().hashCode());
hashCode = prime * hashCode + ((getPlanTypes() == null) ? 0 : getPlanTypes().hashCode());
hashCode = prime * hashCode + ((getDurations() == null) ? 0 : getDurations().hashCode());
hashCode = prime * hashCode + ((getCurrencies() == null) ? 0 : getCurrencies().hashCode());
hashCode = prime * hashCode + ((getDescriptions() == null) ? 0 : getDescriptions().hashCode());
hashCode = prime * hashCode + ((getServiceCodes() == null) ? 0 : getServiceCodes().hashCode());
hashCode = prime * hashCode + ((getUsageTypes() == null) ? 0 : getUsageTypes().hashCode());
hashCode = prime * hashCode + ((getOperations() == null) ? 0 : getOperations().hashCode());
hashCode = prime * hashCode + ((getFilters() == null) ? 0 : getFilters().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
return hashCode;
}
@Override
public DescribeSavingsPlansOfferingsRequest clone() {
return (DescribeSavingsPlansOfferingsRequest) super.clone();
}
}