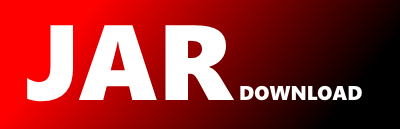
com.amazonaws.services.savingsplans.model.DescribeSavingsPlansOfferingRatesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-savingsplans Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.savingsplans.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeSavingsPlansOfferingRatesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The IDs of the offerings.
*
*/
private java.util.List savingsPlanOfferingIds;
/**
*
* The payment options.
*
*/
private java.util.List savingsPlanPaymentOptions;
/**
*
* The plan types.
*
*/
private java.util.List savingsPlanTypes;
/**
*
* The Amazon Web Services products.
*
*/
private java.util.List products;
/**
*
* The services.
*
*/
private java.util.List serviceCodes;
/**
*
* The usage details of the line item in the billing report.
*
*/
private java.util.List usageTypes;
/**
*
* The specific Amazon Web Services operation for the line item in the billing report.
*
*/
private java.util.List operations;
/**
*
* The filters.
*
*/
private java.util.List filters;
/**
*
* The token for the next page of results.
*
*/
private String nextToken;
/**
*
* The maximum number of results to return with a single call. To retrieve additional results, make another call
* with the returned token value.
*
*/
private Integer maxResults;
/**
*
* The IDs of the offerings.
*
*
* @return The IDs of the offerings.
*/
public java.util.List getSavingsPlanOfferingIds() {
return savingsPlanOfferingIds;
}
/**
*
* The IDs of the offerings.
*
*
* @param savingsPlanOfferingIds
* The IDs of the offerings.
*/
public void setSavingsPlanOfferingIds(java.util.Collection savingsPlanOfferingIds) {
if (savingsPlanOfferingIds == null) {
this.savingsPlanOfferingIds = null;
return;
}
this.savingsPlanOfferingIds = new java.util.ArrayList(savingsPlanOfferingIds);
}
/**
*
* The IDs of the offerings.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSavingsPlanOfferingIds(java.util.Collection)} or
* {@link #withSavingsPlanOfferingIds(java.util.Collection)} if you want to override the existing values.
*
*
* @param savingsPlanOfferingIds
* The IDs of the offerings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withSavingsPlanOfferingIds(String... savingsPlanOfferingIds) {
if (this.savingsPlanOfferingIds == null) {
setSavingsPlanOfferingIds(new java.util.ArrayList(savingsPlanOfferingIds.length));
}
for (String ele : savingsPlanOfferingIds) {
this.savingsPlanOfferingIds.add(ele);
}
return this;
}
/**
*
* The IDs of the offerings.
*
*
* @param savingsPlanOfferingIds
* The IDs of the offerings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withSavingsPlanOfferingIds(java.util.Collection savingsPlanOfferingIds) {
setSavingsPlanOfferingIds(savingsPlanOfferingIds);
return this;
}
/**
*
* The payment options.
*
*
* @return The payment options.
* @see SavingsPlanPaymentOption
*/
public java.util.List getSavingsPlanPaymentOptions() {
return savingsPlanPaymentOptions;
}
/**
*
* The payment options.
*
*
* @param savingsPlanPaymentOptions
* The payment options.
* @see SavingsPlanPaymentOption
*/
public void setSavingsPlanPaymentOptions(java.util.Collection savingsPlanPaymentOptions) {
if (savingsPlanPaymentOptions == null) {
this.savingsPlanPaymentOptions = null;
return;
}
this.savingsPlanPaymentOptions = new java.util.ArrayList(savingsPlanPaymentOptions);
}
/**
*
* The payment options.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSavingsPlanPaymentOptions(java.util.Collection)} or
* {@link #withSavingsPlanPaymentOptions(java.util.Collection)} if you want to override the existing values.
*
*
* @param savingsPlanPaymentOptions
* The payment options.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanPaymentOption
*/
public DescribeSavingsPlansOfferingRatesRequest withSavingsPlanPaymentOptions(String... savingsPlanPaymentOptions) {
if (this.savingsPlanPaymentOptions == null) {
setSavingsPlanPaymentOptions(new java.util.ArrayList(savingsPlanPaymentOptions.length));
}
for (String ele : savingsPlanPaymentOptions) {
this.savingsPlanPaymentOptions.add(ele);
}
return this;
}
/**
*
* The payment options.
*
*
* @param savingsPlanPaymentOptions
* The payment options.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanPaymentOption
*/
public DescribeSavingsPlansOfferingRatesRequest withSavingsPlanPaymentOptions(java.util.Collection savingsPlanPaymentOptions) {
setSavingsPlanPaymentOptions(savingsPlanPaymentOptions);
return this;
}
/**
*
* The payment options.
*
*
* @param savingsPlanPaymentOptions
* The payment options.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanPaymentOption
*/
public DescribeSavingsPlansOfferingRatesRequest withSavingsPlanPaymentOptions(SavingsPlanPaymentOption... savingsPlanPaymentOptions) {
java.util.ArrayList savingsPlanPaymentOptionsCopy = new java.util.ArrayList(savingsPlanPaymentOptions.length);
for (SavingsPlanPaymentOption value : savingsPlanPaymentOptions) {
savingsPlanPaymentOptionsCopy.add(value.toString());
}
if (getSavingsPlanPaymentOptions() == null) {
setSavingsPlanPaymentOptions(savingsPlanPaymentOptionsCopy);
} else {
getSavingsPlanPaymentOptions().addAll(savingsPlanPaymentOptionsCopy);
}
return this;
}
/**
*
* The plan types.
*
*
* @return The plan types.
* @see SavingsPlanType
*/
public java.util.List getSavingsPlanTypes() {
return savingsPlanTypes;
}
/**
*
* The plan types.
*
*
* @param savingsPlanTypes
* The plan types.
* @see SavingsPlanType
*/
public void setSavingsPlanTypes(java.util.Collection savingsPlanTypes) {
if (savingsPlanTypes == null) {
this.savingsPlanTypes = null;
return;
}
this.savingsPlanTypes = new java.util.ArrayList(savingsPlanTypes);
}
/**
*
* The plan types.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSavingsPlanTypes(java.util.Collection)} or {@link #withSavingsPlanTypes(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param savingsPlanTypes
* The plan types.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanType
*/
public DescribeSavingsPlansOfferingRatesRequest withSavingsPlanTypes(String... savingsPlanTypes) {
if (this.savingsPlanTypes == null) {
setSavingsPlanTypes(new java.util.ArrayList(savingsPlanTypes.length));
}
for (String ele : savingsPlanTypes) {
this.savingsPlanTypes.add(ele);
}
return this;
}
/**
*
* The plan types.
*
*
* @param savingsPlanTypes
* The plan types.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanType
*/
public DescribeSavingsPlansOfferingRatesRequest withSavingsPlanTypes(java.util.Collection savingsPlanTypes) {
setSavingsPlanTypes(savingsPlanTypes);
return this;
}
/**
*
* The plan types.
*
*
* @param savingsPlanTypes
* The plan types.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanType
*/
public DescribeSavingsPlansOfferingRatesRequest withSavingsPlanTypes(SavingsPlanType... savingsPlanTypes) {
java.util.ArrayList savingsPlanTypesCopy = new java.util.ArrayList(savingsPlanTypes.length);
for (SavingsPlanType value : savingsPlanTypes) {
savingsPlanTypesCopy.add(value.toString());
}
if (getSavingsPlanTypes() == null) {
setSavingsPlanTypes(savingsPlanTypesCopy);
} else {
getSavingsPlanTypes().addAll(savingsPlanTypesCopy);
}
return this;
}
/**
*
* The Amazon Web Services products.
*
*
* @return The Amazon Web Services products.
* @see SavingsPlanProductType
*/
public java.util.List getProducts() {
return products;
}
/**
*
* The Amazon Web Services products.
*
*
* @param products
* The Amazon Web Services products.
* @see SavingsPlanProductType
*/
public void setProducts(java.util.Collection products) {
if (products == null) {
this.products = null;
return;
}
this.products = new java.util.ArrayList(products);
}
/**
*
* The Amazon Web Services products.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setProducts(java.util.Collection)} or {@link #withProducts(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param products
* The Amazon Web Services products.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanProductType
*/
public DescribeSavingsPlansOfferingRatesRequest withProducts(String... products) {
if (this.products == null) {
setProducts(new java.util.ArrayList(products.length));
}
for (String ele : products) {
this.products.add(ele);
}
return this;
}
/**
*
* The Amazon Web Services products.
*
*
* @param products
* The Amazon Web Services products.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanProductType
*/
public DescribeSavingsPlansOfferingRatesRequest withProducts(java.util.Collection products) {
setProducts(products);
return this;
}
/**
*
* The Amazon Web Services products.
*
*
* @param products
* The Amazon Web Services products.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanProductType
*/
public DescribeSavingsPlansOfferingRatesRequest withProducts(SavingsPlanProductType... products) {
java.util.ArrayList productsCopy = new java.util.ArrayList(products.length);
for (SavingsPlanProductType value : products) {
productsCopy.add(value.toString());
}
if (getProducts() == null) {
setProducts(productsCopy);
} else {
getProducts().addAll(productsCopy);
}
return this;
}
/**
*
* The services.
*
*
* @return The services.
* @see SavingsPlanRateServiceCode
*/
public java.util.List getServiceCodes() {
return serviceCodes;
}
/**
*
* The services.
*
*
* @param serviceCodes
* The services.
* @see SavingsPlanRateServiceCode
*/
public void setServiceCodes(java.util.Collection serviceCodes) {
if (serviceCodes == null) {
this.serviceCodes = null;
return;
}
this.serviceCodes = new java.util.ArrayList(serviceCodes);
}
/**
*
* The services.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServiceCodes(java.util.Collection)} or {@link #withServiceCodes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param serviceCodes
* The services.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanRateServiceCode
*/
public DescribeSavingsPlansOfferingRatesRequest withServiceCodes(String... serviceCodes) {
if (this.serviceCodes == null) {
setServiceCodes(new java.util.ArrayList(serviceCodes.length));
}
for (String ele : serviceCodes) {
this.serviceCodes.add(ele);
}
return this;
}
/**
*
* The services.
*
*
* @param serviceCodes
* The services.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanRateServiceCode
*/
public DescribeSavingsPlansOfferingRatesRequest withServiceCodes(java.util.Collection serviceCodes) {
setServiceCodes(serviceCodes);
return this;
}
/**
*
* The services.
*
*
* @param serviceCodes
* The services.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SavingsPlanRateServiceCode
*/
public DescribeSavingsPlansOfferingRatesRequest withServiceCodes(SavingsPlanRateServiceCode... serviceCodes) {
java.util.ArrayList serviceCodesCopy = new java.util.ArrayList(serviceCodes.length);
for (SavingsPlanRateServiceCode value : serviceCodes) {
serviceCodesCopy.add(value.toString());
}
if (getServiceCodes() == null) {
setServiceCodes(serviceCodesCopy);
} else {
getServiceCodes().addAll(serviceCodesCopy);
}
return this;
}
/**
*
* The usage details of the line item in the billing report.
*
*
* @return The usage details of the line item in the billing report.
*/
public java.util.List getUsageTypes() {
return usageTypes;
}
/**
*
* The usage details of the line item in the billing report.
*
*
* @param usageTypes
* The usage details of the line item in the billing report.
*/
public void setUsageTypes(java.util.Collection usageTypes) {
if (usageTypes == null) {
this.usageTypes = null;
return;
}
this.usageTypes = new java.util.ArrayList(usageTypes);
}
/**
*
* The usage details of the line item in the billing report.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUsageTypes(java.util.Collection)} or {@link #withUsageTypes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param usageTypes
* The usage details of the line item in the billing report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withUsageTypes(String... usageTypes) {
if (this.usageTypes == null) {
setUsageTypes(new java.util.ArrayList(usageTypes.length));
}
for (String ele : usageTypes) {
this.usageTypes.add(ele);
}
return this;
}
/**
*
* The usage details of the line item in the billing report.
*
*
* @param usageTypes
* The usage details of the line item in the billing report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withUsageTypes(java.util.Collection usageTypes) {
setUsageTypes(usageTypes);
return this;
}
/**
*
* The specific Amazon Web Services operation for the line item in the billing report.
*
*
* @return The specific Amazon Web Services operation for the line item in the billing report.
*/
public java.util.List getOperations() {
return operations;
}
/**
*
* The specific Amazon Web Services operation for the line item in the billing report.
*
*
* @param operations
* The specific Amazon Web Services operation for the line item in the billing report.
*/
public void setOperations(java.util.Collection operations) {
if (operations == null) {
this.operations = null;
return;
}
this.operations = new java.util.ArrayList(operations);
}
/**
*
* The specific Amazon Web Services operation for the line item in the billing report.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOperations(java.util.Collection)} or {@link #withOperations(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param operations
* The specific Amazon Web Services operation for the line item in the billing report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withOperations(String... operations) {
if (this.operations == null) {
setOperations(new java.util.ArrayList(operations.length));
}
for (String ele : operations) {
this.operations.add(ele);
}
return this;
}
/**
*
* The specific Amazon Web Services operation for the line item in the billing report.
*
*
* @param operations
* The specific Amazon Web Services operation for the line item in the billing report.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withOperations(java.util.Collection operations) {
setOperations(operations);
return this;
}
/**
*
* The filters.
*
*
* @return The filters.
*/
public java.util.List getFilters() {
return filters;
}
/**
*
* The filters.
*
*
* @param filters
* The filters.
*/
public void setFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
return;
}
this.filters = new java.util.ArrayList(filters);
}
/**
*
* The filters.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFilters(java.util.Collection)} or {@link #withFilters(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param filters
* The filters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withFilters(SavingsPlanOfferingRateFilterElement... filters) {
if (this.filters == null) {
setFilters(new java.util.ArrayList(filters.length));
}
for (SavingsPlanOfferingRateFilterElement ele : filters) {
this.filters.add(ele);
}
return this;
}
/**
*
* The filters.
*
*
* @param filters
* The filters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withFilters(java.util.Collection filters) {
setFilters(filters);
return this;
}
/**
*
* The token for the next page of results.
*
*
* @param nextToken
* The token for the next page of results.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* The token for the next page of results.
*
*
* @return The token for the next page of results.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* The token for the next page of results.
*
*
* @param nextToken
* The token for the next page of results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* The maximum number of results to return with a single call. To retrieve additional results, make another call
* with the returned token value.
*
*
* @param maxResults
* The maximum number of results to return with a single call. To retrieve additional results, make another
* call with the returned token value.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of results to return with a single call. To retrieve additional results, make another call
* with the returned token value.
*
*
* @return The maximum number of results to return with a single call. To retrieve additional results, make another
* call with the returned token value.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of results to return with a single call. To retrieve additional results, make another call
* with the returned token value.
*
*
* @param maxResults
* The maximum number of results to return with a single call. To retrieve additional results, make another
* call with the returned token value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSavingsPlansOfferingRatesRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSavingsPlanOfferingIds() != null)
sb.append("SavingsPlanOfferingIds: ").append(getSavingsPlanOfferingIds()).append(",");
if (getSavingsPlanPaymentOptions() != null)
sb.append("SavingsPlanPaymentOptions: ").append(getSavingsPlanPaymentOptions()).append(",");
if (getSavingsPlanTypes() != null)
sb.append("SavingsPlanTypes: ").append(getSavingsPlanTypes()).append(",");
if (getProducts() != null)
sb.append("Products: ").append(getProducts()).append(",");
if (getServiceCodes() != null)
sb.append("ServiceCodes: ").append(getServiceCodes()).append(",");
if (getUsageTypes() != null)
sb.append("UsageTypes: ").append(getUsageTypes()).append(",");
if (getOperations() != null)
sb.append("Operations: ").append(getOperations()).append(",");
if (getFilters() != null)
sb.append("Filters: ").append(getFilters()).append(",");
if (getNextToken() != null)
sb.append("NextToken: ").append(getNextToken()).append(",");
if (getMaxResults() != null)
sb.append("MaxResults: ").append(getMaxResults());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeSavingsPlansOfferingRatesRequest == false)
return false;
DescribeSavingsPlansOfferingRatesRequest other = (DescribeSavingsPlansOfferingRatesRequest) obj;
if (other.getSavingsPlanOfferingIds() == null ^ this.getSavingsPlanOfferingIds() == null)
return false;
if (other.getSavingsPlanOfferingIds() != null && other.getSavingsPlanOfferingIds().equals(this.getSavingsPlanOfferingIds()) == false)
return false;
if (other.getSavingsPlanPaymentOptions() == null ^ this.getSavingsPlanPaymentOptions() == null)
return false;
if (other.getSavingsPlanPaymentOptions() != null && other.getSavingsPlanPaymentOptions().equals(this.getSavingsPlanPaymentOptions()) == false)
return false;
if (other.getSavingsPlanTypes() == null ^ this.getSavingsPlanTypes() == null)
return false;
if (other.getSavingsPlanTypes() != null && other.getSavingsPlanTypes().equals(this.getSavingsPlanTypes()) == false)
return false;
if (other.getProducts() == null ^ this.getProducts() == null)
return false;
if (other.getProducts() != null && other.getProducts().equals(this.getProducts()) == false)
return false;
if (other.getServiceCodes() == null ^ this.getServiceCodes() == null)
return false;
if (other.getServiceCodes() != null && other.getServiceCodes().equals(this.getServiceCodes()) == false)
return false;
if (other.getUsageTypes() == null ^ this.getUsageTypes() == null)
return false;
if (other.getUsageTypes() != null && other.getUsageTypes().equals(this.getUsageTypes()) == false)
return false;
if (other.getOperations() == null ^ this.getOperations() == null)
return false;
if (other.getOperations() != null && other.getOperations().equals(this.getOperations()) == false)
return false;
if (other.getFilters() == null ^ this.getFilters() == null)
return false;
if (other.getFilters() != null && other.getFilters().equals(this.getFilters()) == false)
return false;
if (other.getNextToken() == null ^ this.getNextToken() == null)
return false;
if (other.getNextToken() != null && other.getNextToken().equals(this.getNextToken()) == false)
return false;
if (other.getMaxResults() == null ^ this.getMaxResults() == null)
return false;
if (other.getMaxResults() != null && other.getMaxResults().equals(this.getMaxResults()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSavingsPlanOfferingIds() == null) ? 0 : getSavingsPlanOfferingIds().hashCode());
hashCode = prime * hashCode + ((getSavingsPlanPaymentOptions() == null) ? 0 : getSavingsPlanPaymentOptions().hashCode());
hashCode = prime * hashCode + ((getSavingsPlanTypes() == null) ? 0 : getSavingsPlanTypes().hashCode());
hashCode = prime * hashCode + ((getProducts() == null) ? 0 : getProducts().hashCode());
hashCode = prime * hashCode + ((getServiceCodes() == null) ? 0 : getServiceCodes().hashCode());
hashCode = prime * hashCode + ((getUsageTypes() == null) ? 0 : getUsageTypes().hashCode());
hashCode = prime * hashCode + ((getOperations() == null) ? 0 : getOperations().hashCode());
hashCode = prime * hashCode + ((getFilters() == null) ? 0 : getFilters().hashCode());
hashCode = prime * hashCode + ((getNextToken() == null) ? 0 : getNextToken().hashCode());
hashCode = prime * hashCode + ((getMaxResults() == null) ? 0 : getMaxResults().hashCode());
return hashCode;
}
@Override
public DescribeSavingsPlansOfferingRatesRequest clone() {
return (DescribeSavingsPlansOfferingRatesRequest) super.clone();
}
}