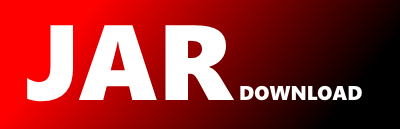
com.amazonaws.services.secretsmanager.AWSSecretsManagerClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-secretsmanager Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.secretsmanager;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.secretsmanager.AWSSecretsManagerClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.secretsmanager.model.*;
import com.amazonaws.services.secretsmanager.model.transform.*;
/**
* Client for accessing AWS Secrets Manager. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
* Amazon Web Services Secrets Manager
*
* Amazon Web Services Secrets Manager provides a service to enable you to store, manage, and retrieve, secrets.
*
*
* This guide provides descriptions of the Secrets Manager API. For more information about using this service, see the
* Amazon Web Services Secrets
* Manager User Guide.
*
*
* API Version
*
*
* This version of the Secrets Manager API Reference documents the Secrets Manager API version 2017-10-17.
*
*
*
* As an alternative to using the API, you can use one of the Amazon Web Services SDKs, which consist of libraries and
* sample code for various programming languages and platforms such as Java, Ruby, .NET, iOS, and Android. The SDKs
* provide a convenient way to create programmatic access to Amazon Web Services Secrets Manager. For example, the SDKs
* provide cryptographically signing requests, managing errors, and retrying requests automatically. For more
* information about the Amazon Web Services SDKs, including downloading and installing them, see Tools for Amazon Web Services.
*
*
*
* We recommend you use the Amazon Web Services SDKs to make programmatic API calls to Secrets Manager. However, you
* also can use the Secrets Manager HTTP Query API to make direct calls to the Secrets Manager web service. To learn
* more about the Secrets Manager HTTP Query API, see Making Query Requests in
* the Amazon Web Services Secrets Manager User Guide.
*
*
* Secrets Manager API supports GET and POST requests for all actions, and doesn't require you to use GET for some
* actions and POST for others. However, GET requests are subject to the limitation size of a URL. Therefore, for
* operations that require larger sizes, use a POST request.
*
*
* Support and Feedback for Amazon Web Services Secrets Manager
*
*
* We welcome your feedback. Send your comments to [email protected], or post your feedback
* and questions in the Amazon Web Services Secrets
* Manager Discussion Forum. For more information about the Amazon Web Services Discussion Forums, see Forums Help.
*
*
* How examples are presented
*
*
* The JSON that Amazon Web Services Secrets Manager expects as your request parameters and the service returns as a
* response to HTTP query requests contain single, long strings without line breaks or white space formatting. The JSON
* shown in the examples displays the code formatted with both line breaks and white space to improve readability. When
* example input parameters can also cause long strings extending beyond the screen, you can insert line breaks to
* enhance readability. You should always submit the input as a single JSON text string.
*
*
* Logging API Requests
*
*
* Amazon Web Services Secrets Manager supports Amazon Web Services CloudTrail, a service that records Amazon Web
* Services API calls for your Amazon Web Services account and delivers log files to an Amazon S3 bucket. By using
* information that's collected by Amazon Web Services CloudTrail, you can determine the requests successfully made to
* Secrets Manager, who made the request, when it was made, and so on. For more about Amazon Web Services Secrets
* Manager and support for Amazon Web Services CloudTrail, see Logging
* Amazon Web Services Secrets Manager Events with Amazon Web Services CloudTrail in the Amazon Web Services
* Secrets Manager User Guide. To learn more about CloudTrail, including enabling it and find your log files, see
* the Amazon
* Web Services CloudTrail User Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AWSSecretsManagerClient extends AmazonWebServiceClient implements AWSSecretsManager {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AWSSecretsManager.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "secretsmanager";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("EncryptionFailure").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.EncryptionFailureExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidParameterException").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.InvalidParameterExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("PublicPolicyException").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.PublicPolicyExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MalformedPolicyDocumentException").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.MalformedPolicyDocumentExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("DecryptionFailure").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.DecryptionFailureExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidRequestException").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.InvalidRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalServiceError").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.InternalServiceErrorExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceExistsException").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.ResourceExistsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidNextTokenException").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.InvalidNextTokenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("PreconditionNotMetException").withExceptionUnmarshaller(
com.amazonaws.services.secretsmanager.model.transform.PreconditionNotMetExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.secretsmanager.model.AWSSecretsManagerException.class));
public static AWSSecretsManagerClientBuilder builder() {
return AWSSecretsManagerClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS Secrets Manager using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSSecretsManagerClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS Secrets Manager using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AWSSecretsManagerClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("secretsmanager.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/secretsmanager/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/secretsmanager/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Turns off automatic rotation, and if a rotation is currently in progress, cancels the rotation.
*
*
* To turn on automatic rotation again, call RotateSecret.
*
*
*
* If you cancel a rotation in progress, it can leave the VersionStage
labels in an unexpected state.
* Depending on the step of the rotation in progress, you might need to remove the staging label
* AWSPENDING
from the partially created version, specified by the VersionId
response
* value. We recommend you also evaluate the partially rotated new version to see if it should be deleted. You can
* delete a version by removing all staging labels from it.
*
*
*
* @param cancelRotateSecretRequest
* @return Result of the CancelRotateSecret operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @sample AWSSecretsManager.CancelRotateSecret
* @see AWS API Documentation
*/
@Override
public CancelRotateSecretResult cancelRotateSecret(CancelRotateSecretRequest request) {
request = beforeClientExecution(request);
return executeCancelRotateSecret(request);
}
@SdkInternalApi
final CancelRotateSecretResult executeCancelRotateSecret(CancelRotateSecretRequest cancelRotateSecretRequest) {
ExecutionContext executionContext = createExecutionContext(cancelRotateSecretRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelRotateSecretRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(cancelRotateSecretRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelRotateSecret");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CancelRotateSecretResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new secret. A secret is a set of credentials, such as a user name and password, that you store
* in an encrypted form in Secrets Manager. The secret also includes the connection information to access a database
* or other service, which Secrets Manager doesn't encrypt. A secret in Secrets Manager consists of both the
* protected secret data and the important information needed to manage the secret.
*
*
* For information about creating a secret in the console, see Create a
* secret.
*
*
* To create a secret, you can provide the secret value to be encrypted in either the SecretString
* parameter or the SecretBinary
parameter, but not both. If you include SecretString
or
* SecretBinary
then Secrets Manager creates an initial secret version and automatically attaches the
* staging label AWSCURRENT
to it.
*
*
* If you don't specify an KMS encryption key, Secrets Manager uses the Amazon Web Services managed key
* aws/secretsmanager
. If this key doesn't already exist in your account, then Secrets Manager creates
* it for you automatically. All users and roles in the Amazon Web Services account automatically have access to use
* aws/secretsmanager
. Creating aws/secretsmanager
can result in a one-time significant
* delay in returning the result.
*
*
* If the secret is in a different Amazon Web Services account from the credentials calling the API, then you can't
* use aws/secretsmanager
to encrypt the secret, and you must create and use a customer managed KMS
* key.
*
*
* @param createSecretRequest
* @return Result of the CreateSecret operation returned by the service.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws LimitExceededException
* The request failed because it would exceed one of the Secrets Manager quotas.
* @throws EncryptionFailureException
* Secrets Manager can't encrypt the protected secret text using the provided KMS key. Check that the KMS
* key is available, enabled, and not in an invalid state. For more information, see Key state: Effect on your KMS
* key.
* @throws ResourceExistsException
* A resource with the ID you requested already exists.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws MalformedPolicyDocumentException
* The resource policy has syntax errors.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws PreconditionNotMetException
* The request failed because you did not complete all the prerequisite steps.
* @sample AWSSecretsManager.CreateSecret
* @see AWS
* API Documentation
*/
@Override
public CreateSecretResult createSecret(CreateSecretRequest request) {
request = beforeClientExecution(request);
return executeCreateSecret(request);
}
@SdkInternalApi
final CreateSecretResult executeCreateSecret(CreateSecretRequest createSecretRequest) {
ExecutionContext executionContext = createExecutionContext(createSecretRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSecretRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSecretRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSecret");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSecretResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the resource-based permission policy attached to the secret. To attach a policy to a secret, use
* PutResourcePolicy.
*
*
* @param deleteResourcePolicyRequest
* @return Result of the DeleteResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InvalidParameterException
* The parameter name is invalid value.
* @sample AWSSecretsManager.DeleteResourcePolicy
* @see AWS API Documentation
*/
@Override
public DeleteResourcePolicyResult deleteResourcePolicy(DeleteResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeDeleteResourcePolicy(request);
}
@SdkInternalApi
final DeleteResourcePolicyResult executeDeleteResourcePolicy(DeleteResourcePolicyRequest deleteResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a secret and all of its versions. You can specify a recovery window during which you can restore the
* secret. The minimum recovery window is 7 days. The default recovery window is 30 days. Secrets Manager attaches a
* DeletionDate
stamp to the secret that specifies the end of the recovery window. At the end of the
* recovery window, Secrets Manager deletes the secret permanently.
*
*
* For information about deleting a secret in the console, see https://docs.aws.amazon.com/secretsmanager/latest/userguide/manage_delete-secret.html.
*
*
* Secrets Manager performs the permanent secret deletion at the end of the waiting period as a background task with
* low priority. There is no guarantee of a specific time after the recovery window for the permanent delete to
* occur.
*
*
* At any time before recovery window ends, you can use RestoreSecret to remove the DeletionDate
* and cancel the deletion of the secret.
*
*
* In a secret scheduled for deletion, you cannot access the encrypted secret value. To access that information,
* first cancel the deletion with RestoreSecret and then retrieve the information.
*
*
* @param deleteSecretRequest
* @return Result of the DeleteSecret operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.DeleteSecret
* @see AWS
* API Documentation
*/
@Override
public DeleteSecretResult deleteSecret(DeleteSecretRequest request) {
request = beforeClientExecution(request);
return executeDeleteSecret(request);
}
@SdkInternalApi
final DeleteSecretResult executeDeleteSecret(DeleteSecretRequest deleteSecretRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSecretRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSecretRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSecretRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSecret");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSecretResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the details of a secret. It does not include the encrypted secret value. Secrets Manager only returns
* fields that have a value in the response.
*
*
* @param describeSecretRequest
* @return Result of the DescribeSecret operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @sample AWSSecretsManager.DescribeSecret
* @see AWS
* API Documentation
*/
@Override
public DescribeSecretResult describeSecret(DescribeSecretRequest request) {
request = beforeClientExecution(request);
return executeDescribeSecret(request);
}
@SdkInternalApi
final DescribeSecretResult executeDescribeSecret(DescribeSecretRequest describeSecretRequest) {
ExecutionContext executionContext = createExecutionContext(describeSecretRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeSecretRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeSecretRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeSecret");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeSecretResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Generates a random password. We recommend that you specify the maximum length and include every character type
* that the system you are generating a password for can support.
*
*
* @param getRandomPasswordRequest
* @return Result of the GetRandomPassword operation returned by the service.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.GetRandomPassword
* @see AWS API Documentation
*/
@Override
public GetRandomPasswordResult getRandomPassword(GetRandomPasswordRequest request) {
request = beforeClientExecution(request);
return executeGetRandomPassword(request);
}
@SdkInternalApi
final GetRandomPasswordResult executeGetRandomPassword(GetRandomPasswordRequest getRandomPasswordRequest) {
ExecutionContext executionContext = createExecutionContext(getRandomPasswordRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetRandomPasswordRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getRandomPasswordRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetRandomPassword");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetRandomPasswordResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the JSON text of the resource-based policy document attached to the secret. For more information about
* permissions policies attached to a secret, see Permissions policies attached to a secret.
*
*
* @param getResourcePolicyRequest
* @return Result of the GetResourcePolicy operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InvalidParameterException
* The parameter name is invalid value.
* @sample AWSSecretsManager.GetResourcePolicy
* @see AWS API Documentation
*/
@Override
public GetResourcePolicyResult getResourcePolicy(GetResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeGetResourcePolicy(request);
}
@SdkInternalApi
final GetResourcePolicyResult executeGetResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the contents of the encrypted fields SecretString
or SecretBinary
from the
* specified version of a secret, whichever contains content.
*
*
* For information about retrieving the secret value in the console, see Retrieve secrets.
*
*
* To run this command, you must have secretsmanager:GetSecretValue
permissions. If the secret is
* encrypted using a customer-managed key instead of the Amazon Web Services managed key
* aws/secretsmanager
, then you also need kms:Decrypt
permissions for that key.
*
*
* @param getSecretValueRequest
* @return Result of the GetSecretValue operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws DecryptionFailureException
* Secrets Manager can't decrypt the protected secret text using the provided KMS key.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.GetSecretValue
* @see AWS
* API Documentation
*/
@Override
public GetSecretValueResult getSecretValue(GetSecretValueRequest request) {
request = beforeClientExecution(request);
return executeGetSecretValue(request);
}
@SdkInternalApi
final GetSecretValueResult executeGetSecretValue(GetSecretValueRequest getSecretValueRequest) {
ExecutionContext executionContext = createExecutionContext(getSecretValueRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSecretValueRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSecretValueRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSecretValue");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSecretValueResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the versions for a secret.
*
*
* To list the secrets in the account, use ListSecrets.
*
*
* To get the secret value from SecretString
or SecretBinary
, call GetSecretValue.
*
*
* Minimum permissions
*
*
* To run this command, you must have secretsmanager:ListSecretVersionIds
permissions.
*
*
* @param listSecretVersionIdsRequest
* @return Result of the ListSecretVersionIds operation returned by the service.
* @throws InvalidNextTokenException
* The NextToken
value is invalid.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @sample AWSSecretsManager.ListSecretVersionIds
* @see AWS API Documentation
*/
@Override
public ListSecretVersionIdsResult listSecretVersionIds(ListSecretVersionIdsRequest request) {
request = beforeClientExecution(request);
return executeListSecretVersionIds(request);
}
@SdkInternalApi
final ListSecretVersionIdsResult executeListSecretVersionIds(ListSecretVersionIdsRequest listSecretVersionIdsRequest) {
ExecutionContext executionContext = createExecutionContext(listSecretVersionIdsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSecretVersionIdsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSecretVersionIdsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSecretVersionIds");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSecretVersionIdsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the secrets that are stored by Secrets Manager in the Amazon Web Services account.
*
*
* To list the versions of a secret, use ListSecretVersionIds.
*
*
* To get the secret value from SecretString
or SecretBinary
, call GetSecretValue.
*
*
* For information about finding secrets in the console, see Enhanced search
* capabilities for secrets in Secrets Manager.
*
*
* Minimum permissions
*
*
* To run this command, you must have secretsmanager:ListSecrets
permissions.
*
*
* @param listSecretsRequest
* @return Result of the ListSecrets operation returned by the service.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InvalidNextTokenException
* The NextToken
value is invalid.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.ListSecrets
* @see AWS API
* Documentation
*/
@Override
public ListSecretsResult listSecrets(ListSecretsRequest request) {
request = beforeClientExecution(request);
return executeListSecrets(request);
}
@SdkInternalApi
final ListSecretsResult executeListSecrets(ListSecretsRequest listSecretsRequest) {
ExecutionContext executionContext = createExecutionContext(listSecretsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSecretsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSecretsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSecrets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSecretsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches a resource-based permission policy to a secret. A resource-based policy is optional. For more
* information, see Authentication and access
* control for Secrets Manager
*
*
* For information about attaching a policy in the console, see Attach a permissions policy to a secret.
*
*
* @param putResourcePolicyRequest
* @return Result of the PutResourcePolicy operation returned by the service.
* @throws MalformedPolicyDocumentException
* The resource policy has syntax errors.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws PublicPolicyException
* The BlockPublicPolicy
parameter is set to true, and the resource policy did not prevent
* broad access to the secret.
* @sample AWSSecretsManager.PutResourcePolicy
* @see AWS API Documentation
*/
@Override
public PutResourcePolicyResult putResourcePolicy(PutResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executePutResourcePolicy(request);
}
@SdkInternalApi
final PutResourcePolicyResult executePutResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(putResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new version with a new encrypted secret value and attaches it to the secret. The version can contain a
* new SecretString
value or a new SecretBinary
value.
*
*
* We recommend you avoid calling PutSecretValue
at a sustained rate of more than once every 10
* minutes. When you update the secret value, Secrets Manager creates a new version of the secret. Secrets Manager
* removes outdated versions when there are more than 100, but it does not remove versions created less than 24
* hours ago. If you call PutSecretValue
more than once every 10 minutes, you create more versions than
* Secrets Manager removes, and you will reach the quota for secret versions.
*
*
* You can specify the staging labels to attach to the new version in VersionStages
. If you don't
* include VersionStages
, then Secrets Manager automatically moves the staging label
* AWSCURRENT
to this version. If this operation creates the first version for the secret, then Secrets
* Manager automatically attaches the staging label AWSCURRENT
to it .
*
*
* If this operation moves the staging label AWSCURRENT
from another version to this version, then
* Secrets Manager also automatically moves the staging label AWSPREVIOUS
to the version that
* AWSCURRENT
was removed from.
*
*
* This operation is idempotent. If a version with a VersionId
with the same value as the
* ClientRequestToken
parameter already exists, and you specify the same secret data, the operation
* succeeds but does nothing. However, if the secret data is different, then the operation fails because you can't
* modify an existing version; you can only create new ones.
*
*
* @param putSecretValueRequest
* @return Result of the PutSecretValue operation returned by the service.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws LimitExceededException
* The request failed because it would exceed one of the Secrets Manager quotas.
* @throws EncryptionFailureException
* Secrets Manager can't encrypt the protected secret text using the provided KMS key. Check that the KMS
* key is available, enabled, and not in an invalid state. For more information, see Key state: Effect on your KMS
* key.
* @throws ResourceExistsException
* A resource with the ID you requested already exists.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.PutSecretValue
* @see AWS
* API Documentation
*/
@Override
public PutSecretValueResult putSecretValue(PutSecretValueRequest request) {
request = beforeClientExecution(request);
return executePutSecretValue(request);
}
@SdkInternalApi
final PutSecretValueResult executePutSecretValue(PutSecretValueRequest putSecretValueRequest) {
ExecutionContext executionContext = createExecutionContext(putSecretValueRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutSecretValueRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putSecretValueRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutSecretValue");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new PutSecretValueResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* For a secret that is replicated to other Regions, deletes the secret replicas from the Regions you specify.
*
*
* @param removeRegionsFromReplicationRequest
* @return Result of the RemoveRegionsFromReplication operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.RemoveRegionsFromReplication
* @see AWS API Documentation
*/
@Override
public RemoveRegionsFromReplicationResult removeRegionsFromReplication(RemoveRegionsFromReplicationRequest request) {
request = beforeClientExecution(request);
return executeRemoveRegionsFromReplication(request);
}
@SdkInternalApi
final RemoveRegionsFromReplicationResult executeRemoveRegionsFromReplication(RemoveRegionsFromReplicationRequest removeRegionsFromReplicationRequest) {
ExecutionContext executionContext = createExecutionContext(removeRegionsFromReplicationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RemoveRegionsFromReplicationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(removeRegionsFromReplicationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RemoveRegionsFromReplication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new RemoveRegionsFromReplicationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Replicates the secret to a new Regions. See Multi-Region secrets.
*
*
* @param replicateSecretToRegionsRequest
* @return Result of the ReplicateSecretToRegions operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.ReplicateSecretToRegions
* @see AWS API Documentation
*/
@Override
public ReplicateSecretToRegionsResult replicateSecretToRegions(ReplicateSecretToRegionsRequest request) {
request = beforeClientExecution(request);
return executeReplicateSecretToRegions(request);
}
@SdkInternalApi
final ReplicateSecretToRegionsResult executeReplicateSecretToRegions(ReplicateSecretToRegionsRequest replicateSecretToRegionsRequest) {
ExecutionContext executionContext = createExecutionContext(replicateSecretToRegionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ReplicateSecretToRegionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(replicateSecretToRegionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ReplicateSecretToRegions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ReplicateSecretToRegionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels the scheduled deletion of a secret by removing the DeletedDate
time stamp. You can access a
* secret again after it has been restored.
*
*
* @param restoreSecretRequest
* @return Result of the RestoreSecret operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.RestoreSecret
* @see AWS
* API Documentation
*/
@Override
public RestoreSecretResult restoreSecret(RestoreSecretRequest request) {
request = beforeClientExecution(request);
return executeRestoreSecret(request);
}
@SdkInternalApi
final RestoreSecretResult executeRestoreSecret(RestoreSecretRequest restoreSecretRequest) {
ExecutionContext executionContext = createExecutionContext(restoreSecretRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RestoreSecretRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(restoreSecretRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RestoreSecret");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RestoreSecretResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Configures and starts the asynchronous process of rotating the secret.
*
*
* If you include the configuration parameters, the operation sets the values for the secret and then immediately
* starts a rotation. If you don't include the configuration parameters, the operation starts a rotation with the
* values already stored in the secret. For more information about rotation, see Rotate secrets.
*
*
* To configure rotation, you include the ARN of an Amazon Web Services Lambda function and the schedule for the
* rotation. The Lambda rotation function creates a new version of the secret and creates or updates the credentials
* on the database or service to match. After testing the new credentials, the function marks the new secret version
* with the staging label AWSCURRENT
. Then anyone who retrieves the secret gets the new version. For
* more information, see How rotation
* works.
*
*
* When rotation is successful, the AWSPENDING
staging label might be attached to the same version as
* the AWSCURRENT
version, or it might not be attached to any version.
*
*
* If the AWSPENDING
staging label is present but not attached to the same version as
* AWSCURRENT
, then any later invocation of RotateSecret
assumes that a previous rotation
* request is still in progress and returns an error.
*
*
* To run this command, you must have secretsmanager:RotateSecret
permissions and
* lambda:InvokeFunction
permissions on the function specified in the secret's metadata.
*
*
* @param rotateSecretRequest
* @return Result of the RotateSecret operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @sample AWSSecretsManager.RotateSecret
* @see AWS
* API Documentation
*/
@Override
public RotateSecretResult rotateSecret(RotateSecretRequest request) {
request = beforeClientExecution(request);
return executeRotateSecret(request);
}
@SdkInternalApi
final RotateSecretResult executeRotateSecret(RotateSecretRequest rotateSecretRequest) {
ExecutionContext executionContext = createExecutionContext(rotateSecretRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RotateSecretRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(rotateSecretRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RotateSecret");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RotateSecretResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the link between the replica secret and the primary secret and promotes the replica to a primary secret
* in the replica Region.
*
*
* You must call this operation from the Region in which you want to promote the replica to a primary secret.
*
*
* @param stopReplicationToReplicaRequest
* @return Result of the StopReplicationToReplica operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.StopReplicationToReplica
* @see AWS API Documentation
*/
@Override
public StopReplicationToReplicaResult stopReplicationToReplica(StopReplicationToReplicaRequest request) {
request = beforeClientExecution(request);
return executeStopReplicationToReplica(request);
}
@SdkInternalApi
final StopReplicationToReplicaResult executeStopReplicationToReplica(StopReplicationToReplicaRequest stopReplicationToReplicaRequest) {
ExecutionContext executionContext = createExecutionContext(stopReplicationToReplicaRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopReplicationToReplicaRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(stopReplicationToReplicaRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopReplicationToReplica");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopReplicationToReplicaResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Attaches tags to a secret. Tags consist of a key name and a value. Tags are part of the secret's metadata. They
* are not associated with specific versions of the secret. This operation appends tags to the existing list of
* tags.
*
*
* The following restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per secret: 50
*
*
* -
*
* Maximum key length: 127 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length: 255 Unicode characters in UTF-8
*
*
* -
*
* Tag keys and values are case sensitive.
*
*
* -
*
* Do not use the aws:
prefix in your tag names or values because Amazon Web Services reserves it for
* Amazon Web Services use. You can't edit or delete tag names or values with this prefix. Tags with this prefix do
* not count against your tags per secret limit.
*
*
* -
*
* If you use your tagging schema across multiple services and resources, other services might have restrictions on
* allowed characters. Generally allowed characters: letters, spaces, and numbers representable in UTF-8, plus the
* following special characters: + - = . _ : / @.
*
*
*
*
*
* If you use tags as part of your security strategy, then adding or removing a tag can change permissions. If
* successfully completing this operation would result in you losing your permissions for this secret, then the
* operation is blocked and returns an Access Denied error.
*
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes specific tags from a secret.
*
*
* This operation is idempotent. If a requested tag is not attached to the secret, no error is returned and the
* secret metadata is unchanged.
*
*
*
* If you use tags as part of your security strategy, then removing a tag can change permissions. If successfully
* completing this operation would result in you losing your permissions for this secret, then the operation is
* blocked and returns an Access Denied error.
*
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the details of a secret, including metadata and the secret value. To change the secret value, you can
* also use PutSecretValue.
*
*
* To change the rotation configuration of a secret, use RotateSecret instead.
*
*
* We recommend you avoid calling UpdateSecret
at a sustained rate of more than once every 10 minutes.
* When you call UpdateSecret
to update the secret value, Secrets Manager creates a new version of the
* secret. Secrets Manager removes outdated versions when there are more than 100, but it does not remove versions
* created less than 24 hours ago. If you update the secret value more than once every 10 minutes, you create more
* versions than Secrets Manager removes, and you will reach the quota for secret versions.
*
*
* If you include SecretString
or SecretBinary
to create a new secret version, Secrets
* Manager automatically attaches the staging label AWSCURRENT
to the new version.
*
*
* If you call this operation with a VersionId
that matches an existing version's
* ClientRequestToken
, the operation results in an error. You can't modify an existing version, you can
* only create a new version. To remove a version, remove all staging labels from it. See
* UpdateSecretVersionStage.
*
*
* If you don't specify an KMS encryption key, Secrets Manager uses the Amazon Web Services managed key
* aws/secretsmanager
. If this key doesn't already exist in your account, then Secrets Manager creates
* it for you automatically. All users and roles in the Amazon Web Services account automatically have access to use
* aws/secretsmanager
. Creating aws/secretsmanager
can result in a one-time significant
* delay in returning the result.
*
*
* If the secret is in a different Amazon Web Services account from the credentials calling the API, then you can't
* use aws/secretsmanager
to encrypt the secret, and you must create and use a customer managed key.
*
*
* To run this command, you must have secretsmanager:UpdateSecret
permissions. If you use a customer
* managed key, you must also have kms:GenerateDataKey
and kms:Decrypt
permissions .
*
*
* @param updateSecretRequest
* @return Result of the UpdateSecret operation returned by the service.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws LimitExceededException
* The request failed because it would exceed one of the Secrets Manager quotas.
* @throws EncryptionFailureException
* Secrets Manager can't encrypt the protected secret text using the provided KMS key. Check that the KMS
* key is available, enabled, and not in an invalid state. For more information, see Key state: Effect on your KMS
* key.
* @throws ResourceExistsException
* A resource with the ID you requested already exists.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws MalformedPolicyDocumentException
* The resource policy has syntax errors.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws PreconditionNotMetException
* The request failed because you did not complete all the prerequisite steps.
* @sample AWSSecretsManager.UpdateSecret
* @see AWS
* API Documentation
*/
@Override
public UpdateSecretResult updateSecret(UpdateSecretRequest request) {
request = beforeClientExecution(request);
return executeUpdateSecret(request);
}
@SdkInternalApi
final UpdateSecretResult executeUpdateSecret(UpdateSecretRequest updateSecretRequest) {
ExecutionContext executionContext = createExecutionContext(updateSecretRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSecretRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateSecretRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateSecret");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateSecretResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the staging labels attached to a version of a secret. Secrets Manager uses staging labels to track a
* version as it progresses through the secret rotation process. Each staging label can be attached to only one
* version at a time. To add a staging label to a version when it is already attached to another version, Secrets
* Manager first removes it from the other version first and then attaches it to this one. For more information
* about versions and staging labels, see Concepts:
* Version.
*
*
* The staging labels that you specify in the VersionStage
parameter are added to the existing list of
* staging labels for the version.
*
*
* You can move the AWSCURRENT
staging label to this version by including it in this call.
*
*
*
* Whenever you move AWSCURRENT
, Secrets Manager automatically moves the label AWSPREVIOUS
* to the version that AWSCURRENT
was removed from.
*
*
*
* If this action results in the last label being removed from a version, then the version is considered to be
* 'deprecated' and can be deleted by Secrets Manager.
*
*
* @param updateSecretVersionStageRequest
* @return Result of the UpdateSecretVersionStage operation returned by the service.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @throws LimitExceededException
* The request failed because it would exceed one of the Secrets Manager quotas.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @sample AWSSecretsManager.UpdateSecretVersionStage
* @see AWS API Documentation
*/
@Override
public UpdateSecretVersionStageResult updateSecretVersionStage(UpdateSecretVersionStageRequest request) {
request = beforeClientExecution(request);
return executeUpdateSecretVersionStage(request);
}
@SdkInternalApi
final UpdateSecretVersionStageResult executeUpdateSecretVersionStage(UpdateSecretVersionStageRequest updateSecretVersionStageRequest) {
ExecutionContext executionContext = createExecutionContext(updateSecretVersionStageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateSecretVersionStageRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateSecretVersionStageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateSecretVersionStage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateSecretVersionStageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Validates that a resource policy does not grant a wide range of principals access to your secret. A
* resource-based policy is optional for secrets.
*
*
* The API performs three checks when validating the policy:
*
*
* -
*
* Sends a call to Zelkova, an automated reasoning engine, to ensure your resource policy does not allow broad access to your
* secret, for example policies that use a wildcard for the principal.
*
*
* -
*
* Checks for correct syntax in a policy.
*
*
* -
*
* Verifies the policy does not lock out a caller.
*
*
*
*
* @param validateResourcePolicyRequest
* @return Result of the ValidateResourcePolicy operation returned by the service.
* @throws MalformedPolicyDocumentException
* The resource policy has syntax errors.
* @throws ResourceNotFoundException
* Secrets Manager can't find the resource that you asked for.
* @throws InvalidParameterException
* The parameter name is invalid value.
* @throws InternalServiceErrorException
* An error occurred on the server side.
* @throws InvalidRequestException
* A parameter value is not valid for the current state of the resource.
*
* Possible causes:
*
*
* -
*
* The secret is scheduled for deletion.
*
*
* -
*
* You tried to enable rotation on a secret that doesn't already have a Lambda function ARN configured and
* you didn't include such an ARN as a parameter in this call.
*
*
* @sample AWSSecretsManager.ValidateResourcePolicy
* @see AWS API Documentation
*/
@Override
public ValidateResourcePolicyResult validateResourcePolicy(ValidateResourcePolicyRequest request) {
request = beforeClientExecution(request);
return executeValidateResourcePolicy(request);
}
@SdkInternalApi
final ValidateResourcePolicyResult executeValidateResourcePolicy(ValidateResourcePolicyRequest validateResourcePolicyRequest) {
ExecutionContext executionContext = createExecutionContext(validateResourcePolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ValidateResourcePolicyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(validateResourcePolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Secrets Manager");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ValidateResourcePolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ValidateResourcePolicyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}