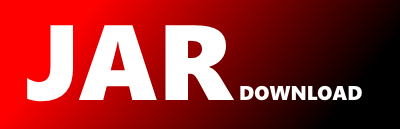
com.amazonaws.services.secretsmanager.model.DescribeSecretResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-secretsmanager Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.secretsmanager.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DescribeSecretResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The ARN of the secret.
*
*/
private String aRN;
/**
*
* The name of the secret.
*
*/
private String name;
/**
*
* The description of the secret.
*
*/
private String description;
/**
*
* The ARN of the KMS key that Secrets Manager uses to encrypt the secret value. If the secret is encrypted with the
* Amazon Web Services managed key aws/secretsmanager
, this field is omitted.
*
*/
private String kmsKeyId;
/**
*
* Specifies whether automatic rotation is turned on for this secret.
*
*
* To turn on rotation, use RotateSecret. To turn off rotation, use CancelRotateSecret.
*
*/
private Boolean rotationEnabled;
/**
*
* The ARN of the Lambda function that Secrets Manager invokes to rotate the secret.
*
*/
private String rotationLambdaARN;
/**
*
* The rotation schedule and Lambda function for this secret. If the secret previously had rotation turned on, but
* it is now turned off, this field shows the previous rotation schedule and rotation function. If the secret never
* had rotation turned on, this field is omitted.
*
*/
private RotationRulesType rotationRules;
/**
*
* The last date and time that Secrets Manager rotated the secret. If the secret isn't configured for rotation,
* Secrets Manager returns null.
*
*/
private java.util.Date lastRotatedDate;
/**
*
* The last date and time that this secret was modified in any way.
*
*/
private java.util.Date lastChangedDate;
/**
*
* The last date that the secret value was retrieved. This value does not include the time. This field is omitted if
* the secret has never been retrieved.
*
*/
private java.util.Date lastAccessedDate;
/**
*
* The date the secret is scheduled for deletion. If it is not scheduled for deletion, this field is omitted. When
* you delete a secret, Secrets Manager requires a recovery window of at least 7 days before deleting the secret.
* Some time after the deleted date, Secrets Manager deletes the secret, including all of its versions.
*
*
* If a secret is scheduled for deletion, then its details, including the encrypted secret value, is not accessible.
* To cancel a scheduled deletion and restore access to the secret, use RestoreSecret.
*
*/
private java.util.Date deletedDate;
/**
*
* The list of tags attached to the secret. To add tags to a secret, use TagResource. To remove tags, use
* UntagResource.
*
*/
private java.util.List tags;
/**
*
* A list of the versions of the secret that have staging labels attached. Versions that don't have staging labels
* are considered deprecated and Secrets Manager can delete them.
*
*
* Secrets Manager uses staging labels to indicate the status of a secret version during rotation. The three staging
* labels for rotation are:
*
*
* -
*
* AWSCURRENT
, which indicates the current version of the secret.
*
*
* -
*
* AWSPENDING
, which indicates the version of the secret that contains new secret information that will
* become the next current version when rotation finishes.
*
*
* During rotation, Secrets Manager creates an AWSPENDING
version ID before creating the new secret
* version. To check if a secret version exists, call GetSecretValue.
*
*
* -
*
* AWSPREVIOUS
, which indicates the previous current version of the secret. You can use this as the
* last known good version.
*
*
*
*
* For more information about rotation and staging labels, see How rotation
* works.
*
*/
private java.util.Map> versionIdsToStages;
/**
*
* The name of the service that created this secret.
*
*/
private String owningService;
/**
*
* The date the secret was created.
*
*/
private java.util.Date createdDate;
/**
*
* The Region the secret is in. If a secret is replicated to other Regions, the replicas are listed in
* ReplicationStatus
.
*
*/
private String primaryRegion;
/**
*
* A list of the replicas of this secret and their status:
*
*
* -
*
* Failed
, which indicates that the replica was not created.
*
*
* -
*
* InProgress
, which indicates that Secrets Manager is in the process of creating the replica.
*
*
* -
*
* InSync
, which indicates that the replica was created.
*
*
*
*/
private java.util.List replicationStatus;
/**
*
* The ARN of the secret.
*
*
* @param aRN
* The ARN of the secret.
*/
public void setARN(String aRN) {
this.aRN = aRN;
}
/**
*
* The ARN of the secret.
*
*
* @return The ARN of the secret.
*/
public String getARN() {
return this.aRN;
}
/**
*
* The ARN of the secret.
*
*
* @param aRN
* The ARN of the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withARN(String aRN) {
setARN(aRN);
return this;
}
/**
*
* The name of the secret.
*
*
* @param name
* The name of the secret.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the secret.
*
*
* @return The name of the secret.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the secret.
*
*
* @param name
* The name of the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withName(String name) {
setName(name);
return this;
}
/**
*
* The description of the secret.
*
*
* @param description
* The description of the secret.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the secret.
*
*
* @return The description of the secret.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the secret.
*
*
* @param description
* The description of the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The ARN of the KMS key that Secrets Manager uses to encrypt the secret value. If the secret is encrypted with the
* Amazon Web Services managed key aws/secretsmanager
, this field is omitted.
*
*
* @param kmsKeyId
* The ARN of the KMS key that Secrets Manager uses to encrypt the secret value. If the secret is encrypted
* with the Amazon Web Services managed key aws/secretsmanager
, this field is omitted.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The ARN of the KMS key that Secrets Manager uses to encrypt the secret value. If the secret is encrypted with the
* Amazon Web Services managed key aws/secretsmanager
, this field is omitted.
*
*
* @return The ARN of the KMS key that Secrets Manager uses to encrypt the secret value. If the secret is encrypted
* with the Amazon Web Services managed key aws/secretsmanager
, this field is omitted.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The ARN of the KMS key that Secrets Manager uses to encrypt the secret value. If the secret is encrypted with the
* Amazon Web Services managed key aws/secretsmanager
, this field is omitted.
*
*
* @param kmsKeyId
* The ARN of the KMS key that Secrets Manager uses to encrypt the secret value. If the secret is encrypted
* with the Amazon Web Services managed key aws/secretsmanager
, this field is omitted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* Specifies whether automatic rotation is turned on for this secret.
*
*
* To turn on rotation, use RotateSecret. To turn off rotation, use CancelRotateSecret.
*
*
* @param rotationEnabled
* Specifies whether automatic rotation is turned on for this secret.
*
* To turn on rotation, use RotateSecret. To turn off rotation, use CancelRotateSecret.
*/
public void setRotationEnabled(Boolean rotationEnabled) {
this.rotationEnabled = rotationEnabled;
}
/**
*
* Specifies whether automatic rotation is turned on for this secret.
*
*
* To turn on rotation, use RotateSecret. To turn off rotation, use CancelRotateSecret.
*
*
* @return Specifies whether automatic rotation is turned on for this secret.
*
* To turn on rotation, use RotateSecret. To turn off rotation, use CancelRotateSecret.
*/
public Boolean getRotationEnabled() {
return this.rotationEnabled;
}
/**
*
* Specifies whether automatic rotation is turned on for this secret.
*
*
* To turn on rotation, use RotateSecret. To turn off rotation, use CancelRotateSecret.
*
*
* @param rotationEnabled
* Specifies whether automatic rotation is turned on for this secret.
*
* To turn on rotation, use RotateSecret. To turn off rotation, use CancelRotateSecret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withRotationEnabled(Boolean rotationEnabled) {
setRotationEnabled(rotationEnabled);
return this;
}
/**
*
* Specifies whether automatic rotation is turned on for this secret.
*
*
* To turn on rotation, use RotateSecret. To turn off rotation, use CancelRotateSecret.
*
*
* @return Specifies whether automatic rotation is turned on for this secret.
*
* To turn on rotation, use RotateSecret. To turn off rotation, use CancelRotateSecret.
*/
public Boolean isRotationEnabled() {
return this.rotationEnabled;
}
/**
*
* The ARN of the Lambda function that Secrets Manager invokes to rotate the secret.
*
*
* @param rotationLambdaARN
* The ARN of the Lambda function that Secrets Manager invokes to rotate the secret.
*/
public void setRotationLambdaARN(String rotationLambdaARN) {
this.rotationLambdaARN = rotationLambdaARN;
}
/**
*
* The ARN of the Lambda function that Secrets Manager invokes to rotate the secret.
*
*
* @return The ARN of the Lambda function that Secrets Manager invokes to rotate the secret.
*/
public String getRotationLambdaARN() {
return this.rotationLambdaARN;
}
/**
*
* The ARN of the Lambda function that Secrets Manager invokes to rotate the secret.
*
*
* @param rotationLambdaARN
* The ARN of the Lambda function that Secrets Manager invokes to rotate the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withRotationLambdaARN(String rotationLambdaARN) {
setRotationLambdaARN(rotationLambdaARN);
return this;
}
/**
*
* The rotation schedule and Lambda function for this secret. If the secret previously had rotation turned on, but
* it is now turned off, this field shows the previous rotation schedule and rotation function. If the secret never
* had rotation turned on, this field is omitted.
*
*
* @param rotationRules
* The rotation schedule and Lambda function for this secret. If the secret previously had rotation turned
* on, but it is now turned off, this field shows the previous rotation schedule and rotation function. If
* the secret never had rotation turned on, this field is omitted.
*/
public void setRotationRules(RotationRulesType rotationRules) {
this.rotationRules = rotationRules;
}
/**
*
* The rotation schedule and Lambda function for this secret. If the secret previously had rotation turned on, but
* it is now turned off, this field shows the previous rotation schedule and rotation function. If the secret never
* had rotation turned on, this field is omitted.
*
*
* @return The rotation schedule and Lambda function for this secret. If the secret previously had rotation turned
* on, but it is now turned off, this field shows the previous rotation schedule and rotation function. If
* the secret never had rotation turned on, this field is omitted.
*/
public RotationRulesType getRotationRules() {
return this.rotationRules;
}
/**
*
* The rotation schedule and Lambda function for this secret. If the secret previously had rotation turned on, but
* it is now turned off, this field shows the previous rotation schedule and rotation function. If the secret never
* had rotation turned on, this field is omitted.
*
*
* @param rotationRules
* The rotation schedule and Lambda function for this secret. If the secret previously had rotation turned
* on, but it is now turned off, this field shows the previous rotation schedule and rotation function. If
* the secret never had rotation turned on, this field is omitted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withRotationRules(RotationRulesType rotationRules) {
setRotationRules(rotationRules);
return this;
}
/**
*
* The last date and time that Secrets Manager rotated the secret. If the secret isn't configured for rotation,
* Secrets Manager returns null.
*
*
* @param lastRotatedDate
* The last date and time that Secrets Manager rotated the secret. If the secret isn't configured for
* rotation, Secrets Manager returns null.
*/
public void setLastRotatedDate(java.util.Date lastRotatedDate) {
this.lastRotatedDate = lastRotatedDate;
}
/**
*
* The last date and time that Secrets Manager rotated the secret. If the secret isn't configured for rotation,
* Secrets Manager returns null.
*
*
* @return The last date and time that Secrets Manager rotated the secret. If the secret isn't configured for
* rotation, Secrets Manager returns null.
*/
public java.util.Date getLastRotatedDate() {
return this.lastRotatedDate;
}
/**
*
* The last date and time that Secrets Manager rotated the secret. If the secret isn't configured for rotation,
* Secrets Manager returns null.
*
*
* @param lastRotatedDate
* The last date and time that Secrets Manager rotated the secret. If the secret isn't configured for
* rotation, Secrets Manager returns null.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withLastRotatedDate(java.util.Date lastRotatedDate) {
setLastRotatedDate(lastRotatedDate);
return this;
}
/**
*
* The last date and time that this secret was modified in any way.
*
*
* @param lastChangedDate
* The last date and time that this secret was modified in any way.
*/
public void setLastChangedDate(java.util.Date lastChangedDate) {
this.lastChangedDate = lastChangedDate;
}
/**
*
* The last date and time that this secret was modified in any way.
*
*
* @return The last date and time that this secret was modified in any way.
*/
public java.util.Date getLastChangedDate() {
return this.lastChangedDate;
}
/**
*
* The last date and time that this secret was modified in any way.
*
*
* @param lastChangedDate
* The last date and time that this secret was modified in any way.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withLastChangedDate(java.util.Date lastChangedDate) {
setLastChangedDate(lastChangedDate);
return this;
}
/**
*
* The last date that the secret value was retrieved. This value does not include the time. This field is omitted if
* the secret has never been retrieved.
*
*
* @param lastAccessedDate
* The last date that the secret value was retrieved. This value does not include the time. This field is
* omitted if the secret has never been retrieved.
*/
public void setLastAccessedDate(java.util.Date lastAccessedDate) {
this.lastAccessedDate = lastAccessedDate;
}
/**
*
* The last date that the secret value was retrieved. This value does not include the time. This field is omitted if
* the secret has never been retrieved.
*
*
* @return The last date that the secret value was retrieved. This value does not include the time. This field is
* omitted if the secret has never been retrieved.
*/
public java.util.Date getLastAccessedDate() {
return this.lastAccessedDate;
}
/**
*
* The last date that the secret value was retrieved. This value does not include the time. This field is omitted if
* the secret has never been retrieved.
*
*
* @param lastAccessedDate
* The last date that the secret value was retrieved. This value does not include the time. This field is
* omitted if the secret has never been retrieved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withLastAccessedDate(java.util.Date lastAccessedDate) {
setLastAccessedDate(lastAccessedDate);
return this;
}
/**
*
* The date the secret is scheduled for deletion. If it is not scheduled for deletion, this field is omitted. When
* you delete a secret, Secrets Manager requires a recovery window of at least 7 days before deleting the secret.
* Some time after the deleted date, Secrets Manager deletes the secret, including all of its versions.
*
*
* If a secret is scheduled for deletion, then its details, including the encrypted secret value, is not accessible.
* To cancel a scheduled deletion and restore access to the secret, use RestoreSecret.
*
*
* @param deletedDate
* The date the secret is scheduled for deletion. If it is not scheduled for deletion, this field is omitted.
* When you delete a secret, Secrets Manager requires a recovery window of at least 7 days before deleting
* the secret. Some time after the deleted date, Secrets Manager deletes the secret, including all of its
* versions.
*
* If a secret is scheduled for deletion, then its details, including the encrypted secret value, is not
* accessible. To cancel a scheduled deletion and restore access to the secret, use RestoreSecret.
*/
public void setDeletedDate(java.util.Date deletedDate) {
this.deletedDate = deletedDate;
}
/**
*
* The date the secret is scheduled for deletion. If it is not scheduled for deletion, this field is omitted. When
* you delete a secret, Secrets Manager requires a recovery window of at least 7 days before deleting the secret.
* Some time after the deleted date, Secrets Manager deletes the secret, including all of its versions.
*
*
* If a secret is scheduled for deletion, then its details, including the encrypted secret value, is not accessible.
* To cancel a scheduled deletion and restore access to the secret, use RestoreSecret.
*
*
* @return The date the secret is scheduled for deletion. If it is not scheduled for deletion, this field is
* omitted. When you delete a secret, Secrets Manager requires a recovery window of at least 7 days before
* deleting the secret. Some time after the deleted date, Secrets Manager deletes the secret, including all
* of its versions.
*
* If a secret is scheduled for deletion, then its details, including the encrypted secret value, is not
* accessible. To cancel a scheduled deletion and restore access to the secret, use RestoreSecret.
*/
public java.util.Date getDeletedDate() {
return this.deletedDate;
}
/**
*
* The date the secret is scheduled for deletion. If it is not scheduled for deletion, this field is omitted. When
* you delete a secret, Secrets Manager requires a recovery window of at least 7 days before deleting the secret.
* Some time after the deleted date, Secrets Manager deletes the secret, including all of its versions.
*
*
* If a secret is scheduled for deletion, then its details, including the encrypted secret value, is not accessible.
* To cancel a scheduled deletion and restore access to the secret, use RestoreSecret.
*
*
* @param deletedDate
* The date the secret is scheduled for deletion. If it is not scheduled for deletion, this field is omitted.
* When you delete a secret, Secrets Manager requires a recovery window of at least 7 days before deleting
* the secret. Some time after the deleted date, Secrets Manager deletes the secret, including all of its
* versions.
*
* If a secret is scheduled for deletion, then its details, including the encrypted secret value, is not
* accessible. To cancel a scheduled deletion and restore access to the secret, use RestoreSecret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withDeletedDate(java.util.Date deletedDate) {
setDeletedDate(deletedDate);
return this;
}
/**
*
* The list of tags attached to the secret. To add tags to a secret, use TagResource. To remove tags, use
* UntagResource.
*
*
* @return The list of tags attached to the secret. To add tags to a secret, use TagResource. To remove tags,
* use UntagResource.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The list of tags attached to the secret. To add tags to a secret, use TagResource. To remove tags, use
* UntagResource.
*
*
* @param tags
* The list of tags attached to the secret. To add tags to a secret, use TagResource. To remove tags,
* use UntagResource.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The list of tags attached to the secret. To add tags to a secret, use TagResource. To remove tags, use
* UntagResource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The list of tags attached to the secret. To add tags to a secret, use TagResource. To remove tags,
* use UntagResource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The list of tags attached to the secret. To add tags to a secret, use TagResource. To remove tags, use
* UntagResource.
*
*
* @param tags
* The list of tags attached to the secret. To add tags to a secret, use TagResource. To remove tags,
* use UntagResource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* A list of the versions of the secret that have staging labels attached. Versions that don't have staging labels
* are considered deprecated and Secrets Manager can delete them.
*
*
* Secrets Manager uses staging labels to indicate the status of a secret version during rotation. The three staging
* labels for rotation are:
*
*
* -
*
* AWSCURRENT
, which indicates the current version of the secret.
*
*
* -
*
* AWSPENDING
, which indicates the version of the secret that contains new secret information that will
* become the next current version when rotation finishes.
*
*
* During rotation, Secrets Manager creates an AWSPENDING
version ID before creating the new secret
* version. To check if a secret version exists, call GetSecretValue.
*
*
* -
*
* AWSPREVIOUS
, which indicates the previous current version of the secret. You can use this as the
* last known good version.
*
*
*
*
* For more information about rotation and staging labels, see How rotation
* works.
*
*
* @return A list of the versions of the secret that have staging labels attached. Versions that don't have staging
* labels are considered deprecated and Secrets Manager can delete them.
*
* Secrets Manager uses staging labels to indicate the status of a secret version during rotation. The three
* staging labels for rotation are:
*
*
* -
*
* AWSCURRENT
, which indicates the current version of the secret.
*
*
* -
*
* AWSPENDING
, which indicates the version of the secret that contains new secret information
* that will become the next current version when rotation finishes.
*
*
* During rotation, Secrets Manager creates an AWSPENDING
version ID before creating the new
* secret version. To check if a secret version exists, call GetSecretValue.
*
*
* -
*
* AWSPREVIOUS
, which indicates the previous current version of the secret. You can use this as
* the last known good version.
*
*
*
*
* For more information about rotation and staging labels, see How rotation
* works.
*/
public java.util.Map> getVersionIdsToStages() {
return versionIdsToStages;
}
/**
*
* A list of the versions of the secret that have staging labels attached. Versions that don't have staging labels
* are considered deprecated and Secrets Manager can delete them.
*
*
* Secrets Manager uses staging labels to indicate the status of a secret version during rotation. The three staging
* labels for rotation are:
*
*
* -
*
* AWSCURRENT
, which indicates the current version of the secret.
*
*
* -
*
* AWSPENDING
, which indicates the version of the secret that contains new secret information that will
* become the next current version when rotation finishes.
*
*
* During rotation, Secrets Manager creates an AWSPENDING
version ID before creating the new secret
* version. To check if a secret version exists, call GetSecretValue.
*
*
* -
*
* AWSPREVIOUS
, which indicates the previous current version of the secret. You can use this as the
* last known good version.
*
*
*
*
* For more information about rotation and staging labels, see How rotation
* works.
*
*
* @param versionIdsToStages
* A list of the versions of the secret that have staging labels attached. Versions that don't have staging
* labels are considered deprecated and Secrets Manager can delete them.
*
* Secrets Manager uses staging labels to indicate the status of a secret version during rotation. The three
* staging labels for rotation are:
*
*
* -
*
* AWSCURRENT
, which indicates the current version of the secret.
*
*
* -
*
* AWSPENDING
, which indicates the version of the secret that contains new secret information
* that will become the next current version when rotation finishes.
*
*
* During rotation, Secrets Manager creates an AWSPENDING
version ID before creating the new
* secret version. To check if a secret version exists, call GetSecretValue.
*
*
* -
*
* AWSPREVIOUS
, which indicates the previous current version of the secret. You can use this as
* the last known good version.
*
*
*
*
* For more information about rotation and staging labels, see How rotation
* works.
*/
public void setVersionIdsToStages(java.util.Map> versionIdsToStages) {
this.versionIdsToStages = versionIdsToStages;
}
/**
*
* A list of the versions of the secret that have staging labels attached. Versions that don't have staging labels
* are considered deprecated and Secrets Manager can delete them.
*
*
* Secrets Manager uses staging labels to indicate the status of a secret version during rotation. The three staging
* labels for rotation are:
*
*
* -
*
* AWSCURRENT
, which indicates the current version of the secret.
*
*
* -
*
* AWSPENDING
, which indicates the version of the secret that contains new secret information that will
* become the next current version when rotation finishes.
*
*
* During rotation, Secrets Manager creates an AWSPENDING
version ID before creating the new secret
* version. To check if a secret version exists, call GetSecretValue.
*
*
* -
*
* AWSPREVIOUS
, which indicates the previous current version of the secret. You can use this as the
* last known good version.
*
*
*
*
* For more information about rotation and staging labels, see How rotation
* works.
*
*
* @param versionIdsToStages
* A list of the versions of the secret that have staging labels attached. Versions that don't have staging
* labels are considered deprecated and Secrets Manager can delete them.
*
* Secrets Manager uses staging labels to indicate the status of a secret version during rotation. The three
* staging labels for rotation are:
*
*
* -
*
* AWSCURRENT
, which indicates the current version of the secret.
*
*
* -
*
* AWSPENDING
, which indicates the version of the secret that contains new secret information
* that will become the next current version when rotation finishes.
*
*
* During rotation, Secrets Manager creates an AWSPENDING
version ID before creating the new
* secret version. To check if a secret version exists, call GetSecretValue.
*
*
* -
*
* AWSPREVIOUS
, which indicates the previous current version of the secret. You can use this as
* the last known good version.
*
*
*
*
* For more information about rotation and staging labels, see How rotation
* works.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withVersionIdsToStages(java.util.Map> versionIdsToStages) {
setVersionIdsToStages(versionIdsToStages);
return this;
}
/**
* Add a single VersionIdsToStages entry
*
* @see DescribeSecretResult#withVersionIdsToStages
* @returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult addVersionIdsToStagesEntry(String key, java.util.List value) {
if (null == this.versionIdsToStages) {
this.versionIdsToStages = new java.util.HashMap>();
}
if (this.versionIdsToStages.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.versionIdsToStages.put(key, value);
return this;
}
/**
* Removes all the entries added into VersionIdsToStages.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult clearVersionIdsToStagesEntries() {
this.versionIdsToStages = null;
return this;
}
/**
*
* The name of the service that created this secret.
*
*
* @param owningService
* The name of the service that created this secret.
*/
public void setOwningService(String owningService) {
this.owningService = owningService;
}
/**
*
* The name of the service that created this secret.
*
*
* @return The name of the service that created this secret.
*/
public String getOwningService() {
return this.owningService;
}
/**
*
* The name of the service that created this secret.
*
*
* @param owningService
* The name of the service that created this secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withOwningService(String owningService) {
setOwningService(owningService);
return this;
}
/**
*
* The date the secret was created.
*
*
* @param createdDate
* The date the secret was created.
*/
public void setCreatedDate(java.util.Date createdDate) {
this.createdDate = createdDate;
}
/**
*
* The date the secret was created.
*
*
* @return The date the secret was created.
*/
public java.util.Date getCreatedDate() {
return this.createdDate;
}
/**
*
* The date the secret was created.
*
*
* @param createdDate
* The date the secret was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withCreatedDate(java.util.Date createdDate) {
setCreatedDate(createdDate);
return this;
}
/**
*
* The Region the secret is in. If a secret is replicated to other Regions, the replicas are listed in
* ReplicationStatus
.
*
*
* @param primaryRegion
* The Region the secret is in. If a secret is replicated to other Regions, the replicas are listed in
* ReplicationStatus
.
*/
public void setPrimaryRegion(String primaryRegion) {
this.primaryRegion = primaryRegion;
}
/**
*
* The Region the secret is in. If a secret is replicated to other Regions, the replicas are listed in
* ReplicationStatus
.
*
*
* @return The Region the secret is in. If a secret is replicated to other Regions, the replicas are listed in
* ReplicationStatus
.
*/
public String getPrimaryRegion() {
return this.primaryRegion;
}
/**
*
* The Region the secret is in. If a secret is replicated to other Regions, the replicas are listed in
* ReplicationStatus
.
*
*
* @param primaryRegion
* The Region the secret is in. If a secret is replicated to other Regions, the replicas are listed in
* ReplicationStatus
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withPrimaryRegion(String primaryRegion) {
setPrimaryRegion(primaryRegion);
return this;
}
/**
*
* A list of the replicas of this secret and their status:
*
*
* -
*
* Failed
, which indicates that the replica was not created.
*
*
* -
*
* InProgress
, which indicates that Secrets Manager is in the process of creating the replica.
*
*
* -
*
* InSync
, which indicates that the replica was created.
*
*
*
*
* @return A list of the replicas of this secret and their status:
*
* -
*
* Failed
, which indicates that the replica was not created.
*
*
* -
*
* InProgress
, which indicates that Secrets Manager is in the process of creating the replica.
*
*
* -
*
* InSync
, which indicates that the replica was created.
*
*
*/
public java.util.List getReplicationStatus() {
return replicationStatus;
}
/**
*
* A list of the replicas of this secret and their status:
*
*
* -
*
* Failed
, which indicates that the replica was not created.
*
*
* -
*
* InProgress
, which indicates that Secrets Manager is in the process of creating the replica.
*
*
* -
*
* InSync
, which indicates that the replica was created.
*
*
*
*
* @param replicationStatus
* A list of the replicas of this secret and their status:
*
* -
*
* Failed
, which indicates that the replica was not created.
*
*
* -
*
* InProgress
, which indicates that Secrets Manager is in the process of creating the replica.
*
*
* -
*
* InSync
, which indicates that the replica was created.
*
*
*/
public void setReplicationStatus(java.util.Collection replicationStatus) {
if (replicationStatus == null) {
this.replicationStatus = null;
return;
}
this.replicationStatus = new java.util.ArrayList(replicationStatus);
}
/**
*
* A list of the replicas of this secret and their status:
*
*
* -
*
* Failed
, which indicates that the replica was not created.
*
*
* -
*
* InProgress
, which indicates that Secrets Manager is in the process of creating the replica.
*
*
* -
*
* InSync
, which indicates that the replica was created.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setReplicationStatus(java.util.Collection)} or {@link #withReplicationStatus(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param replicationStatus
* A list of the replicas of this secret and their status:
*
* -
*
* Failed
, which indicates that the replica was not created.
*
*
* -
*
* InProgress
, which indicates that Secrets Manager is in the process of creating the replica.
*
*
* -
*
* InSync
, which indicates that the replica was created.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withReplicationStatus(ReplicationStatusType... replicationStatus) {
if (this.replicationStatus == null) {
setReplicationStatus(new java.util.ArrayList(replicationStatus.length));
}
for (ReplicationStatusType ele : replicationStatus) {
this.replicationStatus.add(ele);
}
return this;
}
/**
*
* A list of the replicas of this secret and their status:
*
*
* -
*
* Failed
, which indicates that the replica was not created.
*
*
* -
*
* InProgress
, which indicates that Secrets Manager is in the process of creating the replica.
*
*
* -
*
* InSync
, which indicates that the replica was created.
*
*
*
*
* @param replicationStatus
* A list of the replicas of this secret and their status:
*
* -
*
* Failed
, which indicates that the replica was not created.
*
*
* -
*
* InProgress
, which indicates that Secrets Manager is in the process of creating the replica.
*
*
* -
*
* InSync
, which indicates that the replica was created.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DescribeSecretResult withReplicationStatus(java.util.Collection replicationStatus) {
setReplicationStatus(replicationStatus);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getARN() != null)
sb.append("ARN: ").append(getARN()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getRotationEnabled() != null)
sb.append("RotationEnabled: ").append(getRotationEnabled()).append(",");
if (getRotationLambdaARN() != null)
sb.append("RotationLambdaARN: ").append(getRotationLambdaARN()).append(",");
if (getRotationRules() != null)
sb.append("RotationRules: ").append(getRotationRules()).append(",");
if (getLastRotatedDate() != null)
sb.append("LastRotatedDate: ").append(getLastRotatedDate()).append(",");
if (getLastChangedDate() != null)
sb.append("LastChangedDate: ").append(getLastChangedDate()).append(",");
if (getLastAccessedDate() != null)
sb.append("LastAccessedDate: ").append(getLastAccessedDate()).append(",");
if (getDeletedDate() != null)
sb.append("DeletedDate: ").append(getDeletedDate()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getVersionIdsToStages() != null)
sb.append("VersionIdsToStages: ").append(getVersionIdsToStages()).append(",");
if (getOwningService() != null)
sb.append("OwningService: ").append(getOwningService()).append(",");
if (getCreatedDate() != null)
sb.append("CreatedDate: ").append(getCreatedDate()).append(",");
if (getPrimaryRegion() != null)
sb.append("PrimaryRegion: ").append(getPrimaryRegion()).append(",");
if (getReplicationStatus() != null)
sb.append("ReplicationStatus: ").append(getReplicationStatus());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeSecretResult == false)
return false;
DescribeSecretResult other = (DescribeSecretResult) obj;
if (other.getARN() == null ^ this.getARN() == null)
return false;
if (other.getARN() != null && other.getARN().equals(this.getARN()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getRotationEnabled() == null ^ this.getRotationEnabled() == null)
return false;
if (other.getRotationEnabled() != null && other.getRotationEnabled().equals(this.getRotationEnabled()) == false)
return false;
if (other.getRotationLambdaARN() == null ^ this.getRotationLambdaARN() == null)
return false;
if (other.getRotationLambdaARN() != null && other.getRotationLambdaARN().equals(this.getRotationLambdaARN()) == false)
return false;
if (other.getRotationRules() == null ^ this.getRotationRules() == null)
return false;
if (other.getRotationRules() != null && other.getRotationRules().equals(this.getRotationRules()) == false)
return false;
if (other.getLastRotatedDate() == null ^ this.getLastRotatedDate() == null)
return false;
if (other.getLastRotatedDate() != null && other.getLastRotatedDate().equals(this.getLastRotatedDate()) == false)
return false;
if (other.getLastChangedDate() == null ^ this.getLastChangedDate() == null)
return false;
if (other.getLastChangedDate() != null && other.getLastChangedDate().equals(this.getLastChangedDate()) == false)
return false;
if (other.getLastAccessedDate() == null ^ this.getLastAccessedDate() == null)
return false;
if (other.getLastAccessedDate() != null && other.getLastAccessedDate().equals(this.getLastAccessedDate()) == false)
return false;
if (other.getDeletedDate() == null ^ this.getDeletedDate() == null)
return false;
if (other.getDeletedDate() != null && other.getDeletedDate().equals(this.getDeletedDate()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getVersionIdsToStages() == null ^ this.getVersionIdsToStages() == null)
return false;
if (other.getVersionIdsToStages() != null && other.getVersionIdsToStages().equals(this.getVersionIdsToStages()) == false)
return false;
if (other.getOwningService() == null ^ this.getOwningService() == null)
return false;
if (other.getOwningService() != null && other.getOwningService().equals(this.getOwningService()) == false)
return false;
if (other.getCreatedDate() == null ^ this.getCreatedDate() == null)
return false;
if (other.getCreatedDate() != null && other.getCreatedDate().equals(this.getCreatedDate()) == false)
return false;
if (other.getPrimaryRegion() == null ^ this.getPrimaryRegion() == null)
return false;
if (other.getPrimaryRegion() != null && other.getPrimaryRegion().equals(this.getPrimaryRegion()) == false)
return false;
if (other.getReplicationStatus() == null ^ this.getReplicationStatus() == null)
return false;
if (other.getReplicationStatus() != null && other.getReplicationStatus().equals(this.getReplicationStatus()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getARN() == null) ? 0 : getARN().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getRotationEnabled() == null) ? 0 : getRotationEnabled().hashCode());
hashCode = prime * hashCode + ((getRotationLambdaARN() == null) ? 0 : getRotationLambdaARN().hashCode());
hashCode = prime * hashCode + ((getRotationRules() == null) ? 0 : getRotationRules().hashCode());
hashCode = prime * hashCode + ((getLastRotatedDate() == null) ? 0 : getLastRotatedDate().hashCode());
hashCode = prime * hashCode + ((getLastChangedDate() == null) ? 0 : getLastChangedDate().hashCode());
hashCode = prime * hashCode + ((getLastAccessedDate() == null) ? 0 : getLastAccessedDate().hashCode());
hashCode = prime * hashCode + ((getDeletedDate() == null) ? 0 : getDeletedDate().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getVersionIdsToStages() == null) ? 0 : getVersionIdsToStages().hashCode());
hashCode = prime * hashCode + ((getOwningService() == null) ? 0 : getOwningService().hashCode());
hashCode = prime * hashCode + ((getCreatedDate() == null) ? 0 : getCreatedDate().hashCode());
hashCode = prime * hashCode + ((getPrimaryRegion() == null) ? 0 : getPrimaryRegion().hashCode());
hashCode = prime * hashCode + ((getReplicationStatus() == null) ? 0 : getReplicationStatus().hashCode());
return hashCode;
}
@Override
public DescribeSecretResult clone() {
try {
return (DescribeSecretResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}