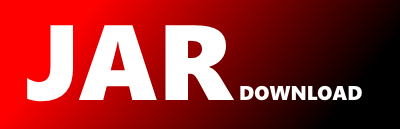
com.amazonaws.services.secretsmanager.model.GetSecretValueResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-secretsmanager Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.secretsmanager.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetSecretValueResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The ARN of the secret.
*
*/
private String aRN;
/**
*
* The friendly name of the secret.
*
*/
private String name;
/**
*
* The unique identifier of this version of the secret.
*
*/
private String versionId;
/**
*
* The decrypted secret value, if the secret value was originally provided as binary data in the form of a byte
* array. The response parameter represents the binary data as a base64-encoded string.
*
*
* If the secret was created by using the Secrets Manager console, or if the secret value was originally provided as
* a string, then this field is omitted. The secret value appears in SecretString
instead.
*
*/
private java.nio.ByteBuffer secretBinary;
/**
*
* The decrypted secret value, if the secret value was originally provided as a string or through the Secrets
* Manager console.
*
*
* If this secret was created by using the console, then Secrets Manager stores the information as a JSON structure
* of key/value pairs.
*
*/
private String secretString;
/**
*
* A list of all of the staging labels currently attached to this version of the secret.
*
*/
private java.util.List versionStages;
/**
*
* The date and time that this version of the secret was created. If you don't specify which version in
* VersionId
or VersionStage
, then Secrets Manager uses the AWSCURRENT
* version.
*
*/
private java.util.Date createdDate;
/**
*
* The ARN of the secret.
*
*
* @param aRN
* The ARN of the secret.
*/
public void setARN(String aRN) {
this.aRN = aRN;
}
/**
*
* The ARN of the secret.
*
*
* @return The ARN of the secret.
*/
public String getARN() {
return this.aRN;
}
/**
*
* The ARN of the secret.
*
*
* @param aRN
* The ARN of the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSecretValueResult withARN(String aRN) {
setARN(aRN);
return this;
}
/**
*
* The friendly name of the secret.
*
*
* @param name
* The friendly name of the secret.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The friendly name of the secret.
*
*
* @return The friendly name of the secret.
*/
public String getName() {
return this.name;
}
/**
*
* The friendly name of the secret.
*
*
* @param name
* The friendly name of the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSecretValueResult withName(String name) {
setName(name);
return this;
}
/**
*
* The unique identifier of this version of the secret.
*
*
* @param versionId
* The unique identifier of this version of the secret.
*/
public void setVersionId(String versionId) {
this.versionId = versionId;
}
/**
*
* The unique identifier of this version of the secret.
*
*
* @return The unique identifier of this version of the secret.
*/
public String getVersionId() {
return this.versionId;
}
/**
*
* The unique identifier of this version of the secret.
*
*
* @param versionId
* The unique identifier of this version of the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSecretValueResult withVersionId(String versionId) {
setVersionId(versionId);
return this;
}
/**
*
* The decrypted secret value, if the secret value was originally provided as binary data in the form of a byte
* array. The response parameter represents the binary data as a base64-encoded string.
*
*
* If the secret was created by using the Secrets Manager console, or if the secret value was originally provided as
* a string, then this field is omitted. The secret value appears in SecretString
instead.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param secretBinary
* The decrypted secret value, if the secret value was originally provided as binary data in the form of a
* byte array. The response parameter represents the binary data as a base64-encoded string.
*
* If the secret was created by using the Secrets Manager console, or if the secret value was originally
* provided as a string, then this field is omitted. The secret value appears in SecretString
* instead.
*/
public void setSecretBinary(java.nio.ByteBuffer secretBinary) {
this.secretBinary = secretBinary;
}
/**
*
* The decrypted secret value, if the secret value was originally provided as binary data in the form of a byte
* array. The response parameter represents the binary data as a base64-encoded string.
*
*
* If the secret was created by using the Secrets Manager console, or if the secret value was originally provided as
* a string, then this field is omitted. The secret value appears in SecretString
instead.
*
*
* {@code ByteBuffer}s are stateful. Calling their {@code get} methods changes their {@code position}. We recommend
* using {@link java.nio.ByteBuffer#asReadOnlyBuffer()} to create a read-only view of the buffer with an independent
* {@code position}, and calling {@code get} methods on this rather than directly on the returned {@code ByteBuffer}.
* Doing so will ensure that anyone else using the {@code ByteBuffer} will not be affected by changes to the
* {@code position}.
*
*
* @return The decrypted secret value, if the secret value was originally provided as binary data in the form of a
* byte array. The response parameter represents the binary data as a base64-encoded string.
*
* If the secret was created by using the Secrets Manager console, or if the secret value was originally
* provided as a string, then this field is omitted. The secret value appears in SecretString
* instead.
*/
public java.nio.ByteBuffer getSecretBinary() {
return this.secretBinary;
}
/**
*
* The decrypted secret value, if the secret value was originally provided as binary data in the form of a byte
* array. The response parameter represents the binary data as a base64-encoded string.
*
*
* If the secret was created by using the Secrets Manager console, or if the secret value was originally provided as
* a string, then this field is omitted. The secret value appears in SecretString
instead.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param secretBinary
* The decrypted secret value, if the secret value was originally provided as binary data in the form of a
* byte array. The response parameter represents the binary data as a base64-encoded string.
*
* If the secret was created by using the Secrets Manager console, or if the secret value was originally
* provided as a string, then this field is omitted. The secret value appears in SecretString
* instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSecretValueResult withSecretBinary(java.nio.ByteBuffer secretBinary) {
setSecretBinary(secretBinary);
return this;
}
/**
*
* The decrypted secret value, if the secret value was originally provided as a string or through the Secrets
* Manager console.
*
*
* If this secret was created by using the console, then Secrets Manager stores the information as a JSON structure
* of key/value pairs.
*
*
* @param secretString
* The decrypted secret value, if the secret value was originally provided as a string or through the Secrets
* Manager console.
*
* If this secret was created by using the console, then Secrets Manager stores the information as a JSON
* structure of key/value pairs.
*/
public void setSecretString(String secretString) {
this.secretString = secretString;
}
/**
*
* The decrypted secret value, if the secret value was originally provided as a string or through the Secrets
* Manager console.
*
*
* If this secret was created by using the console, then Secrets Manager stores the information as a JSON structure
* of key/value pairs.
*
*
* @return The decrypted secret value, if the secret value was originally provided as a string or through the
* Secrets Manager console.
*
* If this secret was created by using the console, then Secrets Manager stores the information as a JSON
* structure of key/value pairs.
*/
public String getSecretString() {
return this.secretString;
}
/**
*
* The decrypted secret value, if the secret value was originally provided as a string or through the Secrets
* Manager console.
*
*
* If this secret was created by using the console, then Secrets Manager stores the information as a JSON structure
* of key/value pairs.
*
*
* @param secretString
* The decrypted secret value, if the secret value was originally provided as a string or through the Secrets
* Manager console.
*
* If this secret was created by using the console, then Secrets Manager stores the information as a JSON
* structure of key/value pairs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSecretValueResult withSecretString(String secretString) {
setSecretString(secretString);
return this;
}
/**
*
* A list of all of the staging labels currently attached to this version of the secret.
*
*
* @return A list of all of the staging labels currently attached to this version of the secret.
*/
public java.util.List getVersionStages() {
return versionStages;
}
/**
*
* A list of all of the staging labels currently attached to this version of the secret.
*
*
* @param versionStages
* A list of all of the staging labels currently attached to this version of the secret.
*/
public void setVersionStages(java.util.Collection versionStages) {
if (versionStages == null) {
this.versionStages = null;
return;
}
this.versionStages = new java.util.ArrayList(versionStages);
}
/**
*
* A list of all of the staging labels currently attached to this version of the secret.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVersionStages(java.util.Collection)} or {@link #withVersionStages(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param versionStages
* A list of all of the staging labels currently attached to this version of the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSecretValueResult withVersionStages(String... versionStages) {
if (this.versionStages == null) {
setVersionStages(new java.util.ArrayList(versionStages.length));
}
for (String ele : versionStages) {
this.versionStages.add(ele);
}
return this;
}
/**
*
* A list of all of the staging labels currently attached to this version of the secret.
*
*
* @param versionStages
* A list of all of the staging labels currently attached to this version of the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSecretValueResult withVersionStages(java.util.Collection versionStages) {
setVersionStages(versionStages);
return this;
}
/**
*
* The date and time that this version of the secret was created. If you don't specify which version in
* VersionId
or VersionStage
, then Secrets Manager uses the AWSCURRENT
* version.
*
*
* @param createdDate
* The date and time that this version of the secret was created. If you don't specify which version in
* VersionId
or VersionStage
, then Secrets Manager uses the AWSCURRENT
* version.
*/
public void setCreatedDate(java.util.Date createdDate) {
this.createdDate = createdDate;
}
/**
*
* The date and time that this version of the secret was created. If you don't specify which version in
* VersionId
or VersionStage
, then Secrets Manager uses the AWSCURRENT
* version.
*
*
* @return The date and time that this version of the secret was created. If you don't specify which version in
* VersionId
or VersionStage
, then Secrets Manager uses the
* AWSCURRENT
version.
*/
public java.util.Date getCreatedDate() {
return this.createdDate;
}
/**
*
* The date and time that this version of the secret was created. If you don't specify which version in
* VersionId
or VersionStage
, then Secrets Manager uses the AWSCURRENT
* version.
*
*
* @param createdDate
* The date and time that this version of the secret was created. If you don't specify which version in
* VersionId
or VersionStage
, then Secrets Manager uses the AWSCURRENT
* version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetSecretValueResult withCreatedDate(java.util.Date createdDate) {
setCreatedDate(createdDate);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getARN() != null)
sb.append("ARN: ").append(getARN()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getVersionId() != null)
sb.append("VersionId: ").append(getVersionId()).append(",");
if (getSecretBinary() != null)
sb.append("SecretBinary: ").append("***Sensitive Data Redacted***").append(",");
if (getSecretString() != null)
sb.append("SecretString: ").append("***Sensitive Data Redacted***").append(",");
if (getVersionStages() != null)
sb.append("VersionStages: ").append(getVersionStages()).append(",");
if (getCreatedDate() != null)
sb.append("CreatedDate: ").append(getCreatedDate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetSecretValueResult == false)
return false;
GetSecretValueResult other = (GetSecretValueResult) obj;
if (other.getARN() == null ^ this.getARN() == null)
return false;
if (other.getARN() != null && other.getARN().equals(this.getARN()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getVersionId() == null ^ this.getVersionId() == null)
return false;
if (other.getVersionId() != null && other.getVersionId().equals(this.getVersionId()) == false)
return false;
if (other.getSecretBinary() == null ^ this.getSecretBinary() == null)
return false;
if (other.getSecretBinary() != null && other.getSecretBinary().equals(this.getSecretBinary()) == false)
return false;
if (other.getSecretString() == null ^ this.getSecretString() == null)
return false;
if (other.getSecretString() != null && other.getSecretString().equals(this.getSecretString()) == false)
return false;
if (other.getVersionStages() == null ^ this.getVersionStages() == null)
return false;
if (other.getVersionStages() != null && other.getVersionStages().equals(this.getVersionStages()) == false)
return false;
if (other.getCreatedDate() == null ^ this.getCreatedDate() == null)
return false;
if (other.getCreatedDate() != null && other.getCreatedDate().equals(this.getCreatedDate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getARN() == null) ? 0 : getARN().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getVersionId() == null) ? 0 : getVersionId().hashCode());
hashCode = prime * hashCode + ((getSecretBinary() == null) ? 0 : getSecretBinary().hashCode());
hashCode = prime * hashCode + ((getSecretString() == null) ? 0 : getSecretString().hashCode());
hashCode = prime * hashCode + ((getVersionStages() == null) ? 0 : getVersionStages().hashCode());
hashCode = prime * hashCode + ((getCreatedDate() == null) ? 0 : getCreatedDate().hashCode());
return hashCode;
}
@Override
public GetSecretValueResult clone() {
try {
return (GetSecretValueResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}