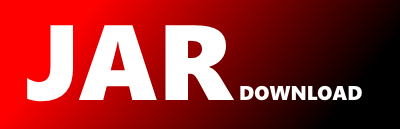
com.amazonaws.services.secretsmanager.model.CreateSecretRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-secretsmanager Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.secretsmanager.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateSecretRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name of the new secret.
*
*
* The secret name can contain ASCII letters, numbers, and the following characters: /_+=.@-
*
*
* Do not end your secret name with a hyphen followed by six characters. If you do so, you risk confusion and
* unexpected results when searching for a secret by partial ARN. Secrets Manager automatically adds a hyphen and
* six random characters after the secret name at the end of the ARN.
*
*/
private String name;
/**
*
* If you include SecretString
or SecretBinary
, then Secrets Manager creates an initial
* version for the secret, and this parameter specifies the unique identifier for the new version.
*
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then you
* can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the value for
* this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a UUID-type value to ensure uniqueness of your
* versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a new
* version of the secret is created.
*
*
* -
*
* If a version with this value already exists and the version SecretString
and
* SecretBinary
values are the same as those in the request, then the request is ignored.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because you
* cannot modify an existing version. Instead, use PutSecretValue to create a new version.
*
*
*
*
* This value becomes the VersionId
of the new version.
*
*/
private String clientRequestToken;
/**
*
* The description of the secret.
*
*/
private String description;
/**
*
* The ARN, key ID, or alias of the KMS key that Secrets Manager uses to encrypt the secret value in the secret. An
* alias is always prefixed by alias/
, for example alias/aws/secretsmanager
. For more
* information, see About
* aliases.
*
*
* To use a KMS key in a different account, use the key ARN or the alias ARN.
*
*
* If you don't specify this value, then Secrets Manager uses the key aws/secretsmanager
. If that key
* doesn't yet exist, then Secrets Manager creates it for you automatically the first time it encrypts the secret
* value.
*
*
* If the secret is in a different Amazon Web Services account from the credentials calling the API, then you can't
* use aws/secretsmanager
to encrypt the secret, and you must create and use a customer managed KMS
* key.
*
*/
private String kmsKeyId;
/**
*
* The binary data to encrypt and store in the new version of the secret. We recommend that you store your binary
* data in a file and then pass the contents of the file as a parameter.
*
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* This parameter is not available in the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*/
private java.nio.ByteBuffer secretBinary;
/**
*
* The text data to encrypt and store in this new version of the secret. We recommend you use a JSON structure of
* key/value pairs for your secret value.
*
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* If you create a secret by using the Secrets Manager console then Secrets Manager puts the protected secret text
* in only the SecretString
parameter. The Secrets Manager console stores the information as a JSON
* structure of key/value pairs that a Lambda rotation function can parse.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*/
private String secretString;
/**
*
* A list of tags to attach to the secret. Each tag is a key and value pair of strings in a JSON text string, for
* example:
*
*
* [{"Key":"CostCenter","Value":"12345"},{"Key":"environment","Value":"production"}]
*
*
* Secrets Manager tag key names are case sensitive. A tag with the key "ABC" is a different tag from one with key
* "abc".
*
*
* If you check tags in permissions policies as part of your security strategy, then adding or removing a tag can
* change permissions. If the completion of this operation would result in you losing your permissions for this
* secret, then Secrets Manager blocks the operation and returns an Access Denied
error. For more
* information, see Control access to secrets using tags and Limit access to identities with tags that match secrets' tags.
*
*
* For information about how to format a JSON parameter for the various command line tool environments, see Using JSON for
* Parameters. If your command-line tool or SDK requires quotation marks around the parameter, you should use
* single quotes to avoid confusion with the double quotes required in the JSON text.
*
*
* For tag quotas and naming restrictions, see Service quotas for
* Tagging in the Amazon Web Services General Reference guide.
*
*/
private java.util.List tags;
/**
*
* A list of Regions and KMS keys to replicate secrets.
*
*/
private java.util.List addReplicaRegions;
/**
*
* Specifies whether to overwrite a secret with the same name in the destination Region. By default, secrets aren't
* overwritten.
*
*/
private Boolean forceOverwriteReplicaSecret;
/**
*
* The name of the new secret.
*
*
* The secret name can contain ASCII letters, numbers, and the following characters: /_+=.@-
*
*
* Do not end your secret name with a hyphen followed by six characters. If you do so, you risk confusion and
* unexpected results when searching for a secret by partial ARN. Secrets Manager automatically adds a hyphen and
* six random characters after the secret name at the end of the ARN.
*
*
* @param name
* The name of the new secret.
*
* The secret name can contain ASCII letters, numbers, and the following characters: /_+=.@-
*
*
* Do not end your secret name with a hyphen followed by six characters. If you do so, you risk confusion and
* unexpected results when searching for a secret by partial ARN. Secrets Manager automatically adds a hyphen
* and six random characters after the secret name at the end of the ARN.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the new secret.
*
*
* The secret name can contain ASCII letters, numbers, and the following characters: /_+=.@-
*
*
* Do not end your secret name with a hyphen followed by six characters. If you do so, you risk confusion and
* unexpected results when searching for a secret by partial ARN. Secrets Manager automatically adds a hyphen and
* six random characters after the secret name at the end of the ARN.
*
*
* @return The name of the new secret.
*
* The secret name can contain ASCII letters, numbers, and the following characters: /_+=.@-
*
*
* Do not end your secret name with a hyphen followed by six characters. If you do so, you risk confusion
* and unexpected results when searching for a secret by partial ARN. Secrets Manager automatically adds a
* hyphen and six random characters after the secret name at the end of the ARN.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the new secret.
*
*
* The secret name can contain ASCII letters, numbers, and the following characters: /_+=.@-
*
*
* Do not end your secret name with a hyphen followed by six characters. If you do so, you risk confusion and
* unexpected results when searching for a secret by partial ARN. Secrets Manager automatically adds a hyphen and
* six random characters after the secret name at the end of the ARN.
*
*
* @param name
* The name of the new secret.
*
* The secret name can contain ASCII letters, numbers, and the following characters: /_+=.@-
*
*
* Do not end your secret name with a hyphen followed by six characters. If you do so, you risk confusion and
* unexpected results when searching for a secret by partial ARN. Secrets Manager automatically adds a hyphen
* and six random characters after the secret name at the end of the ARN.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withName(String name) {
setName(name);
return this;
}
/**
*
* If you include SecretString
or SecretBinary
, then Secrets Manager creates an initial
* version for the secret, and this parameter specifies the unique identifier for the new version.
*
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then you
* can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the value for
* this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a UUID-type value to ensure uniqueness of your
* versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a new
* version of the secret is created.
*
*
* -
*
* If a version with this value already exists and the version SecretString
and
* SecretBinary
values are the same as those in the request, then the request is ignored.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because you
* cannot modify an existing version. Instead, use PutSecretValue to create a new version.
*
*
*
*
* This value becomes the VersionId
of the new version.
*
*
* @param clientRequestToken
* If you include SecretString
or SecretBinary
, then Secrets Manager creates an
* initial version for the secret, and this parameter specifies the unique identifier for the new version.
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then
* you can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the
* value for this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a
* UUID-type value to ensure
* uniqueness of your versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a
* new version of the secret is created.
*
*
* -
*
* If a version with this value already exists and the version SecretString
and
* SecretBinary
values are the same as those in the request, then the request is ignored.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because
* you cannot modify an existing version. Instead, use PutSecretValue to create a new version.
*
*
*
*
* This value becomes the VersionId
of the new version.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* If you include SecretString
or SecretBinary
, then Secrets Manager creates an initial
* version for the secret, and this parameter specifies the unique identifier for the new version.
*
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then you
* can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the value for
* this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a UUID-type value to ensure uniqueness of your
* versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a new
* version of the secret is created.
*
*
* -
*
* If a version with this value already exists and the version SecretString
and
* SecretBinary
values are the same as those in the request, then the request is ignored.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because you
* cannot modify an existing version. Instead, use PutSecretValue to create a new version.
*
*
*
*
* This value becomes the VersionId
of the new version.
*
*
* @return If you include SecretString
or SecretBinary
, then Secrets Manager creates an
* initial version for the secret, and this parameter specifies the unique identifier for the new version.
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation,
* then you can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it
* as the value for this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation
* of duplicate versions if there are failures and retries during a rotation. We recommend that you generate
* a UUID-type value to ensure
* uniqueness of your versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a
* new version of the secret is created.
*
*
* -
*
* If a version with this value already exists and the version SecretString
and
* SecretBinary
values are the same as those in the request, then the request is ignored.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because
* you cannot modify an existing version. Instead, use PutSecretValue to create a new version.
*
*
*
*
* This value becomes the VersionId
of the new version.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* If you include SecretString
or SecretBinary
, then Secrets Manager creates an initial
* version for the secret, and this parameter specifies the unique identifier for the new version.
*
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then you
* can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the value for
* this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a UUID-type value to ensure uniqueness of your
* versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a new
* version of the secret is created.
*
*
* -
*
* If a version with this value already exists and the version SecretString
and
* SecretBinary
values are the same as those in the request, then the request is ignored.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because you
* cannot modify an existing version. Instead, use PutSecretValue to create a new version.
*
*
*
*
* This value becomes the VersionId
of the new version.
*
*
* @param clientRequestToken
* If you include SecretString
or SecretBinary
, then Secrets Manager creates an
* initial version for the secret, and this parameter specifies the unique identifier for the new version.
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then
* you can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the
* value for this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a
* UUID-type value to ensure
* uniqueness of your versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a
* new version of the secret is created.
*
*
* -
*
* If a version with this value already exists and the version SecretString
and
* SecretBinary
values are the same as those in the request, then the request is ignored.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because
* you cannot modify an existing version. Instead, use PutSecretValue to create a new version.
*
*
*
*
* This value becomes the VersionId
of the new version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* The description of the secret.
*
*
* @param description
* The description of the secret.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the secret.
*
*
* @return The description of the secret.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the secret.
*
*
* @param description
* The description of the secret.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The ARN, key ID, or alias of the KMS key that Secrets Manager uses to encrypt the secret value in the secret. An
* alias is always prefixed by alias/
, for example alias/aws/secretsmanager
. For more
* information, see About
* aliases.
*
*
* To use a KMS key in a different account, use the key ARN or the alias ARN.
*
*
* If you don't specify this value, then Secrets Manager uses the key aws/secretsmanager
. If that key
* doesn't yet exist, then Secrets Manager creates it for you automatically the first time it encrypts the secret
* value.
*
*
* If the secret is in a different Amazon Web Services account from the credentials calling the API, then you can't
* use aws/secretsmanager
to encrypt the secret, and you must create and use a customer managed KMS
* key.
*
*
* @param kmsKeyId
* The ARN, key ID, or alias of the KMS key that Secrets Manager uses to encrypt the secret value in the
* secret. An alias is always prefixed by alias/
, for example
* alias/aws/secretsmanager
. For more information, see About aliases.
*
* To use a KMS key in a different account, use the key ARN or the alias ARN.
*
*
* If you don't specify this value, then Secrets Manager uses the key aws/secretsmanager
. If
* that key doesn't yet exist, then Secrets Manager creates it for you automatically the first time it
* encrypts the secret value.
*
*
* If the secret is in a different Amazon Web Services account from the credentials calling the API, then you
* can't use aws/secretsmanager
to encrypt the secret, and you must create and use a customer
* managed KMS key.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The ARN, key ID, or alias of the KMS key that Secrets Manager uses to encrypt the secret value in the secret. An
* alias is always prefixed by alias/
, for example alias/aws/secretsmanager
. For more
* information, see About
* aliases.
*
*
* To use a KMS key in a different account, use the key ARN or the alias ARN.
*
*
* If you don't specify this value, then Secrets Manager uses the key aws/secretsmanager
. If that key
* doesn't yet exist, then Secrets Manager creates it for you automatically the first time it encrypts the secret
* value.
*
*
* If the secret is in a different Amazon Web Services account from the credentials calling the API, then you can't
* use aws/secretsmanager
to encrypt the secret, and you must create and use a customer managed KMS
* key.
*
*
* @return The ARN, key ID, or alias of the KMS key that Secrets Manager uses to encrypt the secret value in the
* secret. An alias is always prefixed by alias/
, for example
* alias/aws/secretsmanager
. For more information, see About aliases.
*
* To use a KMS key in a different account, use the key ARN or the alias ARN.
*
*
* If you don't specify this value, then Secrets Manager uses the key aws/secretsmanager
. If
* that key doesn't yet exist, then Secrets Manager creates it for you automatically the first time it
* encrypts the secret value.
*
*
* If the secret is in a different Amazon Web Services account from the credentials calling the API, then
* you can't use aws/secretsmanager
to encrypt the secret, and you must create and use a
* customer managed KMS key.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The ARN, key ID, or alias of the KMS key that Secrets Manager uses to encrypt the secret value in the secret. An
* alias is always prefixed by alias/
, for example alias/aws/secretsmanager
. For more
* information, see About
* aliases.
*
*
* To use a KMS key in a different account, use the key ARN or the alias ARN.
*
*
* If you don't specify this value, then Secrets Manager uses the key aws/secretsmanager
. If that key
* doesn't yet exist, then Secrets Manager creates it for you automatically the first time it encrypts the secret
* value.
*
*
* If the secret is in a different Amazon Web Services account from the credentials calling the API, then you can't
* use aws/secretsmanager
to encrypt the secret, and you must create and use a customer managed KMS
* key.
*
*
* @param kmsKeyId
* The ARN, key ID, or alias of the KMS key that Secrets Manager uses to encrypt the secret value in the
* secret. An alias is always prefixed by alias/
, for example
* alias/aws/secretsmanager
. For more information, see About aliases.
*
* To use a KMS key in a different account, use the key ARN or the alias ARN.
*
*
* If you don't specify this value, then Secrets Manager uses the key aws/secretsmanager
. If
* that key doesn't yet exist, then Secrets Manager creates it for you automatically the first time it
* encrypts the secret value.
*
*
* If the secret is in a different Amazon Web Services account from the credentials calling the API, then you
* can't use aws/secretsmanager
to encrypt the secret, and you must create and use a customer
* managed KMS key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* The binary data to encrypt and store in the new version of the secret. We recommend that you store your binary
* data in a file and then pass the contents of the file as a parameter.
*
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* This parameter is not available in the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param secretBinary
* The binary data to encrypt and store in the new version of the secret. We recommend that you store your
* binary data in a file and then pass the contents of the file as a parameter.
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* This parameter is not available in the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*/
public void setSecretBinary(java.nio.ByteBuffer secretBinary) {
this.secretBinary = secretBinary;
}
/**
*
* The binary data to encrypt and store in the new version of the secret. We recommend that you store your binary
* data in a file and then pass the contents of the file as a parameter.
*
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* This parameter is not available in the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* {@code ByteBuffer}s are stateful. Calling their {@code get} methods changes their {@code position}. We recommend
* using {@link java.nio.ByteBuffer#asReadOnlyBuffer()} to create a read-only view of the buffer with an independent
* {@code position}, and calling {@code get} methods on this rather than directly on the returned {@code ByteBuffer}.
* Doing so will ensure that anyone else using the {@code ByteBuffer} will not be affected by changes to the
* {@code position}.
*
*
* @return The binary data to encrypt and store in the new version of the secret. We recommend that you store your
* binary data in a file and then pass the contents of the file as a parameter.
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* This parameter is not available in the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail
* log entries. If you create your own log entries, you must also avoid logging the information in this
* field.
*/
public java.nio.ByteBuffer getSecretBinary() {
return this.secretBinary;
}
/**
*
* The binary data to encrypt and store in the new version of the secret. We recommend that you store your binary
* data in a file and then pass the contents of the file as a parameter.
*
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* This parameter is not available in the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param secretBinary
* The binary data to encrypt and store in the new version of the secret. We recommend that you store your
* binary data in a file and then pass the contents of the file as a parameter.
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* This parameter is not available in the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withSecretBinary(java.nio.ByteBuffer secretBinary) {
setSecretBinary(secretBinary);
return this;
}
/**
*
* The text data to encrypt and store in this new version of the secret. We recommend you use a JSON structure of
* key/value pairs for your secret value.
*
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* If you create a secret by using the Secrets Manager console then Secrets Manager puts the protected secret text
* in only the SecretString
parameter. The Secrets Manager console stores the information as a JSON
* structure of key/value pairs that a Lambda rotation function can parse.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @param secretString
* The text data to encrypt and store in this new version of the secret. We recommend you use a JSON
* structure of key/value pairs for your secret value.
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* If you create a secret by using the Secrets Manager console then Secrets Manager puts the protected secret
* text in only the SecretString
parameter. The Secrets Manager console stores the information
* as a JSON structure of key/value pairs that a Lambda rotation function can parse.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*/
public void setSecretString(String secretString) {
this.secretString = secretString;
}
/**
*
* The text data to encrypt and store in this new version of the secret. We recommend you use a JSON structure of
* key/value pairs for your secret value.
*
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* If you create a secret by using the Secrets Manager console then Secrets Manager puts the protected secret text
* in only the SecretString
parameter. The Secrets Manager console stores the information as a JSON
* structure of key/value pairs that a Lambda rotation function can parse.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @return The text data to encrypt and store in this new version of the secret. We recommend you use a JSON
* structure of key/value pairs for your secret value.
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* If you create a secret by using the Secrets Manager console then Secrets Manager puts the protected
* secret text in only the SecretString
parameter. The Secrets Manager console stores the
* information as a JSON structure of key/value pairs that a Lambda rotation function can parse.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail
* log entries. If you create your own log entries, you must also avoid logging the information in this
* field.
*/
public String getSecretString() {
return this.secretString;
}
/**
*
* The text data to encrypt and store in this new version of the secret. We recommend you use a JSON structure of
* key/value pairs for your secret value.
*
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* If you create a secret by using the Secrets Manager console then Secrets Manager puts the protected secret text
* in only the SecretString
parameter. The Secrets Manager console stores the information as a JSON
* structure of key/value pairs that a Lambda rotation function can parse.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @param secretString
* The text data to encrypt and store in this new version of the secret. We recommend you use a JSON
* structure of key/value pairs for your secret value.
*
* Either SecretString
or SecretBinary
must have a value, but not both.
*
*
* If you create a secret by using the Secrets Manager console then Secrets Manager puts the protected secret
* text in only the SecretString
parameter. The Secrets Manager console stores the information
* as a JSON structure of key/value pairs that a Lambda rotation function can parse.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withSecretString(String secretString) {
setSecretString(secretString);
return this;
}
/**
*
* A list of tags to attach to the secret. Each tag is a key and value pair of strings in a JSON text string, for
* example:
*
*
* [{"Key":"CostCenter","Value":"12345"},{"Key":"environment","Value":"production"}]
*
*
* Secrets Manager tag key names are case sensitive. A tag with the key "ABC" is a different tag from one with key
* "abc".
*
*
* If you check tags in permissions policies as part of your security strategy, then adding or removing a tag can
* change permissions. If the completion of this operation would result in you losing your permissions for this
* secret, then Secrets Manager blocks the operation and returns an Access Denied
error. For more
* information, see Control access to secrets using tags and Limit access to identities with tags that match secrets' tags.
*
*
* For information about how to format a JSON parameter for the various command line tool environments, see Using JSON for
* Parameters. If your command-line tool or SDK requires quotation marks around the parameter, you should use
* single quotes to avoid confusion with the double quotes required in the JSON text.
*
*
* For tag quotas and naming restrictions, see Service quotas for
* Tagging in the Amazon Web Services General Reference guide.
*
*
* @return A list of tags to attach to the secret. Each tag is a key and value pair of strings in a JSON text
* string, for example:
*
* [{"Key":"CostCenter","Value":"12345"},{"Key":"environment","Value":"production"}]
*
*
* Secrets Manager tag key names are case sensitive. A tag with the key "ABC" is a different tag from one
* with key "abc".
*
*
* If you check tags in permissions policies as part of your security strategy, then adding or removing a
* tag can change permissions. If the completion of this operation would result in you losing your
* permissions for this secret, then Secrets Manager blocks the operation and returns an
* Access Denied
error. For more information, see Control access to secrets using tags and Limit access to identities with tags that match secrets' tags.
*
*
* For information about how to format a JSON parameter for the various command line tool environments, see
* Using
* JSON for Parameters. If your command-line tool or SDK requires quotation marks around the parameter,
* you should use single quotes to avoid confusion with the double quotes required in the JSON text.
*
*
* For tag quotas and naming restrictions, see Service quotas for
* Tagging in the Amazon Web Services General Reference guide.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* A list of tags to attach to the secret. Each tag is a key and value pair of strings in a JSON text string, for
* example:
*
*
* [{"Key":"CostCenter","Value":"12345"},{"Key":"environment","Value":"production"}]
*
*
* Secrets Manager tag key names are case sensitive. A tag with the key "ABC" is a different tag from one with key
* "abc".
*
*
* If you check tags in permissions policies as part of your security strategy, then adding or removing a tag can
* change permissions. If the completion of this operation would result in you losing your permissions for this
* secret, then Secrets Manager blocks the operation and returns an Access Denied
error. For more
* information, see Control access to secrets using tags and Limit access to identities with tags that match secrets' tags.
*
*
* For information about how to format a JSON parameter for the various command line tool environments, see Using JSON for
* Parameters. If your command-line tool or SDK requires quotation marks around the parameter, you should use
* single quotes to avoid confusion with the double quotes required in the JSON text.
*
*
* For tag quotas and naming restrictions, see Service quotas for
* Tagging in the Amazon Web Services General Reference guide.
*
*
* @param tags
* A list of tags to attach to the secret. Each tag is a key and value pair of strings in a JSON text string,
* for example:
*
* [{"Key":"CostCenter","Value":"12345"},{"Key":"environment","Value":"production"}]
*
*
* Secrets Manager tag key names are case sensitive. A tag with the key "ABC" is a different tag from one
* with key "abc".
*
*
* If you check tags in permissions policies as part of your security strategy, then adding or removing a tag
* can change permissions. If the completion of this operation would result in you losing your permissions
* for this secret, then Secrets Manager blocks the operation and returns an Access Denied
* error. For more information, see Control access to secrets using tags and Limit access to identities with tags that match secrets' tags.
*
*
* For information about how to format a JSON parameter for the various command line tool environments, see
* Using
* JSON for Parameters. If your command-line tool or SDK requires quotation marks around the parameter,
* you should use single quotes to avoid confusion with the double quotes required in the JSON text.
*
*
* For tag quotas and naming restrictions, see Service quotas for
* Tagging in the Amazon Web Services General Reference guide.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* A list of tags to attach to the secret. Each tag is a key and value pair of strings in a JSON text string, for
* example:
*
*
* [{"Key":"CostCenter","Value":"12345"},{"Key":"environment","Value":"production"}]
*
*
* Secrets Manager tag key names are case sensitive. A tag with the key "ABC" is a different tag from one with key
* "abc".
*
*
* If you check tags in permissions policies as part of your security strategy, then adding or removing a tag can
* change permissions. If the completion of this operation would result in you losing your permissions for this
* secret, then Secrets Manager blocks the operation and returns an Access Denied
error. For more
* information, see Control access to secrets using tags and Limit access to identities with tags that match secrets' tags.
*
*
* For information about how to format a JSON parameter for the various command line tool environments, see Using JSON for
* Parameters. If your command-line tool or SDK requires quotation marks around the parameter, you should use
* single quotes to avoid confusion with the double quotes required in the JSON text.
*
*
* For tag quotas and naming restrictions, see Service quotas for
* Tagging in the Amazon Web Services General Reference guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags to attach to the secret. Each tag is a key and value pair of strings in a JSON text string,
* for example:
*
* [{"Key":"CostCenter","Value":"12345"},{"Key":"environment","Value":"production"}]
*
*
* Secrets Manager tag key names are case sensitive. A tag with the key "ABC" is a different tag from one
* with key "abc".
*
*
* If you check tags in permissions policies as part of your security strategy, then adding or removing a tag
* can change permissions. If the completion of this operation would result in you losing your permissions
* for this secret, then Secrets Manager blocks the operation and returns an Access Denied
* error. For more information, see Control access to secrets using tags and Limit access to identities with tags that match secrets' tags.
*
*
* For information about how to format a JSON parameter for the various command line tool environments, see
* Using
* JSON for Parameters. If your command-line tool or SDK requires quotation marks around the parameter,
* you should use single quotes to avoid confusion with the double quotes required in the JSON text.
*
*
* For tag quotas and naming restrictions, see Service quotas for
* Tagging in the Amazon Web Services General Reference guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags to attach to the secret. Each tag is a key and value pair of strings in a JSON text string, for
* example:
*
*
* [{"Key":"CostCenter","Value":"12345"},{"Key":"environment","Value":"production"}]
*
*
* Secrets Manager tag key names are case sensitive. A tag with the key "ABC" is a different tag from one with key
* "abc".
*
*
* If you check tags in permissions policies as part of your security strategy, then adding or removing a tag can
* change permissions. If the completion of this operation would result in you losing your permissions for this
* secret, then Secrets Manager blocks the operation and returns an Access Denied
error. For more
* information, see Control access to secrets using tags and Limit access to identities with tags that match secrets' tags.
*
*
* For information about how to format a JSON parameter for the various command line tool environments, see Using JSON for
* Parameters. If your command-line tool or SDK requires quotation marks around the parameter, you should use
* single quotes to avoid confusion with the double quotes required in the JSON text.
*
*
* For tag quotas and naming restrictions, see Service quotas for
* Tagging in the Amazon Web Services General Reference guide.
*
*
* @param tags
* A list of tags to attach to the secret. Each tag is a key and value pair of strings in a JSON text string,
* for example:
*
* [{"Key":"CostCenter","Value":"12345"},{"Key":"environment","Value":"production"}]
*
*
* Secrets Manager tag key names are case sensitive. A tag with the key "ABC" is a different tag from one
* with key "abc".
*
*
* If you check tags in permissions policies as part of your security strategy, then adding or removing a tag
* can change permissions. If the completion of this operation would result in you losing your permissions
* for this secret, then Secrets Manager blocks the operation and returns an Access Denied
* error. For more information, see Control access to secrets using tags and Limit access to identities with tags that match secrets' tags.
*
*
* For information about how to format a JSON parameter for the various command line tool environments, see
* Using
* JSON for Parameters. If your command-line tool or SDK requires quotation marks around the parameter,
* you should use single quotes to avoid confusion with the double quotes required in the JSON text.
*
*
* For tag quotas and naming restrictions, see Service quotas for
* Tagging in the Amazon Web Services General Reference guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* A list of Regions and KMS keys to replicate secrets.
*
*
* @return A list of Regions and KMS keys to replicate secrets.
*/
public java.util.List getAddReplicaRegions() {
return addReplicaRegions;
}
/**
*
* A list of Regions and KMS keys to replicate secrets.
*
*
* @param addReplicaRegions
* A list of Regions and KMS keys to replicate secrets.
*/
public void setAddReplicaRegions(java.util.Collection addReplicaRegions) {
if (addReplicaRegions == null) {
this.addReplicaRegions = null;
return;
}
this.addReplicaRegions = new java.util.ArrayList(addReplicaRegions);
}
/**
*
* A list of Regions and KMS keys to replicate secrets.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAddReplicaRegions(java.util.Collection)} or {@link #withAddReplicaRegions(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param addReplicaRegions
* A list of Regions and KMS keys to replicate secrets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withAddReplicaRegions(ReplicaRegionType... addReplicaRegions) {
if (this.addReplicaRegions == null) {
setAddReplicaRegions(new java.util.ArrayList(addReplicaRegions.length));
}
for (ReplicaRegionType ele : addReplicaRegions) {
this.addReplicaRegions.add(ele);
}
return this;
}
/**
*
* A list of Regions and KMS keys to replicate secrets.
*
*
* @param addReplicaRegions
* A list of Regions and KMS keys to replicate secrets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withAddReplicaRegions(java.util.Collection addReplicaRegions) {
setAddReplicaRegions(addReplicaRegions);
return this;
}
/**
*
* Specifies whether to overwrite a secret with the same name in the destination Region. By default, secrets aren't
* overwritten.
*
*
* @param forceOverwriteReplicaSecret
* Specifies whether to overwrite a secret with the same name in the destination Region. By default, secrets
* aren't overwritten.
*/
public void setForceOverwriteReplicaSecret(Boolean forceOverwriteReplicaSecret) {
this.forceOverwriteReplicaSecret = forceOverwriteReplicaSecret;
}
/**
*
* Specifies whether to overwrite a secret with the same name in the destination Region. By default, secrets aren't
* overwritten.
*
*
* @return Specifies whether to overwrite a secret with the same name in the destination Region. By default, secrets
* aren't overwritten.
*/
public Boolean getForceOverwriteReplicaSecret() {
return this.forceOverwriteReplicaSecret;
}
/**
*
* Specifies whether to overwrite a secret with the same name in the destination Region. By default, secrets aren't
* overwritten.
*
*
* @param forceOverwriteReplicaSecret
* Specifies whether to overwrite a secret with the same name in the destination Region. By default, secrets
* aren't overwritten.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateSecretRequest withForceOverwriteReplicaSecret(Boolean forceOverwriteReplicaSecret) {
setForceOverwriteReplicaSecret(forceOverwriteReplicaSecret);
return this;
}
/**
*
* Specifies whether to overwrite a secret with the same name in the destination Region. By default, secrets aren't
* overwritten.
*
*
* @return Specifies whether to overwrite a secret with the same name in the destination Region. By default, secrets
* aren't overwritten.
*/
public Boolean isForceOverwriteReplicaSecret() {
return this.forceOverwriteReplicaSecret;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getSecretBinary() != null)
sb.append("SecretBinary: ").append("***Sensitive Data Redacted***").append(",");
if (getSecretString() != null)
sb.append("SecretString: ").append("***Sensitive Data Redacted***").append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getAddReplicaRegions() != null)
sb.append("AddReplicaRegions: ").append(getAddReplicaRegions()).append(",");
if (getForceOverwriteReplicaSecret() != null)
sb.append("ForceOverwriteReplicaSecret: ").append(getForceOverwriteReplicaSecret());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateSecretRequest == false)
return false;
CreateSecretRequest other = (CreateSecretRequest) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getSecretBinary() == null ^ this.getSecretBinary() == null)
return false;
if (other.getSecretBinary() != null && other.getSecretBinary().equals(this.getSecretBinary()) == false)
return false;
if (other.getSecretString() == null ^ this.getSecretString() == null)
return false;
if (other.getSecretString() != null && other.getSecretString().equals(this.getSecretString()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getAddReplicaRegions() == null ^ this.getAddReplicaRegions() == null)
return false;
if (other.getAddReplicaRegions() != null && other.getAddReplicaRegions().equals(this.getAddReplicaRegions()) == false)
return false;
if (other.getForceOverwriteReplicaSecret() == null ^ this.getForceOverwriteReplicaSecret() == null)
return false;
if (other.getForceOverwriteReplicaSecret() != null && other.getForceOverwriteReplicaSecret().equals(this.getForceOverwriteReplicaSecret()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getSecretBinary() == null) ? 0 : getSecretBinary().hashCode());
hashCode = prime * hashCode + ((getSecretString() == null) ? 0 : getSecretString().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getAddReplicaRegions() == null) ? 0 : getAddReplicaRegions().hashCode());
hashCode = prime * hashCode + ((getForceOverwriteReplicaSecret() == null) ? 0 : getForceOverwriteReplicaSecret().hashCode());
return hashCode;
}
@Override
public CreateSecretRequest clone() {
return (CreateSecretRequest) super.clone();
}
}