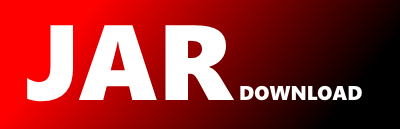
com.amazonaws.services.secretsmanager.model.PutSecretValueRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-secretsmanager Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.secretsmanager.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PutSecretValueRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The ARN or name of the secret to add a new version to.
*
*
* For an ARN, we recommend that you specify a complete ARN rather than a partial ARN. See Finding
* a secret from a partial ARN.
*
*
* If the secret doesn't already exist, use CreateSecret
instead.
*
*/
private String secretId;
/**
*
* A unique identifier for the new version of the secret.
*
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then you
* can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the value for
* this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a UUID-type value to ensure uniqueness of your
* versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a new
* version of the secret is created.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
or
* SecretBinary
values are the same as those in the request then the request is ignored. The operation
* is idempotent.
*
*
* -
*
* If a version with this value already exists and the version of the SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because you
* can't modify a secret version. You can only create new versions to store new secret values.
*
*
*
*
* This value becomes the VersionId
of the new version.
*
*/
private String clientRequestToken;
/**
*
* The binary data to encrypt and store in the new version of the secret. To use this parameter in the command-line
* tools, we recommend that you store your binary data in a file and then pass the contents of the file as a
* parameter.
*
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* You can't access this value from the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*/
private java.nio.ByteBuffer secretBinary;
/**
*
* The text to encrypt and store in the new version of the secret.
*
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* We recommend you create the secret string as JSON key/value pairs, as shown in the example.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*/
private String secretString;
/**
*
* A list of staging labels to attach to this version of the secret. Secrets Manager uses staging labels to track
* versions of a secret through the rotation process.
*
*
* If you specify a staging label that's already associated with a different version of the same secret, then
* Secrets Manager removes the label from the other version and attaches it to this version. If you specify
* AWSCURRENT
, and it is already attached to another version, then Secrets Manager also moves the
* staging label AWSPREVIOUS
to the version that AWSCURRENT
was removed from.
*
*
* If you don't include VersionStages
, then Secrets Manager automatically moves the staging label
* AWSCURRENT
to this version.
*
*/
private java.util.List versionStages;
/**
*
* A unique identifier that indicates the source of the request. For cross-account rotation (when you rotate a
* secret in one account by using a Lambda rotation function in another account) and the Lambda rotation function
* assumes an IAM role to call Secrets Manager, Secrets Manager validates the identity with the rotation token. For
* more information, see How rotation works.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*/
private String rotationToken;
/**
*
* The ARN or name of the secret to add a new version to.
*
*
* For an ARN, we recommend that you specify a complete ARN rather than a partial ARN. See Finding
* a secret from a partial ARN.
*
*
* If the secret doesn't already exist, use CreateSecret
instead.
*
*
* @param secretId
* The ARN or name of the secret to add a new version to.
*
* For an ARN, we recommend that you specify a complete ARN rather than a partial ARN. See Finding a secret from a partial ARN.
*
*
* If the secret doesn't already exist, use CreateSecret
instead.
*/
public void setSecretId(String secretId) {
this.secretId = secretId;
}
/**
*
* The ARN or name of the secret to add a new version to.
*
*
* For an ARN, we recommend that you specify a complete ARN rather than a partial ARN. See Finding
* a secret from a partial ARN.
*
*
* If the secret doesn't already exist, use CreateSecret
instead.
*
*
* @return The ARN or name of the secret to add a new version to.
*
* For an ARN, we recommend that you specify a complete ARN rather than a partial ARN. See Finding a secret from a partial ARN.
*
*
* If the secret doesn't already exist, use CreateSecret
instead.
*/
public String getSecretId() {
return this.secretId;
}
/**
*
* The ARN or name of the secret to add a new version to.
*
*
* For an ARN, we recommend that you specify a complete ARN rather than a partial ARN. See Finding
* a secret from a partial ARN.
*
*
* If the secret doesn't already exist, use CreateSecret
instead.
*
*
* @param secretId
* The ARN or name of the secret to add a new version to.
*
* For an ARN, we recommend that you specify a complete ARN rather than a partial ARN. See Finding a secret from a partial ARN.
*
*
* If the secret doesn't already exist, use CreateSecret
instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutSecretValueRequest withSecretId(String secretId) {
setSecretId(secretId);
return this;
}
/**
*
* A unique identifier for the new version of the secret.
*
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then you
* can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the value for
* this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a UUID-type value to ensure uniqueness of your
* versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a new
* version of the secret is created.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
or
* SecretBinary
values are the same as those in the request then the request is ignored. The operation
* is idempotent.
*
*
* -
*
* If a version with this value already exists and the version of the SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because you
* can't modify a secret version. You can only create new versions to store new secret values.
*
*
*
*
* This value becomes the VersionId
of the new version.
*
*
* @param clientRequestToken
* A unique identifier for the new version of the secret.
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then
* you can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the
* value for this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a
* UUID-type value to ensure
* uniqueness of your versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a
* new version of the secret is created.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
or
* SecretBinary
values are the same as those in the request then the request is ignored. The
* operation is idempotent.
*
*
* -
*
* If a version with this value already exists and the version of the SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because
* you can't modify a secret version. You can only create new versions to store new secret values.
*
*
*
*
* This value becomes the VersionId
of the new version.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* A unique identifier for the new version of the secret.
*
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then you
* can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the value for
* this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a UUID-type value to ensure uniqueness of your
* versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a new
* version of the secret is created.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
or
* SecretBinary
values are the same as those in the request then the request is ignored. The operation
* is idempotent.
*
*
* -
*
* If a version with this value already exists and the version of the SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because you
* can't modify a secret version. You can only create new versions to store new secret values.
*
*
*
*
* This value becomes the VersionId
of the new version.
*
*
* @return A unique identifier for the new version of the secret.
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation,
* then you can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it
* as the value for this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation
* of duplicate versions if there are failures and retries during a rotation. We recommend that you generate
* a UUID-type value to ensure
* uniqueness of your versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a
* new version of the secret is created.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
or
* SecretBinary
values are the same as those in the request then the request is ignored. The
* operation is idempotent.
*
*
* -
*
* If a version with this value already exists and the version of the SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because
* you can't modify a secret version. You can only create new versions to store new secret values.
*
*
*
*
* This value becomes the VersionId
of the new version.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* A unique identifier for the new version of the secret.
*
*
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then you
* can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the value for
* this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a UUID-type value to ensure uniqueness of your
* versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a new
* version of the secret is created.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
or
* SecretBinary
values are the same as those in the request then the request is ignored. The operation
* is idempotent.
*
*
* -
*
* If a version with this value already exists and the version of the SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because you
* can't modify a secret version. You can only create new versions to store new secret values.
*
*
*
*
* This value becomes the VersionId
of the new version.
*
*
* @param clientRequestToken
* A unique identifier for the new version of the secret.
*
* If you use the Amazon Web Services CLI or one of the Amazon Web Services SDKs to call this operation, then
* you can leave this parameter empty. The CLI or SDK generates a random UUID for you and includes it as the
* value for this parameter in the request.
*
*
*
* If you generate a raw HTTP request to the Secrets Manager service endpoint, then you must generate a
* ClientRequestToken
and include it in the request.
*
*
* This value helps ensure idempotency. Secrets Manager uses this value to prevent the accidental creation of
* duplicate versions if there are failures and retries during a rotation. We recommend that you generate a
* UUID-type value to ensure
* uniqueness of your versions within the specified secret.
*
*
* -
*
* If the ClientRequestToken
value isn't already associated with a version of the secret then a
* new version of the secret is created.
*
*
* -
*
* If a version with this value already exists and that version's SecretString
or
* SecretBinary
values are the same as those in the request then the request is ignored. The
* operation is idempotent.
*
*
* -
*
* If a version with this value already exists and the version of the SecretString
and
* SecretBinary
values are different from those in the request, then the request fails because
* you can't modify a secret version. You can only create new versions to store new secret values.
*
*
*
*
* This value becomes the VersionId
of the new version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutSecretValueRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* The binary data to encrypt and store in the new version of the secret. To use this parameter in the command-line
* tools, we recommend that you store your binary data in a file and then pass the contents of the file as a
* parameter.
*
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* You can't access this value from the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param secretBinary
* The binary data to encrypt and store in the new version of the secret. To use this parameter in the
* command-line tools, we recommend that you store your binary data in a file and then pass the contents of
* the file as a parameter.
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* You can't access this value from the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*/
public void setSecretBinary(java.nio.ByteBuffer secretBinary) {
this.secretBinary = secretBinary;
}
/**
*
* The binary data to encrypt and store in the new version of the secret. To use this parameter in the command-line
* tools, we recommend that you store your binary data in a file and then pass the contents of the file as a
* parameter.
*
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* You can't access this value from the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* {@code ByteBuffer}s are stateful. Calling their {@code get} methods changes their {@code position}. We recommend
* using {@link java.nio.ByteBuffer#asReadOnlyBuffer()} to create a read-only view of the buffer with an independent
* {@code position}, and calling {@code get} methods on this rather than directly on the returned {@code ByteBuffer}.
* Doing so will ensure that anyone else using the {@code ByteBuffer} will not be affected by changes to the
* {@code position}.
*
*
* @return The binary data to encrypt and store in the new version of the secret. To use this parameter in the
* command-line tools, we recommend that you store your binary data in a file and then pass the contents of
* the file as a parameter.
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* You can't access this value from the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail
* log entries. If you create your own log entries, you must also avoid logging the information in this
* field.
*/
public java.nio.ByteBuffer getSecretBinary() {
return this.secretBinary;
}
/**
*
* The binary data to encrypt and store in the new version of the secret. To use this parameter in the command-line
* tools, we recommend that you store your binary data in a file and then pass the contents of the file as a
* parameter.
*
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* You can't access this value from the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* The AWS SDK for Java performs a Base64 encoding on this field before sending this request to the AWS service.
* Users of the SDK should not perform Base64 encoding on this field.
*
*
* Warning: ByteBuffers returned by the SDK are mutable. Changes to the content or position of the byte buffer will
* be seen by all objects that have a reference to this object. It is recommended to call ByteBuffer.duplicate() or
* ByteBuffer.asReadOnlyBuffer() before using or reading from the buffer. This behavior will be changed in a future
* major version of the SDK.
*
*
* @param secretBinary
* The binary data to encrypt and store in the new version of the secret. To use this parameter in the
* command-line tools, we recommend that you store your binary data in a file and then pass the contents of
* the file as a parameter.
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* You can't access this value from the Secrets Manager console.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutSecretValueRequest withSecretBinary(java.nio.ByteBuffer secretBinary) {
setSecretBinary(secretBinary);
return this;
}
/**
*
* The text to encrypt and store in the new version of the secret.
*
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* We recommend you create the secret string as JSON key/value pairs, as shown in the example.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @param secretString
* The text to encrypt and store in the new version of the secret.
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* We recommend you create the secret string as JSON key/value pairs, as shown in the example.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*/
public void setSecretString(String secretString) {
this.secretString = secretString;
}
/**
*
* The text to encrypt and store in the new version of the secret.
*
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* We recommend you create the secret string as JSON key/value pairs, as shown in the example.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @return The text to encrypt and store in the new version of the secret.
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* We recommend you create the secret string as JSON key/value pairs, as shown in the example.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail
* log entries. If you create your own log entries, you must also avoid logging the information in this
* field.
*/
public String getSecretString() {
return this.secretString;
}
/**
*
* The text to encrypt and store in the new version of the secret.
*
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* We recommend you create the secret string as JSON key/value pairs, as shown in the example.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @param secretString
* The text to encrypt and store in the new version of the secret.
*
* You must include SecretBinary
or SecretString
, but not both.
*
*
* We recommend you create the secret string as JSON key/value pairs, as shown in the example.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutSecretValueRequest withSecretString(String secretString) {
setSecretString(secretString);
return this;
}
/**
*
* A list of staging labels to attach to this version of the secret. Secrets Manager uses staging labels to track
* versions of a secret through the rotation process.
*
*
* If you specify a staging label that's already associated with a different version of the same secret, then
* Secrets Manager removes the label from the other version and attaches it to this version. If you specify
* AWSCURRENT
, and it is already attached to another version, then Secrets Manager also moves the
* staging label AWSPREVIOUS
to the version that AWSCURRENT
was removed from.
*
*
* If you don't include VersionStages
, then Secrets Manager automatically moves the staging label
* AWSCURRENT
to this version.
*
*
* @return A list of staging labels to attach to this version of the secret. Secrets Manager uses staging labels to
* track versions of a secret through the rotation process.
*
* If you specify a staging label that's already associated with a different version of the same secret,
* then Secrets Manager removes the label from the other version and attaches it to this version. If you
* specify AWSCURRENT
, and it is already attached to another version, then Secrets Manager also
* moves the staging label AWSPREVIOUS
to the version that AWSCURRENT
was removed
* from.
*
*
* If you don't include VersionStages
, then Secrets Manager automatically moves the staging
* label AWSCURRENT
to this version.
*/
public java.util.List getVersionStages() {
return versionStages;
}
/**
*
* A list of staging labels to attach to this version of the secret. Secrets Manager uses staging labels to track
* versions of a secret through the rotation process.
*
*
* If you specify a staging label that's already associated with a different version of the same secret, then
* Secrets Manager removes the label from the other version and attaches it to this version. If you specify
* AWSCURRENT
, and it is already attached to another version, then Secrets Manager also moves the
* staging label AWSPREVIOUS
to the version that AWSCURRENT
was removed from.
*
*
* If you don't include VersionStages
, then Secrets Manager automatically moves the staging label
* AWSCURRENT
to this version.
*
*
* @param versionStages
* A list of staging labels to attach to this version of the secret. Secrets Manager uses staging labels to
* track versions of a secret through the rotation process.
*
* If you specify a staging label that's already associated with a different version of the same secret, then
* Secrets Manager removes the label from the other version and attaches it to this version. If you specify
* AWSCURRENT
, and it is already attached to another version, then Secrets Manager also moves
* the staging label AWSPREVIOUS
to the version that AWSCURRENT
was removed from.
*
*
* If you don't include VersionStages
, then Secrets Manager automatically moves the staging
* label AWSCURRENT
to this version.
*/
public void setVersionStages(java.util.Collection versionStages) {
if (versionStages == null) {
this.versionStages = null;
return;
}
this.versionStages = new java.util.ArrayList(versionStages);
}
/**
*
* A list of staging labels to attach to this version of the secret. Secrets Manager uses staging labels to track
* versions of a secret through the rotation process.
*
*
* If you specify a staging label that's already associated with a different version of the same secret, then
* Secrets Manager removes the label from the other version and attaches it to this version. If you specify
* AWSCURRENT
, and it is already attached to another version, then Secrets Manager also moves the
* staging label AWSPREVIOUS
to the version that AWSCURRENT
was removed from.
*
*
* If you don't include VersionStages
, then Secrets Manager automatically moves the staging label
* AWSCURRENT
to this version.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVersionStages(java.util.Collection)} or {@link #withVersionStages(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param versionStages
* A list of staging labels to attach to this version of the secret. Secrets Manager uses staging labels to
* track versions of a secret through the rotation process.
*
* If you specify a staging label that's already associated with a different version of the same secret, then
* Secrets Manager removes the label from the other version and attaches it to this version. If you specify
* AWSCURRENT
, and it is already attached to another version, then Secrets Manager also moves
* the staging label AWSPREVIOUS
to the version that AWSCURRENT
was removed from.
*
*
* If you don't include VersionStages
, then Secrets Manager automatically moves the staging
* label AWSCURRENT
to this version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutSecretValueRequest withVersionStages(String... versionStages) {
if (this.versionStages == null) {
setVersionStages(new java.util.ArrayList(versionStages.length));
}
for (String ele : versionStages) {
this.versionStages.add(ele);
}
return this;
}
/**
*
* A list of staging labels to attach to this version of the secret. Secrets Manager uses staging labels to track
* versions of a secret through the rotation process.
*
*
* If you specify a staging label that's already associated with a different version of the same secret, then
* Secrets Manager removes the label from the other version and attaches it to this version. If you specify
* AWSCURRENT
, and it is already attached to another version, then Secrets Manager also moves the
* staging label AWSPREVIOUS
to the version that AWSCURRENT
was removed from.
*
*
* If you don't include VersionStages
, then Secrets Manager automatically moves the staging label
* AWSCURRENT
to this version.
*
*
* @param versionStages
* A list of staging labels to attach to this version of the secret. Secrets Manager uses staging labels to
* track versions of a secret through the rotation process.
*
* If you specify a staging label that's already associated with a different version of the same secret, then
* Secrets Manager removes the label from the other version and attaches it to this version. If you specify
* AWSCURRENT
, and it is already attached to another version, then Secrets Manager also moves
* the staging label AWSPREVIOUS
to the version that AWSCURRENT
was removed from.
*
*
* If you don't include VersionStages
, then Secrets Manager automatically moves the staging
* label AWSCURRENT
to this version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutSecretValueRequest withVersionStages(java.util.Collection versionStages) {
setVersionStages(versionStages);
return this;
}
/**
*
* A unique identifier that indicates the source of the request. For cross-account rotation (when you rotate a
* secret in one account by using a Lambda rotation function in another account) and the Lambda rotation function
* assumes an IAM role to call Secrets Manager, Secrets Manager validates the identity with the rotation token. For
* more information, see How rotation works.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @param rotationToken
* A unique identifier that indicates the source of the request. For cross-account rotation (when you rotate
* a secret in one account by using a Lambda rotation function in another account) and the Lambda rotation
* function assumes an IAM role to call Secrets Manager, Secrets Manager validates the identity with the
* rotation token. For more information, see How rotation
* works.
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*/
public void setRotationToken(String rotationToken) {
this.rotationToken = rotationToken;
}
/**
*
* A unique identifier that indicates the source of the request. For cross-account rotation (when you rotate a
* secret in one account by using a Lambda rotation function in another account) and the Lambda rotation function
* assumes an IAM role to call Secrets Manager, Secrets Manager validates the identity with the rotation token. For
* more information, see How rotation works.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @return A unique identifier that indicates the source of the request. For cross-account rotation (when you rotate
* a secret in one account by using a Lambda rotation function in another account) and the Lambda rotation
* function assumes an IAM role to call Secrets Manager, Secrets Manager validates the identity with the
* rotation token. For more information, see How rotation
* works.
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail
* log entries. If you create your own log entries, you must also avoid logging the information in this
* field.
*/
public String getRotationToken() {
return this.rotationToken;
}
/**
*
* A unique identifier that indicates the source of the request. For cross-account rotation (when you rotate a
* secret in one account by using a Lambda rotation function in another account) and the Lambda rotation function
* assumes an IAM role to call Secrets Manager, Secrets Manager validates the identity with the rotation token. For
* more information, see How rotation works.
*
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
*
*
* @param rotationToken
* A unique identifier that indicates the source of the request. For cross-account rotation (when you rotate
* a secret in one account by using a Lambda rotation function in another account) and the Lambda rotation
* function assumes an IAM role to call Secrets Manager, Secrets Manager validates the identity with the
* rotation token. For more information, see How rotation
* works.
*
* Sensitive: This field contains sensitive information, so the service does not include it in CloudTrail log
* entries. If you create your own log entries, you must also avoid logging the information in this field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PutSecretValueRequest withRotationToken(String rotationToken) {
setRotationToken(rotationToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSecretId() != null)
sb.append("SecretId: ").append(getSecretId()).append(",");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken()).append(",");
if (getSecretBinary() != null)
sb.append("SecretBinary: ").append("***Sensitive Data Redacted***").append(",");
if (getSecretString() != null)
sb.append("SecretString: ").append("***Sensitive Data Redacted***").append(",");
if (getVersionStages() != null)
sb.append("VersionStages: ").append(getVersionStages()).append(",");
if (getRotationToken() != null)
sb.append("RotationToken: ").append("***Sensitive Data Redacted***");
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PutSecretValueRequest == false)
return false;
PutSecretValueRequest other = (PutSecretValueRequest) obj;
if (other.getSecretId() == null ^ this.getSecretId() == null)
return false;
if (other.getSecretId() != null && other.getSecretId().equals(this.getSecretId()) == false)
return false;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getSecretBinary() == null ^ this.getSecretBinary() == null)
return false;
if (other.getSecretBinary() != null && other.getSecretBinary().equals(this.getSecretBinary()) == false)
return false;
if (other.getSecretString() == null ^ this.getSecretString() == null)
return false;
if (other.getSecretString() != null && other.getSecretString().equals(this.getSecretString()) == false)
return false;
if (other.getVersionStages() == null ^ this.getVersionStages() == null)
return false;
if (other.getVersionStages() != null && other.getVersionStages().equals(this.getVersionStages()) == false)
return false;
if (other.getRotationToken() == null ^ this.getRotationToken() == null)
return false;
if (other.getRotationToken() != null && other.getRotationToken().equals(this.getRotationToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSecretId() == null) ? 0 : getSecretId().hashCode());
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getSecretBinary() == null) ? 0 : getSecretBinary().hashCode());
hashCode = prime * hashCode + ((getSecretString() == null) ? 0 : getSecretString().hashCode());
hashCode = prime * hashCode + ((getVersionStages() == null) ? 0 : getVersionStages().hashCode());
hashCode = prime * hashCode + ((getRotationToken() == null) ? 0 : getRotationToken().hashCode());
return hashCode;
}
@Override
public PutSecretValueRequest clone() {
return (PutSecretValueRequest) super.clone();
}
}