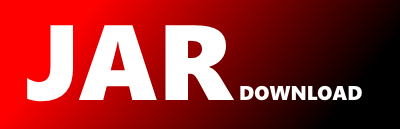
com.amazonaws.services.securityhub.model.AwsRdsDbInstanceDetails Maven / Gradle / Ivy
Show all versions of aws-java-sdk-securityhub Show documentation
/*
* Copyright 2015-2020 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.securityhub.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains the details of an Amazon RDS DB instance.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AwsRdsDbInstanceDetails implements Serializable, Cloneable, StructuredPojo {
/**
*
* The AWS Identity and Access Management (IAM) roles associated with the DB instance.
*
*/
private java.util.List associatedRoles;
/**
*
* The identifier of the CA certificate for this DB instance.
*
*/
private String cACertificateIdentifier;
/**
*
* If the DB instance is a member of a DB cluster, contains the name of the DB cluster that the DB instance is a
* member of.
*
*/
private String dBClusterIdentifier;
/**
*
* Contains a user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*
*/
private String dBInstanceIdentifier;
/**
*
* Contains the name of the compute and memory capacity class of the DB instance.
*
*/
private String dBInstanceClass;
/**
*
* Specifies the port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a
* different port than the DB cluster port.
*
*/
private Integer dbInstancePort;
/**
*
* The AWS Region-unique, immutable identifier for the DB instance. This identifier is found in AWS CloudTrail log
* entries whenever the AWS KMS key for the DB instance is accessed.
*
*/
private String dbiResourceId;
/**
*
* The meaning of this parameter differs according to the database engine you use.
*
*
* MySQL, MariaDB, SQL Server, PostgreSQL
*
*
* Contains the name of the initial database of this instance that was provided at create time, if one was specified
* when the DB instance was created. This same name is returned for the life of the DB instance.
*
*
* Oracle
*
*
* Contains the Oracle System ID (SID) of the created DB instance. Not shown when the returned parameters do not
* apply to an Oracle DB instance.
*
*/
private String dBName;
/**
*
* Indicates whether the DB instance has deletion protection enabled.
*
*
* When deletion protection is enabled, the database cannot be deleted.
*
*/
private Boolean deletionProtection;
/**
*
* Specifies the connection endpoint.
*
*/
private AwsRdsDbInstanceEndpoint endpoint;
/**
*
* Provides the name of the database engine to use for this DB instance.
*
*/
private String engine;
/**
*
* Indicates the database engine version.
*
*/
private String engineVersion;
/**
*
* True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
*
* IAM database authentication can be enabled for the following database engines.
*
*
* -
*
* For MySQL 5.6, minor version 5.6.34 or higher
*
*
* -
*
* For MySQL 5.7, minor version 5.7.16 or higher
*
*
* -
*
* Aurora 5.6 or higher
*
*
*
*/
private Boolean iAMDatabaseAuthenticationEnabled;
/**
*
* Provides the date and time the DB instance was created.
*
*/
private String instanceCreateTime;
/**
*
* If StorageEncrypted
is true, the AWS KMS key identifier for the encrypted DB instance.
*
*/
private String kmsKeyId;
/**
*
* Specifies the accessibility options for the DB instance.
*
*
* A value of true specifies an Internet-facing instance with a publicly resolvable DNS name, which resolves to a
* public IP address.
*
*
* A value of false specifies an internal instance with a DNS name that resolves to a private IP address.
*
*/
private Boolean publiclyAccessible;
/**
*
* Specifies whether the DB instance is encrypted.
*
*/
private Boolean storageEncrypted;
/**
*
* The ARN from the key store with which the instance is associated for TDE encryption.
*
*/
private String tdeCredentialArn;
/**
*
* A list of VPC security groups that the DB instance belongs to.
*
*/
private java.util.List vpcSecurityGroups;
/**
*
* The AWS Identity and Access Management (IAM) roles associated with the DB instance.
*
*
* @return The AWS Identity and Access Management (IAM) roles associated with the DB instance.
*/
public java.util.List getAssociatedRoles() {
return associatedRoles;
}
/**
*
* The AWS Identity and Access Management (IAM) roles associated with the DB instance.
*
*
* @param associatedRoles
* The AWS Identity and Access Management (IAM) roles associated with the DB instance.
*/
public void setAssociatedRoles(java.util.Collection associatedRoles) {
if (associatedRoles == null) {
this.associatedRoles = null;
return;
}
this.associatedRoles = new java.util.ArrayList(associatedRoles);
}
/**
*
* The AWS Identity and Access Management (IAM) roles associated with the DB instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAssociatedRoles(java.util.Collection)} or {@link #withAssociatedRoles(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param associatedRoles
* The AWS Identity and Access Management (IAM) roles associated with the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withAssociatedRoles(AwsRdsDbInstanceAssociatedRole... associatedRoles) {
if (this.associatedRoles == null) {
setAssociatedRoles(new java.util.ArrayList(associatedRoles.length));
}
for (AwsRdsDbInstanceAssociatedRole ele : associatedRoles) {
this.associatedRoles.add(ele);
}
return this;
}
/**
*
* The AWS Identity and Access Management (IAM) roles associated with the DB instance.
*
*
* @param associatedRoles
* The AWS Identity and Access Management (IAM) roles associated with the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withAssociatedRoles(java.util.Collection associatedRoles) {
setAssociatedRoles(associatedRoles);
return this;
}
/**
*
* The identifier of the CA certificate for this DB instance.
*
*
* @param cACertificateIdentifier
* The identifier of the CA certificate for this DB instance.
*/
public void setCACertificateIdentifier(String cACertificateIdentifier) {
this.cACertificateIdentifier = cACertificateIdentifier;
}
/**
*
* The identifier of the CA certificate for this DB instance.
*
*
* @return The identifier of the CA certificate for this DB instance.
*/
public String getCACertificateIdentifier() {
return this.cACertificateIdentifier;
}
/**
*
* The identifier of the CA certificate for this DB instance.
*
*
* @param cACertificateIdentifier
* The identifier of the CA certificate for this DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withCACertificateIdentifier(String cACertificateIdentifier) {
setCACertificateIdentifier(cACertificateIdentifier);
return this;
}
/**
*
* If the DB instance is a member of a DB cluster, contains the name of the DB cluster that the DB instance is a
* member of.
*
*
* @param dBClusterIdentifier
* If the DB instance is a member of a DB cluster, contains the name of the DB cluster that the DB instance
* is a member of.
*/
public void setDBClusterIdentifier(String dBClusterIdentifier) {
this.dBClusterIdentifier = dBClusterIdentifier;
}
/**
*
* If the DB instance is a member of a DB cluster, contains the name of the DB cluster that the DB instance is a
* member of.
*
*
* @return If the DB instance is a member of a DB cluster, contains the name of the DB cluster that the DB instance
* is a member of.
*/
public String getDBClusterIdentifier() {
return this.dBClusterIdentifier;
}
/**
*
* If the DB instance is a member of a DB cluster, contains the name of the DB cluster that the DB instance is a
* member of.
*
*
* @param dBClusterIdentifier
* If the DB instance is a member of a DB cluster, contains the name of the DB cluster that the DB instance
* is a member of.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withDBClusterIdentifier(String dBClusterIdentifier) {
setDBClusterIdentifier(dBClusterIdentifier);
return this;
}
/**
*
* Contains a user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*
*
* @param dBInstanceIdentifier
* Contains a user-supplied database identifier. This identifier is the unique key that identifies a DB
* instance.
*/
public void setDBInstanceIdentifier(String dBInstanceIdentifier) {
this.dBInstanceIdentifier = dBInstanceIdentifier;
}
/**
*
* Contains a user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*
*
* @return Contains a user-supplied database identifier. This identifier is the unique key that identifies a DB
* instance.
*/
public String getDBInstanceIdentifier() {
return this.dBInstanceIdentifier;
}
/**
*
* Contains a user-supplied database identifier. This identifier is the unique key that identifies a DB instance.
*
*
* @param dBInstanceIdentifier
* Contains a user-supplied database identifier. This identifier is the unique key that identifies a DB
* instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withDBInstanceIdentifier(String dBInstanceIdentifier) {
setDBInstanceIdentifier(dBInstanceIdentifier);
return this;
}
/**
*
* Contains the name of the compute and memory capacity class of the DB instance.
*
*
* @param dBInstanceClass
* Contains the name of the compute and memory capacity class of the DB instance.
*/
public void setDBInstanceClass(String dBInstanceClass) {
this.dBInstanceClass = dBInstanceClass;
}
/**
*
* Contains the name of the compute and memory capacity class of the DB instance.
*
*
* @return Contains the name of the compute and memory capacity class of the DB instance.
*/
public String getDBInstanceClass() {
return this.dBInstanceClass;
}
/**
*
* Contains the name of the compute and memory capacity class of the DB instance.
*
*
* @param dBInstanceClass
* Contains the name of the compute and memory capacity class of the DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withDBInstanceClass(String dBInstanceClass) {
setDBInstanceClass(dBInstanceClass);
return this;
}
/**
*
* Specifies the port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a
* different port than the DB cluster port.
*
*
* @param dbInstancePort
* Specifies the port that the DB instance listens on. If the DB instance is part of a DB cluster, this can
* be a different port than the DB cluster port.
*/
public void setDbInstancePort(Integer dbInstancePort) {
this.dbInstancePort = dbInstancePort;
}
/**
*
* Specifies the port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a
* different port than the DB cluster port.
*
*
* @return Specifies the port that the DB instance listens on. If the DB instance is part of a DB cluster, this can
* be a different port than the DB cluster port.
*/
public Integer getDbInstancePort() {
return this.dbInstancePort;
}
/**
*
* Specifies the port that the DB instance listens on. If the DB instance is part of a DB cluster, this can be a
* different port than the DB cluster port.
*
*
* @param dbInstancePort
* Specifies the port that the DB instance listens on. If the DB instance is part of a DB cluster, this can
* be a different port than the DB cluster port.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withDbInstancePort(Integer dbInstancePort) {
setDbInstancePort(dbInstancePort);
return this;
}
/**
*
* The AWS Region-unique, immutable identifier for the DB instance. This identifier is found in AWS CloudTrail log
* entries whenever the AWS KMS key for the DB instance is accessed.
*
*
* @param dbiResourceId
* The AWS Region-unique, immutable identifier for the DB instance. This identifier is found in AWS
* CloudTrail log entries whenever the AWS KMS key for the DB instance is accessed.
*/
public void setDbiResourceId(String dbiResourceId) {
this.dbiResourceId = dbiResourceId;
}
/**
*
* The AWS Region-unique, immutable identifier for the DB instance. This identifier is found in AWS CloudTrail log
* entries whenever the AWS KMS key for the DB instance is accessed.
*
*
* @return The AWS Region-unique, immutable identifier for the DB instance. This identifier is found in AWS
* CloudTrail log entries whenever the AWS KMS key for the DB instance is accessed.
*/
public String getDbiResourceId() {
return this.dbiResourceId;
}
/**
*
* The AWS Region-unique, immutable identifier for the DB instance. This identifier is found in AWS CloudTrail log
* entries whenever the AWS KMS key for the DB instance is accessed.
*
*
* @param dbiResourceId
* The AWS Region-unique, immutable identifier for the DB instance. This identifier is found in AWS
* CloudTrail log entries whenever the AWS KMS key for the DB instance is accessed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withDbiResourceId(String dbiResourceId) {
setDbiResourceId(dbiResourceId);
return this;
}
/**
*
* The meaning of this parameter differs according to the database engine you use.
*
*
* MySQL, MariaDB, SQL Server, PostgreSQL
*
*
* Contains the name of the initial database of this instance that was provided at create time, if one was specified
* when the DB instance was created. This same name is returned for the life of the DB instance.
*
*
* Oracle
*
*
* Contains the Oracle System ID (SID) of the created DB instance. Not shown when the returned parameters do not
* apply to an Oracle DB instance.
*
*
* @param dBName
* The meaning of this parameter differs according to the database engine you use.
*
* MySQL, MariaDB, SQL Server, PostgreSQL
*
*
* Contains the name of the initial database of this instance that was provided at create time, if one was
* specified when the DB instance was created. This same name is returned for the life of the DB instance.
*
*
* Oracle
*
*
* Contains the Oracle System ID (SID) of the created DB instance. Not shown when the returned parameters do
* not apply to an Oracle DB instance.
*/
public void setDBName(String dBName) {
this.dBName = dBName;
}
/**
*
* The meaning of this parameter differs according to the database engine you use.
*
*
* MySQL, MariaDB, SQL Server, PostgreSQL
*
*
* Contains the name of the initial database of this instance that was provided at create time, if one was specified
* when the DB instance was created. This same name is returned for the life of the DB instance.
*
*
* Oracle
*
*
* Contains the Oracle System ID (SID) of the created DB instance. Not shown when the returned parameters do not
* apply to an Oracle DB instance.
*
*
* @return The meaning of this parameter differs according to the database engine you use.
*
* MySQL, MariaDB, SQL Server, PostgreSQL
*
*
* Contains the name of the initial database of this instance that was provided at create time, if one was
* specified when the DB instance was created. This same name is returned for the life of the DB instance.
*
*
* Oracle
*
*
* Contains the Oracle System ID (SID) of the created DB instance. Not shown when the returned parameters do
* not apply to an Oracle DB instance.
*/
public String getDBName() {
return this.dBName;
}
/**
*
* The meaning of this parameter differs according to the database engine you use.
*
*
* MySQL, MariaDB, SQL Server, PostgreSQL
*
*
* Contains the name of the initial database of this instance that was provided at create time, if one was specified
* when the DB instance was created. This same name is returned for the life of the DB instance.
*
*
* Oracle
*
*
* Contains the Oracle System ID (SID) of the created DB instance. Not shown when the returned parameters do not
* apply to an Oracle DB instance.
*
*
* @param dBName
* The meaning of this parameter differs according to the database engine you use.
*
* MySQL, MariaDB, SQL Server, PostgreSQL
*
*
* Contains the name of the initial database of this instance that was provided at create time, if one was
* specified when the DB instance was created. This same name is returned for the life of the DB instance.
*
*
* Oracle
*
*
* Contains the Oracle System ID (SID) of the created DB instance. Not shown when the returned parameters do
* not apply to an Oracle DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withDBName(String dBName) {
setDBName(dBName);
return this;
}
/**
*
* Indicates whether the DB instance has deletion protection enabled.
*
*
* When deletion protection is enabled, the database cannot be deleted.
*
*
* @param deletionProtection
* Indicates whether the DB instance has deletion protection enabled.
*
* When deletion protection is enabled, the database cannot be deleted.
*/
public void setDeletionProtection(Boolean deletionProtection) {
this.deletionProtection = deletionProtection;
}
/**
*
* Indicates whether the DB instance has deletion protection enabled.
*
*
* When deletion protection is enabled, the database cannot be deleted.
*
*
* @return Indicates whether the DB instance has deletion protection enabled.
*
* When deletion protection is enabled, the database cannot be deleted.
*/
public Boolean getDeletionProtection() {
return this.deletionProtection;
}
/**
*
* Indicates whether the DB instance has deletion protection enabled.
*
*
* When deletion protection is enabled, the database cannot be deleted.
*
*
* @param deletionProtection
* Indicates whether the DB instance has deletion protection enabled.
*
* When deletion protection is enabled, the database cannot be deleted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withDeletionProtection(Boolean deletionProtection) {
setDeletionProtection(deletionProtection);
return this;
}
/**
*
* Indicates whether the DB instance has deletion protection enabled.
*
*
* When deletion protection is enabled, the database cannot be deleted.
*
*
* @return Indicates whether the DB instance has deletion protection enabled.
*
* When deletion protection is enabled, the database cannot be deleted.
*/
public Boolean isDeletionProtection() {
return this.deletionProtection;
}
/**
*
* Specifies the connection endpoint.
*
*
* @param endpoint
* Specifies the connection endpoint.
*/
public void setEndpoint(AwsRdsDbInstanceEndpoint endpoint) {
this.endpoint = endpoint;
}
/**
*
* Specifies the connection endpoint.
*
*
* @return Specifies the connection endpoint.
*/
public AwsRdsDbInstanceEndpoint getEndpoint() {
return this.endpoint;
}
/**
*
* Specifies the connection endpoint.
*
*
* @param endpoint
* Specifies the connection endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withEndpoint(AwsRdsDbInstanceEndpoint endpoint) {
setEndpoint(endpoint);
return this;
}
/**
*
* Provides the name of the database engine to use for this DB instance.
*
*
* @param engine
* Provides the name of the database engine to use for this DB instance.
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
*
* Provides the name of the database engine to use for this DB instance.
*
*
* @return Provides the name of the database engine to use for this DB instance.
*/
public String getEngine() {
return this.engine;
}
/**
*
* Provides the name of the database engine to use for this DB instance.
*
*
* @param engine
* Provides the name of the database engine to use for this DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withEngine(String engine) {
setEngine(engine);
return this;
}
/**
*
* Indicates the database engine version.
*
*
* @param engineVersion
* Indicates the database engine version.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* Indicates the database engine version.
*
*
* @return Indicates the database engine version.
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* Indicates the database engine version.
*
*
* @param engineVersion
* Indicates the database engine version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
*
* IAM database authentication can be enabled for the following database engines.
*
*
* -
*
* For MySQL 5.6, minor version 5.6.34 or higher
*
*
* -
*
* For MySQL 5.7, minor version 5.7.16 or higher
*
*
* -
*
* Aurora 5.6 or higher
*
*
*
*
* @param iAMDatabaseAuthenticationEnabled
* True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
* IAM database authentication can be enabled for the following database engines.
*
*
* -
*
* For MySQL 5.6, minor version 5.6.34 or higher
*
*
* -
*
* For MySQL 5.7, minor version 5.7.16 or higher
*
*
* -
*
* Aurora 5.6 or higher
*
*
*/
public void setIAMDatabaseAuthenticationEnabled(Boolean iAMDatabaseAuthenticationEnabled) {
this.iAMDatabaseAuthenticationEnabled = iAMDatabaseAuthenticationEnabled;
}
/**
*
* True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
*
* IAM database authentication can be enabled for the following database engines.
*
*
* -
*
* For MySQL 5.6, minor version 5.6.34 or higher
*
*
* -
*
* For MySQL 5.7, minor version 5.7.16 or higher
*
*
* -
*
* Aurora 5.6 or higher
*
*
*
*
* @return True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
* IAM database authentication can be enabled for the following database engines.
*
*
* -
*
* For MySQL 5.6, minor version 5.6.34 or higher
*
*
* -
*
* For MySQL 5.7, minor version 5.7.16 or higher
*
*
* -
*
* Aurora 5.6 or higher
*
*
*/
public Boolean getIAMDatabaseAuthenticationEnabled() {
return this.iAMDatabaseAuthenticationEnabled;
}
/**
*
* True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
*
* IAM database authentication can be enabled for the following database engines.
*
*
* -
*
* For MySQL 5.6, minor version 5.6.34 or higher
*
*
* -
*
* For MySQL 5.7, minor version 5.7.16 or higher
*
*
* -
*
* Aurora 5.6 or higher
*
*
*
*
* @param iAMDatabaseAuthenticationEnabled
* True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
* IAM database authentication can be enabled for the following database engines.
*
*
* -
*
* For MySQL 5.6, minor version 5.6.34 or higher
*
*
* -
*
* For MySQL 5.7, minor version 5.7.16 or higher
*
*
* -
*
* Aurora 5.6 or higher
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withIAMDatabaseAuthenticationEnabled(Boolean iAMDatabaseAuthenticationEnabled) {
setIAMDatabaseAuthenticationEnabled(iAMDatabaseAuthenticationEnabled);
return this;
}
/**
*
* True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
*
* IAM database authentication can be enabled for the following database engines.
*
*
* -
*
* For MySQL 5.6, minor version 5.6.34 or higher
*
*
* -
*
* For MySQL 5.7, minor version 5.7.16 or higher
*
*
* -
*
* Aurora 5.6 or higher
*
*
*
*
* @return True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
* IAM database authentication can be enabled for the following database engines.
*
*
* -
*
* For MySQL 5.6, minor version 5.6.34 or higher
*
*
* -
*
* For MySQL 5.7, minor version 5.7.16 or higher
*
*
* -
*
* Aurora 5.6 or higher
*
*
*/
public Boolean isIAMDatabaseAuthenticationEnabled() {
return this.iAMDatabaseAuthenticationEnabled;
}
/**
*
* Provides the date and time the DB instance was created.
*
*
* @param instanceCreateTime
* Provides the date and time the DB instance was created.
*/
public void setInstanceCreateTime(String instanceCreateTime) {
this.instanceCreateTime = instanceCreateTime;
}
/**
*
* Provides the date and time the DB instance was created.
*
*
* @return Provides the date and time the DB instance was created.
*/
public String getInstanceCreateTime() {
return this.instanceCreateTime;
}
/**
*
* Provides the date and time the DB instance was created.
*
*
* @param instanceCreateTime
* Provides the date and time the DB instance was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withInstanceCreateTime(String instanceCreateTime) {
setInstanceCreateTime(instanceCreateTime);
return this;
}
/**
*
* If StorageEncrypted
is true, the AWS KMS key identifier for the encrypted DB instance.
*
*
* @param kmsKeyId
* If StorageEncrypted
is true, the AWS KMS key identifier for the encrypted DB instance.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* If StorageEncrypted
is true, the AWS KMS key identifier for the encrypted DB instance.
*
*
* @return If StorageEncrypted
is true, the AWS KMS key identifier for the encrypted DB instance.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* If StorageEncrypted
is true, the AWS KMS key identifier for the encrypted DB instance.
*
*
* @param kmsKeyId
* If StorageEncrypted
is true, the AWS KMS key identifier for the encrypted DB instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* Specifies the accessibility options for the DB instance.
*
*
* A value of true specifies an Internet-facing instance with a publicly resolvable DNS name, which resolves to a
* public IP address.
*
*
* A value of false specifies an internal instance with a DNS name that resolves to a private IP address.
*
*
* @param publiclyAccessible
* Specifies the accessibility options for the DB instance.
*
* A value of true specifies an Internet-facing instance with a publicly resolvable DNS name, which resolves
* to a public IP address.
*
*
* A value of false specifies an internal instance with a DNS name that resolves to a private IP address.
*/
public void setPubliclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
}
/**
*
* Specifies the accessibility options for the DB instance.
*
*
* A value of true specifies an Internet-facing instance with a publicly resolvable DNS name, which resolves to a
* public IP address.
*
*
* A value of false specifies an internal instance with a DNS name that resolves to a private IP address.
*
*
* @return Specifies the accessibility options for the DB instance.
*
* A value of true specifies an Internet-facing instance with a publicly resolvable DNS name, which resolves
* to a public IP address.
*
*
* A value of false specifies an internal instance with a DNS name that resolves to a private IP address.
*/
public Boolean getPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* Specifies the accessibility options for the DB instance.
*
*
* A value of true specifies an Internet-facing instance with a publicly resolvable DNS name, which resolves to a
* public IP address.
*
*
* A value of false specifies an internal instance with a DNS name that resolves to a private IP address.
*
*
* @param publiclyAccessible
* Specifies the accessibility options for the DB instance.
*
* A value of true specifies an Internet-facing instance with a publicly resolvable DNS name, which resolves
* to a public IP address.
*
*
* A value of false specifies an internal instance with a DNS name that resolves to a private IP address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withPubliclyAccessible(Boolean publiclyAccessible) {
setPubliclyAccessible(publiclyAccessible);
return this;
}
/**
*
* Specifies the accessibility options for the DB instance.
*
*
* A value of true specifies an Internet-facing instance with a publicly resolvable DNS name, which resolves to a
* public IP address.
*
*
* A value of false specifies an internal instance with a DNS name that resolves to a private IP address.
*
*
* @return Specifies the accessibility options for the DB instance.
*
* A value of true specifies an Internet-facing instance with a publicly resolvable DNS name, which resolves
* to a public IP address.
*
*
* A value of false specifies an internal instance with a DNS name that resolves to a private IP address.
*/
public Boolean isPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* Specifies whether the DB instance is encrypted.
*
*
* @param storageEncrypted
* Specifies whether the DB instance is encrypted.
*/
public void setStorageEncrypted(Boolean storageEncrypted) {
this.storageEncrypted = storageEncrypted;
}
/**
*
* Specifies whether the DB instance is encrypted.
*
*
* @return Specifies whether the DB instance is encrypted.
*/
public Boolean getStorageEncrypted() {
return this.storageEncrypted;
}
/**
*
* Specifies whether the DB instance is encrypted.
*
*
* @param storageEncrypted
* Specifies whether the DB instance is encrypted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withStorageEncrypted(Boolean storageEncrypted) {
setStorageEncrypted(storageEncrypted);
return this;
}
/**
*
* Specifies whether the DB instance is encrypted.
*
*
* @return Specifies whether the DB instance is encrypted.
*/
public Boolean isStorageEncrypted() {
return this.storageEncrypted;
}
/**
*
* The ARN from the key store with which the instance is associated for TDE encryption.
*
*
* @param tdeCredentialArn
* The ARN from the key store with which the instance is associated for TDE encryption.
*/
public void setTdeCredentialArn(String tdeCredentialArn) {
this.tdeCredentialArn = tdeCredentialArn;
}
/**
*
* The ARN from the key store with which the instance is associated for TDE encryption.
*
*
* @return The ARN from the key store with which the instance is associated for TDE encryption.
*/
public String getTdeCredentialArn() {
return this.tdeCredentialArn;
}
/**
*
* The ARN from the key store with which the instance is associated for TDE encryption.
*
*
* @param tdeCredentialArn
* The ARN from the key store with which the instance is associated for TDE encryption.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withTdeCredentialArn(String tdeCredentialArn) {
setTdeCredentialArn(tdeCredentialArn);
return this;
}
/**
*
* A list of VPC security groups that the DB instance belongs to.
*
*
* @return A list of VPC security groups that the DB instance belongs to.
*/
public java.util.List getVpcSecurityGroups() {
return vpcSecurityGroups;
}
/**
*
* A list of VPC security groups that the DB instance belongs to.
*
*
* @param vpcSecurityGroups
* A list of VPC security groups that the DB instance belongs to.
*/
public void setVpcSecurityGroups(java.util.Collection vpcSecurityGroups) {
if (vpcSecurityGroups == null) {
this.vpcSecurityGroups = null;
return;
}
this.vpcSecurityGroups = new java.util.ArrayList(vpcSecurityGroups);
}
/**
*
* A list of VPC security groups that the DB instance belongs to.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVpcSecurityGroups(java.util.Collection)} or {@link #withVpcSecurityGroups(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param vpcSecurityGroups
* A list of VPC security groups that the DB instance belongs to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withVpcSecurityGroups(AwsRdsDbInstanceVpcSecurityGroup... vpcSecurityGroups) {
if (this.vpcSecurityGroups == null) {
setVpcSecurityGroups(new java.util.ArrayList(vpcSecurityGroups.length));
}
for (AwsRdsDbInstanceVpcSecurityGroup ele : vpcSecurityGroups) {
this.vpcSecurityGroups.add(ele);
}
return this;
}
/**
*
* A list of VPC security groups that the DB instance belongs to.
*
*
* @param vpcSecurityGroups
* A list of VPC security groups that the DB instance belongs to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsRdsDbInstanceDetails withVpcSecurityGroups(java.util.Collection vpcSecurityGroups) {
setVpcSecurityGroups(vpcSecurityGroups);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAssociatedRoles() != null)
sb.append("AssociatedRoles: ").append(getAssociatedRoles()).append(",");
if (getCACertificateIdentifier() != null)
sb.append("CACertificateIdentifier: ").append(getCACertificateIdentifier()).append(",");
if (getDBClusterIdentifier() != null)
sb.append("DBClusterIdentifier: ").append(getDBClusterIdentifier()).append(",");
if (getDBInstanceIdentifier() != null)
sb.append("DBInstanceIdentifier: ").append(getDBInstanceIdentifier()).append(",");
if (getDBInstanceClass() != null)
sb.append("DBInstanceClass: ").append(getDBInstanceClass()).append(",");
if (getDbInstancePort() != null)
sb.append("DbInstancePort: ").append(getDbInstancePort()).append(",");
if (getDbiResourceId() != null)
sb.append("DbiResourceId: ").append(getDbiResourceId()).append(",");
if (getDBName() != null)
sb.append("DBName: ").append(getDBName()).append(",");
if (getDeletionProtection() != null)
sb.append("DeletionProtection: ").append(getDeletionProtection()).append(",");
if (getEndpoint() != null)
sb.append("Endpoint: ").append(getEndpoint()).append(",");
if (getEngine() != null)
sb.append("Engine: ").append(getEngine()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getIAMDatabaseAuthenticationEnabled() != null)
sb.append("IAMDatabaseAuthenticationEnabled: ").append(getIAMDatabaseAuthenticationEnabled()).append(",");
if (getInstanceCreateTime() != null)
sb.append("InstanceCreateTime: ").append(getInstanceCreateTime()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getPubliclyAccessible() != null)
sb.append("PubliclyAccessible: ").append(getPubliclyAccessible()).append(",");
if (getStorageEncrypted() != null)
sb.append("StorageEncrypted: ").append(getStorageEncrypted()).append(",");
if (getTdeCredentialArn() != null)
sb.append("TdeCredentialArn: ").append(getTdeCredentialArn()).append(",");
if (getVpcSecurityGroups() != null)
sb.append("VpcSecurityGroups: ").append(getVpcSecurityGroups());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AwsRdsDbInstanceDetails == false)
return false;
AwsRdsDbInstanceDetails other = (AwsRdsDbInstanceDetails) obj;
if (other.getAssociatedRoles() == null ^ this.getAssociatedRoles() == null)
return false;
if (other.getAssociatedRoles() != null && other.getAssociatedRoles().equals(this.getAssociatedRoles()) == false)
return false;
if (other.getCACertificateIdentifier() == null ^ this.getCACertificateIdentifier() == null)
return false;
if (other.getCACertificateIdentifier() != null && other.getCACertificateIdentifier().equals(this.getCACertificateIdentifier()) == false)
return false;
if (other.getDBClusterIdentifier() == null ^ this.getDBClusterIdentifier() == null)
return false;
if (other.getDBClusterIdentifier() != null && other.getDBClusterIdentifier().equals(this.getDBClusterIdentifier()) == false)
return false;
if (other.getDBInstanceIdentifier() == null ^ this.getDBInstanceIdentifier() == null)
return false;
if (other.getDBInstanceIdentifier() != null && other.getDBInstanceIdentifier().equals(this.getDBInstanceIdentifier()) == false)
return false;
if (other.getDBInstanceClass() == null ^ this.getDBInstanceClass() == null)
return false;
if (other.getDBInstanceClass() != null && other.getDBInstanceClass().equals(this.getDBInstanceClass()) == false)
return false;
if (other.getDbInstancePort() == null ^ this.getDbInstancePort() == null)
return false;
if (other.getDbInstancePort() != null && other.getDbInstancePort().equals(this.getDbInstancePort()) == false)
return false;
if (other.getDbiResourceId() == null ^ this.getDbiResourceId() == null)
return false;
if (other.getDbiResourceId() != null && other.getDbiResourceId().equals(this.getDbiResourceId()) == false)
return false;
if (other.getDBName() == null ^ this.getDBName() == null)
return false;
if (other.getDBName() != null && other.getDBName().equals(this.getDBName()) == false)
return false;
if (other.getDeletionProtection() == null ^ this.getDeletionProtection() == null)
return false;
if (other.getDeletionProtection() != null && other.getDeletionProtection().equals(this.getDeletionProtection()) == false)
return false;
if (other.getEndpoint() == null ^ this.getEndpoint() == null)
return false;
if (other.getEndpoint() != null && other.getEndpoint().equals(this.getEndpoint()) == false)
return false;
if (other.getEngine() == null ^ this.getEngine() == null)
return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getIAMDatabaseAuthenticationEnabled() == null ^ this.getIAMDatabaseAuthenticationEnabled() == null)
return false;
if (other.getIAMDatabaseAuthenticationEnabled() != null
&& other.getIAMDatabaseAuthenticationEnabled().equals(this.getIAMDatabaseAuthenticationEnabled()) == false)
return false;
if (other.getInstanceCreateTime() == null ^ this.getInstanceCreateTime() == null)
return false;
if (other.getInstanceCreateTime() != null && other.getInstanceCreateTime().equals(this.getInstanceCreateTime()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getPubliclyAccessible() == null ^ this.getPubliclyAccessible() == null)
return false;
if (other.getPubliclyAccessible() != null && other.getPubliclyAccessible().equals(this.getPubliclyAccessible()) == false)
return false;
if (other.getStorageEncrypted() == null ^ this.getStorageEncrypted() == null)
return false;
if (other.getStorageEncrypted() != null && other.getStorageEncrypted().equals(this.getStorageEncrypted()) == false)
return false;
if (other.getTdeCredentialArn() == null ^ this.getTdeCredentialArn() == null)
return false;
if (other.getTdeCredentialArn() != null && other.getTdeCredentialArn().equals(this.getTdeCredentialArn()) == false)
return false;
if (other.getVpcSecurityGroups() == null ^ this.getVpcSecurityGroups() == null)
return false;
if (other.getVpcSecurityGroups() != null && other.getVpcSecurityGroups().equals(this.getVpcSecurityGroups()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAssociatedRoles() == null) ? 0 : getAssociatedRoles().hashCode());
hashCode = prime * hashCode + ((getCACertificateIdentifier() == null) ? 0 : getCACertificateIdentifier().hashCode());
hashCode = prime * hashCode + ((getDBClusterIdentifier() == null) ? 0 : getDBClusterIdentifier().hashCode());
hashCode = prime * hashCode + ((getDBInstanceIdentifier() == null) ? 0 : getDBInstanceIdentifier().hashCode());
hashCode = prime * hashCode + ((getDBInstanceClass() == null) ? 0 : getDBInstanceClass().hashCode());
hashCode = prime * hashCode + ((getDbInstancePort() == null) ? 0 : getDbInstancePort().hashCode());
hashCode = prime * hashCode + ((getDbiResourceId() == null) ? 0 : getDbiResourceId().hashCode());
hashCode = prime * hashCode + ((getDBName() == null) ? 0 : getDBName().hashCode());
hashCode = prime * hashCode + ((getDeletionProtection() == null) ? 0 : getDeletionProtection().hashCode());
hashCode = prime * hashCode + ((getEndpoint() == null) ? 0 : getEndpoint().hashCode());
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getIAMDatabaseAuthenticationEnabled() == null) ? 0 : getIAMDatabaseAuthenticationEnabled().hashCode());
hashCode = prime * hashCode + ((getInstanceCreateTime() == null) ? 0 : getInstanceCreateTime().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getPubliclyAccessible() == null) ? 0 : getPubliclyAccessible().hashCode());
hashCode = prime * hashCode + ((getStorageEncrypted() == null) ? 0 : getStorageEncrypted().hashCode());
hashCode = prime * hashCode + ((getTdeCredentialArn() == null) ? 0 : getTdeCredentialArn().hashCode());
hashCode = prime * hashCode + ((getVpcSecurityGroups() == null) ? 0 : getVpcSecurityGroups().hashCode());
return hashCode;
}
@Override
public AwsRdsDbInstanceDetails clone() {
try {
return (AwsRdsDbInstanceDetails) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.securityhub.model.transform.AwsRdsDbInstanceDetailsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}