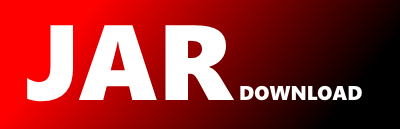
com.amazonaws.services.securityhub.model.AwsEcsServiceDetails Maven / Gradle / Ivy
Show all versions of aws-java-sdk-securityhub Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.securityhub.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides details about a service within an ECS cluster.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AwsEcsServiceDetails implements Serializable, Cloneable, StructuredPojo {
/**
*
* The capacity provider strategy that the service uses.
*
*/
private java.util.List capacityProviderStrategy;
/**
*
* The ARN of the cluster that hosts the service.
*
*/
private String cluster;
/**
*
* Deployment parameters for the service. Includes the number of tasks that run and the order in which to start and
* stop tasks.
*
*/
private AwsEcsServiceDeploymentConfigurationDetails deploymentConfiguration;
/**
*
* Contains the deployment controller type that the service uses.
*
*/
private AwsEcsServiceDeploymentControllerDetails deploymentController;
/**
*
* The number of instantiations of the task definition to run on the service.
*
*/
private Integer desiredCount;
/**
*
* Whether to enable Amazon ECS managed tags for the tasks in the service.
*
*/
private Boolean enableEcsManagedTags;
/**
*
* Whether the execute command functionality is enabled for the service.
*
*/
private Boolean enableExecuteCommand;
/**
*
* After a task starts, the amount of time in seconds that the Amazon ECS service scheduler ignores unhealthy
* Elastic Load Balancing target health checks.
*
*/
private Integer healthCheckGracePeriodSeconds;
/**
*
* The launch type that the service uses.
*
*
* Valid values: EC2
| FARGATE
| EXTERNAL
*
*/
private String launchType;
/**
*
* Information about the load balancers that the service uses.
*
*/
private java.util.List loadBalancers;
/**
*
* The name of the service.
*
*/
private String name;
/**
*
* For tasks that use the awsvpc
networking mode, the VPC subnet and security group configuration.
*
*/
private AwsEcsServiceNetworkConfigurationDetails networkConfiguration;
/**
*
* The placement constraints for the tasks in the service.
*
*/
private java.util.List placementConstraints;
/**
*
* Information about how tasks for the service are placed.
*
*/
private java.util.List placementStrategies;
/**
*
* The platform version on which to run the service. Only specified for tasks that are hosted on Fargate. If a
* platform version is not specified, the LATEST
platform version is used by default.
*
*/
private String platformVersion;
/**
*
* Indicates whether to propagate the tags from the task definition to the task or from the service to the task. If
* no value is provided, then tags are not propagated.
*
*
* Valid values: TASK_DEFINITION
| SERVICE
*
*/
private String propagateTags;
/**
*
* The ARN of the IAM role that is associated with the service. The role allows the Amazon ECS container agent to
* register container instances with an Elastic Load Balancing load balancer.
*
*/
private String role;
/**
*
* The scheduling strategy to use for the service.
*
*
* The REPLICA
scheduling strategy places and maintains the desired number of tasks across the cluster.
* By default, the service scheduler spreads tasks across Availability Zones. Task placement strategies and
* constraints are used to customize task placement decisions.
*
*
* The DAEMON
scheduling strategy deploys exactly one task on each active container instance that meets
* all of the task placement constraints that are specified in the cluster. The service scheduler also evaluates the
* task placement constraints for running tasks and stops tasks that do not meet the placement constraints.
*
*
* Valid values: REPLICA
| DAEMON
*
*/
private String schedulingStrategy;
/**
*
* The ARN of the service.
*
*/
private String serviceArn;
/**
*
* The name of the service.
*
*
* The name can contain up to 255 characters. It can use letters, numbers, underscores, and hyphens.
*
*/
private String serviceName;
/**
*
* Information about the service discovery registries to assign to the service.
*
*/
private java.util.List serviceRegistries;
/**
*
* The task definition to use for tasks in the service.
*
*/
private String taskDefinition;
/**
*
* The capacity provider strategy that the service uses.
*
*
* @return The capacity provider strategy that the service uses.
*/
public java.util.List getCapacityProviderStrategy() {
return capacityProviderStrategy;
}
/**
*
* The capacity provider strategy that the service uses.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy that the service uses.
*/
public void setCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
if (capacityProviderStrategy == null) {
this.capacityProviderStrategy = null;
return;
}
this.capacityProviderStrategy = new java.util.ArrayList(capacityProviderStrategy);
}
/**
*
* The capacity provider strategy that the service uses.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCapacityProviderStrategy(java.util.Collection)} or
* {@link #withCapacityProviderStrategy(java.util.Collection)} if you want to override the existing values.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy that the service uses.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withCapacityProviderStrategy(AwsEcsServiceCapacityProviderStrategyDetails... capacityProviderStrategy) {
if (this.capacityProviderStrategy == null) {
setCapacityProviderStrategy(new java.util.ArrayList(capacityProviderStrategy.length));
}
for (AwsEcsServiceCapacityProviderStrategyDetails ele : capacityProviderStrategy) {
this.capacityProviderStrategy.add(ele);
}
return this;
}
/**
*
* The capacity provider strategy that the service uses.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy that the service uses.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
setCapacityProviderStrategy(capacityProviderStrategy);
return this;
}
/**
*
* The ARN of the cluster that hosts the service.
*
*
* @param cluster
* The ARN of the cluster that hosts the service.
*/
public void setCluster(String cluster) {
this.cluster = cluster;
}
/**
*
* The ARN of the cluster that hosts the service.
*
*
* @return The ARN of the cluster that hosts the service.
*/
public String getCluster() {
return this.cluster;
}
/**
*
* The ARN of the cluster that hosts the service.
*
*
* @param cluster
* The ARN of the cluster that hosts the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withCluster(String cluster) {
setCluster(cluster);
return this;
}
/**
*
* Deployment parameters for the service. Includes the number of tasks that run and the order in which to start and
* stop tasks.
*
*
* @param deploymentConfiguration
* Deployment parameters for the service. Includes the number of tasks that run and the order in which to
* start and stop tasks.
*/
public void setDeploymentConfiguration(AwsEcsServiceDeploymentConfigurationDetails deploymentConfiguration) {
this.deploymentConfiguration = deploymentConfiguration;
}
/**
*
* Deployment parameters for the service. Includes the number of tasks that run and the order in which to start and
* stop tasks.
*
*
* @return Deployment parameters for the service. Includes the number of tasks that run and the order in which to
* start and stop tasks.
*/
public AwsEcsServiceDeploymentConfigurationDetails getDeploymentConfiguration() {
return this.deploymentConfiguration;
}
/**
*
* Deployment parameters for the service. Includes the number of tasks that run and the order in which to start and
* stop tasks.
*
*
* @param deploymentConfiguration
* Deployment parameters for the service. Includes the number of tasks that run and the order in which to
* start and stop tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withDeploymentConfiguration(AwsEcsServiceDeploymentConfigurationDetails deploymentConfiguration) {
setDeploymentConfiguration(deploymentConfiguration);
return this;
}
/**
*
* Contains the deployment controller type that the service uses.
*
*
* @param deploymentController
* Contains the deployment controller type that the service uses.
*/
public void setDeploymentController(AwsEcsServiceDeploymentControllerDetails deploymentController) {
this.deploymentController = deploymentController;
}
/**
*
* Contains the deployment controller type that the service uses.
*
*
* @return Contains the deployment controller type that the service uses.
*/
public AwsEcsServiceDeploymentControllerDetails getDeploymentController() {
return this.deploymentController;
}
/**
*
* Contains the deployment controller type that the service uses.
*
*
* @param deploymentController
* Contains the deployment controller type that the service uses.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withDeploymentController(AwsEcsServiceDeploymentControllerDetails deploymentController) {
setDeploymentController(deploymentController);
return this;
}
/**
*
* The number of instantiations of the task definition to run on the service.
*
*
* @param desiredCount
* The number of instantiations of the task definition to run on the service.
*/
public void setDesiredCount(Integer desiredCount) {
this.desiredCount = desiredCount;
}
/**
*
* The number of instantiations of the task definition to run on the service.
*
*
* @return The number of instantiations of the task definition to run on the service.
*/
public Integer getDesiredCount() {
return this.desiredCount;
}
/**
*
* The number of instantiations of the task definition to run on the service.
*
*
* @param desiredCount
* The number of instantiations of the task definition to run on the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withDesiredCount(Integer desiredCount) {
setDesiredCount(desiredCount);
return this;
}
/**
*
* Whether to enable Amazon ECS managed tags for the tasks in the service.
*
*
* @param enableEcsManagedTags
* Whether to enable Amazon ECS managed tags for the tasks in the service.
*/
public void setEnableEcsManagedTags(Boolean enableEcsManagedTags) {
this.enableEcsManagedTags = enableEcsManagedTags;
}
/**
*
* Whether to enable Amazon ECS managed tags for the tasks in the service.
*
*
* @return Whether to enable Amazon ECS managed tags for the tasks in the service.
*/
public Boolean getEnableEcsManagedTags() {
return this.enableEcsManagedTags;
}
/**
*
* Whether to enable Amazon ECS managed tags for the tasks in the service.
*
*
* @param enableEcsManagedTags
* Whether to enable Amazon ECS managed tags for the tasks in the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withEnableEcsManagedTags(Boolean enableEcsManagedTags) {
setEnableEcsManagedTags(enableEcsManagedTags);
return this;
}
/**
*
* Whether to enable Amazon ECS managed tags for the tasks in the service.
*
*
* @return Whether to enable Amazon ECS managed tags for the tasks in the service.
*/
public Boolean isEnableEcsManagedTags() {
return this.enableEcsManagedTags;
}
/**
*
* Whether the execute command functionality is enabled for the service.
*
*
* @param enableExecuteCommand
* Whether the execute command functionality is enabled for the service.
*/
public void setEnableExecuteCommand(Boolean enableExecuteCommand) {
this.enableExecuteCommand = enableExecuteCommand;
}
/**
*
* Whether the execute command functionality is enabled for the service.
*
*
* @return Whether the execute command functionality is enabled for the service.
*/
public Boolean getEnableExecuteCommand() {
return this.enableExecuteCommand;
}
/**
*
* Whether the execute command functionality is enabled for the service.
*
*
* @param enableExecuteCommand
* Whether the execute command functionality is enabled for the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withEnableExecuteCommand(Boolean enableExecuteCommand) {
setEnableExecuteCommand(enableExecuteCommand);
return this;
}
/**
*
* Whether the execute command functionality is enabled for the service.
*
*
* @return Whether the execute command functionality is enabled for the service.
*/
public Boolean isEnableExecuteCommand() {
return this.enableExecuteCommand;
}
/**
*
* After a task starts, the amount of time in seconds that the Amazon ECS service scheduler ignores unhealthy
* Elastic Load Balancing target health checks.
*
*
* @param healthCheckGracePeriodSeconds
* After a task starts, the amount of time in seconds that the Amazon ECS service scheduler ignores unhealthy
* Elastic Load Balancing target health checks.
*/
public void setHealthCheckGracePeriodSeconds(Integer healthCheckGracePeriodSeconds) {
this.healthCheckGracePeriodSeconds = healthCheckGracePeriodSeconds;
}
/**
*
* After a task starts, the amount of time in seconds that the Amazon ECS service scheduler ignores unhealthy
* Elastic Load Balancing target health checks.
*
*
* @return After a task starts, the amount of time in seconds that the Amazon ECS service scheduler ignores
* unhealthy Elastic Load Balancing target health checks.
*/
public Integer getHealthCheckGracePeriodSeconds() {
return this.healthCheckGracePeriodSeconds;
}
/**
*
* After a task starts, the amount of time in seconds that the Amazon ECS service scheduler ignores unhealthy
* Elastic Load Balancing target health checks.
*
*
* @param healthCheckGracePeriodSeconds
* After a task starts, the amount of time in seconds that the Amazon ECS service scheduler ignores unhealthy
* Elastic Load Balancing target health checks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withHealthCheckGracePeriodSeconds(Integer healthCheckGracePeriodSeconds) {
setHealthCheckGracePeriodSeconds(healthCheckGracePeriodSeconds);
return this;
}
/**
*
* The launch type that the service uses.
*
*
* Valid values: EC2
| FARGATE
| EXTERNAL
*
*
* @param launchType
* The launch type that the service uses.
*
* Valid values: EC2
| FARGATE
| EXTERNAL
*/
public void setLaunchType(String launchType) {
this.launchType = launchType;
}
/**
*
* The launch type that the service uses.
*
*
* Valid values: EC2
| FARGATE
| EXTERNAL
*
*
* @return The launch type that the service uses.
*
* Valid values: EC2
| FARGATE
| EXTERNAL
*/
public String getLaunchType() {
return this.launchType;
}
/**
*
* The launch type that the service uses.
*
*
* Valid values: EC2
| FARGATE
| EXTERNAL
*
*
* @param launchType
* The launch type that the service uses.
*
* Valid values: EC2
| FARGATE
| EXTERNAL
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withLaunchType(String launchType) {
setLaunchType(launchType);
return this;
}
/**
*
* Information about the load balancers that the service uses.
*
*
* @return Information about the load balancers that the service uses.
*/
public java.util.List getLoadBalancers() {
return loadBalancers;
}
/**
*
* Information about the load balancers that the service uses.
*
*
* @param loadBalancers
* Information about the load balancers that the service uses.
*/
public void setLoadBalancers(java.util.Collection loadBalancers) {
if (loadBalancers == null) {
this.loadBalancers = null;
return;
}
this.loadBalancers = new java.util.ArrayList(loadBalancers);
}
/**
*
* Information about the load balancers that the service uses.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLoadBalancers(java.util.Collection)} or {@link #withLoadBalancers(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param loadBalancers
* Information about the load balancers that the service uses.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withLoadBalancers(AwsEcsServiceLoadBalancersDetails... loadBalancers) {
if (this.loadBalancers == null) {
setLoadBalancers(new java.util.ArrayList(loadBalancers.length));
}
for (AwsEcsServiceLoadBalancersDetails ele : loadBalancers) {
this.loadBalancers.add(ele);
}
return this;
}
/**
*
* Information about the load balancers that the service uses.
*
*
* @param loadBalancers
* Information about the load balancers that the service uses.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withLoadBalancers(java.util.Collection loadBalancers) {
setLoadBalancers(loadBalancers);
return this;
}
/**
*
* The name of the service.
*
*
* @param name
* The name of the service.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the service.
*
*
* @return The name of the service.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the service.
*
*
* @param name
* The name of the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withName(String name) {
setName(name);
return this;
}
/**
*
* For tasks that use the awsvpc
networking mode, the VPC subnet and security group configuration.
*
*
* @param networkConfiguration
* For tasks that use the awsvpc
networking mode, the VPC subnet and security group
* configuration.
*/
public void setNetworkConfiguration(AwsEcsServiceNetworkConfigurationDetails networkConfiguration) {
this.networkConfiguration = networkConfiguration;
}
/**
*
* For tasks that use the awsvpc
networking mode, the VPC subnet and security group configuration.
*
*
* @return For tasks that use the awsvpc
networking mode, the VPC subnet and security group
* configuration.
*/
public AwsEcsServiceNetworkConfigurationDetails getNetworkConfiguration() {
return this.networkConfiguration;
}
/**
*
* For tasks that use the awsvpc
networking mode, the VPC subnet and security group configuration.
*
*
* @param networkConfiguration
* For tasks that use the awsvpc
networking mode, the VPC subnet and security group
* configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withNetworkConfiguration(AwsEcsServiceNetworkConfigurationDetails networkConfiguration) {
setNetworkConfiguration(networkConfiguration);
return this;
}
/**
*
* The placement constraints for the tasks in the service.
*
*
* @return The placement constraints for the tasks in the service.
*/
public java.util.List getPlacementConstraints() {
return placementConstraints;
}
/**
*
* The placement constraints for the tasks in the service.
*
*
* @param placementConstraints
* The placement constraints for the tasks in the service.
*/
public void setPlacementConstraints(java.util.Collection placementConstraints) {
if (placementConstraints == null) {
this.placementConstraints = null;
return;
}
this.placementConstraints = new java.util.ArrayList(placementConstraints);
}
/**
*
* The placement constraints for the tasks in the service.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementConstraints(java.util.Collection)} or {@link #withPlacementConstraints(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param placementConstraints
* The placement constraints for the tasks in the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withPlacementConstraints(AwsEcsServicePlacementConstraintsDetails... placementConstraints) {
if (this.placementConstraints == null) {
setPlacementConstraints(new java.util.ArrayList(placementConstraints.length));
}
for (AwsEcsServicePlacementConstraintsDetails ele : placementConstraints) {
this.placementConstraints.add(ele);
}
return this;
}
/**
*
* The placement constraints for the tasks in the service.
*
*
* @param placementConstraints
* The placement constraints for the tasks in the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withPlacementConstraints(java.util.Collection placementConstraints) {
setPlacementConstraints(placementConstraints);
return this;
}
/**
*
* Information about how tasks for the service are placed.
*
*
* @return Information about how tasks for the service are placed.
*/
public java.util.List getPlacementStrategies() {
return placementStrategies;
}
/**
*
* Information about how tasks for the service are placed.
*
*
* @param placementStrategies
* Information about how tasks for the service are placed.
*/
public void setPlacementStrategies(java.util.Collection placementStrategies) {
if (placementStrategies == null) {
this.placementStrategies = null;
return;
}
this.placementStrategies = new java.util.ArrayList(placementStrategies);
}
/**
*
* Information about how tasks for the service are placed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementStrategies(java.util.Collection)} or {@link #withPlacementStrategies(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param placementStrategies
* Information about how tasks for the service are placed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withPlacementStrategies(AwsEcsServicePlacementStrategiesDetails... placementStrategies) {
if (this.placementStrategies == null) {
setPlacementStrategies(new java.util.ArrayList(placementStrategies.length));
}
for (AwsEcsServicePlacementStrategiesDetails ele : placementStrategies) {
this.placementStrategies.add(ele);
}
return this;
}
/**
*
* Information about how tasks for the service are placed.
*
*
* @param placementStrategies
* Information about how tasks for the service are placed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withPlacementStrategies(java.util.Collection placementStrategies) {
setPlacementStrategies(placementStrategies);
return this;
}
/**
*
* The platform version on which to run the service. Only specified for tasks that are hosted on Fargate. If a
* platform version is not specified, the LATEST
platform version is used by default.
*
*
* @param platformVersion
* The platform version on which to run the service. Only specified for tasks that are hosted on Fargate. If
* a platform version is not specified, the LATEST
platform version is used by default.
*/
public void setPlatformVersion(String platformVersion) {
this.platformVersion = platformVersion;
}
/**
*
* The platform version on which to run the service. Only specified for tasks that are hosted on Fargate. If a
* platform version is not specified, the LATEST
platform version is used by default.
*
*
* @return The platform version on which to run the service. Only specified for tasks that are hosted on Fargate. If
* a platform version is not specified, the LATEST
platform version is used by default.
*/
public String getPlatformVersion() {
return this.platformVersion;
}
/**
*
* The platform version on which to run the service. Only specified for tasks that are hosted on Fargate. If a
* platform version is not specified, the LATEST
platform version is used by default.
*
*
* @param platformVersion
* The platform version on which to run the service. Only specified for tasks that are hosted on Fargate. If
* a platform version is not specified, the LATEST
platform version is used by default.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withPlatformVersion(String platformVersion) {
setPlatformVersion(platformVersion);
return this;
}
/**
*
* Indicates whether to propagate the tags from the task definition to the task or from the service to the task. If
* no value is provided, then tags are not propagated.
*
*
* Valid values: TASK_DEFINITION
| SERVICE
*
*
* @param propagateTags
* Indicates whether to propagate the tags from the task definition to the task or from the service to the
* task. If no value is provided, then tags are not propagated.
*
* Valid values: TASK_DEFINITION
| SERVICE
*/
public void setPropagateTags(String propagateTags) {
this.propagateTags = propagateTags;
}
/**
*
* Indicates whether to propagate the tags from the task definition to the task or from the service to the task. If
* no value is provided, then tags are not propagated.
*
*
* Valid values: TASK_DEFINITION
| SERVICE
*
*
* @return Indicates whether to propagate the tags from the task definition to the task or from the service to the
* task. If no value is provided, then tags are not propagated.
*
* Valid values: TASK_DEFINITION
| SERVICE
*/
public String getPropagateTags() {
return this.propagateTags;
}
/**
*
* Indicates whether to propagate the tags from the task definition to the task or from the service to the task. If
* no value is provided, then tags are not propagated.
*
*
* Valid values: TASK_DEFINITION
| SERVICE
*
*
* @param propagateTags
* Indicates whether to propagate the tags from the task definition to the task or from the service to the
* task. If no value is provided, then tags are not propagated.
*
* Valid values: TASK_DEFINITION
| SERVICE
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withPropagateTags(String propagateTags) {
setPropagateTags(propagateTags);
return this;
}
/**
*
* The ARN of the IAM role that is associated with the service. The role allows the Amazon ECS container agent to
* register container instances with an Elastic Load Balancing load balancer.
*
*
* @param role
* The ARN of the IAM role that is associated with the service. The role allows the Amazon ECS container
* agent to register container instances with an Elastic Load Balancing load balancer.
*/
public void setRole(String role) {
this.role = role;
}
/**
*
* The ARN of the IAM role that is associated with the service. The role allows the Amazon ECS container agent to
* register container instances with an Elastic Load Balancing load balancer.
*
*
* @return The ARN of the IAM role that is associated with the service. The role allows the Amazon ECS container
* agent to register container instances with an Elastic Load Balancing load balancer.
*/
public String getRole() {
return this.role;
}
/**
*
* The ARN of the IAM role that is associated with the service. The role allows the Amazon ECS container agent to
* register container instances with an Elastic Load Balancing load balancer.
*
*
* @param role
* The ARN of the IAM role that is associated with the service. The role allows the Amazon ECS container
* agent to register container instances with an Elastic Load Balancing load balancer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withRole(String role) {
setRole(role);
return this;
}
/**
*
* The scheduling strategy to use for the service.
*
*
* The REPLICA
scheduling strategy places and maintains the desired number of tasks across the cluster.
* By default, the service scheduler spreads tasks across Availability Zones. Task placement strategies and
* constraints are used to customize task placement decisions.
*
*
* The DAEMON
scheduling strategy deploys exactly one task on each active container instance that meets
* all of the task placement constraints that are specified in the cluster. The service scheduler also evaluates the
* task placement constraints for running tasks and stops tasks that do not meet the placement constraints.
*
*
* Valid values: REPLICA
| DAEMON
*
*
* @param schedulingStrategy
* The scheduling strategy to use for the service.
*
* The REPLICA
scheduling strategy places and maintains the desired number of tasks across the
* cluster. By default, the service scheduler spreads tasks across Availability Zones. Task placement
* strategies and constraints are used to customize task placement decisions.
*
*
* The DAEMON
scheduling strategy deploys exactly one task on each active container instance
* that meets all of the task placement constraints that are specified in the cluster. The service scheduler
* also evaluates the task placement constraints for running tasks and stops tasks that do not meet the
* placement constraints.
*
*
* Valid values: REPLICA
| DAEMON
*/
public void setSchedulingStrategy(String schedulingStrategy) {
this.schedulingStrategy = schedulingStrategy;
}
/**
*
* The scheduling strategy to use for the service.
*
*
* The REPLICA
scheduling strategy places and maintains the desired number of tasks across the cluster.
* By default, the service scheduler spreads tasks across Availability Zones. Task placement strategies and
* constraints are used to customize task placement decisions.
*
*
* The DAEMON
scheduling strategy deploys exactly one task on each active container instance that meets
* all of the task placement constraints that are specified in the cluster. The service scheduler also evaluates the
* task placement constraints for running tasks and stops tasks that do not meet the placement constraints.
*
*
* Valid values: REPLICA
| DAEMON
*
*
* @return The scheduling strategy to use for the service.
*
* The REPLICA
scheduling strategy places and maintains the desired number of tasks across the
* cluster. By default, the service scheduler spreads tasks across Availability Zones. Task placement
* strategies and constraints are used to customize task placement decisions.
*
*
* The DAEMON
scheduling strategy deploys exactly one task on each active container instance
* that meets all of the task placement constraints that are specified in the cluster. The service scheduler
* also evaluates the task placement constraints for running tasks and stops tasks that do not meet the
* placement constraints.
*
*
* Valid values: REPLICA
| DAEMON
*/
public String getSchedulingStrategy() {
return this.schedulingStrategy;
}
/**
*
* The scheduling strategy to use for the service.
*
*
* The REPLICA
scheduling strategy places and maintains the desired number of tasks across the cluster.
* By default, the service scheduler spreads tasks across Availability Zones. Task placement strategies and
* constraints are used to customize task placement decisions.
*
*
* The DAEMON
scheduling strategy deploys exactly one task on each active container instance that meets
* all of the task placement constraints that are specified in the cluster. The service scheduler also evaluates the
* task placement constraints for running tasks and stops tasks that do not meet the placement constraints.
*
*
* Valid values: REPLICA
| DAEMON
*
*
* @param schedulingStrategy
* The scheduling strategy to use for the service.
*
* The REPLICA
scheduling strategy places and maintains the desired number of tasks across the
* cluster. By default, the service scheduler spreads tasks across Availability Zones. Task placement
* strategies and constraints are used to customize task placement decisions.
*
*
* The DAEMON
scheduling strategy deploys exactly one task on each active container instance
* that meets all of the task placement constraints that are specified in the cluster. The service scheduler
* also evaluates the task placement constraints for running tasks and stops tasks that do not meet the
* placement constraints.
*
*
* Valid values: REPLICA
| DAEMON
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withSchedulingStrategy(String schedulingStrategy) {
setSchedulingStrategy(schedulingStrategy);
return this;
}
/**
*
* The ARN of the service.
*
*
* @param serviceArn
* The ARN of the service.
*/
public void setServiceArn(String serviceArn) {
this.serviceArn = serviceArn;
}
/**
*
* The ARN of the service.
*
*
* @return The ARN of the service.
*/
public String getServiceArn() {
return this.serviceArn;
}
/**
*
* The ARN of the service.
*
*
* @param serviceArn
* The ARN of the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withServiceArn(String serviceArn) {
setServiceArn(serviceArn);
return this;
}
/**
*
* The name of the service.
*
*
* The name can contain up to 255 characters. It can use letters, numbers, underscores, and hyphens.
*
*
* @param serviceName
* The name of the service.
*
* The name can contain up to 255 characters. It can use letters, numbers, underscores, and hyphens.
*/
public void setServiceName(String serviceName) {
this.serviceName = serviceName;
}
/**
*
* The name of the service.
*
*
* The name can contain up to 255 characters. It can use letters, numbers, underscores, and hyphens.
*
*
* @return The name of the service.
*
* The name can contain up to 255 characters. It can use letters, numbers, underscores, and hyphens.
*/
public String getServiceName() {
return this.serviceName;
}
/**
*
* The name of the service.
*
*
* The name can contain up to 255 characters. It can use letters, numbers, underscores, and hyphens.
*
*
* @param serviceName
* The name of the service.
*
* The name can contain up to 255 characters. It can use letters, numbers, underscores, and hyphens.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withServiceName(String serviceName) {
setServiceName(serviceName);
return this;
}
/**
*
* Information about the service discovery registries to assign to the service.
*
*
* @return Information about the service discovery registries to assign to the service.
*/
public java.util.List getServiceRegistries() {
return serviceRegistries;
}
/**
*
* Information about the service discovery registries to assign to the service.
*
*
* @param serviceRegistries
* Information about the service discovery registries to assign to the service.
*/
public void setServiceRegistries(java.util.Collection serviceRegistries) {
if (serviceRegistries == null) {
this.serviceRegistries = null;
return;
}
this.serviceRegistries = new java.util.ArrayList(serviceRegistries);
}
/**
*
* Information about the service discovery registries to assign to the service.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServiceRegistries(java.util.Collection)} or {@link #withServiceRegistries(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param serviceRegistries
* Information about the service discovery registries to assign to the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withServiceRegistries(AwsEcsServiceServiceRegistriesDetails... serviceRegistries) {
if (this.serviceRegistries == null) {
setServiceRegistries(new java.util.ArrayList(serviceRegistries.length));
}
for (AwsEcsServiceServiceRegistriesDetails ele : serviceRegistries) {
this.serviceRegistries.add(ele);
}
return this;
}
/**
*
* Information about the service discovery registries to assign to the service.
*
*
* @param serviceRegistries
* Information about the service discovery registries to assign to the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withServiceRegistries(java.util.Collection serviceRegistries) {
setServiceRegistries(serviceRegistries);
return this;
}
/**
*
* The task definition to use for tasks in the service.
*
*
* @param taskDefinition
* The task definition to use for tasks in the service.
*/
public void setTaskDefinition(String taskDefinition) {
this.taskDefinition = taskDefinition;
}
/**
*
* The task definition to use for tasks in the service.
*
*
* @return The task definition to use for tasks in the service.
*/
public String getTaskDefinition() {
return this.taskDefinition;
}
/**
*
* The task definition to use for tasks in the service.
*
*
* @param taskDefinition
* The task definition to use for tasks in the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsServiceDetails withTaskDefinition(String taskDefinition) {
setTaskDefinition(taskDefinition);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCapacityProviderStrategy() != null)
sb.append("CapacityProviderStrategy: ").append(getCapacityProviderStrategy()).append(",");
if (getCluster() != null)
sb.append("Cluster: ").append(getCluster()).append(",");
if (getDeploymentConfiguration() != null)
sb.append("DeploymentConfiguration: ").append(getDeploymentConfiguration()).append(",");
if (getDeploymentController() != null)
sb.append("DeploymentController: ").append(getDeploymentController()).append(",");
if (getDesiredCount() != null)
sb.append("DesiredCount: ").append(getDesiredCount()).append(",");
if (getEnableEcsManagedTags() != null)
sb.append("EnableEcsManagedTags: ").append(getEnableEcsManagedTags()).append(",");
if (getEnableExecuteCommand() != null)
sb.append("EnableExecuteCommand: ").append(getEnableExecuteCommand()).append(",");
if (getHealthCheckGracePeriodSeconds() != null)
sb.append("HealthCheckGracePeriodSeconds: ").append(getHealthCheckGracePeriodSeconds()).append(",");
if (getLaunchType() != null)
sb.append("LaunchType: ").append(getLaunchType()).append(",");
if (getLoadBalancers() != null)
sb.append("LoadBalancers: ").append(getLoadBalancers()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getNetworkConfiguration() != null)
sb.append("NetworkConfiguration: ").append(getNetworkConfiguration()).append(",");
if (getPlacementConstraints() != null)
sb.append("PlacementConstraints: ").append(getPlacementConstraints()).append(",");
if (getPlacementStrategies() != null)
sb.append("PlacementStrategies: ").append(getPlacementStrategies()).append(",");
if (getPlatformVersion() != null)
sb.append("PlatformVersion: ").append(getPlatformVersion()).append(",");
if (getPropagateTags() != null)
sb.append("PropagateTags: ").append(getPropagateTags()).append(",");
if (getRole() != null)
sb.append("Role: ").append(getRole()).append(",");
if (getSchedulingStrategy() != null)
sb.append("SchedulingStrategy: ").append(getSchedulingStrategy()).append(",");
if (getServiceArn() != null)
sb.append("ServiceArn: ").append(getServiceArn()).append(",");
if (getServiceName() != null)
sb.append("ServiceName: ").append(getServiceName()).append(",");
if (getServiceRegistries() != null)
sb.append("ServiceRegistries: ").append(getServiceRegistries()).append(",");
if (getTaskDefinition() != null)
sb.append("TaskDefinition: ").append(getTaskDefinition());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AwsEcsServiceDetails == false)
return false;
AwsEcsServiceDetails other = (AwsEcsServiceDetails) obj;
if (other.getCapacityProviderStrategy() == null ^ this.getCapacityProviderStrategy() == null)
return false;
if (other.getCapacityProviderStrategy() != null && other.getCapacityProviderStrategy().equals(this.getCapacityProviderStrategy()) == false)
return false;
if (other.getCluster() == null ^ this.getCluster() == null)
return false;
if (other.getCluster() != null && other.getCluster().equals(this.getCluster()) == false)
return false;
if (other.getDeploymentConfiguration() == null ^ this.getDeploymentConfiguration() == null)
return false;
if (other.getDeploymentConfiguration() != null && other.getDeploymentConfiguration().equals(this.getDeploymentConfiguration()) == false)
return false;
if (other.getDeploymentController() == null ^ this.getDeploymentController() == null)
return false;
if (other.getDeploymentController() != null && other.getDeploymentController().equals(this.getDeploymentController()) == false)
return false;
if (other.getDesiredCount() == null ^ this.getDesiredCount() == null)
return false;
if (other.getDesiredCount() != null && other.getDesiredCount().equals(this.getDesiredCount()) == false)
return false;
if (other.getEnableEcsManagedTags() == null ^ this.getEnableEcsManagedTags() == null)
return false;
if (other.getEnableEcsManagedTags() != null && other.getEnableEcsManagedTags().equals(this.getEnableEcsManagedTags()) == false)
return false;
if (other.getEnableExecuteCommand() == null ^ this.getEnableExecuteCommand() == null)
return false;
if (other.getEnableExecuteCommand() != null && other.getEnableExecuteCommand().equals(this.getEnableExecuteCommand()) == false)
return false;
if (other.getHealthCheckGracePeriodSeconds() == null ^ this.getHealthCheckGracePeriodSeconds() == null)
return false;
if (other.getHealthCheckGracePeriodSeconds() != null
&& other.getHealthCheckGracePeriodSeconds().equals(this.getHealthCheckGracePeriodSeconds()) == false)
return false;
if (other.getLaunchType() == null ^ this.getLaunchType() == null)
return false;
if (other.getLaunchType() != null && other.getLaunchType().equals(this.getLaunchType()) == false)
return false;
if (other.getLoadBalancers() == null ^ this.getLoadBalancers() == null)
return false;
if (other.getLoadBalancers() != null && other.getLoadBalancers().equals(this.getLoadBalancers()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getNetworkConfiguration() == null ^ this.getNetworkConfiguration() == null)
return false;
if (other.getNetworkConfiguration() != null && other.getNetworkConfiguration().equals(this.getNetworkConfiguration()) == false)
return false;
if (other.getPlacementConstraints() == null ^ this.getPlacementConstraints() == null)
return false;
if (other.getPlacementConstraints() != null && other.getPlacementConstraints().equals(this.getPlacementConstraints()) == false)
return false;
if (other.getPlacementStrategies() == null ^ this.getPlacementStrategies() == null)
return false;
if (other.getPlacementStrategies() != null && other.getPlacementStrategies().equals(this.getPlacementStrategies()) == false)
return false;
if (other.getPlatformVersion() == null ^ this.getPlatformVersion() == null)
return false;
if (other.getPlatformVersion() != null && other.getPlatformVersion().equals(this.getPlatformVersion()) == false)
return false;
if (other.getPropagateTags() == null ^ this.getPropagateTags() == null)
return false;
if (other.getPropagateTags() != null && other.getPropagateTags().equals(this.getPropagateTags()) == false)
return false;
if (other.getRole() == null ^ this.getRole() == null)
return false;
if (other.getRole() != null && other.getRole().equals(this.getRole()) == false)
return false;
if (other.getSchedulingStrategy() == null ^ this.getSchedulingStrategy() == null)
return false;
if (other.getSchedulingStrategy() != null && other.getSchedulingStrategy().equals(this.getSchedulingStrategy()) == false)
return false;
if (other.getServiceArn() == null ^ this.getServiceArn() == null)
return false;
if (other.getServiceArn() != null && other.getServiceArn().equals(this.getServiceArn()) == false)
return false;
if (other.getServiceName() == null ^ this.getServiceName() == null)
return false;
if (other.getServiceName() != null && other.getServiceName().equals(this.getServiceName()) == false)
return false;
if (other.getServiceRegistries() == null ^ this.getServiceRegistries() == null)
return false;
if (other.getServiceRegistries() != null && other.getServiceRegistries().equals(this.getServiceRegistries()) == false)
return false;
if (other.getTaskDefinition() == null ^ this.getTaskDefinition() == null)
return false;
if (other.getTaskDefinition() != null && other.getTaskDefinition().equals(this.getTaskDefinition()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCapacityProviderStrategy() == null) ? 0 : getCapacityProviderStrategy().hashCode());
hashCode = prime * hashCode + ((getCluster() == null) ? 0 : getCluster().hashCode());
hashCode = prime * hashCode + ((getDeploymentConfiguration() == null) ? 0 : getDeploymentConfiguration().hashCode());
hashCode = prime * hashCode + ((getDeploymentController() == null) ? 0 : getDeploymentController().hashCode());
hashCode = prime * hashCode + ((getDesiredCount() == null) ? 0 : getDesiredCount().hashCode());
hashCode = prime * hashCode + ((getEnableEcsManagedTags() == null) ? 0 : getEnableEcsManagedTags().hashCode());
hashCode = prime * hashCode + ((getEnableExecuteCommand() == null) ? 0 : getEnableExecuteCommand().hashCode());
hashCode = prime * hashCode + ((getHealthCheckGracePeriodSeconds() == null) ? 0 : getHealthCheckGracePeriodSeconds().hashCode());
hashCode = prime * hashCode + ((getLaunchType() == null) ? 0 : getLaunchType().hashCode());
hashCode = prime * hashCode + ((getLoadBalancers() == null) ? 0 : getLoadBalancers().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getNetworkConfiguration() == null) ? 0 : getNetworkConfiguration().hashCode());
hashCode = prime * hashCode + ((getPlacementConstraints() == null) ? 0 : getPlacementConstraints().hashCode());
hashCode = prime * hashCode + ((getPlacementStrategies() == null) ? 0 : getPlacementStrategies().hashCode());
hashCode = prime * hashCode + ((getPlatformVersion() == null) ? 0 : getPlatformVersion().hashCode());
hashCode = prime * hashCode + ((getPropagateTags() == null) ? 0 : getPropagateTags().hashCode());
hashCode = prime * hashCode + ((getRole() == null) ? 0 : getRole().hashCode());
hashCode = prime * hashCode + ((getSchedulingStrategy() == null) ? 0 : getSchedulingStrategy().hashCode());
hashCode = prime * hashCode + ((getServiceArn() == null) ? 0 : getServiceArn().hashCode());
hashCode = prime * hashCode + ((getServiceName() == null) ? 0 : getServiceName().hashCode());
hashCode = prime * hashCode + ((getServiceRegistries() == null) ? 0 : getServiceRegistries().hashCode());
hashCode = prime * hashCode + ((getTaskDefinition() == null) ? 0 : getTaskDefinition().hashCode());
return hashCode;
}
@Override
public AwsEcsServiceDetails clone() {
try {
return (AwsEcsServiceDetails) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.securityhub.model.transform.AwsEcsServiceDetailsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}