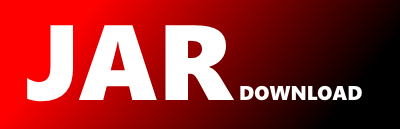
com.amazonaws.services.securityhub.model.AwsEcsTaskDefinitionContainerDefinitionsDetails Maven / Gradle / Ivy
Show all versions of aws-java-sdk-securityhub Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.securityhub.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A container definition that describes a container in the task.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AwsEcsTaskDefinitionContainerDefinitionsDetails implements Serializable, Cloneable, StructuredPojo {
/**
*
* The command that is passed to the container.
*
*/
private java.util.List command;
/**
*
* The number of CPU units reserved for the container.
*
*/
private Integer cpu;
/**
*
* The dependencies that are defined for container startup and shutdown.
*
*/
private java.util.List dependsOn;
/**
*
* Whether to disable networking within the container.
*
*/
private Boolean disableNetworking;
/**
*
* A list of DNS search domains that are presented to the container.
*
*/
private java.util.List dnsSearchDomains;
/**
*
* A list of DNS servers that are presented to the container.
*
*/
private java.util.List dnsServers;
/**
*
* A key-value map of labels to add to the container.
*
*/
private java.util.Map dockerLabels;
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
*
*/
private java.util.List dockerSecurityOptions;
/**
*
* The entry point that is passed to the container.
*
*/
private java.util.List entryPoint;
/**
*
* The environment variables to pass to a container.
*
*/
private java.util.List environment;
/**
*
* A list of files containing the environment variables to pass to a container.
*
*/
private java.util.List environmentFiles;
/**
*
* Whether the container is essential. All tasks must have at least one essential container.
*
*/
private Boolean essential;
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
*
*/
private java.util.List extraHosts;
/**
*
* The FireLens configuration for the container. Specifies and configures a log router for container logs.
*
*/
private AwsEcsTaskDefinitionContainerDefinitionsFirelensConfigurationDetails firelensConfiguration;
/**
*
* The container health check command and associated configuration parameters for the container.
*
*/
private AwsEcsTaskDefinitionContainerDefinitionsHealthCheckDetails healthCheck;
/**
*
* The hostname to use for the container.
*
*/
private String hostname;
/**
*
* The image used to start the container.
*
*/
private String image;
/**
*
* If set to true, then containerized applications can be deployed that require stdin
or a
* tty
to be allocated.
*
*/
private Boolean interactive;
/**
*
* A list of links for the container in the form container_name:alias
. Allows
* containers to communicate with each other without the need for port mappings.
*
*/
private java.util.List links;
/**
*
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities.
*
*/
private AwsEcsTaskDefinitionContainerDefinitionsLinuxParametersDetails linuxParameters;
/**
*
* The log configuration specification for the container.
*
*/
private AwsEcsTaskDefinitionContainerDefinitionsLogConfigurationDetails logConfiguration;
/**
*
* The amount (in MiB) of memory to present to the container. If the container attempts to exceed the memory
* specified here, the container is shut down. The total amount of memory reserved for all containers within a task
* must be lower than the task memory value, if one is specified.
*
*/
private Integer memory;
/**
*
* The soft limit (in MiB) of memory to reserve for the container.
*
*/
private Integer memoryReservation;
/**
*
* The mount points for the data volumes in the container.
*
*/
private java.util.List mountPoints;
/**
*
* The name of the container.
*
*/
private String name;
/**
*
* The list of port mappings for the container.
*
*/
private java.util.List portMappings;
/**
*
* Whether the container is given elevated privileges on the host container instance. The elevated privileges are
* similar to the root user.
*
*/
private Boolean privileged;
/**
*
* Whether to allocate a TTY to the container.
*
*/
private Boolean pseudoTerminal;
/**
*
* Whether the container is given read-only access to its root file system.
*
*/
private Boolean readonlyRootFilesystem;
/**
*
* The private repository authentication credentials to use.
*
*/
private AwsEcsTaskDefinitionContainerDefinitionsRepositoryCredentialsDetails repositoryCredentials;
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*/
private java.util.List resourceRequirements;
/**
*
* The secrets to pass to the container.
*
*/
private java.util.List secrets;
/**
*
* The number of seconds to wait before giving up on resolving dependencies for a container.
*
*/
private Integer startTimeout;
/**
*
* The number of seconds to wait before the container is stopped if it doesn't shut down normally on its own.
*
*/
private Integer stopTimeout;
/**
*
* A list of namespaced kernel parameters to set in the container.
*
*/
private java.util.List systemControls;
/**
*
* A list of ulimits to set in the container.
*
*/
private java.util.List ulimits;
/**
*
* The user to use inside the container.
*
*
* The value can use one of the following formats.
*
*
* -
*
* user
*
*
* -
*
* user
: group
*
*
* -
*
* uid
*
*
* -
*
* uid
: gid
*
*
* -
*
* user
: gid
*
*
* -
*
* uid
: group
*
*
*
*/
private String user;
/**
*
* Data volumes to mount from another container.
*
*/
private java.util.List volumesFrom;
/**
*
* The working directory in which to run commands inside the container.
*
*/
private String workingDirectory;
/**
*
* The command that is passed to the container.
*
*
* @return The command that is passed to the container.
*/
public java.util.List getCommand() {
return command;
}
/**
*
* The command that is passed to the container.
*
*
* @param command
* The command that is passed to the container.
*/
public void setCommand(java.util.Collection command) {
if (command == null) {
this.command = null;
return;
}
this.command = new java.util.ArrayList(command);
}
/**
*
* The command that is passed to the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCommand(java.util.Collection)} or {@link #withCommand(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param command
* The command that is passed to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withCommand(String... command) {
if (this.command == null) {
setCommand(new java.util.ArrayList(command.length));
}
for (String ele : command) {
this.command.add(ele);
}
return this;
}
/**
*
* The command that is passed to the container.
*
*
* @param command
* The command that is passed to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withCommand(java.util.Collection command) {
setCommand(command);
return this;
}
/**
*
* The number of CPU units reserved for the container.
*
*
* @param cpu
* The number of CPU units reserved for the container.
*/
public void setCpu(Integer cpu) {
this.cpu = cpu;
}
/**
*
* The number of CPU units reserved for the container.
*
*
* @return The number of CPU units reserved for the container.
*/
public Integer getCpu() {
return this.cpu;
}
/**
*
* The number of CPU units reserved for the container.
*
*
* @param cpu
* The number of CPU units reserved for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withCpu(Integer cpu) {
setCpu(cpu);
return this;
}
/**
*
* The dependencies that are defined for container startup and shutdown.
*
*
* @return The dependencies that are defined for container startup and shutdown.
*/
public java.util.List getDependsOn() {
return dependsOn;
}
/**
*
* The dependencies that are defined for container startup and shutdown.
*
*
* @param dependsOn
* The dependencies that are defined for container startup and shutdown.
*/
public void setDependsOn(java.util.Collection dependsOn) {
if (dependsOn == null) {
this.dependsOn = null;
return;
}
this.dependsOn = new java.util.ArrayList(dependsOn);
}
/**
*
* The dependencies that are defined for container startup and shutdown.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDependsOn(java.util.Collection)} or {@link #withDependsOn(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dependsOn
* The dependencies that are defined for container startup and shutdown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDependsOn(AwsEcsTaskDefinitionContainerDefinitionsDependsOnDetails... dependsOn) {
if (this.dependsOn == null) {
setDependsOn(new java.util.ArrayList(dependsOn.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsDependsOnDetails ele : dependsOn) {
this.dependsOn.add(ele);
}
return this;
}
/**
*
* The dependencies that are defined for container startup and shutdown.
*
*
* @param dependsOn
* The dependencies that are defined for container startup and shutdown.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDependsOn(
java.util.Collection dependsOn) {
setDependsOn(dependsOn);
return this;
}
/**
*
* Whether to disable networking within the container.
*
*
* @param disableNetworking
* Whether to disable networking within the container.
*/
public void setDisableNetworking(Boolean disableNetworking) {
this.disableNetworking = disableNetworking;
}
/**
*
* Whether to disable networking within the container.
*
*
* @return Whether to disable networking within the container.
*/
public Boolean getDisableNetworking() {
return this.disableNetworking;
}
/**
*
* Whether to disable networking within the container.
*
*
* @param disableNetworking
* Whether to disable networking within the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDisableNetworking(Boolean disableNetworking) {
setDisableNetworking(disableNetworking);
return this;
}
/**
*
* Whether to disable networking within the container.
*
*
* @return Whether to disable networking within the container.
*/
public Boolean isDisableNetworking() {
return this.disableNetworking;
}
/**
*
* A list of DNS search domains that are presented to the container.
*
*
* @return A list of DNS search domains that are presented to the container.
*/
public java.util.List getDnsSearchDomains() {
return dnsSearchDomains;
}
/**
*
* A list of DNS search domains that are presented to the container.
*
*
* @param dnsSearchDomains
* A list of DNS search domains that are presented to the container.
*/
public void setDnsSearchDomains(java.util.Collection dnsSearchDomains) {
if (dnsSearchDomains == null) {
this.dnsSearchDomains = null;
return;
}
this.dnsSearchDomains = new java.util.ArrayList(dnsSearchDomains);
}
/**
*
* A list of DNS search domains that are presented to the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDnsSearchDomains(java.util.Collection)} or {@link #withDnsSearchDomains(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param dnsSearchDomains
* A list of DNS search domains that are presented to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDnsSearchDomains(String... dnsSearchDomains) {
if (this.dnsSearchDomains == null) {
setDnsSearchDomains(new java.util.ArrayList(dnsSearchDomains.length));
}
for (String ele : dnsSearchDomains) {
this.dnsSearchDomains.add(ele);
}
return this;
}
/**
*
* A list of DNS search domains that are presented to the container.
*
*
* @param dnsSearchDomains
* A list of DNS search domains that are presented to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDnsSearchDomains(java.util.Collection dnsSearchDomains) {
setDnsSearchDomains(dnsSearchDomains);
return this;
}
/**
*
* A list of DNS servers that are presented to the container.
*
*
* @return A list of DNS servers that are presented to the container.
*/
public java.util.List getDnsServers() {
return dnsServers;
}
/**
*
* A list of DNS servers that are presented to the container.
*
*
* @param dnsServers
* A list of DNS servers that are presented to the container.
*/
public void setDnsServers(java.util.Collection dnsServers) {
if (dnsServers == null) {
this.dnsServers = null;
return;
}
this.dnsServers = new java.util.ArrayList(dnsServers);
}
/**
*
* A list of DNS servers that are presented to the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDnsServers(java.util.Collection)} or {@link #withDnsServers(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dnsServers
* A list of DNS servers that are presented to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDnsServers(String... dnsServers) {
if (this.dnsServers == null) {
setDnsServers(new java.util.ArrayList(dnsServers.length));
}
for (String ele : dnsServers) {
this.dnsServers.add(ele);
}
return this;
}
/**
*
* A list of DNS servers that are presented to the container.
*
*
* @param dnsServers
* A list of DNS servers that are presented to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDnsServers(java.util.Collection dnsServers) {
setDnsServers(dnsServers);
return this;
}
/**
*
* A key-value map of labels to add to the container.
*
*
* @return A key-value map of labels to add to the container.
*/
public java.util.Map getDockerLabels() {
return dockerLabels;
}
/**
*
* A key-value map of labels to add to the container.
*
*
* @param dockerLabels
* A key-value map of labels to add to the container.
*/
public void setDockerLabels(java.util.Map dockerLabels) {
this.dockerLabels = dockerLabels;
}
/**
*
* A key-value map of labels to add to the container.
*
*
* @param dockerLabels
* A key-value map of labels to add to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDockerLabels(java.util.Map dockerLabels) {
setDockerLabels(dockerLabels);
return this;
}
/**
* Add a single DockerLabels entry
*
* @see AwsEcsTaskDefinitionContainerDefinitionsDetails#withDockerLabels
* @returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails addDockerLabelsEntry(String key, String value) {
if (null == this.dockerLabels) {
this.dockerLabels = new java.util.HashMap();
}
if (this.dockerLabels.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.dockerLabels.put(key, value);
return this;
}
/**
* Removes all the entries added into DockerLabels.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails clearDockerLabelsEntries() {
this.dockerLabels = null;
return this;
}
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
*
*
* @return A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
*/
public java.util.List getDockerSecurityOptions() {
return dockerSecurityOptions;
}
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
*
*
* @param dockerSecurityOptions
* A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
*/
public void setDockerSecurityOptions(java.util.Collection dockerSecurityOptions) {
if (dockerSecurityOptions == null) {
this.dockerSecurityOptions = null;
return;
}
this.dockerSecurityOptions = new java.util.ArrayList(dockerSecurityOptions);
}
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDockerSecurityOptions(java.util.Collection)} or
* {@link #withDockerSecurityOptions(java.util.Collection)} if you want to override the existing values.
*
*
* @param dockerSecurityOptions
* A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDockerSecurityOptions(String... dockerSecurityOptions) {
if (this.dockerSecurityOptions == null) {
setDockerSecurityOptions(new java.util.ArrayList(dockerSecurityOptions.length));
}
for (String ele : dockerSecurityOptions) {
this.dockerSecurityOptions.add(ele);
}
return this;
}
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
*
*
* @param dockerSecurityOptions
* A list of strings to provide custom labels for SELinux and AppArmor multi-level security systems.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withDockerSecurityOptions(java.util.Collection dockerSecurityOptions) {
setDockerSecurityOptions(dockerSecurityOptions);
return this;
}
/**
*
* The entry point that is passed to the container.
*
*
* @return The entry point that is passed to the container.
*/
public java.util.List getEntryPoint() {
return entryPoint;
}
/**
*
* The entry point that is passed to the container.
*
*
* @param entryPoint
* The entry point that is passed to the container.
*/
public void setEntryPoint(java.util.Collection entryPoint) {
if (entryPoint == null) {
this.entryPoint = null;
return;
}
this.entryPoint = new java.util.ArrayList(entryPoint);
}
/**
*
* The entry point that is passed to the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEntryPoint(java.util.Collection)} or {@link #withEntryPoint(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param entryPoint
* The entry point that is passed to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withEntryPoint(String... entryPoint) {
if (this.entryPoint == null) {
setEntryPoint(new java.util.ArrayList(entryPoint.length));
}
for (String ele : entryPoint) {
this.entryPoint.add(ele);
}
return this;
}
/**
*
* The entry point that is passed to the container.
*
*
* @param entryPoint
* The entry point that is passed to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withEntryPoint(java.util.Collection entryPoint) {
setEntryPoint(entryPoint);
return this;
}
/**
*
* The environment variables to pass to a container.
*
*
* @return The environment variables to pass to a container.
*/
public java.util.List getEnvironment() {
return environment;
}
/**
*
* The environment variables to pass to a container.
*
*
* @param environment
* The environment variables to pass to a container.
*/
public void setEnvironment(java.util.Collection environment) {
if (environment == null) {
this.environment = null;
return;
}
this.environment = new java.util.ArrayList(environment);
}
/**
*
* The environment variables to pass to a container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEnvironment(java.util.Collection)} or {@link #withEnvironment(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param environment
* The environment variables to pass to a container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withEnvironment(AwsEcsTaskDefinitionContainerDefinitionsEnvironmentDetails... environment) {
if (this.environment == null) {
setEnvironment(new java.util.ArrayList(environment.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsEnvironmentDetails ele : environment) {
this.environment.add(ele);
}
return this;
}
/**
*
* The environment variables to pass to a container.
*
*
* @param environment
* The environment variables to pass to a container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withEnvironment(
java.util.Collection environment) {
setEnvironment(environment);
return this;
}
/**
*
* A list of files containing the environment variables to pass to a container.
*
*
* @return A list of files containing the environment variables to pass to a container.
*/
public java.util.List getEnvironmentFiles() {
return environmentFiles;
}
/**
*
* A list of files containing the environment variables to pass to a container.
*
*
* @param environmentFiles
* A list of files containing the environment variables to pass to a container.
*/
public void setEnvironmentFiles(java.util.Collection environmentFiles) {
if (environmentFiles == null) {
this.environmentFiles = null;
return;
}
this.environmentFiles = new java.util.ArrayList(environmentFiles);
}
/**
*
* A list of files containing the environment variables to pass to a container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEnvironmentFiles(java.util.Collection)} or {@link #withEnvironmentFiles(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param environmentFiles
* A list of files containing the environment variables to pass to a container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withEnvironmentFiles(
AwsEcsTaskDefinitionContainerDefinitionsEnvironmentFilesDetails... environmentFiles) {
if (this.environmentFiles == null) {
setEnvironmentFiles(new java.util.ArrayList(environmentFiles.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsEnvironmentFilesDetails ele : environmentFiles) {
this.environmentFiles.add(ele);
}
return this;
}
/**
*
* A list of files containing the environment variables to pass to a container.
*
*
* @param environmentFiles
* A list of files containing the environment variables to pass to a container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withEnvironmentFiles(
java.util.Collection environmentFiles) {
setEnvironmentFiles(environmentFiles);
return this;
}
/**
*
* Whether the container is essential. All tasks must have at least one essential container.
*
*
* @param essential
* Whether the container is essential. All tasks must have at least one essential container.
*/
public void setEssential(Boolean essential) {
this.essential = essential;
}
/**
*
* Whether the container is essential. All tasks must have at least one essential container.
*
*
* @return Whether the container is essential. All tasks must have at least one essential container.
*/
public Boolean getEssential() {
return this.essential;
}
/**
*
* Whether the container is essential. All tasks must have at least one essential container.
*
*
* @param essential
* Whether the container is essential. All tasks must have at least one essential container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withEssential(Boolean essential) {
setEssential(essential);
return this;
}
/**
*
* Whether the container is essential. All tasks must have at least one essential container.
*
*
* @return Whether the container is essential. All tasks must have at least one essential container.
*/
public Boolean isEssential() {
return this.essential;
}
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
*
*
* @return A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
*/
public java.util.List getExtraHosts() {
return extraHosts;
}
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
*
*
* @param extraHosts
* A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
*/
public void setExtraHosts(java.util.Collection extraHosts) {
if (extraHosts == null) {
this.extraHosts = null;
return;
}
this.extraHosts = new java.util.ArrayList(extraHosts);
}
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExtraHosts(java.util.Collection)} or {@link #withExtraHosts(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param extraHosts
* A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withExtraHosts(AwsEcsTaskDefinitionContainerDefinitionsExtraHostsDetails... extraHosts) {
if (this.extraHosts == null) {
setExtraHosts(new java.util.ArrayList(extraHosts.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsExtraHostsDetails ele : extraHosts) {
this.extraHosts.add(ele);
}
return this;
}
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
*
*
* @param extraHosts
* A list of hostnames and IP address mappings to append to the /etc/hosts file on the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withExtraHosts(
java.util.Collection extraHosts) {
setExtraHosts(extraHosts);
return this;
}
/**
*
* The FireLens configuration for the container. Specifies and configures a log router for container logs.
*
*
* @param firelensConfiguration
* The FireLens configuration for the container. Specifies and configures a log router for container logs.
*/
public void setFirelensConfiguration(AwsEcsTaskDefinitionContainerDefinitionsFirelensConfigurationDetails firelensConfiguration) {
this.firelensConfiguration = firelensConfiguration;
}
/**
*
* The FireLens configuration for the container. Specifies and configures a log router for container logs.
*
*
* @return The FireLens configuration for the container. Specifies and configures a log router for container logs.
*/
public AwsEcsTaskDefinitionContainerDefinitionsFirelensConfigurationDetails getFirelensConfiguration() {
return this.firelensConfiguration;
}
/**
*
* The FireLens configuration for the container. Specifies and configures a log router for container logs.
*
*
* @param firelensConfiguration
* The FireLens configuration for the container. Specifies and configures a log router for container logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withFirelensConfiguration(
AwsEcsTaskDefinitionContainerDefinitionsFirelensConfigurationDetails firelensConfiguration) {
setFirelensConfiguration(firelensConfiguration);
return this;
}
/**
*
* The container health check command and associated configuration parameters for the container.
*
*
* @param healthCheck
* The container health check command and associated configuration parameters for the container.
*/
public void setHealthCheck(AwsEcsTaskDefinitionContainerDefinitionsHealthCheckDetails healthCheck) {
this.healthCheck = healthCheck;
}
/**
*
* The container health check command and associated configuration parameters for the container.
*
*
* @return The container health check command and associated configuration parameters for the container.
*/
public AwsEcsTaskDefinitionContainerDefinitionsHealthCheckDetails getHealthCheck() {
return this.healthCheck;
}
/**
*
* The container health check command and associated configuration parameters for the container.
*
*
* @param healthCheck
* The container health check command and associated configuration parameters for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withHealthCheck(AwsEcsTaskDefinitionContainerDefinitionsHealthCheckDetails healthCheck) {
setHealthCheck(healthCheck);
return this;
}
/**
*
* The hostname to use for the container.
*
*
* @param hostname
* The hostname to use for the container.
*/
public void setHostname(String hostname) {
this.hostname = hostname;
}
/**
*
* The hostname to use for the container.
*
*
* @return The hostname to use for the container.
*/
public String getHostname() {
return this.hostname;
}
/**
*
* The hostname to use for the container.
*
*
* @param hostname
* The hostname to use for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withHostname(String hostname) {
setHostname(hostname);
return this;
}
/**
*
* The image used to start the container.
*
*
* @param image
* The image used to start the container.
*/
public void setImage(String image) {
this.image = image;
}
/**
*
* The image used to start the container.
*
*
* @return The image used to start the container.
*/
public String getImage() {
return this.image;
}
/**
*
* The image used to start the container.
*
*
* @param image
* The image used to start the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withImage(String image) {
setImage(image);
return this;
}
/**
*
* If set to true, then containerized applications can be deployed that require stdin
or a
* tty
to be allocated.
*
*
* @param interactive
* If set to true, then containerized applications can be deployed that require stdin
or a
* tty
to be allocated.
*/
public void setInteractive(Boolean interactive) {
this.interactive = interactive;
}
/**
*
* If set to true, then containerized applications can be deployed that require stdin
or a
* tty
to be allocated.
*
*
* @return If set to true, then containerized applications can be deployed that require stdin
or a
* tty
to be allocated.
*/
public Boolean getInteractive() {
return this.interactive;
}
/**
*
* If set to true, then containerized applications can be deployed that require stdin
or a
* tty
to be allocated.
*
*
* @param interactive
* If set to true, then containerized applications can be deployed that require stdin
or a
* tty
to be allocated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withInteractive(Boolean interactive) {
setInteractive(interactive);
return this;
}
/**
*
* If set to true, then containerized applications can be deployed that require stdin
or a
* tty
to be allocated.
*
*
* @return If set to true, then containerized applications can be deployed that require stdin
or a
* tty
to be allocated.
*/
public Boolean isInteractive() {
return this.interactive;
}
/**
*
* A list of links for the container in the form container_name:alias
. Allows
* containers to communicate with each other without the need for port mappings.
*
*
* @return A list of links for the container in the form container_name:alias
. Allows
* containers to communicate with each other without the need for port mappings.
*/
public java.util.List getLinks() {
return links;
}
/**
*
* A list of links for the container in the form container_name:alias
. Allows
* containers to communicate with each other without the need for port mappings.
*
*
* @param links
* A list of links for the container in the form container_name:alias
. Allows
* containers to communicate with each other without the need for port mappings.
*/
public void setLinks(java.util.Collection links) {
if (links == null) {
this.links = null;
return;
}
this.links = new java.util.ArrayList(links);
}
/**
*
* A list of links for the container in the form container_name:alias
. Allows
* containers to communicate with each other without the need for port mappings.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLinks(java.util.Collection)} or {@link #withLinks(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param links
* A list of links for the container in the form container_name:alias
. Allows
* containers to communicate with each other without the need for port mappings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withLinks(String... links) {
if (this.links == null) {
setLinks(new java.util.ArrayList(links.length));
}
for (String ele : links) {
this.links.add(ele);
}
return this;
}
/**
*
* A list of links for the container in the form container_name:alias
. Allows
* containers to communicate with each other without the need for port mappings.
*
*
* @param links
* A list of links for the container in the form container_name:alias
. Allows
* containers to communicate with each other without the need for port mappings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withLinks(java.util.Collection links) {
setLinks(links);
return this;
}
/**
*
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities.
*
*
* @param linuxParameters
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities.
*/
public void setLinuxParameters(AwsEcsTaskDefinitionContainerDefinitionsLinuxParametersDetails linuxParameters) {
this.linuxParameters = linuxParameters;
}
/**
*
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities.
*
*
* @return Linux-specific modifications that are applied to the container, such as Linux kernel capabilities.
*/
public AwsEcsTaskDefinitionContainerDefinitionsLinuxParametersDetails getLinuxParameters() {
return this.linuxParameters;
}
/**
*
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities.
*
*
* @param linuxParameters
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withLinuxParameters(AwsEcsTaskDefinitionContainerDefinitionsLinuxParametersDetails linuxParameters) {
setLinuxParameters(linuxParameters);
return this;
}
/**
*
* The log configuration specification for the container.
*
*
* @param logConfiguration
* The log configuration specification for the container.
*/
public void setLogConfiguration(AwsEcsTaskDefinitionContainerDefinitionsLogConfigurationDetails logConfiguration) {
this.logConfiguration = logConfiguration;
}
/**
*
* The log configuration specification for the container.
*
*
* @return The log configuration specification for the container.
*/
public AwsEcsTaskDefinitionContainerDefinitionsLogConfigurationDetails getLogConfiguration() {
return this.logConfiguration;
}
/**
*
* The log configuration specification for the container.
*
*
* @param logConfiguration
* The log configuration specification for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withLogConfiguration(AwsEcsTaskDefinitionContainerDefinitionsLogConfigurationDetails logConfiguration) {
setLogConfiguration(logConfiguration);
return this;
}
/**
*
* The amount (in MiB) of memory to present to the container. If the container attempts to exceed the memory
* specified here, the container is shut down. The total amount of memory reserved for all containers within a task
* must be lower than the task memory value, if one is specified.
*
*
* @param memory
* The amount (in MiB) of memory to present to the container. If the container attempts to exceed the memory
* specified here, the container is shut down. The total amount of memory reserved for all containers within
* a task must be lower than the task memory value, if one is specified.
*/
public void setMemory(Integer memory) {
this.memory = memory;
}
/**
*
* The amount (in MiB) of memory to present to the container. If the container attempts to exceed the memory
* specified here, the container is shut down. The total amount of memory reserved for all containers within a task
* must be lower than the task memory value, if one is specified.
*
*
* @return The amount (in MiB) of memory to present to the container. If the container attempts to exceed the memory
* specified here, the container is shut down. The total amount of memory reserved for all containers within
* a task must be lower than the task memory value, if one is specified.
*/
public Integer getMemory() {
return this.memory;
}
/**
*
* The amount (in MiB) of memory to present to the container. If the container attempts to exceed the memory
* specified here, the container is shut down. The total amount of memory reserved for all containers within a task
* must be lower than the task memory value, if one is specified.
*
*
* @param memory
* The amount (in MiB) of memory to present to the container. If the container attempts to exceed the memory
* specified here, the container is shut down. The total amount of memory reserved for all containers within
* a task must be lower than the task memory value, if one is specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withMemory(Integer memory) {
setMemory(memory);
return this;
}
/**
*
* The soft limit (in MiB) of memory to reserve for the container.
*
*
* @param memoryReservation
* The soft limit (in MiB) of memory to reserve for the container.
*/
public void setMemoryReservation(Integer memoryReservation) {
this.memoryReservation = memoryReservation;
}
/**
*
* The soft limit (in MiB) of memory to reserve for the container.
*
*
* @return The soft limit (in MiB) of memory to reserve for the container.
*/
public Integer getMemoryReservation() {
return this.memoryReservation;
}
/**
*
* The soft limit (in MiB) of memory to reserve for the container.
*
*
* @param memoryReservation
* The soft limit (in MiB) of memory to reserve for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withMemoryReservation(Integer memoryReservation) {
setMemoryReservation(memoryReservation);
return this;
}
/**
*
* The mount points for the data volumes in the container.
*
*
* @return The mount points for the data volumes in the container.
*/
public java.util.List getMountPoints() {
return mountPoints;
}
/**
*
* The mount points for the data volumes in the container.
*
*
* @param mountPoints
* The mount points for the data volumes in the container.
*/
public void setMountPoints(java.util.Collection mountPoints) {
if (mountPoints == null) {
this.mountPoints = null;
return;
}
this.mountPoints = new java.util.ArrayList(mountPoints);
}
/**
*
* The mount points for the data volumes in the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMountPoints(java.util.Collection)} or {@link #withMountPoints(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param mountPoints
* The mount points for the data volumes in the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withMountPoints(AwsEcsTaskDefinitionContainerDefinitionsMountPointsDetails... mountPoints) {
if (this.mountPoints == null) {
setMountPoints(new java.util.ArrayList(mountPoints.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsMountPointsDetails ele : mountPoints) {
this.mountPoints.add(ele);
}
return this;
}
/**
*
* The mount points for the data volumes in the container.
*
*
* @param mountPoints
* The mount points for the data volumes in the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withMountPoints(
java.util.Collection mountPoints) {
setMountPoints(mountPoints);
return this;
}
/**
*
* The name of the container.
*
*
* @param name
* The name of the container.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the container.
*
*
* @return The name of the container.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the container.
*
*
* @param name
* The name of the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withName(String name) {
setName(name);
return this;
}
/**
*
* The list of port mappings for the container.
*
*
* @return The list of port mappings for the container.
*/
public java.util.List getPortMappings() {
return portMappings;
}
/**
*
* The list of port mappings for the container.
*
*
* @param portMappings
* The list of port mappings for the container.
*/
public void setPortMappings(java.util.Collection portMappings) {
if (portMappings == null) {
this.portMappings = null;
return;
}
this.portMappings = new java.util.ArrayList(portMappings);
}
/**
*
* The list of port mappings for the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPortMappings(java.util.Collection)} or {@link #withPortMappings(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param portMappings
* The list of port mappings for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withPortMappings(AwsEcsTaskDefinitionContainerDefinitionsPortMappingsDetails... portMappings) {
if (this.portMappings == null) {
setPortMappings(new java.util.ArrayList(portMappings.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsPortMappingsDetails ele : portMappings) {
this.portMappings.add(ele);
}
return this;
}
/**
*
* The list of port mappings for the container.
*
*
* @param portMappings
* The list of port mappings for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withPortMappings(
java.util.Collection portMappings) {
setPortMappings(portMappings);
return this;
}
/**
*
* Whether the container is given elevated privileges on the host container instance. The elevated privileges are
* similar to the root user.
*
*
* @param privileged
* Whether the container is given elevated privileges on the host container instance. The elevated privileges
* are similar to the root user.
*/
public void setPrivileged(Boolean privileged) {
this.privileged = privileged;
}
/**
*
* Whether the container is given elevated privileges on the host container instance. The elevated privileges are
* similar to the root user.
*
*
* @return Whether the container is given elevated privileges on the host container instance. The elevated
* privileges are similar to the root user.
*/
public Boolean getPrivileged() {
return this.privileged;
}
/**
*
* Whether the container is given elevated privileges on the host container instance. The elevated privileges are
* similar to the root user.
*
*
* @param privileged
* Whether the container is given elevated privileges on the host container instance. The elevated privileges
* are similar to the root user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withPrivileged(Boolean privileged) {
setPrivileged(privileged);
return this;
}
/**
*
* Whether the container is given elevated privileges on the host container instance. The elevated privileges are
* similar to the root user.
*
*
* @return Whether the container is given elevated privileges on the host container instance. The elevated
* privileges are similar to the root user.
*/
public Boolean isPrivileged() {
return this.privileged;
}
/**
*
* Whether to allocate a TTY to the container.
*
*
* @param pseudoTerminal
* Whether to allocate a TTY to the container.
*/
public void setPseudoTerminal(Boolean pseudoTerminal) {
this.pseudoTerminal = pseudoTerminal;
}
/**
*
* Whether to allocate a TTY to the container.
*
*
* @return Whether to allocate a TTY to the container.
*/
public Boolean getPseudoTerminal() {
return this.pseudoTerminal;
}
/**
*
* Whether to allocate a TTY to the container.
*
*
* @param pseudoTerminal
* Whether to allocate a TTY to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withPseudoTerminal(Boolean pseudoTerminal) {
setPseudoTerminal(pseudoTerminal);
return this;
}
/**
*
* Whether to allocate a TTY to the container.
*
*
* @return Whether to allocate a TTY to the container.
*/
public Boolean isPseudoTerminal() {
return this.pseudoTerminal;
}
/**
*
* Whether the container is given read-only access to its root file system.
*
*
* @param readonlyRootFilesystem
* Whether the container is given read-only access to its root file system.
*/
public void setReadonlyRootFilesystem(Boolean readonlyRootFilesystem) {
this.readonlyRootFilesystem = readonlyRootFilesystem;
}
/**
*
* Whether the container is given read-only access to its root file system.
*
*
* @return Whether the container is given read-only access to its root file system.
*/
public Boolean getReadonlyRootFilesystem() {
return this.readonlyRootFilesystem;
}
/**
*
* Whether the container is given read-only access to its root file system.
*
*
* @param readonlyRootFilesystem
* Whether the container is given read-only access to its root file system.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withReadonlyRootFilesystem(Boolean readonlyRootFilesystem) {
setReadonlyRootFilesystem(readonlyRootFilesystem);
return this;
}
/**
*
* Whether the container is given read-only access to its root file system.
*
*
* @return Whether the container is given read-only access to its root file system.
*/
public Boolean isReadonlyRootFilesystem() {
return this.readonlyRootFilesystem;
}
/**
*
* The private repository authentication credentials to use.
*
*
* @param repositoryCredentials
* The private repository authentication credentials to use.
*/
public void setRepositoryCredentials(AwsEcsTaskDefinitionContainerDefinitionsRepositoryCredentialsDetails repositoryCredentials) {
this.repositoryCredentials = repositoryCredentials;
}
/**
*
* The private repository authentication credentials to use.
*
*
* @return The private repository authentication credentials to use.
*/
public AwsEcsTaskDefinitionContainerDefinitionsRepositoryCredentialsDetails getRepositoryCredentials() {
return this.repositoryCredentials;
}
/**
*
* The private repository authentication credentials to use.
*
*
* @param repositoryCredentials
* The private repository authentication credentials to use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withRepositoryCredentials(
AwsEcsTaskDefinitionContainerDefinitionsRepositoryCredentialsDetails repositoryCredentials) {
setRepositoryCredentials(repositoryCredentials);
return this;
}
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*
* @return The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*/
public java.util.List getResourceRequirements() {
return resourceRequirements;
}
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*
* @param resourceRequirements
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*/
public void setResourceRequirements(java.util.Collection resourceRequirements) {
if (resourceRequirements == null) {
this.resourceRequirements = null;
return;
}
this.resourceRequirements = new java.util.ArrayList(resourceRequirements);
}
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceRequirements(java.util.Collection)} or {@link #withResourceRequirements(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param resourceRequirements
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withResourceRequirements(
AwsEcsTaskDefinitionContainerDefinitionsResourceRequirementsDetails... resourceRequirements) {
if (this.resourceRequirements == null) {
setResourceRequirements(new java.util.ArrayList(resourceRequirements.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsResourceRequirementsDetails ele : resourceRequirements) {
this.resourceRequirements.add(ele);
}
return this;
}
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*
* @param resourceRequirements
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withResourceRequirements(
java.util.Collection resourceRequirements) {
setResourceRequirements(resourceRequirements);
return this;
}
/**
*
* The secrets to pass to the container.
*
*
* @return The secrets to pass to the container.
*/
public java.util.List getSecrets() {
return secrets;
}
/**
*
* The secrets to pass to the container.
*
*
* @param secrets
* The secrets to pass to the container.
*/
public void setSecrets(java.util.Collection secrets) {
if (secrets == null) {
this.secrets = null;
return;
}
this.secrets = new java.util.ArrayList(secrets);
}
/**
*
* The secrets to pass to the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecrets(java.util.Collection)} or {@link #withSecrets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param secrets
* The secrets to pass to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withSecrets(AwsEcsTaskDefinitionContainerDefinitionsSecretsDetails... secrets) {
if (this.secrets == null) {
setSecrets(new java.util.ArrayList(secrets.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsSecretsDetails ele : secrets) {
this.secrets.add(ele);
}
return this;
}
/**
*
* The secrets to pass to the container.
*
*
* @param secrets
* The secrets to pass to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withSecrets(java.util.Collection secrets) {
setSecrets(secrets);
return this;
}
/**
*
* The number of seconds to wait before giving up on resolving dependencies for a container.
*
*
* @param startTimeout
* The number of seconds to wait before giving up on resolving dependencies for a container.
*/
public void setStartTimeout(Integer startTimeout) {
this.startTimeout = startTimeout;
}
/**
*
* The number of seconds to wait before giving up on resolving dependencies for a container.
*
*
* @return The number of seconds to wait before giving up on resolving dependencies for a container.
*/
public Integer getStartTimeout() {
return this.startTimeout;
}
/**
*
* The number of seconds to wait before giving up on resolving dependencies for a container.
*
*
* @param startTimeout
* The number of seconds to wait before giving up on resolving dependencies for a container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withStartTimeout(Integer startTimeout) {
setStartTimeout(startTimeout);
return this;
}
/**
*
* The number of seconds to wait before the container is stopped if it doesn't shut down normally on its own.
*
*
* @param stopTimeout
* The number of seconds to wait before the container is stopped if it doesn't shut down normally on its own.
*/
public void setStopTimeout(Integer stopTimeout) {
this.stopTimeout = stopTimeout;
}
/**
*
* The number of seconds to wait before the container is stopped if it doesn't shut down normally on its own.
*
*
* @return The number of seconds to wait before the container is stopped if it doesn't shut down normally on its
* own.
*/
public Integer getStopTimeout() {
return this.stopTimeout;
}
/**
*
* The number of seconds to wait before the container is stopped if it doesn't shut down normally on its own.
*
*
* @param stopTimeout
* The number of seconds to wait before the container is stopped if it doesn't shut down normally on its own.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withStopTimeout(Integer stopTimeout) {
setStopTimeout(stopTimeout);
return this;
}
/**
*
* A list of namespaced kernel parameters to set in the container.
*
*
* @return A list of namespaced kernel parameters to set in the container.
*/
public java.util.List getSystemControls() {
return systemControls;
}
/**
*
* A list of namespaced kernel parameters to set in the container.
*
*
* @param systemControls
* A list of namespaced kernel parameters to set in the container.
*/
public void setSystemControls(java.util.Collection systemControls) {
if (systemControls == null) {
this.systemControls = null;
return;
}
this.systemControls = new java.util.ArrayList(systemControls);
}
/**
*
* A list of namespaced kernel parameters to set in the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSystemControls(java.util.Collection)} or {@link #withSystemControls(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param systemControls
* A list of namespaced kernel parameters to set in the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withSystemControls(AwsEcsTaskDefinitionContainerDefinitionsSystemControlsDetails... systemControls) {
if (this.systemControls == null) {
setSystemControls(new java.util.ArrayList(systemControls.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsSystemControlsDetails ele : systemControls) {
this.systemControls.add(ele);
}
return this;
}
/**
*
* A list of namespaced kernel parameters to set in the container.
*
*
* @param systemControls
* A list of namespaced kernel parameters to set in the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withSystemControls(
java.util.Collection systemControls) {
setSystemControls(systemControls);
return this;
}
/**
*
* A list of ulimits to set in the container.
*
*
* @return A list of ulimits to set in the container.
*/
public java.util.List getUlimits() {
return ulimits;
}
/**
*
* A list of ulimits to set in the container.
*
*
* @param ulimits
* A list of ulimits to set in the container.
*/
public void setUlimits(java.util.Collection ulimits) {
if (ulimits == null) {
this.ulimits = null;
return;
}
this.ulimits = new java.util.ArrayList(ulimits);
}
/**
*
* A list of ulimits to set in the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUlimits(java.util.Collection)} or {@link #withUlimits(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param ulimits
* A list of ulimits to set in the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withUlimits(AwsEcsTaskDefinitionContainerDefinitionsUlimitsDetails... ulimits) {
if (this.ulimits == null) {
setUlimits(new java.util.ArrayList(ulimits.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsUlimitsDetails ele : ulimits) {
this.ulimits.add(ele);
}
return this;
}
/**
*
* A list of ulimits to set in the container.
*
*
* @param ulimits
* A list of ulimits to set in the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withUlimits(java.util.Collection ulimits) {
setUlimits(ulimits);
return this;
}
/**
*
* The user to use inside the container.
*
*
* The value can use one of the following formats.
*
*
* -
*
* user
*
*
* -
*
* user
: group
*
*
* -
*
* uid
*
*
* -
*
* uid
: gid
*
*
* -
*
* user
: gid
*
*
* -
*
* uid
: group
*
*
*
*
* @param user
* The user to use inside the container.
*
* The value can use one of the following formats.
*
*
* -
*
* user
*
*
* -
*
* user
: group
*
*
* -
*
* uid
*
*
* -
*
* uid
: gid
*
*
* -
*
* user
: gid
*
*
* -
*
* uid
: group
*
*
*/
public void setUser(String user) {
this.user = user;
}
/**
*
* The user to use inside the container.
*
*
* The value can use one of the following formats.
*
*
* -
*
* user
*
*
* -
*
* user
: group
*
*
* -
*
* uid
*
*
* -
*
* uid
: gid
*
*
* -
*
* user
: gid
*
*
* -
*
* uid
: group
*
*
*
*
* @return The user to use inside the container.
*
* The value can use one of the following formats.
*
*
* -
*
* user
*
*
* -
*
* user
: group
*
*
* -
*
* uid
*
*
* -
*
* uid
: gid
*
*
* -
*
* user
: gid
*
*
* -
*
* uid
: group
*
*
*/
public String getUser() {
return this.user;
}
/**
*
* The user to use inside the container.
*
*
* The value can use one of the following formats.
*
*
* -
*
* user
*
*
* -
*
* user
: group
*
*
* -
*
* uid
*
*
* -
*
* uid
: gid
*
*
* -
*
* user
: gid
*
*
* -
*
* uid
: group
*
*
*
*
* @param user
* The user to use inside the container.
*
* The value can use one of the following formats.
*
*
* -
*
* user
*
*
* -
*
* user
: group
*
*
* -
*
* uid
*
*
* -
*
* uid
: gid
*
*
* -
*
* user
: gid
*
*
* -
*
* uid
: group
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withUser(String user) {
setUser(user);
return this;
}
/**
*
* Data volumes to mount from another container.
*
*
* @return Data volumes to mount from another container.
*/
public java.util.List getVolumesFrom() {
return volumesFrom;
}
/**
*
* Data volumes to mount from another container.
*
*
* @param volumesFrom
* Data volumes to mount from another container.
*/
public void setVolumesFrom(java.util.Collection volumesFrom) {
if (volumesFrom == null) {
this.volumesFrom = null;
return;
}
this.volumesFrom = new java.util.ArrayList(volumesFrom);
}
/**
*
* Data volumes to mount from another container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVolumesFrom(java.util.Collection)} or {@link #withVolumesFrom(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param volumesFrom
* Data volumes to mount from another container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withVolumesFrom(AwsEcsTaskDefinitionContainerDefinitionsVolumesFromDetails... volumesFrom) {
if (this.volumesFrom == null) {
setVolumesFrom(new java.util.ArrayList(volumesFrom.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsVolumesFromDetails ele : volumesFrom) {
this.volumesFrom.add(ele);
}
return this;
}
/**
*
* Data volumes to mount from another container.
*
*
* @param volumesFrom
* Data volumes to mount from another container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withVolumesFrom(
java.util.Collection volumesFrom) {
setVolumesFrom(volumesFrom);
return this;
}
/**
*
* The working directory in which to run commands inside the container.
*
*
* @param workingDirectory
* The working directory in which to run commands inside the container.
*/
public void setWorkingDirectory(String workingDirectory) {
this.workingDirectory = workingDirectory;
}
/**
*
* The working directory in which to run commands inside the container.
*
*
* @return The working directory in which to run commands inside the container.
*/
public String getWorkingDirectory() {
return this.workingDirectory;
}
/**
*
* The working directory in which to run commands inside the container.
*
*
* @param workingDirectory
* The working directory in which to run commands inside the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionContainerDefinitionsDetails withWorkingDirectory(String workingDirectory) {
setWorkingDirectory(workingDirectory);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCommand() != null)
sb.append("Command: ").append(getCommand()).append(",");
if (getCpu() != null)
sb.append("Cpu: ").append(getCpu()).append(",");
if (getDependsOn() != null)
sb.append("DependsOn: ").append(getDependsOn()).append(",");
if (getDisableNetworking() != null)
sb.append("DisableNetworking: ").append(getDisableNetworking()).append(",");
if (getDnsSearchDomains() != null)
sb.append("DnsSearchDomains: ").append(getDnsSearchDomains()).append(",");
if (getDnsServers() != null)
sb.append("DnsServers: ").append(getDnsServers()).append(",");
if (getDockerLabels() != null)
sb.append("DockerLabels: ").append(getDockerLabels()).append(",");
if (getDockerSecurityOptions() != null)
sb.append("DockerSecurityOptions: ").append(getDockerSecurityOptions()).append(",");
if (getEntryPoint() != null)
sb.append("EntryPoint: ").append(getEntryPoint()).append(",");
if (getEnvironment() != null)
sb.append("Environment: ").append(getEnvironment()).append(",");
if (getEnvironmentFiles() != null)
sb.append("EnvironmentFiles: ").append(getEnvironmentFiles()).append(",");
if (getEssential() != null)
sb.append("Essential: ").append(getEssential()).append(",");
if (getExtraHosts() != null)
sb.append("ExtraHosts: ").append(getExtraHosts()).append(",");
if (getFirelensConfiguration() != null)
sb.append("FirelensConfiguration: ").append(getFirelensConfiguration()).append(",");
if (getHealthCheck() != null)
sb.append("HealthCheck: ").append(getHealthCheck()).append(",");
if (getHostname() != null)
sb.append("Hostname: ").append(getHostname()).append(",");
if (getImage() != null)
sb.append("Image: ").append(getImage()).append(",");
if (getInteractive() != null)
sb.append("Interactive: ").append(getInteractive()).append(",");
if (getLinks() != null)
sb.append("Links: ").append(getLinks()).append(",");
if (getLinuxParameters() != null)
sb.append("LinuxParameters: ").append(getLinuxParameters()).append(",");
if (getLogConfiguration() != null)
sb.append("LogConfiguration: ").append(getLogConfiguration()).append(",");
if (getMemory() != null)
sb.append("Memory: ").append(getMemory()).append(",");
if (getMemoryReservation() != null)
sb.append("MemoryReservation: ").append(getMemoryReservation()).append(",");
if (getMountPoints() != null)
sb.append("MountPoints: ").append(getMountPoints()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getPortMappings() != null)
sb.append("PortMappings: ").append(getPortMappings()).append(",");
if (getPrivileged() != null)
sb.append("Privileged: ").append(getPrivileged()).append(",");
if (getPseudoTerminal() != null)
sb.append("PseudoTerminal: ").append(getPseudoTerminal()).append(",");
if (getReadonlyRootFilesystem() != null)
sb.append("ReadonlyRootFilesystem: ").append(getReadonlyRootFilesystem()).append(",");
if (getRepositoryCredentials() != null)
sb.append("RepositoryCredentials: ").append(getRepositoryCredentials()).append(",");
if (getResourceRequirements() != null)
sb.append("ResourceRequirements: ").append(getResourceRequirements()).append(",");
if (getSecrets() != null)
sb.append("Secrets: ").append(getSecrets()).append(",");
if (getStartTimeout() != null)
sb.append("StartTimeout: ").append(getStartTimeout()).append(",");
if (getStopTimeout() != null)
sb.append("StopTimeout: ").append(getStopTimeout()).append(",");
if (getSystemControls() != null)
sb.append("SystemControls: ").append(getSystemControls()).append(",");
if (getUlimits() != null)
sb.append("Ulimits: ").append(getUlimits()).append(",");
if (getUser() != null)
sb.append("User: ").append(getUser()).append(",");
if (getVolumesFrom() != null)
sb.append("VolumesFrom: ").append(getVolumesFrom()).append(",");
if (getWorkingDirectory() != null)
sb.append("WorkingDirectory: ").append(getWorkingDirectory());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AwsEcsTaskDefinitionContainerDefinitionsDetails == false)
return false;
AwsEcsTaskDefinitionContainerDefinitionsDetails other = (AwsEcsTaskDefinitionContainerDefinitionsDetails) obj;
if (other.getCommand() == null ^ this.getCommand() == null)
return false;
if (other.getCommand() != null && other.getCommand().equals(this.getCommand()) == false)
return false;
if (other.getCpu() == null ^ this.getCpu() == null)
return false;
if (other.getCpu() != null && other.getCpu().equals(this.getCpu()) == false)
return false;
if (other.getDependsOn() == null ^ this.getDependsOn() == null)
return false;
if (other.getDependsOn() != null && other.getDependsOn().equals(this.getDependsOn()) == false)
return false;
if (other.getDisableNetworking() == null ^ this.getDisableNetworking() == null)
return false;
if (other.getDisableNetworking() != null && other.getDisableNetworking().equals(this.getDisableNetworking()) == false)
return false;
if (other.getDnsSearchDomains() == null ^ this.getDnsSearchDomains() == null)
return false;
if (other.getDnsSearchDomains() != null && other.getDnsSearchDomains().equals(this.getDnsSearchDomains()) == false)
return false;
if (other.getDnsServers() == null ^ this.getDnsServers() == null)
return false;
if (other.getDnsServers() != null && other.getDnsServers().equals(this.getDnsServers()) == false)
return false;
if (other.getDockerLabels() == null ^ this.getDockerLabels() == null)
return false;
if (other.getDockerLabels() != null && other.getDockerLabels().equals(this.getDockerLabels()) == false)
return false;
if (other.getDockerSecurityOptions() == null ^ this.getDockerSecurityOptions() == null)
return false;
if (other.getDockerSecurityOptions() != null && other.getDockerSecurityOptions().equals(this.getDockerSecurityOptions()) == false)
return false;
if (other.getEntryPoint() == null ^ this.getEntryPoint() == null)
return false;
if (other.getEntryPoint() != null && other.getEntryPoint().equals(this.getEntryPoint()) == false)
return false;
if (other.getEnvironment() == null ^ this.getEnvironment() == null)
return false;
if (other.getEnvironment() != null && other.getEnvironment().equals(this.getEnvironment()) == false)
return false;
if (other.getEnvironmentFiles() == null ^ this.getEnvironmentFiles() == null)
return false;
if (other.getEnvironmentFiles() != null && other.getEnvironmentFiles().equals(this.getEnvironmentFiles()) == false)
return false;
if (other.getEssential() == null ^ this.getEssential() == null)
return false;
if (other.getEssential() != null && other.getEssential().equals(this.getEssential()) == false)
return false;
if (other.getExtraHosts() == null ^ this.getExtraHosts() == null)
return false;
if (other.getExtraHosts() != null && other.getExtraHosts().equals(this.getExtraHosts()) == false)
return false;
if (other.getFirelensConfiguration() == null ^ this.getFirelensConfiguration() == null)
return false;
if (other.getFirelensConfiguration() != null && other.getFirelensConfiguration().equals(this.getFirelensConfiguration()) == false)
return false;
if (other.getHealthCheck() == null ^ this.getHealthCheck() == null)
return false;
if (other.getHealthCheck() != null && other.getHealthCheck().equals(this.getHealthCheck()) == false)
return false;
if (other.getHostname() == null ^ this.getHostname() == null)
return false;
if (other.getHostname() != null && other.getHostname().equals(this.getHostname()) == false)
return false;
if (other.getImage() == null ^ this.getImage() == null)
return false;
if (other.getImage() != null && other.getImage().equals(this.getImage()) == false)
return false;
if (other.getInteractive() == null ^ this.getInteractive() == null)
return false;
if (other.getInteractive() != null && other.getInteractive().equals(this.getInteractive()) == false)
return false;
if (other.getLinks() == null ^ this.getLinks() == null)
return false;
if (other.getLinks() != null && other.getLinks().equals(this.getLinks()) == false)
return false;
if (other.getLinuxParameters() == null ^ this.getLinuxParameters() == null)
return false;
if (other.getLinuxParameters() != null && other.getLinuxParameters().equals(this.getLinuxParameters()) == false)
return false;
if (other.getLogConfiguration() == null ^ this.getLogConfiguration() == null)
return false;
if (other.getLogConfiguration() != null && other.getLogConfiguration().equals(this.getLogConfiguration()) == false)
return false;
if (other.getMemory() == null ^ this.getMemory() == null)
return false;
if (other.getMemory() != null && other.getMemory().equals(this.getMemory()) == false)
return false;
if (other.getMemoryReservation() == null ^ this.getMemoryReservation() == null)
return false;
if (other.getMemoryReservation() != null && other.getMemoryReservation().equals(this.getMemoryReservation()) == false)
return false;
if (other.getMountPoints() == null ^ this.getMountPoints() == null)
return false;
if (other.getMountPoints() != null && other.getMountPoints().equals(this.getMountPoints()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getPortMappings() == null ^ this.getPortMappings() == null)
return false;
if (other.getPortMappings() != null && other.getPortMappings().equals(this.getPortMappings()) == false)
return false;
if (other.getPrivileged() == null ^ this.getPrivileged() == null)
return false;
if (other.getPrivileged() != null && other.getPrivileged().equals(this.getPrivileged()) == false)
return false;
if (other.getPseudoTerminal() == null ^ this.getPseudoTerminal() == null)
return false;
if (other.getPseudoTerminal() != null && other.getPseudoTerminal().equals(this.getPseudoTerminal()) == false)
return false;
if (other.getReadonlyRootFilesystem() == null ^ this.getReadonlyRootFilesystem() == null)
return false;
if (other.getReadonlyRootFilesystem() != null && other.getReadonlyRootFilesystem().equals(this.getReadonlyRootFilesystem()) == false)
return false;
if (other.getRepositoryCredentials() == null ^ this.getRepositoryCredentials() == null)
return false;
if (other.getRepositoryCredentials() != null && other.getRepositoryCredentials().equals(this.getRepositoryCredentials()) == false)
return false;
if (other.getResourceRequirements() == null ^ this.getResourceRequirements() == null)
return false;
if (other.getResourceRequirements() != null && other.getResourceRequirements().equals(this.getResourceRequirements()) == false)
return false;
if (other.getSecrets() == null ^ this.getSecrets() == null)
return false;
if (other.getSecrets() != null && other.getSecrets().equals(this.getSecrets()) == false)
return false;
if (other.getStartTimeout() == null ^ this.getStartTimeout() == null)
return false;
if (other.getStartTimeout() != null && other.getStartTimeout().equals(this.getStartTimeout()) == false)
return false;
if (other.getStopTimeout() == null ^ this.getStopTimeout() == null)
return false;
if (other.getStopTimeout() != null && other.getStopTimeout().equals(this.getStopTimeout()) == false)
return false;
if (other.getSystemControls() == null ^ this.getSystemControls() == null)
return false;
if (other.getSystemControls() != null && other.getSystemControls().equals(this.getSystemControls()) == false)
return false;
if (other.getUlimits() == null ^ this.getUlimits() == null)
return false;
if (other.getUlimits() != null && other.getUlimits().equals(this.getUlimits()) == false)
return false;
if (other.getUser() == null ^ this.getUser() == null)
return false;
if (other.getUser() != null && other.getUser().equals(this.getUser()) == false)
return false;
if (other.getVolumesFrom() == null ^ this.getVolumesFrom() == null)
return false;
if (other.getVolumesFrom() != null && other.getVolumesFrom().equals(this.getVolumesFrom()) == false)
return false;
if (other.getWorkingDirectory() == null ^ this.getWorkingDirectory() == null)
return false;
if (other.getWorkingDirectory() != null && other.getWorkingDirectory().equals(this.getWorkingDirectory()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCommand() == null) ? 0 : getCommand().hashCode());
hashCode = prime * hashCode + ((getCpu() == null) ? 0 : getCpu().hashCode());
hashCode = prime * hashCode + ((getDependsOn() == null) ? 0 : getDependsOn().hashCode());
hashCode = prime * hashCode + ((getDisableNetworking() == null) ? 0 : getDisableNetworking().hashCode());
hashCode = prime * hashCode + ((getDnsSearchDomains() == null) ? 0 : getDnsSearchDomains().hashCode());
hashCode = prime * hashCode + ((getDnsServers() == null) ? 0 : getDnsServers().hashCode());
hashCode = prime * hashCode + ((getDockerLabels() == null) ? 0 : getDockerLabels().hashCode());
hashCode = prime * hashCode + ((getDockerSecurityOptions() == null) ? 0 : getDockerSecurityOptions().hashCode());
hashCode = prime * hashCode + ((getEntryPoint() == null) ? 0 : getEntryPoint().hashCode());
hashCode = prime * hashCode + ((getEnvironment() == null) ? 0 : getEnvironment().hashCode());
hashCode = prime * hashCode + ((getEnvironmentFiles() == null) ? 0 : getEnvironmentFiles().hashCode());
hashCode = prime * hashCode + ((getEssential() == null) ? 0 : getEssential().hashCode());
hashCode = prime * hashCode + ((getExtraHosts() == null) ? 0 : getExtraHosts().hashCode());
hashCode = prime * hashCode + ((getFirelensConfiguration() == null) ? 0 : getFirelensConfiguration().hashCode());
hashCode = prime * hashCode + ((getHealthCheck() == null) ? 0 : getHealthCheck().hashCode());
hashCode = prime * hashCode + ((getHostname() == null) ? 0 : getHostname().hashCode());
hashCode = prime * hashCode + ((getImage() == null) ? 0 : getImage().hashCode());
hashCode = prime * hashCode + ((getInteractive() == null) ? 0 : getInteractive().hashCode());
hashCode = prime * hashCode + ((getLinks() == null) ? 0 : getLinks().hashCode());
hashCode = prime * hashCode + ((getLinuxParameters() == null) ? 0 : getLinuxParameters().hashCode());
hashCode = prime * hashCode + ((getLogConfiguration() == null) ? 0 : getLogConfiguration().hashCode());
hashCode = prime * hashCode + ((getMemory() == null) ? 0 : getMemory().hashCode());
hashCode = prime * hashCode + ((getMemoryReservation() == null) ? 0 : getMemoryReservation().hashCode());
hashCode = prime * hashCode + ((getMountPoints() == null) ? 0 : getMountPoints().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getPortMappings() == null) ? 0 : getPortMappings().hashCode());
hashCode = prime * hashCode + ((getPrivileged() == null) ? 0 : getPrivileged().hashCode());
hashCode = prime * hashCode + ((getPseudoTerminal() == null) ? 0 : getPseudoTerminal().hashCode());
hashCode = prime * hashCode + ((getReadonlyRootFilesystem() == null) ? 0 : getReadonlyRootFilesystem().hashCode());
hashCode = prime * hashCode + ((getRepositoryCredentials() == null) ? 0 : getRepositoryCredentials().hashCode());
hashCode = prime * hashCode + ((getResourceRequirements() == null) ? 0 : getResourceRequirements().hashCode());
hashCode = prime * hashCode + ((getSecrets() == null) ? 0 : getSecrets().hashCode());
hashCode = prime * hashCode + ((getStartTimeout() == null) ? 0 : getStartTimeout().hashCode());
hashCode = prime * hashCode + ((getStopTimeout() == null) ? 0 : getStopTimeout().hashCode());
hashCode = prime * hashCode + ((getSystemControls() == null) ? 0 : getSystemControls().hashCode());
hashCode = prime * hashCode + ((getUlimits() == null) ? 0 : getUlimits().hashCode());
hashCode = prime * hashCode + ((getUser() == null) ? 0 : getUser().hashCode());
hashCode = prime * hashCode + ((getVolumesFrom() == null) ? 0 : getVolumesFrom().hashCode());
hashCode = prime * hashCode + ((getWorkingDirectory() == null) ? 0 : getWorkingDirectory().hashCode());
return hashCode;
}
@Override
public AwsEcsTaskDefinitionContainerDefinitionsDetails clone() {
try {
return (AwsEcsTaskDefinitionContainerDefinitionsDetails) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.securityhub.model.transform.AwsEcsTaskDefinitionContainerDefinitionsDetailsMarshaller.getInstance().marshall(this,
protocolMarshaller);
}
}