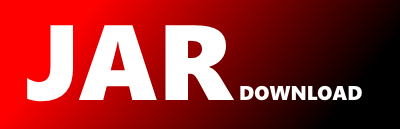
com.amazonaws.services.securityhub.model.AwsEcsTaskDefinitionDetails Maven / Gradle / Ivy
Show all versions of aws-java-sdk-securityhub Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.securityhub.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Details about a task definition. A task definition describes the container and volume definitions of an Amazon
* Elastic Container Service task.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AwsEcsTaskDefinitionDetails implements Serializable, Cloneable, StructuredPojo {
/**
*
* The container definitions that describe the containers that make up the task.
*
*/
private java.util.List containerDefinitions;
/**
*
* The number of CPU units used by the task.Valid values are as follows:
*
*
* -
*
* 256 (.25 vCPU)
*
*
* -
*
* 512 (.5 vCPU)
*
*
* -
*
* 1024 (1 vCPU)
*
*
* -
*
* 2048 (2 vCPU)
*
*
* -
*
* 4096 (4 vCPU)
*
*
*
*/
private String cpu;
/**
*
* The ARN of the task execution role that grants the container agent permission to make API calls on behalf of the
* container user.
*
*/
private String executionRoleArn;
/**
*
* The name of a family that this task definition is registered to.
*
*/
private String family;
/**
*
* The Elastic Inference accelerators to use for the containers in the task.
*
*/
private java.util.List inferenceAccelerators;
/**
*
* The inter-process communication (IPC) resource namespace to use for the containers in the task. Valid values are
* as follows:
*
*
* -
*
* host
*
*
* -
*
* none
*
*
* -
*
* task
*
*
*
*/
private String ipcMode;
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* For tasks that are hosted on Amazon EC2, you can provide a task-level memory value or a container-level memory
* value. For tasks that are hosted on Fargate, you must use one of the specified values in the Amazon Elastic Container Service Developer Guide , which determines
* your range of supported values for the Cpu
and Memory
parameters.
*
*/
private String memory;
/**
*
* The Docker networking mode to use for the containers in the task. Valid values are as follows:
*
*
* -
*
* awsvpc
*
*
* -
*
* bridge
*
*
* -
*
* host
*
*
* -
*
* none
*
*
*
*/
private String networkMode;
/**
*
* The process namespace to use for the containers in the task. Valid values are host
or
* task
.
*
*/
private String pidMode;
/**
*
* The placement constraint objects to use for tasks.
*
*/
private java.util.List placementConstraints;
/**
*
* The configuration details for the App Mesh proxy.
*
*/
private AwsEcsTaskDefinitionProxyConfigurationDetails proxyConfiguration;
/**
*
* The task launch types that the task definition was validated against.
*
*/
private java.util.List requiresCompatibilities;
/**
*
* The short name or ARN of the IAM role that grants containers in the task permission to call Amazon Web Services
* API operations on your behalf.
*
*/
private String taskRoleArn;
/**
*
* The data volume definitions for the task.
*
*/
private java.util.List volumes;
/**
*
* The container definitions that describe the containers that make up the task.
*
*
* @return The container definitions that describe the containers that make up the task.
*/
public java.util.List getContainerDefinitions() {
return containerDefinitions;
}
/**
*
* The container definitions that describe the containers that make up the task.
*
*
* @param containerDefinitions
* The container definitions that describe the containers that make up the task.
*/
public void setContainerDefinitions(java.util.Collection containerDefinitions) {
if (containerDefinitions == null) {
this.containerDefinitions = null;
return;
}
this.containerDefinitions = new java.util.ArrayList(containerDefinitions);
}
/**
*
* The container definitions that describe the containers that make up the task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setContainerDefinitions(java.util.Collection)} or {@link #withContainerDefinitions(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param containerDefinitions
* The container definitions that describe the containers that make up the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withContainerDefinitions(AwsEcsTaskDefinitionContainerDefinitionsDetails... containerDefinitions) {
if (this.containerDefinitions == null) {
setContainerDefinitions(new java.util.ArrayList(containerDefinitions.length));
}
for (AwsEcsTaskDefinitionContainerDefinitionsDetails ele : containerDefinitions) {
this.containerDefinitions.add(ele);
}
return this;
}
/**
*
* The container definitions that describe the containers that make up the task.
*
*
* @param containerDefinitions
* The container definitions that describe the containers that make up the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withContainerDefinitions(java.util.Collection containerDefinitions) {
setContainerDefinitions(containerDefinitions);
return this;
}
/**
*
* The number of CPU units used by the task.Valid values are as follows:
*
*
* -
*
* 256 (.25 vCPU)
*
*
* -
*
* 512 (.5 vCPU)
*
*
* -
*
* 1024 (1 vCPU)
*
*
* -
*
* 2048 (2 vCPU)
*
*
* -
*
* 4096 (4 vCPU)
*
*
*
*
* @param cpu
* The number of CPU units used by the task.Valid values are as follows:
*
* -
*
* 256 (.25 vCPU)
*
*
* -
*
* 512 (.5 vCPU)
*
*
* -
*
* 1024 (1 vCPU)
*
*
* -
*
* 2048 (2 vCPU)
*
*
* -
*
* 4096 (4 vCPU)
*
*
*/
public void setCpu(String cpu) {
this.cpu = cpu;
}
/**
*
* The number of CPU units used by the task.Valid values are as follows:
*
*
* -
*
* 256 (.25 vCPU)
*
*
* -
*
* 512 (.5 vCPU)
*
*
* -
*
* 1024 (1 vCPU)
*
*
* -
*
* 2048 (2 vCPU)
*
*
* -
*
* 4096 (4 vCPU)
*
*
*
*
* @return The number of CPU units used by the task.Valid values are as follows:
*
* -
*
* 256 (.25 vCPU)
*
*
* -
*
* 512 (.5 vCPU)
*
*
* -
*
* 1024 (1 vCPU)
*
*
* -
*
* 2048 (2 vCPU)
*
*
* -
*
* 4096 (4 vCPU)
*
*
*/
public String getCpu() {
return this.cpu;
}
/**
*
* The number of CPU units used by the task.Valid values are as follows:
*
*
* -
*
* 256 (.25 vCPU)
*
*
* -
*
* 512 (.5 vCPU)
*
*
* -
*
* 1024 (1 vCPU)
*
*
* -
*
* 2048 (2 vCPU)
*
*
* -
*
* 4096 (4 vCPU)
*
*
*
*
* @param cpu
* The number of CPU units used by the task.Valid values are as follows:
*
* -
*
* 256 (.25 vCPU)
*
*
* -
*
* 512 (.5 vCPU)
*
*
* -
*
* 1024 (1 vCPU)
*
*
* -
*
* 2048 (2 vCPU)
*
*
* -
*
* 4096 (4 vCPU)
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withCpu(String cpu) {
setCpu(cpu);
return this;
}
/**
*
* The ARN of the task execution role that grants the container agent permission to make API calls on behalf of the
* container user.
*
*
* @param executionRoleArn
* The ARN of the task execution role that grants the container agent permission to make API calls on behalf
* of the container user.
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The ARN of the task execution role that grants the container agent permission to make API calls on behalf of the
* container user.
*
*
* @return The ARN of the task execution role that grants the container agent permission to make API calls on behalf
* of the container user.
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The ARN of the task execution role that grants the container agent permission to make API calls on behalf of the
* container user.
*
*
* @param executionRoleArn
* The ARN of the task execution role that grants the container agent permission to make API calls on behalf
* of the container user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
*
* The name of a family that this task definition is registered to.
*
*
* @param family
* The name of a family that this task definition is registered to.
*/
public void setFamily(String family) {
this.family = family;
}
/**
*
* The name of a family that this task definition is registered to.
*
*
* @return The name of a family that this task definition is registered to.
*/
public String getFamily() {
return this.family;
}
/**
*
* The name of a family that this task definition is registered to.
*
*
* @param family
* The name of a family that this task definition is registered to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withFamily(String family) {
setFamily(family);
return this;
}
/**
*
* The Elastic Inference accelerators to use for the containers in the task.
*
*
* @return The Elastic Inference accelerators to use for the containers in the task.
*/
public java.util.List getInferenceAccelerators() {
return inferenceAccelerators;
}
/**
*
* The Elastic Inference accelerators to use for the containers in the task.
*
*
* @param inferenceAccelerators
* The Elastic Inference accelerators to use for the containers in the task.
*/
public void setInferenceAccelerators(java.util.Collection inferenceAccelerators) {
if (inferenceAccelerators == null) {
this.inferenceAccelerators = null;
return;
}
this.inferenceAccelerators = new java.util.ArrayList(inferenceAccelerators);
}
/**
*
* The Elastic Inference accelerators to use for the containers in the task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInferenceAccelerators(java.util.Collection)} or
* {@link #withInferenceAccelerators(java.util.Collection)} if you want to override the existing values.
*
*
* @param inferenceAccelerators
* The Elastic Inference accelerators to use for the containers in the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withInferenceAccelerators(AwsEcsTaskDefinitionInferenceAcceleratorsDetails... inferenceAccelerators) {
if (this.inferenceAccelerators == null) {
setInferenceAccelerators(new java.util.ArrayList(inferenceAccelerators.length));
}
for (AwsEcsTaskDefinitionInferenceAcceleratorsDetails ele : inferenceAccelerators) {
this.inferenceAccelerators.add(ele);
}
return this;
}
/**
*
* The Elastic Inference accelerators to use for the containers in the task.
*
*
* @param inferenceAccelerators
* The Elastic Inference accelerators to use for the containers in the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withInferenceAccelerators(java.util.Collection inferenceAccelerators) {
setInferenceAccelerators(inferenceAccelerators);
return this;
}
/**
*
* The inter-process communication (IPC) resource namespace to use for the containers in the task. Valid values are
* as follows:
*
*
* -
*
* host
*
*
* -
*
* none
*
*
* -
*
* task
*
*
*
*
* @param ipcMode
* The inter-process communication (IPC) resource namespace to use for the containers in the task. Valid
* values are as follows:
*
* -
*
* host
*
*
* -
*
* none
*
*
* -
*
* task
*
*
*/
public void setIpcMode(String ipcMode) {
this.ipcMode = ipcMode;
}
/**
*
* The inter-process communication (IPC) resource namespace to use for the containers in the task. Valid values are
* as follows:
*
*
* -
*
* host
*
*
* -
*
* none
*
*
* -
*
* task
*
*
*
*
* @return The inter-process communication (IPC) resource namespace to use for the containers in the task. Valid
* values are as follows:
*
* -
*
* host
*
*
* -
*
* none
*
*
* -
*
* task
*
*
*/
public String getIpcMode() {
return this.ipcMode;
}
/**
*
* The inter-process communication (IPC) resource namespace to use for the containers in the task. Valid values are
* as follows:
*
*
* -
*
* host
*
*
* -
*
* none
*
*
* -
*
* task
*
*
*
*
* @param ipcMode
* The inter-process communication (IPC) resource namespace to use for the containers in the task. Valid
* values are as follows:
*
* -
*
* host
*
*
* -
*
* none
*
*
* -
*
* task
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withIpcMode(String ipcMode) {
setIpcMode(ipcMode);
return this;
}
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* For tasks that are hosted on Amazon EC2, you can provide a task-level memory value or a container-level memory
* value. For tasks that are hosted on Fargate, you must use one of the specified values in the Amazon Elastic Container Service Developer Guide , which determines
* your range of supported values for the Cpu
and Memory
parameters.
*
*
* @param memory
* The amount (in MiB) of memory used by the task.
*
* For tasks that are hosted on Amazon EC2, you can provide a task-level memory value or a container-level
* memory value. For tasks that are hosted on Fargate, you must use one of the specified values in the Amazon Elastic Container Service Developer Guide , which
* determines your range of supported values for the Cpu
and Memory
parameters.
*/
public void setMemory(String memory) {
this.memory = memory;
}
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* For tasks that are hosted on Amazon EC2, you can provide a task-level memory value or a container-level memory
* value. For tasks that are hosted on Fargate, you must use one of the specified values in the Amazon Elastic Container Service Developer Guide , which determines
* your range of supported values for the Cpu
and Memory
parameters.
*
*
* @return The amount (in MiB) of memory used by the task.
*
* For tasks that are hosted on Amazon EC2, you can provide a task-level memory value or a container-level
* memory value. For tasks that are hosted on Fargate, you must use one of the specified values in the Amazon Elastic Container Service Developer Guide , which
* determines your range of supported values for the Cpu
and Memory
parameters.
*/
public String getMemory() {
return this.memory;
}
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* For tasks that are hosted on Amazon EC2, you can provide a task-level memory value or a container-level memory
* value. For tasks that are hosted on Fargate, you must use one of the specified values in the Amazon Elastic Container Service Developer Guide , which determines
* your range of supported values for the Cpu
and Memory
parameters.
*
*
* @param memory
* The amount (in MiB) of memory used by the task.
*
* For tasks that are hosted on Amazon EC2, you can provide a task-level memory value or a container-level
* memory value. For tasks that are hosted on Fargate, you must use one of the specified values in the Amazon Elastic Container Service Developer Guide , which
* determines your range of supported values for the Cpu
and Memory
parameters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withMemory(String memory) {
setMemory(memory);
return this;
}
/**
*
* The Docker networking mode to use for the containers in the task. Valid values are as follows:
*
*
* -
*
* awsvpc
*
*
* -
*
* bridge
*
*
* -
*
* host
*
*
* -
*
* none
*
*
*
*
* @param networkMode
* The Docker networking mode to use for the containers in the task. Valid values are as follows:
*
* -
*
* awsvpc
*
*
* -
*
* bridge
*
*
* -
*
* host
*
*
* -
*
* none
*
*
*/
public void setNetworkMode(String networkMode) {
this.networkMode = networkMode;
}
/**
*
* The Docker networking mode to use for the containers in the task. Valid values are as follows:
*
*
* -
*
* awsvpc
*
*
* -
*
* bridge
*
*
* -
*
* host
*
*
* -
*
* none
*
*
*
*
* @return The Docker networking mode to use for the containers in the task. Valid values are as follows:
*
* -
*
* awsvpc
*
*
* -
*
* bridge
*
*
* -
*
* host
*
*
* -
*
* none
*
*
*/
public String getNetworkMode() {
return this.networkMode;
}
/**
*
* The Docker networking mode to use for the containers in the task. Valid values are as follows:
*
*
* -
*
* awsvpc
*
*
* -
*
* bridge
*
*
* -
*
* host
*
*
* -
*
* none
*
*
*
*
* @param networkMode
* The Docker networking mode to use for the containers in the task. Valid values are as follows:
*
* -
*
* awsvpc
*
*
* -
*
* bridge
*
*
* -
*
* host
*
*
* -
*
* none
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withNetworkMode(String networkMode) {
setNetworkMode(networkMode);
return this;
}
/**
*
* The process namespace to use for the containers in the task. Valid values are host
or
* task
.
*
*
* @param pidMode
* The process namespace to use for the containers in the task. Valid values are host
or
* task
.
*/
public void setPidMode(String pidMode) {
this.pidMode = pidMode;
}
/**
*
* The process namespace to use for the containers in the task. Valid values are host
or
* task
.
*
*
* @return The process namespace to use for the containers in the task. Valid values are host
or
* task
.
*/
public String getPidMode() {
return this.pidMode;
}
/**
*
* The process namespace to use for the containers in the task. Valid values are host
or
* task
.
*
*
* @param pidMode
* The process namespace to use for the containers in the task. Valid values are host
or
* task
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withPidMode(String pidMode) {
setPidMode(pidMode);
return this;
}
/**
*
* The placement constraint objects to use for tasks.
*
*
* @return The placement constraint objects to use for tasks.
*/
public java.util.List getPlacementConstraints() {
return placementConstraints;
}
/**
*
* The placement constraint objects to use for tasks.
*
*
* @param placementConstraints
* The placement constraint objects to use for tasks.
*/
public void setPlacementConstraints(java.util.Collection placementConstraints) {
if (placementConstraints == null) {
this.placementConstraints = null;
return;
}
this.placementConstraints = new java.util.ArrayList(placementConstraints);
}
/**
*
* The placement constraint objects to use for tasks.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementConstraints(java.util.Collection)} or {@link #withPlacementConstraints(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param placementConstraints
* The placement constraint objects to use for tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withPlacementConstraints(AwsEcsTaskDefinitionPlacementConstraintsDetails... placementConstraints) {
if (this.placementConstraints == null) {
setPlacementConstraints(new java.util.ArrayList(placementConstraints.length));
}
for (AwsEcsTaskDefinitionPlacementConstraintsDetails ele : placementConstraints) {
this.placementConstraints.add(ele);
}
return this;
}
/**
*
* The placement constraint objects to use for tasks.
*
*
* @param placementConstraints
* The placement constraint objects to use for tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withPlacementConstraints(java.util.Collection placementConstraints) {
setPlacementConstraints(placementConstraints);
return this;
}
/**
*
* The configuration details for the App Mesh proxy.
*
*
* @param proxyConfiguration
* The configuration details for the App Mesh proxy.
*/
public void setProxyConfiguration(AwsEcsTaskDefinitionProxyConfigurationDetails proxyConfiguration) {
this.proxyConfiguration = proxyConfiguration;
}
/**
*
* The configuration details for the App Mesh proxy.
*
*
* @return The configuration details for the App Mesh proxy.
*/
public AwsEcsTaskDefinitionProxyConfigurationDetails getProxyConfiguration() {
return this.proxyConfiguration;
}
/**
*
* The configuration details for the App Mesh proxy.
*
*
* @param proxyConfiguration
* The configuration details for the App Mesh proxy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withProxyConfiguration(AwsEcsTaskDefinitionProxyConfigurationDetails proxyConfiguration) {
setProxyConfiguration(proxyConfiguration);
return this;
}
/**
*
* The task launch types that the task definition was validated against.
*
*
* @return The task launch types that the task definition was validated against.
*/
public java.util.List getRequiresCompatibilities() {
return requiresCompatibilities;
}
/**
*
* The task launch types that the task definition was validated against.
*
*
* @param requiresCompatibilities
* The task launch types that the task definition was validated against.
*/
public void setRequiresCompatibilities(java.util.Collection requiresCompatibilities) {
if (requiresCompatibilities == null) {
this.requiresCompatibilities = null;
return;
}
this.requiresCompatibilities = new java.util.ArrayList(requiresCompatibilities);
}
/**
*
* The task launch types that the task definition was validated against.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRequiresCompatibilities(java.util.Collection)} or
* {@link #withRequiresCompatibilities(java.util.Collection)} if you want to override the existing values.
*
*
* @param requiresCompatibilities
* The task launch types that the task definition was validated against.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withRequiresCompatibilities(String... requiresCompatibilities) {
if (this.requiresCompatibilities == null) {
setRequiresCompatibilities(new java.util.ArrayList(requiresCompatibilities.length));
}
for (String ele : requiresCompatibilities) {
this.requiresCompatibilities.add(ele);
}
return this;
}
/**
*
* The task launch types that the task definition was validated against.
*
*
* @param requiresCompatibilities
* The task launch types that the task definition was validated against.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withRequiresCompatibilities(java.util.Collection requiresCompatibilities) {
setRequiresCompatibilities(requiresCompatibilities);
return this;
}
/**
*
* The short name or ARN of the IAM role that grants containers in the task permission to call Amazon Web Services
* API operations on your behalf.
*
*
* @param taskRoleArn
* The short name or ARN of the IAM role that grants containers in the task permission to call Amazon Web
* Services API operations on your behalf.
*/
public void setTaskRoleArn(String taskRoleArn) {
this.taskRoleArn = taskRoleArn;
}
/**
*
* The short name or ARN of the IAM role that grants containers in the task permission to call Amazon Web Services
* API operations on your behalf.
*
*
* @return The short name or ARN of the IAM role that grants containers in the task permission to call Amazon Web
* Services API operations on your behalf.
*/
public String getTaskRoleArn() {
return this.taskRoleArn;
}
/**
*
* The short name or ARN of the IAM role that grants containers in the task permission to call Amazon Web Services
* API operations on your behalf.
*
*
* @param taskRoleArn
* The short name or ARN of the IAM role that grants containers in the task permission to call Amazon Web
* Services API operations on your behalf.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withTaskRoleArn(String taskRoleArn) {
setTaskRoleArn(taskRoleArn);
return this;
}
/**
*
* The data volume definitions for the task.
*
*
* @return The data volume definitions for the task.
*/
public java.util.List getVolumes() {
return volumes;
}
/**
*
* The data volume definitions for the task.
*
*
* @param volumes
* The data volume definitions for the task.
*/
public void setVolumes(java.util.Collection volumes) {
if (volumes == null) {
this.volumes = null;
return;
}
this.volumes = new java.util.ArrayList(volumes);
}
/**
*
* The data volume definitions for the task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVolumes(java.util.Collection)} or {@link #withVolumes(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param volumes
* The data volume definitions for the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withVolumes(AwsEcsTaskDefinitionVolumesDetails... volumes) {
if (this.volumes == null) {
setVolumes(new java.util.ArrayList(volumes.length));
}
for (AwsEcsTaskDefinitionVolumesDetails ele : volumes) {
this.volumes.add(ele);
}
return this;
}
/**
*
* The data volume definitions for the task.
*
*
* @param volumes
* The data volume definitions for the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AwsEcsTaskDefinitionDetails withVolumes(java.util.Collection volumes) {
setVolumes(volumes);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getContainerDefinitions() != null)
sb.append("ContainerDefinitions: ").append(getContainerDefinitions()).append(",");
if (getCpu() != null)
sb.append("Cpu: ").append(getCpu()).append(",");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getFamily() != null)
sb.append("Family: ").append(getFamily()).append(",");
if (getInferenceAccelerators() != null)
sb.append("InferenceAccelerators: ").append(getInferenceAccelerators()).append(",");
if (getIpcMode() != null)
sb.append("IpcMode: ").append(getIpcMode()).append(",");
if (getMemory() != null)
sb.append("Memory: ").append(getMemory()).append(",");
if (getNetworkMode() != null)
sb.append("NetworkMode: ").append(getNetworkMode()).append(",");
if (getPidMode() != null)
sb.append("PidMode: ").append(getPidMode()).append(",");
if (getPlacementConstraints() != null)
sb.append("PlacementConstraints: ").append(getPlacementConstraints()).append(",");
if (getProxyConfiguration() != null)
sb.append("ProxyConfiguration: ").append(getProxyConfiguration()).append(",");
if (getRequiresCompatibilities() != null)
sb.append("RequiresCompatibilities: ").append(getRequiresCompatibilities()).append(",");
if (getTaskRoleArn() != null)
sb.append("TaskRoleArn: ").append(getTaskRoleArn()).append(",");
if (getVolumes() != null)
sb.append("Volumes: ").append(getVolumes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AwsEcsTaskDefinitionDetails == false)
return false;
AwsEcsTaskDefinitionDetails other = (AwsEcsTaskDefinitionDetails) obj;
if (other.getContainerDefinitions() == null ^ this.getContainerDefinitions() == null)
return false;
if (other.getContainerDefinitions() != null && other.getContainerDefinitions().equals(this.getContainerDefinitions()) == false)
return false;
if (other.getCpu() == null ^ this.getCpu() == null)
return false;
if (other.getCpu() != null && other.getCpu().equals(this.getCpu()) == false)
return false;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getFamily() == null ^ this.getFamily() == null)
return false;
if (other.getFamily() != null && other.getFamily().equals(this.getFamily()) == false)
return false;
if (other.getInferenceAccelerators() == null ^ this.getInferenceAccelerators() == null)
return false;
if (other.getInferenceAccelerators() != null && other.getInferenceAccelerators().equals(this.getInferenceAccelerators()) == false)
return false;
if (other.getIpcMode() == null ^ this.getIpcMode() == null)
return false;
if (other.getIpcMode() != null && other.getIpcMode().equals(this.getIpcMode()) == false)
return false;
if (other.getMemory() == null ^ this.getMemory() == null)
return false;
if (other.getMemory() != null && other.getMemory().equals(this.getMemory()) == false)
return false;
if (other.getNetworkMode() == null ^ this.getNetworkMode() == null)
return false;
if (other.getNetworkMode() != null && other.getNetworkMode().equals(this.getNetworkMode()) == false)
return false;
if (other.getPidMode() == null ^ this.getPidMode() == null)
return false;
if (other.getPidMode() != null && other.getPidMode().equals(this.getPidMode()) == false)
return false;
if (other.getPlacementConstraints() == null ^ this.getPlacementConstraints() == null)
return false;
if (other.getPlacementConstraints() != null && other.getPlacementConstraints().equals(this.getPlacementConstraints()) == false)
return false;
if (other.getProxyConfiguration() == null ^ this.getProxyConfiguration() == null)
return false;
if (other.getProxyConfiguration() != null && other.getProxyConfiguration().equals(this.getProxyConfiguration()) == false)
return false;
if (other.getRequiresCompatibilities() == null ^ this.getRequiresCompatibilities() == null)
return false;
if (other.getRequiresCompatibilities() != null && other.getRequiresCompatibilities().equals(this.getRequiresCompatibilities()) == false)
return false;
if (other.getTaskRoleArn() == null ^ this.getTaskRoleArn() == null)
return false;
if (other.getTaskRoleArn() != null && other.getTaskRoleArn().equals(this.getTaskRoleArn()) == false)
return false;
if (other.getVolumes() == null ^ this.getVolumes() == null)
return false;
if (other.getVolumes() != null && other.getVolumes().equals(this.getVolumes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getContainerDefinitions() == null) ? 0 : getContainerDefinitions().hashCode());
hashCode = prime * hashCode + ((getCpu() == null) ? 0 : getCpu().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getFamily() == null) ? 0 : getFamily().hashCode());
hashCode = prime * hashCode + ((getInferenceAccelerators() == null) ? 0 : getInferenceAccelerators().hashCode());
hashCode = prime * hashCode + ((getIpcMode() == null) ? 0 : getIpcMode().hashCode());
hashCode = prime * hashCode + ((getMemory() == null) ? 0 : getMemory().hashCode());
hashCode = prime * hashCode + ((getNetworkMode() == null) ? 0 : getNetworkMode().hashCode());
hashCode = prime * hashCode + ((getPidMode() == null) ? 0 : getPidMode().hashCode());
hashCode = prime * hashCode + ((getPlacementConstraints() == null) ? 0 : getPlacementConstraints().hashCode());
hashCode = prime * hashCode + ((getProxyConfiguration() == null) ? 0 : getProxyConfiguration().hashCode());
hashCode = prime * hashCode + ((getRequiresCompatibilities() == null) ? 0 : getRequiresCompatibilities().hashCode());
hashCode = prime * hashCode + ((getTaskRoleArn() == null) ? 0 : getTaskRoleArn().hashCode());
hashCode = prime * hashCode + ((getVolumes() == null) ? 0 : getVolumes().hashCode());
return hashCode;
}
@Override
public AwsEcsTaskDefinitionDetails clone() {
try {
return (AwsEcsTaskDefinitionDetails) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.securityhub.model.transform.AwsEcsTaskDefinitionDetailsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}