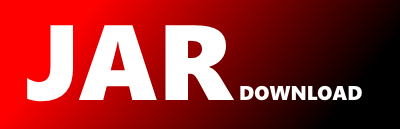
com.amazonaws.services.securityhub.model.StandardsControlAssociationDetail Maven / Gradle / Ivy
Show all versions of aws-java-sdk-securityhub Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.securityhub.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides details about a control's enablement status in a specified standard.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StandardsControlAssociationDetail implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of a security standard.
*
*/
private String standardsArn;
/**
*
* The unique identifier of a security control across standards. Values for this field typically consist of an
* Amazon Web Service name and a number, such as APIGateway.3.
*
*/
private String securityControlId;
/**
*
* The ARN of a security control across standards, such as
* arn:aws:securityhub:eu-central-1:123456789012:security-control/S3.1
. This parameter doesn't mention
* a specific standard.
*
*/
private String securityControlArn;
/**
*
* Specifies whether a control is enabled or disabled in a specified standard.
*
*/
private String associationStatus;
/**
*
* The requirement that underlies a control in the compliance framework related to the standard.
*
*/
private java.util.List relatedRequirements;
/**
*
* The time at which the enablement status of the control in the specified standard was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The reason for updating the enablement status of a control in a specified standard.
*
*/
private String updatedReason;
/**
*
* The title of a control. This field may reference a specific standard.
*
*/
private String standardsControlTitle;
/**
*
* The description of a control. This typically summarizes how Security Hub evaluates the control and the conditions
* under which it produces a failed finding. This parameter may reference a specific standard.
*
*/
private String standardsControlDescription;
/**
*
* Provides the input parameter that Security Hub uses to call the UpdateStandardsControl API. This API can be used to enable or disable a control in a specified standard.
*
*/
private java.util.List standardsControlArns;
/**
*
* The Amazon Resource Name (ARN) of a security standard.
*
*
* @param standardsArn
* The Amazon Resource Name (ARN) of a security standard.
*/
public void setStandardsArn(String standardsArn) {
this.standardsArn = standardsArn;
}
/**
*
* The Amazon Resource Name (ARN) of a security standard.
*
*
* @return The Amazon Resource Name (ARN) of a security standard.
*/
public String getStandardsArn() {
return this.standardsArn;
}
/**
*
* The Amazon Resource Name (ARN) of a security standard.
*
*
* @param standardsArn
* The Amazon Resource Name (ARN) of a security standard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withStandardsArn(String standardsArn) {
setStandardsArn(standardsArn);
return this;
}
/**
*
* The unique identifier of a security control across standards. Values for this field typically consist of an
* Amazon Web Service name and a number, such as APIGateway.3.
*
*
* @param securityControlId
* The unique identifier of a security control across standards. Values for this field typically consist of
* an Amazon Web Service name and a number, such as APIGateway.3.
*/
public void setSecurityControlId(String securityControlId) {
this.securityControlId = securityControlId;
}
/**
*
* The unique identifier of a security control across standards. Values for this field typically consist of an
* Amazon Web Service name and a number, such as APIGateway.3.
*
*
* @return The unique identifier of a security control across standards. Values for this field typically consist of
* an Amazon Web Service name and a number, such as APIGateway.3.
*/
public String getSecurityControlId() {
return this.securityControlId;
}
/**
*
* The unique identifier of a security control across standards. Values for this field typically consist of an
* Amazon Web Service name and a number, such as APIGateway.3.
*
*
* @param securityControlId
* The unique identifier of a security control across standards. Values for this field typically consist of
* an Amazon Web Service name and a number, such as APIGateway.3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withSecurityControlId(String securityControlId) {
setSecurityControlId(securityControlId);
return this;
}
/**
*
* The ARN of a security control across standards, such as
* arn:aws:securityhub:eu-central-1:123456789012:security-control/S3.1
. This parameter doesn't mention
* a specific standard.
*
*
* @param securityControlArn
* The ARN of a security control across standards, such as
* arn:aws:securityhub:eu-central-1:123456789012:security-control/S3.1
. This parameter doesn't
* mention a specific standard.
*/
public void setSecurityControlArn(String securityControlArn) {
this.securityControlArn = securityControlArn;
}
/**
*
* The ARN of a security control across standards, such as
* arn:aws:securityhub:eu-central-1:123456789012:security-control/S3.1
. This parameter doesn't mention
* a specific standard.
*
*
* @return The ARN of a security control across standards, such as
* arn:aws:securityhub:eu-central-1:123456789012:security-control/S3.1
. This parameter doesn't
* mention a specific standard.
*/
public String getSecurityControlArn() {
return this.securityControlArn;
}
/**
*
* The ARN of a security control across standards, such as
* arn:aws:securityhub:eu-central-1:123456789012:security-control/S3.1
. This parameter doesn't mention
* a specific standard.
*
*
* @param securityControlArn
* The ARN of a security control across standards, such as
* arn:aws:securityhub:eu-central-1:123456789012:security-control/S3.1
. This parameter doesn't
* mention a specific standard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withSecurityControlArn(String securityControlArn) {
setSecurityControlArn(securityControlArn);
return this;
}
/**
*
* Specifies whether a control is enabled or disabled in a specified standard.
*
*
* @param associationStatus
* Specifies whether a control is enabled or disabled in a specified standard.
* @see AssociationStatus
*/
public void setAssociationStatus(String associationStatus) {
this.associationStatus = associationStatus;
}
/**
*
* Specifies whether a control is enabled or disabled in a specified standard.
*
*
* @return Specifies whether a control is enabled or disabled in a specified standard.
* @see AssociationStatus
*/
public String getAssociationStatus() {
return this.associationStatus;
}
/**
*
* Specifies whether a control is enabled or disabled in a specified standard.
*
*
* @param associationStatus
* Specifies whether a control is enabled or disabled in a specified standard.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationStatus
*/
public StandardsControlAssociationDetail withAssociationStatus(String associationStatus) {
setAssociationStatus(associationStatus);
return this;
}
/**
*
* Specifies whether a control is enabled or disabled in a specified standard.
*
*
* @param associationStatus
* Specifies whether a control is enabled or disabled in a specified standard.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssociationStatus
*/
public StandardsControlAssociationDetail withAssociationStatus(AssociationStatus associationStatus) {
this.associationStatus = associationStatus.toString();
return this;
}
/**
*
* The requirement that underlies a control in the compliance framework related to the standard.
*
*
* @return The requirement that underlies a control in the compliance framework related to the standard.
*/
public java.util.List getRelatedRequirements() {
return relatedRequirements;
}
/**
*
* The requirement that underlies a control in the compliance framework related to the standard.
*
*
* @param relatedRequirements
* The requirement that underlies a control in the compliance framework related to the standard.
*/
public void setRelatedRequirements(java.util.Collection relatedRequirements) {
if (relatedRequirements == null) {
this.relatedRequirements = null;
return;
}
this.relatedRequirements = new java.util.ArrayList(relatedRequirements);
}
/**
*
* The requirement that underlies a control in the compliance framework related to the standard.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRelatedRequirements(java.util.Collection)} or {@link #withRelatedRequirements(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param relatedRequirements
* The requirement that underlies a control in the compliance framework related to the standard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withRelatedRequirements(String... relatedRequirements) {
if (this.relatedRequirements == null) {
setRelatedRequirements(new java.util.ArrayList(relatedRequirements.length));
}
for (String ele : relatedRequirements) {
this.relatedRequirements.add(ele);
}
return this;
}
/**
*
* The requirement that underlies a control in the compliance framework related to the standard.
*
*
* @param relatedRequirements
* The requirement that underlies a control in the compliance framework related to the standard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withRelatedRequirements(java.util.Collection relatedRequirements) {
setRelatedRequirements(relatedRequirements);
return this;
}
/**
*
* The time at which the enablement status of the control in the specified standard was last updated.
*
*
* @param updatedAt
* The time at which the enablement status of the control in the specified standard was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The time at which the enablement status of the control in the specified standard was last updated.
*
*
* @return The time at which the enablement status of the control in the specified standard was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The time at which the enablement status of the control in the specified standard was last updated.
*
*
* @param updatedAt
* The time at which the enablement status of the control in the specified standard was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
*
* The reason for updating the enablement status of a control in a specified standard.
*
*
* @param updatedReason
* The reason for updating the enablement status of a control in a specified standard.
*/
public void setUpdatedReason(String updatedReason) {
this.updatedReason = updatedReason;
}
/**
*
* The reason for updating the enablement status of a control in a specified standard.
*
*
* @return The reason for updating the enablement status of a control in a specified standard.
*/
public String getUpdatedReason() {
return this.updatedReason;
}
/**
*
* The reason for updating the enablement status of a control in a specified standard.
*
*
* @param updatedReason
* The reason for updating the enablement status of a control in a specified standard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withUpdatedReason(String updatedReason) {
setUpdatedReason(updatedReason);
return this;
}
/**
*
* The title of a control. This field may reference a specific standard.
*
*
* @param standardsControlTitle
* The title of a control. This field may reference a specific standard.
*/
public void setStandardsControlTitle(String standardsControlTitle) {
this.standardsControlTitle = standardsControlTitle;
}
/**
*
* The title of a control. This field may reference a specific standard.
*
*
* @return The title of a control. This field may reference a specific standard.
*/
public String getStandardsControlTitle() {
return this.standardsControlTitle;
}
/**
*
* The title of a control. This field may reference a specific standard.
*
*
* @param standardsControlTitle
* The title of a control. This field may reference a specific standard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withStandardsControlTitle(String standardsControlTitle) {
setStandardsControlTitle(standardsControlTitle);
return this;
}
/**
*
* The description of a control. This typically summarizes how Security Hub evaluates the control and the conditions
* under which it produces a failed finding. This parameter may reference a specific standard.
*
*
* @param standardsControlDescription
* The description of a control. This typically summarizes how Security Hub evaluates the control and the
* conditions under which it produces a failed finding. This parameter may reference a specific standard.
*/
public void setStandardsControlDescription(String standardsControlDescription) {
this.standardsControlDescription = standardsControlDescription;
}
/**
*
* The description of a control. This typically summarizes how Security Hub evaluates the control and the conditions
* under which it produces a failed finding. This parameter may reference a specific standard.
*
*
* @return The description of a control. This typically summarizes how Security Hub evaluates the control and the
* conditions under which it produces a failed finding. This parameter may reference a specific standard.
*/
public String getStandardsControlDescription() {
return this.standardsControlDescription;
}
/**
*
* The description of a control. This typically summarizes how Security Hub evaluates the control and the conditions
* under which it produces a failed finding. This parameter may reference a specific standard.
*
*
* @param standardsControlDescription
* The description of a control. This typically summarizes how Security Hub evaluates the control and the
* conditions under which it produces a failed finding. This parameter may reference a specific standard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withStandardsControlDescription(String standardsControlDescription) {
setStandardsControlDescription(standardsControlDescription);
return this;
}
/**
*
* Provides the input parameter that Security Hub uses to call the UpdateStandardsControl API. This API can be used to enable or disable a control in a specified standard.
*
*
* @return Provides the input parameter that Security Hub uses to call the UpdateStandardsControl API. This API can be used to enable or disable a control in a specified
* standard.
*/
public java.util.List getStandardsControlArns() {
return standardsControlArns;
}
/**
*
* Provides the input parameter that Security Hub uses to call the UpdateStandardsControl API. This API can be used to enable or disable a control in a specified standard.
*
*
* @param standardsControlArns
* Provides the input parameter that Security Hub uses to call the UpdateStandardsControl API. This API can be used to enable or disable a control in a specified
* standard.
*/
public void setStandardsControlArns(java.util.Collection standardsControlArns) {
if (standardsControlArns == null) {
this.standardsControlArns = null;
return;
}
this.standardsControlArns = new java.util.ArrayList(standardsControlArns);
}
/**
*
* Provides the input parameter that Security Hub uses to call the UpdateStandardsControl API. This API can be used to enable or disable a control in a specified standard.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setStandardsControlArns(java.util.Collection)} or {@link #withStandardsControlArns(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param standardsControlArns
* Provides the input parameter that Security Hub uses to call the UpdateStandardsControl API. This API can be used to enable or disable a control in a specified
* standard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withStandardsControlArns(String... standardsControlArns) {
if (this.standardsControlArns == null) {
setStandardsControlArns(new java.util.ArrayList(standardsControlArns.length));
}
for (String ele : standardsControlArns) {
this.standardsControlArns.add(ele);
}
return this;
}
/**
*
* Provides the input parameter that Security Hub uses to call the UpdateStandardsControl API. This API can be used to enable or disable a control in a specified standard.
*
*
* @param standardsControlArns
* Provides the input parameter that Security Hub uses to call the UpdateStandardsControl API. This API can be used to enable or disable a control in a specified
* standard.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StandardsControlAssociationDetail withStandardsControlArns(java.util.Collection standardsControlArns) {
setStandardsControlArns(standardsControlArns);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStandardsArn() != null)
sb.append("StandardsArn: ").append(getStandardsArn()).append(",");
if (getSecurityControlId() != null)
sb.append("SecurityControlId: ").append(getSecurityControlId()).append(",");
if (getSecurityControlArn() != null)
sb.append("SecurityControlArn: ").append(getSecurityControlArn()).append(",");
if (getAssociationStatus() != null)
sb.append("AssociationStatus: ").append(getAssociationStatus()).append(",");
if (getRelatedRequirements() != null)
sb.append("RelatedRequirements: ").append(getRelatedRequirements()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt()).append(",");
if (getUpdatedReason() != null)
sb.append("UpdatedReason: ").append(getUpdatedReason()).append(",");
if (getStandardsControlTitle() != null)
sb.append("StandardsControlTitle: ").append(getStandardsControlTitle()).append(",");
if (getStandardsControlDescription() != null)
sb.append("StandardsControlDescription: ").append(getStandardsControlDescription()).append(",");
if (getStandardsControlArns() != null)
sb.append("StandardsControlArns: ").append(getStandardsControlArns());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StandardsControlAssociationDetail == false)
return false;
StandardsControlAssociationDetail other = (StandardsControlAssociationDetail) obj;
if (other.getStandardsArn() == null ^ this.getStandardsArn() == null)
return false;
if (other.getStandardsArn() != null && other.getStandardsArn().equals(this.getStandardsArn()) == false)
return false;
if (other.getSecurityControlId() == null ^ this.getSecurityControlId() == null)
return false;
if (other.getSecurityControlId() != null && other.getSecurityControlId().equals(this.getSecurityControlId()) == false)
return false;
if (other.getSecurityControlArn() == null ^ this.getSecurityControlArn() == null)
return false;
if (other.getSecurityControlArn() != null && other.getSecurityControlArn().equals(this.getSecurityControlArn()) == false)
return false;
if (other.getAssociationStatus() == null ^ this.getAssociationStatus() == null)
return false;
if (other.getAssociationStatus() != null && other.getAssociationStatus().equals(this.getAssociationStatus()) == false)
return false;
if (other.getRelatedRequirements() == null ^ this.getRelatedRequirements() == null)
return false;
if (other.getRelatedRequirements() != null && other.getRelatedRequirements().equals(this.getRelatedRequirements()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
if (other.getUpdatedReason() == null ^ this.getUpdatedReason() == null)
return false;
if (other.getUpdatedReason() != null && other.getUpdatedReason().equals(this.getUpdatedReason()) == false)
return false;
if (other.getStandardsControlTitle() == null ^ this.getStandardsControlTitle() == null)
return false;
if (other.getStandardsControlTitle() != null && other.getStandardsControlTitle().equals(this.getStandardsControlTitle()) == false)
return false;
if (other.getStandardsControlDescription() == null ^ this.getStandardsControlDescription() == null)
return false;
if (other.getStandardsControlDescription() != null && other.getStandardsControlDescription().equals(this.getStandardsControlDescription()) == false)
return false;
if (other.getStandardsControlArns() == null ^ this.getStandardsControlArns() == null)
return false;
if (other.getStandardsControlArns() != null && other.getStandardsControlArns().equals(this.getStandardsControlArns()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStandardsArn() == null) ? 0 : getStandardsArn().hashCode());
hashCode = prime * hashCode + ((getSecurityControlId() == null) ? 0 : getSecurityControlId().hashCode());
hashCode = prime * hashCode + ((getSecurityControlArn() == null) ? 0 : getSecurityControlArn().hashCode());
hashCode = prime * hashCode + ((getAssociationStatus() == null) ? 0 : getAssociationStatus().hashCode());
hashCode = prime * hashCode + ((getRelatedRequirements() == null) ? 0 : getRelatedRequirements().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getUpdatedReason() == null) ? 0 : getUpdatedReason().hashCode());
hashCode = prime * hashCode + ((getStandardsControlTitle() == null) ? 0 : getStandardsControlTitle().hashCode());
hashCode = prime * hashCode + ((getStandardsControlDescription() == null) ? 0 : getStandardsControlDescription().hashCode());
hashCode = prime * hashCode + ((getStandardsControlArns() == null) ? 0 : getStandardsControlArns().hashCode());
return hashCode;
}
@Override
public StandardsControlAssociationDetail clone() {
try {
return (StandardsControlAssociationDetail) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.securityhub.model.transform.StandardsControlAssociationDetailMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}