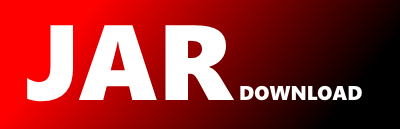
com.amazonaws.services.securityhub.model.ClassificationResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-securityhub Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.securityhub.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Details about the sensitive data that was detected on the resource.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ClassificationResult implements Serializable, Cloneable, StructuredPojo {
/**
*
* The type of content that the finding applies to.
*
*/
private String mimeType;
/**
*
* The total size in bytes of the affected data.
*
*/
private Long sizeClassified;
/**
*
* Indicates whether there are additional occurrences of sensitive data that are not included in the finding. This
* occurs when the number of occurrences exceeds the maximum that can be included.
*
*/
private Boolean additionalOccurrences;
/**
*
* The current status of the sensitive data detection.
*
*/
private ClassificationStatus status;
/**
*
* Provides details about sensitive data that was identified based on built-in configuration.
*
*/
private java.util.List sensitiveData;
/**
*
* Provides details about sensitive data that was identified based on customer-defined configuration.
*
*/
private CustomDataIdentifiersResult customDataIdentifiers;
/**
*
* The type of content that the finding applies to.
*
*
* @param mimeType
* The type of content that the finding applies to.
*/
public void setMimeType(String mimeType) {
this.mimeType = mimeType;
}
/**
*
* The type of content that the finding applies to.
*
*
* @return The type of content that the finding applies to.
*/
public String getMimeType() {
return this.mimeType;
}
/**
*
* The type of content that the finding applies to.
*
*
* @param mimeType
* The type of content that the finding applies to.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClassificationResult withMimeType(String mimeType) {
setMimeType(mimeType);
return this;
}
/**
*
* The total size in bytes of the affected data.
*
*
* @param sizeClassified
* The total size in bytes of the affected data.
*/
public void setSizeClassified(Long sizeClassified) {
this.sizeClassified = sizeClassified;
}
/**
*
* The total size in bytes of the affected data.
*
*
* @return The total size in bytes of the affected data.
*/
public Long getSizeClassified() {
return this.sizeClassified;
}
/**
*
* The total size in bytes of the affected data.
*
*
* @param sizeClassified
* The total size in bytes of the affected data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClassificationResult withSizeClassified(Long sizeClassified) {
setSizeClassified(sizeClassified);
return this;
}
/**
*
* Indicates whether there are additional occurrences of sensitive data that are not included in the finding. This
* occurs when the number of occurrences exceeds the maximum that can be included.
*
*
* @param additionalOccurrences
* Indicates whether there are additional occurrences of sensitive data that are not included in the finding.
* This occurs when the number of occurrences exceeds the maximum that can be included.
*/
public void setAdditionalOccurrences(Boolean additionalOccurrences) {
this.additionalOccurrences = additionalOccurrences;
}
/**
*
* Indicates whether there are additional occurrences of sensitive data that are not included in the finding. This
* occurs when the number of occurrences exceeds the maximum that can be included.
*
*
* @return Indicates whether there are additional occurrences of sensitive data that are not included in the
* finding. This occurs when the number of occurrences exceeds the maximum that can be included.
*/
public Boolean getAdditionalOccurrences() {
return this.additionalOccurrences;
}
/**
*
* Indicates whether there are additional occurrences of sensitive data that are not included in the finding. This
* occurs when the number of occurrences exceeds the maximum that can be included.
*
*
* @param additionalOccurrences
* Indicates whether there are additional occurrences of sensitive data that are not included in the finding.
* This occurs when the number of occurrences exceeds the maximum that can be included.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClassificationResult withAdditionalOccurrences(Boolean additionalOccurrences) {
setAdditionalOccurrences(additionalOccurrences);
return this;
}
/**
*
* Indicates whether there are additional occurrences of sensitive data that are not included in the finding. This
* occurs when the number of occurrences exceeds the maximum that can be included.
*
*
* @return Indicates whether there are additional occurrences of sensitive data that are not included in the
* finding. This occurs when the number of occurrences exceeds the maximum that can be included.
*/
public Boolean isAdditionalOccurrences() {
return this.additionalOccurrences;
}
/**
*
* The current status of the sensitive data detection.
*
*
* @param status
* The current status of the sensitive data detection.
*/
public void setStatus(ClassificationStatus status) {
this.status = status;
}
/**
*
* The current status of the sensitive data detection.
*
*
* @return The current status of the sensitive data detection.
*/
public ClassificationStatus getStatus() {
return this.status;
}
/**
*
* The current status of the sensitive data detection.
*
*
* @param status
* The current status of the sensitive data detection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClassificationResult withStatus(ClassificationStatus status) {
setStatus(status);
return this;
}
/**
*
* Provides details about sensitive data that was identified based on built-in configuration.
*
*
* @return Provides details about sensitive data that was identified based on built-in configuration.
*/
public java.util.List getSensitiveData() {
return sensitiveData;
}
/**
*
* Provides details about sensitive data that was identified based on built-in configuration.
*
*
* @param sensitiveData
* Provides details about sensitive data that was identified based on built-in configuration.
*/
public void setSensitiveData(java.util.Collection sensitiveData) {
if (sensitiveData == null) {
this.sensitiveData = null;
return;
}
this.sensitiveData = new java.util.ArrayList(sensitiveData);
}
/**
*
* Provides details about sensitive data that was identified based on built-in configuration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSensitiveData(java.util.Collection)} or {@link #withSensitiveData(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param sensitiveData
* Provides details about sensitive data that was identified based on built-in configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClassificationResult withSensitiveData(SensitiveDataResult... sensitiveData) {
if (this.sensitiveData == null) {
setSensitiveData(new java.util.ArrayList(sensitiveData.length));
}
for (SensitiveDataResult ele : sensitiveData) {
this.sensitiveData.add(ele);
}
return this;
}
/**
*
* Provides details about sensitive data that was identified based on built-in configuration.
*
*
* @param sensitiveData
* Provides details about sensitive data that was identified based on built-in configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClassificationResult withSensitiveData(java.util.Collection sensitiveData) {
setSensitiveData(sensitiveData);
return this;
}
/**
*
* Provides details about sensitive data that was identified based on customer-defined configuration.
*
*
* @param customDataIdentifiers
* Provides details about sensitive data that was identified based on customer-defined configuration.
*/
public void setCustomDataIdentifiers(CustomDataIdentifiersResult customDataIdentifiers) {
this.customDataIdentifiers = customDataIdentifiers;
}
/**
*
* Provides details about sensitive data that was identified based on customer-defined configuration.
*
*
* @return Provides details about sensitive data that was identified based on customer-defined configuration.
*/
public CustomDataIdentifiersResult getCustomDataIdentifiers() {
return this.customDataIdentifiers;
}
/**
*
* Provides details about sensitive data that was identified based on customer-defined configuration.
*
*
* @param customDataIdentifiers
* Provides details about sensitive data that was identified based on customer-defined configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ClassificationResult withCustomDataIdentifiers(CustomDataIdentifiersResult customDataIdentifiers) {
setCustomDataIdentifiers(customDataIdentifiers);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMimeType() != null)
sb.append("MimeType: ").append(getMimeType()).append(",");
if (getSizeClassified() != null)
sb.append("SizeClassified: ").append(getSizeClassified()).append(",");
if (getAdditionalOccurrences() != null)
sb.append("AdditionalOccurrences: ").append(getAdditionalOccurrences()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getSensitiveData() != null)
sb.append("SensitiveData: ").append(getSensitiveData()).append(",");
if (getCustomDataIdentifiers() != null)
sb.append("CustomDataIdentifiers: ").append(getCustomDataIdentifiers());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ClassificationResult == false)
return false;
ClassificationResult other = (ClassificationResult) obj;
if (other.getMimeType() == null ^ this.getMimeType() == null)
return false;
if (other.getMimeType() != null && other.getMimeType().equals(this.getMimeType()) == false)
return false;
if (other.getSizeClassified() == null ^ this.getSizeClassified() == null)
return false;
if (other.getSizeClassified() != null && other.getSizeClassified().equals(this.getSizeClassified()) == false)
return false;
if (other.getAdditionalOccurrences() == null ^ this.getAdditionalOccurrences() == null)
return false;
if (other.getAdditionalOccurrences() != null && other.getAdditionalOccurrences().equals(this.getAdditionalOccurrences()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getSensitiveData() == null ^ this.getSensitiveData() == null)
return false;
if (other.getSensitiveData() != null && other.getSensitiveData().equals(this.getSensitiveData()) == false)
return false;
if (other.getCustomDataIdentifiers() == null ^ this.getCustomDataIdentifiers() == null)
return false;
if (other.getCustomDataIdentifiers() != null && other.getCustomDataIdentifiers().equals(this.getCustomDataIdentifiers()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMimeType() == null) ? 0 : getMimeType().hashCode());
hashCode = prime * hashCode + ((getSizeClassified() == null) ? 0 : getSizeClassified().hashCode());
hashCode = prime * hashCode + ((getAdditionalOccurrences() == null) ? 0 : getAdditionalOccurrences().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getSensitiveData() == null) ? 0 : getSensitiveData().hashCode());
hashCode = prime * hashCode + ((getCustomDataIdentifiers() == null) ? 0 : getCustomDataIdentifiers().hashCode());
return hashCode;
}
@Override
public ClassificationResult clone() {
try {
return (ClassificationResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.securityhub.model.transform.ClassificationResultMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}